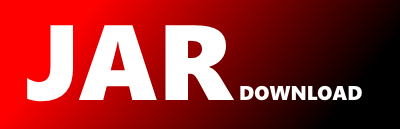
com.amazonaws.services.ecs.model.TaskOverride Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The overrides that are associated with a task.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TaskOverride implements Serializable, Cloneable, StructuredPojo {
/**
*
* One or more container overrides that are sent to a task.
*
*/
private com.amazonaws.internal.SdkInternalList containerOverrides;
/**
*
* The CPU override for the task.
*
*/
private String cpu;
/**
*
* The Elastic Inference accelerator override for the task.
*
*/
private com.amazonaws.internal.SdkInternalList inferenceAcceleratorOverrides;
/**
*
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*/
private String executionRoleArn;
/**
*
* The memory override for the task.
*
*/
private String memory;
/**
*
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this task
* are granted the permissions that are specified in this role. For more information, see IAM Role for Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*/
private String taskRoleArn;
/**
*
* The ephemeral storage setting override for the task.
*
*
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
*/
private EphemeralStorage ephemeralStorage;
/**
*
* One or more container overrides that are sent to a task.
*
*
* @return One or more container overrides that are sent to a task.
*/
public java.util.List getContainerOverrides() {
if (containerOverrides == null) {
containerOverrides = new com.amazonaws.internal.SdkInternalList();
}
return containerOverrides;
}
/**
*
* One or more container overrides that are sent to a task.
*
*
* @param containerOverrides
* One or more container overrides that are sent to a task.
*/
public void setContainerOverrides(java.util.Collection containerOverrides) {
if (containerOverrides == null) {
this.containerOverrides = null;
return;
}
this.containerOverrides = new com.amazonaws.internal.SdkInternalList(containerOverrides);
}
/**
*
* One or more container overrides that are sent to a task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainerOverrides(java.util.Collection)} or {@link #withContainerOverrides(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param containerOverrides
* One or more container overrides that are sent to a task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withContainerOverrides(ContainerOverride... containerOverrides) {
if (this.containerOverrides == null) {
setContainerOverrides(new com.amazonaws.internal.SdkInternalList(containerOverrides.length));
}
for (ContainerOverride ele : containerOverrides) {
this.containerOverrides.add(ele);
}
return this;
}
/**
*
* One or more container overrides that are sent to a task.
*
*
* @param containerOverrides
* One or more container overrides that are sent to a task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withContainerOverrides(java.util.Collection containerOverrides) {
setContainerOverrides(containerOverrides);
return this;
}
/**
*
* The CPU override for the task.
*
*
* @param cpu
* The CPU override for the task.
*/
public void setCpu(String cpu) {
this.cpu = cpu;
}
/**
*
* The CPU override for the task.
*
*
* @return The CPU override for the task.
*/
public String getCpu() {
return this.cpu;
}
/**
*
* The CPU override for the task.
*
*
* @param cpu
* The CPU override for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withCpu(String cpu) {
setCpu(cpu);
return this;
}
/**
*
* The Elastic Inference accelerator override for the task.
*
*
* @return The Elastic Inference accelerator override for the task.
*/
public java.util.List getInferenceAcceleratorOverrides() {
if (inferenceAcceleratorOverrides == null) {
inferenceAcceleratorOverrides = new com.amazonaws.internal.SdkInternalList();
}
return inferenceAcceleratorOverrides;
}
/**
*
* The Elastic Inference accelerator override for the task.
*
*
* @param inferenceAcceleratorOverrides
* The Elastic Inference accelerator override for the task.
*/
public void setInferenceAcceleratorOverrides(java.util.Collection inferenceAcceleratorOverrides) {
if (inferenceAcceleratorOverrides == null) {
this.inferenceAcceleratorOverrides = null;
return;
}
this.inferenceAcceleratorOverrides = new com.amazonaws.internal.SdkInternalList(inferenceAcceleratorOverrides);
}
/**
*
* The Elastic Inference accelerator override for the task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInferenceAcceleratorOverrides(java.util.Collection)} or
* {@link #withInferenceAcceleratorOverrides(java.util.Collection)} if you want to override the existing values.
*
*
* @param inferenceAcceleratorOverrides
* The Elastic Inference accelerator override for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withInferenceAcceleratorOverrides(InferenceAcceleratorOverride... inferenceAcceleratorOverrides) {
if (this.inferenceAcceleratorOverrides == null) {
setInferenceAcceleratorOverrides(new com.amazonaws.internal.SdkInternalList(inferenceAcceleratorOverrides.length));
}
for (InferenceAcceleratorOverride ele : inferenceAcceleratorOverrides) {
this.inferenceAcceleratorOverrides.add(ele);
}
return this;
}
/**
*
* The Elastic Inference accelerator override for the task.
*
*
* @param inferenceAcceleratorOverrides
* The Elastic Inference accelerator override for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withInferenceAcceleratorOverrides(java.util.Collection inferenceAcceleratorOverrides) {
setInferenceAcceleratorOverrides(inferenceAcceleratorOverrides);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see
* Amazon
* ECS task execution IAM role in the Amazon Elastic Container Service Developer Guide.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the task execution role override for the task. For more information,
* see Amazon
* ECS task execution IAM role in the Amazon Elastic Container Service Developer Guide.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see
* Amazon
* ECS task execution IAM role in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* The memory override for the task.
*
*
* @param memory
* The memory override for the task.
*/
public void setMemory(String memory) {
this.memory = memory;
}
/**
*
* The memory override for the task.
*
*
* @return The memory override for the task.
*/
public String getMemory() {
return this.memory;
}
/**
*
* The memory override for the task.
*
*
* @param memory
* The memory override for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withMemory(String memory) {
setMemory(memory);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this task
* are granted the permissions that are specified in this role. For more information, see IAM Role for Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param taskRoleArn
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this
* task are granted the permissions that are specified in this role. For more information, see IAM Role for
* Tasks in the Amazon Elastic Container Service Developer Guide.
*/
public void setTaskRoleArn(String taskRoleArn) {
this.taskRoleArn = taskRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this task
* are granted the permissions that are specified in this role. For more information, see IAM Role for Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in
* this task are granted the permissions that are specified in this role. For more information, see IAM Role for
* Tasks in the Amazon Elastic Container Service Developer Guide.
*/
public String getTaskRoleArn() {
return this.taskRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this task
* are granted the permissions that are specified in this role. For more information, see IAM Role for Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param taskRoleArn
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this
* task are granted the permissions that are specified in this role. For more information, see IAM Role for
* Tasks in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withTaskRoleArn(String taskRoleArn) {
setTaskRoleArn(taskRoleArn);
return this;
}
/**
*
* The ephemeral storage setting override for the task.
*
*
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
*
* @param ephemeralStorage
* The ephemeral storage setting override for the task.
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*/
public void setEphemeralStorage(EphemeralStorage ephemeralStorage) {
this.ephemeralStorage = ephemeralStorage;
}
/**
*
* The ephemeral storage setting override for the task.
*
*
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
*
* @return The ephemeral storage setting override for the task.
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*/
public EphemeralStorage getEphemeralStorage() {
return this.ephemeralStorage;
}
/**
*
* The ephemeral storage setting override for the task.
*
*
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
*
* @param ephemeralStorage
* The ephemeral storage setting override for the task.
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskOverride withEphemeralStorage(EphemeralStorage ephemeralStorage) {
setEphemeralStorage(ephemeralStorage);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getContainerOverrides() != null)
sb.append("ContainerOverrides: ").append(getContainerOverrides()).append(",");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getInferenceAcceleratorOverrides() != null)
sb.append("InferenceAcceleratorOverrides: ").append(getInferenceAcceleratorOverrides()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getTaskRoleArn() != null)
sb.append("TaskRoleArn: ").append(getTaskRoleArn()).append(",");
if (getEphemeralStorage() != null)
sb.append("EphemeralStorage: ").append(getEphemeralStorage());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TaskOverride == false)
return false;
TaskOverride other = (TaskOverride) obj;
if (other.getContainerOverrides() == null ^ this.getContainerOverrides() == null)
return false;
if (other.getContainerOverrides() != null && other.getContainerOverrides().equals(this.getContainerOverrides()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getInferenceAcceleratorOverrides() == null ^ this.getInferenceAcceleratorOverrides() == null)
return false;
if (other.getInferenceAcceleratorOverrides() != null
&& other.getInferenceAcceleratorOverrides().equals(this.getInferenceAcceleratorOverrides()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getTaskRoleArn() == null ^ this.getTaskRoleArn() == null)
return false;
if (other.getTaskRoleArn() != null && other.getTaskRoleArn().equals(this.getTaskRoleArn()) == false)
return false;
if (other.getEphemeralStorage() == null ^ this.getEphemeralStorage() == null)
return false;
if (other.getEphemeralStorage() != null && other.getEphemeralStorage().equals(this.getEphemeralStorage()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getContainerOverrides() == null) ? 0 : getContainerOverrides().hashCode());
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getInferenceAcceleratorOverrides() == null) ? 0 : getInferenceAcceleratorOverrides().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getTaskRoleArn() == null) ? 0 : getTaskRoleArn().hashCode());
hashCode = prime * hashCode + ((getEphemeralStorage() == null) ? 0 : getEphemeralStorage().hashCode());
return hashCode;
}
@Override
public TaskOverride clone() {
try {
return (TaskOverride) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.TaskOverrideMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}