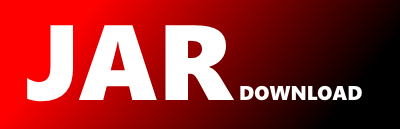
com.amazonaws.services.elasticache.model.CreateCacheClusterRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input of a CreateCacheCluster operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateCacheClusterRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*/
private String cacheClusterId;
/**
*
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the cluster
* is added to the specified replication group as a read replica; otherwise, the cluster is a standalone primary
* that is not part of any replication group.
*
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the cluster is
* created in Availability Zones that provide the best spread of read replicas across Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*/
private String replicationGroupId;
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*/
private String aZMode;
/**
*
* The EC2 Availability Zone in which the cluster is created.
*
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create your
* nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*
*/
private String preferredAvailabilityZone;
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability Zones
* that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead, or
* repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*/
private com.amazonaws.internal.SdkInternalList preferredAvailabilityZones;
/**
*
* The initial number of cache nodes that the cluster has.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase Request
* form at http://aws.amazon.com/contact-us
* /elasticache-node-limit-request/.
*
*/
private Integer numCacheNodes;
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported on Redis
* OSS version 2.8.22 and later.
*
*
*
*/
private String cacheNodeType;
/**
*
* The name of the cache engine to be used for this cluster.
*
*
* Valid values for this parameter are: memcached
| redis
*
*/
private String engine;
/**
*
* The version number of the cache engine to be used for this cluster. To view the supported cache engine versions,
* use the DescribeCacheEngineVersions operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster or replication group and create it anew with the
* earlier engine version.
*
*/
private String engineVersion;
/**
*
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*
*/
private String cacheParameterGroupName;
/**
*
* The name of the subnet group to be used for the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*/
private String cacheSubnetGroupName;
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon VPC).
*
*/
private com.amazonaws.internal.SdkInternalList cacheSecurityGroupNames;
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*/
private com.amazonaws.internal.SdkInternalList securityGroupIds;
/**
*
* A list of tags to be added to this resource.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon S3
* object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*/
private com.amazonaws.internal.SdkInternalList snapshotArns;
/**
*
* The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot status
* changes to restoring
while the new node group (shard) is being created.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*/
private String snapshotName;
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*/
private String preferredMaintenanceWindow;
/**
*
* The port number on which each of the cache nodes accepts connections.
*
*/
private Integer port;
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are
* sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*/
private String notificationTopicArn;
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*/
private Boolean autoMinorVersionUpgrade;
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if you
* set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before being deleted.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*
*/
private Integer snapshotRetentionLimit;
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*/
private String snapshotWindow;
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable special
* characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*/
private String authToken;
/**
*
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
*
*/
private String outpostMode;
/**
*
* The outpost ARN in which the cache cluster is created.
*
*/
private String preferredOutpostArn;
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*/
private com.amazonaws.internal.SdkInternalList preferredOutpostArns;
/**
*
* Specifies the destination, format and type of the logs.
*
*/
private com.amazonaws.internal.SdkInternalList logDeliveryConfigurations;
/**
*
* A flag that enables in-transit encryption when set to true.
*
*/
private Boolean transitEncryptionEnabled;
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*/
private String networkType;
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*/
private String ipDiscovery;
/**
* Default constructor for CreateCacheClusterRequest object. Callers should use the setter or fluent setter
* (with...) methods to initialize the object after creating it.
*/
public CreateCacheClusterRequest() {
}
/**
* Constructs a new CreateCacheClusterRequest object. Callers should use the setter or fluent setter (with...)
* methods to initialize any additional object members.
*
* @param cacheClusterId
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
* @param numCacheNodes
* The initial number of cache nodes that the cluster has.
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase
* Request form at http://aws.amazon
* .com/contact-us/elasticache-node-limit-request/.
* @param cacheNodeType
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine
* version 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
,
* cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported
* on Redis OSS version 2.8.22 and later.
*
*
* @param engine
* The name of the cache engine to be used for this cluster.
*
* Valid values for this parameter are: memcached
| redis
* @param cacheSecurityGroupNames
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*/
public CreateCacheClusterRequest(String cacheClusterId, Integer numCacheNodes, String cacheNodeType, String engine,
java.util.List cacheSecurityGroupNames) {
setCacheClusterId(cacheClusterId);
setNumCacheNodes(numCacheNodes);
setCacheNodeType(cacheNodeType);
setEngine(engine);
setCacheSecurityGroupNames(cacheSecurityGroupNames);
}
/**
*
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @param cacheClusterId
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public void setCacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
}
/**
*
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The node group (shard) identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public String getCacheClusterId() {
return this.cacheClusterId;
}
/**
*
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @param cacheClusterId
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 50 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withCacheClusterId(String cacheClusterId) {
setCacheClusterId(cacheClusterId);
return this;
}
/**
*
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the cluster
* is added to the specified replication group as a read replica; otherwise, the cluster is a standalone primary
* that is not part of any replication group.
*
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the cluster is
* created in Availability Zones that provide the best spread of read replicas across Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param replicationGroupId
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the
* cluster is added to the specified replication group as a read replica; otherwise, the cluster is a
* standalone primary that is not part of any replication group.
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the
* cluster is created in Availability Zones that provide the best spread of read replicas across Availability
* Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
/**
*
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the cluster
* is added to the specified replication group as a read replica; otherwise, the cluster is a standalone primary
* that is not part of any replication group.
*
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the cluster is
* created in Availability Zones that provide the best spread of read replicas across Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The ID of the replication group to which this cluster should belong. If this parameter is specified, the
* cluster is added to the specified replication group as a read replica; otherwise, the cluster is a
* standalone primary that is not part of any replication group.
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the
* cluster is created in Availability Zones that provide the best spread of read replicas across
* Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String getReplicationGroupId() {
return this.replicationGroupId;
}
/**
*
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the cluster
* is added to the specified replication group as a read replica; otherwise, the cluster is a standalone primary
* that is not part of any replication group.
*
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the cluster is
* created in Availability Zones that provide the best spread of read replicas across Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param replicationGroupId
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the
* cluster is added to the specified replication group as a read replica; otherwise, the cluster is a
* standalone primary that is not part of any replication group.
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the
* cluster is created in Availability Zones that provide the best spread of read replicas across Availability
* Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withReplicationGroupId(String replicationGroupId) {
setReplicationGroupId(replicationGroupId);
return this;
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @param aZMode
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created
* across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
*/
public void setAZMode(String aZMode) {
this.aZMode = aZMode;
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @return Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or
* created across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
*/
public String getAZMode() {
return this.aZMode;
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @param aZMode
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created
* across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AZMode
*/
public CreateCacheClusterRequest withAZMode(String aZMode) {
setAZMode(aZMode);
return this;
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @param aZMode
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created
* across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
*/
public void setAZMode(AZMode aZMode) {
withAZMode(aZMode);
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @param aZMode
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created
* across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AZMode
*/
public CreateCacheClusterRequest withAZMode(AZMode aZMode) {
this.aZMode = aZMode.toString();
return this;
}
/**
*
* The EC2 Availability Zone in which the cluster is created.
*
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create your
* nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*
*
* @param preferredAvailabilityZone
* The EC2 Availability Zone in which the cluster is created.
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create
* your nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*/
public void setPreferredAvailabilityZone(String preferredAvailabilityZone) {
this.preferredAvailabilityZone = preferredAvailabilityZone;
}
/**
*
* The EC2 Availability Zone in which the cluster is created.
*
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create your
* nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*
*
* @return The EC2 Availability Zone in which the cluster is created.
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create
* your nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*/
public String getPreferredAvailabilityZone() {
return this.preferredAvailabilityZone;
}
/**
*
* The EC2 Availability Zone in which the cluster is created.
*
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create your
* nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*
*
* @param preferredAvailabilityZone
* The EC2 Availability Zone in which the cluster is created.
*
* All nodes belonging to this cluster are placed in the preferred Availability Zone. If you want to create
* your nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredAvailabilityZone(String preferredAvailabilityZone) {
setPreferredAvailabilityZone(preferredAvailabilityZone);
return this;
}
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability Zones
* that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead, or
* repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* @return A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is
* not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*/
public java.util.List getPreferredAvailabilityZones() {
if (preferredAvailabilityZones == null) {
preferredAvailabilityZones = new com.amazonaws.internal.SdkInternalList();
}
return preferredAvailabilityZones;
}
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability Zones
* that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead, or
* repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* @param preferredAvailabilityZones
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is
* not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*/
public void setPreferredAvailabilityZones(java.util.Collection preferredAvailabilityZones) {
if (preferredAvailabilityZones == null) {
this.preferredAvailabilityZones = null;
return;
}
this.preferredAvailabilityZones = new com.amazonaws.internal.SdkInternalList(preferredAvailabilityZones);
}
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability Zones
* that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead, or
* repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPreferredAvailabilityZones(java.util.Collection)} or
* {@link #withPreferredAvailabilityZones(java.util.Collection)} if you want to override the existing values.
*
*
* @param preferredAvailabilityZones
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is
* not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredAvailabilityZones(String... preferredAvailabilityZones) {
if (this.preferredAvailabilityZones == null) {
setPreferredAvailabilityZones(new com.amazonaws.internal.SdkInternalList(preferredAvailabilityZones.length));
}
for (String ele : preferredAvailabilityZones) {
this.preferredAvailabilityZones.add(ele);
}
return this;
}
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability Zones
* that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead, or
* repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* @param preferredAvailabilityZones
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is
* not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredAvailabilityZones(java.util.Collection preferredAvailabilityZones) {
setPreferredAvailabilityZones(preferredAvailabilityZones);
return this;
}
/**
*
* The initial number of cache nodes that the cluster has.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase Request
* form at http://aws.amazon.com/contact-us
* /elasticache-node-limit-request/.
*
*
* @param numCacheNodes
* The initial number of cache nodes that the cluster has.
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase
* Request form at http://aws.amazon
* .com/contact-us/elasticache-node-limit-request/.
*/
public void setNumCacheNodes(Integer numCacheNodes) {
this.numCacheNodes = numCacheNodes;
}
/**
*
* The initial number of cache nodes that the cluster has.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase Request
* form at http://aws.amazon.com/contact-us
* /elasticache-node-limit-request/.
*
*
* @return The initial number of cache nodes that the cluster has.
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase
* Request form at http://aws.amazon
* .com/contact-us/elasticache-node-limit-request/.
*/
public Integer getNumCacheNodes() {
return this.numCacheNodes;
}
/**
*
* The initial number of cache nodes that the cluster has.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase Request
* form at http://aws.amazon.com/contact-us
* /elasticache-node-limit-request/.
*
*
* @param numCacheNodes
* The initial number of cache nodes that the cluster has.
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
* If you need more than 40 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase
* Request form at http://aws.amazon
* .com/contact-us/elasticache-node-limit-request/.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withNumCacheNodes(Integer numCacheNodes) {
setNumCacheNodes(numCacheNodes);
return this;
}
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported on Redis
* OSS version 2.8.22 and later.
*
*
*
*
* @param cacheNodeType
* The compute and memory capacity of the nodes in the node group (shard).
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine
* version 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
,
* cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported
* on Redis OSS version 2.8.22 and later.
*
*
*/
public void setCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
}
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported on Redis
* OSS version 2.8.22 and later.
*
*
*
*
* @return The compute and memory capacity of the nodes in the node group (shard).
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine
* version 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
,
* cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported
* on Redis OSS version 2.8.22 and later.
*
*
*/
public String getCacheNodeType() {
return this.cacheNodeType;
}
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported on Redis
* OSS version 2.8.22 and later.
*
*
*
*
* @param cacheNodeType
* The compute and memory capacity of the nodes in the node group (shard).
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine
* version 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
,
* cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported
* on Redis OSS version 2.8.22 and later.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withCacheNodeType(String cacheNodeType) {
setCacheNodeType(cacheNodeType);
return this;
}
/**
*
* The name of the cache engine to be used for this cluster.
*
*
* Valid values for this parameter are: memcached
| redis
*
*
* @param engine
* The name of the cache engine to be used for this cluster.
*
* Valid values for this parameter are: memcached
| redis
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The name of the cache engine to be used for this cluster.
*
*
* Valid values for this parameter are: memcached
| redis
*
*
* @return The name of the cache engine to be used for this cluster.
*
* Valid values for this parameter are: memcached
| redis
*/
public String getEngine() {
return this.engine;
}
/**
*
* The name of the cache engine to be used for this cluster.
*
*
* Valid values for this parameter are: memcached
| redis
*
*
* @param engine
* The name of the cache engine to be used for this cluster.
*
* Valid values for this parameter are: memcached
| redis
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The version number of the cache engine to be used for this cluster. To view the supported cache engine versions,
* use the DescribeCacheEngineVersions operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster or replication group and create it anew with the
* earlier engine version.
*
*
* @param engineVersion
* The version number of the cache engine to be used for this cluster. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster or replication group and
* create it anew with the earlier engine version.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The version number of the cache engine to be used for this cluster. To view the supported cache engine versions,
* use the DescribeCacheEngineVersions operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster or replication group and create it anew with the
* earlier engine version.
*
*
* @return The version number of the cache engine to be used for this cluster. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster or replication group and
* create it anew with the earlier engine version.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The version number of the cache engine to be used for this cluster. To view the supported cache engine versions,
* use the DescribeCacheEngineVersions operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster or replication group and create it anew with the
* earlier engine version.
*
*
* @param engineVersion
* The version number of the cache engine to be used for this cluster. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster or replication group and
* create it anew with the earlier engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*
*
* @param cacheParameterGroupName
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*/
public void setCacheParameterGroupName(String cacheParameterGroupName) {
this.cacheParameterGroupName = cacheParameterGroupName;
}
/**
*
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*
*
* @return The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*/
public String getCacheParameterGroupName() {
return this.cacheParameterGroupName;
}
/**
*
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*
*
* @param cacheParameterGroupName
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withCacheParameterGroupName(String cacheParameterGroupName) {
setCacheParameterGroupName(cacheParameterGroupName);
return this;
}
/**
*
* The name of the subnet group to be used for the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*
* @param cacheSubnetGroupName
* The name of the subnet group to be used for the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you
* start creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*/
public void setCacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
}
/**
*
* The name of the subnet group to be used for the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*
* @return The name of the subnet group to be used for the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you
* start creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*/
public String getCacheSubnetGroupName() {
return this.cacheSubnetGroupName;
}
/**
*
* The name of the subnet group to be used for the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*
* @param cacheSubnetGroupName
* The name of the subnet group to be used for the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you
* start creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withCacheSubnetGroupName(String cacheSubnetGroupName) {
setCacheSubnetGroupName(cacheSubnetGroupName);
return this;
}
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @return A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*/
public java.util.List getCacheSecurityGroupNames() {
if (cacheSecurityGroupNames == null) {
cacheSecurityGroupNames = new com.amazonaws.internal.SdkInternalList();
}
return cacheSecurityGroupNames;
}
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param cacheSecurityGroupNames
* A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*/
public void setCacheSecurityGroupNames(java.util.Collection cacheSecurityGroupNames) {
if (cacheSecurityGroupNames == null) {
this.cacheSecurityGroupNames = null;
return;
}
this.cacheSecurityGroupNames = new com.amazonaws.internal.SdkInternalList(cacheSecurityGroupNames);
}
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCacheSecurityGroupNames(java.util.Collection)} or
* {@link #withCacheSecurityGroupNames(java.util.Collection)} if you want to override the existing values.
*
*
* @param cacheSecurityGroupNames
* A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withCacheSecurityGroupNames(String... cacheSecurityGroupNames) {
if (this.cacheSecurityGroupNames == null) {
setCacheSecurityGroupNames(new com.amazonaws.internal.SdkInternalList(cacheSecurityGroupNames.length));
}
for (String ele : cacheSecurityGroupNames) {
this.cacheSecurityGroupNames.add(ele);
}
return this;
}
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param cacheSecurityGroupNames
* A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withCacheSecurityGroupNames(java.util.Collection cacheSecurityGroupNames) {
setCacheSecurityGroupNames(cacheSecurityGroupNames);
return this;
}
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @return One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return securityGroupIds;
}
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param securityGroupIds
* One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new com.amazonaws.internal.SdkInternalList(securityGroupIds);
}
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new com.amazonaws.internal.SdkInternalList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param securityGroupIds
* One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* A list of tags to be added to this resource.
*
*
* @return A list of tags to be added to this resource.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* A list of tags to be added to this resource.
*
*
* @param tags
* A list of tags to be added to this resource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* A list of tags to be added to this resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to be added to this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to be added to this resource.
*
*
* @param tags
* A list of tags to be added to this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon S3
* object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* @return A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis
* OSS RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard).
* The Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
public java.util.List getSnapshotArns() {
if (snapshotArns == null) {
snapshotArns = new com.amazonaws.internal.SdkInternalList();
}
return snapshotArns;
}
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon S3
* object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* @param snapshotArns
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS
* RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The
* Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
public void setSnapshotArns(java.util.Collection snapshotArns) {
if (snapshotArns == null) {
this.snapshotArns = null;
return;
}
this.snapshotArns = new com.amazonaws.internal.SdkInternalList(snapshotArns);
}
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon S3
* object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSnapshotArns(java.util.Collection)} or {@link #withSnapshotArns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param snapshotArns
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS
* RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The
* Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSnapshotArns(String... snapshotArns) {
if (this.snapshotArns == null) {
setSnapshotArns(new com.amazonaws.internal.SdkInternalList(snapshotArns.length));
}
for (String ele : snapshotArns) {
this.snapshotArns.add(ele);
}
return this;
}
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon S3
* object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* @param snapshotArns
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis OSS
* RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The
* Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSnapshotArns(java.util.Collection snapshotArns) {
setSnapshotArns(snapshotArns);
return this;
}
/**
*
* The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot status
* changes to restoring
while the new node group (shard) is being created.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param snapshotName
* The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot
* status changes to restoring
while the new node group (shard) is being created.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public void setSnapshotName(String snapshotName) {
this.snapshotName = snapshotName;
}
/**
*
* The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot status
* changes to restoring
while the new node group (shard) is being created.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot
* status changes to restoring
while the new node group (shard) is being created.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String getSnapshotName() {
return this.snapshotName;
}
/**
*
* The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot status
* changes to restoring
while the new node group (shard) is being created.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param snapshotName
* The name of a Redis OSS snapshot from which to restore data into the new node group (shard). The snapshot
* status changes to restoring
while the new node group (shard) is being created.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSnapshotName(String snapshotName) {
setSnapshotName(snapshotName);
return this;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* @param preferredMaintenanceWindow
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period.
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*/
public String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* @param preferredMaintenanceWindow
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
setPreferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
*
* The port number on which each of the cache nodes accepts connections.
*
*
* @param port
* The port number on which each of the cache nodes accepts connections.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port number on which each of the cache nodes accepts connections.
*
*
* @return The port number on which each of the cache nodes accepts connections.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port number on which each of the cache nodes accepts connections.
*
*
* @param port
* The port number on which each of the cache nodes accepts connections.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are
* sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*
* @param notificationTopicArn
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which
* notifications are sent.
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*/
public void setNotificationTopicArn(String notificationTopicArn) {
this.notificationTopicArn = notificationTopicArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are
* sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which
* notifications are sent.
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*/
public String getNotificationTopicArn() {
return this.notificationTopicArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are
* sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*
* @param notificationTopicArn
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which
* notifications are sent.
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withNotificationTopicArn(String notificationTopicArn) {
setNotificationTopicArn(notificationTopicArn);
return this;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @param autoMinorVersionUpgrade
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in
* to the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to
* opt-in to the next auto minor version upgrade campaign. This parameter is disabled for previous
* versions.
*/
public Boolean getAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @param autoMinorVersionUpgrade
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in
* to the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
setAutoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to
* opt-in to the next auto minor version upgrade campaign. This parameter is disabled for previous
* versions.
*/
public Boolean isAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if you
* set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before being deleted.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*
*
* @param snapshotRetentionLimit
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if
* you set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before
* being deleted.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*/
public void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if you
* set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before being deleted.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*
*
* @return The number of days for which ElastiCache retains automatic snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before
* being deleted.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*/
public Integer getSnapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if you
* set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before being deleted.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*
*
* @param snapshotRetentionLimit
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if
* you set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before
* being deleted.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
setSnapshotRetentionLimit(snapshotRetentionLimit);
return this;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param snapshotWindow
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public void setSnapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String getSnapshotWindow() {
return this.snapshotWindow;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param snapshotWindow
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withSnapshotWindow(String snapshotWindow) {
setSnapshotWindow(snapshotWindow);
return this;
}
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable special
* characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*
* @param authToken
* Reserved parameter. The password used to access a password protected server.
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable
* special characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*/
public void setAuthToken(String authToken) {
this.authToken = authToken;
}
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable special
* characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*
* @return Reserved parameter. The password used to access a password protected server.
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable
* special characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*/
public String getAuthToken() {
return this.authToken;
}
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable special
* characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*
* @param authToken
* Reserved parameter. The password used to access a password protected server.
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable
* special characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withAuthToken(String authToken) {
setAuthToken(authToken);
return this;
}
/**
*
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
*
*
* @param outpostMode
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
* @see OutpostMode
*/
public void setOutpostMode(String outpostMode) {
this.outpostMode = outpostMode;
}
/**
*
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
*
*
* @return Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
* @see OutpostMode
*/
public String getOutpostMode() {
return this.outpostMode;
}
/**
*
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
*
*
* @param outpostMode
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OutpostMode
*/
public CreateCacheClusterRequest withOutpostMode(String outpostMode) {
setOutpostMode(outpostMode);
return this;
}
/**
*
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
*
*
* @param outpostMode
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
* @see OutpostMode
*/
public void setOutpostMode(OutpostMode outpostMode) {
withOutpostMode(outpostMode);
}
/**
*
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
*
*
* @param outpostMode
* Specifies whether the nodes in the cluster are created in a single outpost or across multiple outposts.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OutpostMode
*/
public CreateCacheClusterRequest withOutpostMode(OutpostMode outpostMode) {
this.outpostMode = outpostMode.toString();
return this;
}
/**
*
* The outpost ARN in which the cache cluster is created.
*
*
* @param preferredOutpostArn
* The outpost ARN in which the cache cluster is created.
*/
public void setPreferredOutpostArn(String preferredOutpostArn) {
this.preferredOutpostArn = preferredOutpostArn;
}
/**
*
* The outpost ARN in which the cache cluster is created.
*
*
* @return The outpost ARN in which the cache cluster is created.
*/
public String getPreferredOutpostArn() {
return this.preferredOutpostArn;
}
/**
*
* The outpost ARN in which the cache cluster is created.
*
*
* @param preferredOutpostArn
* The outpost ARN in which the cache cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredOutpostArn(String preferredOutpostArn) {
setPreferredOutpostArn(preferredOutpostArn);
return this;
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* @return The outpost ARNs in which the cache cluster is created.
*/
public java.util.List getPreferredOutpostArns() {
if (preferredOutpostArns == null) {
preferredOutpostArns = new com.amazonaws.internal.SdkInternalList();
}
return preferredOutpostArns;
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* @param preferredOutpostArns
* The outpost ARNs in which the cache cluster is created.
*/
public void setPreferredOutpostArns(java.util.Collection preferredOutpostArns) {
if (preferredOutpostArns == null) {
this.preferredOutpostArns = null;
return;
}
this.preferredOutpostArns = new com.amazonaws.internal.SdkInternalList(preferredOutpostArns);
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPreferredOutpostArns(java.util.Collection)} or {@link #withPreferredOutpostArns(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param preferredOutpostArns
* The outpost ARNs in which the cache cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredOutpostArns(String... preferredOutpostArns) {
if (this.preferredOutpostArns == null) {
setPreferredOutpostArns(new com.amazonaws.internal.SdkInternalList(preferredOutpostArns.length));
}
for (String ele : preferredOutpostArns) {
this.preferredOutpostArns.add(ele);
}
return this;
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* @param preferredOutpostArns
* The outpost ARNs in which the cache cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withPreferredOutpostArns(java.util.Collection preferredOutpostArns) {
setPreferredOutpostArns(preferredOutpostArns);
return this;
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* @return Specifies the destination, format and type of the logs.
*/
public java.util.List getLogDeliveryConfigurations() {
if (logDeliveryConfigurations == null) {
logDeliveryConfigurations = new com.amazonaws.internal.SdkInternalList();
}
return logDeliveryConfigurations;
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* @param logDeliveryConfigurations
* Specifies the destination, format and type of the logs.
*/
public void setLogDeliveryConfigurations(java.util.Collection logDeliveryConfigurations) {
if (logDeliveryConfigurations == null) {
this.logDeliveryConfigurations = null;
return;
}
this.logDeliveryConfigurations = new com.amazonaws.internal.SdkInternalList(logDeliveryConfigurations);
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLogDeliveryConfigurations(java.util.Collection)} or
* {@link #withLogDeliveryConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param logDeliveryConfigurations
* Specifies the destination, format and type of the logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withLogDeliveryConfigurations(LogDeliveryConfigurationRequest... logDeliveryConfigurations) {
if (this.logDeliveryConfigurations == null) {
setLogDeliveryConfigurations(new com.amazonaws.internal.SdkInternalList(logDeliveryConfigurations.length));
}
for (LogDeliveryConfigurationRequest ele : logDeliveryConfigurations) {
this.logDeliveryConfigurations.add(ele);
}
return this;
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* @param logDeliveryConfigurations
* Specifies the destination, format and type of the logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withLogDeliveryConfigurations(java.util.Collection logDeliveryConfigurations) {
setLogDeliveryConfigurations(logDeliveryConfigurations);
return this;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @param transitEncryptionEnabled
* A flag that enables in-transit encryption when set to true.
*/
public void setTransitEncryptionEnabled(Boolean transitEncryptionEnabled) {
this.transitEncryptionEnabled = transitEncryptionEnabled;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @return A flag that enables in-transit encryption when set to true.
*/
public Boolean getTransitEncryptionEnabled() {
return this.transitEncryptionEnabled;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @param transitEncryptionEnabled
* A flag that enables in-transit encryption when set to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCacheClusterRequest withTransitEncryptionEnabled(Boolean transitEncryptionEnabled) {
setTransitEncryptionEnabled(transitEncryptionEnabled);
return this;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @return A flag that enables in-transit encryption when set to true.
*/
public Boolean isTransitEncryptionEnabled() {
return this.transitEncryptionEnabled;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* @param networkType
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public void setNetworkType(String networkType) {
this.networkType = networkType;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public String getNetworkType() {
return this.networkType;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* @param networkType
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NetworkType
*/
public CreateCacheClusterRequest withNetworkType(String networkType) {
setNetworkType(networkType);
return this;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* @param networkType
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public void setNetworkType(NetworkType networkType) {
withNetworkType(networkType);
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* @param networkType
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NetworkType
*/
public CreateCacheClusterRequest withNetworkType(NetworkType networkType) {
this.networkType = networkType.toString();
return this;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public void setIpDiscovery(String ipDiscovery) {
this.ipDiscovery = ipDiscovery;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @return The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public String getIpDiscovery() {
return this.ipDiscovery;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpDiscovery
*/
public CreateCacheClusterRequest withIpDiscovery(String ipDiscovery) {
setIpDiscovery(ipDiscovery);
return this;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public void setIpDiscovery(IpDiscovery ipDiscovery) {
withIpDiscovery(ipDiscovery);
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* @param ipDiscovery
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpDiscovery
*/
public CreateCacheClusterRequest withIpDiscovery(IpDiscovery ipDiscovery) {
this.ipDiscovery = ipDiscovery.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCacheClusterId() != null)
sb.append("CacheClusterId: ").append(getCacheClusterId()).append(",");
if (getReplicationGroupId() != null)
sb.append("ReplicationGroupId: ").append(getReplicationGroupId()).append(",");
if (getAZMode() != null)
sb.append("AZMode: ").append(getAZMode()).append(",");
if (getPreferredAvailabilityZone() != null)
sb.append("PreferredAvailabilityZone: ").append(getPreferredAvailabilityZone()).append(",");
if (getPreferredAvailabilityZones() != null)
sb.append("PreferredAvailabilityZones: ").append(getPreferredAvailabilityZones()).append(",");
if (getNumCacheNodes() != null)
sb.append("NumCacheNodes: ").append(getNumCacheNodes()).append(",");
if (getCacheNodeType() != null)
sb.append("CacheNodeType: ").append(getCacheNodeType()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getCacheParameterGroupName() != null)
sb.append("CacheParameterGroupName: ").append(getCacheParameterGroupName()).append(",");
if (getCacheSubnetGroupName() != null)
sb.append("CacheSubnetGroupName: ").append(getCacheSubnetGroupName()).append(",");
if (getCacheSecurityGroupNames() != null)
sb.append("CacheSecurityGroupNames: ").append(getCacheSecurityGroupNames()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getSnapshotArns() != null)
sb.append("SnapshotArns: ").append(getSnapshotArns()).append(",");
if (getSnapshotName() != null)
sb.append("SnapshotName: ").append(getSnapshotName()).append(",");
if (getPreferredMaintenanceWindow() != null)
sb.append("PreferredMaintenanceWindow: ").append(getPreferredMaintenanceWindow()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getNotificationTopicArn() != null)
sb.append("NotificationTopicArn: ").append(getNotificationTopicArn()).append(",");
if (getAutoMinorVersionUpgrade() != null)
sb.append("AutoMinorVersionUpgrade: ").append(getAutoMinorVersionUpgrade()).append(",");
if (getSnapshotRetentionLimit() != null)
sb.append("SnapshotRetentionLimit: ").append(getSnapshotRetentionLimit()).append(",");
if (getSnapshotWindow() != null)
sb.append("SnapshotWindow: ").append(getSnapshotWindow()).append(",");
if (getAuthToken() != null)
sb.append("AuthToken: ").append(getAuthToken()).append(",");
if (getOutpostMode() != null)
sb.append("OutpostMode: ").append(getOutpostMode()).append(",");
if (getPreferredOutpostArn() != null)
sb.append("PreferredOutpostArn: ").append(getPreferredOutpostArn()).append(",");
if (getPreferredOutpostArns() != null)
sb.append("PreferredOutpostArns: ").append(getPreferredOutpostArns()).append(",");
if (getLogDeliveryConfigurations() != null)
sb.append("LogDeliveryConfigurations: ").append(getLogDeliveryConfigurations()).append(",");
if (getTransitEncryptionEnabled() != null)
sb.append("TransitEncryptionEnabled: ").append(getTransitEncryptionEnabled()).append(",");
if (getNetworkType() != null)
sb.append("NetworkType: ").append(getNetworkType()).append(",");
if (getIpDiscovery() != null)
sb.append("IpDiscovery: ").append(getIpDiscovery());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateCacheClusterRequest == false)
return false;
CreateCacheClusterRequest other = (CreateCacheClusterRequest) obj;
if (other.getCacheClusterId() == null ^ this.getCacheClusterId() == null)
return false;
if (other.getCacheClusterId() != null && other.getCacheClusterId().equals(this.getCacheClusterId()) == false)
return false;
if (other.getReplicationGroupId() == null ^ this.getReplicationGroupId() == null)
return false;
if (other.getReplicationGroupId() != null && other.getReplicationGroupId().equals(this.getReplicationGroupId()) == false)
return false;
if (other.getAZMode() == null ^ this.getAZMode() == null)
return false;
if (other.getAZMode() != null && other.getAZMode().equals(this.getAZMode()) == false)
return false;
if (other.getPreferredAvailabilityZone() == null ^ this.getPreferredAvailabilityZone() == null)
return false;
if (other.getPreferredAvailabilityZone() != null && other.getPreferredAvailabilityZone().equals(this.getPreferredAvailabilityZone()) == false)
return false;
if (other.getPreferredAvailabilityZones() == null ^ this.getPreferredAvailabilityZones() == null)
return false;
if (other.getPreferredAvailabilityZones() != null && other.getPreferredAvailabilityZones().equals(this.getPreferredAvailabilityZones()) == false)
return false;
if (other.getNumCacheNodes() == null ^ this.getNumCacheNodes() == null)
return false;
if (other.getNumCacheNodes() != null && other.getNumCacheNodes().equals(this.getNumCacheNodes()) == false)
return false;
if (other.getCacheNodeType() == null ^ this.getCacheNodeType() == null)
return false;
if (other.getCacheNodeType() != null && other.getCacheNodeType().equals(this.getCacheNodeType()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getCacheParameterGroupName() == null ^ this.getCacheParameterGroupName() == null)
return false;
if (other.getCacheParameterGroupName() != null && other.getCacheParameterGroupName().equals(this.getCacheParameterGroupName()) == false)
return false;
if (other.getCacheSubnetGroupName() == null ^ this.getCacheSubnetGroupName() == null)
return false;
if (other.getCacheSubnetGroupName() != null && other.getCacheSubnetGroupName().equals(this.getCacheSubnetGroupName()) == false)
return false;
if (other.getCacheSecurityGroupNames() == null ^ this.getCacheSecurityGroupNames() == null)
return false;
if (other.getCacheSecurityGroupNames() != null && other.getCacheSecurityGroupNames().equals(this.getCacheSecurityGroupNames()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getSnapshotArns() == null ^ this.getSnapshotArns() == null)
return false;
if (other.getSnapshotArns() != null && other.getSnapshotArns().equals(this.getSnapshotArns()) == false)
return false;
if (other.getSnapshotName() == null ^ this.getSnapshotName() == null)
return false;
if (other.getSnapshotName() != null && other.getSnapshotName().equals(this.getSnapshotName()) == false)
return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null)
return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getNotificationTopicArn() == null ^ this.getNotificationTopicArn() == null)
return false;
if (other.getNotificationTopicArn() != null && other.getNotificationTopicArn().equals(this.getNotificationTopicArn()) == false)
return false;
if (other.getAutoMinorVersionUpgrade() == null ^ this.getAutoMinorVersionUpgrade() == null)
return false;
if (other.getAutoMinorVersionUpgrade() != null && other.getAutoMinorVersionUpgrade().equals(this.getAutoMinorVersionUpgrade()) == false)
return false;
if (other.getSnapshotRetentionLimit() == null ^ this.getSnapshotRetentionLimit() == null)
return false;
if (other.getSnapshotRetentionLimit() != null && other.getSnapshotRetentionLimit().equals(this.getSnapshotRetentionLimit()) == false)
return false;
if (other.getSnapshotWindow() == null ^ this.getSnapshotWindow() == null)
return false;
if (other.getSnapshotWindow() != null && other.getSnapshotWindow().equals(this.getSnapshotWindow()) == false)
return false;
if (other.getAuthToken() == null ^ this.getAuthToken() == null)
return false;
if (other.getAuthToken() != null && other.getAuthToken().equals(this.getAuthToken()) == false)
return false;
if (other.getOutpostMode() == null ^ this.getOutpostMode() == null)
return false;
if (other.getOutpostMode() != null && other.getOutpostMode().equals(this.getOutpostMode()) == false)
return false;
if (other.getPreferredOutpostArn() == null ^ this.getPreferredOutpostArn() == null)
return false;
if (other.getPreferredOutpostArn() != null && other.getPreferredOutpostArn().equals(this.getPreferredOutpostArn()) == false)
return false;
if (other.getPreferredOutpostArns() == null ^ this.getPreferredOutpostArns() == null)
return false;
if (other.getPreferredOutpostArns() != null && other.getPreferredOutpostArns().equals(this.getPreferredOutpostArns()) == false)
return false;
if (other.getLogDeliveryConfigurations() == null ^ this.getLogDeliveryConfigurations() == null)
return false;
if (other.getLogDeliveryConfigurations() != null && other.getLogDeliveryConfigurations().equals(this.getLogDeliveryConfigurations()) == false)
return false;
if (other.getTransitEncryptionEnabled() == null ^ this.getTransitEncryptionEnabled() == null)
return false;
if (other.getTransitEncryptionEnabled() != null && other.getTransitEncryptionEnabled().equals(this.getTransitEncryptionEnabled()) == false)
return false;
if (other.getNetworkType() == null ^ this.getNetworkType() == null)
return false;
if (other.getNetworkType() != null && other.getNetworkType().equals(this.getNetworkType()) == false)
return false;
if (other.getIpDiscovery() == null ^ this.getIpDiscovery() == null)
return false;
if (other.getIpDiscovery() != null && other.getIpDiscovery().equals(this.getIpDiscovery()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCacheClusterId() == null) ? 0 : getCacheClusterId().hashCode());
hashCode = prime * hashCode + ((getReplicationGroupId() == null) ? 0 : getReplicationGroupId().hashCode());
hashCode = prime * hashCode + ((getAZMode() == null) ? 0 : getAZMode().hashCode());
hashCode = prime * hashCode + ((getPreferredAvailabilityZone() == null) ? 0 : getPreferredAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getPreferredAvailabilityZones() == null) ? 0 : getPreferredAvailabilityZones().hashCode());
hashCode = prime * hashCode + ((getNumCacheNodes() == null) ? 0 : getNumCacheNodes().hashCode());
hashCode = prime * hashCode + ((getCacheNodeType() == null) ? 0 : getCacheNodeType().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getCacheParameterGroupName() == null) ? 0 : getCacheParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getCacheSubnetGroupName() == null) ? 0 : getCacheSubnetGroupName().hashCode());
hashCode = prime * hashCode + ((getCacheSecurityGroupNames() == null) ? 0 : getCacheSecurityGroupNames().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getSnapshotArns() == null) ? 0 : getSnapshotArns().hashCode());
hashCode = prime * hashCode + ((getSnapshotName() == null) ? 0 : getSnapshotName().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getNotificationTopicArn() == null) ? 0 : getNotificationTopicArn().hashCode());
hashCode = prime * hashCode + ((getAutoMinorVersionUpgrade() == null) ? 0 : getAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getSnapshotRetentionLimit() == null) ? 0 : getSnapshotRetentionLimit().hashCode());
hashCode = prime * hashCode + ((getSnapshotWindow() == null) ? 0 : getSnapshotWindow().hashCode());
hashCode = prime * hashCode + ((getAuthToken() == null) ? 0 : getAuthToken().hashCode());
hashCode = prime * hashCode + ((getOutpostMode() == null) ? 0 : getOutpostMode().hashCode());
hashCode = prime * hashCode + ((getPreferredOutpostArn() == null) ? 0 : getPreferredOutpostArn().hashCode());
hashCode = prime * hashCode + ((getPreferredOutpostArns() == null) ? 0 : getPreferredOutpostArns().hashCode());
hashCode = prime * hashCode + ((getLogDeliveryConfigurations() == null) ? 0 : getLogDeliveryConfigurations().hashCode());
hashCode = prime * hashCode + ((getTransitEncryptionEnabled() == null) ? 0 : getTransitEncryptionEnabled().hashCode());
hashCode = prime * hashCode + ((getNetworkType() == null) ? 0 : getNetworkType().hashCode());
hashCode = prime * hashCode + ((getIpDiscovery() == null) ? 0 : getIpDiscovery().hashCode());
return hashCode;
}
@Override
public CreateCacheClusterRequest clone() {
return (CreateCacheClusterRequest) super.clone();
}
}