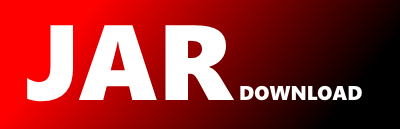
com.amazonaws.services.elasticfilesystem.model.CreateAccessPointResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-efs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticfilesystem.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Provides a description of an EFS file system access point.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAccessPointResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The opaque string specified in the request to ensure idempotent creation.
*
*/
private String clientToken;
/**
*
* The name of the access point. This is the value of the Name
tag.
*
*/
private String name;
/**
*
* The tags associated with the access point, presented as an array of Tag objects.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The ID of the access point, assigned by Amazon EFS.
*
*/
private String accessPointId;
/**
*
* The unique Amazon Resource Name (ARN) associated with the access point.
*
*/
private String accessPointArn;
/**
*
* The ID of the EFS file system that the access point applies to.
*
*/
private String fileSystemId;
/**
*
* The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point that is
* used for all file operations by NFS clients using the access point.
*
*/
private PosixUser posixUser;
/**
*
* The directory on the EFS file system that the access point exposes as the root directory to NFS clients using the
* access point.
*
*/
private RootDirectory rootDirectory;
/**
*
* Identifies the Amazon Web Services account that owns the access point resource.
*
*/
private String ownerId;
/**
*
* Identifies the lifecycle phase of the access point.
*
*/
private String lifeCycleState;
/**
*
* The opaque string specified in the request to ensure idempotent creation.
*
*
* @param clientToken
* The opaque string specified in the request to ensure idempotent creation.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* The opaque string specified in the request to ensure idempotent creation.
*
*
* @return The opaque string specified in the request to ensure idempotent creation.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* The opaque string specified in the request to ensure idempotent creation.
*
*
* @param clientToken
* The opaque string specified in the request to ensure idempotent creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The name of the access point. This is the value of the Name
tag.
*
*
* @param name
* The name of the access point. This is the value of the Name
tag.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the access point. This is the value of the Name
tag.
*
*
* @return The name of the access point. This is the value of the Name
tag.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the access point. This is the value of the Name
tag.
*
*
* @param name
* The name of the access point. This is the value of the Name
tag.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withName(String name) {
setName(name);
return this;
}
/**
*
* The tags associated with the access point, presented as an array of Tag objects.
*
*
* @return The tags associated with the access point, presented as an array of Tag objects.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The tags associated with the access point, presented as an array of Tag objects.
*
*
* @param tags
* The tags associated with the access point, presented as an array of Tag objects.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The tags associated with the access point, presented as an array of Tag objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags associated with the access point, presented as an array of Tag objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags associated with the access point, presented as an array of Tag objects.
*
*
* @param tags
* The tags associated with the access point, presented as an array of Tag objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The ID of the access point, assigned by Amazon EFS.
*
*
* @param accessPointId
* The ID of the access point, assigned by Amazon EFS.
*/
public void setAccessPointId(String accessPointId) {
this.accessPointId = accessPointId;
}
/**
*
* The ID of the access point, assigned by Amazon EFS.
*
*
* @return The ID of the access point, assigned by Amazon EFS.
*/
public String getAccessPointId() {
return this.accessPointId;
}
/**
*
* The ID of the access point, assigned by Amazon EFS.
*
*
* @param accessPointId
* The ID of the access point, assigned by Amazon EFS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withAccessPointId(String accessPointId) {
setAccessPointId(accessPointId);
return this;
}
/**
*
* The unique Amazon Resource Name (ARN) associated with the access point.
*
*
* @param accessPointArn
* The unique Amazon Resource Name (ARN) associated with the access point.
*/
public void setAccessPointArn(String accessPointArn) {
this.accessPointArn = accessPointArn;
}
/**
*
* The unique Amazon Resource Name (ARN) associated with the access point.
*
*
* @return The unique Amazon Resource Name (ARN) associated with the access point.
*/
public String getAccessPointArn() {
return this.accessPointArn;
}
/**
*
* The unique Amazon Resource Name (ARN) associated with the access point.
*
*
* @param accessPointArn
* The unique Amazon Resource Name (ARN) associated with the access point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withAccessPointArn(String accessPointArn) {
setAccessPointArn(accessPointArn);
return this;
}
/**
*
* The ID of the EFS file system that the access point applies to.
*
*
* @param fileSystemId
* The ID of the EFS file system that the access point applies to.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The ID of the EFS file system that the access point applies to.
*
*
* @return The ID of the EFS file system that the access point applies to.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The ID of the EFS file system that the access point applies to.
*
*
* @param fileSystemId
* The ID of the EFS file system that the access point applies to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point that is
* used for all file operations by NFS clients using the access point.
*
*
* @param posixUser
* The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point that
* is used for all file operations by NFS clients using the access point.
*/
public void setPosixUser(PosixUser posixUser) {
this.posixUser = posixUser;
}
/**
*
* The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point that is
* used for all file operations by NFS clients using the access point.
*
*
* @return The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point
* that is used for all file operations by NFS clients using the access point.
*/
public PosixUser getPosixUser() {
return this.posixUser;
}
/**
*
* The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point that is
* used for all file operations by NFS clients using the access point.
*
*
* @param posixUser
* The full POSIX identity, including the user ID, group ID, and secondary group IDs on the access point that
* is used for all file operations by NFS clients using the access point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withPosixUser(PosixUser posixUser) {
setPosixUser(posixUser);
return this;
}
/**
*
* The directory on the EFS file system that the access point exposes as the root directory to NFS clients using the
* access point.
*
*
* @param rootDirectory
* The directory on the EFS file system that the access point exposes as the root directory to NFS clients
* using the access point.
*/
public void setRootDirectory(RootDirectory rootDirectory) {
this.rootDirectory = rootDirectory;
}
/**
*
* The directory on the EFS file system that the access point exposes as the root directory to NFS clients using the
* access point.
*
*
* @return The directory on the EFS file system that the access point exposes as the root directory to NFS clients
* using the access point.
*/
public RootDirectory getRootDirectory() {
return this.rootDirectory;
}
/**
*
* The directory on the EFS file system that the access point exposes as the root directory to NFS clients using the
* access point.
*
*
* @param rootDirectory
* The directory on the EFS file system that the access point exposes as the root directory to NFS clients
* using the access point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withRootDirectory(RootDirectory rootDirectory) {
setRootDirectory(rootDirectory);
return this;
}
/**
*
* Identifies the Amazon Web Services account that owns the access point resource.
*
*
* @param ownerId
* Identifies the Amazon Web Services account that owns the access point resource.
*/
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
/**
*
* Identifies the Amazon Web Services account that owns the access point resource.
*
*
* @return Identifies the Amazon Web Services account that owns the access point resource.
*/
public String getOwnerId() {
return this.ownerId;
}
/**
*
* Identifies the Amazon Web Services account that owns the access point resource.
*
*
* @param ownerId
* Identifies the Amazon Web Services account that owns the access point resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessPointResult withOwnerId(String ownerId) {
setOwnerId(ownerId);
return this;
}
/**
*
* Identifies the lifecycle phase of the access point.
*
*
* @param lifeCycleState
* Identifies the lifecycle phase of the access point.
* @see LifeCycleState
*/
public void setLifeCycleState(String lifeCycleState) {
this.lifeCycleState = lifeCycleState;
}
/**
*
* Identifies the lifecycle phase of the access point.
*
*
* @return Identifies the lifecycle phase of the access point.
* @see LifeCycleState
*/
public String getLifeCycleState() {
return this.lifeCycleState;
}
/**
*
* Identifies the lifecycle phase of the access point.
*
*
* @param lifeCycleState
* Identifies the lifecycle phase of the access point.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LifeCycleState
*/
public CreateAccessPointResult withLifeCycleState(String lifeCycleState) {
setLifeCycleState(lifeCycleState);
return this;
}
/**
*
* Identifies the lifecycle phase of the access point.
*
*
* @param lifeCycleState
* Identifies the lifecycle phase of the access point.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LifeCycleState
*/
public CreateAccessPointResult withLifeCycleState(LifeCycleState lifeCycleState) {
this.lifeCycleState = lifeCycleState.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAccessPointId() != null)
sb.append("AccessPointId: ").append(getAccessPointId()).append(",");
if (getAccessPointArn() != null)
sb.append("AccessPointArn: ").append(getAccessPointArn()).append(",");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getPosixUser() != null)
sb.append("PosixUser: ").append(getPosixUser()).append(",");
if (getRootDirectory() != null)
sb.append("RootDirectory: ").append(getRootDirectory()).append(",");
if (getOwnerId() != null)
sb.append("OwnerId: ").append(getOwnerId()).append(",");
if (getLifeCycleState() != null)
sb.append("LifeCycleState: ").append(getLifeCycleState());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAccessPointResult == false)
return false;
CreateAccessPointResult other = (CreateAccessPointResult) obj;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAccessPointId() == null ^ this.getAccessPointId() == null)
return false;
if (other.getAccessPointId() != null && other.getAccessPointId().equals(this.getAccessPointId()) == false)
return false;
if (other.getAccessPointArn() == null ^ this.getAccessPointArn() == null)
return false;
if (other.getAccessPointArn() != null && other.getAccessPointArn().equals(this.getAccessPointArn()) == false)
return false;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getPosixUser() == null ^ this.getPosixUser() == null)
return false;
if (other.getPosixUser() != null && other.getPosixUser().equals(this.getPosixUser()) == false)
return false;
if (other.getRootDirectory() == null ^ this.getRootDirectory() == null)
return false;
if (other.getRootDirectory() != null && other.getRootDirectory().equals(this.getRootDirectory()) == false)
return false;
if (other.getOwnerId() == null ^ this.getOwnerId() == null)
return false;
if (other.getOwnerId() != null && other.getOwnerId().equals(this.getOwnerId()) == false)
return false;
if (other.getLifeCycleState() == null ^ this.getLifeCycleState() == null)
return false;
if (other.getLifeCycleState() != null && other.getLifeCycleState().equals(this.getLifeCycleState()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAccessPointId() == null) ? 0 : getAccessPointId().hashCode());
hashCode = prime * hashCode + ((getAccessPointArn() == null) ? 0 : getAccessPointArn().hashCode());
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getPosixUser() == null) ? 0 : getPosixUser().hashCode());
hashCode = prime * hashCode + ((getRootDirectory() == null) ? 0 : getRootDirectory().hashCode());
hashCode = prime * hashCode + ((getOwnerId() == null) ? 0 : getOwnerId().hashCode());
hashCode = prime * hashCode + ((getLifeCycleState() == null) ? 0 : getLifeCycleState().hashCode());
return hashCode;
}
@Override
public CreateAccessPointResult clone() {
try {
return (CreateAccessPointResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}