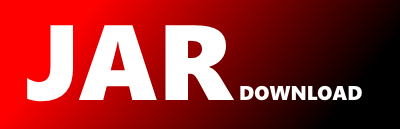
com.amazonaws.services.elasticache.model.ModifyServerlessCacheRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyServerlessCacheRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* User-provided identifier for the serverless cache to be modified.
*
*/
private String serverlessCacheName;
/**
*
* User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
*
*/
private String description;
/**
*
* Modify the cache usage limit for the serverless cache.
*
*/
private CacheUsageLimits cacheUsageLimits;
/**
*
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache. Available for
* Redis OSS only. Default is NULL.
*
*/
private Boolean removeUserGroup;
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL - the existing UserGroup is not removed.
*
*/
private String userGroupId;
/**
*
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means the
* current VPC security groups will be removed. This security group is used to authorize traffic access for the VPC
* end-point (private-link). Default = NULL - the existing list of VPC security groups is not removed.
*
*/
private com.amazonaws.internal.SdkInternalList securityGroupIds;
/**
*
* The number of days for which Elasticache retains automatic snapshots before deleting them. Available for Redis
* OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will not be removed
* or modified. The maximum value allowed is 35 days.
*
*/
private Integer snapshotRetentionLimit;
/**
*
* The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available for
* Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time configured for the
* cluster is not removed.
*
*/
private String dailySnapshotTime;
/**
*
* User-provided identifier for the serverless cache to be modified.
*
*
* @param serverlessCacheName
* User-provided identifier for the serverless cache to be modified.
*/
public void setServerlessCacheName(String serverlessCacheName) {
this.serverlessCacheName = serverlessCacheName;
}
/**
*
* User-provided identifier for the serverless cache to be modified.
*
*
* @return User-provided identifier for the serverless cache to be modified.
*/
public String getServerlessCacheName() {
return this.serverlessCacheName;
}
/**
*
* User-provided identifier for the serverless cache to be modified.
*
*
* @param serverlessCacheName
* User-provided identifier for the serverless cache to be modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withServerlessCacheName(String serverlessCacheName) {
setServerlessCacheName(serverlessCacheName);
return this;
}
/**
*
* User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
*
*
* @param description
* User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
*
*
* @return User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
*/
public String getDescription() {
return this.description;
}
/**
*
* User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
*
*
* @param description
* User provided description for the serverless cache. Default = NULL, i.e. the existing description is not
* removed/modified. The description has a maximum length of 255 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Modify the cache usage limit for the serverless cache.
*
*
* @param cacheUsageLimits
* Modify the cache usage limit for the serverless cache.
*/
public void setCacheUsageLimits(CacheUsageLimits cacheUsageLimits) {
this.cacheUsageLimits = cacheUsageLimits;
}
/**
*
* Modify the cache usage limit for the serverless cache.
*
*
* @return Modify the cache usage limit for the serverless cache.
*/
public CacheUsageLimits getCacheUsageLimits() {
return this.cacheUsageLimits;
}
/**
*
* Modify the cache usage limit for the serverless cache.
*
*
* @param cacheUsageLimits
* Modify the cache usage limit for the serverless cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withCacheUsageLimits(CacheUsageLimits cacheUsageLimits) {
setCacheUsageLimits(cacheUsageLimits);
return this;
}
/**
*
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache. Available for
* Redis OSS only. Default is NULL.
*
*
* @param removeUserGroup
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache.
* Available for Redis OSS only. Default is NULL.
*/
public void setRemoveUserGroup(Boolean removeUserGroup) {
this.removeUserGroup = removeUserGroup;
}
/**
*
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache. Available for
* Redis OSS only. Default is NULL.
*
*
* @return The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache.
* Available for Redis OSS only. Default is NULL.
*/
public Boolean getRemoveUserGroup() {
return this.removeUserGroup;
}
/**
*
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache. Available for
* Redis OSS only. Default is NULL.
*
*
* @param removeUserGroup
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache.
* Available for Redis OSS only. Default is NULL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withRemoveUserGroup(Boolean removeUserGroup) {
setRemoveUserGroup(removeUserGroup);
return this;
}
/**
*
* The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache. Available for
* Redis OSS only. Default is NULL.
*
*
* @return The identifier of the UserGroup to be removed from association with the Redis OSS serverless cache.
* Available for Redis OSS only. Default is NULL.
*/
public Boolean isRemoveUserGroup() {
return this.removeUserGroup;
}
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL - the existing UserGroup is not removed.
*
*
* @param userGroupId
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only.
* Default is NULL - the existing UserGroup is not removed.
*/
public void setUserGroupId(String userGroupId) {
this.userGroupId = userGroupId;
}
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL - the existing UserGroup is not removed.
*
*
* @return The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only.
* Default is NULL - the existing UserGroup is not removed.
*/
public String getUserGroupId() {
return this.userGroupId;
}
/**
*
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only. Default
* is NULL - the existing UserGroup is not removed.
*
*
* @param userGroupId
* The identifier of the UserGroup to be associated with the serverless cache. Available for Redis OSS only.
* Default is NULL - the existing UserGroup is not removed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withUserGroupId(String userGroupId) {
setUserGroupId(userGroupId);
return this;
}
/**
*
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means the
* current VPC security groups will be removed. This security group is used to authorize traffic access for the VPC
* end-point (private-link). Default = NULL - the existing list of VPC security groups is not removed.
*
*
* @return The new list of VPC security groups to be associated with the serverless cache. Populating this list
* means the current VPC security groups will be removed. This security group is used to authorize traffic
* access for the VPC end-point (private-link). Default = NULL - the existing list of VPC security groups is
* not removed.
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return securityGroupIds;
}
/**
*
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means the
* current VPC security groups will be removed. This security group is used to authorize traffic access for the VPC
* end-point (private-link). Default = NULL - the existing list of VPC security groups is not removed.
*
*
* @param securityGroupIds
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means
* the current VPC security groups will be removed. This security group is used to authorize traffic access
* for the VPC end-point (private-link). Default = NULL - the existing list of VPC security groups is not
* removed.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new com.amazonaws.internal.SdkInternalList(securityGroupIds);
}
/**
*
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means the
* current VPC security groups will be removed. This security group is used to authorize traffic access for the VPC
* end-point (private-link). Default = NULL - the existing list of VPC security groups is not removed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means
* the current VPC security groups will be removed. This security group is used to authorize traffic access
* for the VPC end-point (private-link). Default = NULL - the existing list of VPC security groups is not
* removed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new com.amazonaws.internal.SdkInternalList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means the
* current VPC security groups will be removed. This security group is used to authorize traffic access for the VPC
* end-point (private-link). Default = NULL - the existing list of VPC security groups is not removed.
*
*
* @param securityGroupIds
* The new list of VPC security groups to be associated with the serverless cache. Populating this list means
* the current VPC security groups will be removed. This security group is used to authorize traffic access
* for the VPC end-point (private-link). Default = NULL - the existing list of VPC security groups is not
* removed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* The number of days for which Elasticache retains automatic snapshots before deleting them. Available for Redis
* OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will not be removed
* or modified. The maximum value allowed is 35 days.
*
*
* @param snapshotRetentionLimit
* The number of days for which Elasticache retains automatic snapshots before deleting them. Available for
* Redis OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will
* not be removed or modified. The maximum value allowed is 35 days.
*/
public void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
/**
*
* The number of days for which Elasticache retains automatic snapshots before deleting them. Available for Redis
* OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will not be removed
* or modified. The maximum value allowed is 35 days.
*
*
* @return The number of days for which Elasticache retains automatic snapshots before deleting them. Available for
* Redis OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will
* not be removed or modified. The maximum value allowed is 35 days.
*/
public Integer getSnapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
*
* The number of days for which Elasticache retains automatic snapshots before deleting them. Available for Redis
* OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will not be removed
* or modified. The maximum value allowed is 35 days.
*
*
* @param snapshotRetentionLimit
* The number of days for which Elasticache retains automatic snapshots before deleting them. Available for
* Redis OSS and Serverless Memcached only. Default = NULL, i.e. the existing snapshot-retention-limit will
* not be removed or modified. The maximum value allowed is 35 days.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
setSnapshotRetentionLimit(snapshotRetentionLimit);
return this;
}
/**
*
* The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available for
* Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time configured for the
* cluster is not removed.
*
*
* @param dailySnapshotTime
* The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available
* for Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time
* configured for the cluster is not removed.
*/
public void setDailySnapshotTime(String dailySnapshotTime) {
this.dailySnapshotTime = dailySnapshotTime;
}
/**
*
* The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available for
* Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time configured for the
* cluster is not removed.
*
*
* @return The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available
* for Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time
* configured for the cluster is not removed.
*/
public String getDailySnapshotTime() {
return this.dailySnapshotTime;
}
/**
*
* The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available for
* Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time configured for the
* cluster is not removed.
*
*
* @param dailySnapshotTime
* The daily time during which Elasticache begins taking a daily snapshot of the serverless cache. Available
* for Redis OSS and Serverless Memcached only. The default is NULL, i.e. the existing snapshot time
* configured for the cluster is not removed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyServerlessCacheRequest withDailySnapshotTime(String dailySnapshotTime) {
setDailySnapshotTime(dailySnapshotTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServerlessCacheName() != null)
sb.append("ServerlessCacheName: ").append(getServerlessCacheName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getCacheUsageLimits() != null)
sb.append("CacheUsageLimits: ").append(getCacheUsageLimits()).append(",");
if (getRemoveUserGroup() != null)
sb.append("RemoveUserGroup: ").append(getRemoveUserGroup()).append(",");
if (getUserGroupId() != null)
sb.append("UserGroupId: ").append(getUserGroupId()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getSnapshotRetentionLimit() != null)
sb.append("SnapshotRetentionLimit: ").append(getSnapshotRetentionLimit()).append(",");
if (getDailySnapshotTime() != null)
sb.append("DailySnapshotTime: ").append(getDailySnapshotTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyServerlessCacheRequest == false)
return false;
ModifyServerlessCacheRequest other = (ModifyServerlessCacheRequest) obj;
if (other.getServerlessCacheName() == null ^ this.getServerlessCacheName() == null)
return false;
if (other.getServerlessCacheName() != null && other.getServerlessCacheName().equals(this.getServerlessCacheName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCacheUsageLimits() == null ^ this.getCacheUsageLimits() == null)
return false;
if (other.getCacheUsageLimits() != null && other.getCacheUsageLimits().equals(this.getCacheUsageLimits()) == false)
return false;
if (other.getRemoveUserGroup() == null ^ this.getRemoveUserGroup() == null)
return false;
if (other.getRemoveUserGroup() != null && other.getRemoveUserGroup().equals(this.getRemoveUserGroup()) == false)
return false;
if (other.getUserGroupId() == null ^ this.getUserGroupId() == null)
return false;
if (other.getUserGroupId() != null && other.getUserGroupId().equals(this.getUserGroupId()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getSnapshotRetentionLimit() == null ^ this.getSnapshotRetentionLimit() == null)
return false;
if (other.getSnapshotRetentionLimit() != null && other.getSnapshotRetentionLimit().equals(this.getSnapshotRetentionLimit()) == false)
return false;
if (other.getDailySnapshotTime() == null ^ this.getDailySnapshotTime() == null)
return false;
if (other.getDailySnapshotTime() != null && other.getDailySnapshotTime().equals(this.getDailySnapshotTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServerlessCacheName() == null) ? 0 : getServerlessCacheName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCacheUsageLimits() == null) ? 0 : getCacheUsageLimits().hashCode());
hashCode = prime * hashCode + ((getRemoveUserGroup() == null) ? 0 : getRemoveUserGroup().hashCode());
hashCode = prime * hashCode + ((getUserGroupId() == null) ? 0 : getUserGroupId().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getSnapshotRetentionLimit() == null) ? 0 : getSnapshotRetentionLimit().hashCode());
hashCode = prime * hashCode + ((getDailySnapshotTime() == null) ? 0 : getDailySnapshotTime().hashCode());
return hashCode;
}
@Override
public ModifyServerlessCacheRequest clone() {
return (ModifyServerlessCacheRequest) super.clone();
}
}