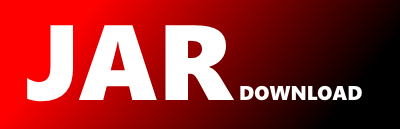
com.amazonaws.services.elasticache.model.NodeGroupConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Node group (shard) configuration options. Each node group (shard) configuration has the following: Slots
, PrimaryAvailabilityZone
, ReplicaAvailabilityZones
, ReplicaCount
.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class NodeGroupConfiguration implements Serializable, Cloneable {
/**
*
* Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
*
*/
private String nodeGroupId;
/**
*
* A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The string is
* in the format startkey-endkey
.
*
*
* Example: "0-3999"
*
*/
private String slots;
/**
*
* The number of read replica nodes in this node group (shard).
*
*/
private Integer replicaCount;
/**
*
* The Availability Zone where the primary node of this node group (shard) is launched.
*
*/
private String primaryAvailabilityZone;
/**
*
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this list must
* match the value of ReplicaCount
or ReplicasPerNodeGroup
if not specified.
*
*/
private com.amazonaws.internal.SdkInternalList replicaAvailabilityZones;
/**
*
* The outpost ARN of the primary node.
*
*/
private String primaryOutpostArn;
/**
*
* The outpost ARN of the node replicas.
*
*/
private com.amazonaws.internal.SdkInternalList replicaOutpostArns;
/**
*
* Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
*
*
* @param nodeGroupId
* Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
*/
public void setNodeGroupId(String nodeGroupId) {
this.nodeGroupId = nodeGroupId;
}
/**
*
* Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
*
*
* @return Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
*/
public String getNodeGroupId() {
return this.nodeGroupId;
}
/**
*
* Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
*
*
* @param nodeGroupId
* Either the ElastiCache (Redis OSS) supplied 4-digit id or a user supplied id for the node group these
* configuration values apply to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withNodeGroupId(String nodeGroupId) {
setNodeGroupId(nodeGroupId);
return this;
}
/**
*
* A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The string is
* in the format startkey-endkey
.
*
*
* Example: "0-3999"
*
*
* @param slots
* A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The
* string is in the format startkey-endkey
.
*
* Example: "0-3999"
*/
public void setSlots(String slots) {
this.slots = slots;
}
/**
*
* A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The string is
* in the format startkey-endkey
.
*
*
* Example: "0-3999"
*
*
* @return A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The
* string is in the format startkey-endkey
.
*
* Example: "0-3999"
*/
public String getSlots() {
return this.slots;
}
/**
*
* A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The string is
* in the format startkey-endkey
.
*
*
* Example: "0-3999"
*
*
* @param slots
* A string that specifies the keyspace for a particular node group. Keyspaces range from 0 to 16,383. The
* string is in the format startkey-endkey
.
*
* Example: "0-3999"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withSlots(String slots) {
setSlots(slots);
return this;
}
/**
*
* The number of read replica nodes in this node group (shard).
*
*
* @param replicaCount
* The number of read replica nodes in this node group (shard).
*/
public void setReplicaCount(Integer replicaCount) {
this.replicaCount = replicaCount;
}
/**
*
* The number of read replica nodes in this node group (shard).
*
*
* @return The number of read replica nodes in this node group (shard).
*/
public Integer getReplicaCount() {
return this.replicaCount;
}
/**
*
* The number of read replica nodes in this node group (shard).
*
*
* @param replicaCount
* The number of read replica nodes in this node group (shard).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withReplicaCount(Integer replicaCount) {
setReplicaCount(replicaCount);
return this;
}
/**
*
* The Availability Zone where the primary node of this node group (shard) is launched.
*
*
* @param primaryAvailabilityZone
* The Availability Zone where the primary node of this node group (shard) is launched.
*/
public void setPrimaryAvailabilityZone(String primaryAvailabilityZone) {
this.primaryAvailabilityZone = primaryAvailabilityZone;
}
/**
*
* The Availability Zone where the primary node of this node group (shard) is launched.
*
*
* @return The Availability Zone where the primary node of this node group (shard) is launched.
*/
public String getPrimaryAvailabilityZone() {
return this.primaryAvailabilityZone;
}
/**
*
* The Availability Zone where the primary node of this node group (shard) is launched.
*
*
* @param primaryAvailabilityZone
* The Availability Zone where the primary node of this node group (shard) is launched.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withPrimaryAvailabilityZone(String primaryAvailabilityZone) {
setPrimaryAvailabilityZone(primaryAvailabilityZone);
return this;
}
/**
*
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this list must
* match the value of ReplicaCount
or ReplicasPerNodeGroup
if not specified.
*
*
* @return A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this
* list must match the value of ReplicaCount
or ReplicasPerNodeGroup
if not
* specified.
*/
public java.util.List getReplicaAvailabilityZones() {
if (replicaAvailabilityZones == null) {
replicaAvailabilityZones = new com.amazonaws.internal.SdkInternalList();
}
return replicaAvailabilityZones;
}
/**
*
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this list must
* match the value of ReplicaCount
or ReplicasPerNodeGroup
if not specified.
*
*
* @param replicaAvailabilityZones
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this
* list must match the value of ReplicaCount
or ReplicasPerNodeGroup
if not
* specified.
*/
public void setReplicaAvailabilityZones(java.util.Collection replicaAvailabilityZones) {
if (replicaAvailabilityZones == null) {
this.replicaAvailabilityZones = null;
return;
}
this.replicaAvailabilityZones = new com.amazonaws.internal.SdkInternalList(replicaAvailabilityZones);
}
/**
*
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this list must
* match the value of ReplicaCount
or ReplicasPerNodeGroup
if not specified.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicaAvailabilityZones(java.util.Collection)} or
* {@link #withReplicaAvailabilityZones(java.util.Collection)} if you want to override the existing values.
*
*
* @param replicaAvailabilityZones
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this
* list must match the value of ReplicaCount
or ReplicasPerNodeGroup
if not
* specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withReplicaAvailabilityZones(String... replicaAvailabilityZones) {
if (this.replicaAvailabilityZones == null) {
setReplicaAvailabilityZones(new com.amazonaws.internal.SdkInternalList(replicaAvailabilityZones.length));
}
for (String ele : replicaAvailabilityZones) {
this.replicaAvailabilityZones.add(ele);
}
return this;
}
/**
*
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this list must
* match the value of ReplicaCount
or ReplicasPerNodeGroup
if not specified.
*
*
* @param replicaAvailabilityZones
* A list of Availability Zones to be used for the read replicas. The number of Availability Zones in this
* list must match the value of ReplicaCount
or ReplicasPerNodeGroup
if not
* specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withReplicaAvailabilityZones(java.util.Collection replicaAvailabilityZones) {
setReplicaAvailabilityZones(replicaAvailabilityZones);
return this;
}
/**
*
* The outpost ARN of the primary node.
*
*
* @param primaryOutpostArn
* The outpost ARN of the primary node.
*/
public void setPrimaryOutpostArn(String primaryOutpostArn) {
this.primaryOutpostArn = primaryOutpostArn;
}
/**
*
* The outpost ARN of the primary node.
*
*
* @return The outpost ARN of the primary node.
*/
public String getPrimaryOutpostArn() {
return this.primaryOutpostArn;
}
/**
*
* The outpost ARN of the primary node.
*
*
* @param primaryOutpostArn
* The outpost ARN of the primary node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withPrimaryOutpostArn(String primaryOutpostArn) {
setPrimaryOutpostArn(primaryOutpostArn);
return this;
}
/**
*
* The outpost ARN of the node replicas.
*
*
* @return The outpost ARN of the node replicas.
*/
public java.util.List getReplicaOutpostArns() {
if (replicaOutpostArns == null) {
replicaOutpostArns = new com.amazonaws.internal.SdkInternalList();
}
return replicaOutpostArns;
}
/**
*
* The outpost ARN of the node replicas.
*
*
* @param replicaOutpostArns
* The outpost ARN of the node replicas.
*/
public void setReplicaOutpostArns(java.util.Collection replicaOutpostArns) {
if (replicaOutpostArns == null) {
this.replicaOutpostArns = null;
return;
}
this.replicaOutpostArns = new com.amazonaws.internal.SdkInternalList(replicaOutpostArns);
}
/**
*
* The outpost ARN of the node replicas.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicaOutpostArns(java.util.Collection)} or {@link #withReplicaOutpostArns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param replicaOutpostArns
* The outpost ARN of the node replicas.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withReplicaOutpostArns(String... replicaOutpostArns) {
if (this.replicaOutpostArns == null) {
setReplicaOutpostArns(new com.amazonaws.internal.SdkInternalList(replicaOutpostArns.length));
}
for (String ele : replicaOutpostArns) {
this.replicaOutpostArns.add(ele);
}
return this;
}
/**
*
* The outpost ARN of the node replicas.
*
*
* @param replicaOutpostArns
* The outpost ARN of the node replicas.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NodeGroupConfiguration withReplicaOutpostArns(java.util.Collection replicaOutpostArns) {
setReplicaOutpostArns(replicaOutpostArns);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNodeGroupId() != null)
sb.append("NodeGroupId: ").append(getNodeGroupId()).append(",");
if (getSlots() != null)
sb.append("Slots: ").append(getSlots()).append(",");
if (getReplicaCount() != null)
sb.append("ReplicaCount: ").append(getReplicaCount()).append(",");
if (getPrimaryAvailabilityZone() != null)
sb.append("PrimaryAvailabilityZone: ").append(getPrimaryAvailabilityZone()).append(",");
if (getReplicaAvailabilityZones() != null)
sb.append("ReplicaAvailabilityZones: ").append(getReplicaAvailabilityZones()).append(",");
if (getPrimaryOutpostArn() != null)
sb.append("PrimaryOutpostArn: ").append(getPrimaryOutpostArn()).append(",");
if (getReplicaOutpostArns() != null)
sb.append("ReplicaOutpostArns: ").append(getReplicaOutpostArns());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof NodeGroupConfiguration == false)
return false;
NodeGroupConfiguration other = (NodeGroupConfiguration) obj;
if (other.getNodeGroupId() == null ^ this.getNodeGroupId() == null)
return false;
if (other.getNodeGroupId() != null && other.getNodeGroupId().equals(this.getNodeGroupId()) == false)
return false;
if (other.getSlots() == null ^ this.getSlots() == null)
return false;
if (other.getSlots() != null && other.getSlots().equals(this.getSlots()) == false)
return false;
if (other.getReplicaCount() == null ^ this.getReplicaCount() == null)
return false;
if (other.getReplicaCount() != null && other.getReplicaCount().equals(this.getReplicaCount()) == false)
return false;
if (other.getPrimaryAvailabilityZone() == null ^ this.getPrimaryAvailabilityZone() == null)
return false;
if (other.getPrimaryAvailabilityZone() != null && other.getPrimaryAvailabilityZone().equals(this.getPrimaryAvailabilityZone()) == false)
return false;
if (other.getReplicaAvailabilityZones() == null ^ this.getReplicaAvailabilityZones() == null)
return false;
if (other.getReplicaAvailabilityZones() != null && other.getReplicaAvailabilityZones().equals(this.getReplicaAvailabilityZones()) == false)
return false;
if (other.getPrimaryOutpostArn() == null ^ this.getPrimaryOutpostArn() == null)
return false;
if (other.getPrimaryOutpostArn() != null && other.getPrimaryOutpostArn().equals(this.getPrimaryOutpostArn()) == false)
return false;
if (other.getReplicaOutpostArns() == null ^ this.getReplicaOutpostArns() == null)
return false;
if (other.getReplicaOutpostArns() != null && other.getReplicaOutpostArns().equals(this.getReplicaOutpostArns()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNodeGroupId() == null) ? 0 : getNodeGroupId().hashCode());
hashCode = prime * hashCode + ((getSlots() == null) ? 0 : getSlots().hashCode());
hashCode = prime * hashCode + ((getReplicaCount() == null) ? 0 : getReplicaCount().hashCode());
hashCode = prime * hashCode + ((getPrimaryAvailabilityZone() == null) ? 0 : getPrimaryAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getReplicaAvailabilityZones() == null) ? 0 : getReplicaAvailabilityZones().hashCode());
hashCode = prime * hashCode + ((getPrimaryOutpostArn() == null) ? 0 : getPrimaryOutpostArn().hashCode());
hashCode = prime * hashCode + ((getReplicaOutpostArns() == null) ? 0 : getReplicaOutpostArns().hashCode());
return hashCode;
}
@Override
public NodeGroupConfiguration clone() {
try {
return (NodeGroupConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}