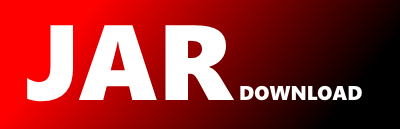
com.amazonaws.services.elasticache.model.ModifyUserGroupResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyUserGroupResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The ID of the user group.
*
*/
private String userGroupId;
/**
*
* Indicates user group status. Can be "creating", "active", "modifying", "deleting".
*
*/
private String status;
/**
*
* The current supported value is Redis user.
*
*/
private String engine;
/**
*
* The list of user IDs that belong to the user group.
*
*/
private com.amazonaws.internal.SdkInternalList userIds;
/**
*
* The minimum engine version required, which is Redis OSS 6.0
*
*/
private String minimumEngineVersion;
/**
*
* A list of updates being applied to the user group.
*
*/
private UserGroupPendingChanges pendingChanges;
/**
*
* A list of replication groups that the user group can access.
*
*/
private com.amazonaws.internal.SdkInternalList replicationGroups;
/**
*
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
*
*/
private com.amazonaws.internal.SdkInternalList serverlessCaches;
/**
*
* The Amazon Resource Name (ARN) of the user group.
*
*/
private String aRN;
/**
*
* The ID of the user group.
*
*
* @param userGroupId
* The ID of the user group.
*/
public void setUserGroupId(String userGroupId) {
this.userGroupId = userGroupId;
}
/**
*
* The ID of the user group.
*
*
* @return The ID of the user group.
*/
public String getUserGroupId() {
return this.userGroupId;
}
/**
*
* The ID of the user group.
*
*
* @param userGroupId
* The ID of the user group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withUserGroupId(String userGroupId) {
setUserGroupId(userGroupId);
return this;
}
/**
*
* Indicates user group status. Can be "creating", "active", "modifying", "deleting".
*
*
* @param status
* Indicates user group status. Can be "creating", "active", "modifying", "deleting".
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Indicates user group status. Can be "creating", "active", "modifying", "deleting".
*
*
* @return Indicates user group status. Can be "creating", "active", "modifying", "deleting".
*/
public String getStatus() {
return this.status;
}
/**
*
* Indicates user group status. Can be "creating", "active", "modifying", "deleting".
*
*
* @param status
* Indicates user group status. Can be "creating", "active", "modifying", "deleting".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current supported value is Redis user.
*
*
* @param engine
* The current supported value is Redis user.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The current supported value is Redis user.
*
*
* @return The current supported value is Redis user.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The current supported value is Redis user.
*
*
* @param engine
* The current supported value is Redis user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The list of user IDs that belong to the user group.
*
*
* @return The list of user IDs that belong to the user group.
*/
public java.util.List getUserIds() {
if (userIds == null) {
userIds = new com.amazonaws.internal.SdkInternalList();
}
return userIds;
}
/**
*
* The list of user IDs that belong to the user group.
*
*
* @param userIds
* The list of user IDs that belong to the user group.
*/
public void setUserIds(java.util.Collection userIds) {
if (userIds == null) {
this.userIds = null;
return;
}
this.userIds = new com.amazonaws.internal.SdkInternalList(userIds);
}
/**
*
* The list of user IDs that belong to the user group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUserIds(java.util.Collection)} or {@link #withUserIds(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param userIds
* The list of user IDs that belong to the user group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withUserIds(String... userIds) {
if (this.userIds == null) {
setUserIds(new com.amazonaws.internal.SdkInternalList(userIds.length));
}
for (String ele : userIds) {
this.userIds.add(ele);
}
return this;
}
/**
*
* The list of user IDs that belong to the user group.
*
*
* @param userIds
* The list of user IDs that belong to the user group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withUserIds(java.util.Collection userIds) {
setUserIds(userIds);
return this;
}
/**
*
* The minimum engine version required, which is Redis OSS 6.0
*
*
* @param minimumEngineVersion
* The minimum engine version required, which is Redis OSS 6.0
*/
public void setMinimumEngineVersion(String minimumEngineVersion) {
this.minimumEngineVersion = minimumEngineVersion;
}
/**
*
* The minimum engine version required, which is Redis OSS 6.0
*
*
* @return The minimum engine version required, which is Redis OSS 6.0
*/
public String getMinimumEngineVersion() {
return this.minimumEngineVersion;
}
/**
*
* The minimum engine version required, which is Redis OSS 6.0
*
*
* @param minimumEngineVersion
* The minimum engine version required, which is Redis OSS 6.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withMinimumEngineVersion(String minimumEngineVersion) {
setMinimumEngineVersion(minimumEngineVersion);
return this;
}
/**
*
* A list of updates being applied to the user group.
*
*
* @param pendingChanges
* A list of updates being applied to the user group.
*/
public void setPendingChanges(UserGroupPendingChanges pendingChanges) {
this.pendingChanges = pendingChanges;
}
/**
*
* A list of updates being applied to the user group.
*
*
* @return A list of updates being applied to the user group.
*/
public UserGroupPendingChanges getPendingChanges() {
return this.pendingChanges;
}
/**
*
* A list of updates being applied to the user group.
*
*
* @param pendingChanges
* A list of updates being applied to the user group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withPendingChanges(UserGroupPendingChanges pendingChanges) {
setPendingChanges(pendingChanges);
return this;
}
/**
*
* A list of replication groups that the user group can access.
*
*
* @return A list of replication groups that the user group can access.
*/
public java.util.List getReplicationGroups() {
if (replicationGroups == null) {
replicationGroups = new com.amazonaws.internal.SdkInternalList();
}
return replicationGroups;
}
/**
*
* A list of replication groups that the user group can access.
*
*
* @param replicationGroups
* A list of replication groups that the user group can access.
*/
public void setReplicationGroups(java.util.Collection replicationGroups) {
if (replicationGroups == null) {
this.replicationGroups = null;
return;
}
this.replicationGroups = new com.amazonaws.internal.SdkInternalList(replicationGroups);
}
/**
*
* A list of replication groups that the user group can access.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicationGroups(java.util.Collection)} or {@link #withReplicationGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param replicationGroups
* A list of replication groups that the user group can access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withReplicationGroups(String... replicationGroups) {
if (this.replicationGroups == null) {
setReplicationGroups(new com.amazonaws.internal.SdkInternalList(replicationGroups.length));
}
for (String ele : replicationGroups) {
this.replicationGroups.add(ele);
}
return this;
}
/**
*
* A list of replication groups that the user group can access.
*
*
* @param replicationGroups
* A list of replication groups that the user group can access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withReplicationGroups(java.util.Collection replicationGroups) {
setReplicationGroups(replicationGroups);
return this;
}
/**
*
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @return Indicates which serverless caches the specified user group is associated with. Available for Redis OSS
* and Serverless Memcached only.
*/
public java.util.List getServerlessCaches() {
if (serverlessCaches == null) {
serverlessCaches = new com.amazonaws.internal.SdkInternalList();
}
return serverlessCaches;
}
/**
*
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param serverlessCaches
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
*/
public void setServerlessCaches(java.util.Collection serverlessCaches) {
if (serverlessCaches == null) {
this.serverlessCaches = null;
return;
}
this.serverlessCaches = new com.amazonaws.internal.SdkInternalList(serverlessCaches);
}
/**
*
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServerlessCaches(java.util.Collection)} or {@link #withServerlessCaches(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param serverlessCaches
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withServerlessCaches(String... serverlessCaches) {
if (this.serverlessCaches == null) {
setServerlessCaches(new com.amazonaws.internal.SdkInternalList(serverlessCaches.length));
}
for (String ele : serverlessCaches) {
this.serverlessCaches.add(ele);
}
return this;
}
/**
*
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param serverlessCaches
* Indicates which serverless caches the specified user group is associated with. Available for Redis OSS and
* Serverless Memcached only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withServerlessCaches(java.util.Collection serverlessCaches) {
setServerlessCaches(serverlessCaches);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the user group.
*
*
* @param aRN
* The Amazon Resource Name (ARN) of the user group.
*/
public void setARN(String aRN) {
this.aRN = aRN;
}
/**
*
* The Amazon Resource Name (ARN) of the user group.
*
*
* @return The Amazon Resource Name (ARN) of the user group.
*/
public String getARN() {
return this.aRN;
}
/**
*
* The Amazon Resource Name (ARN) of the user group.
*
*
* @param aRN
* The Amazon Resource Name (ARN) of the user group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyUserGroupResult withARN(String aRN) {
setARN(aRN);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUserGroupId() != null)
sb.append("UserGroupId: ").append(getUserGroupId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getUserIds() != null)
sb.append("UserIds: ").append(getUserIds()).append(",");
if (getMinimumEngineVersion() != null)
sb.append("MinimumEngineVersion: ").append(getMinimumEngineVersion()).append(",");
if (getPendingChanges() != null)
sb.append("PendingChanges: ").append(getPendingChanges()).append(",");
if (getReplicationGroups() != null)
sb.append("ReplicationGroups: ").append(getReplicationGroups()).append(",");
if (getServerlessCaches() != null)
sb.append("ServerlessCaches: ").append(getServerlessCaches()).append(",");
if (getARN() != null)
sb.append("ARN: ").append(getARN());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyUserGroupResult == false)
return false;
ModifyUserGroupResult other = (ModifyUserGroupResult) obj;
if (other.getUserGroupId() == null ^ this.getUserGroupId() == null)
return false;
if (other.getUserGroupId() != null && other.getUserGroupId().equals(this.getUserGroupId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getUserIds() == null ^ this.getUserIds() == null)
return false;
if (other.getUserIds() != null && other.getUserIds().equals(this.getUserIds()) == false)
return false;
if (other.getMinimumEngineVersion() == null ^ this.getMinimumEngineVersion() == null)
return false;
if (other.getMinimumEngineVersion() != null && other.getMinimumEngineVersion().equals(this.getMinimumEngineVersion()) == false)
return false;
if (other.getPendingChanges() == null ^ this.getPendingChanges() == null)
return false;
if (other.getPendingChanges() != null && other.getPendingChanges().equals(this.getPendingChanges()) == false)
return false;
if (other.getReplicationGroups() == null ^ this.getReplicationGroups() == null)
return false;
if (other.getReplicationGroups() != null && other.getReplicationGroups().equals(this.getReplicationGroups()) == false)
return false;
if (other.getServerlessCaches() == null ^ this.getServerlessCaches() == null)
return false;
if (other.getServerlessCaches() != null && other.getServerlessCaches().equals(this.getServerlessCaches()) == false)
return false;
if (other.getARN() == null ^ this.getARN() == null)
return false;
if (other.getARN() != null && other.getARN().equals(this.getARN()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUserGroupId() == null) ? 0 : getUserGroupId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getUserIds() == null) ? 0 : getUserIds().hashCode());
hashCode = prime * hashCode + ((getMinimumEngineVersion() == null) ? 0 : getMinimumEngineVersion().hashCode());
hashCode = prime * hashCode + ((getPendingChanges() == null) ? 0 : getPendingChanges().hashCode());
hashCode = prime * hashCode + ((getReplicationGroups() == null) ? 0 : getReplicationGroups().hashCode());
hashCode = prime * hashCode + ((getServerlessCaches() == null) ? 0 : getServerlessCaches().hashCode());
hashCode = prime * hashCode + ((getARN() == null) ? 0 : getARN().hashCode());
return hashCode;
}
@Override
public ModifyUserGroupResult clone() {
try {
return (ModifyUserGroupResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}