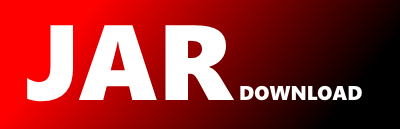
com.amazonaws.services.elasticache.model.BatchApplyUpdateActionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class BatchApplyUpdateActionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The replication group IDs
*
*/
private com.amazonaws.internal.SdkInternalList replicationGroupIds;
/**
*
* The cache cluster IDs
*
*/
private com.amazonaws.internal.SdkInternalList cacheClusterIds;
/**
*
* The unique ID of the service update
*
*/
private String serviceUpdateName;
/**
*
* The replication group IDs
*
*
* @return The replication group IDs
*/
public java.util.List getReplicationGroupIds() {
if (replicationGroupIds == null) {
replicationGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return replicationGroupIds;
}
/**
*
* The replication group IDs
*
*
* @param replicationGroupIds
* The replication group IDs
*/
public void setReplicationGroupIds(java.util.Collection replicationGroupIds) {
if (replicationGroupIds == null) {
this.replicationGroupIds = null;
return;
}
this.replicationGroupIds = new com.amazonaws.internal.SdkInternalList(replicationGroupIds);
}
/**
*
* The replication group IDs
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicationGroupIds(java.util.Collection)} or {@link #withReplicationGroupIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param replicationGroupIds
* The replication group IDs
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BatchApplyUpdateActionRequest withReplicationGroupIds(String... replicationGroupIds) {
if (this.replicationGroupIds == null) {
setReplicationGroupIds(new com.amazonaws.internal.SdkInternalList(replicationGroupIds.length));
}
for (String ele : replicationGroupIds) {
this.replicationGroupIds.add(ele);
}
return this;
}
/**
*
* The replication group IDs
*
*
* @param replicationGroupIds
* The replication group IDs
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BatchApplyUpdateActionRequest withReplicationGroupIds(java.util.Collection replicationGroupIds) {
setReplicationGroupIds(replicationGroupIds);
return this;
}
/**
*
* The cache cluster IDs
*
*
* @return The cache cluster IDs
*/
public java.util.List getCacheClusterIds() {
if (cacheClusterIds == null) {
cacheClusterIds = new com.amazonaws.internal.SdkInternalList();
}
return cacheClusterIds;
}
/**
*
* The cache cluster IDs
*
*
* @param cacheClusterIds
* The cache cluster IDs
*/
public void setCacheClusterIds(java.util.Collection cacheClusterIds) {
if (cacheClusterIds == null) {
this.cacheClusterIds = null;
return;
}
this.cacheClusterIds = new com.amazonaws.internal.SdkInternalList(cacheClusterIds);
}
/**
*
* The cache cluster IDs
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCacheClusterIds(java.util.Collection)} or {@link #withCacheClusterIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param cacheClusterIds
* The cache cluster IDs
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BatchApplyUpdateActionRequest withCacheClusterIds(String... cacheClusterIds) {
if (this.cacheClusterIds == null) {
setCacheClusterIds(new com.amazonaws.internal.SdkInternalList(cacheClusterIds.length));
}
for (String ele : cacheClusterIds) {
this.cacheClusterIds.add(ele);
}
return this;
}
/**
*
* The cache cluster IDs
*
*
* @param cacheClusterIds
* The cache cluster IDs
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BatchApplyUpdateActionRequest withCacheClusterIds(java.util.Collection cacheClusterIds) {
setCacheClusterIds(cacheClusterIds);
return this;
}
/**
*
* The unique ID of the service update
*
*
* @param serviceUpdateName
* The unique ID of the service update
*/
public void setServiceUpdateName(String serviceUpdateName) {
this.serviceUpdateName = serviceUpdateName;
}
/**
*
* The unique ID of the service update
*
*
* @return The unique ID of the service update
*/
public String getServiceUpdateName() {
return this.serviceUpdateName;
}
/**
*
* The unique ID of the service update
*
*
* @param serviceUpdateName
* The unique ID of the service update
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BatchApplyUpdateActionRequest withServiceUpdateName(String serviceUpdateName) {
setServiceUpdateName(serviceUpdateName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationGroupIds() != null)
sb.append("ReplicationGroupIds: ").append(getReplicationGroupIds()).append(",");
if (getCacheClusterIds() != null)
sb.append("CacheClusterIds: ").append(getCacheClusterIds()).append(",");
if (getServiceUpdateName() != null)
sb.append("ServiceUpdateName: ").append(getServiceUpdateName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof BatchApplyUpdateActionRequest == false)
return false;
BatchApplyUpdateActionRequest other = (BatchApplyUpdateActionRequest) obj;
if (other.getReplicationGroupIds() == null ^ this.getReplicationGroupIds() == null)
return false;
if (other.getReplicationGroupIds() != null && other.getReplicationGroupIds().equals(this.getReplicationGroupIds()) == false)
return false;
if (other.getCacheClusterIds() == null ^ this.getCacheClusterIds() == null)
return false;
if (other.getCacheClusterIds() != null && other.getCacheClusterIds().equals(this.getCacheClusterIds()) == false)
return false;
if (other.getServiceUpdateName() == null ^ this.getServiceUpdateName() == null)
return false;
if (other.getServiceUpdateName() != null && other.getServiceUpdateName().equals(this.getServiceUpdateName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationGroupIds() == null) ? 0 : getReplicationGroupIds().hashCode());
hashCode = prime * hashCode + ((getCacheClusterIds() == null) ? 0 : getCacheClusterIds().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateName() == null) ? 0 : getServiceUpdateName().hashCode());
return hashCode;
}
@Override
public BatchApplyUpdateActionRequest clone() {
return (BatchApplyUpdateActionRequest) super.clone();
}
}