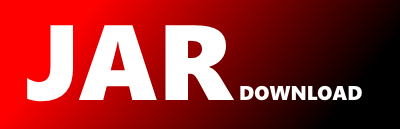
com.amazonaws.services.elasticache.model.ModifyCacheClusterRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input of a ModifyCacheCluster
operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyCacheClusterRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The cluster identifier. This value is stored as a lowercase string.
*
*/
private String cacheClusterId;
/**
*
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is greater
* than the sum of the number of current cache nodes and the number of cache nodes pending creation (which may be
* zero), more nodes are added. If the value is less than the number of existing cache nodes, nodes are removed. If
* the value is equal to the number of current cache nodes, any pending add or remove requests are canceled.
*
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide the IDs
* of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window, whether by
* adding or removing nodes in accordance with the scale out architecture, is not queued. The customer's latest
* request to add or remove nodes to the cluster overrides any previous pending operations to modify the number of
* cache nodes in the cluster. For example, a request to remove 2 nodes would override a previous pending operation
* to remove 3 nodes. Similarly, a request to add 2 nodes would override a previous pending operation to remove 3
* nodes and vice versa. As Memcached cache nodes may now be provisioned in different Availability Zones with
* flexible cache node placement, a request to add nodes does not automatically override a previous pending
* operation to add nodes. The customer can modify the previous pending operation to add more nodes or explicitly
* cancel the pending request and retry the new request. To cancel pending operations to modify the number of cache
* nodes in a cluster, use the ModifyCacheCluster
request and set NumCacheNodes
equal to
* the number of cache nodes currently in the cluster.
*
*
*/
private Integer numCacheNodes;
/**
*
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This parameter is
* only valid when NumCacheNodes
is less than the existing number of cache nodes. The number of cache
* node IDs supplied in this parameter must match the difference between the existing number of cache nodes in the
* cluster or pending cache nodes, whichever is greater, and the value of NumCacheNodes
in the request.
*
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in this
* ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*
*/
private com.amazonaws.internal.SdkInternalList cacheNodeIdsToRemove;
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*/
private String aZMode;
/**
*
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the number
* of active cache nodes and the number of cache nodes pending creation (which may be zero). The number of
* Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
(3 + 2)
* and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and want to
* add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an Availability Zone
* for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to cancel all
* pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any nodes
* pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone. Only
* newly created nodes can be located in different Availability Zones. For guidance on how to move existing
* Memcached nodes to different Availability Zones, see the Availability Zone Considerations section of Cache Node
* Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*
*/
private com.amazonaws.internal.SdkInternalList newAvailabilityZones;
/**
*
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*
*/
private com.amazonaws.internal.SdkInternalList cacheSecurityGroupNames;
/**
*
* Specifies the VPC Security Groups associated with the cluster.
*
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*/
private com.amazonaws.internal.SdkInternalList securityGroupIds;
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*/
private String preferredMaintenanceWindow;
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
*
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*
*/
private String notificationTopicArn;
/**
*
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as soon as
* possible for parameters when the ApplyImmediately
parameter is specified as true
for
* this request.
*
*/
private String cacheParameterGroupName;
/**
*
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is active
* .
*
*
* Valid values: active
| inactive
*
*/
private String notificationTopicStatus;
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the cluster.
*
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next failure
* reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*
*/
private Boolean applyImmediately;
/**
*
* The upgraded version of the cache engine to be run on the cache nodes.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster and create it anew with the earlier engine version.
*
*/
private String engineVersion;
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*/
private Boolean autoMinorVersionUpgrade;
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*/
private Integer snapshotRetentionLimit;
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*/
private String snapshotWindow;
/**
*
* A valid cache node type that you want to scale this cluster up to.
*
*/
private String cacheNodeType;
/**
*
* Reserved parameter. The password used to access a password protected server. This parameter must be specified
* with the auth-token-update
parameter. Password constraints:
*
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*
*/
private String authToken;
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with Redis OSS
* AUTH
*
*/
private String authTokenUpdateStrategy;
/**
*
* Specifies the destination, format and type of the logs.
*
*/
private com.amazonaws.internal.SdkInternalList logDeliveryConfigurations;
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*/
private String ipDiscovery;
/**
* Default constructor for ModifyCacheClusterRequest object. Callers should use the setter or fluent setter
* (with...) methods to initialize the object after creating it.
*/
public ModifyCacheClusterRequest() {
}
/**
* Constructs a new ModifyCacheClusterRequest object. Callers should use the setter or fluent setter (with...)
* methods to initialize any additional object members.
*
* @param cacheClusterId
* The cluster identifier. This value is stored as a lowercase string.
*/
public ModifyCacheClusterRequest(String cacheClusterId) {
setCacheClusterId(cacheClusterId);
}
/**
*
* The cluster identifier. This value is stored as a lowercase string.
*
*
* @param cacheClusterId
* The cluster identifier. This value is stored as a lowercase string.
*/
public void setCacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
}
/**
*
* The cluster identifier. This value is stored as a lowercase string.
*
*
* @return The cluster identifier. This value is stored as a lowercase string.
*/
public String getCacheClusterId() {
return this.cacheClusterId;
}
/**
*
* The cluster identifier. This value is stored as a lowercase string.
*
*
* @param cacheClusterId
* The cluster identifier. This value is stored as a lowercase string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheClusterId(String cacheClusterId) {
setCacheClusterId(cacheClusterId);
return this;
}
/**
*
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is greater
* than the sum of the number of current cache nodes and the number of cache nodes pending creation (which may be
* zero), more nodes are added. If the value is less than the number of existing cache nodes, nodes are removed. If
* the value is equal to the number of current cache nodes, any pending add or remove requests are canceled.
*
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide the IDs
* of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window, whether by
* adding or removing nodes in accordance with the scale out architecture, is not queued. The customer's latest
* request to add or remove nodes to the cluster overrides any previous pending operations to modify the number of
* cache nodes in the cluster. For example, a request to remove 2 nodes would override a previous pending operation
* to remove 3 nodes. Similarly, a request to add 2 nodes would override a previous pending operation to remove 3
* nodes and vice versa. As Memcached cache nodes may now be provisioned in different Availability Zones with
* flexible cache node placement, a request to add nodes does not automatically override a previous pending
* operation to add nodes. The customer can modify the previous pending operation to add more nodes or explicitly
* cancel the pending request and retry the new request. To cancel pending operations to modify the number of cache
* nodes in a cluster, use the ModifyCacheCluster
request and set NumCacheNodes
equal to
* the number of cache nodes currently in the cluster.
*
*
*
* @param numCacheNodes
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is
* greater than the sum of the number of current cache nodes and the number of cache nodes pending creation
* (which may be zero), more nodes are added. If the value is less than the number of existing cache nodes,
* nodes are removed. If the value is equal to the number of current cache nodes, any pending add or remove
* requests are canceled.
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide
* the IDs of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window,
* whether by adding or removing nodes in accordance with the scale out architecture, is not queued. The
* customer's latest request to add or remove nodes to the cluster overrides any previous pending operations
* to modify the number of cache nodes in the cluster. For example, a request to remove 2 nodes would
* override a previous pending operation to remove 3 nodes. Similarly, a request to add 2 nodes would
* override a previous pending operation to remove 3 nodes and vice versa. As Memcached cache nodes may now
* be provisioned in different Availability Zones with flexible cache node placement, a request to add nodes
* does not automatically override a previous pending operation to add nodes. The customer can modify the
* previous pending operation to add more nodes or explicitly cancel the pending request and retry the new
* request. To cancel pending operations to modify the number of cache nodes in a cluster, use the
* ModifyCacheCluster
request and set NumCacheNodes
equal to the number of cache
* nodes currently in the cluster.
*
*/
public void setNumCacheNodes(Integer numCacheNodes) {
this.numCacheNodes = numCacheNodes;
}
/**
*
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is greater
* than the sum of the number of current cache nodes and the number of cache nodes pending creation (which may be
* zero), more nodes are added. If the value is less than the number of existing cache nodes, nodes are removed. If
* the value is equal to the number of current cache nodes, any pending add or remove requests are canceled.
*
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide the IDs
* of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window, whether by
* adding or removing nodes in accordance with the scale out architecture, is not queued. The customer's latest
* request to add or remove nodes to the cluster overrides any previous pending operations to modify the number of
* cache nodes in the cluster. For example, a request to remove 2 nodes would override a previous pending operation
* to remove 3 nodes. Similarly, a request to add 2 nodes would override a previous pending operation to remove 3
* nodes and vice versa. As Memcached cache nodes may now be provisioned in different Availability Zones with
* flexible cache node placement, a request to add nodes does not automatically override a previous pending
* operation to add nodes. The customer can modify the previous pending operation to add more nodes or explicitly
* cancel the pending request and retry the new request. To cancel pending operations to modify the number of cache
* nodes in a cluster, use the ModifyCacheCluster
request and set NumCacheNodes
equal to
* the number of cache nodes currently in the cluster.
*
*
*
* @return The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is
* greater than the sum of the number of current cache nodes and the number of cache nodes pending creation
* (which may be zero), more nodes are added. If the value is less than the number of existing cache nodes,
* nodes are removed. If the value is equal to the number of current cache nodes, any pending add or remove
* requests are canceled.
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide
* the IDs of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window,
* whether by adding or removing nodes in accordance with the scale out architecture, is not queued. The
* customer's latest request to add or remove nodes to the cluster overrides any previous pending operations
* to modify the number of cache nodes in the cluster. For example, a request to remove 2 nodes would
* override a previous pending operation to remove 3 nodes. Similarly, a request to add 2 nodes would
* override a previous pending operation to remove 3 nodes and vice versa. As Memcached cache nodes may now
* be provisioned in different Availability Zones with flexible cache node placement, a request to add nodes
* does not automatically override a previous pending operation to add nodes. The customer can modify the
* previous pending operation to add more nodes or explicitly cancel the pending request and retry the new
* request. To cancel pending operations to modify the number of cache nodes in a cluster, use the
* ModifyCacheCluster
request and set NumCacheNodes
equal to the number of cache
* nodes currently in the cluster.
*
*/
public Integer getNumCacheNodes() {
return this.numCacheNodes;
}
/**
*
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is greater
* than the sum of the number of current cache nodes and the number of cache nodes pending creation (which may be
* zero), more nodes are added. If the value is less than the number of existing cache nodes, nodes are removed. If
* the value is equal to the number of current cache nodes, any pending add or remove requests are canceled.
*
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide the IDs
* of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window, whether by
* adding or removing nodes in accordance with the scale out architecture, is not queued. The customer's latest
* request to add or remove nodes to the cluster overrides any previous pending operations to modify the number of
* cache nodes in the cluster. For example, a request to remove 2 nodes would override a previous pending operation
* to remove 3 nodes. Similarly, a request to add 2 nodes would override a previous pending operation to remove 3
* nodes and vice versa. As Memcached cache nodes may now be provisioned in different Availability Zones with
* flexible cache node placement, a request to add nodes does not automatically override a previous pending
* operation to add nodes. The customer can modify the previous pending operation to add more nodes or explicitly
* cancel the pending request and retry the new request. To cancel pending operations to modify the number of cache
* nodes in a cluster, use the ModifyCacheCluster
request and set NumCacheNodes
equal to
* the number of cache nodes currently in the cluster.
*
*
*
* @param numCacheNodes
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is
* greater than the sum of the number of current cache nodes and the number of cache nodes pending creation
* (which may be zero), more nodes are added. If the value is less than the number of existing cache nodes,
* nodes are removed. If the value is equal to the number of current cache nodes, any pending add or remove
* requests are canceled.
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide
* the IDs of the specific cache nodes to remove.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window,
* whether by adding or removing nodes in accordance with the scale out architecture, is not queued. The
* customer's latest request to add or remove nodes to the cluster overrides any previous pending operations
* to modify the number of cache nodes in the cluster. For example, a request to remove 2 nodes would
* override a previous pending operation to remove 3 nodes. Similarly, a request to add 2 nodes would
* override a previous pending operation to remove 3 nodes and vice versa. As Memcached cache nodes may now
* be provisioned in different Availability Zones with flexible cache node placement, a request to add nodes
* does not automatically override a previous pending operation to add nodes. The customer can modify the
* previous pending operation to add more nodes or explicitly cancel the pending request and retry the new
* request. To cancel pending operations to modify the number of cache nodes in a cluster, use the
* ModifyCacheCluster
request and set NumCacheNodes
equal to the number of cache
* nodes currently in the cluster.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withNumCacheNodes(Integer numCacheNodes) {
setNumCacheNodes(numCacheNodes);
return this;
}
/**
*
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This parameter is
* only valid when NumCacheNodes
is less than the existing number of cache nodes. The number of cache
* node IDs supplied in this parameter must match the difference between the existing number of cache nodes in the
* cluster or pending cache nodes, whichever is greater, and the value of NumCacheNodes
in the request.
*
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in this
* ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*
*
* @return A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This
* parameter is only valid when NumCacheNodes
is less than the existing number of cache nodes.
* The number of cache node IDs supplied in this parameter must match the difference between the existing
* number of cache nodes in the cluster or pending cache nodes, whichever is greater, and the value of
* NumCacheNodes
in the request.
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in
* this ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*/
public java.util.List getCacheNodeIdsToRemove() {
if (cacheNodeIdsToRemove == null) {
cacheNodeIdsToRemove = new com.amazonaws.internal.SdkInternalList();
}
return cacheNodeIdsToRemove;
}
/**
*
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This parameter is
* only valid when NumCacheNodes
is less than the existing number of cache nodes. The number of cache
* node IDs supplied in this parameter must match the difference between the existing number of cache nodes in the
* cluster or pending cache nodes, whichever is greater, and the value of NumCacheNodes
in the request.
*
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in this
* ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*
*
* @param cacheNodeIdsToRemove
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This
* parameter is only valid when NumCacheNodes
is less than the existing number of cache nodes.
* The number of cache node IDs supplied in this parameter must match the difference between the existing
* number of cache nodes in the cluster or pending cache nodes, whichever is greater, and the value of
* NumCacheNodes
in the request.
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in
* this ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*/
public void setCacheNodeIdsToRemove(java.util.Collection cacheNodeIdsToRemove) {
if (cacheNodeIdsToRemove == null) {
this.cacheNodeIdsToRemove = null;
return;
}
this.cacheNodeIdsToRemove = new com.amazonaws.internal.SdkInternalList(cacheNodeIdsToRemove);
}
/**
*
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This parameter is
* only valid when NumCacheNodes
is less than the existing number of cache nodes. The number of cache
* node IDs supplied in this parameter must match the difference between the existing number of cache nodes in the
* cluster or pending cache nodes, whichever is greater, and the value of NumCacheNodes
in the request.
*
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in this
* ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCacheNodeIdsToRemove(java.util.Collection)} or {@link #withCacheNodeIdsToRemove(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param cacheNodeIdsToRemove
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This
* parameter is only valid when NumCacheNodes
is less than the existing number of cache nodes.
* The number of cache node IDs supplied in this parameter must match the difference between the existing
* number of cache nodes in the cluster or pending cache nodes, whichever is greater, and the value of
* NumCacheNodes
in the request.
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in
* this ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheNodeIdsToRemove(String... cacheNodeIdsToRemove) {
if (this.cacheNodeIdsToRemove == null) {
setCacheNodeIdsToRemove(new com.amazonaws.internal.SdkInternalList(cacheNodeIdsToRemove.length));
}
for (String ele : cacheNodeIdsToRemove) {
this.cacheNodeIdsToRemove.add(ele);
}
return this;
}
/**
*
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This parameter is
* only valid when NumCacheNodes
is less than the existing number of cache nodes. The number of cache
* node IDs supplied in this parameter must match the difference between the existing number of cache nodes in the
* cluster or pending cache nodes, whichever is greater, and the value of NumCacheNodes
in the request.
*
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in this
* ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*
*
* @param cacheNodeIdsToRemove
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This
* parameter is only valid when NumCacheNodes
is less than the existing number of cache nodes.
* The number of cache node IDs supplied in this parameter must match the difference between the existing
* number of cache nodes in the cluster or pending cache nodes, whichever is greater, and the value of
* NumCacheNodes
in the request.
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in
* this ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheNodeIdsToRemove(java.util.Collection cacheNodeIdsToRemove) {
setCacheNodeIdsToRemove(cacheNodeIdsToRemove);
return this;
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* @param aZMode
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @see AZMode
*/
public void setAZMode(String aZMode) {
this.aZMode = aZMode;
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* @return Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone
* or created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @see AZMode
*/
public String getAZMode() {
return this.aZMode;
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* @param aZMode
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AZMode
*/
public ModifyCacheClusterRequest withAZMode(String aZMode) {
setAZMode(aZMode);
return this;
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* @param aZMode
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @see AZMode
*/
public void setAZMode(AZMode aZMode) {
withAZMode(aZMode);
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* @param aZMode
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AZMode
*/
public ModifyCacheClusterRequest withAZMode(AZMode aZMode) {
this.aZMode = aZMode.toString();
return this;
}
/**
*
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the number
* of active cache nodes and the number of cache nodes pending creation (which may be zero). The number of
* Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
(3 + 2)
* and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and want to
* add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an Availability Zone
* for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to cancel all
* pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any nodes
* pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone. Only
* newly created nodes can be located in different Availability Zones. For guidance on how to move existing
* Memcached nodes to different Availability Zones, see the Availability Zone Considerations section of Cache Node
* Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*
*
* @return
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of
* the number of active cache nodes and the number of cache nodes pending creation (which may be zero). The
* number of Availability Zones supplied in this list must match the cache nodes being added in this
* request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
* (3 + 2) and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and
* want to add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an
* Availability Zone for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to
* cancel all pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any
* nodes pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability
* Zone. Only newly created nodes can be located in different Availability Zones. For guidance on how to
* move existing Memcached nodes to different Availability Zones, see the Availability Zone
* Considerations section of Cache
* Node Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*/
public java.util.List getNewAvailabilityZones() {
if (newAvailabilityZones == null) {
newAvailabilityZones = new com.amazonaws.internal.SdkInternalList();
}
return newAvailabilityZones;
}
/**
*
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the number
* of active cache nodes and the number of cache nodes pending creation (which may be zero). The number of
* Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
(3 + 2)
* and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and want to
* add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an Availability Zone
* for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to cancel all
* pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any nodes
* pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone. Only
* newly created nodes can be located in different Availability Zones. For guidance on how to move existing
* Memcached nodes to different Availability Zones, see the Availability Zone Considerations section of Cache Node
* Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*
*
* @param newAvailabilityZones
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the
* number of active cache nodes and the number of cache nodes pending creation (which may be zero). The
* number of Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
* (3 + 2) and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and
* want to add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an
* Availability Zone for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to
* cancel all pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any
* nodes pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone.
* Only newly created nodes can be located in different Availability Zones. For guidance on how to move
* existing Memcached nodes to different Availability Zones, see the Availability Zone Considerations
* section of Cache
* Node Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*/
public void setNewAvailabilityZones(java.util.Collection newAvailabilityZones) {
if (newAvailabilityZones == null) {
this.newAvailabilityZones = null;
return;
}
this.newAvailabilityZones = new com.amazonaws.internal.SdkInternalList(newAvailabilityZones);
}
/**
*
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the number
* of active cache nodes and the number of cache nodes pending creation (which may be zero). The number of
* Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
(3 + 2)
* and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and want to
* add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an Availability Zone
* for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to cancel all
* pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any nodes
* pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone. Only
* newly created nodes can be located in different Availability Zones. For guidance on how to move existing
* Memcached nodes to different Availability Zones, see the Availability Zone Considerations section of Cache Node
* Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNewAvailabilityZones(java.util.Collection)} or {@link #withNewAvailabilityZones(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param newAvailabilityZones
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the
* number of active cache nodes and the number of cache nodes pending creation (which may be zero). The
* number of Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
* (3 + 2) and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and
* want to add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an
* Availability Zone for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to
* cancel all pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any
* nodes pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone.
* Only newly created nodes can be located in different Availability Zones. For guidance on how to move
* existing Memcached nodes to different Availability Zones, see the Availability Zone Considerations
* section of Cache
* Node Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withNewAvailabilityZones(String... newAvailabilityZones) {
if (this.newAvailabilityZones == null) {
setNewAvailabilityZones(new com.amazonaws.internal.SdkInternalList(newAvailabilityZones.length));
}
for (String ele : newAvailabilityZones) {
this.newAvailabilityZones.add(ele);
}
return this;
}
/**
*
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the number
* of active cache nodes and the number of cache nodes pending creation (which may be zero). The number of
* Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
(3 + 2)
* and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and want to
* add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an Availability Zone
* for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to cancel all
* pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any nodes
* pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone. Only
* newly created nodes can be located in different Availability Zones. For guidance on how to move existing
* Memcached nodes to different Availability Zones, see the Availability Zone Considerations section of Cache Node
* Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*
*
* @param newAvailabilityZones
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the
* number of active cache nodes and the number of cache nodes pending creation (which may be zero). The
* number of Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
* (3 + 2) and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and
* want to add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an
* Availability Zone for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to
* cancel all pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any
* nodes pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone.
* Only newly created nodes can be located in different Availability Zones. For guidance on how to move
* existing Memcached nodes to different Availability Zones, see the Availability Zone Considerations
* section of Cache
* Node Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withNewAvailabilityZones(java.util.Collection newAvailabilityZones) {
setNewAvailabilityZones(newAvailabilityZones);
return this;
}
/**
*
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*
*
* @return A list of cache security group names to authorize on this cluster. This change is asynchronously applied
* as soon as possible.
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*/
public java.util.List getCacheSecurityGroupNames() {
if (cacheSecurityGroupNames == null) {
cacheSecurityGroupNames = new com.amazonaws.internal.SdkInternalList();
}
return cacheSecurityGroupNames;
}
/**
*
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*
*
* @param cacheSecurityGroupNames
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied
* as soon as possible.
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*/
public void setCacheSecurityGroupNames(java.util.Collection cacheSecurityGroupNames) {
if (cacheSecurityGroupNames == null) {
this.cacheSecurityGroupNames = null;
return;
}
this.cacheSecurityGroupNames = new com.amazonaws.internal.SdkInternalList(cacheSecurityGroupNames);
}
/**
*
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCacheSecurityGroupNames(java.util.Collection)} or
* {@link #withCacheSecurityGroupNames(java.util.Collection)} if you want to override the existing values.
*
*
* @param cacheSecurityGroupNames
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied
* as soon as possible.
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheSecurityGroupNames(String... cacheSecurityGroupNames) {
if (this.cacheSecurityGroupNames == null) {
setCacheSecurityGroupNames(new com.amazonaws.internal.SdkInternalList(cacheSecurityGroupNames.length));
}
for (String ele : cacheSecurityGroupNames) {
this.cacheSecurityGroupNames.add(ele);
}
return this;
}
/**
*
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*
*
* @param cacheSecurityGroupNames
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied
* as soon as possible.
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheSecurityGroupNames(java.util.Collection cacheSecurityGroupNames) {
setCacheSecurityGroupNames(cacheSecurityGroupNames);
return this;
}
/**
*
* Specifies the VPC Security Groups associated with the cluster.
*
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @return Specifies the VPC Security Groups associated with the cluster.
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon
* VPC).
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return securityGroupIds;
}
/**
*
* Specifies the VPC Security Groups associated with the cluster.
*
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param securityGroupIds
* Specifies the VPC Security Groups associated with the cluster.
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon
* VPC).
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new com.amazonaws.internal.SdkInternalList(securityGroupIds);
}
/**
*
* Specifies the VPC Security Groups associated with the cluster.
*
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* Specifies the VPC Security Groups associated with the cluster.
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon
* VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new com.amazonaws.internal.SdkInternalList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* Specifies the VPC Security Groups associated with the cluster.
*
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param securityGroupIds
* Specifies the VPC Security Groups associated with the cluster.
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon
* VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @param preferredMaintenanceWindow
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @param preferredMaintenanceWindow
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
setPreferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
*
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*
*
* @param notificationTopicArn
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*/
public void setNotificationTopicArn(String notificationTopicArn) {
this.notificationTopicArn = notificationTopicArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
*
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*/
public String getNotificationTopicArn() {
return this.notificationTopicArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
*
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*
*
* @param notificationTopicArn
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withNotificationTopicArn(String notificationTopicArn) {
setNotificationTopicArn(notificationTopicArn);
return this;
}
/**
*
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as soon as
* possible for parameters when the ApplyImmediately
parameter is specified as true
for
* this request.
*
*
* @param cacheParameterGroupName
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as
* soon as possible for parameters when the ApplyImmediately
parameter is specified as
* true
for this request.
*/
public void setCacheParameterGroupName(String cacheParameterGroupName) {
this.cacheParameterGroupName = cacheParameterGroupName;
}
/**
*
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as soon as
* possible for parameters when the ApplyImmediately
parameter is specified as true
for
* this request.
*
*
* @return The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as
* soon as possible for parameters when the ApplyImmediately
parameter is specified as
* true
for this request.
*/
public String getCacheParameterGroupName() {
return this.cacheParameterGroupName;
}
/**
*
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as soon as
* possible for parameters when the ApplyImmediately
parameter is specified as true
for
* this request.
*
*
* @param cacheParameterGroupName
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as
* soon as possible for parameters when the ApplyImmediately
parameter is specified as
* true
for this request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheParameterGroupName(String cacheParameterGroupName) {
setCacheParameterGroupName(cacheParameterGroupName);
return this;
}
/**
*
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is active
* .
*
*
* Valid values: active
| inactive
*
*
* @param notificationTopicStatus
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is
* active
.
*
* Valid values: active
| inactive
*/
public void setNotificationTopicStatus(String notificationTopicStatus) {
this.notificationTopicStatus = notificationTopicStatus;
}
/**
*
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is active
* .
*
*
* Valid values: active
| inactive
*
*
* @return The status of the Amazon SNS notification topic. Notifications are sent only if the status is
* active
.
*
* Valid values: active
| inactive
*/
public String getNotificationTopicStatus() {
return this.notificationTopicStatus;
}
/**
*
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is active
* .
*
*
* Valid values: active
| inactive
*
*
* @param notificationTopicStatus
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is
* active
.
*
* Valid values: active
| inactive
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withNotificationTopicStatus(String notificationTopicStatus) {
setNotificationTopicStatus(notificationTopicStatus);
return this;
}
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the cluster.
*
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next failure
* reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*
*
* @param applyImmediately
* If true
, this parameter causes the modifications in this request and any pending
* modifications to be applied, asynchronously and as soon as possible, regardless of the
* PreferredMaintenanceWindow
setting for the cluster.
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next
* failure reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*/
public void setApplyImmediately(Boolean applyImmediately) {
this.applyImmediately = applyImmediately;
}
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the cluster.
*
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next failure
* reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*
*
* @return If true
, this parameter causes the modifications in this request and any pending
* modifications to be applied, asynchronously and as soon as possible, regardless of the
* PreferredMaintenanceWindow
setting for the cluster.
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next
* failure reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*/
public Boolean getApplyImmediately() {
return this.applyImmediately;
}
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the cluster.
*
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next failure
* reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*
*
* @param applyImmediately
* If true
, this parameter causes the modifications in this request and any pending
* modifications to be applied, asynchronously and as soon as possible, regardless of the
* PreferredMaintenanceWindow
setting for the cluster.
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next
* failure reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withApplyImmediately(Boolean applyImmediately) {
setApplyImmediately(applyImmediately);
return this;
}
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the cluster.
*
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next failure
* reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*
*
* @return If true
, this parameter causes the modifications in this request and any pending
* modifications to be applied, asynchronously and as soon as possible, regardless of the
* PreferredMaintenanceWindow
setting for the cluster.
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next
* failure reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*/
public Boolean isApplyImmediately() {
return this.applyImmediately;
}
/**
*
* The upgraded version of the cache engine to be run on the cache nodes.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster and create it anew with the earlier engine version.
*
*
* @param engineVersion
* The upgraded version of the cache engine to be run on the cache nodes.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster and create it anew with the
* earlier engine version.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The upgraded version of the cache engine to be run on the cache nodes.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster and create it anew with the earlier engine version.
*
*
* @return The upgraded version of the cache engine to be run on the cache nodes.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster and create it anew with the
* earlier engine version.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The upgraded version of the cache engine to be run on the cache nodes.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster and create it anew with the earlier engine version.
*
*
* @param engineVersion
* The upgraded version of the cache engine to be run on the cache nodes.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster and create it anew with the
* earlier engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @param autoMinorVersionUpgrade
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in
* to the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to
* opt-in to the next auto minor version upgrade campaign. This parameter is disabled for previous
* versions.
*/
public Boolean getAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @param autoMinorVersionUpgrade
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in
* to the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
setAutoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to
* opt-in to the next auto minor version upgrade campaign. This parameter is disabled for previous
* versions.
*/
public Boolean isAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*
* @param snapshotRetentionLimit
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*/
public void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*
* @return The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*/
public Integer getSnapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*
* @param snapshotRetentionLimit
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
setSnapshotRetentionLimit(snapshotRetentionLimit);
return this;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* @param snapshotWindow
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*/
public void setSnapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*/
public String getSnapshotWindow() {
return this.snapshotWindow;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* @param snapshotWindow
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withSnapshotWindow(String snapshotWindow) {
setSnapshotWindow(snapshotWindow);
return this;
}
/**
*
* A valid cache node type that you want to scale this cluster up to.
*
*
* @param cacheNodeType
* A valid cache node type that you want to scale this cluster up to.
*/
public void setCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
}
/**
*
* A valid cache node type that you want to scale this cluster up to.
*
*
* @return A valid cache node type that you want to scale this cluster up to.
*/
public String getCacheNodeType() {
return this.cacheNodeType;
}
/**
*
* A valid cache node type that you want to scale this cluster up to.
*
*
* @param cacheNodeType
* A valid cache node type that you want to scale this cluster up to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withCacheNodeType(String cacheNodeType) {
setCacheNodeType(cacheNodeType);
return this;
}
/**
*
* Reserved parameter. The password used to access a password protected server. This parameter must be specified
* with the auth-token-update
parameter. Password constraints:
*
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*
*
* @param authToken
* Reserved parameter. The password used to access a password protected server. This parameter must be
* specified with the auth-token-update
parameter. Password constraints:
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*/
public void setAuthToken(String authToken) {
this.authToken = authToken;
}
/**
*
* Reserved parameter. The password used to access a password protected server. This parameter must be specified
* with the auth-token-update
parameter. Password constraints:
*
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*
*
* @return Reserved parameter. The password used to access a password protected server. This parameter must be
* specified with the auth-token-update
parameter. Password constraints:
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*/
public String getAuthToken() {
return this.authToken;
}
/**
*
* Reserved parameter. The password used to access a password protected server. This parameter must be specified
* with the auth-token-update
parameter. Password constraints:
*
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*
*
* @param authToken
* Reserved parameter. The password used to access a password protected server. This parameter must be
* specified with the auth-token-update
parameter. Password constraints:
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withAuthToken(String authToken) {
setAuthToken(authToken);
return this;
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with Redis OSS
* AUTH
*
*
* @param authTokenUpdateStrategy
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with
* Redis OSS AUTH
* @see AuthTokenUpdateStrategyType
*/
public void setAuthTokenUpdateStrategy(String authTokenUpdateStrategy) {
this.authTokenUpdateStrategy = authTokenUpdateStrategy;
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with Redis OSS
* AUTH
*
*
* @return Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with
* Redis OSS AUTH
* @see AuthTokenUpdateStrategyType
*/
public String getAuthTokenUpdateStrategy() {
return this.authTokenUpdateStrategy;
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with Redis OSS
* AUTH
*
*
* @param authTokenUpdateStrategy
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with
* Redis OSS AUTH
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthTokenUpdateStrategyType
*/
public ModifyCacheClusterRequest withAuthTokenUpdateStrategy(String authTokenUpdateStrategy) {
setAuthTokenUpdateStrategy(authTokenUpdateStrategy);
return this;
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with Redis OSS
* AUTH
*
*
* @param authTokenUpdateStrategy
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with
* Redis OSS AUTH
* @see AuthTokenUpdateStrategyType
*/
public void setAuthTokenUpdateStrategy(AuthTokenUpdateStrategyType authTokenUpdateStrategy) {
withAuthTokenUpdateStrategy(authTokenUpdateStrategy);
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with Redis OSS
* AUTH
*
*
* @param authTokenUpdateStrategy
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* ROTATE - default, if no update strategy is provided
*
*
* -
*
* SET - allowed only after ROTATE
*
*
* -
*
* DELETE - allowed only when transitioning to RBAC
*
*
*
*
* For more information, see Authenticating Users with
* Redis OSS AUTH
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthTokenUpdateStrategyType
*/
public ModifyCacheClusterRequest withAuthTokenUpdateStrategy(AuthTokenUpdateStrategyType authTokenUpdateStrategy) {
this.authTokenUpdateStrategy = authTokenUpdateStrategy.toString();
return this;
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* @return Specifies the destination, format and type of the logs.
*/
public java.util.List getLogDeliveryConfigurations() {
if (logDeliveryConfigurations == null) {
logDeliveryConfigurations = new com.amazonaws.internal.SdkInternalList();
}
return logDeliveryConfigurations;
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* @param logDeliveryConfigurations
* Specifies the destination, format and type of the logs.
*/
public void setLogDeliveryConfigurations(java.util.Collection logDeliveryConfigurations) {
if (logDeliveryConfigurations == null) {
this.logDeliveryConfigurations = null;
return;
}
this.logDeliveryConfigurations = new com.amazonaws.internal.SdkInternalList(logDeliveryConfigurations);
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLogDeliveryConfigurations(java.util.Collection)} or
* {@link #withLogDeliveryConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param logDeliveryConfigurations
* Specifies the destination, format and type of the logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withLogDeliveryConfigurations(LogDeliveryConfigurationRequest... logDeliveryConfigurations) {
if (this.logDeliveryConfigurations == null) {
setLogDeliveryConfigurations(new com.amazonaws.internal.SdkInternalList(logDeliveryConfigurations.length));
}
for (LogDeliveryConfigurationRequest ele : logDeliveryConfigurations) {
this.logDeliveryConfigurations.add(ele);
}
return this;
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* @param logDeliveryConfigurations
* Specifies the destination, format and type of the logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCacheClusterRequest withLogDeliveryConfigurations(java.util.Collection logDeliveryConfigurations) {
setLogDeliveryConfigurations(logDeliveryConfigurations);
return this;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public void setIpDiscovery(String ipDiscovery) {
this.ipDiscovery = ipDiscovery;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @return The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public String getIpDiscovery() {
return this.ipDiscovery;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpDiscovery
*/
public ModifyCacheClusterRequest withIpDiscovery(String ipDiscovery) {
setIpDiscovery(ipDiscovery);
return this;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public void setIpDiscovery(IpDiscovery ipDiscovery) {
withIpDiscovery(ipDiscovery);
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpDiscovery
*/
public ModifyCacheClusterRequest withIpDiscovery(IpDiscovery ipDiscovery) {
this.ipDiscovery = ipDiscovery.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCacheClusterId() != null)
sb.append("CacheClusterId: ").append(getCacheClusterId()).append(",");
if (getNumCacheNodes() != null)
sb.append("NumCacheNodes: ").append(getNumCacheNodes()).append(",");
if (getCacheNodeIdsToRemove() != null)
sb.append("CacheNodeIdsToRemove: ").append(getCacheNodeIdsToRemove()).append(",");
if (getAZMode() != null)
sb.append("AZMode: ").append(getAZMode()).append(",");
if (getNewAvailabilityZones() != null)
sb.append("NewAvailabilityZones: ").append(getNewAvailabilityZones()).append(",");
if (getCacheSecurityGroupNames() != null)
sb.append("CacheSecurityGroupNames: ").append(getCacheSecurityGroupNames()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getPreferredMaintenanceWindow() != null)
sb.append("PreferredMaintenanceWindow: ").append(getPreferredMaintenanceWindow()).append(",");
if (getNotificationTopicArn() != null)
sb.append("NotificationTopicArn: ").append(getNotificationTopicArn()).append(",");
if (getCacheParameterGroupName() != null)
sb.append("CacheParameterGroupName: ").append(getCacheParameterGroupName()).append(",");
if (getNotificationTopicStatus() != null)
sb.append("NotificationTopicStatus: ").append(getNotificationTopicStatus()).append(",");
if (getApplyImmediately() != null)
sb.append("ApplyImmediately: ").append(getApplyImmediately()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAutoMinorVersionUpgrade() != null)
sb.append("AutoMinorVersionUpgrade: ").append(getAutoMinorVersionUpgrade()).append(",");
if (getSnapshotRetentionLimit() != null)
sb.append("SnapshotRetentionLimit: ").append(getSnapshotRetentionLimit()).append(",");
if (getSnapshotWindow() != null)
sb.append("SnapshotWindow: ").append(getSnapshotWindow()).append(",");
if (getCacheNodeType() != null)
sb.append("CacheNodeType: ").append(getCacheNodeType()).append(",");
if (getAuthToken() != null)
sb.append("AuthToken: ").append(getAuthToken()).append(",");
if (getAuthTokenUpdateStrategy() != null)
sb.append("AuthTokenUpdateStrategy: ").append(getAuthTokenUpdateStrategy()).append(",");
if (getLogDeliveryConfigurations() != null)
sb.append("LogDeliveryConfigurations: ").append(getLogDeliveryConfigurations()).append(",");
if (getIpDiscovery() != null)
sb.append("IpDiscovery: ").append(getIpDiscovery());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyCacheClusterRequest == false)
return false;
ModifyCacheClusterRequest other = (ModifyCacheClusterRequest) obj;
if (other.getCacheClusterId() == null ^ this.getCacheClusterId() == null)
return false;
if (other.getCacheClusterId() != null && other.getCacheClusterId().equals(this.getCacheClusterId()) == false)
return false;
if (other.getNumCacheNodes() == null ^ this.getNumCacheNodes() == null)
return false;
if (other.getNumCacheNodes() != null && other.getNumCacheNodes().equals(this.getNumCacheNodes()) == false)
return false;
if (other.getCacheNodeIdsToRemove() == null ^ this.getCacheNodeIdsToRemove() == null)
return false;
if (other.getCacheNodeIdsToRemove() != null && other.getCacheNodeIdsToRemove().equals(this.getCacheNodeIdsToRemove()) == false)
return false;
if (other.getAZMode() == null ^ this.getAZMode() == null)
return false;
if (other.getAZMode() != null && other.getAZMode().equals(this.getAZMode()) == false)
return false;
if (other.getNewAvailabilityZones() == null ^ this.getNewAvailabilityZones() == null)
return false;
if (other.getNewAvailabilityZones() != null && other.getNewAvailabilityZones().equals(this.getNewAvailabilityZones()) == false)
return false;
if (other.getCacheSecurityGroupNames() == null ^ this.getCacheSecurityGroupNames() == null)
return false;
if (other.getCacheSecurityGroupNames() != null && other.getCacheSecurityGroupNames().equals(this.getCacheSecurityGroupNames()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null)
return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false)
return false;
if (other.getNotificationTopicArn() == null ^ this.getNotificationTopicArn() == null)
return false;
if (other.getNotificationTopicArn() != null && other.getNotificationTopicArn().equals(this.getNotificationTopicArn()) == false)
return false;
if (other.getCacheParameterGroupName() == null ^ this.getCacheParameterGroupName() == null)
return false;
if (other.getCacheParameterGroupName() != null && other.getCacheParameterGroupName().equals(this.getCacheParameterGroupName()) == false)
return false;
if (other.getNotificationTopicStatus() == null ^ this.getNotificationTopicStatus() == null)
return false;
if (other.getNotificationTopicStatus() != null && other.getNotificationTopicStatus().equals(this.getNotificationTopicStatus()) == false)
return false;
if (other.getApplyImmediately() == null ^ this.getApplyImmediately() == null)
return false;
if (other.getApplyImmediately() != null && other.getApplyImmediately().equals(this.getApplyImmediately()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAutoMinorVersionUpgrade() == null ^ this.getAutoMinorVersionUpgrade() == null)
return false;
if (other.getAutoMinorVersionUpgrade() != null && other.getAutoMinorVersionUpgrade().equals(this.getAutoMinorVersionUpgrade()) == false)
return false;
if (other.getSnapshotRetentionLimit() == null ^ this.getSnapshotRetentionLimit() == null)
return false;
if (other.getSnapshotRetentionLimit() != null && other.getSnapshotRetentionLimit().equals(this.getSnapshotRetentionLimit()) == false)
return false;
if (other.getSnapshotWindow() == null ^ this.getSnapshotWindow() == null)
return false;
if (other.getSnapshotWindow() != null && other.getSnapshotWindow().equals(this.getSnapshotWindow()) == false)
return false;
if (other.getCacheNodeType() == null ^ this.getCacheNodeType() == null)
return false;
if (other.getCacheNodeType() != null && other.getCacheNodeType().equals(this.getCacheNodeType()) == false)
return false;
if (other.getAuthToken() == null ^ this.getAuthToken() == null)
return false;
if (other.getAuthToken() != null && other.getAuthToken().equals(this.getAuthToken()) == false)
return false;
if (other.getAuthTokenUpdateStrategy() == null ^ this.getAuthTokenUpdateStrategy() == null)
return false;
if (other.getAuthTokenUpdateStrategy() != null && other.getAuthTokenUpdateStrategy().equals(this.getAuthTokenUpdateStrategy()) == false)
return false;
if (other.getLogDeliveryConfigurations() == null ^ this.getLogDeliveryConfigurations() == null)
return false;
if (other.getLogDeliveryConfigurations() != null && other.getLogDeliveryConfigurations().equals(this.getLogDeliveryConfigurations()) == false)
return false;
if (other.getIpDiscovery() == null ^ this.getIpDiscovery() == null)
return false;
if (other.getIpDiscovery() != null && other.getIpDiscovery().equals(this.getIpDiscovery()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCacheClusterId() == null) ? 0 : getCacheClusterId().hashCode());
hashCode = prime * hashCode + ((getNumCacheNodes() == null) ? 0 : getNumCacheNodes().hashCode());
hashCode = prime * hashCode + ((getCacheNodeIdsToRemove() == null) ? 0 : getCacheNodeIdsToRemove().hashCode());
hashCode = prime * hashCode + ((getAZMode() == null) ? 0 : getAZMode().hashCode());
hashCode = prime * hashCode + ((getNewAvailabilityZones() == null) ? 0 : getNewAvailabilityZones().hashCode());
hashCode = prime * hashCode + ((getCacheSecurityGroupNames() == null) ? 0 : getCacheSecurityGroupNames().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getNotificationTopicArn() == null) ? 0 : getNotificationTopicArn().hashCode());
hashCode = prime * hashCode + ((getCacheParameterGroupName() == null) ? 0 : getCacheParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getNotificationTopicStatus() == null) ? 0 : getNotificationTopicStatus().hashCode());
hashCode = prime * hashCode + ((getApplyImmediately() == null) ? 0 : getApplyImmediately().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAutoMinorVersionUpgrade() == null) ? 0 : getAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getSnapshotRetentionLimit() == null) ? 0 : getSnapshotRetentionLimit().hashCode());
hashCode = prime * hashCode + ((getSnapshotWindow() == null) ? 0 : getSnapshotWindow().hashCode());
hashCode = prime * hashCode + ((getCacheNodeType() == null) ? 0 : getCacheNodeType().hashCode());
hashCode = prime * hashCode + ((getAuthToken() == null) ? 0 : getAuthToken().hashCode());
hashCode = prime * hashCode + ((getAuthTokenUpdateStrategy() == null) ? 0 : getAuthTokenUpdateStrategy().hashCode());
hashCode = prime * hashCode + ((getLogDeliveryConfigurations() == null) ? 0 : getLogDeliveryConfigurations().hashCode());
hashCode = prime * hashCode + ((getIpDiscovery() == null) ? 0 : getIpDiscovery().hashCode());
return hashCode;
}
@Override
public ModifyCacheClusterRequest clone() {
return (ModifyCacheClusterRequest) super.clone();
}
}