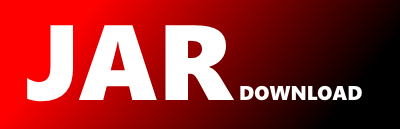
com.amazonaws.services.elasticache.AmazonElastiCacheAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticache;
import com.amazonaws.services.elasticache.model.*;
/**
* Interface for accessing Amazon ElastiCache asynchronously. Each asynchronous
* method will return a Java Future object representing the asynchronous
* operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Amazon ElastiCache
*
* Amazon ElastiCache is a web service that makes it easier to set up, operate,
* and scale a distributed cache in the cloud.
*
*
* With ElastiCache, customers gain all of the benefits of a high-performance,
* in-memory cache with far less of the administrative burden of launching and
* managing a distributed cache. The service makes setup, scaling, and cluster
* failure handling much simpler than in a self-managed cache deployment.
*
*
* In addition, through integration with Amazon CloudWatch, customers get
* enhanced visibility into the key performance statistics associated with their
* cache and can receive alarms if a part of their cache runs hot.
*
*/
public interface AmazonElastiCacheAsync extends AmazonElastiCache {
/**
*
* The AddTagsToResource action adds up to 10 cost allocation tags to
* the named resource. A cost allocation tag is a key-value pair
* where the key and value are case-sensitive. Cost allocation tags can be
* used to categorize and track your AWS costs.
*
*
* When you apply tags to your ElastiCache resources, AWS generates a cost
* allocation report as a comma-separated value (CSV) file with your usage
* and costs aggregated by your tags. You can apply tags that represent
* business categories (such as cost centers, application names, or owners)
* to organize your costs across multiple services. For more information,
* see Using Cost Allocation Tags in Amazon ElastiCache in the
* ElastiCache User Guide.
*
*
* @param addTagsToResourceRequest
* Represents the input of an AddTagsToResource action.
* @return A Java Future containing the result of the AddTagsToResource
* operation returned by the service.
* @sample AmazonElastiCacheAsync.AddTagsToResource
*/
java.util.concurrent.Future addTagsToResourceAsync(
AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* The AddTagsToResource action adds up to 10 cost allocation tags to
* the named resource. A cost allocation tag is a key-value pair
* where the key and value are case-sensitive. Cost allocation tags can be
* used to categorize and track your AWS costs.
*
*
* When you apply tags to your ElastiCache resources, AWS generates a cost
* allocation report as a comma-separated value (CSV) file with your usage
* and costs aggregated by your tags. You can apply tags that represent
* business categories (such as cost centers, application names, or owners)
* to organize your costs across multiple services. For more information,
* see Using Cost Allocation Tags in Amazon ElastiCache in the
* ElastiCache User Guide.
*
*
* @param addTagsToResourceRequest
* Represents the input of an AddTagsToResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.AddTagsToResource
*/
java.util.concurrent.Future addTagsToResourceAsync(
AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The AuthorizeCacheSecurityGroupIngress action allows network
* ingress to a cache security group. Applications using ElastiCache must be
* running on Amazon EC2, and Amazon EC2 security groups are used as the
* authorization mechanism.
*
*
*
* You cannot authorize ingress from an Amazon EC2 security group in one
* region to an ElastiCache cluster in another region.
*
*
*
* @param authorizeCacheSecurityGroupIngressRequest
* Represents the input of an
* AuthorizeCacheSecurityGroupIngress action.
* @return A Java Future containing the result of the
* AuthorizeCacheSecurityGroupIngress operation returned by the
* service.
* @sample AmazonElastiCacheAsync.AuthorizeCacheSecurityGroupIngress
*/
java.util.concurrent.Future authorizeCacheSecurityGroupIngressAsync(
AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest);
/**
*
* The AuthorizeCacheSecurityGroupIngress action allows network
* ingress to a cache security group. Applications using ElastiCache must be
* running on Amazon EC2, and Amazon EC2 security groups are used as the
* authorization mechanism.
*
*
*
* You cannot authorize ingress from an Amazon EC2 security group in one
* region to an ElastiCache cluster in another region.
*
*
*
* @param authorizeCacheSecurityGroupIngressRequest
* Represents the input of an
* AuthorizeCacheSecurityGroupIngress action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* AuthorizeCacheSecurityGroupIngress operation returned by the
* service.
* @sample AmazonElastiCacheAsyncHandler.AuthorizeCacheSecurityGroupIngress
*/
java.util.concurrent.Future authorizeCacheSecurityGroupIngressAsync(
AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CopySnapshot action makes a copy of an existing snapshot.
*
*
*
* Users or groups that have permissions to use the CopySnapshot API
* can create their own Amazon S3 buckets and copy snapshots to it. To
* control access to your snapshots, use an IAM policy to control who has
* the ability to use the CopySnapshot API. For more information
* about using IAM to control the use of ElastiCache APIs, see Exporting Snapshots and Authentication & Access Control.
*
*
*
* Erorr Message:
*
*
* -
*
* Error Message: The authenticated user does not have sufficient
* permissions to perform the desired activity.
*
*
* Solution: Contact your system administrator to get the needed
* permissions.
*
*
*
*
* @param copySnapshotRequest
* Represents the input of a CopySnapshotMessage action.
* @return A Java Future containing the result of the CopySnapshot operation
* returned by the service.
* @sample AmazonElastiCacheAsync.CopySnapshot
*/
java.util.concurrent.Future copySnapshotAsync(
CopySnapshotRequest copySnapshotRequest);
/**
*
* The CopySnapshot action makes a copy of an existing snapshot.
*
*
*
* Users or groups that have permissions to use the CopySnapshot API
* can create their own Amazon S3 buckets and copy snapshots to it. To
* control access to your snapshots, use an IAM policy to control who has
* the ability to use the CopySnapshot API. For more information
* about using IAM to control the use of ElastiCache APIs, see Exporting Snapshots and Authentication & Access Control.
*
*
*
* Erorr Message:
*
*
* -
*
* Error Message: The authenticated user does not have sufficient
* permissions to perform the desired activity.
*
*
* Solution: Contact your system administrator to get the needed
* permissions.
*
*
*
*
* @param copySnapshotRequest
* Represents the input of a CopySnapshotMessage action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopySnapshot operation
* returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CopySnapshot
*/
java.util.concurrent.Future copySnapshotAsync(
CopySnapshotRequest copySnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CreateCacheCluster action creates a cache cluster. All nodes
* in the cache cluster run the same protocol-compliant cache engine
* software, either Memcached or Redis.
*
*
* @param createCacheClusterRequest
* Represents the input of a CreateCacheCluster action.
* @return A Java Future containing the result of the CreateCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsync.CreateCacheCluster
*/
java.util.concurrent.Future createCacheClusterAsync(
CreateCacheClusterRequest createCacheClusterRequest);
/**
*
* The CreateCacheCluster action creates a cache cluster. All nodes
* in the cache cluster run the same protocol-compliant cache engine
* software, either Memcached or Redis.
*
*
* @param createCacheClusterRequest
* Represents the input of a CreateCacheCluster action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CreateCacheCluster
*/
java.util.concurrent.Future createCacheClusterAsync(
CreateCacheClusterRequest createCacheClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CreateCacheParameterGroup action creates a new cache parameter
* group. A cache parameter group is a collection of parameters that you
* apply to all of the nodes in a cache cluster.
*
*
* @param createCacheParameterGroupRequest
* Represents the input of a CreateCacheParameterGroup action.
* @return A Java Future containing the result of the
* CreateCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsync.CreateCacheParameterGroup
*/
java.util.concurrent.Future createCacheParameterGroupAsync(
CreateCacheParameterGroupRequest createCacheParameterGroupRequest);
/**
*
* The CreateCacheParameterGroup action creates a new cache parameter
* group. A cache parameter group is a collection of parameters that you
* apply to all of the nodes in a cache cluster.
*
*
* @param createCacheParameterGroupRequest
* Represents the input of a CreateCacheParameterGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CreateCacheParameterGroup
*/
java.util.concurrent.Future createCacheParameterGroupAsync(
CreateCacheParameterGroupRequest createCacheParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CreateCacheSecurityGroup action creates a new cache security
* group. Use a cache security group to control access to one or more cache
* clusters.
*
*
* Cache security groups are only used when you are creating a cache cluster
* outside of an Amazon Virtual Private Cloud (VPC). If you are creating a
* cache cluster inside of a VPC, use a cache subnet group instead. For more
* information, see CreateCacheSubnetGroup.
*
*
* @param createCacheSecurityGroupRequest
* Represents the input of a CreateCacheSecurityGroup action.
* @return A Java Future containing the result of the
* CreateCacheSecurityGroup operation returned by the service.
* @sample AmazonElastiCacheAsync.CreateCacheSecurityGroup
*/
java.util.concurrent.Future createCacheSecurityGroupAsync(
CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest);
/**
*
* The CreateCacheSecurityGroup action creates a new cache security
* group. Use a cache security group to control access to one or more cache
* clusters.
*
*
* Cache security groups are only used when you are creating a cache cluster
* outside of an Amazon Virtual Private Cloud (VPC). If you are creating a
* cache cluster inside of a VPC, use a cache subnet group instead. For more
* information, see CreateCacheSubnetGroup.
*
*
* @param createCacheSecurityGroupRequest
* Represents the input of a CreateCacheSecurityGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateCacheSecurityGroup operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CreateCacheSecurityGroup
*/
java.util.concurrent.Future createCacheSecurityGroupAsync(
CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CreateCacheSubnetGroup action creates a new cache subnet
* group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon
* Virtual Private Cloud (VPC).
*
*
* @param createCacheSubnetGroupRequest
* Represents the input of a CreateCacheSubnetGroup action.
* @return A Java Future containing the result of the CreateCacheSubnetGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsync.CreateCacheSubnetGroup
*/
java.util.concurrent.Future createCacheSubnetGroupAsync(
CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest);
/**
*
* The CreateCacheSubnetGroup action creates a new cache subnet
* group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon
* Virtual Private Cloud (VPC).
*
*
* @param createCacheSubnetGroupRequest
* Represents the input of a CreateCacheSubnetGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCacheSubnetGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CreateCacheSubnetGroup
*/
java.util.concurrent.Future createCacheSubnetGroupAsync(
CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CreateReplicationGroup action creates a replication group. A
* replication group is a collection of cache clusters, where one of the
* cache clusters is a read/write primary and the others are read-only
* replicas. Writes to the primary are automatically propagated to the
* replicas.
*
*
* When you create a replication group, you must specify an existing cache
* cluster that is in the primary role. When the replication group has been
* successfully created, you can add one or more read replica replicas to
* it, up to a total of five read replicas.
*
*
*
* This action is valid only for Redis.
*
*
*
* @param createReplicationGroupRequest
* Represents the input of a CreateReplicationGroup action.
* @return A Java Future containing the result of the CreateReplicationGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsync.CreateReplicationGroup
*/
java.util.concurrent.Future createReplicationGroupAsync(
CreateReplicationGroupRequest createReplicationGroupRequest);
/**
*
* The CreateReplicationGroup action creates a replication group. A
* replication group is a collection of cache clusters, where one of the
* cache clusters is a read/write primary and the others are read-only
* replicas. Writes to the primary are automatically propagated to the
* replicas.
*
*
* When you create a replication group, you must specify an existing cache
* cluster that is in the primary role. When the replication group has been
* successfully created, you can add one or more read replica replicas to
* it, up to a total of five read replicas.
*
*
*
* This action is valid only for Redis.
*
*
*
* @param createReplicationGroupRequest
* Represents the input of a CreateReplicationGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CreateReplicationGroup
*/
java.util.concurrent.Future createReplicationGroupAsync(
CreateReplicationGroupRequest createReplicationGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The CreateSnapshot action creates a copy of an entire cache
* cluster at a specific moment in time.
*
*
* @param createSnapshotRequest
* Represents the input of a CreateSnapshot action.
* @return A Java Future containing the result of the CreateSnapshot
* operation returned by the service.
* @sample AmazonElastiCacheAsync.CreateSnapshot
*/
java.util.concurrent.Future createSnapshotAsync(
CreateSnapshotRequest createSnapshotRequest);
/**
*
* The CreateSnapshot action creates a copy of an entire cache
* cluster at a specific moment in time.
*
*
* @param createSnapshotRequest
* Represents the input of a CreateSnapshot action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.CreateSnapshot
*/
java.util.concurrent.Future createSnapshotAsync(
CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteCacheCluster action deletes a previously provisioned
* cache cluster. DeleteCacheCluster deletes all associated cache
* nodes, node endpoints and the cache cluster itself. When you receive a
* successful response from this action, Amazon ElastiCache immediately
* begins deleting the cache cluster; you cannot cancel or revert this
* action.
*
*
* This API cannot be used to delete a cache cluster that is the last read
* replica of a replication group that has Multi-AZ mode enabled.
*
*
* @param deleteCacheClusterRequest
* Represents the input of a DeleteCacheCluster action.
* @return A Java Future containing the result of the DeleteCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DeleteCacheCluster
*/
java.util.concurrent.Future deleteCacheClusterAsync(
DeleteCacheClusterRequest deleteCacheClusterRequest);
/**
*
* The DeleteCacheCluster action deletes a previously provisioned
* cache cluster. DeleteCacheCluster deletes all associated cache
* nodes, node endpoints and the cache cluster itself. When you receive a
* successful response from this action, Amazon ElastiCache immediately
* begins deleting the cache cluster; you cannot cancel or revert this
* action.
*
*
* This API cannot be used to delete a cache cluster that is the last read
* replica of a replication group that has Multi-AZ mode enabled.
*
*
* @param deleteCacheClusterRequest
* Represents the input of a DeleteCacheCluster action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DeleteCacheCluster
*/
java.util.concurrent.Future deleteCacheClusterAsync(
DeleteCacheClusterRequest deleteCacheClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteCacheParameterGroup action deletes the specified cache
* parameter group. You cannot delete a cache parameter group if it is
* associated with any cache clusters.
*
*
* @param deleteCacheParameterGroupRequest
* Represents the input of a DeleteCacheParameterGroup action.
* @return A Java Future containing the result of the
* DeleteCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsync.DeleteCacheParameterGroup
*/
java.util.concurrent.Future deleteCacheParameterGroupAsync(
DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest);
/**
*
* The DeleteCacheParameterGroup action deletes the specified cache
* parameter group. You cannot delete a cache parameter group if it is
* associated with any cache clusters.
*
*
* @param deleteCacheParameterGroupRequest
* Represents the input of a DeleteCacheParameterGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DeleteCacheParameterGroup
*/
java.util.concurrent.Future deleteCacheParameterGroupAsync(
DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteCacheSecurityGroup action deletes a cache security
* group.
*
*
*
* You cannot delete a cache security group if it is associated with any
* cache clusters.
*
*
*
* @param deleteCacheSecurityGroupRequest
* Represents the input of a DeleteCacheSecurityGroup action.
* @return A Java Future containing the result of the
* DeleteCacheSecurityGroup operation returned by the service.
* @sample AmazonElastiCacheAsync.DeleteCacheSecurityGroup
*/
java.util.concurrent.Future deleteCacheSecurityGroupAsync(
DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest);
/**
*
* The DeleteCacheSecurityGroup action deletes a cache security
* group.
*
*
*
* You cannot delete a cache security group if it is associated with any
* cache clusters.
*
*
*
* @param deleteCacheSecurityGroupRequest
* Represents the input of a DeleteCacheSecurityGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteCacheSecurityGroup operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DeleteCacheSecurityGroup
*/
java.util.concurrent.Future deleteCacheSecurityGroupAsync(
DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteCacheSubnetGroup action deletes a cache subnet group.
*
*
*
* You cannot delete a cache subnet group if it is associated with any cache
* clusters.
*
*
*
* @param deleteCacheSubnetGroupRequest
* Represents the input of a DeleteCacheSubnetGroup action.
* @return A Java Future containing the result of the DeleteCacheSubnetGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DeleteCacheSubnetGroup
*/
java.util.concurrent.Future deleteCacheSubnetGroupAsync(
DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest);
/**
*
* The DeleteCacheSubnetGroup action deletes a cache subnet group.
*
*
*
* You cannot delete a cache subnet group if it is associated with any cache
* clusters.
*
*
*
* @param deleteCacheSubnetGroupRequest
* Represents the input of a DeleteCacheSubnetGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCacheSubnetGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DeleteCacheSubnetGroup
*/
java.util.concurrent.Future deleteCacheSubnetGroupAsync(
DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteReplicationGroup action deletes an existing replication
* group. By default, this action deletes the entire replication group,
* including the primary cluster and all of the read replicas. You can
* optionally delete only the read replicas, while retaining the primary
* cluster.
*
*
* When you receive a successful response from this action, Amazon
* ElastiCache immediately begins deleting the selected resources; you
* cannot cancel or revert this action.
*
*
* @param deleteReplicationGroupRequest
* Represents the input of a DeleteReplicationGroup action.
* @return A Java Future containing the result of the DeleteReplicationGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DeleteReplicationGroup
*/
java.util.concurrent.Future deleteReplicationGroupAsync(
DeleteReplicationGroupRequest deleteReplicationGroupRequest);
/**
*
* The DeleteReplicationGroup action deletes an existing replication
* group. By default, this action deletes the entire replication group,
* including the primary cluster and all of the read replicas. You can
* optionally delete only the read replicas, while retaining the primary
* cluster.
*
*
* When you receive a successful response from this action, Amazon
* ElastiCache immediately begins deleting the selected resources; you
* cannot cancel or revert this action.
*
*
* @param deleteReplicationGroupRequest
* Represents the input of a DeleteReplicationGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DeleteReplicationGroup
*/
java.util.concurrent.Future deleteReplicationGroupAsync(
DeleteReplicationGroupRequest deleteReplicationGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteSnapshot action deletes an existing snapshot. When you
* receive a successful response from this action, ElastiCache immediately
* begins deleting the snapshot; you cannot cancel or revert this action.
*
*
* @param deleteSnapshotRequest
* Represents the input of a DeleteSnapshot action.
* @return A Java Future containing the result of the DeleteSnapshot
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DeleteSnapshot
*/
java.util.concurrent.Future deleteSnapshotAsync(
DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* The DeleteSnapshot action deletes an existing snapshot. When you
* receive a successful response from this action, ElastiCache immediately
* begins deleting the snapshot; you cannot cancel or revert this action.
*
*
* @param deleteSnapshotRequest
* Represents the input of a DeleteSnapshot action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshot
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DeleteSnapshot
*/
java.util.concurrent.Future deleteSnapshotAsync(
DeleteSnapshotRequest deleteSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeCacheClusters action returns information about all
* provisioned cache clusters if no cache cluster identifier is specified,
* or about a specific cache cluster if a cache cluster identifier is
* supplied.
*
*
* By default, abbreviated information about the cache clusters(s) will be
* returned. You can use the optional ShowDetails flag to retrieve
* detailed information about the cache nodes associated with the cache
* clusters. These details include the DNS address and port for the cache
* node endpoint.
*
*
* If the cluster is in the CREATING state, only cluster level information
* will be displayed until all of the nodes are successfully provisioned.
*
*
* If the cluster is in the DELETING state, only cluster level information
* will be displayed.
*
*
* If cache nodes are currently being added to the cache cluster, node
* endpoint information and creation time for the additional nodes will not
* be displayed until they are completely provisioned. When the cache
* cluster state is available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cache cluster, no
* endpoint information for the removed nodes is displayed.
*
*
* @param describeCacheClustersRequest
* Represents the input of a DescribeCacheClusters action.
* @return A Java Future containing the result of the DescribeCacheClusters
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeCacheClusters
*/
java.util.concurrent.Future describeCacheClustersAsync(
DescribeCacheClustersRequest describeCacheClustersRequest);
/**
*
* The DescribeCacheClusters action returns information about all
* provisioned cache clusters if no cache cluster identifier is specified,
* or about a specific cache cluster if a cache cluster identifier is
* supplied.
*
*
* By default, abbreviated information about the cache clusters(s) will be
* returned. You can use the optional ShowDetails flag to retrieve
* detailed information about the cache nodes associated with the cache
* clusters. These details include the DNS address and port for the cache
* node endpoint.
*
*
* If the cluster is in the CREATING state, only cluster level information
* will be displayed until all of the nodes are successfully provisioned.
*
*
* If the cluster is in the DELETING state, only cluster level information
* will be displayed.
*
*
* If cache nodes are currently being added to the cache cluster, node
* endpoint information and creation time for the additional nodes will not
* be displayed until they are completely provisioned. When the cache
* cluster state is available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cache cluster, no
* endpoint information for the removed nodes is displayed.
*
*
* @param describeCacheClustersRequest
* Represents the input of a DescribeCacheClusters action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCacheClusters
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeCacheClusters
*/
java.util.concurrent.Future describeCacheClustersAsync(
DescribeCacheClustersRequest describeCacheClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCacheClusters operation.
*
* @see #describeCacheClustersAsync(DescribeCacheClustersRequest)
*/
java.util.concurrent.Future describeCacheClustersAsync();
/**
* Simplified method form for invoking the DescribeCacheClusters operation
* with an AsyncHandler.
*
* @see #describeCacheClustersAsync(DescribeCacheClustersRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCacheClustersAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeCacheEngineVersions action returns a list of the
* available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest
* Represents the input of a DescribeCacheEngineVersions
* action.
* @return A Java Future containing the result of the
* DescribeCacheEngineVersions operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeCacheEngineVersions
*/
java.util.concurrent.Future describeCacheEngineVersionsAsync(
DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest);
/**
*
* The DescribeCacheEngineVersions action returns a list of the
* available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest
* Represents the input of a DescribeCacheEngineVersions
* action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeCacheEngineVersions operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeCacheEngineVersions
*/
java.util.concurrent.Future describeCacheEngineVersionsAsync(
DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCacheEngineVersions
* operation.
*
* @see #describeCacheEngineVersionsAsync(DescribeCacheEngineVersionsRequest)
*/
java.util.concurrent.Future describeCacheEngineVersionsAsync();
/**
* Simplified method form for invoking the DescribeCacheEngineVersions
* operation with an AsyncHandler.
*
* @see #describeCacheEngineVersionsAsync(DescribeCacheEngineVersionsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCacheEngineVersionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeCacheParameterGroups action returns a list of cache
* parameter group descriptions. If a cache parameter group name is
* specified, the list will contain only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest
* Represents the input of a DescribeCacheParameterGroups
* action.
* @return A Java Future containing the result of the
* DescribeCacheParameterGroups operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeCacheParameterGroups
*/
java.util.concurrent.Future describeCacheParameterGroupsAsync(
DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest);
/**
*
* The DescribeCacheParameterGroups action returns a list of cache
* parameter group descriptions. If a cache parameter group name is
* specified, the list will contain only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest
* Represents the input of a DescribeCacheParameterGroups
* action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeCacheParameterGroups operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeCacheParameterGroups
*/
java.util.concurrent.Future describeCacheParameterGroupsAsync(
DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCacheParameterGroups
* operation.
*
* @see #describeCacheParameterGroupsAsync(DescribeCacheParameterGroupsRequest)
*/
java.util.concurrent.Future describeCacheParameterGroupsAsync();
/**
* Simplified method form for invoking the DescribeCacheParameterGroups
* operation with an AsyncHandler.
*
* @see #describeCacheParameterGroupsAsync(DescribeCacheParameterGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCacheParameterGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeCacheParameters action returns the detailed parameter
* list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest
* Represents the input of a DescribeCacheParameters action.
* @return A Java Future containing the result of the
* DescribeCacheParameters operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeCacheParameters
*/
java.util.concurrent.Future describeCacheParametersAsync(
DescribeCacheParametersRequest describeCacheParametersRequest);
/**
*
* The DescribeCacheParameters action returns the detailed parameter
* list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest
* Represents the input of a DescribeCacheParameters action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeCacheParameters operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeCacheParameters
*/
java.util.concurrent.Future describeCacheParametersAsync(
DescribeCacheParametersRequest describeCacheParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeCacheSecurityGroups action returns a list of cache
* security group descriptions. If a cache security group name is specified,
* the list will contain only the description of that group.
*
*
* @param describeCacheSecurityGroupsRequest
* Represents the input of a DescribeCacheSecurityGroups
* action.
* @return A Java Future containing the result of the
* DescribeCacheSecurityGroups operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeCacheSecurityGroups
*/
java.util.concurrent.Future describeCacheSecurityGroupsAsync(
DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest);
/**
*
* The DescribeCacheSecurityGroups action returns a list of cache
* security group descriptions. If a cache security group name is specified,
* the list will contain only the description of that group.
*
*
* @param describeCacheSecurityGroupsRequest
* Represents the input of a DescribeCacheSecurityGroups
* action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeCacheSecurityGroups operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeCacheSecurityGroups
*/
java.util.concurrent.Future describeCacheSecurityGroupsAsync(
DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCacheSecurityGroups
* operation.
*
* @see #describeCacheSecurityGroupsAsync(DescribeCacheSecurityGroupsRequest)
*/
java.util.concurrent.Future describeCacheSecurityGroupsAsync();
/**
* Simplified method form for invoking the DescribeCacheSecurityGroups
* operation with an AsyncHandler.
*
* @see #describeCacheSecurityGroupsAsync(DescribeCacheSecurityGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCacheSecurityGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeCacheSubnetGroups action returns a list of cache
* subnet group descriptions. If a subnet group name is specified, the list
* will contain only the description of that group.
*
*
* @param describeCacheSubnetGroupsRequest
* Represents the input of a DescribeCacheSubnetGroups action.
* @return A Java Future containing the result of the
* DescribeCacheSubnetGroups operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeCacheSubnetGroups
*/
java.util.concurrent.Future describeCacheSubnetGroupsAsync(
DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest);
/**
*
* The DescribeCacheSubnetGroups action returns a list of cache
* subnet group descriptions. If a subnet group name is specified, the list
* will contain only the description of that group.
*
*
* @param describeCacheSubnetGroupsRequest
* Represents the input of a DescribeCacheSubnetGroups action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeCacheSubnetGroups operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeCacheSubnetGroups
*/
java.util.concurrent.Future describeCacheSubnetGroupsAsync(
DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCacheSubnetGroups
* operation.
*
* @see #describeCacheSubnetGroupsAsync(DescribeCacheSubnetGroupsRequest)
*/
java.util.concurrent.Future describeCacheSubnetGroupsAsync();
/**
* Simplified method form for invoking the DescribeCacheSubnetGroups
* operation with an AsyncHandler.
*
* @see #describeCacheSubnetGroupsAsync(DescribeCacheSubnetGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCacheSubnetGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeEngineDefaultParameters action returns the default
* engine and system parameter information for the specified cache engine.
*
*
* @param describeEngineDefaultParametersRequest
* Represents the input of a DescribeEngineDefaultParameters
* action.
* @return A Java Future containing the result of the
* DescribeEngineDefaultParameters operation returned by the
* service.
* @sample AmazonElastiCacheAsync.DescribeEngineDefaultParameters
*/
java.util.concurrent.Future describeEngineDefaultParametersAsync(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest);
/**
*
* The DescribeEngineDefaultParameters action returns the default
* engine and system parameter information for the specified cache engine.
*
*
* @param describeEngineDefaultParametersRequest
* Represents the input of a DescribeEngineDefaultParameters
* action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeEngineDefaultParameters operation returned by the
* service.
* @sample AmazonElastiCacheAsyncHandler.DescribeEngineDefaultParameters
*/
java.util.concurrent.Future describeEngineDefaultParametersAsync(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeEvents action returns events related to cache
* clusters, cache security groups, and cache parameter groups. You can
* obtain events specific to a particular cache cluster, cache security
* group, or cache parameter group by providing the name as a parameter.
*
*
* By default, only the events occurring within the last hour are returned;
* however, you can retrieve up to 14 days' worth of events if necessary.
*
*
* @param describeEventsRequest
* Represents the input of a DescribeEvents action.
* @return A Java Future containing the result of the DescribeEvents
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeEvents
*/
java.util.concurrent.Future describeEventsAsync(
DescribeEventsRequest describeEventsRequest);
/**
*
* The DescribeEvents action returns events related to cache
* clusters, cache security groups, and cache parameter groups. You can
* obtain events specific to a particular cache cluster, cache security
* group, or cache parameter group by providing the name as a parameter.
*
*
* By default, only the events occurring within the last hour are returned;
* however, you can retrieve up to 14 days' worth of events if necessary.
*
*
* @param describeEventsRequest
* Represents the input of a DescribeEvents action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeEvents
*/
java.util.concurrent.Future describeEventsAsync(
DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEvents operation.
*
* @see #describeEventsAsync(DescribeEventsRequest)
*/
java.util.concurrent.Future describeEventsAsync();
/**
* Simplified method form for invoking the DescribeEvents operation with an
* AsyncHandler.
*
* @see #describeEventsAsync(DescribeEventsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeReplicationGroups action returns information about a
* particular replication group. If no identifier is specified,
* DescribeReplicationGroups returns information about all
* replication groups.
*
*
* @param describeReplicationGroupsRequest
* Represents the input of a DescribeReplicationGroups action.
* @return A Java Future containing the result of the
* DescribeReplicationGroups operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeReplicationGroups
*/
java.util.concurrent.Future describeReplicationGroupsAsync(
DescribeReplicationGroupsRequest describeReplicationGroupsRequest);
/**
*
* The DescribeReplicationGroups action returns information about a
* particular replication group. If no identifier is specified,
* DescribeReplicationGroups returns information about all
* replication groups.
*
*
* @param describeReplicationGroupsRequest
* Represents the input of a DescribeReplicationGroups action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeReplicationGroups operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeReplicationGroups
*/
java.util.concurrent.Future describeReplicationGroupsAsync(
DescribeReplicationGroupsRequest describeReplicationGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeReplicationGroups
* operation.
*
* @see #describeReplicationGroupsAsync(DescribeReplicationGroupsRequest)
*/
java.util.concurrent.Future describeReplicationGroupsAsync();
/**
* Simplified method form for invoking the DescribeReplicationGroups
* operation with an AsyncHandler.
*
* @see #describeReplicationGroupsAsync(DescribeReplicationGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeReplicationGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeReservedCacheNodes action returns information about
* reserved cache nodes for this account, or about a specified reserved
* cache node.
*
*
* @param describeReservedCacheNodesRequest
* Represents the input of a DescribeReservedCacheNodes
* action.
* @return A Java Future containing the result of the
* DescribeReservedCacheNodes operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeReservedCacheNodes
*/
java.util.concurrent.Future describeReservedCacheNodesAsync(
DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest);
/**
*
* The DescribeReservedCacheNodes action returns information about
* reserved cache nodes for this account, or about a specified reserved
* cache node.
*
*
* @param describeReservedCacheNodesRequest
* Represents the input of a DescribeReservedCacheNodes
* action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeReservedCacheNodes operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeReservedCacheNodes
*/
java.util.concurrent.Future describeReservedCacheNodesAsync(
DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeReservedCacheNodes
* operation.
*
* @see #describeReservedCacheNodesAsync(DescribeReservedCacheNodesRequest)
*/
java.util.concurrent.Future describeReservedCacheNodesAsync();
/**
* Simplified method form for invoking the DescribeReservedCacheNodes
* operation with an AsyncHandler.
*
* @see #describeReservedCacheNodesAsync(DescribeReservedCacheNodesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeReservedCacheNodesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeReservedCacheNodesOfferings action lists available
* reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest
* Represents the input of a
* DescribeReservedCacheNodesOfferings action.
* @return A Java Future containing the result of the
* DescribeReservedCacheNodesOfferings operation returned by the
* service.
* @sample AmazonElastiCacheAsync.DescribeReservedCacheNodesOfferings
*/
java.util.concurrent.Future describeReservedCacheNodesOfferingsAsync(
DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest);
/**
*
* The DescribeReservedCacheNodesOfferings action lists available
* reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest
* Represents the input of a
* DescribeReservedCacheNodesOfferings action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeReservedCacheNodesOfferings operation returned by the
* service.
* @sample AmazonElastiCacheAsyncHandler.DescribeReservedCacheNodesOfferings
*/
java.util.concurrent.Future describeReservedCacheNodesOfferingsAsync(
DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the
* DescribeReservedCacheNodesOfferings operation.
*
* @see #describeReservedCacheNodesOfferingsAsync(DescribeReservedCacheNodesOfferingsRequest)
*/
java.util.concurrent.Future describeReservedCacheNodesOfferingsAsync();
/**
* Simplified method form for invoking the
* DescribeReservedCacheNodesOfferings operation with an AsyncHandler.
*
* @see #describeReservedCacheNodesOfferingsAsync(DescribeReservedCacheNodesOfferingsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeReservedCacheNodesOfferingsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DescribeSnapshots action returns information about cache
* cluster snapshots. By default, DescribeSnapshots lists all of your
* snapshots; it can optionally describe a single snapshot, or just the
* snapshots associated with a particular cache cluster.
*
*
* @param describeSnapshotsRequest
* Represents the input of a DescribeSnapshotsMessage action.
* @return A Java Future containing the result of the DescribeSnapshots
* operation returned by the service.
* @sample AmazonElastiCacheAsync.DescribeSnapshots
*/
java.util.concurrent.Future describeSnapshotsAsync(
DescribeSnapshotsRequest describeSnapshotsRequest);
/**
*
* The DescribeSnapshots action returns information about cache
* cluster snapshots. By default, DescribeSnapshots lists all of your
* snapshots; it can optionally describe a single snapshot, or just the
* snapshots associated with a particular cache cluster.
*
*
* @param describeSnapshotsRequest
* Represents the input of a DescribeSnapshotsMessage action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSnapshots
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.DescribeSnapshots
*/
java.util.concurrent.Future describeSnapshotsAsync(
DescribeSnapshotsRequest describeSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeSnapshots operation.
*
* @see #describeSnapshotsAsync(DescribeSnapshotsRequest)
*/
java.util.concurrent.Future describeSnapshotsAsync();
/**
* Simplified method form for invoking the DescribeSnapshots operation with
* an AsyncHandler.
*
* @see #describeSnapshotsAsync(DescribeSnapshotsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeSnapshotsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ListAllowedNodeTypeModifications
action lists all
* available node types that you can scale your Redis cluster's or
* replication group's current node type up to.
*
*
* When you use the ModifyCacheCluster
or
* ModifyReplicationGroup
APIs to scale up your cluster or
* replication group, the value of the CacheNodeType parameter must
* be one of the node types returned by this action.
*
*
* @param listAllowedNodeTypeModificationsRequest
* The input parameters for the
* ListAllowedNodeTypeModifications action.
* @return A Java Future containing the result of the
* ListAllowedNodeTypeModifications operation returned by the
* service.
* @sample AmazonElastiCacheAsync.ListAllowedNodeTypeModifications
*/
java.util.concurrent.Future listAllowedNodeTypeModificationsAsync(
ListAllowedNodeTypeModificationsRequest listAllowedNodeTypeModificationsRequest);
/**
*
* The ListAllowedNodeTypeModifications
action lists all
* available node types that you can scale your Redis cluster's or
* replication group's current node type up to.
*
*
* When you use the ModifyCacheCluster
or
* ModifyReplicationGroup
APIs to scale up your cluster or
* replication group, the value of the CacheNodeType parameter must
* be one of the node types returned by this action.
*
*
* @param listAllowedNodeTypeModificationsRequest
* The input parameters for the
* ListAllowedNodeTypeModifications action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListAllowedNodeTypeModifications operation returned by the
* service.
* @sample AmazonElastiCacheAsyncHandler.ListAllowedNodeTypeModifications
*/
java.util.concurrent.Future listAllowedNodeTypeModificationsAsync(
ListAllowedNodeTypeModificationsRequest listAllowedNodeTypeModificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListAllowedNodeTypeModifications
* operation.
*
* @see #listAllowedNodeTypeModificationsAsync(ListAllowedNodeTypeModificationsRequest)
*/
java.util.concurrent.Future listAllowedNodeTypeModificationsAsync();
/**
* Simplified method form for invoking the ListAllowedNodeTypeModifications
* operation with an AsyncHandler.
*
* @see #listAllowedNodeTypeModificationsAsync(ListAllowedNodeTypeModificationsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listAllowedNodeTypeModificationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ListTagsForResource action lists all cost allocation tags
* currently on the named resource. A cost allocation tag is a
* key-value pair where the key is case-sensitive and the value is optional.
* Cost allocation tags can be used to categorize and track your AWS costs.
*
*
* You can have a maximum of 10 cost allocation tags on an ElastiCache
* resource. For more information, see Using Cost Allocation Tags in Amazon ElastiCache.
*
*
* @param listTagsForResourceRequest
* The input parameters for the ListTagsForResource action.
* @return A Java Future containing the result of the ListTagsForResource
* operation returned by the service.
* @sample AmazonElastiCacheAsync.ListTagsForResource
*/
java.util.concurrent.Future listTagsForResourceAsync(
ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* The ListTagsForResource action lists all cost allocation tags
* currently on the named resource. A cost allocation tag is a
* key-value pair where the key is case-sensitive and the value is optional.
* Cost allocation tags can be used to categorize and track your AWS costs.
*
*
* You can have a maximum of 10 cost allocation tags on an ElastiCache
* resource. For more information, see Using Cost Allocation Tags in Amazon ElastiCache.
*
*
* @param listTagsForResourceRequest
* The input parameters for the ListTagsForResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.ListTagsForResource
*/
java.util.concurrent.Future listTagsForResourceAsync(
ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ModifyCacheCluster action modifies the settings for a cache
* cluster. You can use this action to change one or more cluster
* configuration parameters by specifying the parameters and the new values.
*
*
* @param modifyCacheClusterRequest
* Represents the input of a ModifyCacheCluster action.
* @return A Java Future containing the result of the ModifyCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsync.ModifyCacheCluster
*/
java.util.concurrent.Future modifyCacheClusterAsync(
ModifyCacheClusterRequest modifyCacheClusterRequest);
/**
*
* The ModifyCacheCluster action modifies the settings for a cache
* cluster. You can use this action to change one or more cluster
* configuration parameters by specifying the parameters and the new values.
*
*
* @param modifyCacheClusterRequest
* Represents the input of a ModifyCacheCluster action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.ModifyCacheCluster
*/
java.util.concurrent.Future modifyCacheClusterAsync(
ModifyCacheClusterRequest modifyCacheClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ModifyCacheParameterGroup action modifies the parameters of a
* cache parameter group. You can modify up to 20 parameters in a single
* request by submitting a list parameter name and value pairs.
*
*
* @param modifyCacheParameterGroupRequest
* Represents the input of a ModifyCacheParameterGroup action.
* @return A Java Future containing the result of the
* ModifyCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsync.ModifyCacheParameterGroup
*/
java.util.concurrent.Future modifyCacheParameterGroupAsync(
ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest);
/**
*
* The ModifyCacheParameterGroup action modifies the parameters of a
* cache parameter group. You can modify up to 20 parameters in a single
* request by submitting a list parameter name and value pairs.
*
*
* @param modifyCacheParameterGroupRequest
* Represents the input of a ModifyCacheParameterGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ModifyCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.ModifyCacheParameterGroup
*/
java.util.concurrent.Future modifyCacheParameterGroupAsync(
ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ModifyCacheSubnetGroup action modifies an existing cache
* subnet group.
*
*
* @param modifyCacheSubnetGroupRequest
* Represents the input of a ModifyCacheSubnetGroup action.
* @return A Java Future containing the result of the ModifyCacheSubnetGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsync.ModifyCacheSubnetGroup
*/
java.util.concurrent.Future modifyCacheSubnetGroupAsync(
ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest);
/**
*
* The ModifyCacheSubnetGroup action modifies an existing cache
* subnet group.
*
*
* @param modifyCacheSubnetGroupRequest
* Represents the input of a ModifyCacheSubnetGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyCacheSubnetGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.ModifyCacheSubnetGroup
*/
java.util.concurrent.Future modifyCacheSubnetGroupAsync(
ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ModifyReplicationGroup action modifies the settings for a
* replication group.
*
*
* @param modifyReplicationGroupRequest
* Represents the input of a ModifyReplicationGroups action.
* @return A Java Future containing the result of the ModifyReplicationGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsync.ModifyReplicationGroup
*/
java.util.concurrent.Future modifyReplicationGroupAsync(
ModifyReplicationGroupRequest modifyReplicationGroupRequest);
/**
*
* The ModifyReplicationGroup action modifies the settings for a
* replication group.
*
*
* @param modifyReplicationGroupRequest
* Represents the input of a ModifyReplicationGroups action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationGroup
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.ModifyReplicationGroup
*/
java.util.concurrent.Future modifyReplicationGroupAsync(
ModifyReplicationGroupRequest modifyReplicationGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The PurchaseReservedCacheNodesOffering action allows you to
* purchase a reserved cache node offering.
*
*
* @param purchaseReservedCacheNodesOfferingRequest
* Represents the input of a
* PurchaseReservedCacheNodesOffering action.
* @return A Java Future containing the result of the
* PurchaseReservedCacheNodesOffering operation returned by the
* service.
* @sample AmazonElastiCacheAsync.PurchaseReservedCacheNodesOffering
*/
java.util.concurrent.Future purchaseReservedCacheNodesOfferingAsync(
PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest);
/**
*
* The PurchaseReservedCacheNodesOffering action allows you to
* purchase a reserved cache node offering.
*
*
* @param purchaseReservedCacheNodesOfferingRequest
* Represents the input of a
* PurchaseReservedCacheNodesOffering action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* PurchaseReservedCacheNodesOffering operation returned by the
* service.
* @sample AmazonElastiCacheAsyncHandler.PurchaseReservedCacheNodesOffering
*/
java.util.concurrent.Future purchaseReservedCacheNodesOfferingAsync(
PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The RebootCacheCluster action reboots some, or all, of the cache
* nodes within a provisioned cache cluster. This API will apply any
* modified cache parameter groups to the cache cluster. The reboot action
* takes place as soon as possible, and results in a momentary outage to the
* cache cluster. During the reboot, the cache cluster status is set to
* REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node being
* rebooted) to be lost.
*
*
* When the reboot is complete, a cache cluster event is created.
*
*
* @param rebootCacheClusterRequest
* Represents the input of a RebootCacheCluster action.
* @return A Java Future containing the result of the RebootCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsync.RebootCacheCluster
*/
java.util.concurrent.Future rebootCacheClusterAsync(
RebootCacheClusterRequest rebootCacheClusterRequest);
/**
*
* The RebootCacheCluster action reboots some, or all, of the cache
* nodes within a provisioned cache cluster. This API will apply any
* modified cache parameter groups to the cache cluster. The reboot action
* takes place as soon as possible, and results in a momentary outage to the
* cache cluster. During the reboot, the cache cluster status is set to
* REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node being
* rebooted) to be lost.
*
*
* When the reboot is complete, a cache cluster event is created.
*
*
* @param rebootCacheClusterRequest
* Represents the input of a RebootCacheCluster action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebootCacheCluster
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.RebootCacheCluster
*/
java.util.concurrent.Future rebootCacheClusterAsync(
RebootCacheClusterRequest rebootCacheClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The RemoveTagsFromResource action removes the tags identified by
* the TagKeys
list from the named resource.
*
*
* @param removeTagsFromResourceRequest
* Represents the input of a RemoveTagsFromResource action.
* @return A Java Future containing the result of the RemoveTagsFromResource
* operation returned by the service.
* @sample AmazonElastiCacheAsync.RemoveTagsFromResource
*/
java.util.concurrent.Future removeTagsFromResourceAsync(
RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* The RemoveTagsFromResource action removes the tags identified by
* the TagKeys
list from the named resource.
*
*
* @param removeTagsFromResourceRequest
* Represents the input of a RemoveTagsFromResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromResource
* operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.RemoveTagsFromResource
*/
java.util.concurrent.Future removeTagsFromResourceAsync(
RemoveTagsFromResourceRequest removeTagsFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ResetCacheParameterGroup action modifies the parameters of a
* cache parameter group to the engine or system default value. You can
* reset specific parameters by submitting a list of parameter names. To
* reset the entire cache parameter group, specify the
* ResetAllParameters and CacheParameterGroupName parameters.
*
*
* @param resetCacheParameterGroupRequest
* Represents the input of a ResetCacheParameterGroup action.
* @return A Java Future containing the result of the
* ResetCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsync.ResetCacheParameterGroup
*/
java.util.concurrent.Future resetCacheParameterGroupAsync(
ResetCacheParameterGroupRequest resetCacheParameterGroupRequest);
/**
*
* The ResetCacheParameterGroup action modifies the parameters of a
* cache parameter group to the engine or system default value. You can
* reset specific parameters by submitting a list of parameter names. To
* reset the entire cache parameter group, specify the
* ResetAllParameters and CacheParameterGroupName parameters.
*
*
* @param resetCacheParameterGroupRequest
* Represents the input of a ResetCacheParameterGroup action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ResetCacheParameterGroup operation returned by the service.
* @sample AmazonElastiCacheAsyncHandler.ResetCacheParameterGroup
*/
java.util.concurrent.Future resetCacheParameterGroupAsync(
ResetCacheParameterGroupRequest resetCacheParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The RevokeCacheSecurityGroupIngress action revokes ingress from a
* cache security group. Use this action to disallow access from an Amazon
* EC2 security group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest
* Represents the input of a RevokeCacheSecurityGroupIngress
* action.
* @return A Java Future containing the result of the
* RevokeCacheSecurityGroupIngress operation returned by the
* service.
* @sample AmazonElastiCacheAsync.RevokeCacheSecurityGroupIngress
*/
java.util.concurrent.Future revokeCacheSecurityGroupIngressAsync(
RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest);
/**
*
* The RevokeCacheSecurityGroupIngress action revokes ingress from a
* cache security group. Use this action to disallow access from an Amazon
* EC2 security group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest
* Represents the input of a RevokeCacheSecurityGroupIngress
* action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RevokeCacheSecurityGroupIngress operation returned by the
* service.
* @sample AmazonElastiCacheAsyncHandler.RevokeCacheSecurityGroupIngress
*/
java.util.concurrent.Future revokeCacheSecurityGroupIngressAsync(
RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}