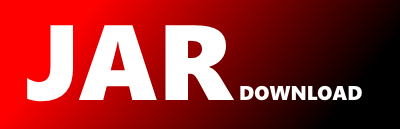
com.amazonaws.services.elasticfilesystem.model.CreateAccessPointRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-efs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticfilesystem.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAccessPointRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
*
*/
private String clientToken;
/**
*
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For more
* information, see Tagging Amazon Web
* Services resources in the Amazon Web Services General Reference Guide.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The ID of the EFS file system that the access point provides access to.
*
*/
private String fileSystemId;
/**
*
* The operating system user and group applied to all file system requests made using the access point.
*
*/
private PosixUser posixUser;
/**
*
* Specifies the directory on the EFS file system that the access point exposes as the root directory of your file
* system to NFS clients using the access point. The clients using the access point can only access the root
* directory and below. If the RootDirectory
> Path
specified does not exist, Amazon
* EFS creates it and applies the CreationInfo
settings when a client connects to an access point. When
* specifying a RootDirectory
, you must provide the Path
, and the
* CreationInfo
.
*
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and permissions
* for the directory. If you do not provide this information, Amazon EFS does not create the root directory. If the
* root directory does not exist, attempts to mount using the access point will fail.
*
*/
private RootDirectory rootDirectory;
/**
*
* A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
*
*
* @param clientToken
* A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
*
*
* @return A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
*
*
* @param clientToken
* A string of up to 64 ASCII characters that Amazon EFS uses to ensure idempotent creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For more
* information, see Tagging Amazon Web
* Services resources in the Amazon Web Services General Reference Guide.
*
*
* @return Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For
* more information, see Tagging
* Amazon Web Services resources in the Amazon Web Services General Reference Guide.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For more
* information, see Tagging Amazon Web
* Services resources in the Amazon Web Services General Reference Guide.
*
*
* @param tags
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For
* more information, see Tagging
* Amazon Web Services resources in the Amazon Web Services General Reference Guide.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For more
* information, see Tagging Amazon Web
* Services resources in the Amazon Web Services General Reference Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For
* more information, see Tagging
* Amazon Web Services resources in the Amazon Web Services General Reference Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For more
* information, see Tagging Amazon Web
* Services resources in the Amazon Web Services General Reference Guide.
*
*
* @param tags
* Creates tags associated with the access point. Each tag is a key-value pair, each key must be unique. For
* more information, see Tagging
* Amazon Web Services resources in the Amazon Web Services General Reference Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The ID of the EFS file system that the access point provides access to.
*
*
* @param fileSystemId
* The ID of the EFS file system that the access point provides access to.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The ID of the EFS file system that the access point provides access to.
*
*
* @return The ID of the EFS file system that the access point provides access to.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The ID of the EFS file system that the access point provides access to.
*
*
* @param fileSystemId
* The ID of the EFS file system that the access point provides access to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointRequest withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* The operating system user and group applied to all file system requests made using the access point.
*
*
* @param posixUser
* The operating system user and group applied to all file system requests made using the access point.
*/
public void setPosixUser(PosixUser posixUser) {
this.posixUser = posixUser;
}
/**
*
* The operating system user and group applied to all file system requests made using the access point.
*
*
* @return The operating system user and group applied to all file system requests made using the access point.
*/
public PosixUser getPosixUser() {
return this.posixUser;
}
/**
*
* The operating system user and group applied to all file system requests made using the access point.
*
*
* @param posixUser
* The operating system user and group applied to all file system requests made using the access point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointRequest withPosixUser(PosixUser posixUser) {
setPosixUser(posixUser);
return this;
}
/**
*
* Specifies the directory on the EFS file system that the access point exposes as the root directory of your file
* system to NFS clients using the access point. The clients using the access point can only access the root
* directory and below. If the RootDirectory
> Path
specified does not exist, Amazon
* EFS creates it and applies the CreationInfo
settings when a client connects to an access point. When
* specifying a RootDirectory
, you must provide the Path
, and the
* CreationInfo
.
*
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and permissions
* for the directory. If you do not provide this information, Amazon EFS does not create the root directory. If the
* root directory does not exist, attempts to mount using the access point will fail.
*
*
* @param rootDirectory
* Specifies the directory on the EFS file system that the access point exposes as the root directory of your
* file system to NFS clients using the access point. The clients using the access point can only access the
* root directory and below. If the RootDirectory
> Path
specified does not
* exist, Amazon EFS creates it and applies the CreationInfo
settings when a client connects to
* an access point. When specifying a RootDirectory
, you must provide the Path
, and
* the CreationInfo
.
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and
* permissions for the directory. If you do not provide this information, Amazon EFS does not create the root
* directory. If the root directory does not exist, attempts to mount using the access point will fail.
*/
public void setRootDirectory(RootDirectory rootDirectory) {
this.rootDirectory = rootDirectory;
}
/**
*
* Specifies the directory on the EFS file system that the access point exposes as the root directory of your file
* system to NFS clients using the access point. The clients using the access point can only access the root
* directory and below. If the RootDirectory
> Path
specified does not exist, Amazon
* EFS creates it and applies the CreationInfo
settings when a client connects to an access point. When
* specifying a RootDirectory
, you must provide the Path
, and the
* CreationInfo
.
*
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and permissions
* for the directory. If you do not provide this information, Amazon EFS does not create the root directory. If the
* root directory does not exist, attempts to mount using the access point will fail.
*
*
* @return Specifies the directory on the EFS file system that the access point exposes as the root directory of
* your file system to NFS clients using the access point. The clients using the access point can only
* access the root directory and below. If the RootDirectory
> Path
specified
* does not exist, Amazon EFS creates it and applies the CreationInfo
settings when a client
* connects to an access point. When specifying a RootDirectory
, you must provide the
* Path
, and the CreationInfo
.
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and
* permissions for the directory. If you do not provide this information, Amazon EFS does not create the
* root directory. If the root directory does not exist, attempts to mount using the access point will fail.
*/
public RootDirectory getRootDirectory() {
return this.rootDirectory;
}
/**
*
* Specifies the directory on the EFS file system that the access point exposes as the root directory of your file
* system to NFS clients using the access point. The clients using the access point can only access the root
* directory and below. If the RootDirectory
> Path
specified does not exist, Amazon
* EFS creates it and applies the CreationInfo
settings when a client connects to an access point. When
* specifying a RootDirectory
, you must provide the Path
, and the
* CreationInfo
.
*
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and permissions
* for the directory. If you do not provide this information, Amazon EFS does not create the root directory. If the
* root directory does not exist, attempts to mount using the access point will fail.
*
*
* @param rootDirectory
* Specifies the directory on the EFS file system that the access point exposes as the root directory of your
* file system to NFS clients using the access point. The clients using the access point can only access the
* root directory and below. If the RootDirectory
> Path
specified does not
* exist, Amazon EFS creates it and applies the CreationInfo
settings when a client connects to
* an access point. When specifying a RootDirectory
, you must provide the Path
, and
* the CreationInfo
.
*
* Amazon EFS creates a root directory only if you have provided the CreationInfo: OwnUid, OwnGID, and
* permissions for the directory. If you do not provide this information, Amazon EFS does not create the root
* directory. If the root directory does not exist, attempts to mount using the access point will fail.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointRequest withRootDirectory(RootDirectory rootDirectory) {
setRootDirectory(rootDirectory);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getPosixUser() != null)
sb.append("PosixUser: ").append(getPosixUser()).append(",");
if (getRootDirectory() != null)
sb.append("RootDirectory: ").append(getRootDirectory());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAccessPointRequest == false)
return false;
CreateAccessPointRequest other = (CreateAccessPointRequest) obj;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getPosixUser() == null ^ this.getPosixUser() == null)
return false;
if (other.getPosixUser() != null && other.getPosixUser().equals(this.getPosixUser()) == false)
return false;
if (other.getRootDirectory() == null ^ this.getRootDirectory() == null)
return false;
if (other.getRootDirectory() != null && other.getRootDirectory().equals(this.getRootDirectory()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getPosixUser() == null) ? 0 : getPosixUser().hashCode());
hashCode = prime * hashCode + ((getRootDirectory() == null) ? 0 : getRootDirectory().hashCode());
return hashCode;
}
@Override
public CreateAccessPointRequest clone() {
return (CreateAccessPointRequest) super.clone();
}
}