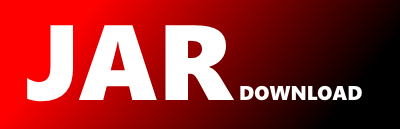
com.amazonaws.services.elasticfilesystem.model.PutLifecycleConfigurationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-efs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticfilesystem.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutLifecycleConfigurationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the file system for which you are creating the LifecycleConfiguration
object (String).
*
*/
private String fileSystemId;
/**
*
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS Lifecycle
* management of the following:
*
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage (Standard
* storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current storage
* class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the General
* Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to primary
* storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the PutLifecycleConfiguration
* API action, Amazon EFS requires that each LifecyclePolicy
object have only a single transition. This
* means that in a request body, LifecyclePolicies
must be structured as an array of
* LifecyclePolicy
objects, one object for each storage transition. See the example requests in the
* following section for more information.
*
*
*/
private com.amazonaws.internal.SdkInternalList lifecyclePolicies;
/**
*
* The ID of the file system for which you are creating the LifecycleConfiguration
object (String).
*
*
* @param fileSystemId
* The ID of the file system for which you are creating the LifecycleConfiguration
object
* (String).
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The ID of the file system for which you are creating the LifecycleConfiguration
object (String).
*
*
* @return The ID of the file system for which you are creating the LifecycleConfiguration
object
* (String).
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The ID of the file system for which you are creating the LifecycleConfiguration
object (String).
*
*
* @param fileSystemId
* The ID of the file system for which you are creating the LifecycleConfiguration
object
* (String).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutLifecycleConfigurationRequest withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS Lifecycle
* management of the following:
*
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage (Standard
* storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current storage
* class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the General
* Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to primary
* storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the PutLifecycleConfiguration
* API action, Amazon EFS requires that each LifecyclePolicy
object have only a single transition. This
* means that in a request body, LifecyclePolicies
must be structured as an array of
* LifecyclePolicy
objects, one object for each storage transition. See the example requests in the
* following section for more information.
*
*
*
* @return An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS
* Lifecycle management of the following:
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage
* (Standard storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current
* storage class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the
* General Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to
* primary storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the
* PutLifecycleConfiguration
API action, Amazon EFS requires that each
* LifecyclePolicy
object have only a single transition. This means that in a request body,
* LifecyclePolicies
must be structured as an array of LifecyclePolicy
objects,
* one object for each storage transition. See the example requests in the following section for more
* information.
*
*/
public java.util.List getLifecyclePolicies() {
if (lifecyclePolicies == null) {
lifecyclePolicies = new com.amazonaws.internal.SdkInternalList();
}
return lifecyclePolicies;
}
/**
*
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS Lifecycle
* management of the following:
*
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage (Standard
* storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current storage
* class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the General
* Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to primary
* storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the PutLifecycleConfiguration
* API action, Amazon EFS requires that each LifecyclePolicy
object have only a single transition. This
* means that in a request body, LifecyclePolicies
must be structured as an array of
* LifecyclePolicy
objects, one object for each storage transition. See the example requests in the
* following section for more information.
*
*
*
* @param lifecyclePolicies
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS
* Lifecycle management of the following:
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage
* (Standard storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current
* storage class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the
* General Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to
* primary storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the
* PutLifecycleConfiguration
API action, Amazon EFS requires that each
* LifecyclePolicy
object have only a single transition. This means that in a request body,
* LifecyclePolicies
must be structured as an array of LifecyclePolicy
objects, one
* object for each storage transition. See the example requests in the following section for more
* information.
*
*/
public void setLifecyclePolicies(java.util.Collection lifecyclePolicies) {
if (lifecyclePolicies == null) {
this.lifecyclePolicies = null;
return;
}
this.lifecyclePolicies = new com.amazonaws.internal.SdkInternalList(lifecyclePolicies);
}
/**
*
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS Lifecycle
* management of the following:
*
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage (Standard
* storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current storage
* class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the General
* Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to primary
* storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the PutLifecycleConfiguration
* API action, Amazon EFS requires that each LifecyclePolicy
object have only a single transition. This
* means that in a request body, LifecyclePolicies
must be structured as an array of
* LifecyclePolicy
objects, one object for each storage transition. See the example requests in the
* following section for more information.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLifecyclePolicies(java.util.Collection)} or {@link #withLifecyclePolicies(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param lifecyclePolicies
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS
* Lifecycle management of the following:
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage
* (Standard storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current
* storage class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the
* General Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to
* primary storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the
* PutLifecycleConfiguration
API action, Amazon EFS requires that each
* LifecyclePolicy
object have only a single transition. This means that in a request body,
* LifecyclePolicies
must be structured as an array of LifecyclePolicy
objects, one
* object for each storage transition. See the example requests in the following section for more
* information.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutLifecycleConfigurationRequest withLifecyclePolicies(LifecyclePolicy... lifecyclePolicies) {
if (this.lifecyclePolicies == null) {
setLifecyclePolicies(new com.amazonaws.internal.SdkInternalList(lifecyclePolicies.length));
}
for (LifecyclePolicy ele : lifecyclePolicies) {
this.lifecyclePolicies.add(ele);
}
return this;
}
/**
*
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS Lifecycle
* management of the following:
*
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage (Standard
* storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current storage
* class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the General
* Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to primary
* storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the PutLifecycleConfiguration
* API action, Amazon EFS requires that each LifecyclePolicy
object have only a single transition. This
* means that in a request body, LifecyclePolicies
must be structured as an array of
* LifecyclePolicy
objects, one object for each storage transition. See the example requests in the
* following section for more information.
*
*
*
* @param lifecyclePolicies
* An array of LifecyclePolicy
objects that define the file system's
* LifecycleConfiguration
object. A LifecycleConfiguration
object informs EFS
* Lifecycle management of the following:
*
* -
*
* TransitionToIA
– When to move files in the file system from primary storage
* (Standard storage class) into the Infrequent Access (IA) storage.
*
*
* -
*
* TransitionToArchive
– When to move files in the file system from their current
* storage class (either IA or Standard storage) into the Archive storage.
*
*
* File systems cannot transition into Archive storage before transitioning into IA storage. Therefore,
* TransitionToArchive must either not be set or must be later than TransitionToIA.
*
*
*
* The Archive storage class is available only for file systems that use the Elastic Throughput mode and the
* General Purpose Performance mode.
*
*
* -
*
* TransitionToPrimaryStorageClass
– Whether to move files in the file system back to
* primary storage (Standard storage class) after they are accessed in IA or Archive storage.
*
*
*
*
*
* When using the put-lifecycle-configuration
CLI command or the
* PutLifecycleConfiguration
API action, Amazon EFS requires that each
* LifecyclePolicy
object have only a single transition. This means that in a request body,
* LifecyclePolicies
must be structured as an array of LifecyclePolicy
objects, one
* object for each storage transition. See the example requests in the following section for more
* information.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutLifecycleConfigurationRequest withLifecyclePolicies(java.util.Collection lifecyclePolicies) {
setLifecyclePolicies(lifecyclePolicies);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getLifecyclePolicies() != null)
sb.append("LifecyclePolicies: ").append(getLifecyclePolicies());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutLifecycleConfigurationRequest == false)
return false;
PutLifecycleConfigurationRequest other = (PutLifecycleConfigurationRequest) obj;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getLifecyclePolicies() == null ^ this.getLifecyclePolicies() == null)
return false;
if (other.getLifecyclePolicies() != null && other.getLifecyclePolicies().equals(this.getLifecyclePolicies()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getLifecyclePolicies() == null) ? 0 : getLifecyclePolicies().hashCode());
return hashCode;
}
@Override
public PutLifecycleConfigurationRequest clone() {
return (PutLifecycleConfigurationRequest) super.clone();
}
}