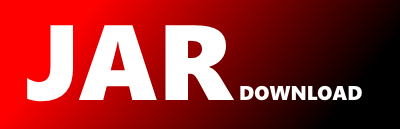
com.amazonaws.services.elasticache.AmazonElastiCache Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.elasticache.model.*;
import com.amazonaws.services.elasticache.waiters.AmazonElastiCacheWaiters;
/**
* Interface for accessing Amazon ElastiCache.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.elasticache.AbstractAmazonElastiCache} instead.
*
*
* Amazon ElastiCache
*
* Amazon ElastiCache is a web service that makes it easier to set up, operate, and scale a distributed cache in the
* cloud.
*
*
* With ElastiCache, customers get all of the benefits of a high-performance, in-memory cache with less of the
* administrative burden involved in launching and managing a distributed cache. The service makes setup, scaling, and
* cluster failure handling much simpler than in a self-managed cache deployment.
*
*
* In addition, through integration with Amazon CloudWatch, customers get enhanced visibility into the key performance
* statistics associated with their cache and can receive alarms if a part of their cache runs hot.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonElastiCache {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "elasticache";
/**
* Overrides the default endpoint for this client ("elasticache.us-east-1.amazonaws.com"). Callers can use this
* method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "elasticache.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "elasticache.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol
* from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "elasticache.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "elasticache.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate
* with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonElastiCache#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource
* -level permissions.
*
*
* For example, you can use cost-allocation tags to your ElastiCache resources, Amazon generates a cost allocation
* report as a comma-separated value (CSV) file with your usage and costs aggregated by your tags. You can apply
* tags that represent business categories (such as cost centers, application names, or owners) to organize your
* costs across multiple services.
*
*
* For more information, see Using Cost Allocation Tags in
* Amazon ElastiCache in the ElastiCache User Guide.
*
*
* @param addTagsToResourceRequest
* Represents the input of an AddTagsToResource operation.
* @return Result of the AddTagsToResource operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Redis OSS and Serverless
* Memcached only.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidARNException
* The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* @sample AmazonElastiCache.AddTagsToResource
* @see AWS
* API Documentation
*/
AddTagsToResourceResult addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Allows network ingress to a cache security group. Applications using ElastiCache must be running on Amazon EC2,
* and Amazon EC2 security groups are used as the authorization mechanism.
*
*
*
* You cannot authorize ingress from an Amazon EC2 security group in one region to an ElastiCache cluster in another
* region.
*
*
*
* @param authorizeCacheSecurityGroupIngressRequest
* Represents the input of an AuthorizeCacheSecurityGroupIngress operation.
* @return Result of the AuthorizeCacheSecurityGroupIngress operation returned by the service.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws AuthorizationAlreadyExistsException
* The specified Amazon EC2 security group is already authorized for the specified cache security group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.AuthorizeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
CacheSecurityGroup authorizeCacheSecurityGroupIngress(AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest);
/**
*
* Apply the service update. For more information on service updates and applying them, see Applying Service
* Updates.
*
*
* @param batchApplyUpdateActionRequest
* @return Result of the BatchApplyUpdateAction operation returned by the service.
* @throws ServiceUpdateNotFoundException
* The service update doesn't exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.BatchApplyUpdateAction
* @see AWS API Documentation
*/
BatchApplyUpdateActionResult batchApplyUpdateAction(BatchApplyUpdateActionRequest batchApplyUpdateActionRequest);
/**
*
* Stop the service update. For more information on service updates and stopping them, see Stopping
* Service Updates.
*
*
* @param batchStopUpdateActionRequest
* @return Result of the BatchStopUpdateAction operation returned by the service.
* @throws ServiceUpdateNotFoundException
* The service update doesn't exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.BatchStopUpdateAction
* @see AWS API Documentation
*/
BatchStopUpdateActionResult batchStopUpdateAction(BatchStopUpdateActionRequest batchStopUpdateActionRequest);
/**
*
* Complete the migration of data.
*
*
* @param completeMigrationRequest
* @return Result of the CompleteMigration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotUnderMigrationException
* The designated replication group is not available for data migration.
* @sample AmazonElastiCache.CompleteMigration
* @see AWS
* API Documentation
*/
ReplicationGroup completeMigration(CompleteMigrationRequest completeMigrationRequest);
/**
*
* Creates a copy of an existing serverless cache’s snapshot. Available for Redis OSS and Serverless Memcached only.
*
*
* @param copyServerlessCacheSnapshotRequest
* @return Result of the CopyServerlessCacheSnapshot operation returned by the service.
* @throws ServerlessCacheSnapshotAlreadyExistsException
* A serverless cache snapshot with this name already exists. Available for Redis OSS and Serverless
* Memcached only.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws ServerlessCacheSnapshotQuotaExceededException
* The number of serverless cache snapshots exceeds the customer snapshot quota. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Redis OSS and Serverless
* Memcached only.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CopyServerlessCacheSnapshot
* @see AWS API Documentation
*/
CopyServerlessCacheSnapshotResult copyServerlessCacheSnapshot(CopyServerlessCacheSnapshotRequest copyServerlessCacheSnapshotRequest);
/**
*
* Makes a copy of an existing snapshot.
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* Users or groups that have permissions to use the CopySnapshot
operation can create their own Amazon
* S3 buckets and copy snapshots to it. To control access to your snapshots, use an IAM policy to control who has
* the ability to use the CopySnapshot
operation. For more information about using IAM to control the
* use of ElastiCache operations, see Exporting Snapshots
* and Authentication & Access
* Control.
*
*
*
* You could receive the following error messages.
*
*
* Error Messages
*
*
* -
*
* Error Message: The S3 bucket %s is outside of the region.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s does not exist.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s is not owned by the authenticated user.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The authenticated user does not have sufficient permissions to perform the desired
* activity.
*
*
* Solution: Contact your system administrator to get the needed permissions.
*
*
* -
*
* Error Message: The S3 bucket %s already contains an object with key %s.
*
*
* Solution: Give the TargetSnapshotName
a new and unique value. If exporting a snapshot, you
* could alternatively create a new Amazon S3 bucket and use this same value for TargetSnapshotName
.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ permissions %s on the S3 Bucket.
*
*
* Solution: Add List and Read permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted WRITE permissions %s on the S3 Bucket.
*
*
* Solution: Add Upload/Delete permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ_ACP permissions %s on the S3 Bucket.
*
*
* Solution: Add View Permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
*
*
* @param copySnapshotRequest
* Represents the input of a CopySnapshotMessage
operation.
* @return Result of the CopySnapshot operation returned by the service.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws InvalidSnapshotStateException
* The current state of the snapshot does not allow the requested operation to occur.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CopySnapshot
* @see AWS API
* Documentation
*/
Snapshot copySnapshot(CopySnapshotRequest copySnapshotRequest);
/**
*
* Creates a cluster. All nodes in the cluster run the same protocol-compliant cache engine software, either
* Memcached or Redis OSS.
*
*
* This operation is not supported for Redis OSS (cluster mode enabled) clusters.
*
*
* @param createCacheClusterRequest
* Represents the input of a CreateCacheCluster operation.
* @return Result of the CreateCacheCluster operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws CacheClusterAlreadyExistsException
* You already have a cluster with the given identifier.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CreateCacheCluster
* @see AWS
* API Documentation
*/
CacheCluster createCacheCluster(CreateCacheClusterRequest createCacheClusterRequest);
/**
*
* Creates a new Amazon ElastiCache cache parameter group. An ElastiCache cache parameter group is a collection of
* parameters and their values that are applied to all of the nodes in any cluster or replication group using the
* CacheParameterGroup.
*
*
* A newly created CacheParameterGroup is an exact duplicate of the default parameter group for the
* CacheParameterGroupFamily. To customize the newly created CacheParameterGroup you can change the values of
* specific parameters. For more information, see:
*
*
* -
*
*
* ModifyCacheParameterGroup in the ElastiCache API Reference.
*
*
* -
*
* Parameters and
* Parameter Groups in the ElastiCache User Guide.
*
*
*
*
* @param createCacheParameterGroupRequest
* Represents the input of a CreateCacheParameterGroup
operation.
* @return Result of the CreateCacheParameterGroup operation returned by the service.
* @throws CacheParameterGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of cache security groups.
* @throws CacheParameterGroupAlreadyExistsException
* A cache parameter group with the requested name already exists.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CreateCacheParameterGroup
* @see AWS API Documentation
*/
CacheParameterGroup createCacheParameterGroup(CreateCacheParameterGroupRequest createCacheParameterGroupRequest);
/**
*
* Creates a new cache security group. Use a cache security group to control access to one or more clusters.
*
*
* Cache security groups are only used when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC). If you are creating a cluster inside of a VPC, use a cache subnet group instead. For more
* information, see CreateCacheSubnetGroup.
*
*
* @param createCacheSecurityGroupRequest
* Represents the input of a CreateCacheSecurityGroup
operation.
* @return Result of the CreateCacheSecurityGroup operation returned by the service.
* @throws CacheSecurityGroupAlreadyExistsException
* A cache security group with the specified name already exists.
* @throws CacheSecurityGroupQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of cache security groups.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CreateCacheSecurityGroup
* @see AWS API Documentation
*/
CacheSecurityGroup createCacheSecurityGroup(CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest);
/**
*
* Creates a new cache subnet group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createCacheSubnetGroupRequest
* Represents the input of a CreateCacheSubnetGroup
operation.
* @return Result of the CreateCacheSubnetGroup operation returned by the service.
* @throws CacheSubnetGroupAlreadyExistsException
* The requested cache subnet group name is already in use by an existing cache subnet group.
* @throws CacheSubnetGroupQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of cache subnet groups.
* @throws CacheSubnetQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a cache subnet
* group.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidSubnetException
* An invalid subnet identifier was specified.
* @throws SubnetNotAllowedException
* At least one subnet ID does not match the other subnet IDs. This mismatch typically occurs when a user
* sets one subnet ID to a regional Availability Zone and a different one to an outpost. Or when a user sets
* the subnet ID to an Outpost when not subscribed on this service.
* @sample AmazonElastiCache.CreateCacheSubnetGroup
* @see AWS API Documentation
*/
CacheSubnetGroup createCacheSubnetGroup(CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest);
/**
*
* Global Datastore for Redis OSS offers fully managed, fast, reliable and secure cross-region replication. Using
* Global Datastore for Redis OSS, you can create cross-region read replica clusters for ElastiCache (Redis OSS) to
* enable low-latency reads and disaster recovery across regions. For more information, see Replication Across
* Regions Using Global Datastore.
*
*
* -
*
* The GlobalReplicationGroupIdSuffix is the name of the Global datastore.
*
*
* -
*
* The PrimaryReplicationGroupId represents the name of the primary cluster that accepts writes and will
* replicate updates to the secondary cluster.
*
*
*
*
* @param createGlobalReplicationGroupRequest
* @return Result of the CreateGlobalReplicationGroup operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws GlobalReplicationGroupAlreadyExistsException
* The Global datastore name already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.CreateGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup createGlobalReplicationGroup(CreateGlobalReplicationGroupRequest createGlobalReplicationGroupRequest);
/**
*
* Creates a Redis OSS (cluster mode disabled) or a Redis OSS (cluster mode enabled) replication group.
*
*
* This API can be used to create a standalone regional replication group or a secondary replication group
* associated with a Global datastore.
*
*
* A Redis OSS (cluster mode disabled) replication group is a collection of nodes, where one of the nodes is a
* read/write primary and the others are read-only replicas. Writes to the primary are asynchronously propagated to
* the replicas.
*
*
* A Redis OSS cluster-mode enabled cluster is comprised of from 1 to 90 shards (API/CLI: node groups). Each shard
* has a primary node and up to 5 read-only replica nodes. The configuration can range from 90 shards and 0 replicas
* to 15 shards and 5 replicas, which is the maximum number or replicas allowed.
*
*
* The node or shard limit can be increased to a maximum of 500 per cluster if the Redis OSS engine version is 5.0.6
* or higher. For example, you can choose to configure a 500 node cluster that ranges between 83 shards (one primary
* and 5 replicas per shard) and 500 shards (single primary and no replicas). Make sure there are enough available
* IP addresses to accommodate the increase. Common pitfalls include the subnets in the subnet group have too small
* a CIDR range or the subnets are shared and heavily used by other clusters. For more information, see Creating a Subnet
* Group. For versions below 5.0.6, the limit is 250 per cluster.
*
*
* To request a limit increase, see Amazon Service Limits and choose
* the limit type Nodes per cluster per instance type.
*
*
* When a Redis OSS (cluster mode disabled) replication group has been successfully created, you can add one or more
* read replicas to it, up to a total of 5 read replicas. If you need to increase or decrease the number of node
* groups (console: shards), you can use ElastiCache (Redis OSS) scaling. For more information, see Scaling ElastiCache (Redis OSS)
* Clusters in the ElastiCache User Guide.
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* @param createReplicationGroupRequest
* Represents the input of a CreateReplicationGroup
operation.
* @return Result of the CreateReplicationGroup operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws ReplicationGroupAlreadyExistsException
* The specified replication group already exists.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CreateReplicationGroup
* @see AWS API Documentation
*/
ReplicationGroup createReplicationGroup(CreateReplicationGroupRequest createReplicationGroupRequest);
/**
*
* Creates a serverless cache.
*
*
* @param createServerlessCacheRequest
* @return Result of the CreateServerlessCache operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheAlreadyExistsException
* A serverless cache with this name already exists.
* @throws ServerlessCacheQuotaForCustomerExceededException
* The number of serverless caches exceeds the customer quota.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidCredentialsException
* You must enter valid credentials.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @sample AmazonElastiCache.CreateServerlessCache
* @see AWS API Documentation
*/
CreateServerlessCacheResult createServerlessCache(CreateServerlessCacheRequest createServerlessCacheRequest);
/**
*
* This API creates a copy of an entire ServerlessCache at a specific moment in time. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param createServerlessCacheSnapshotRequest
* @return Result of the CreateServerlessCacheSnapshot operation returned by the service.
* @throws ServerlessCacheSnapshotAlreadyExistsException
* A serverless cache snapshot with this name already exists. Available for Redis OSS and Serverless
* Memcached only.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotQuotaExceededException
* The number of serverless cache snapshots exceeds the customer snapshot quota. Available for Redis OSS and
* Serverless Memcached only.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.CreateServerlessCacheSnapshot
* @see AWS API Documentation
*/
CreateServerlessCacheSnapshotResult createServerlessCacheSnapshot(CreateServerlessCacheSnapshotRequest createServerlessCacheSnapshotRequest);
/**
*
* Creates a copy of an entire cluster or replication group at a specific moment in time.
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* @param createSnapshotRequest
* Represents the input of a CreateSnapshot
operation.
* @return Result of the CreateSnapshot operation returned by the service.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws SnapshotFeatureNotSupportedException
* You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.CreateSnapshot
* @see AWS API
* Documentation
*/
Snapshot createSnapshot(CreateSnapshotRequest createSnapshotRequest);
/**
*
* For Redis OSS engine version 6.0 onwards: Creates a Redis OSS user. For more information, see Using Role Based Access
* Control (RBAC).
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws UserAlreadyExistsException
* A user with this ID already exists.
* @throws UserQuotaExceededException
* The quota of users has been exceeded.
* @throws DuplicateUserNameException
* A user with this username already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @sample AmazonElastiCache.CreateUser
* @see AWS API
* Documentation
*/
CreateUserResult createUser(CreateUserRequest createUserRequest);
/**
*
* For Redis OSS engine version 6.0 onwards: Creates a Redis OSS user group. For more information, see Using Role Based Access
* Control (RBAC)
*
*
* @param createUserGroupRequest
* @return Result of the CreateUserGroup operation returned by the service.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws DuplicateUserNameException
* A user with this username already exists.
* @throws UserGroupAlreadyExistsException
* The user group with this ID already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws DefaultUserRequiredException
* You must add default user to a user group.
* @throws UserGroupQuotaExceededException
* The number of users exceeds the user group limit.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @sample AmazonElastiCache.CreateUserGroup
* @see AWS
* API Documentation
*/
CreateUserGroupResult createUserGroup(CreateUserGroupRequest createUserGroupRequest);
/**
*
* Decreases the number of node groups in a Global datastore
*
*
* @param decreaseNodeGroupsInGlobalReplicationGroupRequest
* @return Result of the DecreaseNodeGroupsInGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DecreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup decreaseNodeGroupsInGlobalReplicationGroup(
DecreaseNodeGroupsInGlobalReplicationGroupRequest decreaseNodeGroupsInGlobalReplicationGroupRequest);
/**
*
* Dynamically decreases the number of replicas in a Redis OSS (cluster mode disabled) replication group or the
* number of replica nodes in one or more node groups (shards) of a Redis OSS (cluster mode enabled) replication
* group. This operation is performed with no cluster down time.
*
*
* @param decreaseReplicaCountRequest
* @return Result of the DecreaseReplicaCount operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws NoOperationException
* The operation was not performed because no changes were required.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DecreaseReplicaCount
* @see AWS API Documentation
*/
ReplicationGroup decreaseReplicaCount(DecreaseReplicaCountRequest decreaseReplicaCountRequest);
/**
*
* Deletes a previously provisioned cluster. DeleteCacheCluster
deletes all associated cache nodes,
* node endpoints and the cluster itself. When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the cluster; you cannot cancel or revert this operation.
*
*
* This operation is not valid for:
*
*
* -
*
* Redis OSS (cluster mode enabled) clusters
*
*
* -
*
* Redis OSS (cluster mode disabled) clusters
*
*
* -
*
* A cluster that is the last read replica of a replication group
*
*
* -
*
* A cluster that is the primary node of a replication group
*
*
* -
*
* A node group (shard) that has Multi-AZ mode enabled
*
*
* -
*
* A cluster from a Redis OSS (cluster mode enabled) replication group
*
*
* -
*
* A cluster that is not in the available
state
*
*
*
*
* @param deleteCacheClusterRequest
* Represents the input of a DeleteCacheCluster
operation.
* @return Result of the DeleteCacheCluster operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws SnapshotFeatureNotSupportedException
* You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DeleteCacheCluster
* @see AWS
* API Documentation
*/
CacheCluster deleteCacheCluster(DeleteCacheClusterRequest deleteCacheClusterRequest);
/**
*
* Deletes the specified cache parameter group. You cannot delete a cache parameter group if it is associated with
* any cache clusters. You cannot delete the default cache parameter groups in your account.
*
*
* @param deleteCacheParameterGroupRequest
* Represents the input of a DeleteCacheParameterGroup
operation.
* @return Result of the DeleteCacheParameterGroup operation returned by the service.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DeleteCacheParameterGroup
* @see AWS API Documentation
*/
DeleteCacheParameterGroupResult deleteCacheParameterGroup(DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest);
/**
*
* Deletes a cache security group.
*
*
*
* You cannot delete a cache security group if it is associated with any clusters.
*
*
*
* @param deleteCacheSecurityGroupRequest
* Represents the input of a DeleteCacheSecurityGroup
operation.
* @return Result of the DeleteCacheSecurityGroup operation returned by the service.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DeleteCacheSecurityGroup
* @see AWS API Documentation
*/
DeleteCacheSecurityGroupResult deleteCacheSecurityGroup(DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest);
/**
*
* Deletes a cache subnet group.
*
*
*
* You cannot delete a default cache subnet group or one that is associated with any clusters.
*
*
*
* @param deleteCacheSubnetGroupRequest
* Represents the input of a DeleteCacheSubnetGroup
operation.
* @return Result of the DeleteCacheSubnetGroup operation returned by the service.
* @throws CacheSubnetGroupInUseException
* The requested cache subnet group is currently in use.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @sample AmazonElastiCache.DeleteCacheSubnetGroup
* @see AWS API Documentation
*/
DeleteCacheSubnetGroupResult deleteCacheSubnetGroup(DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest);
/**
*
* Deleting a Global datastore is a two-step process:
*
*
* -
*
* First, you must DisassociateGlobalReplicationGroup to remove the secondary clusters in the Global
* datastore.
*
*
* -
*
* Once the Global datastore contains only the primary cluster, you can use the
* DeleteGlobalReplicationGroup
API to delete the Global datastore while retainining the primary
* cluster using RetainPrimaryReplicationGroup=true
.
*
*
*
*
* Since the Global Datastore has only a primary cluster, you can delete the Global Datastore while retaining the
* primary by setting RetainPrimaryReplicationGroup=true
. The primary cluster is never deleted when
* deleting a Global Datastore. It can only be deleted when it no longer is associated with any Global Datastore.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
* @param deleteGlobalReplicationGroupRequest
* @return Result of the DeleteGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.DeleteGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup deleteGlobalReplicationGroup(DeleteGlobalReplicationGroupRequest deleteGlobalReplicationGroupRequest);
/**
*
* Deletes an existing replication group. By default, this operation deletes the entire replication group, including
* the primary/primaries and all of the read replicas. If the replication group has only one primary, you can
* optionally delete only the read replicas, while retaining the primary by setting
* RetainPrimaryCluster=true
.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
*
* -
*
* CreateSnapshot
permission is required to create a final snapshot. Without this permission, the API
* call will fail with an Access Denied
exception.
*
*
* -
*
* This operation is valid for Redis OSS only.
*
*
*
*
*
* @param deleteReplicationGroupRequest
* Represents the input of a DeleteReplicationGroup
operation.
* @return Result of the DeleteReplicationGroup operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws SnapshotFeatureNotSupportedException
* You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DeleteReplicationGroup
* @see AWS API Documentation
*/
ReplicationGroup deleteReplicationGroup(DeleteReplicationGroupRequest deleteReplicationGroupRequest);
/**
*
* Deletes a specified existing serverless cache.
*
*
*
* CreateServerlessCacheSnapshot
permission is required to create a final snapshot. Without this
* permission, the API call will fail with an Access Denied
exception.
*
*
*
* @param deleteServerlessCacheRequest
* @return Result of the DeleteServerlessCache operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotAlreadyExistsException
* A serverless cache snapshot with this name already exists. Available for Redis OSS and Serverless
* Memcached only.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidCredentialsException
* You must enter valid credentials.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @sample AmazonElastiCache.DeleteServerlessCache
* @see AWS API Documentation
*/
DeleteServerlessCacheResult deleteServerlessCache(DeleteServerlessCacheRequest deleteServerlessCacheRequest);
/**
*
* Deletes an existing serverless cache snapshot. Available for Redis OSS and Serverless Memcached only.
*
*
* @param deleteServerlessCacheSnapshotRequest
* @return Result of the DeleteServerlessCacheSnapshot operation returned by the service.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Redis OSS and Serverless
* Memcached only.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.DeleteServerlessCacheSnapshot
* @see AWS API Documentation
*/
DeleteServerlessCacheSnapshotResult deleteServerlessCacheSnapshot(DeleteServerlessCacheSnapshotRequest deleteServerlessCacheSnapshotRequest);
/**
*
* Deletes an existing snapshot. When you receive a successful response from this operation, ElastiCache immediately
* begins deleting the snapshot; you cannot cancel or revert this operation.
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* @param deleteSnapshotRequest
* Represents the input of a DeleteSnapshot
operation.
* @return Result of the DeleteSnapshot operation returned by the service.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws InvalidSnapshotStateException
* The current state of the snapshot does not allow the requested operation to occur.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DeleteSnapshot
* @see AWS API
* Documentation
*/
Snapshot deleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* For Redis OSS engine version 6.0 onwards: Deletes a user. The user will be removed from all user groups and in
* turn removed from all replication groups. For more information, see Using Role Based Access
* Control (RBAC).
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws InvalidUserStateException
* The user is not in active state.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws DefaultUserAssociatedToUserGroupException
* The default user assigned to the user group.
* @sample AmazonElastiCache.DeleteUser
* @see AWS API
* Documentation
*/
DeleteUserResult deleteUser(DeleteUserRequest deleteUserRequest);
/**
*
* For Redis OSS engine version 6.0 onwards: Deletes a user group. The user group must first be disassociated from
* the replication group before it can be deleted. For more information, see Using Role Based Access
* Control (RBAC).
*
*
* @param deleteUserGroupRequest
* @return Result of the DeleteUserGroup operation returned by the service.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.DeleteUserGroup
* @see AWS
* API Documentation
*/
DeleteUserGroupResult deleteUserGroup(DeleteUserGroupRequest deleteUserGroupRequest);
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cache cluster if a cluster identifier is supplied.
*
*
* By default, abbreviated information about the clusters is returned. You can use the optional
* ShowCacheNodeInfo flag to retrieve detailed information about the cache nodes associated with the
* clusters. These details include the DNS address and port for the cache node endpoint.
*
*
* If the cluster is in the creating state, only cluster-level information is displayed until all of the
* nodes are successfully provisioned.
*
*
* If the cluster is in the deleting state, only cluster-level information is displayed.
*
*
* If cache nodes are currently being added to the cluster, node endpoint information and creation time for the
* additional nodes are not displayed until they are completely provisioned. When the cluster state is
* available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cluster, no endpoint information for the removed nodes is
* displayed.
*
*
* @param describeCacheClustersRequest
* Represents the input of a DescribeCacheClusters
operation.
* @return Result of the DescribeCacheClusters operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeCacheClusters
* @see AWS API Documentation
*/
DescribeCacheClustersResult describeCacheClusters(DescribeCacheClustersRequest describeCacheClustersRequest);
/**
* Simplified method form for invoking the DescribeCacheClusters operation.
*
* @see #describeCacheClusters(DescribeCacheClustersRequest)
*/
DescribeCacheClustersResult describeCacheClusters();
/**
*
* Returns a list of the available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest
* Represents the input of a DescribeCacheEngineVersions
operation.
* @return Result of the DescribeCacheEngineVersions operation returned by the service.
* @sample AmazonElastiCache.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
DescribeCacheEngineVersionsResult describeCacheEngineVersions(DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest);
/**
* Simplified method form for invoking the DescribeCacheEngineVersions operation.
*
* @see #describeCacheEngineVersions(DescribeCacheEngineVersionsRequest)
*/
DescribeCacheEngineVersionsResult describeCacheEngineVersions();
/**
*
* Returns a list of cache parameter group descriptions. If a cache parameter group name is specified, the list
* contains only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest
* Represents the input of a DescribeCacheParameterGroups
operation.
* @return Result of the DescribeCacheParameterGroups operation returned by the service.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
DescribeCacheParameterGroupsResult describeCacheParameterGroups(DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest);
/**
* Simplified method form for invoking the DescribeCacheParameterGroups operation.
*
* @see #describeCacheParameterGroups(DescribeCacheParameterGroupsRequest)
*/
DescribeCacheParameterGroupsResult describeCacheParameterGroups();
/**
*
* Returns the detailed parameter list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest
* Represents the input of a DescribeCacheParameters
operation.
* @return Result of the DescribeCacheParameters operation returned by the service.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeCacheParameters
* @see AWS API Documentation
*/
DescribeCacheParametersResult describeCacheParameters(DescribeCacheParametersRequest describeCacheParametersRequest);
/**
*
* Returns a list of cache security group descriptions. If a cache security group name is specified, the list
* contains only the description of that group. This applicable only when you have ElastiCache in Classic setup
*
*
* @param describeCacheSecurityGroupsRequest
* Represents the input of a DescribeCacheSecurityGroups
operation.
* @return Result of the DescribeCacheSecurityGroups operation returned by the service.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeCacheSecurityGroups
* @see AWS API Documentation
*/
DescribeCacheSecurityGroupsResult describeCacheSecurityGroups(DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest);
/**
* Simplified method form for invoking the DescribeCacheSecurityGroups operation.
*
* @see #describeCacheSecurityGroups(DescribeCacheSecurityGroupsRequest)
*/
DescribeCacheSecurityGroupsResult describeCacheSecurityGroups();
/**
*
* Returns a list of cache subnet group descriptions. If a subnet group name is specified, the list contains only
* the description of that group. This is applicable only when you have ElastiCache in VPC setup. All ElastiCache
* clusters now launch in VPC by default.
*
*
* @param describeCacheSubnetGroupsRequest
* Represents the input of a DescribeCacheSubnetGroups
operation.
* @return Result of the DescribeCacheSubnetGroups operation returned by the service.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @sample AmazonElastiCache.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
DescribeCacheSubnetGroupsResult describeCacheSubnetGroups(DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest);
/**
* Simplified method form for invoking the DescribeCacheSubnetGroups operation.
*
* @see #describeCacheSubnetGroups(DescribeCacheSubnetGroupsRequest)
*/
DescribeCacheSubnetGroupsResult describeCacheSubnetGroups();
/**
*
* Returns the default engine and system parameter information for the specified cache engine.
*
*
* @param describeEngineDefaultParametersRequest
* Represents the input of a DescribeEngineDefaultParameters
operation.
* @return Result of the DescribeEngineDefaultParameters operation returned by the service.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
EngineDefaults describeEngineDefaultParameters(DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest);
/**
*
* Returns events related to clusters, cache security groups, and cache parameter groups. You can obtain events
* specific to a particular cluster, cache security group, or cache parameter group by providing the name as a
* parameter.
*
*
* By default, only the events occurring within the last hour are returned; however, you can retrieve up to 14 days'
* worth of events if necessary.
*
*
* @param describeEventsRequest
* Represents the input of a DescribeEvents
operation.
* @return Result of the DescribeEvents operation returned by the service.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeEvents
* @see AWS API
* Documentation
*/
DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest);
/**
* Simplified method form for invoking the DescribeEvents operation.
*
* @see #describeEvents(DescribeEventsRequest)
*/
DescribeEventsResult describeEvents();
/**
*
* Returns information about a particular global replication group. If no identifier is specified, returns
* information about all Global datastores.
*
*
* @param describeGlobalReplicationGroupsRequest
* @return Result of the DescribeGlobalReplicationGroups operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeGlobalReplicationGroups
* @see AWS API Documentation
*/
DescribeGlobalReplicationGroupsResult describeGlobalReplicationGroups(DescribeGlobalReplicationGroupsRequest describeGlobalReplicationGroupsRequest);
/**
*
* Returns information about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups
returns information about all replication groups.
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* @param describeReplicationGroupsRequest
* Represents the input of a DescribeReplicationGroups
operation.
* @return Result of the DescribeReplicationGroups operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeReplicationGroups
* @see AWS API Documentation
*/
DescribeReplicationGroupsResult describeReplicationGroups(DescribeReplicationGroupsRequest describeReplicationGroupsRequest);
/**
* Simplified method form for invoking the DescribeReplicationGroups operation.
*
* @see #describeReplicationGroups(DescribeReplicationGroupsRequest)
*/
DescribeReplicationGroupsResult describeReplicationGroups();
/**
*
* Returns information about reserved cache nodes for this account, or about a specified reserved cache node.
*
*
* @param describeReservedCacheNodesRequest
* Represents the input of a DescribeReservedCacheNodes
operation.
* @return Result of the DescribeReservedCacheNodes operation returned by the service.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
DescribeReservedCacheNodesResult describeReservedCacheNodes(DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest);
/**
* Simplified method form for invoking the DescribeReservedCacheNodes operation.
*
* @see #describeReservedCacheNodes(DescribeReservedCacheNodesRequest)
*/
DescribeReservedCacheNodesResult describeReservedCacheNodes();
/**
*
* Lists available reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest
* Represents the input of a DescribeReservedCacheNodesOfferings
operation.
* @return Result of the DescribeReservedCacheNodesOfferings operation returned by the service.
* @throws ReservedCacheNodesOfferingNotFoundException
* The requested cache node offering does not exist.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
DescribeReservedCacheNodesOfferingsResult describeReservedCacheNodesOfferings(
DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest);
/**
* Simplified method form for invoking the DescribeReservedCacheNodesOfferings operation.
*
* @see #describeReservedCacheNodesOfferings(DescribeReservedCacheNodesOfferingsRequest)
*/
DescribeReservedCacheNodesOfferingsResult describeReservedCacheNodesOfferings();
/**
*
* Returns information about serverless cache snapshots. By default, this API lists all of the customer’s serverless
* cache snapshots. It can also describe a single serverless cache snapshot, or the snapshots associated with a
* particular serverless cache. Available for Redis OSS and Serverless Memcached only.
*
*
* @param describeServerlessCacheSnapshotsRequest
* @return Result of the DescribeServerlessCacheSnapshots operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeServerlessCacheSnapshots
* @see AWS API Documentation
*/
DescribeServerlessCacheSnapshotsResult describeServerlessCacheSnapshots(DescribeServerlessCacheSnapshotsRequest describeServerlessCacheSnapshotsRequest);
/**
*
* Returns information about a specific serverless cache. If no identifier is specified, then the API returns
* information on all the serverless caches belonging to this Amazon Web Services account.
*
*
* @param describeServerlessCachesRequest
* @return Result of the DescribeServerlessCaches operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeServerlessCaches
* @see AWS API Documentation
*/
DescribeServerlessCachesResult describeServerlessCaches(DescribeServerlessCachesRequest describeServerlessCachesRequest);
/**
*
* Returns details of the service updates
*
*
* @param describeServiceUpdatesRequest
* @return Result of the DescribeServiceUpdates operation returned by the service.
* @throws ServiceUpdateNotFoundException
* The service update doesn't exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeServiceUpdates
* @see AWS API Documentation
*/
DescribeServiceUpdatesResult describeServiceUpdates(DescribeServiceUpdatesRequest describeServiceUpdatesRequest);
/**
*
* Returns information about cluster or replication group snapshots. By default, DescribeSnapshots
* lists all of your snapshots; it can optionally describe a single snapshot, or just the snapshots associated with
* a particular cache cluster.
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* @param describeSnapshotsRequest
* Represents the input of a DescribeSnapshotsMessage
operation.
* @return Result of the DescribeSnapshots operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeSnapshots
* @see AWS
* API Documentation
*/
DescribeSnapshotsResult describeSnapshots(DescribeSnapshotsRequest describeSnapshotsRequest);
/**
* Simplified method form for invoking the DescribeSnapshots operation.
*
* @see #describeSnapshots(DescribeSnapshotsRequest)
*/
DescribeSnapshotsResult describeSnapshots();
/**
*
* Returns details of the update actions
*
*
* @param describeUpdateActionsRequest
* @return Result of the DescribeUpdateActions operation returned by the service.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeUpdateActions
* @see AWS API Documentation
*/
DescribeUpdateActionsResult describeUpdateActions(DescribeUpdateActionsRequest describeUpdateActionsRequest);
/**
*
* Returns a list of user groups.
*
*
* @param describeUserGroupsRequest
* @return Result of the DescribeUserGroups operation returned by the service.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeUserGroups
* @see AWS
* API Documentation
*/
DescribeUserGroupsResult describeUserGroups(DescribeUserGroupsRequest describeUserGroupsRequest);
/**
*
* Returns a list of users.
*
*
* @param describeUsersRequest
* @return Result of the DescribeUsers operation returned by the service.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DescribeUsers
* @see AWS API
* Documentation
*/
DescribeUsersResult describeUsers(DescribeUsersRequest describeUsersRequest);
/**
*
* Remove a secondary cluster from the Global datastore using the Global datastore name. The secondary cluster will
* no longer receive updates from the primary cluster, but will remain as a standalone cluster in that Amazon
* region.
*
*
* @param disassociateGlobalReplicationGroupRequest
* @return Result of the DisassociateGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.DisassociateGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup disassociateGlobalReplicationGroup(DisassociateGlobalReplicationGroupRequest disassociateGlobalReplicationGroupRequest);
/**
*
* Provides the functionality to export the serverless cache snapshot data to Amazon S3. Available for Redis OSS
* only.
*
*
* @param exportServerlessCacheSnapshotRequest
* @return Result of the ExportServerlessCacheSnapshot operation returned by the service.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Redis OSS and Serverless
* Memcached only.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.ExportServerlessCacheSnapshot
* @see AWS API Documentation
*/
ExportServerlessCacheSnapshotResult exportServerlessCacheSnapshot(ExportServerlessCacheSnapshotRequest exportServerlessCacheSnapshotRequest);
/**
*
* Used to failover the primary region to a secondary region. The secondary region will become primary, and all
* other clusters will become secondary.
*
*
* @param failoverGlobalReplicationGroupRequest
* @return Result of the FailoverGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.FailoverGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup failoverGlobalReplicationGroup(FailoverGlobalReplicationGroupRequest failoverGlobalReplicationGroupRequest);
/**
*
* Increase the number of node groups in the Global datastore
*
*
* @param increaseNodeGroupsInGlobalReplicationGroupRequest
* @return Result of the IncreaseNodeGroupsInGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.IncreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup increaseNodeGroupsInGlobalReplicationGroup(
IncreaseNodeGroupsInGlobalReplicationGroupRequest increaseNodeGroupsInGlobalReplicationGroupRequest);
/**
*
* Dynamically increases the number of replicas in a Redis OSS (cluster mode disabled) replication group or the
* number of replica nodes in one or more node groups (shards) of a Redis OSS (cluster mode enabled) replication
* group. This operation is performed with no cluster down time.
*
*
* @param increaseReplicaCountRequest
* @return Result of the IncreaseReplicaCount operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws NoOperationException
* The operation was not performed because no changes were required.
* @throws InvalidKMSKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.IncreaseReplicaCount
* @see AWS API Documentation
*/
ReplicationGroup increaseReplicaCount(IncreaseReplicaCountRequest increaseReplicaCountRequest);
/**
*
* Lists all available node types that you can scale your Redis OSS cluster's or replication group's current node
* type.
*
*
* When you use the ModifyCacheCluster
or ModifyReplicationGroup
operations to scale your
* cluster or replication group, the value of the CacheNodeType
parameter must be one of the node types
* returned by this operation.
*
*
* @param listAllowedNodeTypeModificationsRequest
* The input parameters for the ListAllowedNodeTypeModifications
operation.
* @return Result of the ListAllowedNodeTypeModifications operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.ListAllowedNodeTypeModifications
* @see AWS API Documentation
*/
ListAllowedNodeTypeModificationsResult listAllowedNodeTypeModifications(ListAllowedNodeTypeModificationsRequest listAllowedNodeTypeModificationsRequest);
/**
* Simplified method form for invoking the ListAllowedNodeTypeModifications operation.
*
* @see #listAllowedNodeTypeModifications(ListAllowedNodeTypeModificationsRequest)
*/
ListAllowedNodeTypeModificationsResult listAllowedNodeTypeModifications();
/**
*
* Lists all tags currently on a named resource.
*
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource
* -level permissions.
*
*
* If the cluster is not in the available state, ListTagsForResource
returns an error.
*
*
* @param listTagsForResourceRequest
* The input parameters for the ListTagsForResource
operation.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Redis OSS and Serverless
* Memcached only.
* @throws InvalidARNException
* The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* @sample AmazonElastiCache.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Modifies the settings for a cluster. You can use this operation to change one or more cluster configuration
* parameters by specifying the parameters and the new values.
*
*
* @param modifyCacheClusterRequest
* Represents the input of a ModifyCacheCluster
operation.
* @return Result of the ModifyCacheCluster operation returned by the service.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.ModifyCacheCluster
* @see AWS
* API Documentation
*/
CacheCluster modifyCacheCluster(ModifyCacheClusterRequest modifyCacheClusterRequest);
/**
*
* Modifies the parameters of a cache parameter group. You can modify up to 20 parameters in a single request by
* submitting a list parameter name and value pairs.
*
*
* @param modifyCacheParameterGroupRequest
* Represents the input of a ModifyCacheParameterGroup
operation.
* @return Result of the ModifyCacheParameterGroup operation returned by the service.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @sample AmazonElastiCache.ModifyCacheParameterGroup
* @see AWS API Documentation
*/
ModifyCacheParameterGroupResult modifyCacheParameterGroup(ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest);
/**
*
* Modifies an existing cache subnet group.
*
*
* @param modifyCacheSubnetGroupRequest
* Represents the input of a ModifyCacheSubnetGroup
operation.
* @return Result of the ModifyCacheSubnetGroup operation returned by the service.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws CacheSubnetQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a cache subnet
* group.
* @throws SubnetInUseException
* The requested subnet is being used by another cache subnet group.
* @throws InvalidSubnetException
* An invalid subnet identifier was specified.
* @throws SubnetNotAllowedException
* At least one subnet ID does not match the other subnet IDs. This mismatch typically occurs when a user
* sets one subnet ID to a regional Availability Zone and a different one to an outpost. Or when a user sets
* the subnet ID to an Outpost when not subscribed on this service.
* @sample AmazonElastiCache.ModifyCacheSubnetGroup
* @see AWS API Documentation
*/
CacheSubnetGroup modifyCacheSubnetGroup(ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest);
/**
*
* Modifies the settings for a Global datastore.
*
*
* @param modifyGlobalReplicationGroupRequest
* @return Result of the ModifyGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.ModifyGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup modifyGlobalReplicationGroup(ModifyGlobalReplicationGroupRequest modifyGlobalReplicationGroupRequest);
/**
*
* Modifies the settings for a replication group. This is limited to Redis OSS 7 and newer.
*
*
* -
*
*
* Scaling for Amazon ElastiCache (Redis OSS) (cluster mode enabled) in the ElastiCache User Guide
*
*
* -
*
* ModifyReplicationGroupShardConfiguration in the ElastiCache API Reference
*
*
*
*
*
* This operation is valid for Redis OSS only.
*
*
*
* @param modifyReplicationGroupRequest
* Represents the input of a ModifyReplicationGroups
operation.
* @return Result of the ModifyReplicationGroup operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws InvalidKMSKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.ModifyReplicationGroup
* @see AWS API Documentation
*/
ReplicationGroup modifyReplicationGroup(ModifyReplicationGroupRequest modifyReplicationGroupRequest);
/**
*
* Modifies a replication group's shards (node groups) by allowing you to add shards, remove shards, or rebalance
* the keyspaces among existing shards.
*
*
* @param modifyReplicationGroupShardConfigurationRequest
* Represents the input for a ModifyReplicationGroupShardConfiguration
operation.
* @return Result of the ModifyReplicationGroupShardConfiguration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidVPCNetworkStateException
* The VPC network is in an invalid state.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws InvalidKMSKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.ModifyReplicationGroupShardConfiguration
* @see AWS API Documentation
*/
ReplicationGroup modifyReplicationGroupShardConfiguration(ModifyReplicationGroupShardConfigurationRequest modifyReplicationGroupShardConfigurationRequest);
/**
*
* This API modifies the attributes of a serverless cache.
*
*
* @param modifyServerlessCacheRequest
* @return Result of the ModifyServerlessCache operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidCredentialsException
* You must enter valid credentials.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @sample AmazonElastiCache.ModifyServerlessCache
* @see AWS API Documentation
*/
ModifyServerlessCacheResult modifyServerlessCache(ModifyServerlessCacheRequest modifyServerlessCacheRequest);
/**
*
* Changes user password(s) and/or access string.
*
*
* @param modifyUserRequest
* @return Result of the ModifyUser operation returned by the service.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws InvalidUserStateException
* The user is not in active state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.ModifyUser
* @see AWS API
* Documentation
*/
ModifyUserResult modifyUser(ModifyUserRequest modifyUserRequest);
/**
*
* Changes the list of users that belong to the user group.
*
*
* @param modifyUserGroupRequest
* @return Result of the ModifyUserGroup operation returned by the service.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws DuplicateUserNameException
* A user with this username already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws DefaultUserRequiredException
* You must add default user to a user group.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.ModifyUserGroup
* @see AWS
* API Documentation
*/
ModifyUserGroupResult modifyUserGroup(ModifyUserGroupRequest modifyUserGroupRequest);
/**
*
* Allows you to purchase a reserved cache node offering. Reserved nodes are not eligible for cancellation and are
* non-refundable. For more information, see Managing Costs with
* Reserved Nodes for Redis OSS or Managing Costs with
* Reserved Nodes for Memcached.
*
*
* @param purchaseReservedCacheNodesOfferingRequest
* Represents the input of a PurchaseReservedCacheNodesOffering
operation.
* @return Result of the PurchaseReservedCacheNodesOffering operation returned by the service.
* @throws ReservedCacheNodesOfferingNotFoundException
* The requested cache node offering does not exist.
* @throws ReservedCacheNodeAlreadyExistsException
* You already have a reservation with the given identifier.
* @throws ReservedCacheNodeQuotaExceededException
* The request cannot be processed because it would exceed the user's cache node quota.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.PurchaseReservedCacheNodesOffering
* @see AWS API Documentation
*/
ReservedCacheNode purchaseReservedCacheNodesOffering(PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest);
/**
*
* Redistribute slots to ensure uniform distribution across existing shards in the cluster.
*
*
* @param rebalanceSlotsInGlobalReplicationGroupRequest
* @return Result of the RebalanceSlotsInGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.RebalanceSlotsInGlobalReplicationGroup
* @see AWS API Documentation
*/
GlobalReplicationGroup rebalanceSlotsInGlobalReplicationGroup(RebalanceSlotsInGlobalReplicationGroupRequest rebalanceSlotsInGlobalReplicationGroupRequest);
/**
*
* Reboots some, or all, of the cache nodes within a provisioned cluster. This operation applies any modified cache
* parameter groups to the cluster. The reboot operation takes place as soon as possible, and results in a momentary
* outage to the cluster. During the reboot, the cluster status is set to REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node being rebooted) to be lost.
*
*
* When the reboot is complete, a cluster event is created.
*
*
* Rebooting a cluster is currently supported on Memcached and Redis OSS (cluster mode disabled) clusters. Rebooting
* is not supported on Redis OSS (cluster mode enabled) clusters.
*
*
* If you make changes to parameters that require a Redis OSS (cluster mode enabled) cluster reboot for the changes
* to be applied, see Rebooting a Cluster
* for an alternate process.
*
*
* @param rebootCacheClusterRequest
* Represents the input of a RebootCacheCluster
operation.
* @return Result of the RebootCacheCluster operation returned by the service.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @sample AmazonElastiCache.RebootCacheCluster
* @see AWS
* API Documentation
*/
CacheCluster rebootCacheCluster(RebootCacheClusterRequest rebootCacheClusterRequest);
/**
*
* Removes the tags identified by the TagKeys
list from the named resource. A tag is a key-value pair
* where the key and value are case-sensitive. You can use tags to categorize and track all your ElastiCache
* resources, with the exception of global replication group. When you add or remove tags on replication groups,
* those actions will be replicated to all nodes in the replication group. For more information, see Resource-level permissions.
*
*
* @param removeTagsFromResourceRequest
* Represents the input of a RemoveTagsFromResource
operation.
* @return Result of the RemoveTagsFromResource operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Redis OSS and Serverless
* Memcached only.
* @throws InvalidARNException
* The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* @throws TagNotFoundException
* The requested tag was not found on this resource.
* @sample AmazonElastiCache.RemoveTagsFromResource
* @see AWS API Documentation
*/
RemoveTagsFromResourceResult removeTagsFromResource(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Modifies the parameters of a cache parameter group to the engine or system default value. You can reset specific
* parameters by submitting a list of parameter names. To reset the entire cache parameter group, specify the
* ResetAllParameters
and CacheParameterGroupName
parameters.
*
*
* @param resetCacheParameterGroupRequest
* Represents the input of a ResetCacheParameterGroup
operation.
* @return Result of the ResetCacheParameterGroup operation returned by the service.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @sample AmazonElastiCache.ResetCacheParameterGroup
* @see AWS API Documentation
*/
ResetCacheParameterGroupResult resetCacheParameterGroup(ResetCacheParameterGroupRequest resetCacheParameterGroupRequest);
/**
*
* Revokes ingress from a cache security group. Use this operation to disallow access from an Amazon EC2 security
* group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest
* Represents the input of a RevokeCacheSecurityGroupIngress
operation.
* @return Result of the RevokeCacheSecurityGroupIngress operation returned by the service.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws AuthorizationNotFoundException
* The specified Amazon EC2 security group is not authorized for the specified cache security group.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.RevokeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
CacheSecurityGroup revokeCacheSecurityGroupIngress(RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest);
/**
*
* Start the migration of data.
*
*
* @param startMigrationRequest
* @return Result of the StartMigration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupAlreadyUnderMigrationException
* The targeted replication group is not available.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.StartMigration
* @see AWS API
* Documentation
*/
ReplicationGroup startMigration(StartMigrationRequest startMigrationRequest);
/**
*
* Represents the input of a TestFailover
operation which tests automatic failover on a specified node
* group (called shard in the console) in a replication group (called cluster in the console).
*
*
* This API is designed for testing the behavior of your application in case of ElastiCache failover. It is not
* designed to be an operational tool for initiating a failover to overcome a problem you may have with the cluster.
* Moreover, in certain conditions such as large-scale operational events, Amazon may block this API.
*
*
* Note the following
*
*
* -
*
* A customer can use this operation to test automatic failover on up to 15 shards (called node groups in the
* ElastiCache API and Amazon CLI) in any rolling 24-hour period.
*
*
* -
*
* If calling this operation on shards in different clusters (called replication groups in the API and CLI), the
* calls can be made concurrently.
*
*
*
* -
*
* If calling this operation multiple times on different shards in the same Redis OSS (cluster mode enabled)
* replication group, the first node replacement must complete before a subsequent call can be made.
*
*
* -
*
* To determine whether the node replacement is complete you can check Events using the Amazon ElastiCache console,
* the Amazon CLI, or the ElastiCache API. Look for the following automatic failover related events, listed here in
* order of occurrance:
*
*
* -
*
* Replication group message: Test Failover API called for node group <node-group-id>
*
*
* -
*
* Cache cluster message:
* Failover from primary node <primary-node-id> to replica node <node-id> completed
*
*
* -
*
* Replication group message:
* Failover from primary node <primary-node-id> to replica node <node-id> completed
*
*
* -
*
* Cache cluster message: Recovering cache nodes <node-id>
*
*
* -
*
* Cache cluster message: Finished recovery for cache nodes <node-id>
*
*
*
*
* For more information see:
*
*
* -
*
* Viewing ElastiCache
* Events in the ElastiCache User Guide
*
*
* -
*
* DescribeEvents
* in the ElastiCache API Reference
*
*
*
*
*
*
* Also see, Testing
* Multi-AZ in the ElastiCache User Guide.
*
*
* @param testFailoverRequest
* @return Result of the TestFailover operation returned by the service.
* @throws APICallRateForCustomerExceededException
* The customer has exceeded the allowed rate of API calls.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws NodeGroupNotFoundException
* The node group specified by the NodeGroupId
parameter could not be found. Please verify that
* the node group exists and that you spelled the NodeGroupId
value correctly.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws TestFailoverNotAvailableException
* The TestFailover
action is not available.
* @throws InvalidKMSKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @sample AmazonElastiCache.TestFailover
* @see AWS API
* Documentation
*/
ReplicationGroup testFailover(TestFailoverRequest testFailoverRequest);
/**
*
* Async API to test connection between source and target replication group.
*
*
* @param testMigrationRequest
* @return Result of the TestMigration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupAlreadyUnderMigrationException
* The targeted replication group is not available.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @sample AmazonElastiCache.TestMigration
* @see AWS API
* Documentation
*/
ReplicationGroup testMigration(TestMigrationRequest testMigrationRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonElastiCacheWaiters waiters();
}