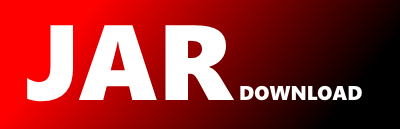
com.amazonaws.services.elasticache.model.ModifyReplicationGroupShardConfigurationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input for a ModifyReplicationGroupShardConfiguration
operation.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyReplicationGroupShardConfigurationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*
*/
private String replicationGroupId;
/**
*
* The number of node groups (shards) that results from the modification of the shard configuration.
*
*/
private Integer nodeGroupCount;
/**
*
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value for
* this parameter is true
.
*
*
* Value: true
*
*/
private Boolean applyImmediately;
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*/
private com.amazonaws.internal.SdkInternalList reshardingConfiguration;
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRemove
* is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
from the
* cluster.
*
*/
private com.amazonaws.internal.SdkInternalList nodeGroupsToRemove;
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRetain
* is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*/
private com.amazonaws.internal.SdkInternalList nodeGroupsToRetain;
/**
*
* The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*
*
* @param replicationGroupId
* The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*/
public void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
/**
*
* The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*
*
* @return The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to
* be configured.
*/
public String getReplicationGroupId() {
return this.replicationGroupId;
}
/**
*
* The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*
*
* @param replicationGroupId
* The name of the Redis OSS (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withReplicationGroupId(String replicationGroupId) {
setReplicationGroupId(replicationGroupId);
return this;
}
/**
*
* The number of node groups (shards) that results from the modification of the shard configuration.
*
*
* @param nodeGroupCount
* The number of node groups (shards) that results from the modification of the shard configuration.
*/
public void setNodeGroupCount(Integer nodeGroupCount) {
this.nodeGroupCount = nodeGroupCount;
}
/**
*
* The number of node groups (shards) that results from the modification of the shard configuration.
*
*
* @return The number of node groups (shards) that results from the modification of the shard configuration.
*/
public Integer getNodeGroupCount() {
return this.nodeGroupCount;
}
/**
*
* The number of node groups (shards) that results from the modification of the shard configuration.
*
*
* @param nodeGroupCount
* The number of node groups (shards) that results from the modification of the shard configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withNodeGroupCount(Integer nodeGroupCount) {
setNodeGroupCount(nodeGroupCount);
return this;
}
/**
*
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value for
* this parameter is true
.
*
*
* Value: true
*
*
* @param applyImmediately
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value
* for this parameter is true
.
*
* Value: true
*/
public void setApplyImmediately(Boolean applyImmediately) {
this.applyImmediately = applyImmediately;
}
/**
*
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value for
* this parameter is true
.
*
*
* Value: true
*
*
* @return Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value
* for this parameter is true
.
*
* Value: true
*/
public Boolean getApplyImmediately() {
return this.applyImmediately;
}
/**
*
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value for
* this parameter is true
.
*
*
* Value: true
*
*
* @param applyImmediately
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value
* for this parameter is true
.
*
* Value: true
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withApplyImmediately(Boolean applyImmediately) {
setApplyImmediately(applyImmediately);
return this;
}
/**
*
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value for
* this parameter is true
.
*
*
* Value: true
*
*
* @return Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value
* for this parameter is true
.
*
* Value: true
*/
public Boolean isApplyImmediately() {
return this.applyImmediately;
}
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* @return Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
*/
public java.util.List getReshardingConfiguration() {
if (reshardingConfiguration == null) {
reshardingConfiguration = new com.amazonaws.internal.SdkInternalList();
}
return reshardingConfiguration;
}
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* @param reshardingConfiguration
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
*/
public void setReshardingConfiguration(java.util.Collection reshardingConfiguration) {
if (reshardingConfiguration == null) {
this.reshardingConfiguration = null;
return;
}
this.reshardingConfiguration = new com.amazonaws.internal.SdkInternalList(reshardingConfiguration);
}
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReshardingConfiguration(java.util.Collection)} or
* {@link #withReshardingConfiguration(java.util.Collection)} if you want to override the existing values.
*
*
* @param reshardingConfiguration
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withReshardingConfiguration(ReshardingConfiguration... reshardingConfiguration) {
if (this.reshardingConfiguration == null) {
setReshardingConfiguration(new com.amazonaws.internal.SdkInternalList(reshardingConfiguration.length));
}
for (ReshardingConfiguration ele : reshardingConfiguration) {
this.reshardingConfiguration.add(ele);
}
return this;
}
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* @param reshardingConfiguration
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withReshardingConfiguration(java.util.Collection reshardingConfiguration) {
setReshardingConfiguration(reshardingConfiguration);
return this;
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRemove
* is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
from the
* cluster.
*
*
* @return If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
*/
public java.util.List getNodeGroupsToRemove() {
if (nodeGroupsToRemove == null) {
nodeGroupsToRemove = new com.amazonaws.internal.SdkInternalList();
}
return nodeGroupsToRemove;
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRemove
* is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
from the
* cluster.
*
*
* @param nodeGroupsToRemove
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
*/
public void setNodeGroupsToRemove(java.util.Collection nodeGroupsToRemove) {
if (nodeGroupsToRemove == null) {
this.nodeGroupsToRemove = null;
return;
}
this.nodeGroupsToRemove = new com.amazonaws.internal.SdkInternalList(nodeGroupsToRemove);
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRemove
* is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
from the
* cluster.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNodeGroupsToRemove(java.util.Collection)} or {@link #withNodeGroupsToRemove(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param nodeGroupsToRemove
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withNodeGroupsToRemove(String... nodeGroupsToRemove) {
if (this.nodeGroupsToRemove == null) {
setNodeGroupsToRemove(new com.amazonaws.internal.SdkInternalList(nodeGroupsToRemove.length));
}
for (String ele : nodeGroupsToRemove) {
this.nodeGroupsToRemove.add(ele);
}
return this;
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRemove
* is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
from the
* cluster.
*
*
* @param nodeGroupsToRemove
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withNodeGroupsToRemove(java.util.Collection nodeGroupsToRemove) {
setNodeGroupsToRemove(nodeGroupsToRemove);
return this;
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRetain
* is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* @return If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*/
public java.util.List getNodeGroupsToRetain() {
if (nodeGroupsToRetain == null) {
nodeGroupsToRetain = new com.amazonaws.internal.SdkInternalList();
}
return nodeGroupsToRetain;
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRetain
* is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* @param nodeGroupsToRetain
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*/
public void setNodeGroupsToRetain(java.util.Collection nodeGroupsToRetain) {
if (nodeGroupsToRetain == null) {
this.nodeGroupsToRetain = null;
return;
}
this.nodeGroupsToRetain = new com.amazonaws.internal.SdkInternalList(nodeGroupsToRetain);
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRetain
* is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNodeGroupsToRetain(java.util.Collection)} or {@link #withNodeGroupsToRetain(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param nodeGroupsToRetain
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withNodeGroupsToRetain(String... nodeGroupsToRetain) {
if (this.nodeGroupsToRetain == null) {
setNodeGroupsToRetain(new com.amazonaws.internal.SdkInternalList(nodeGroupsToRetain.length));
}
for (String ele : nodeGroupsToRetain) {
this.nodeGroupsToRetain.add(ele);
}
return this;
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRetain
* is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* @param nodeGroupsToRetain
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache (Redis OSS) will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyReplicationGroupShardConfigurationRequest withNodeGroupsToRetain(java.util.Collection nodeGroupsToRetain) {
setNodeGroupsToRetain(nodeGroupsToRetain);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationGroupId() != null)
sb.append("ReplicationGroupId: ").append(getReplicationGroupId()).append(",");
if (getNodeGroupCount() != null)
sb.append("NodeGroupCount: ").append(getNodeGroupCount()).append(",");
if (getApplyImmediately() != null)
sb.append("ApplyImmediately: ").append(getApplyImmediately()).append(",");
if (getReshardingConfiguration() != null)
sb.append("ReshardingConfiguration: ").append(getReshardingConfiguration()).append(",");
if (getNodeGroupsToRemove() != null)
sb.append("NodeGroupsToRemove: ").append(getNodeGroupsToRemove()).append(",");
if (getNodeGroupsToRetain() != null)
sb.append("NodeGroupsToRetain: ").append(getNodeGroupsToRetain());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyReplicationGroupShardConfigurationRequest == false)
return false;
ModifyReplicationGroupShardConfigurationRequest other = (ModifyReplicationGroupShardConfigurationRequest) obj;
if (other.getReplicationGroupId() == null ^ this.getReplicationGroupId() == null)
return false;
if (other.getReplicationGroupId() != null && other.getReplicationGroupId().equals(this.getReplicationGroupId()) == false)
return false;
if (other.getNodeGroupCount() == null ^ this.getNodeGroupCount() == null)
return false;
if (other.getNodeGroupCount() != null && other.getNodeGroupCount().equals(this.getNodeGroupCount()) == false)
return false;
if (other.getApplyImmediately() == null ^ this.getApplyImmediately() == null)
return false;
if (other.getApplyImmediately() != null && other.getApplyImmediately().equals(this.getApplyImmediately()) == false)
return false;
if (other.getReshardingConfiguration() == null ^ this.getReshardingConfiguration() == null)
return false;
if (other.getReshardingConfiguration() != null && other.getReshardingConfiguration().equals(this.getReshardingConfiguration()) == false)
return false;
if (other.getNodeGroupsToRemove() == null ^ this.getNodeGroupsToRemove() == null)
return false;
if (other.getNodeGroupsToRemove() != null && other.getNodeGroupsToRemove().equals(this.getNodeGroupsToRemove()) == false)
return false;
if (other.getNodeGroupsToRetain() == null ^ this.getNodeGroupsToRetain() == null)
return false;
if (other.getNodeGroupsToRetain() != null && other.getNodeGroupsToRetain().equals(this.getNodeGroupsToRetain()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationGroupId() == null) ? 0 : getReplicationGroupId().hashCode());
hashCode = prime * hashCode + ((getNodeGroupCount() == null) ? 0 : getNodeGroupCount().hashCode());
hashCode = prime * hashCode + ((getApplyImmediately() == null) ? 0 : getApplyImmediately().hashCode());
hashCode = prime * hashCode + ((getReshardingConfiguration() == null) ? 0 : getReshardingConfiguration().hashCode());
hashCode = prime * hashCode + ((getNodeGroupsToRemove() == null) ? 0 : getNodeGroupsToRemove().hashCode());
hashCode = prime * hashCode + ((getNodeGroupsToRetain() == null) ? 0 : getNodeGroupsToRetain().hashCode());
return hashCode;
}
@Override
public ModifyReplicationGroupShardConfigurationRequest clone() {
return (ModifyReplicationGroupShardConfigurationRequest) super.clone();
}
}