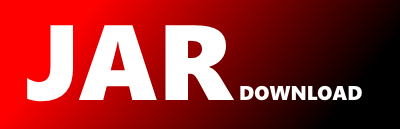
com.amazonaws.services.elasticfilesystem.model.CreateMountTargetResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-efs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticfilesystem.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Provides a description of a mount target.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateMountTargetResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* Amazon Web Services account ID that owns the resource.
*
*/
private String ownerId;
/**
*
* System-assigned mount target ID.
*
*/
private String mountTargetId;
/**
*
* The ID of the file system for which the mount target is intended.
*
*/
private String fileSystemId;
/**
*
* The ID of the mount target's subnet.
*
*/
private String subnetId;
/**
*
* Lifecycle state of the mount target.
*
*/
private String lifeCycleState;
/**
*
* Address at which the file system can be mounted by using the mount target.
*
*/
private String ipAddress;
/**
*
* The ID of the network interface that Amazon EFS created when it created the mount target.
*
*/
private String networkInterfaceId;
/**
*
* The unique and consistent identifier of the Availability Zone that the mount target resides in. For example,
* use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every Amazon Web
* Services account.
*
*/
private String availabilityZoneId;
/**
*
* The name of the Availability Zone in which the mount target is located. Availability Zones are independently
* mapped to names for each Amazon Web Services account. For example, the Availability Zone us-east-1a
* for your Amazon Web Services account might not be the same location as us-east-1a
for another Amazon
* Web Services account.
*
*/
private String availabilityZoneName;
/**
*
* The virtual private cloud (VPC) ID that the mount target is configured in.
*
*/
private String vpcId;
/**
*
* Amazon Web Services account ID that owns the resource.
*
*
* @param ownerId
* Amazon Web Services account ID that owns the resource.
*/
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
/**
*
* Amazon Web Services account ID that owns the resource.
*
*
* @return Amazon Web Services account ID that owns the resource.
*/
public String getOwnerId() {
return this.ownerId;
}
/**
*
* Amazon Web Services account ID that owns the resource.
*
*
* @param ownerId
* Amazon Web Services account ID that owns the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withOwnerId(String ownerId) {
setOwnerId(ownerId);
return this;
}
/**
*
* System-assigned mount target ID.
*
*
* @param mountTargetId
* System-assigned mount target ID.
*/
public void setMountTargetId(String mountTargetId) {
this.mountTargetId = mountTargetId;
}
/**
*
* System-assigned mount target ID.
*
*
* @return System-assigned mount target ID.
*/
public String getMountTargetId() {
return this.mountTargetId;
}
/**
*
* System-assigned mount target ID.
*
*
* @param mountTargetId
* System-assigned mount target ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withMountTargetId(String mountTargetId) {
setMountTargetId(mountTargetId);
return this;
}
/**
*
* The ID of the file system for which the mount target is intended.
*
*
* @param fileSystemId
* The ID of the file system for which the mount target is intended.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The ID of the file system for which the mount target is intended.
*
*
* @return The ID of the file system for which the mount target is intended.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The ID of the file system for which the mount target is intended.
*
*
* @param fileSystemId
* The ID of the file system for which the mount target is intended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* The ID of the mount target's subnet.
*
*
* @param subnetId
* The ID of the mount target's subnet.
*/
public void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
/**
*
* The ID of the mount target's subnet.
*
*
* @return The ID of the mount target's subnet.
*/
public String getSubnetId() {
return this.subnetId;
}
/**
*
* The ID of the mount target's subnet.
*
*
* @param subnetId
* The ID of the mount target's subnet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withSubnetId(String subnetId) {
setSubnetId(subnetId);
return this;
}
/**
*
* Lifecycle state of the mount target.
*
*
* @param lifeCycleState
* Lifecycle state of the mount target.
* @see LifeCycleState
*/
public void setLifeCycleState(String lifeCycleState) {
this.lifeCycleState = lifeCycleState;
}
/**
*
* Lifecycle state of the mount target.
*
*
* @return Lifecycle state of the mount target.
* @see LifeCycleState
*/
public String getLifeCycleState() {
return this.lifeCycleState;
}
/**
*
* Lifecycle state of the mount target.
*
*
* @param lifeCycleState
* Lifecycle state of the mount target.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LifeCycleState
*/
public CreateMountTargetResult withLifeCycleState(String lifeCycleState) {
setLifeCycleState(lifeCycleState);
return this;
}
/**
*
* Lifecycle state of the mount target.
*
*
* @param lifeCycleState
* Lifecycle state of the mount target.
* @see LifeCycleState
*/
public void setLifeCycleState(LifeCycleState lifeCycleState) {
withLifeCycleState(lifeCycleState);
}
/**
*
* Lifecycle state of the mount target.
*
*
* @param lifeCycleState
* Lifecycle state of the mount target.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LifeCycleState
*/
public CreateMountTargetResult withLifeCycleState(LifeCycleState lifeCycleState) {
this.lifeCycleState = lifeCycleState.toString();
return this;
}
/**
*
* Address at which the file system can be mounted by using the mount target.
*
*
* @param ipAddress
* Address at which the file system can be mounted by using the mount target.
*/
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
/**
*
* Address at which the file system can be mounted by using the mount target.
*
*
* @return Address at which the file system can be mounted by using the mount target.
*/
public String getIpAddress() {
return this.ipAddress;
}
/**
*
* Address at which the file system can be mounted by using the mount target.
*
*
* @param ipAddress
* Address at which the file system can be mounted by using the mount target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withIpAddress(String ipAddress) {
setIpAddress(ipAddress);
return this;
}
/**
*
* The ID of the network interface that Amazon EFS created when it created the mount target.
*
*
* @param networkInterfaceId
* The ID of the network interface that Amazon EFS created when it created the mount target.
*/
public void setNetworkInterfaceId(String networkInterfaceId) {
this.networkInterfaceId = networkInterfaceId;
}
/**
*
* The ID of the network interface that Amazon EFS created when it created the mount target.
*
*
* @return The ID of the network interface that Amazon EFS created when it created the mount target.
*/
public String getNetworkInterfaceId() {
return this.networkInterfaceId;
}
/**
*
* The ID of the network interface that Amazon EFS created when it created the mount target.
*
*
* @param networkInterfaceId
* The ID of the network interface that Amazon EFS created when it created the mount target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withNetworkInterfaceId(String networkInterfaceId) {
setNetworkInterfaceId(networkInterfaceId);
return this;
}
/**
*
* The unique and consistent identifier of the Availability Zone that the mount target resides in. For example,
* use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every Amazon Web
* Services account.
*
*
* @param availabilityZoneId
* The unique and consistent identifier of the Availability Zone that the mount target resides in. For
* example, use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every
* Amazon Web Services account.
*/
public void setAvailabilityZoneId(String availabilityZoneId) {
this.availabilityZoneId = availabilityZoneId;
}
/**
*
* The unique and consistent identifier of the Availability Zone that the mount target resides in. For example,
* use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every Amazon Web
* Services account.
*
*
* @return The unique and consistent identifier of the Availability Zone that the mount target resides in. For
* example, use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every
* Amazon Web Services account.
*/
public String getAvailabilityZoneId() {
return this.availabilityZoneId;
}
/**
*
* The unique and consistent identifier of the Availability Zone that the mount target resides in. For example,
* use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every Amazon Web
* Services account.
*
*
* @param availabilityZoneId
* The unique and consistent identifier of the Availability Zone that the mount target resides in. For
* example, use1-az1
is an AZ ID for the us-east-1 Region and it has the same location in every
* Amazon Web Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withAvailabilityZoneId(String availabilityZoneId) {
setAvailabilityZoneId(availabilityZoneId);
return this;
}
/**
*
* The name of the Availability Zone in which the mount target is located. Availability Zones are independently
* mapped to names for each Amazon Web Services account. For example, the Availability Zone us-east-1a
* for your Amazon Web Services account might not be the same location as us-east-1a
for another Amazon
* Web Services account.
*
*
* @param availabilityZoneName
* The name of the Availability Zone in which the mount target is located. Availability Zones are
* independently mapped to names for each Amazon Web Services account. For example, the Availability Zone
* us-east-1a
for your Amazon Web Services account might not be the same location as
* us-east-1a
for another Amazon Web Services account.
*/
public void setAvailabilityZoneName(String availabilityZoneName) {
this.availabilityZoneName = availabilityZoneName;
}
/**
*
* The name of the Availability Zone in which the mount target is located. Availability Zones are independently
* mapped to names for each Amazon Web Services account. For example, the Availability Zone us-east-1a
* for your Amazon Web Services account might not be the same location as us-east-1a
for another Amazon
* Web Services account.
*
*
* @return The name of the Availability Zone in which the mount target is located. Availability Zones are
* independently mapped to names for each Amazon Web Services account. For example, the Availability Zone
* us-east-1a
for your Amazon Web Services account might not be the same location as
* us-east-1a
for another Amazon Web Services account.
*/
public String getAvailabilityZoneName() {
return this.availabilityZoneName;
}
/**
*
* The name of the Availability Zone in which the mount target is located. Availability Zones are independently
* mapped to names for each Amazon Web Services account. For example, the Availability Zone us-east-1a
* for your Amazon Web Services account might not be the same location as us-east-1a
for another Amazon
* Web Services account.
*
*
* @param availabilityZoneName
* The name of the Availability Zone in which the mount target is located. Availability Zones are
* independently mapped to names for each Amazon Web Services account. For example, the Availability Zone
* us-east-1a
for your Amazon Web Services account might not be the same location as
* us-east-1a
for another Amazon Web Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withAvailabilityZoneName(String availabilityZoneName) {
setAvailabilityZoneName(availabilityZoneName);
return this;
}
/**
*
* The virtual private cloud (VPC) ID that the mount target is configured in.
*
*
* @param vpcId
* The virtual private cloud (VPC) ID that the mount target is configured in.
*/
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
/**
*
* The virtual private cloud (VPC) ID that the mount target is configured in.
*
*
* @return The virtual private cloud (VPC) ID that the mount target is configured in.
*/
public String getVpcId() {
return this.vpcId;
}
/**
*
* The virtual private cloud (VPC) ID that the mount target is configured in.
*
*
* @param vpcId
* The virtual private cloud (VPC) ID that the mount target is configured in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMountTargetResult withVpcId(String vpcId) {
setVpcId(vpcId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOwnerId() != null)
sb.append("OwnerId: ").append(getOwnerId()).append(",");
if (getMountTargetId() != null)
sb.append("MountTargetId: ").append(getMountTargetId()).append(",");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getSubnetId() != null)
sb.append("SubnetId: ").append(getSubnetId()).append(",");
if (getLifeCycleState() != null)
sb.append("LifeCycleState: ").append(getLifeCycleState()).append(",");
if (getIpAddress() != null)
sb.append("IpAddress: ").append(getIpAddress()).append(",");
if (getNetworkInterfaceId() != null)
sb.append("NetworkInterfaceId: ").append(getNetworkInterfaceId()).append(",");
if (getAvailabilityZoneId() != null)
sb.append("AvailabilityZoneId: ").append(getAvailabilityZoneId()).append(",");
if (getAvailabilityZoneName() != null)
sb.append("AvailabilityZoneName: ").append(getAvailabilityZoneName()).append(",");
if (getVpcId() != null)
sb.append("VpcId: ").append(getVpcId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateMountTargetResult == false)
return false;
CreateMountTargetResult other = (CreateMountTargetResult) obj;
if (other.getOwnerId() == null ^ this.getOwnerId() == null)
return false;
if (other.getOwnerId() != null && other.getOwnerId().equals(this.getOwnerId()) == false)
return false;
if (other.getMountTargetId() == null ^ this.getMountTargetId() == null)
return false;
if (other.getMountTargetId() != null && other.getMountTargetId().equals(this.getMountTargetId()) == false)
return false;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getSubnetId() == null ^ this.getSubnetId() == null)
return false;
if (other.getSubnetId() != null && other.getSubnetId().equals(this.getSubnetId()) == false)
return false;
if (other.getLifeCycleState() == null ^ this.getLifeCycleState() == null)
return false;
if (other.getLifeCycleState() != null && other.getLifeCycleState().equals(this.getLifeCycleState()) == false)
return false;
if (other.getIpAddress() == null ^ this.getIpAddress() == null)
return false;
if (other.getIpAddress() != null && other.getIpAddress().equals(this.getIpAddress()) == false)
return false;
if (other.getNetworkInterfaceId() == null ^ this.getNetworkInterfaceId() == null)
return false;
if (other.getNetworkInterfaceId() != null && other.getNetworkInterfaceId().equals(this.getNetworkInterfaceId()) == false)
return false;
if (other.getAvailabilityZoneId() == null ^ this.getAvailabilityZoneId() == null)
return false;
if (other.getAvailabilityZoneId() != null && other.getAvailabilityZoneId().equals(this.getAvailabilityZoneId()) == false)
return false;
if (other.getAvailabilityZoneName() == null ^ this.getAvailabilityZoneName() == null)
return false;
if (other.getAvailabilityZoneName() != null && other.getAvailabilityZoneName().equals(this.getAvailabilityZoneName()) == false)
return false;
if (other.getVpcId() == null ^ this.getVpcId() == null)
return false;
if (other.getVpcId() != null && other.getVpcId().equals(this.getVpcId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOwnerId() == null) ? 0 : getOwnerId().hashCode());
hashCode = prime * hashCode + ((getMountTargetId() == null) ? 0 : getMountTargetId().hashCode());
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getSubnetId() == null) ? 0 : getSubnetId().hashCode());
hashCode = prime * hashCode + ((getLifeCycleState() == null) ? 0 : getLifeCycleState().hashCode());
hashCode = prime * hashCode + ((getIpAddress() == null) ? 0 : getIpAddress().hashCode());
hashCode = prime * hashCode + ((getNetworkInterfaceId() == null) ? 0 : getNetworkInterfaceId().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneId() == null) ? 0 : getAvailabilityZoneId().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneName() == null) ? 0 : getAvailabilityZoneName().hashCode());
hashCode = prime * hashCode + ((getVpcId() == null) ? 0 : getVpcId().hashCode());
return hashCode;
}
@Override
public CreateMountTargetResult clone() {
try {
return (CreateMountTargetResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}