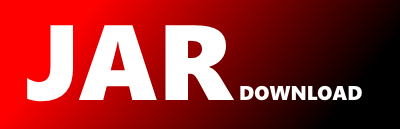
com.amazonaws.services.elasticfilesystem.model.FileSystemDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk-efs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticfilesystem.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A description of the file system.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FileSystemDescription implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Web Services account that created the file system.
*
*/
private String ownerId;
/**
*
* The opaque string specified in the request.
*
*/
private String creationToken;
/**
*
* The ID of the file system, assigned by Amazon EFS.
*
*/
private String fileSystemId;
/**
*
* The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
.
* Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
*
*/
private String fileSystemArn;
/**
*
* The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
*
*/
private java.util.Date creationTime;
/**
*
* The lifecycle phase of the file system.
*
*/
private String lifeCycleState;
/**
*
* You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in this
* field.
*
*/
private String name;
/**
*
* The current number of mount targets that the file system has. For more information, see CreateMountTarget.
*
*/
private Integer numberOfMountTargets;
/**
*
* The latest known metered size (in bytes) of data stored in the file system, in its Value
field, and
* the time at which that size was determined in its Timestamp
field. The Timestamp
value
* is the integer number of seconds since 1970-01-01T00:00:00Z. The SizeInBytes
value doesn't represent
* the size of a consistent snapshot of the file system, but it is eventually consistent when there are no writes to
* the file system. That is, SizeInBytes
represents actual size only if the file system is not modified
* for a period longer than a couple of hours. Otherwise, the value is not the exact size that the file system was
* at any point in time.
*
*/
private FileSystemSize sizeInBytes;
/**
*
* The Performance mode of the file system.
*
*/
private String performanceMode;
/**
*
* A Boolean value that, if true, indicates that the file system is encrypted.
*
*/
private Boolean encrypted;
/**
*
* The ID of an KMS key used to protect the encrypted file system.
*
*/
private String kmsKeyId;
/**
*
* Displays the file system's throughput mode. For more information, see Throughput modes in the
* Amazon EFS User Guide.
*
*/
private String throughputMode;
/**
*
* The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems using
* ThroughputMode
set to provisioned
.
*
*/
private Double provisionedThroughputInMibps;
/**
*
* Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid only for
* One Zone file systems. For more information, see Using EFS storage classes in the
* Amazon EFS User Guide.
*
*/
private String availabilityZoneName;
/**
*
* The unique and consistent identifier of the Availability Zone in which the file system is located, and is valid
* only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for the us-east-1
* Amazon Web Services Region, and it has the same location in every Amazon Web Services account.
*
*/
private String availabilityZoneId;
/**
*
* The tags associated with the file system, presented as an array of Tag
objects.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* Describes the protection on the file system.
*
*/
private FileSystemProtectionDescription fileSystemProtection;
/**
*
* The Amazon Web Services account that created the file system.
*
*
* @param ownerId
* The Amazon Web Services account that created the file system.
*/
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
/**
*
* The Amazon Web Services account that created the file system.
*
*
* @return The Amazon Web Services account that created the file system.
*/
public String getOwnerId() {
return this.ownerId;
}
/**
*
* The Amazon Web Services account that created the file system.
*
*
* @param ownerId
* The Amazon Web Services account that created the file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withOwnerId(String ownerId) {
setOwnerId(ownerId);
return this;
}
/**
*
* The opaque string specified in the request.
*
*
* @param creationToken
* The opaque string specified in the request.
*/
public void setCreationToken(String creationToken) {
this.creationToken = creationToken;
}
/**
*
* The opaque string specified in the request.
*
*
* @return The opaque string specified in the request.
*/
public String getCreationToken() {
return this.creationToken;
}
/**
*
* The opaque string specified in the request.
*
*
* @param creationToken
* The opaque string specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withCreationToken(String creationToken) {
setCreationToken(creationToken);
return this;
}
/**
*
* The ID of the file system, assigned by Amazon EFS.
*
*
* @param fileSystemId
* The ID of the file system, assigned by Amazon EFS.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The ID of the file system, assigned by Amazon EFS.
*
*
* @return The ID of the file system, assigned by Amazon EFS.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The ID of the file system, assigned by Amazon EFS.
*
*
* @param fileSystemId
* The ID of the file system, assigned by Amazon EFS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
.
* Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
*
*
* @param fileSystemArn
* The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
.
* Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
*/
public void setFileSystemArn(String fileSystemArn) {
this.fileSystemArn = fileSystemArn;
}
/**
*
* The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
.
* Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
*
*
* @return The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
* . Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
*/
public String getFileSystemArn() {
return this.fileSystemArn;
}
/**
*
* The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
.
* Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
*
*
* @param fileSystemArn
* The Amazon Resource Name (ARN) for the EFS file system, in the format
* arn:aws:elasticfilesystem:region:account-id:file-system/file-system-id
.
* Example with sample data:
* arn:aws:elasticfilesystem:us-west-2:1111333322228888:file-system/fs-01234567
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withFileSystemArn(String fileSystemArn) {
setFileSystemArn(fileSystemArn);
return this;
}
/**
*
* The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
*
*
* @param creationTime
* The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
*
*
* @return The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
*
*
* @param creationTime
* The time that the file system was created, in seconds (since 1970-01-01T00:00:00Z).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The lifecycle phase of the file system.
*
*
* @param lifeCycleState
* The lifecycle phase of the file system.
* @see LifeCycleState
*/
public void setLifeCycleState(String lifeCycleState) {
this.lifeCycleState = lifeCycleState;
}
/**
*
* The lifecycle phase of the file system.
*
*
* @return The lifecycle phase of the file system.
* @see LifeCycleState
*/
public String getLifeCycleState() {
return this.lifeCycleState;
}
/**
*
* The lifecycle phase of the file system.
*
*
* @param lifeCycleState
* The lifecycle phase of the file system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LifeCycleState
*/
public FileSystemDescription withLifeCycleState(String lifeCycleState) {
setLifeCycleState(lifeCycleState);
return this;
}
/**
*
* The lifecycle phase of the file system.
*
*
* @param lifeCycleState
* The lifecycle phase of the file system.
* @see LifeCycleState
*/
public void setLifeCycleState(LifeCycleState lifeCycleState) {
withLifeCycleState(lifeCycleState);
}
/**
*
* The lifecycle phase of the file system.
*
*
* @param lifeCycleState
* The lifecycle phase of the file system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LifeCycleState
*/
public FileSystemDescription withLifeCycleState(LifeCycleState lifeCycleState) {
this.lifeCycleState = lifeCycleState.toString();
return this;
}
/**
*
* You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in this
* field.
*
*
* @param name
* You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in
* this field.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in this
* field.
*
*
* @return You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in
* this field.
*/
public String getName() {
return this.name;
}
/**
*
* You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in this
* field.
*
*
* @param name
* You can add tags to a file system, including a Name
tag. For more information, see
* CreateFileSystem. If the file system has a Name
tag, Amazon EFS returns the value in
* this field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withName(String name) {
setName(name);
return this;
}
/**
*
* The current number of mount targets that the file system has. For more information, see CreateMountTarget.
*
*
* @param numberOfMountTargets
* The current number of mount targets that the file system has. For more information, see
* CreateMountTarget.
*/
public void setNumberOfMountTargets(Integer numberOfMountTargets) {
this.numberOfMountTargets = numberOfMountTargets;
}
/**
*
* The current number of mount targets that the file system has. For more information, see CreateMountTarget.
*
*
* @return The current number of mount targets that the file system has. For more information, see
* CreateMountTarget.
*/
public Integer getNumberOfMountTargets() {
return this.numberOfMountTargets;
}
/**
*
* The current number of mount targets that the file system has. For more information, see CreateMountTarget.
*
*
* @param numberOfMountTargets
* The current number of mount targets that the file system has. For more information, see
* CreateMountTarget.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withNumberOfMountTargets(Integer numberOfMountTargets) {
setNumberOfMountTargets(numberOfMountTargets);
return this;
}
/**
*
* The latest known metered size (in bytes) of data stored in the file system, in its Value
field, and
* the time at which that size was determined in its Timestamp
field. The Timestamp
value
* is the integer number of seconds since 1970-01-01T00:00:00Z. The SizeInBytes
value doesn't represent
* the size of a consistent snapshot of the file system, but it is eventually consistent when there are no writes to
* the file system. That is, SizeInBytes
represents actual size only if the file system is not modified
* for a period longer than a couple of hours. Otherwise, the value is not the exact size that the file system was
* at any point in time.
*
*
* @param sizeInBytes
* The latest known metered size (in bytes) of data stored in the file system, in its Value
* field, and the time at which that size was determined in its Timestamp
field. The
* Timestamp
value is the integer number of seconds since 1970-01-01T00:00:00Z. The
* SizeInBytes
value doesn't represent the size of a consistent snapshot of the file system, but
* it is eventually consistent when there are no writes to the file system. That is, SizeInBytes
* represents actual size only if the file system is not modified for a period longer than a couple of hours.
* Otherwise, the value is not the exact size that the file system was at any point in time.
*/
public void setSizeInBytes(FileSystemSize sizeInBytes) {
this.sizeInBytes = sizeInBytes;
}
/**
*
* The latest known metered size (in bytes) of data stored in the file system, in its Value
field, and
* the time at which that size was determined in its Timestamp
field. The Timestamp
value
* is the integer number of seconds since 1970-01-01T00:00:00Z. The SizeInBytes
value doesn't represent
* the size of a consistent snapshot of the file system, but it is eventually consistent when there are no writes to
* the file system. That is, SizeInBytes
represents actual size only if the file system is not modified
* for a period longer than a couple of hours. Otherwise, the value is not the exact size that the file system was
* at any point in time.
*
*
* @return The latest known metered size (in bytes) of data stored in the file system, in its Value
* field, and the time at which that size was determined in its Timestamp
field. The
* Timestamp
value is the integer number of seconds since 1970-01-01T00:00:00Z. The
* SizeInBytes
value doesn't represent the size of a consistent snapshot of the file system,
* but it is eventually consistent when there are no writes to the file system. That is,
* SizeInBytes
represents actual size only if the file system is not modified for a period
* longer than a couple of hours. Otherwise, the value is not the exact size that the file system was at any
* point in time.
*/
public FileSystemSize getSizeInBytes() {
return this.sizeInBytes;
}
/**
*
* The latest known metered size (in bytes) of data stored in the file system, in its Value
field, and
* the time at which that size was determined in its Timestamp
field. The Timestamp
value
* is the integer number of seconds since 1970-01-01T00:00:00Z. The SizeInBytes
value doesn't represent
* the size of a consistent snapshot of the file system, but it is eventually consistent when there are no writes to
* the file system. That is, SizeInBytes
represents actual size only if the file system is not modified
* for a period longer than a couple of hours. Otherwise, the value is not the exact size that the file system was
* at any point in time.
*
*
* @param sizeInBytes
* The latest known metered size (in bytes) of data stored in the file system, in its Value
* field, and the time at which that size was determined in its Timestamp
field. The
* Timestamp
value is the integer number of seconds since 1970-01-01T00:00:00Z. The
* SizeInBytes
value doesn't represent the size of a consistent snapshot of the file system, but
* it is eventually consistent when there are no writes to the file system. That is, SizeInBytes
* represents actual size only if the file system is not modified for a period longer than a couple of hours.
* Otherwise, the value is not the exact size that the file system was at any point in time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withSizeInBytes(FileSystemSize sizeInBytes) {
setSizeInBytes(sizeInBytes);
return this;
}
/**
*
* The Performance mode of the file system.
*
*
* @param performanceMode
* The Performance mode of the file system.
* @see PerformanceMode
*/
public void setPerformanceMode(String performanceMode) {
this.performanceMode = performanceMode;
}
/**
*
* The Performance mode of the file system.
*
*
* @return The Performance mode of the file system.
* @see PerformanceMode
*/
public String getPerformanceMode() {
return this.performanceMode;
}
/**
*
* The Performance mode of the file system.
*
*
* @param performanceMode
* The Performance mode of the file system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PerformanceMode
*/
public FileSystemDescription withPerformanceMode(String performanceMode) {
setPerformanceMode(performanceMode);
return this;
}
/**
*
* The Performance mode of the file system.
*
*
* @param performanceMode
* The Performance mode of the file system.
* @see PerformanceMode
*/
public void setPerformanceMode(PerformanceMode performanceMode) {
withPerformanceMode(performanceMode);
}
/**
*
* The Performance mode of the file system.
*
*
* @param performanceMode
* The Performance mode of the file system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PerformanceMode
*/
public FileSystemDescription withPerformanceMode(PerformanceMode performanceMode) {
this.performanceMode = performanceMode.toString();
return this;
}
/**
*
* A Boolean value that, if true, indicates that the file system is encrypted.
*
*
* @param encrypted
* A Boolean value that, if true, indicates that the file system is encrypted.
*/
public void setEncrypted(Boolean encrypted) {
this.encrypted = encrypted;
}
/**
*
* A Boolean value that, if true, indicates that the file system is encrypted.
*
*
* @return A Boolean value that, if true, indicates that the file system is encrypted.
*/
public Boolean getEncrypted() {
return this.encrypted;
}
/**
*
* A Boolean value that, if true, indicates that the file system is encrypted.
*
*
* @param encrypted
* A Boolean value that, if true, indicates that the file system is encrypted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withEncrypted(Boolean encrypted) {
setEncrypted(encrypted);
return this;
}
/**
*
* A Boolean value that, if true, indicates that the file system is encrypted.
*
*
* @return A Boolean value that, if true, indicates that the file system is encrypted.
*/
public Boolean isEncrypted() {
return this.encrypted;
}
/**
*
* The ID of an KMS key used to protect the encrypted file system.
*
*
* @param kmsKeyId
* The ID of an KMS key used to protect the encrypted file system.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The ID of an KMS key used to protect the encrypted file system.
*
*
* @return The ID of an KMS key used to protect the encrypted file system.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The ID of an KMS key used to protect the encrypted file system.
*
*
* @param kmsKeyId
* The ID of an KMS key used to protect the encrypted file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* Displays the file system's throughput mode. For more information, see Throughput modes in the
* Amazon EFS User Guide.
*
*
* @param throughputMode
* Displays the file system's throughput mode. For more information, see Throughput modes in
* the Amazon EFS User Guide.
* @see ThroughputMode
*/
public void setThroughputMode(String throughputMode) {
this.throughputMode = throughputMode;
}
/**
*
* Displays the file system's throughput mode. For more information, see Throughput modes in the
* Amazon EFS User Guide.
*
*
* @return Displays the file system's throughput mode. For more information, see Throughput modes
* in the Amazon EFS User Guide.
* @see ThroughputMode
*/
public String getThroughputMode() {
return this.throughputMode;
}
/**
*
* Displays the file system's throughput mode. For more information, see Throughput modes in the
* Amazon EFS User Guide.
*
*
* @param throughputMode
* Displays the file system's throughput mode. For more information, see Throughput modes in
* the Amazon EFS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ThroughputMode
*/
public FileSystemDescription withThroughputMode(String throughputMode) {
setThroughputMode(throughputMode);
return this;
}
/**
*
* Displays the file system's throughput mode. For more information, see Throughput modes in the
* Amazon EFS User Guide.
*
*
* @param throughputMode
* Displays the file system's throughput mode. For more information, see Throughput modes in
* the Amazon EFS User Guide.
* @see ThroughputMode
*/
public void setThroughputMode(ThroughputMode throughputMode) {
withThroughputMode(throughputMode);
}
/**
*
* Displays the file system's throughput mode. For more information, see Throughput modes in the
* Amazon EFS User Guide.
*
*
* @param throughputMode
* Displays the file system's throughput mode. For more information, see Throughput modes in
* the Amazon EFS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ThroughputMode
*/
public FileSystemDescription withThroughputMode(ThroughputMode throughputMode) {
this.throughputMode = throughputMode.toString();
return this;
}
/**
*
* The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems using
* ThroughputMode
set to provisioned
.
*
*
* @param provisionedThroughputInMibps
* The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems using
* ThroughputMode
set to provisioned
.
*/
public void setProvisionedThroughputInMibps(Double provisionedThroughputInMibps) {
this.provisionedThroughputInMibps = provisionedThroughputInMibps;
}
/**
*
* The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems using
* ThroughputMode
set to provisioned
.
*
*
* @return The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems
* using ThroughputMode
set to provisioned
.
*/
public Double getProvisionedThroughputInMibps() {
return this.provisionedThroughputInMibps;
}
/**
*
* The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems using
* ThroughputMode
set to provisioned
.
*
*
* @param provisionedThroughputInMibps
* The amount of provisioned throughput, measured in MiBps, for the file system. Valid for file systems using
* ThroughputMode
set to provisioned
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withProvisionedThroughputInMibps(Double provisionedThroughputInMibps) {
setProvisionedThroughputInMibps(provisionedThroughputInMibps);
return this;
}
/**
*
* Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid only for
* One Zone file systems. For more information, see Using EFS storage classes in the
* Amazon EFS User Guide.
*
*
* @param availabilityZoneName
* Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid only
* for One Zone file systems. For more information, see Using EFS storage classes in the
* Amazon EFS User Guide.
*/
public void setAvailabilityZoneName(String availabilityZoneName) {
this.availabilityZoneName = availabilityZoneName;
}
/**
*
* Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid only for
* One Zone file systems. For more information, see Using EFS storage classes in the
* Amazon EFS User Guide.
*
*
* @return Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid
* only for One Zone file systems. For more information, see Using EFS storage classes in
* the Amazon EFS User Guide.
*/
public String getAvailabilityZoneName() {
return this.availabilityZoneName;
}
/**
*
* Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid only for
* One Zone file systems. For more information, see Using EFS storage classes in the
* Amazon EFS User Guide.
*
*
* @param availabilityZoneName
* Describes the Amazon Web Services Availability Zone in which the file system is located, and is valid only
* for One Zone file systems. For more information, see Using EFS storage classes in the
* Amazon EFS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withAvailabilityZoneName(String availabilityZoneName) {
setAvailabilityZoneName(availabilityZoneName);
return this;
}
/**
*
* The unique and consistent identifier of the Availability Zone in which the file system is located, and is valid
* only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for the us-east-1
* Amazon Web Services Region, and it has the same location in every Amazon Web Services account.
*
*
* @param availabilityZoneId
* The unique and consistent identifier of the Availability Zone in which the file system is located, and is
* valid only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for
* the us-east-1 Amazon Web Services Region, and it has the same location in every Amazon Web Services
* account.
*/
public void setAvailabilityZoneId(String availabilityZoneId) {
this.availabilityZoneId = availabilityZoneId;
}
/**
*
* The unique and consistent identifier of the Availability Zone in which the file system is located, and is valid
* only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for the us-east-1
* Amazon Web Services Region, and it has the same location in every Amazon Web Services account.
*
*
* @return The unique and consistent identifier of the Availability Zone in which the file system is located, and is
* valid only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for
* the us-east-1 Amazon Web Services Region, and it has the same location in every Amazon Web Services
* account.
*/
public String getAvailabilityZoneId() {
return this.availabilityZoneId;
}
/**
*
* The unique and consistent identifier of the Availability Zone in which the file system is located, and is valid
* only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for the us-east-1
* Amazon Web Services Region, and it has the same location in every Amazon Web Services account.
*
*
* @param availabilityZoneId
* The unique and consistent identifier of the Availability Zone in which the file system is located, and is
* valid only for One Zone file systems. For example, use1-az1
is an Availability Zone ID for
* the us-east-1 Amazon Web Services Region, and it has the same location in every Amazon Web Services
* account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withAvailabilityZoneId(String availabilityZoneId) {
setAvailabilityZoneId(availabilityZoneId);
return this;
}
/**
*
* The tags associated with the file system, presented as an array of Tag
objects.
*
*
* @return The tags associated with the file system, presented as an array of Tag
objects.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The tags associated with the file system, presented as an array of Tag
objects.
*
*
* @param tags
* The tags associated with the file system, presented as an array of Tag
objects.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The tags associated with the file system, presented as an array of Tag
objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags associated with the file system, presented as an array of Tag
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags associated with the file system, presented as an array of Tag
objects.
*
*
* @param tags
* The tags associated with the file system, presented as an array of Tag
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Describes the protection on the file system.
*
*
* @param fileSystemProtection
* Describes the protection on the file system.
*/
public void setFileSystemProtection(FileSystemProtectionDescription fileSystemProtection) {
this.fileSystemProtection = fileSystemProtection;
}
/**
*
* Describes the protection on the file system.
*
*
* @return Describes the protection on the file system.
*/
public FileSystemProtectionDescription getFileSystemProtection() {
return this.fileSystemProtection;
}
/**
*
* Describes the protection on the file system.
*
*
* @param fileSystemProtection
* Describes the protection on the file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileSystemDescription withFileSystemProtection(FileSystemProtectionDescription fileSystemProtection) {
setFileSystemProtection(fileSystemProtection);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOwnerId() != null)
sb.append("OwnerId: ").append(getOwnerId()).append(",");
if (getCreationToken() != null)
sb.append("CreationToken: ").append(getCreationToken()).append(",");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getFileSystemArn() != null)
sb.append("FileSystemArn: ").append(getFileSystemArn()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLifeCycleState() != null)
sb.append("LifeCycleState: ").append(getLifeCycleState()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getNumberOfMountTargets() != null)
sb.append("NumberOfMountTargets: ").append(getNumberOfMountTargets()).append(",");
if (getSizeInBytes() != null)
sb.append("SizeInBytes: ").append(getSizeInBytes()).append(",");
if (getPerformanceMode() != null)
sb.append("PerformanceMode: ").append(getPerformanceMode()).append(",");
if (getEncrypted() != null)
sb.append("Encrypted: ").append(getEncrypted()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getThroughputMode() != null)
sb.append("ThroughputMode: ").append(getThroughputMode()).append(",");
if (getProvisionedThroughputInMibps() != null)
sb.append("ProvisionedThroughputInMibps: ").append(getProvisionedThroughputInMibps()).append(",");
if (getAvailabilityZoneName() != null)
sb.append("AvailabilityZoneName: ").append(getAvailabilityZoneName()).append(",");
if (getAvailabilityZoneId() != null)
sb.append("AvailabilityZoneId: ").append(getAvailabilityZoneId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getFileSystemProtection() != null)
sb.append("FileSystemProtection: ").append(getFileSystemProtection());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FileSystemDescription == false)
return false;
FileSystemDescription other = (FileSystemDescription) obj;
if (other.getOwnerId() == null ^ this.getOwnerId() == null)
return false;
if (other.getOwnerId() != null && other.getOwnerId().equals(this.getOwnerId()) == false)
return false;
if (other.getCreationToken() == null ^ this.getCreationToken() == null)
return false;
if (other.getCreationToken() != null && other.getCreationToken().equals(this.getCreationToken()) == false)
return false;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getFileSystemArn() == null ^ this.getFileSystemArn() == null)
return false;
if (other.getFileSystemArn() != null && other.getFileSystemArn().equals(this.getFileSystemArn()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLifeCycleState() == null ^ this.getLifeCycleState() == null)
return false;
if (other.getLifeCycleState() != null && other.getLifeCycleState().equals(this.getLifeCycleState()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getNumberOfMountTargets() == null ^ this.getNumberOfMountTargets() == null)
return false;
if (other.getNumberOfMountTargets() != null && other.getNumberOfMountTargets().equals(this.getNumberOfMountTargets()) == false)
return false;
if (other.getSizeInBytes() == null ^ this.getSizeInBytes() == null)
return false;
if (other.getSizeInBytes() != null && other.getSizeInBytes().equals(this.getSizeInBytes()) == false)
return false;
if (other.getPerformanceMode() == null ^ this.getPerformanceMode() == null)
return false;
if (other.getPerformanceMode() != null && other.getPerformanceMode().equals(this.getPerformanceMode()) == false)
return false;
if (other.getEncrypted() == null ^ this.getEncrypted() == null)
return false;
if (other.getEncrypted() != null && other.getEncrypted().equals(this.getEncrypted()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getThroughputMode() == null ^ this.getThroughputMode() == null)
return false;
if (other.getThroughputMode() != null && other.getThroughputMode().equals(this.getThroughputMode()) == false)
return false;
if (other.getProvisionedThroughputInMibps() == null ^ this.getProvisionedThroughputInMibps() == null)
return false;
if (other.getProvisionedThroughputInMibps() != null && other.getProvisionedThroughputInMibps().equals(this.getProvisionedThroughputInMibps()) == false)
return false;
if (other.getAvailabilityZoneName() == null ^ this.getAvailabilityZoneName() == null)
return false;
if (other.getAvailabilityZoneName() != null && other.getAvailabilityZoneName().equals(this.getAvailabilityZoneName()) == false)
return false;
if (other.getAvailabilityZoneId() == null ^ this.getAvailabilityZoneId() == null)
return false;
if (other.getAvailabilityZoneId() != null && other.getAvailabilityZoneId().equals(this.getAvailabilityZoneId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getFileSystemProtection() == null ^ this.getFileSystemProtection() == null)
return false;
if (other.getFileSystemProtection() != null && other.getFileSystemProtection().equals(this.getFileSystemProtection()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOwnerId() == null) ? 0 : getOwnerId().hashCode());
hashCode = prime * hashCode + ((getCreationToken() == null) ? 0 : getCreationToken().hashCode());
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getFileSystemArn() == null) ? 0 : getFileSystemArn().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLifeCycleState() == null) ? 0 : getLifeCycleState().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getNumberOfMountTargets() == null) ? 0 : getNumberOfMountTargets().hashCode());
hashCode = prime * hashCode + ((getSizeInBytes() == null) ? 0 : getSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getPerformanceMode() == null) ? 0 : getPerformanceMode().hashCode());
hashCode = prime * hashCode + ((getEncrypted() == null) ? 0 : getEncrypted().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getThroughputMode() == null) ? 0 : getThroughputMode().hashCode());
hashCode = prime * hashCode + ((getProvisionedThroughputInMibps() == null) ? 0 : getProvisionedThroughputInMibps().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneName() == null) ? 0 : getAvailabilityZoneName().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneId() == null) ? 0 : getAvailabilityZoneId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getFileSystemProtection() == null) ? 0 : getFileSystemProtection().hashCode());
return hashCode;
}
@Override
public FileSystemDescription clone() {
try {
return (FileSystemDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.elasticfilesystem.model.transform.FileSystemDescriptionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}