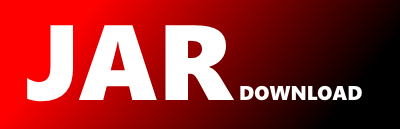
com.amazonaws.services.elasticache.model.ReplicationGroupPendingModifiedValues Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The settings to be applied to the Redis OSS replication group, either immediately or during the next maintenance
* window.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ReplicationGroupPendingModifiedValues implements Serializable, Cloneable {
/**
*
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or during
* the next maintenance window.
*
*/
private String primaryClusterId;
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*/
private String automaticFailoverStatus;
/**
*
* The status of an online resharding operation.
*
*/
private ReshardingStatus resharding;
/**
*
* The auth token status
*
*/
private String authTokenStatus;
/**
*
* The user group being modified.
*
*/
private UserGroupsUpdateStatus userGroups;
/**
*
* The log delivery configurations being modified
*
*/
private com.amazonaws.internal.SdkInternalList logDeliveryConfigurations;
/**
*
* A flag that enables in-transit encryption when set to true.
*
*/
private Boolean transitEncryptionEnabled;
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*/
private String transitEncryptionMode;
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*/
private String clusterMode;
/**
*
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or during
* the next maintenance window.
*
*
* @param primaryClusterId
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or
* during the next maintenance window.
*/
public void setPrimaryClusterId(String primaryClusterId) {
this.primaryClusterId = primaryClusterId;
}
/**
*
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or during
* the next maintenance window.
*
*
* @return The primary cluster ID that is applied immediately (if --apply-immediately
was specified),
* or during the next maintenance window.
*/
public String getPrimaryClusterId() {
return this.primaryClusterId;
}
/**
*
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or during
* the next maintenance window.
*
*
* @param primaryClusterId
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or
* during the next maintenance window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationGroupPendingModifiedValues withPrimaryClusterId(String primaryClusterId) {
setPrimaryClusterId(primaryClusterId);
return this;
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* @param automaticFailoverStatus
* Indicates the status of automatic failover for this Redis OSS replication group.
* @see PendingAutomaticFailoverStatus
*/
public void setAutomaticFailoverStatus(String automaticFailoverStatus) {
this.automaticFailoverStatus = automaticFailoverStatus;
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* @return Indicates the status of automatic failover for this Redis OSS replication group.
* @see PendingAutomaticFailoverStatus
*/
public String getAutomaticFailoverStatus() {
return this.automaticFailoverStatus;
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* @param automaticFailoverStatus
* Indicates the status of automatic failover for this Redis OSS replication group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PendingAutomaticFailoverStatus
*/
public ReplicationGroupPendingModifiedValues withAutomaticFailoverStatus(String automaticFailoverStatus) {
setAutomaticFailoverStatus(automaticFailoverStatus);
return this;
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* @param automaticFailoverStatus
* Indicates the status of automatic failover for this Redis OSS replication group.
* @see PendingAutomaticFailoverStatus
*/
public void setAutomaticFailoverStatus(PendingAutomaticFailoverStatus automaticFailoverStatus) {
withAutomaticFailoverStatus(automaticFailoverStatus);
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* @param automaticFailoverStatus
* Indicates the status of automatic failover for this Redis OSS replication group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PendingAutomaticFailoverStatus
*/
public ReplicationGroupPendingModifiedValues withAutomaticFailoverStatus(PendingAutomaticFailoverStatus automaticFailoverStatus) {
this.automaticFailoverStatus = automaticFailoverStatus.toString();
return this;
}
/**
*
* The status of an online resharding operation.
*
*
* @param resharding
* The status of an online resharding operation.
*/
public void setResharding(ReshardingStatus resharding) {
this.resharding = resharding;
}
/**
*
* The status of an online resharding operation.
*
*
* @return The status of an online resharding operation.
*/
public ReshardingStatus getResharding() {
return this.resharding;
}
/**
*
* The status of an online resharding operation.
*
*
* @param resharding
* The status of an online resharding operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationGroupPendingModifiedValues withResharding(ReshardingStatus resharding) {
setResharding(resharding);
return this;
}
/**
*
* The auth token status
*
*
* @param authTokenStatus
* The auth token status
* @see AuthTokenUpdateStatus
*/
public void setAuthTokenStatus(String authTokenStatus) {
this.authTokenStatus = authTokenStatus;
}
/**
*
* The auth token status
*
*
* @return The auth token status
* @see AuthTokenUpdateStatus
*/
public String getAuthTokenStatus() {
return this.authTokenStatus;
}
/**
*
* The auth token status
*
*
* @param authTokenStatus
* The auth token status
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthTokenUpdateStatus
*/
public ReplicationGroupPendingModifiedValues withAuthTokenStatus(String authTokenStatus) {
setAuthTokenStatus(authTokenStatus);
return this;
}
/**
*
* The auth token status
*
*
* @param authTokenStatus
* The auth token status
* @see AuthTokenUpdateStatus
*/
public void setAuthTokenStatus(AuthTokenUpdateStatus authTokenStatus) {
withAuthTokenStatus(authTokenStatus);
}
/**
*
* The auth token status
*
*
* @param authTokenStatus
* The auth token status
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthTokenUpdateStatus
*/
public ReplicationGroupPendingModifiedValues withAuthTokenStatus(AuthTokenUpdateStatus authTokenStatus) {
this.authTokenStatus = authTokenStatus.toString();
return this;
}
/**
*
* The user group being modified.
*
*
* @param userGroups
* The user group being modified.
*/
public void setUserGroups(UserGroupsUpdateStatus userGroups) {
this.userGroups = userGroups;
}
/**
*
* The user group being modified.
*
*
* @return The user group being modified.
*/
public UserGroupsUpdateStatus getUserGroups() {
return this.userGroups;
}
/**
*
* The user group being modified.
*
*
* @param userGroups
* The user group being modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationGroupPendingModifiedValues withUserGroups(UserGroupsUpdateStatus userGroups) {
setUserGroups(userGroups);
return this;
}
/**
*
* The log delivery configurations being modified
*
*
* @return The log delivery configurations being modified
*/
public java.util.List getLogDeliveryConfigurations() {
if (logDeliveryConfigurations == null) {
logDeliveryConfigurations = new com.amazonaws.internal.SdkInternalList();
}
return logDeliveryConfigurations;
}
/**
*
* The log delivery configurations being modified
*
*
* @param logDeliveryConfigurations
* The log delivery configurations being modified
*/
public void setLogDeliveryConfigurations(java.util.Collection logDeliveryConfigurations) {
if (logDeliveryConfigurations == null) {
this.logDeliveryConfigurations = null;
return;
}
this.logDeliveryConfigurations = new com.amazonaws.internal.SdkInternalList(logDeliveryConfigurations);
}
/**
*
* The log delivery configurations being modified
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLogDeliveryConfigurations(java.util.Collection)} or
* {@link #withLogDeliveryConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param logDeliveryConfigurations
* The log delivery configurations being modified
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationGroupPendingModifiedValues withLogDeliveryConfigurations(PendingLogDeliveryConfiguration... logDeliveryConfigurations) {
if (this.logDeliveryConfigurations == null) {
setLogDeliveryConfigurations(new com.amazonaws.internal.SdkInternalList(logDeliveryConfigurations.length));
}
for (PendingLogDeliveryConfiguration ele : logDeliveryConfigurations) {
this.logDeliveryConfigurations.add(ele);
}
return this;
}
/**
*
* The log delivery configurations being modified
*
*
* @param logDeliveryConfigurations
* The log delivery configurations being modified
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationGroupPendingModifiedValues withLogDeliveryConfigurations(java.util.Collection logDeliveryConfigurations) {
setLogDeliveryConfigurations(logDeliveryConfigurations);
return this;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @param transitEncryptionEnabled
* A flag that enables in-transit encryption when set to true.
*/
public void setTransitEncryptionEnabled(Boolean transitEncryptionEnabled) {
this.transitEncryptionEnabled = transitEncryptionEnabled;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @return A flag that enables in-transit encryption when set to true.
*/
public Boolean getTransitEncryptionEnabled() {
return this.transitEncryptionEnabled;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @param transitEncryptionEnabled
* A flag that enables in-transit encryption when set to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationGroupPendingModifiedValues withTransitEncryptionEnabled(Boolean transitEncryptionEnabled) {
setTransitEncryptionEnabled(transitEncryptionEnabled);
return this;
}
/**
*
* A flag that enables in-transit encryption when set to true.
*
*
* @return A flag that enables in-transit encryption when set to true.
*/
public Boolean isTransitEncryptionEnabled() {
return this.transitEncryptionEnabled;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* @param transitEncryptionMode
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public void setTransitEncryptionMode(String transitEncryptionMode) {
this.transitEncryptionMode = transitEncryptionMode;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public String getTransitEncryptionMode() {
return this.transitEncryptionMode;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* @param transitEncryptionMode
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransitEncryptionMode
*/
public ReplicationGroupPendingModifiedValues withTransitEncryptionMode(String transitEncryptionMode) {
setTransitEncryptionMode(transitEncryptionMode);
return this;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* @param transitEncryptionMode
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public void setTransitEncryptionMode(TransitEncryptionMode transitEncryptionMode) {
withTransitEncryptionMode(transitEncryptionMode);
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* @param transitEncryptionMode
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransitEncryptionMode
*/
public ReplicationGroupPendingModifiedValues withTransitEncryptionMode(TransitEncryptionMode transitEncryptionMode) {
this.transitEncryptionMode = transitEncryptionMode.toString();
return this;
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* @param clusterMode
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public void setClusterMode(String clusterMode) {
this.clusterMode = clusterMode;
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* @return Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public String getClusterMode() {
return this.clusterMode;
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* @param clusterMode
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ClusterMode
*/
public ReplicationGroupPendingModifiedValues withClusterMode(String clusterMode) {
setClusterMode(clusterMode);
return this;
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* @param clusterMode
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public void setClusterMode(ClusterMode clusterMode) {
withClusterMode(clusterMode);
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* @param clusterMode
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ClusterMode
*/
public ReplicationGroupPendingModifiedValues withClusterMode(ClusterMode clusterMode) {
this.clusterMode = clusterMode.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPrimaryClusterId() != null)
sb.append("PrimaryClusterId: ").append(getPrimaryClusterId()).append(",");
if (getAutomaticFailoverStatus() != null)
sb.append("AutomaticFailoverStatus: ").append(getAutomaticFailoverStatus()).append(",");
if (getResharding() != null)
sb.append("Resharding: ").append(getResharding()).append(",");
if (getAuthTokenStatus() != null)
sb.append("AuthTokenStatus: ").append(getAuthTokenStatus()).append(",");
if (getUserGroups() != null)
sb.append("UserGroups: ").append(getUserGroups()).append(",");
if (getLogDeliveryConfigurations() != null)
sb.append("LogDeliveryConfigurations: ").append(getLogDeliveryConfigurations()).append(",");
if (getTransitEncryptionEnabled() != null)
sb.append("TransitEncryptionEnabled: ").append(getTransitEncryptionEnabled()).append(",");
if (getTransitEncryptionMode() != null)
sb.append("TransitEncryptionMode: ").append(getTransitEncryptionMode()).append(",");
if (getClusterMode() != null)
sb.append("ClusterMode: ").append(getClusterMode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ReplicationGroupPendingModifiedValues == false)
return false;
ReplicationGroupPendingModifiedValues other = (ReplicationGroupPendingModifiedValues) obj;
if (other.getPrimaryClusterId() == null ^ this.getPrimaryClusterId() == null)
return false;
if (other.getPrimaryClusterId() != null && other.getPrimaryClusterId().equals(this.getPrimaryClusterId()) == false)
return false;
if (other.getAutomaticFailoverStatus() == null ^ this.getAutomaticFailoverStatus() == null)
return false;
if (other.getAutomaticFailoverStatus() != null && other.getAutomaticFailoverStatus().equals(this.getAutomaticFailoverStatus()) == false)
return false;
if (other.getResharding() == null ^ this.getResharding() == null)
return false;
if (other.getResharding() != null && other.getResharding().equals(this.getResharding()) == false)
return false;
if (other.getAuthTokenStatus() == null ^ this.getAuthTokenStatus() == null)
return false;
if (other.getAuthTokenStatus() != null && other.getAuthTokenStatus().equals(this.getAuthTokenStatus()) == false)
return false;
if (other.getUserGroups() == null ^ this.getUserGroups() == null)
return false;
if (other.getUserGroups() != null && other.getUserGroups().equals(this.getUserGroups()) == false)
return false;
if (other.getLogDeliveryConfigurations() == null ^ this.getLogDeliveryConfigurations() == null)
return false;
if (other.getLogDeliveryConfigurations() != null && other.getLogDeliveryConfigurations().equals(this.getLogDeliveryConfigurations()) == false)
return false;
if (other.getTransitEncryptionEnabled() == null ^ this.getTransitEncryptionEnabled() == null)
return false;
if (other.getTransitEncryptionEnabled() != null && other.getTransitEncryptionEnabled().equals(this.getTransitEncryptionEnabled()) == false)
return false;
if (other.getTransitEncryptionMode() == null ^ this.getTransitEncryptionMode() == null)
return false;
if (other.getTransitEncryptionMode() != null && other.getTransitEncryptionMode().equals(this.getTransitEncryptionMode()) == false)
return false;
if (other.getClusterMode() == null ^ this.getClusterMode() == null)
return false;
if (other.getClusterMode() != null && other.getClusterMode().equals(this.getClusterMode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPrimaryClusterId() == null) ? 0 : getPrimaryClusterId().hashCode());
hashCode = prime * hashCode + ((getAutomaticFailoverStatus() == null) ? 0 : getAutomaticFailoverStatus().hashCode());
hashCode = prime * hashCode + ((getResharding() == null) ? 0 : getResharding().hashCode());
hashCode = prime * hashCode + ((getAuthTokenStatus() == null) ? 0 : getAuthTokenStatus().hashCode());
hashCode = prime * hashCode + ((getUserGroups() == null) ? 0 : getUserGroups().hashCode());
hashCode = prime * hashCode + ((getLogDeliveryConfigurations() == null) ? 0 : getLogDeliveryConfigurations().hashCode());
hashCode = prime * hashCode + ((getTransitEncryptionEnabled() == null) ? 0 : getTransitEncryptionEnabled().hashCode());
hashCode = prime * hashCode + ((getTransitEncryptionMode() == null) ? 0 : getTransitEncryptionMode().hashCode());
hashCode = prime * hashCode + ((getClusterMode() == null) ? 0 : getClusterMode().hashCode());
return hashCode;
}
@Override
public ReplicationGroupPendingModifiedValues clone() {
try {
return (ReplicationGroupPendingModifiedValues) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}