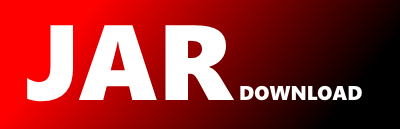
com.amazonaws.services.elasticache.model.ConfigureShard Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Node group (shard) configuration options when adding or removing replicas. Each node group (shard) configuration has
* the following members: NodeGroupId, NewReplicaCount, and PreferredAvailabilityZones.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ConfigureShard implements Serializable, Cloneable {
/**
*
* The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication groups,
* the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's (shard's) id, see Finding a Shard's Id.
*
*/
private String nodeGroupId;
/**
*
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group you are
* working with.
*
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary node
* fails)
*
*
*
*/
private Integer newReplicaCount;
/**
*
* A list of PreferredAvailabilityZone
strings that specify which availability zones the replication
* group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must equal the value of
* NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for each of
* the replicas.
*
*/
private com.amazonaws.internal.SdkInternalList preferredAvailabilityZones;
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*/
private com.amazonaws.internal.SdkInternalList preferredOutpostArns;
/**
*
* The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication groups,
* the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's (shard's) id, see Finding a Shard's Id.
*
*
* @param nodeGroupId
* The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication
* groups, the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's
* (shard's) id, see Finding a Shard's
* Id.
*/
public void setNodeGroupId(String nodeGroupId) {
this.nodeGroupId = nodeGroupId;
}
/**
*
* The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication groups,
* the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's (shard's) id, see Finding a Shard's Id.
*
*
* @return The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication
* groups, the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's
* (shard's) id, see Finding a Shard's
* Id.
*/
public String getNodeGroupId() {
return this.nodeGroupId;
}
/**
*
* The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication groups,
* the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's (shard's) id, see Finding a Shard's Id.
*
*
* @param nodeGroupId
* The 4-digit id for the node group you are configuring. For Redis OSS (cluster mode disabled) replication
* groups, the node group id is always 0001. To find a Redis OSS (cluster mode enabled)'s node group's
* (shard's) id, see Finding a Shard's
* Id.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigureShard withNodeGroupId(String nodeGroupId) {
setNodeGroupId(nodeGroupId);
return this;
}
/**
*
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group you are
* working with.
*
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary node
* fails)
*
*
*
*
* @param newReplicaCount
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group
* you are working with.
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary
* node fails)
*
*
*/
public void setNewReplicaCount(Integer newReplicaCount) {
this.newReplicaCount = newReplicaCount;
}
/**
*
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group you are
* working with.
*
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary node
* fails)
*
*
*
*
* @return The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group
* you are working with.
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary
* node fails)
*
*
*/
public Integer getNewReplicaCount() {
return this.newReplicaCount;
}
/**
*
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group you are
* working with.
*
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary node
* fails)
*
*
*
*
* @param newReplicaCount
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Redis OSS replication group
* you are working with.
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your primary
* node fails)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigureShard withNewReplicaCount(Integer newReplicaCount) {
setNewReplicaCount(newReplicaCount);
return this;
}
/**
*
* A list of PreferredAvailabilityZone
strings that specify which availability zones the replication
* group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must equal the value of
* NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for each of
* the replicas.
*
*
* @return A list of PreferredAvailabilityZone
strings that specify which availability zones the
* replication group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must
* equal the value of NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for
* each of the replicas.
*/
public java.util.List getPreferredAvailabilityZones() {
if (preferredAvailabilityZones == null) {
preferredAvailabilityZones = new com.amazonaws.internal.SdkInternalList();
}
return preferredAvailabilityZones;
}
/**
*
* A list of PreferredAvailabilityZone
strings that specify which availability zones the replication
* group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must equal the value of
* NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for each of
* the replicas.
*
*
* @param preferredAvailabilityZones
* A list of PreferredAvailabilityZone
strings that specify which availability zones the
* replication group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must
* equal the value of NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for
* each of the replicas.
*/
public void setPreferredAvailabilityZones(java.util.Collection preferredAvailabilityZones) {
if (preferredAvailabilityZones == null) {
this.preferredAvailabilityZones = null;
return;
}
this.preferredAvailabilityZones = new com.amazonaws.internal.SdkInternalList(preferredAvailabilityZones);
}
/**
*
* A list of PreferredAvailabilityZone
strings that specify which availability zones the replication
* group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must equal the value of
* NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for each of
* the replicas.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPreferredAvailabilityZones(java.util.Collection)} or
* {@link #withPreferredAvailabilityZones(java.util.Collection)} if you want to override the existing values.
*
*
* @param preferredAvailabilityZones
* A list of PreferredAvailabilityZone
strings that specify which availability zones the
* replication group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must
* equal the value of NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for
* each of the replicas.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigureShard withPreferredAvailabilityZones(String... preferredAvailabilityZones) {
if (this.preferredAvailabilityZones == null) {
setPreferredAvailabilityZones(new com.amazonaws.internal.SdkInternalList(preferredAvailabilityZones.length));
}
for (String ele : preferredAvailabilityZones) {
this.preferredAvailabilityZones.add(ele);
}
return this;
}
/**
*
* A list of PreferredAvailabilityZone
strings that specify which availability zones the replication
* group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must equal the value of
* NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for each of
* the replicas.
*
*
* @param preferredAvailabilityZones
* A list of PreferredAvailabilityZone
strings that specify which availability zones the
* replication group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must
* equal the value of NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache (Redis OSS) selects the availability zone for
* each of the replicas.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigureShard withPreferredAvailabilityZones(java.util.Collection preferredAvailabilityZones) {
setPreferredAvailabilityZones(preferredAvailabilityZones);
return this;
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* @return The outpost ARNs in which the cache cluster is created.
*/
public java.util.List getPreferredOutpostArns() {
if (preferredOutpostArns == null) {
preferredOutpostArns = new com.amazonaws.internal.SdkInternalList();
}
return preferredOutpostArns;
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* @param preferredOutpostArns
* The outpost ARNs in which the cache cluster is created.
*/
public void setPreferredOutpostArns(java.util.Collection preferredOutpostArns) {
if (preferredOutpostArns == null) {
this.preferredOutpostArns = null;
return;
}
this.preferredOutpostArns = new com.amazonaws.internal.SdkInternalList(preferredOutpostArns);
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPreferredOutpostArns(java.util.Collection)} or {@link #withPreferredOutpostArns(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param preferredOutpostArns
* The outpost ARNs in which the cache cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigureShard withPreferredOutpostArns(String... preferredOutpostArns) {
if (this.preferredOutpostArns == null) {
setPreferredOutpostArns(new com.amazonaws.internal.SdkInternalList(preferredOutpostArns.length));
}
for (String ele : preferredOutpostArns) {
this.preferredOutpostArns.add(ele);
}
return this;
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* @param preferredOutpostArns
* The outpost ARNs in which the cache cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigureShard withPreferredOutpostArns(java.util.Collection preferredOutpostArns) {
setPreferredOutpostArns(preferredOutpostArns);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNodeGroupId() != null)
sb.append("NodeGroupId: ").append(getNodeGroupId()).append(",");
if (getNewReplicaCount() != null)
sb.append("NewReplicaCount: ").append(getNewReplicaCount()).append(",");
if (getPreferredAvailabilityZones() != null)
sb.append("PreferredAvailabilityZones: ").append(getPreferredAvailabilityZones()).append(",");
if (getPreferredOutpostArns() != null)
sb.append("PreferredOutpostArns: ").append(getPreferredOutpostArns());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ConfigureShard == false)
return false;
ConfigureShard other = (ConfigureShard) obj;
if (other.getNodeGroupId() == null ^ this.getNodeGroupId() == null)
return false;
if (other.getNodeGroupId() != null && other.getNodeGroupId().equals(this.getNodeGroupId()) == false)
return false;
if (other.getNewReplicaCount() == null ^ this.getNewReplicaCount() == null)
return false;
if (other.getNewReplicaCount() != null && other.getNewReplicaCount().equals(this.getNewReplicaCount()) == false)
return false;
if (other.getPreferredAvailabilityZones() == null ^ this.getPreferredAvailabilityZones() == null)
return false;
if (other.getPreferredAvailabilityZones() != null && other.getPreferredAvailabilityZones().equals(this.getPreferredAvailabilityZones()) == false)
return false;
if (other.getPreferredOutpostArns() == null ^ this.getPreferredOutpostArns() == null)
return false;
if (other.getPreferredOutpostArns() != null && other.getPreferredOutpostArns().equals(this.getPreferredOutpostArns()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNodeGroupId() == null) ? 0 : getNodeGroupId().hashCode());
hashCode = prime * hashCode + ((getNewReplicaCount() == null) ? 0 : getNewReplicaCount().hashCode());
hashCode = prime * hashCode + ((getPreferredAvailabilityZones() == null) ? 0 : getPreferredAvailabilityZones().hashCode());
hashCode = prime * hashCode + ((getPreferredOutpostArns() == null) ? 0 : getPreferredOutpostArns().hashCode());
return hashCode;
}
@Override
public ConfigureShard clone() {
try {
return (ConfigureShard) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}