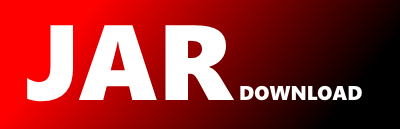
com.amazonaws.services.elasticache.model.CreateServerlessCacheRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateServerlessCacheRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
*
*/
private String serverlessCacheName;
/**
*
* User-provided description for the serverless cache. The default is NULL, i.e. if no description is provided then
* an empty string will be returned. The maximum length is 255 characters.
*
*/
private String description;
/**
*
* The name of the cache engine to be used for creating the serverless cache.
*
*/
private String engine;
/**
*
* The version of the cache engine that will be used to create the serverless cache.
*
*/
private String majorEngineVersion;
/**
*
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
*
*/
private CacheUsageLimits cacheUsageLimits;
/**
*
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default service key
* is used.
*
*/
private String kmsKeyId;
/**
*
* A list of the one or more VPC security groups to be associated with the serverless cache. The security group will
* authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the
* VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*/
private com.amazonaws.internal.SdkInternalList securityGroupIds;
/**
*
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
*
*/
private com.amazonaws.internal.SdkInternalList snapshotArnsToRestore;
/**
*
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL.
*
*/
private String userGroupId;
/**
*
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All
* the subnetIds must belong to the same VPC.
*
*/
private com.amazonaws.internal.SdkInternalList subnetIds;
/**
*
* The number of snapshots that will be retained for the serverless cache that is being created. As new snapshots
* beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available for Redis OSS and
* Serverless Memcached only.
*
*/
private Integer snapshotRetentionLimit;
/**
*
* The daily time that snapshots will be created from the new serverless cache. By default this number is populated
* with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS and Serverless
* Memcached only.
*
*/
private String dailySnapshotTime;
/**
*
* User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
*
*
* @param serverlessCacheName
* User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
*/
public void setServerlessCacheName(String serverlessCacheName) {
this.serverlessCacheName = serverlessCacheName;
}
/**
*
* User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
*
*
* @return User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
*/
public String getServerlessCacheName() {
return this.serverlessCacheName;
}
/**
*
* User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
*
*
* @param serverlessCacheName
* User-provided identifier for the serverless cache. This parameter is stored as a lowercase string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withServerlessCacheName(String serverlessCacheName) {
setServerlessCacheName(serverlessCacheName);
return this;
}
/**
*
* User-provided description for the serverless cache. The default is NULL, i.e. if no description is provided then
* an empty string will be returned. The maximum length is 255 characters.
*
*
* @param description
* User-provided description for the serverless cache. The default is NULL, i.e. if no description is
* provided then an empty string will be returned. The maximum length is 255 characters.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* User-provided description for the serverless cache. The default is NULL, i.e. if no description is provided then
* an empty string will be returned. The maximum length is 255 characters.
*
*
* @return User-provided description for the serverless cache. The default is NULL, i.e. if no description is
* provided then an empty string will be returned. The maximum length is 255 characters.
*/
public String getDescription() {
return this.description;
}
/**
*
* User-provided description for the serverless cache. The default is NULL, i.e. if no description is provided then
* an empty string will be returned. The maximum length is 255 characters.
*
*
* @param description
* User-provided description for the serverless cache. The default is NULL, i.e. if no description is
* provided then an empty string will be returned. The maximum length is 255 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The name of the cache engine to be used for creating the serverless cache.
*
*
* @param engine
* The name of the cache engine to be used for creating the serverless cache.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The name of the cache engine to be used for creating the serverless cache.
*
*
* @return The name of the cache engine to be used for creating the serverless cache.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The name of the cache engine to be used for creating the serverless cache.
*
*
* @param engine
* The name of the cache engine to be used for creating the serverless cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The version of the cache engine that will be used to create the serverless cache.
*
*
* @param majorEngineVersion
* The version of the cache engine that will be used to create the serverless cache.
*/
public void setMajorEngineVersion(String majorEngineVersion) {
this.majorEngineVersion = majorEngineVersion;
}
/**
*
* The version of the cache engine that will be used to create the serverless cache.
*
*
* @return The version of the cache engine that will be used to create the serverless cache.
*/
public String getMajorEngineVersion() {
return this.majorEngineVersion;
}
/**
*
* The version of the cache engine that will be used to create the serverless cache.
*
*
* @param majorEngineVersion
* The version of the cache engine that will be used to create the serverless cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withMajorEngineVersion(String majorEngineVersion) {
setMajorEngineVersion(majorEngineVersion);
return this;
}
/**
*
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
*
*
* @param cacheUsageLimits
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
*/
public void setCacheUsageLimits(CacheUsageLimits cacheUsageLimits) {
this.cacheUsageLimits = cacheUsageLimits;
}
/**
*
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
*
*
* @return Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
*/
public CacheUsageLimits getCacheUsageLimits() {
return this.cacheUsageLimits;
}
/**
*
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
*
*
* @param cacheUsageLimits
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withCacheUsageLimits(CacheUsageLimits cacheUsageLimits) {
setCacheUsageLimits(cacheUsageLimits);
return this;
}
/**
*
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default service key
* is used.
*
*
* @param kmsKeyId
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default
* service key is used.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default service key
* is used.
*
*
* @return ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default
* service key is used.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default service key
* is used.
*
*
* @param kmsKeyId
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default
* service key is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* A list of the one or more VPC security groups to be associated with the serverless cache. The security group will
* authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the
* VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*
* @return A list of the one or more VPC security groups to be associated with the serverless cache. The security
* group will authorize traffic access for the VPC end-point (private-link). If no other information is
* given this will be the VPC’s Default Security Group that is associated with the cluster VPC end-point.
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return securityGroupIds;
}
/**
*
* A list of the one or more VPC security groups to be associated with the serverless cache. The security group will
* authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the
* VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*
* @param securityGroupIds
* A list of the one or more VPC security groups to be associated with the serverless cache. The security
* group will authorize traffic access for the VPC end-point (private-link). If no other information is given
* this will be the VPC’s Default Security Group that is associated with the cluster VPC end-point.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new com.amazonaws.internal.SdkInternalList(securityGroupIds);
}
/**
*
* A list of the one or more VPC security groups to be associated with the serverless cache. The security group will
* authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the
* VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* A list of the one or more VPC security groups to be associated with the serverless cache. The security
* group will authorize traffic access for the VPC end-point (private-link). If no other information is given
* this will be the VPC’s Default Security Group that is associated with the cluster VPC end-point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new com.amazonaws.internal.SdkInternalList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* A list of the one or more VPC security groups to be associated with the serverless cache. The security group will
* authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the
* VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*
* @param securityGroupIds
* A list of the one or more VPC security groups to be associated with the serverless cache. The security
* group will authorize traffic access for the VPC end-point (private-link). If no other information is given
* this will be the VPC’s Default Security Group that is associated with the cluster VPC end-point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @return The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS
* and Serverless Memcached only.
*/
public java.util.List getSnapshotArnsToRestore() {
if (snapshotArnsToRestore == null) {
snapshotArnsToRestore = new com.amazonaws.internal.SdkInternalList();
}
return snapshotArnsToRestore;
}
/**
*
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param snapshotArnsToRestore
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
*/
public void setSnapshotArnsToRestore(java.util.Collection snapshotArnsToRestore) {
if (snapshotArnsToRestore == null) {
this.snapshotArnsToRestore = null;
return;
}
this.snapshotArnsToRestore = new com.amazonaws.internal.SdkInternalList(snapshotArnsToRestore);
}
/**
*
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSnapshotArnsToRestore(java.util.Collection)} or
* {@link #withSnapshotArnsToRestore(java.util.Collection)} if you want to override the existing values.
*
*
* @param snapshotArnsToRestore
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSnapshotArnsToRestore(String... snapshotArnsToRestore) {
if (this.snapshotArnsToRestore == null) {
setSnapshotArnsToRestore(new com.amazonaws.internal.SdkInternalList(snapshotArnsToRestore.length));
}
for (String ele : snapshotArnsToRestore) {
this.snapshotArnsToRestore.add(ele);
}
return this;
}
/**
*
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param snapshotArnsToRestore
* The ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis OSS and
* Serverless Memcached only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSnapshotArnsToRestore(java.util.Collection snapshotArnsToRestore) {
setSnapshotArnsToRestore(snapshotArnsToRestore);
return this;
}
/**
*
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*
*
* @return The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*
*
* @param tags
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
*
*
* @param tags
* The list of tags (key, value) pairs to be added to the serverless cache resource. Default is NULL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL.
*
*
* @param userGroupId
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only.
* Default is NULL.
*/
public void setUserGroupId(String userGroupId) {
this.userGroupId = userGroupId;
}
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL.
*
*
* @return The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only.
* Default is NULL.
*/
public String getUserGroupId() {
return this.userGroupId;
}
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL.
*
*
* @param userGroupId
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only.
* Default is NULL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withUserGroupId(String userGroupId) {
setUserGroupId(userGroupId);
return this;
}
/**
*
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All
* the subnetIds must belong to the same VPC.
*
*
* @return A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be
* deployed. All the subnetIds must belong to the same VPC.
*/
public java.util.List getSubnetIds() {
if (subnetIds == null) {
subnetIds = new com.amazonaws.internal.SdkInternalList();
}
return subnetIds;
}
/**
*
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All
* the subnetIds must belong to the same VPC.
*
*
* @param subnetIds
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed.
* All the subnetIds must belong to the same VPC.
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new com.amazonaws.internal.SdkInternalList(subnetIds);
}
/**
*
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All
* the subnetIds must belong to the same VPC.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed.
* All the subnetIds must belong to the same VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new com.amazonaws.internal.SdkInternalList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
*
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All
* the subnetIds must belong to the same VPC.
*
*
* @param subnetIds
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed.
* All the subnetIds must belong to the same VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
*
* The number of snapshots that will be retained for the serverless cache that is being created. As new snapshots
* beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param snapshotRetentionLimit
* The number of snapshots that will be retained for the serverless cache that is being created. As new
* snapshots beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available
* for Redis OSS and Serverless Memcached only.
*/
public void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
/**
*
* The number of snapshots that will be retained for the serverless cache that is being created. As new snapshots
* beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @return The number of snapshots that will be retained for the serverless cache that is being created. As new
* snapshots beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available
* for Redis OSS and Serverless Memcached only.
*/
public Integer getSnapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
*
* The number of snapshots that will be retained for the serverless cache that is being created. As new snapshots
* beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @param snapshotRetentionLimit
* The number of snapshots that will be retained for the serverless cache that is being created. As new
* snapshots beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available
* for Redis OSS and Serverless Memcached only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
setSnapshotRetentionLimit(snapshotRetentionLimit);
return this;
}
/**
*
* The daily time that snapshots will be created from the new serverless cache. By default this number is populated
* with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS and Serverless
* Memcached only.
*
*
* @param dailySnapshotTime
* The daily time that snapshots will be created from the new serverless cache. By default this number is
* populated with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS
* and Serverless Memcached only.
*/
public void setDailySnapshotTime(String dailySnapshotTime) {
this.dailySnapshotTime = dailySnapshotTime;
}
/**
*
* The daily time that snapshots will be created from the new serverless cache. By default this number is populated
* with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS and Serverless
* Memcached only.
*
*
* @return The daily time that snapshots will be created from the new serverless cache. By default this number is
* populated with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS
* and Serverless Memcached only.
*/
public String getDailySnapshotTime() {
return this.dailySnapshotTime;
}
/**
*
* The daily time that snapshots will be created from the new serverless cache. By default this number is populated
* with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS and Serverless
* Memcached only.
*
*
* @param dailySnapshotTime
* The daily time that snapshots will be created from the new serverless cache. By default this number is
* populated with 0, i.e. no snapshots will be created on an automatic daily basis. Available for Redis OSS
* and Serverless Memcached only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServerlessCacheRequest withDailySnapshotTime(String dailySnapshotTime) {
setDailySnapshotTime(dailySnapshotTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServerlessCacheName() != null)
sb.append("ServerlessCacheName: ").append(getServerlessCacheName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getMajorEngineVersion() != null)
sb.append("MajorEngineVersion: ").append(getMajorEngineVersion()).append(",");
if (getCacheUsageLimits() != null)
sb.append("CacheUsageLimits: ").append(getCacheUsageLimits()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getSnapshotArnsToRestore() != null)
sb.append("SnapshotArnsToRestore: ").append(getSnapshotArnsToRestore()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getUserGroupId() != null)
sb.append("UserGroupId: ").append(getUserGroupId()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getSnapshotRetentionLimit() != null)
sb.append("SnapshotRetentionLimit: ").append(getSnapshotRetentionLimit()).append(",");
if (getDailySnapshotTime() != null)
sb.append("DailySnapshotTime: ").append(getDailySnapshotTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateServerlessCacheRequest == false)
return false;
CreateServerlessCacheRequest other = (CreateServerlessCacheRequest) obj;
if (other.getServerlessCacheName() == null ^ this.getServerlessCacheName() == null)
return false;
if (other.getServerlessCacheName() != null && other.getServerlessCacheName().equals(this.getServerlessCacheName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getMajorEngineVersion() == null ^ this.getMajorEngineVersion() == null)
return false;
if (other.getMajorEngineVersion() != null && other.getMajorEngineVersion().equals(this.getMajorEngineVersion()) == false)
return false;
if (other.getCacheUsageLimits() == null ^ this.getCacheUsageLimits() == null)
return false;
if (other.getCacheUsageLimits() != null && other.getCacheUsageLimits().equals(this.getCacheUsageLimits()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getSnapshotArnsToRestore() == null ^ this.getSnapshotArnsToRestore() == null)
return false;
if (other.getSnapshotArnsToRestore() != null && other.getSnapshotArnsToRestore().equals(this.getSnapshotArnsToRestore()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getUserGroupId() == null ^ this.getUserGroupId() == null)
return false;
if (other.getUserGroupId() != null && other.getUserGroupId().equals(this.getUserGroupId()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getSnapshotRetentionLimit() == null ^ this.getSnapshotRetentionLimit() == null)
return false;
if (other.getSnapshotRetentionLimit() != null && other.getSnapshotRetentionLimit().equals(this.getSnapshotRetentionLimit()) == false)
return false;
if (other.getDailySnapshotTime() == null ^ this.getDailySnapshotTime() == null)
return false;
if (other.getDailySnapshotTime() != null && other.getDailySnapshotTime().equals(this.getDailySnapshotTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServerlessCacheName() == null) ? 0 : getServerlessCacheName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getMajorEngineVersion() == null) ? 0 : getMajorEngineVersion().hashCode());
hashCode = prime * hashCode + ((getCacheUsageLimits() == null) ? 0 : getCacheUsageLimits().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getSnapshotArnsToRestore() == null) ? 0 : getSnapshotArnsToRestore().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getUserGroupId() == null) ? 0 : getUserGroupId().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getSnapshotRetentionLimit() == null) ? 0 : getSnapshotRetentionLimit().hashCode());
hashCode = prime * hashCode + ((getDailySnapshotTime() == null) ? 0 : getDailySnapshotTime().hashCode());
return hashCode;
}
@Override
public CreateServerlessCacheRequest clone() {
return (CreateServerlessCacheRequest) super.clone();
}
}