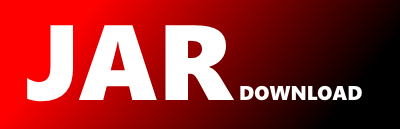
com.amazonaws.services.elasticache.model.NodeGroupMemberUpdateStatus Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The status of the service update on the node group member
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class NodeGroupMemberUpdateStatus implements Serializable, Cloneable {
/**
*
* The cache cluster ID
*
*/
private String cacheClusterId;
/**
*
* The node ID of the cache cluster
*
*/
private String cacheNodeId;
/**
*
* The update status of the node
*
*/
private String nodeUpdateStatus;
/**
*
* The deletion date of the node
*
*/
private java.util.Date nodeDeletionDate;
/**
*
* The start date of the update for a node
*
*/
private java.util.Date nodeUpdateStartDate;
/**
*
* The end date of the update for a node
*
*/
private java.util.Date nodeUpdateEndDate;
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*/
private String nodeUpdateInitiatedBy;
/**
*
* The date when the update is triggered
*
*/
private java.util.Date nodeUpdateInitiatedDate;
/**
*
* The date when the NodeUpdateStatus was last modified
*
*/
private java.util.Date nodeUpdateStatusModifiedDate;
/**
*
* The cache cluster ID
*
*
* @param cacheClusterId
* The cache cluster ID
*/
public void setCacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
}
/**
*
* The cache cluster ID
*
*
* @return The cache cluster ID
*/
public String getCacheClusterId() {
return this.cacheClusterId;
}
/**
*
* The cache cluster ID
*
*
* @param cacheClusterId
* The cache cluster ID
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withCacheClusterId(String cacheClusterId) {
setCacheClusterId(cacheClusterId);
return this;
}
/**
*
* The node ID of the cache cluster
*
*
* @param cacheNodeId
* The node ID of the cache cluster
*/
public void setCacheNodeId(String cacheNodeId) {
this.cacheNodeId = cacheNodeId;
}
/**
*
* The node ID of the cache cluster
*
*
* @return The node ID of the cache cluster
*/
public String getCacheNodeId() {
return this.cacheNodeId;
}
/**
*
* The node ID of the cache cluster
*
*
* @param cacheNodeId
* The node ID of the cache cluster
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withCacheNodeId(String cacheNodeId) {
setCacheNodeId(cacheNodeId);
return this;
}
/**
*
* The update status of the node
*
*
* @param nodeUpdateStatus
* The update status of the node
* @see NodeUpdateStatus
*/
public void setNodeUpdateStatus(String nodeUpdateStatus) {
this.nodeUpdateStatus = nodeUpdateStatus;
}
/**
*
* The update status of the node
*
*
* @return The update status of the node
* @see NodeUpdateStatus
*/
public String getNodeUpdateStatus() {
return this.nodeUpdateStatus;
}
/**
*
* The update status of the node
*
*
* @param nodeUpdateStatus
* The update status of the node
* @return Returns a reference to this object so that method calls can be chained together.
* @see NodeUpdateStatus
*/
public NodeGroupMemberUpdateStatus withNodeUpdateStatus(String nodeUpdateStatus) {
setNodeUpdateStatus(nodeUpdateStatus);
return this;
}
/**
*
* The update status of the node
*
*
* @param nodeUpdateStatus
* The update status of the node
* @return Returns a reference to this object so that method calls can be chained together.
* @see NodeUpdateStatus
*/
public NodeGroupMemberUpdateStatus withNodeUpdateStatus(NodeUpdateStatus nodeUpdateStatus) {
this.nodeUpdateStatus = nodeUpdateStatus.toString();
return this;
}
/**
*
* The deletion date of the node
*
*
* @param nodeDeletionDate
* The deletion date of the node
*/
public void setNodeDeletionDate(java.util.Date nodeDeletionDate) {
this.nodeDeletionDate = nodeDeletionDate;
}
/**
*
* The deletion date of the node
*
*
* @return The deletion date of the node
*/
public java.util.Date getNodeDeletionDate() {
return this.nodeDeletionDate;
}
/**
*
* The deletion date of the node
*
*
* @param nodeDeletionDate
* The deletion date of the node
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withNodeDeletionDate(java.util.Date nodeDeletionDate) {
setNodeDeletionDate(nodeDeletionDate);
return this;
}
/**
*
* The start date of the update for a node
*
*
* @param nodeUpdateStartDate
* The start date of the update for a node
*/
public void setNodeUpdateStartDate(java.util.Date nodeUpdateStartDate) {
this.nodeUpdateStartDate = nodeUpdateStartDate;
}
/**
*
* The start date of the update for a node
*
*
* @return The start date of the update for a node
*/
public java.util.Date getNodeUpdateStartDate() {
return this.nodeUpdateStartDate;
}
/**
*
* The start date of the update for a node
*
*
* @param nodeUpdateStartDate
* The start date of the update for a node
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withNodeUpdateStartDate(java.util.Date nodeUpdateStartDate) {
setNodeUpdateStartDate(nodeUpdateStartDate);
return this;
}
/**
*
* The end date of the update for a node
*
*
* @param nodeUpdateEndDate
* The end date of the update for a node
*/
public void setNodeUpdateEndDate(java.util.Date nodeUpdateEndDate) {
this.nodeUpdateEndDate = nodeUpdateEndDate;
}
/**
*
* The end date of the update for a node
*
*
* @return The end date of the update for a node
*/
public java.util.Date getNodeUpdateEndDate() {
return this.nodeUpdateEndDate;
}
/**
*
* The end date of the update for a node
*
*
* @param nodeUpdateEndDate
* The end date of the update for a node
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withNodeUpdateEndDate(java.util.Date nodeUpdateEndDate) {
setNodeUpdateEndDate(nodeUpdateEndDate);
return this;
}
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*
* @param nodeUpdateInitiatedBy
* Reflects whether the update was initiated by the customer or automatically applied
* @see NodeUpdateInitiatedBy
*/
public void setNodeUpdateInitiatedBy(String nodeUpdateInitiatedBy) {
this.nodeUpdateInitiatedBy = nodeUpdateInitiatedBy;
}
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*
* @return Reflects whether the update was initiated by the customer or automatically applied
* @see NodeUpdateInitiatedBy
*/
public String getNodeUpdateInitiatedBy() {
return this.nodeUpdateInitiatedBy;
}
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*
* @param nodeUpdateInitiatedBy
* Reflects whether the update was initiated by the customer or automatically applied
* @return Returns a reference to this object so that method calls can be chained together.
* @see NodeUpdateInitiatedBy
*/
public NodeGroupMemberUpdateStatus withNodeUpdateInitiatedBy(String nodeUpdateInitiatedBy) {
setNodeUpdateInitiatedBy(nodeUpdateInitiatedBy);
return this;
}
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*
* @param nodeUpdateInitiatedBy
* Reflects whether the update was initiated by the customer or automatically applied
* @return Returns a reference to this object so that method calls can be chained together.
* @see NodeUpdateInitiatedBy
*/
public NodeGroupMemberUpdateStatus withNodeUpdateInitiatedBy(NodeUpdateInitiatedBy nodeUpdateInitiatedBy) {
this.nodeUpdateInitiatedBy = nodeUpdateInitiatedBy.toString();
return this;
}
/**
*
* The date when the update is triggered
*
*
* @param nodeUpdateInitiatedDate
* The date when the update is triggered
*/
public void setNodeUpdateInitiatedDate(java.util.Date nodeUpdateInitiatedDate) {
this.nodeUpdateInitiatedDate = nodeUpdateInitiatedDate;
}
/**
*
* The date when the update is triggered
*
*
* @return The date when the update is triggered
*/
public java.util.Date getNodeUpdateInitiatedDate() {
return this.nodeUpdateInitiatedDate;
}
/**
*
* The date when the update is triggered
*
*
* @param nodeUpdateInitiatedDate
* The date when the update is triggered
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withNodeUpdateInitiatedDate(java.util.Date nodeUpdateInitiatedDate) {
setNodeUpdateInitiatedDate(nodeUpdateInitiatedDate);
return this;
}
/**
*
* The date when the NodeUpdateStatus was last modified
*
*
* @param nodeUpdateStatusModifiedDate
* The date when the NodeUpdateStatus was last modified
*/
public void setNodeUpdateStatusModifiedDate(java.util.Date nodeUpdateStatusModifiedDate) {
this.nodeUpdateStatusModifiedDate = nodeUpdateStatusModifiedDate;
}
/**
*
* The date when the NodeUpdateStatus was last modified
*
*
* @return The date when the NodeUpdateStatus was last modified
*/
public java.util.Date getNodeUpdateStatusModifiedDate() {
return this.nodeUpdateStatusModifiedDate;
}
/**
*
* The date when the NodeUpdateStatus was last modified
*
*
* @param nodeUpdateStatusModifiedDate
* The date when the NodeUpdateStatus was last modified
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupMemberUpdateStatus withNodeUpdateStatusModifiedDate(java.util.Date nodeUpdateStatusModifiedDate) {
setNodeUpdateStatusModifiedDate(nodeUpdateStatusModifiedDate);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCacheClusterId() != null)
sb.append("CacheClusterId: ").append(getCacheClusterId()).append(",");
if (getCacheNodeId() != null)
sb.append("CacheNodeId: ").append(getCacheNodeId()).append(",");
if (getNodeUpdateStatus() != null)
sb.append("NodeUpdateStatus: ").append(getNodeUpdateStatus()).append(",");
if (getNodeDeletionDate() != null)
sb.append("NodeDeletionDate: ").append(getNodeDeletionDate()).append(",");
if (getNodeUpdateStartDate() != null)
sb.append("NodeUpdateStartDate: ").append(getNodeUpdateStartDate()).append(",");
if (getNodeUpdateEndDate() != null)
sb.append("NodeUpdateEndDate: ").append(getNodeUpdateEndDate()).append(",");
if (getNodeUpdateInitiatedBy() != null)
sb.append("NodeUpdateInitiatedBy: ").append(getNodeUpdateInitiatedBy()).append(",");
if (getNodeUpdateInitiatedDate() != null)
sb.append("NodeUpdateInitiatedDate: ").append(getNodeUpdateInitiatedDate()).append(",");
if (getNodeUpdateStatusModifiedDate() != null)
sb.append("NodeUpdateStatusModifiedDate: ").append(getNodeUpdateStatusModifiedDate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof NodeGroupMemberUpdateStatus == false)
return false;
NodeGroupMemberUpdateStatus other = (NodeGroupMemberUpdateStatus) obj;
if (other.getCacheClusterId() == null ^ this.getCacheClusterId() == null)
return false;
if (other.getCacheClusterId() != null && other.getCacheClusterId().equals(this.getCacheClusterId()) == false)
return false;
if (other.getCacheNodeId() == null ^ this.getCacheNodeId() == null)
return false;
if (other.getCacheNodeId() != null && other.getCacheNodeId().equals(this.getCacheNodeId()) == false)
return false;
if (other.getNodeUpdateStatus() == null ^ this.getNodeUpdateStatus() == null)
return false;
if (other.getNodeUpdateStatus() != null && other.getNodeUpdateStatus().equals(this.getNodeUpdateStatus()) == false)
return false;
if (other.getNodeDeletionDate() == null ^ this.getNodeDeletionDate() == null)
return false;
if (other.getNodeDeletionDate() != null && other.getNodeDeletionDate().equals(this.getNodeDeletionDate()) == false)
return false;
if (other.getNodeUpdateStartDate() == null ^ this.getNodeUpdateStartDate() == null)
return false;
if (other.getNodeUpdateStartDate() != null && other.getNodeUpdateStartDate().equals(this.getNodeUpdateStartDate()) == false)
return false;
if (other.getNodeUpdateEndDate() == null ^ this.getNodeUpdateEndDate() == null)
return false;
if (other.getNodeUpdateEndDate() != null && other.getNodeUpdateEndDate().equals(this.getNodeUpdateEndDate()) == false)
return false;
if (other.getNodeUpdateInitiatedBy() == null ^ this.getNodeUpdateInitiatedBy() == null)
return false;
if (other.getNodeUpdateInitiatedBy() != null && other.getNodeUpdateInitiatedBy().equals(this.getNodeUpdateInitiatedBy()) == false)
return false;
if (other.getNodeUpdateInitiatedDate() == null ^ this.getNodeUpdateInitiatedDate() == null)
return false;
if (other.getNodeUpdateInitiatedDate() != null && other.getNodeUpdateInitiatedDate().equals(this.getNodeUpdateInitiatedDate()) == false)
return false;
if (other.getNodeUpdateStatusModifiedDate() == null ^ this.getNodeUpdateStatusModifiedDate() == null)
return false;
if (other.getNodeUpdateStatusModifiedDate() != null && other.getNodeUpdateStatusModifiedDate().equals(this.getNodeUpdateStatusModifiedDate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCacheClusterId() == null) ? 0 : getCacheClusterId().hashCode());
hashCode = prime * hashCode + ((getCacheNodeId() == null) ? 0 : getCacheNodeId().hashCode());
hashCode = prime * hashCode + ((getNodeUpdateStatus() == null) ? 0 : getNodeUpdateStatus().hashCode());
hashCode = prime * hashCode + ((getNodeDeletionDate() == null) ? 0 : getNodeDeletionDate().hashCode());
hashCode = prime * hashCode + ((getNodeUpdateStartDate() == null) ? 0 : getNodeUpdateStartDate().hashCode());
hashCode = prime * hashCode + ((getNodeUpdateEndDate() == null) ? 0 : getNodeUpdateEndDate().hashCode());
hashCode = prime * hashCode + ((getNodeUpdateInitiatedBy() == null) ? 0 : getNodeUpdateInitiatedBy().hashCode());
hashCode = prime * hashCode + ((getNodeUpdateInitiatedDate() == null) ? 0 : getNodeUpdateInitiatedDate().hashCode());
hashCode = prime * hashCode + ((getNodeUpdateStatusModifiedDate() == null) ? 0 : getNodeUpdateStatusModifiedDate().hashCode());
return hashCode;
}
@Override
public NodeGroupMemberUpdateStatus clone() {
try {
return (NodeGroupMemberUpdateStatus) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}