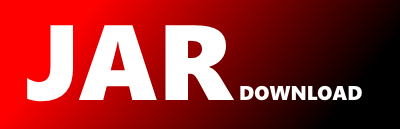
com.amazonaws.services.elasticfilesystem.AmazonElasticFileSystemClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-efs Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticfilesystem;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.elasticfilesystem.AmazonElasticFileSystemClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.elasticfilesystem.model.*;
import com.amazonaws.services.elasticfilesystem.model.transform.*;
/**
* Client for accessing EFS. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* Amazon Elastic File System
*
* Amazon Elastic File System (Amazon EFS) provides simple, scalable file storage for use with Amazon EC2 Linux and Mac
* instances in the Amazon Web Services Cloud. With Amazon EFS, storage capacity is elastic, growing and shrinking
* automatically as you add and remove files, so your applications have the storage they need, when they need it. For
* more information, see the Amazon Elastic File
* System API Reference and the Amazon Elastic
* File System User Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonElasticFileSystemClient extends AmazonWebServiceClient implements AmazonElasticFileSystem {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonElasticFileSystem.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "elasticfilesystem";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IpAddressInUse").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.IpAddressInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("FileSystemInUse").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.FileSystemInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("FileSystemAlreadyExists").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.FileSystemAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessPointAlreadyExists").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.AccessPointAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IncorrectFileSystemLifeCycleState").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.IncorrectFileSystemLifeCycleStateExceptionUnmarshaller
.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessPointLimitExceeded").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.AccessPointLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NoFreeAddressesInSubnet").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.NoFreeAddressesInSubnetExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("FileSystemNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.FileSystemNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientThroughputCapacity").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.InsufficientThroughputCapacityExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("SecurityGroupLimitExceeded").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.SecurityGroupLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("PolicyNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.PolicyNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("FileSystemLimitExceeded").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.FileSystemLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedAvailabilityZone").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.UnsupportedAvailabilityZoneExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessPointNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.AccessPointNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MountTargetConflict").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.MountTargetConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidPolicyException").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.InvalidPolicyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("SecurityGroupNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.SecurityGroupNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NetworkInterfaceLimitExceeded").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.NetworkInterfaceLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ReplicationNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.ReplicationNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MountTargetNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.MountTargetNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("SubnetNotFound").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.SubnetNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IncorrectMountTargetState").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.IncorrectMountTargetStateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThroughputLimitExceeded").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.ThroughputLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DependencyTimeout").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.DependencyTimeoutExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AvailabilityZonesMismatch").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.AvailabilityZonesMismatchExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequests").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.TooManyRequestsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequest").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerError").withExceptionUnmarshaller(
com.amazonaws.services.elasticfilesystem.model.transform.InternalServerErrorExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.elasticfilesystem.model.AmazonElasticFileSystemException.class));
/**
* Constructs a new client to invoke service methods on EFS. A credentials provider chain will be used that searches
* for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonElasticFileSystemClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on EFS. A credentials provider chain will be used that searches
* for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to EFS (ex: proxy settings, retry
* counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonElasticFileSystemClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on EFS using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AmazonElasticFileSystemClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonElasticFileSystemClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on EFS using the specified AWS account credentials and client
* configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to EFS (ex: proxy settings, retry
* counts, etc.).
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonElasticFileSystemClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonElasticFileSystemClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on EFS using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonElasticFileSystemClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on EFS using the specified AWS account credentials provider and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to EFS (ex: proxy settings, retry
* counts, etc.).
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonElasticFileSystemClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonElasticFileSystemClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on EFS using the specified AWS account credentials provider,
* client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to EFS (ex: proxy settings, retry
* counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonElasticFileSystemClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonElasticFileSystemClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonElasticFileSystemClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonElasticFileSystemClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AmazonElasticFileSystemClientBuilder builder() {
return AmazonElasticFileSystemClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on EFS using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonElasticFileSystemClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on EFS using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonElasticFileSystemClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("https://elasticfilesystem.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/elasticfilesystem/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/elasticfilesystem/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates an EFS access point. An access point is an application-specific view into an EFS file system that applies
* an operating system user and group, and a file system path, to any file system request made through the access
* point. The operating system user and group override any identity information provided by the NFS client. The file
* system path is exposed as the access point's root directory. Applications using the access point can only access
* data in its own directory and below. To learn more, see Mounting a file system using EFS access
* points.
*
*
* This operation requires permissions for the elasticfilesystem:CreateAccessPoint
action.
*
*
* @param createAccessPointRequest
* @return Result of the CreateAccessPoint operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws AccessPointAlreadyExistsException
* Returned if the access point you are trying to create already exists, with the creation token you
* provided in the request.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws AccessPointLimitExceededException
* Returned if the Amazon Web Services account has already created the maximum number of access points
* allowed per file system.
* @sample AmazonElasticFileSystem.CreateAccessPoint
* @see AWS API Documentation
*/
@Override
public CreateAccessPointResult createAccessPoint(CreateAccessPointRequest request) {
request = beforeClientExecution(request);
return executeCreateAccessPoint(request);
}
@SdkInternalApi
final CreateAccessPointResult executeCreateAccessPoint(CreateAccessPointRequest createAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(createAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAccessPointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAccessPointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new, empty file system. The operation requires a creation token in the request that Amazon EFS uses to
* ensure idempotent creation (calling the operation with same creation token has no effect). If a file system does
* not currently exist that is owned by the caller's Amazon Web Services account with the specified creation token,
* this operation does the following:
*
*
* -
*
* Creates a new, empty file system. The file system will have an Amazon EFS assigned ID, and an initial lifecycle
* state creating
.
*
*
* -
*
* Returns with the description of the created file system.
*
*
*
*
* Otherwise, this operation returns a FileSystemAlreadyExists
error with the ID of the existing file
* system.
*
*
*
* For basic use cases, you can use a randomly generated UUID for the creation token.
*
*
*
* The idempotent operation allows you to retry a CreateFileSystem
call without risk of creating an
* extra file system. This can happen when an initial call fails in a way that leaves it uncertain whether or not a
* file system was actually created. An example might be that a transport level timeout occurred or your connection
* was reset. As long as you use the same creation token, if the initial call had succeeded in creating a file
* system, the client can learn of its existence from the FileSystemAlreadyExists
error.
*
*
* For more information, see Creating a file system in the Amazon EFS User Guide.
*
*
*
* The CreateFileSystem
call returns while the file system's lifecycle state is still
* creating
. You can check the file system creation status by calling the DescribeFileSystems
* operation, which among other things returns the file system state.
*
*
*
* This operation accepts an optional PerformanceMode
parameter that you choose for your file system.
* We recommend generalPurpose
performance mode for most file systems. File systems using the
* maxIO
performance mode can scale to higher levels of aggregate throughput and operations per second
* with a tradeoff of slightly higher latencies for most file operations. The performance mode can't be changed
* after the file system has been created. For more information, see Amazon EFS performance
* modes.
*
*
* You can set the throughput mode for the file system using the ThroughputMode
parameter.
*
*
* After the file system is fully created, Amazon EFS sets its lifecycle state to available
, at which
* point you can create one or more mount targets for the file system in your VPC. For more information, see
* CreateMountTarget. You mount your Amazon EFS file system on an EC2 instances in your VPC by using the
* mount target. For more information, see Amazon EFS: How it Works.
*
*
* This operation requires permissions for the elasticfilesystem:CreateFileSystem
action.
*
*
* @param createFileSystemRequest
* @return Result of the CreateFileSystem operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemAlreadyExistsException
* Returned if the file system you are trying to create already exists, with the creation token you
* provided.
* @throws FileSystemLimitExceededException
* Returned if the Amazon Web Services account has already created the maximum number of file systems
* allowed per account.
* @throws InsufficientThroughputCapacityException
* Returned if there's not enough capacity to provision additional throughput. This value might be returned
* when you try to create a file system in provisioned throughput mode, when you attempt to increase the
* provisioned throughput of an existing file system, or when you attempt to change an existing file system
* from bursting to provisioned throughput mode. Try again later.
* @throws ThroughputLimitExceededException
* Returned if the throughput mode or amount of provisioned throughput can't be changed because the
* throughput limit of 1024 MiB/s has been reached.
* @throws UnsupportedAvailabilityZoneException
* Returned if the requested Amazon EFS functionality is not available in the specified Availability Zone.
* @sample AmazonElasticFileSystem.CreateFileSystem
* @see AWS API Documentation
*/
@Override
public CreateFileSystemResult createFileSystem(CreateFileSystemRequest request) {
request = beforeClientExecution(request);
return executeCreateFileSystem(request);
}
@SdkInternalApi
final CreateFileSystemResult executeCreateFileSystem(CreateFileSystemRequest createFileSystemRequest) {
ExecutionContext executionContext = createExecutionContext(createFileSystemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFileSystemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFileSystemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFileSystem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFileSystemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a mount target for a file system. You can then mount the file system on EC2 instances by using the mount
* target.
*
*
* You can create one mount target in each Availability Zone in your VPC. All EC2 instances in a VPC within a given
* Availability Zone share a single mount target for a given file system. If you have multiple subnets in an
* Availability Zone, you create a mount target in one of the subnets. EC2 instances do not need to be in the same
* subnet as the mount target in order to access their file system.
*
*
* You can create only one mount target for an EFS file system using One Zone storage classes. You must create that
* mount target in the same Availability Zone in which the file system is located. Use the
* AvailabilityZoneName
and AvailabiltyZoneId
properties in the DescribeFileSystems
* response object to get this information. Use the subnetId
associated with the file system's
* Availability Zone when creating the mount target.
*
*
* For more information, see Amazon EFS: How
* it Works.
*
*
* To create a mount target for a file system, the file system's lifecycle state must be available
. For
* more information, see DescribeFileSystems.
*
*
* In the request, provide the following:
*
*
* -
*
* The file system ID for which you are creating the mount target.
*
*
* -
*
* A subnet ID, which determines the following:
*
*
* -
*
* The VPC in which Amazon EFS creates the mount target
*
*
* -
*
* The Availability Zone in which Amazon EFS creates the mount target
*
*
* -
*
* The IP address range from which Amazon EFS selects the IP address of the mount target (if you don't specify an IP
* address in the request)
*
*
*
*
*
*
* After creating the mount target, Amazon EFS returns a response that includes, a MountTargetId
and an
* IpAddress
. You use this IP address when mounting the file system in an EC2 instance. You can also
* use the mount target's DNS name when mounting the file system. The EC2 instance on which you mount the file
* system by using the mount target can resolve the mount target's DNS name to its IP address. For more information,
* see How it
* Works: Implementation Overview.
*
*
* Note that you can create mount targets for a file system in only one VPC, and there can be only one mount target
* per Availability Zone. That is, if the file system already has one or more mount targets created for it, the
* subnet specified in the request to add another mount target must meet the following requirements:
*
*
* -
*
* Must belong to the same VPC as the subnets of the existing mount targets
*
*
* -
*
* Must not be in the same Availability Zone as any of the subnets of the existing mount targets
*
*
*
*
* If the request satisfies the requirements, Amazon EFS does the following:
*
*
* -
*
* Creates a new mount target in the specified subnet.
*
*
* -
*
* Also creates a new network interface in the subnet as follows:
*
*
* -
*
* If the request provides an IpAddress
, Amazon EFS assigns that IP address to the network interface.
* Otherwise, Amazon EFS assigns a free address in the subnet (in the same way that the Amazon EC2
* CreateNetworkInterface
call does when a request does not specify a primary private IP address).
*
*
* -
*
* If the request provides SecurityGroups
, this network interface is associated with those security
* groups. Otherwise, it belongs to the default security group for the subnet's VPC.
*
*
* -
*
* Assigns the description Mount target fsmt-id for file system fs-id
where
* fsmt-id
is the mount target ID, and fs-id
is the
* FileSystemId
.
*
*
* -
*
* Sets the requesterManaged
property of the network interface to true
, and the
* requesterId
value to EFS
.
*
*
*
*
* Each Amazon EFS mount target has one corresponding requester-managed EC2 network interface. After the network
* interface is created, Amazon EFS sets the NetworkInterfaceId
field in the mount target's description
* to the network interface ID, and the IpAddress
field to its address. If network interface creation
* fails, the entire CreateMountTarget
operation fails.
*
*
*
*
*
* The CreateMountTarget
call returns only after creating the network interface, but while the mount
* target state is still creating
, you can check the mount target creation status by calling the
* DescribeMountTargets operation, which among other things returns the mount target state.
*
*
*
* We recommend that you create a mount target in each of the Availability Zones. There are cost considerations for
* using a file system in an Availability Zone through a mount target created in another Availability Zone. For more
* information, see Amazon EFS. In addition, by always using a mount target
* local to the instance's Availability Zone, you eliminate a partial failure scenario. If the Availability Zone in
* which your mount target is created goes down, then you can't access your file system through that mount target.
*
*
* This operation requires permissions for the following action on the file system:
*
*
* -
*
* elasticfilesystem:CreateMountTarget
*
*
*
*
* This operation also requires permissions for the following Amazon EC2 actions:
*
*
* -
*
* ec2:DescribeSubnets
*
*
* -
*
* ec2:DescribeNetworkInterfaces
*
*
* -
*
* ec2:CreateNetworkInterface
*
*
*
*
* @param createMountTargetRequest
* @return Result of the CreateMountTarget operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @throws MountTargetConflictException
* Returned if the mount target would violate one of the specified restrictions based on the file system's
* existing mount targets.
* @throws SubnetNotFoundException
* Returned if there is no subnet with ID SubnetId
provided in the request.
* @throws NoFreeAddressesInSubnetException
* Returned if IpAddress
was not specified in the request and there are no free IP addresses in
* the subnet.
* @throws IpAddressInUseException
* Returned if the request specified an IpAddress
that is already in use in the subnet.
* @throws NetworkInterfaceLimitExceededException
* The calling account has reached the limit for elastic network interfaces for the specific Amazon Web
* Services Region. The client should try to delete some elastic network interfaces or get the account limit
* raised. For more information, see Amazon VPC
* Limits in the Amazon VPC User Guide (see the Network interfaces per VPC entry in the table).
* @throws SecurityGroupLimitExceededException
* Returned if the size of SecurityGroups
specified in the request is greater than five.
* @throws SecurityGroupNotFoundException
* Returned if one of the specified security groups doesn't exist in the subnet's VPC.
* @throws UnsupportedAvailabilityZoneException
* Returned if the requested Amazon EFS functionality is not available in the specified Availability Zone.
* @throws AvailabilityZonesMismatchException
* Returned if the Availability Zone that was specified for a mount target is different from the
* Availability Zone that was specified for One Zone storage classes. For more information, see Regional and One Zone
* storage redundancy.
* @sample AmazonElasticFileSystem.CreateMountTarget
* @see AWS API Documentation
*/
@Override
public CreateMountTargetResult createMountTarget(CreateMountTargetRequest request) {
request = beforeClientExecution(request);
return executeCreateMountTarget(request);
}
@SdkInternalApi
final CreateMountTargetResult executeCreateMountTarget(CreateMountTargetRequest createMountTargetRequest) {
ExecutionContext executionContext = createExecutionContext(createMountTargetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMountTargetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMountTargetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMountTarget");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMountTargetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a replication configuration that replicates an existing EFS file system to a new, read-only file system.
* For more information, see Amazon EFS
* replication. The replication configuration specifies the following:
*
*
* -
*
* Source file system - an existing EFS file system that you want replicated. The source file system cannot
* be a destination file system in an existing replication configuration.
*
*
* -
*
* Destination file system configuration - the configuration of the destination file system to which the
* source file system will be replicated. There can only be one destination file system in a replication
* configuration.
*
*
* -
*
* Amazon Web Services Region - The Amazon Web Services Region in which the destination file system is
* created. EFS Replication is available in all Amazon Web Services Region that Amazon EFS is available in, except
* the following regions: Asia Pacific (Hong Kong) Europe (Milan), Middle East (Bahrain), Africa (Cape Town), and
* Asia Pacific (Jakarta).
*
*
* -
*
* Availability zone - If you want the destination file system to use One Zone availability and durability,
* you must specify the Availability Zone to create the file system in. For more information about EFS storage
* classes, see Amazon EFS storage
* classes in the Amazon EFS User Guide.
*
*
* -
*
* Encryption - All destination file systems are created with encryption at rest enabled. You can specify the
* KMS key that is used to encrypt the destination file system. Your service-managed KMS key for Amazon EFS is used
* if you don't specify a KMS key. You cannot change this after the file system is created.
*
*
*
*
*
*
* The following properties are set by default:
*
*
* -
*
* Performance mode - The destination file system's performance mode will match that of the source file
* system, unless the destination file system uses One Zone storage. In that case, the General Purpose
* performance mode is used. The Performance mode cannot be changed.
*
*
* -
*
* Throughput mode - The destination file system use the Bursting throughput mode by default. You can modify
* the throughput mode once the file system is created.
*
*
*
*
* The following properties are turned off by default:
*
*
* -
*
* Lifecycle management - EFS lifecycle management and intelligent tiering are not enabled on the destination
* file system. You can enable EFS lifecycle management and intelligent tiering after the destination file system is
* created.
*
*
* -
*
* Automatic backups - Automatic daily backups not enabled on the destination file system. You can change
* this setting after the file system is created.
*
*
*
*
* For more information, see Amazon EFS
* replication.
*
*
* @param createReplicationConfigurationRequest
* @return Result of the CreateReplicationConfiguration operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @throws ValidationException
* Returned if the Backup service is not available in the Amazon Web Services Region in which the request
* was made.
* @throws ReplicationNotFoundException
* Returned if the specified file system did not have a replication configuration.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws UnsupportedAvailabilityZoneException
* Returned if the requested Amazon EFS functionality is not available in the specified Availability Zone.
* @throws FileSystemLimitExceededException
* Returned if the Amazon Web Services account has already created the maximum number of file systems
* allowed per account.
* @throws InsufficientThroughputCapacityException
* Returned if there's not enough capacity to provision additional throughput. This value might be returned
* when you try to create a file system in provisioned throughput mode, when you attempt to increase the
* provisioned throughput of an existing file system, or when you attempt to change an existing file system
* from bursting to provisioned throughput mode. Try again later.
* @throws ThroughputLimitExceededException
* Returned if the throughput mode or amount of provisioned throughput can't be changed because the
* throughput limit of 1024 MiB/s has been reached.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @sample AmazonElasticFileSystem.CreateReplicationConfiguration
* @see AWS API Documentation
*/
@Override
public CreateReplicationConfigurationResult createReplicationConfiguration(CreateReplicationConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateReplicationConfiguration(request);
}
@SdkInternalApi
final CreateReplicationConfigurationResult executeCreateReplicationConfiguration(CreateReplicationConfigurationRequest createReplicationConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createReplicationConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateReplicationConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createReplicationConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateReplicationConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateReplicationConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* DEPRECATED - CreateTags is deprecated and not maintained. Please use the API action to create tags for EFS
* resources.
*
*
*
* Creates or overwrites tags associated with a file system. Each tag is a key-value pair. If a tag key specified in
* the request already exists on the file system, this operation overwrites its value with the value provided in the
* request. If you add the Name
tag to your file system, Amazon EFS returns it in the response to the
* DescribeFileSystems operation.
*
*
* This operation requires permission for the elasticfilesystem:CreateTags
action.
*
*
* @param createTagsRequest
* @return Result of the CreateTags operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.CreateTags
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public CreateTagsResult createTags(CreateTagsRequest request) {
request = beforeClientExecution(request);
return executeCreateTags(request);
}
@SdkInternalApi
final CreateTagsResult executeCreateTags(CreateTagsRequest createTagsRequest) {
ExecutionContext executionContext = createExecutionContext(createTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified access point. After deletion is complete, new clients can no longer connect to the access
* points. Clients connected to the access point at the time of deletion will continue to function until they
* terminate their connection.
*
*
* This operation requires permissions for the elasticfilesystem:DeleteAccessPoint
action.
*
*
* @param deleteAccessPointRequest
* @return Result of the DeleteAccessPoint operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws AccessPointNotFoundException
* Returned if the specified AccessPointId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DeleteAccessPoint
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointResult deleteAccessPoint(DeleteAccessPointRequest request) {
request = beforeClientExecution(request);
return executeDeleteAccessPoint(request);
}
@SdkInternalApi
final DeleteAccessPointResult executeDeleteAccessPoint(DeleteAccessPointRequest deleteAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccessPointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAccessPointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a file system, permanently severing access to its contents. Upon return, the file system no longer exists
* and you can't access any contents of the deleted file system.
*
*
* You can't delete a file system that is in use. That is, if the file system has any mount targets, you must first
* delete them. For more information, see DescribeMountTargets and DeleteMountTarget.
*
*
*
* The DeleteFileSystem
call returns while the file system state is still deleting
. You
* can check the file system deletion status by calling the DescribeFileSystems operation, which returns a
* list of file systems in your account. If you pass file system ID or creation token for the deleted file system,
* the DescribeFileSystems returns a 404 FileSystemNotFound
error.
*
*
*
* This operation requires permissions for the elasticfilesystem:DeleteFileSystem
action.
*
*
* @param deleteFileSystemRequest
* @return Result of the DeleteFileSystem operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws FileSystemInUseException
* Returned if a file system has mount targets.
* @sample AmazonElasticFileSystem.DeleteFileSystem
* @see AWS API Documentation
*/
@Override
public DeleteFileSystemResult deleteFileSystem(DeleteFileSystemRequest request) {
request = beforeClientExecution(request);
return executeDeleteFileSystem(request);
}
@SdkInternalApi
final DeleteFileSystemResult executeDeleteFileSystem(DeleteFileSystemRequest deleteFileSystemRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFileSystemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFileSystemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFileSystemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFileSystem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFileSystemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the FileSystemPolicy
for the specified file system. The default
* FileSystemPolicy
goes into effect once the existing policy is deleted. For more information about
* the default file system policy, see Using Resource-based Policies with
* EFS.
*
*
* This operation requires permissions for the elasticfilesystem:DeleteFileSystemPolicy
action.
*
*
* @param deleteFileSystemPolicyRequest
* @return Result of the DeleteFileSystemPolicy operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @sample AmazonElasticFileSystem.DeleteFileSystemPolicy
* @see AWS API Documentation
*/
@Override
public DeleteFileSystemPolicyResult deleteFileSystemPolicy(DeleteFileSystemPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteFileSystemPolicy(request);
}
@SdkInternalApi
final DeleteFileSystemPolicyResult executeDeleteFileSystemPolicy(DeleteFileSystemPolicyRequest deleteFileSystemPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFileSystemPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFileSystemPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFileSystemPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFileSystemPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteFileSystemPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified mount target.
*
*
* This operation forcibly breaks any mounts of the file system by using the mount target that is being deleted,
* which might disrupt instances or applications using those mounts. To avoid applications getting cut off abruptly,
* you might consider unmounting any mounts of the mount target, if feasible. The operation also deletes the
* associated network interface. Uncommitted writes might be lost, but breaking a mount target using this operation
* does not corrupt the file system itself. The file system you created remains. You can mount an EC2 instance in
* your VPC by using another mount target.
*
*
* This operation requires permissions for the following action on the file system:
*
*
* -
*
* elasticfilesystem:DeleteMountTarget
*
*
*
*
*
* The DeleteMountTarget
call returns while the mount target state is still deleting
. You
* can check the mount target deletion by calling the DescribeMountTargets operation, which returns a list of
* mount target descriptions for the given file system.
*
*
*
* The operation also requires permissions for the following Amazon EC2 action on the mount target's network
* interface:
*
*
* -
*
* ec2:DeleteNetworkInterface
*
*
*
*
* @param deleteMountTargetRequest
* @return Result of the DeleteMountTarget operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws DependencyTimeoutException
* The service timed out trying to fulfill the request, and the client should try the call again.
* @throws MountTargetNotFoundException
* Returned if there is no mount target with the specified ID found in the caller's Amazon Web Services
* account.
* @sample AmazonElasticFileSystem.DeleteMountTarget
* @see AWS API Documentation
*/
@Override
public DeleteMountTargetResult deleteMountTarget(DeleteMountTargetRequest request) {
request = beforeClientExecution(request);
return executeDeleteMountTarget(request);
}
@SdkInternalApi
final DeleteMountTargetResult executeDeleteMountTarget(DeleteMountTargetRequest deleteMountTargetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMountTargetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMountTargetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMountTargetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMountTarget");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMountTargetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing replication configuration. To delete a replication configuration, you must make the request
* from the Amazon Web Services Region in which the destination file system is located. Deleting a replication
* configuration ends the replication process. You can write to the destination file system once it's status becomes
* Writeable
.
*
*
* @param deleteReplicationConfigurationRequest
* @return Result of the DeleteReplicationConfiguration operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws ReplicationNotFoundException
* Returned if the specified file system did not have a replication configuration.
* @sample AmazonElasticFileSystem.DeleteReplicationConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteReplicationConfigurationResult deleteReplicationConfiguration(DeleteReplicationConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteReplicationConfiguration(request);
}
@SdkInternalApi
final DeleteReplicationConfigurationResult executeDeleteReplicationConfiguration(DeleteReplicationConfigurationRequest deleteReplicationConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteReplicationConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteReplicationConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteReplicationConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteReplicationConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteReplicationConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* DEPRECATED - DeleteTags is deprecated and not maintained. Please use the API action to remove tags from EFS
* resources.
*
*
*
* Deletes the specified tags from a file system. If the DeleteTags
request includes a tag key that
* doesn't exist, Amazon EFS ignores it and doesn't cause an error. For more information about tags and related
* restrictions, see Tag
* restrictions in the Billing and Cost Management User Guide.
*
*
* This operation requires permissions for the elasticfilesystem:DeleteTags
action.
*
*
* @param deleteTagsRequest
* @return Result of the DeleteTags operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DeleteTags
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DeleteTagsResult deleteTags(DeleteTagsRequest request) {
request = beforeClientExecution(request);
return executeDeleteTags(request);
}
@SdkInternalApi
final DeleteTagsResult executeDeleteTags(DeleteTagsRequest deleteTagsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of a specific Amazon EFS access point if the AccessPointId
is provided. If
* you provide an EFS FileSystemId
, it returns descriptions of all access points for that file system.
* You can provide either an AccessPointId
or a FileSystemId
in the request, but not both.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeAccessPoints
action.
*
*
* @param describeAccessPointsRequest
* @return Result of the DescribeAccessPoints operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws AccessPointNotFoundException
* Returned if the specified AccessPointId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DescribeAccessPoints
* @see AWS API Documentation
*/
@Override
public DescribeAccessPointsResult describeAccessPoints(DescribeAccessPointsRequest request) {
request = beforeClientExecution(request);
return executeDescribeAccessPoints(request);
}
@SdkInternalApi
final DescribeAccessPointsResult executeDescribeAccessPoints(DescribeAccessPointsRequest describeAccessPointsRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccessPointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAccessPointsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAccessPointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAccessPoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeAccessPointsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the account preferences settings for the Amazon Web Services account associated with the user making the
* request, in the current Amazon Web Services Region. For more information, see Managing Amazon EFS resource IDs.
*
*
* @param describeAccountPreferencesRequest
* @return Result of the DescribeAccountPreferences operation returned by the service.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @sample AmazonElasticFileSystem.DescribeAccountPreferences
* @see AWS API Documentation
*/
@Override
public DescribeAccountPreferencesResult describeAccountPreferences(DescribeAccountPreferencesRequest request) {
request = beforeClientExecution(request);
return executeDescribeAccountPreferences(request);
}
@SdkInternalApi
final DescribeAccountPreferencesResult executeDescribeAccountPreferences(DescribeAccountPreferencesRequest describeAccountPreferencesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccountPreferencesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAccountPreferencesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAccountPreferencesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAccountPreferences");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAccountPreferencesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the backup policy for the specified EFS file system.
*
*
* @param describeBackupPolicyRequest
* @return Result of the DescribeBackupPolicy operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws PolicyNotFoundException
* Returned if the default file system policy is in effect for the EFS file system specified.
* @throws ValidationException
* Returned if the Backup service is not available in the Amazon Web Services Region in which the request
* was made.
* @sample AmazonElasticFileSystem.DescribeBackupPolicy
* @see AWS API Documentation
*/
@Override
public DescribeBackupPolicyResult describeBackupPolicy(DescribeBackupPolicyRequest request) {
request = beforeClientExecution(request);
return executeDescribeBackupPolicy(request);
}
@SdkInternalApi
final DescribeBackupPolicyResult executeDescribeBackupPolicy(DescribeBackupPolicyRequest describeBackupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(describeBackupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBackupPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeBackupPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBackupPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeBackupPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the FileSystemPolicy
for the specified EFS file system.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeFileSystemPolicy
action.
*
*
* @param describeFileSystemPolicyRequest
* @return Result of the DescribeFileSystemPolicy operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws PolicyNotFoundException
* Returned if the default file system policy is in effect for the EFS file system specified.
* @sample AmazonElasticFileSystem.DescribeFileSystemPolicy
* @see AWS API Documentation
*/
@Override
public DescribeFileSystemPolicyResult describeFileSystemPolicy(DescribeFileSystemPolicyRequest request) {
request = beforeClientExecution(request);
return executeDescribeFileSystemPolicy(request);
}
@SdkInternalApi
final DescribeFileSystemPolicyResult executeDescribeFileSystemPolicy(DescribeFileSystemPolicyRequest describeFileSystemPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(describeFileSystemPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFileSystemPolicyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeFileSystemPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFileSystemPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeFileSystemPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of a specific Amazon EFS file system if either the file system CreationToken
* or the FileSystemId
is provided. Otherwise, it returns descriptions of all file systems owned by the
* caller's Amazon Web Services account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all file system descriptions, you can optionally specify the MaxItems
parameter to
* limit the number of descriptions in a response. Currently, this number is automatically set to 10. If more file
* system descriptions remain, Amazon EFS returns a NextMarker
, an opaque token, in the response. In
* this case, you should send a subsequent request with the Marker
request parameter set to the value
* of NextMarker
.
*
*
* To retrieve a list of your file system descriptions, this operation is used in an iterative process, where
* DescribeFileSystems
is called first without the Marker
and then the operation continues
* to call it with the Marker
parameter set to the value of the NextMarker
from the
* previous response until the response has no NextMarker
.
*
*
* The order of file systems returned in the response of one DescribeFileSystems
call and the order of
* file systems returned across the responses of a multi-call iteration is unspecified.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeFileSystems
action.
*
*
* @param describeFileSystemsRequest
* @return Result of the DescribeFileSystems operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DescribeFileSystems
* @see AWS API Documentation
*/
@Override
public DescribeFileSystemsResult describeFileSystems(DescribeFileSystemsRequest request) {
request = beforeClientExecution(request);
return executeDescribeFileSystems(request);
}
@SdkInternalApi
final DescribeFileSystemsResult executeDescribeFileSystems(DescribeFileSystemsRequest describeFileSystemsRequest) {
ExecutionContext executionContext = createExecutionContext(describeFileSystemsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFileSystemsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFileSystemsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFileSystems");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFileSystemsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeFileSystemsResult describeFileSystems() {
return describeFileSystems(new DescribeFileSystemsRequest());
}
/**
*
* Returns the current LifecycleConfiguration
object for the specified Amazon EFS file system. EFS
* lifecycle management uses the LifecycleConfiguration
object to identify which files to move to the
* EFS Infrequent Access (IA) storage class. For a file system without a LifecycleConfiguration
object,
* the call returns an empty array in the response.
*
*
* When EFS Intelligent Tiering is enabled, TransitionToPrimaryStorageClass
has a value of
* AFTER_1_ACCESS
.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeLifecycleConfiguration
* operation.
*
*
* @param describeLifecycleConfigurationRequest
* @return Result of the DescribeLifecycleConfiguration operation returned by the service.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DescribeLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeLifecycleConfigurationResult describeLifecycleConfiguration(DescribeLifecycleConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeLifecycleConfiguration(request);
}
@SdkInternalApi
final DescribeLifecycleConfigurationResult executeDescribeLifecycleConfiguration(DescribeLifecycleConfigurationRequest describeLifecycleConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeLifecycleConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLifecycleConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeLifecycleConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeLifecycleConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeLifecycleConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the security groups currently in effect for a mount target. This operation requires that the network
* interface of the mount target has been created and the lifecycle state of the mount target is not
* deleted
.
*
*
* This operation requires permissions for the following actions:
*
*
* -
*
* elasticfilesystem:DescribeMountTargetSecurityGroups
action on the mount target's file system.
*
*
* -
*
* ec2:DescribeNetworkInterfaceAttribute
action on the mount target's network interface.
*
*
*
*
* @param describeMountTargetSecurityGroupsRequest
* @return Result of the DescribeMountTargetSecurityGroups operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws MountTargetNotFoundException
* Returned if there is no mount target with the specified ID found in the caller's Amazon Web Services
* account.
* @throws IncorrectMountTargetStateException
* Returned if the mount target is not in the correct state for the operation.
* @sample AmazonElasticFileSystem.DescribeMountTargetSecurityGroups
* @see AWS API Documentation
*/
@Override
public DescribeMountTargetSecurityGroupsResult describeMountTargetSecurityGroups(DescribeMountTargetSecurityGroupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeMountTargetSecurityGroups(request);
}
@SdkInternalApi
final DescribeMountTargetSecurityGroupsResult executeDescribeMountTargetSecurityGroups(
DescribeMountTargetSecurityGroupsRequest describeMountTargetSecurityGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeMountTargetSecurityGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMountTargetSecurityGroupsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeMountTargetSecurityGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeMountTargetSecurityGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeMountTargetSecurityGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the descriptions of all the current mount targets, or a specific mount target, for a file system. When
* requesting all of the current mount targets, the order of mount targets returned in the response is unspecified.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeMountTargets
action, on either
* the file system ID that you specify in FileSystemId
, or on the file system of the mount target that
* you specify in MountTargetId
.
*
*
* @param describeMountTargetsRequest
* @return Result of the DescribeMountTargets operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws MountTargetNotFoundException
* Returned if there is no mount target with the specified ID found in the caller's Amazon Web Services
* account.
* @throws AccessPointNotFoundException
* Returned if the specified AccessPointId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DescribeMountTargets
* @see AWS API Documentation
*/
@Override
public DescribeMountTargetsResult describeMountTargets(DescribeMountTargetsRequest request) {
request = beforeClientExecution(request);
return executeDescribeMountTargets(request);
}
@SdkInternalApi
final DescribeMountTargetsResult executeDescribeMountTargets(DescribeMountTargetsRequest describeMountTargetsRequest) {
ExecutionContext executionContext = createExecutionContext(describeMountTargetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMountTargetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeMountTargetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeMountTargets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeMountTargetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the replication configurations for either a specific file system, or all configurations for the Amazon
* Web Services account in an Amazon Web Services Region if a file system is not specified.
*
*
* @param describeReplicationConfigurationsRequest
* @return Result of the DescribeReplicationConfigurations operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws ReplicationNotFoundException
* Returned if the specified file system did not have a replication configuration.
* @throws ValidationException
* Returned if the Backup service is not available in the Amazon Web Services Region in which the request
* was made.
* @sample AmazonElasticFileSystem.DescribeReplicationConfigurations
* @see AWS API Documentation
*/
@Override
public DescribeReplicationConfigurationsResult describeReplicationConfigurations(DescribeReplicationConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeDescribeReplicationConfigurations(request);
}
@SdkInternalApi
final DescribeReplicationConfigurationsResult executeDescribeReplicationConfigurations(
DescribeReplicationConfigurationsRequest describeReplicationConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReplicationConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReplicationConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeReplicationConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReplicationConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeReplicationConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* DEPRECATED - The DeleteTags action is deprecated and not maintained. Please use the API action to remove tags
* from EFS resources.
*
*
*
* Returns the tags associated with a file system. The order of tags returned in the response of one
* DescribeTags
call and the order of tags returned across the responses of a multiple-call iteration
* (when using pagination) is unspecified.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeTags
action.
*
*
* @param describeTagsRequest
* @return Result of the DescribeTags operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.DescribeTags
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DescribeTagsResult describeTags(DescribeTagsRequest request) {
request = beforeClientExecution(request);
return executeDescribeTags(request);
}
@SdkInternalApi
final DescribeTagsResult executeDescribeTags(DescribeTagsRequest describeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(describeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all tags for a top-level EFS resource. You must provide the ID of the resource that you want to retrieve
* the tags for.
*
*
* This operation requires permissions for the elasticfilesystem:DescribeAccessPoints
action.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws AccessPointNotFoundException
* Returned if the specified AccessPointId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the set of security groups in effect for a mount target.
*
*
* When you create a mount target, Amazon EFS also creates a new network interface. For more information, see
* CreateMountTarget. This operation replaces the security groups in effect for the network interface
* associated with a mount target, with the SecurityGroups
provided in the request. This operation
* requires that the network interface of the mount target has been created and the lifecycle state of the mount
* target is not deleted
.
*
*
* The operation requires permissions for the following actions:
*
*
* -
*
* elasticfilesystem:ModifyMountTargetSecurityGroups
action on the mount target's file system.
*
*
* -
*
* ec2:ModifyNetworkInterfaceAttribute
action on the mount target's network interface.
*
*
*
*
* @param modifyMountTargetSecurityGroupsRequest
* @return Result of the ModifyMountTargetSecurityGroups operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws MountTargetNotFoundException
* Returned if there is no mount target with the specified ID found in the caller's Amazon Web Services
* account.
* @throws IncorrectMountTargetStateException
* Returned if the mount target is not in the correct state for the operation.
* @throws SecurityGroupLimitExceededException
* Returned if the size of SecurityGroups
specified in the request is greater than five.
* @throws SecurityGroupNotFoundException
* Returned if one of the specified security groups doesn't exist in the subnet's VPC.
* @sample AmazonElasticFileSystem.ModifyMountTargetSecurityGroups
* @see AWS API Documentation
*/
@Override
public ModifyMountTargetSecurityGroupsResult modifyMountTargetSecurityGroups(ModifyMountTargetSecurityGroupsRequest request) {
request = beforeClientExecution(request);
return executeModifyMountTargetSecurityGroups(request);
}
@SdkInternalApi
final ModifyMountTargetSecurityGroupsResult executeModifyMountTargetSecurityGroups(
ModifyMountTargetSecurityGroupsRequest modifyMountTargetSecurityGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(modifyMountTargetSecurityGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ModifyMountTargetSecurityGroupsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(modifyMountTargetSecurityGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ModifyMountTargetSecurityGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ModifyMountTargetSecurityGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Use this operation to set the account preference in the current Amazon Web Services Region to use long 17
* character (63 bit) or short 8 character (32 bit) resource IDs for new EFS file system and mount target resources.
* All existing resource IDs are not affected by any changes you make. You can set the ID preference during the
* opt-in period as EFS transitions to long resource IDs. For more information, see Managing Amazon EFS resource
* IDs.
*
*
*
* Starting in October, 2021, you will receive an error if you try to set the account preference to use the short 8
* character format resource ID. Contact Amazon Web Services support if you receive an error and need to use short
* IDs for file system and mount target resources.
*
*
*
* @param putAccountPreferencesRequest
* @return Result of the PutAccountPreferences operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @sample AmazonElasticFileSystem.PutAccountPreferences
* @see AWS API Documentation
*/
@Override
public PutAccountPreferencesResult putAccountPreferences(PutAccountPreferencesRequest request) {
request = beforeClientExecution(request);
return executePutAccountPreferences(request);
}
@SdkInternalApi
final PutAccountPreferencesResult executePutAccountPreferences(PutAccountPreferencesRequest putAccountPreferencesRequest) {
ExecutionContext executionContext = createExecutionContext(putAccountPreferencesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutAccountPreferencesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putAccountPreferencesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutAccountPreferences");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutAccountPreferencesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the file system's backup policy. Use this action to start or stop automatic backups of the file system.
*
*
* @param putBackupPolicyRequest
* @return Result of the PutBackupPolicy operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws ValidationException
* Returned if the Backup service is not available in the Amazon Web Services Region in which the request
* was made.
* @sample AmazonElasticFileSystem.PutBackupPolicy
* @see AWS API Documentation
*/
@Override
public PutBackupPolicyResult putBackupPolicy(PutBackupPolicyRequest request) {
request = beforeClientExecution(request);
return executePutBackupPolicy(request);
}
@SdkInternalApi
final PutBackupPolicyResult executePutBackupPolicy(PutBackupPolicyRequest putBackupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putBackupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutBackupPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putBackupPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBackupPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutBackupPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Applies an Amazon EFS FileSystemPolicy
to an Amazon EFS file system. A file system policy is an IAM
* resource-based policy and can contain multiple policy statements. A file system always has exactly one file
* system policy, which can be the default policy or an explicit policy set or updated using this API operation. EFS
* file system policies have a 20,000 character limit. When an explicit policy is set, it overrides the default
* policy. For more information about the default file system policy, see Default
* EFS File System Policy.
*
*
*
* EFS file system policies have a 20,000 character limit.
*
*
*
* This operation requires permissions for the elasticfilesystem:PutFileSystemPolicy
action.
*
*
* @param putFileSystemPolicyRequest
* @return Result of the PutFileSystemPolicy operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws InvalidPolicyException
* Returned if the FileSystemPolicy
is is malformed or contains an error such as an invalid
* parameter value or a missing required parameter. Returned in the case of a policy lockout safety check
* error.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @sample AmazonElasticFileSystem.PutFileSystemPolicy
* @see AWS API Documentation
*/
@Override
public PutFileSystemPolicyResult putFileSystemPolicy(PutFileSystemPolicyRequest request) {
request = beforeClientExecution(request);
return executePutFileSystemPolicy(request);
}
@SdkInternalApi
final PutFileSystemPolicyResult executePutFileSystemPolicy(PutFileSystemPolicyRequest putFileSystemPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putFileSystemPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutFileSystemPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putFileSystemPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutFileSystemPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutFileSystemPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables lifecycle management by creating a new LifecycleConfiguration
object. A
* LifecycleConfiguration
object defines when files in an Amazon EFS file system are automatically
* transitioned to the lower-cost EFS Infrequent Access (IA) storage class. To enable EFS Intelligent Tiering, set
* the value of TransitionToPrimaryStorageClass
to AFTER_1_ACCESS
. For more information,
* see EFS Lifecycle
* Management.
*
*
* Each Amazon EFS file system supports one lifecycle configuration, which applies to all files in the file system.
* If a LifecycleConfiguration
object already exists for the specified file system, a
* PutLifecycleConfiguration
call modifies the existing configuration. A
* PutLifecycleConfiguration
call with an empty LifecyclePolicies
array in the request
* body deletes any existing LifecycleConfiguration
and turns off lifecycle management for the file
* system.
*
*
* In the request, specify the following:
*
*
* -
*
* The ID for the file system for which you are enabling, disabling, or modifying lifecycle management.
*
*
* -
*
* A LifecyclePolicies
array of LifecyclePolicy
objects that define when files are moved
* to the IA storage class. Amazon EFS requires that each LifecyclePolicy
object have only have a
* single transition, so the LifecyclePolicies
array needs to be structured with separate
* LifecyclePolicy
objects. See the example requests in the following section for more information.
*
*
*
*
* This operation requires permissions for the elasticfilesystem:PutLifecycleConfiguration
operation.
*
*
* To apply a LifecycleConfiguration
object to an encrypted file system, you need the same Key
* Management Service permissions as when you created the encrypted file system.
*
*
* @param putLifecycleConfigurationRequest
* @return Result of the PutLifecycleConfiguration operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @sample AmazonElasticFileSystem.PutLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public PutLifecycleConfigurationResult putLifecycleConfiguration(PutLifecycleConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutLifecycleConfiguration(request);
}
@SdkInternalApi
final PutLifecycleConfigurationResult executePutLifecycleConfiguration(PutLifecycleConfigurationRequest putLifecycleConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putLifecycleConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutLifecycleConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putLifecycleConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutLifecycleConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutLifecycleConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a tag for an EFS resource. You can create tags for EFS file systems and access points using this API
* operation.
*
*
* This operation requires permissions for the elasticfilesystem:TagResource
action.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws AccessPointNotFoundException
* Returned if the specified AccessPointId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from an EFS resource. You can remove tags from EFS file systems and access points using this API
* operation.
*
*
* This operation requires permissions for the elasticfilesystem:UntagResource
action.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws AccessPointNotFoundException
* Returned if the specified AccessPointId
value doesn't exist in the requester's Amazon Web
* Services account.
* @sample AmazonElasticFileSystem.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the throughput mode or the amount of provisioned throughput of an existing file system.
*
*
* @param updateFileSystemRequest
* @return Result of the UpdateFileSystem operation returned by the service.
* @throws BadRequestException
* Returned if the request is malformed or contains an error such as an invalid parameter value or a missing
* required parameter.
* @throws FileSystemNotFoundException
* Returned if the specified FileSystemId
value doesn't exist in the requester's Amazon Web
* Services account.
* @throws IncorrectFileSystemLifeCycleStateException
* Returned if the file system's lifecycle state is not "available".
* @throws InsufficientThroughputCapacityException
* Returned if there's not enough capacity to provision additional throughput. This value might be returned
* when you try to create a file system in provisioned throughput mode, when you attempt to increase the
* provisioned throughput of an existing file system, or when you attempt to change an existing file system
* from bursting to provisioned throughput mode. Try again later.
* @throws InternalServerErrorException
* Returned if an error occurred on the server side.
* @throws ThroughputLimitExceededException
* Returned if the throughput mode or amount of provisioned throughput can't be changed because the
* throughput limit of 1024 MiB/s has been reached.
* @throws TooManyRequestsException
* Returned if you don’t wait at least 24 hours before changing the throughput mode, or decreasing the
* Provisioned Throughput value.
* @sample AmazonElasticFileSystem.UpdateFileSystem
* @see AWS API Documentation
*/
@Override
public UpdateFileSystemResult updateFileSystem(UpdateFileSystemRequest request) {
request = beforeClientExecution(request);
return executeUpdateFileSystem(request);
}
@SdkInternalApi
final UpdateFileSystemResult executeUpdateFileSystem(UpdateFileSystemRequest updateFileSystemRequest) {
ExecutionContext executionContext = createExecutionContext(updateFileSystemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFileSystemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateFileSystemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EFS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFileSystem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateFileSystemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}