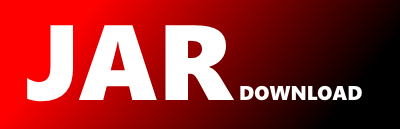
com.amazonaws.services.elasticache.model.ServiceUpdate Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* An update that you can apply to your Redis OSS clusters.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceUpdate implements Serializable, Cloneable {
/**
*
* The unique ID of the service update
*
*/
private String serviceUpdateName;
/**
*
* The date when the service update is initially available
*
*/
private java.util.Date serviceUpdateReleaseDate;
/**
*
* The date after which the service update is no longer available
*
*/
private java.util.Date serviceUpdateEndDate;
/**
*
* The severity of the service update
*
*/
private String serviceUpdateSeverity;
/**
*
* The recommendend date to apply the service update in order to ensure compliance. For information on compliance,
* see Self-Service Security Updates for Compliance.
*
*/
private java.util.Date serviceUpdateRecommendedApplyByDate;
/**
*
* The status of the service update
*
*/
private String serviceUpdateStatus;
/**
*
* Provides details of the service update
*
*/
private String serviceUpdateDescription;
/**
*
* Reflects the nature of the service update
*
*/
private String serviceUpdateType;
/**
*
* The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
*
*/
private String engine;
/**
*
* The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
*
*/
private String engineVersion;
/**
*
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*
*/
private Boolean autoUpdateAfterRecommendedApplyByDate;
/**
*
* The estimated length of time the service update will take
*
*/
private String estimatedUpdateTime;
/**
*
* The unique ID of the service update
*
*
* @param serviceUpdateName
* The unique ID of the service update
*/
public void setServiceUpdateName(String serviceUpdateName) {
this.serviceUpdateName = serviceUpdateName;
}
/**
*
* The unique ID of the service update
*
*
* @return The unique ID of the service update
*/
public String getServiceUpdateName() {
return this.serviceUpdateName;
}
/**
*
* The unique ID of the service update
*
*
* @param serviceUpdateName
* The unique ID of the service update
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withServiceUpdateName(String serviceUpdateName) {
setServiceUpdateName(serviceUpdateName);
return this;
}
/**
*
* The date when the service update is initially available
*
*
* @param serviceUpdateReleaseDate
* The date when the service update is initially available
*/
public void setServiceUpdateReleaseDate(java.util.Date serviceUpdateReleaseDate) {
this.serviceUpdateReleaseDate = serviceUpdateReleaseDate;
}
/**
*
* The date when the service update is initially available
*
*
* @return The date when the service update is initially available
*/
public java.util.Date getServiceUpdateReleaseDate() {
return this.serviceUpdateReleaseDate;
}
/**
*
* The date when the service update is initially available
*
*
* @param serviceUpdateReleaseDate
* The date when the service update is initially available
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withServiceUpdateReleaseDate(java.util.Date serviceUpdateReleaseDate) {
setServiceUpdateReleaseDate(serviceUpdateReleaseDate);
return this;
}
/**
*
* The date after which the service update is no longer available
*
*
* @param serviceUpdateEndDate
* The date after which the service update is no longer available
*/
public void setServiceUpdateEndDate(java.util.Date serviceUpdateEndDate) {
this.serviceUpdateEndDate = serviceUpdateEndDate;
}
/**
*
* The date after which the service update is no longer available
*
*
* @return The date after which the service update is no longer available
*/
public java.util.Date getServiceUpdateEndDate() {
return this.serviceUpdateEndDate;
}
/**
*
* The date after which the service update is no longer available
*
*
* @param serviceUpdateEndDate
* The date after which the service update is no longer available
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withServiceUpdateEndDate(java.util.Date serviceUpdateEndDate) {
setServiceUpdateEndDate(serviceUpdateEndDate);
return this;
}
/**
*
* The severity of the service update
*
*
* @param serviceUpdateSeverity
* The severity of the service update
* @see ServiceUpdateSeverity
*/
public void setServiceUpdateSeverity(String serviceUpdateSeverity) {
this.serviceUpdateSeverity = serviceUpdateSeverity;
}
/**
*
* The severity of the service update
*
*
* @return The severity of the service update
* @see ServiceUpdateSeverity
*/
public String getServiceUpdateSeverity() {
return this.serviceUpdateSeverity;
}
/**
*
* The severity of the service update
*
*
* @param serviceUpdateSeverity
* The severity of the service update
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceUpdateSeverity
*/
public ServiceUpdate withServiceUpdateSeverity(String serviceUpdateSeverity) {
setServiceUpdateSeverity(serviceUpdateSeverity);
return this;
}
/**
*
* The severity of the service update
*
*
* @param serviceUpdateSeverity
* The severity of the service update
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceUpdateSeverity
*/
public ServiceUpdate withServiceUpdateSeverity(ServiceUpdateSeverity serviceUpdateSeverity) {
this.serviceUpdateSeverity = serviceUpdateSeverity.toString();
return this;
}
/**
*
* The recommendend date to apply the service update in order to ensure compliance. For information on compliance,
* see Self-Service Security Updates for Compliance.
*
*
* @param serviceUpdateRecommendedApplyByDate
* The recommendend date to apply the service update in order to ensure compliance. For information on
* compliance, see Self-Service Security Updates for Compliance.
*/
public void setServiceUpdateRecommendedApplyByDate(java.util.Date serviceUpdateRecommendedApplyByDate) {
this.serviceUpdateRecommendedApplyByDate = serviceUpdateRecommendedApplyByDate;
}
/**
*
* The recommendend date to apply the service update in order to ensure compliance. For information on compliance,
* see Self-Service Security Updates for Compliance.
*
*
* @return The recommendend date to apply the service update in order to ensure compliance. For information on
* compliance, see Self-Service Security Updates for Compliance.
*/
public java.util.Date getServiceUpdateRecommendedApplyByDate() {
return this.serviceUpdateRecommendedApplyByDate;
}
/**
*
* The recommendend date to apply the service update in order to ensure compliance. For information on compliance,
* see Self-Service Security Updates for Compliance.
*
*
* @param serviceUpdateRecommendedApplyByDate
* The recommendend date to apply the service update in order to ensure compliance. For information on
* compliance, see Self-Service Security Updates for Compliance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withServiceUpdateRecommendedApplyByDate(java.util.Date serviceUpdateRecommendedApplyByDate) {
setServiceUpdateRecommendedApplyByDate(serviceUpdateRecommendedApplyByDate);
return this;
}
/**
*
* The status of the service update
*
*
* @param serviceUpdateStatus
* The status of the service update
* @see ServiceUpdateStatus
*/
public void setServiceUpdateStatus(String serviceUpdateStatus) {
this.serviceUpdateStatus = serviceUpdateStatus;
}
/**
*
* The status of the service update
*
*
* @return The status of the service update
* @see ServiceUpdateStatus
*/
public String getServiceUpdateStatus() {
return this.serviceUpdateStatus;
}
/**
*
* The status of the service update
*
*
* @param serviceUpdateStatus
* The status of the service update
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceUpdateStatus
*/
public ServiceUpdate withServiceUpdateStatus(String serviceUpdateStatus) {
setServiceUpdateStatus(serviceUpdateStatus);
return this;
}
/**
*
* The status of the service update
*
*
* @param serviceUpdateStatus
* The status of the service update
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceUpdateStatus
*/
public ServiceUpdate withServiceUpdateStatus(ServiceUpdateStatus serviceUpdateStatus) {
this.serviceUpdateStatus = serviceUpdateStatus.toString();
return this;
}
/**
*
* Provides details of the service update
*
*
* @param serviceUpdateDescription
* Provides details of the service update
*/
public void setServiceUpdateDescription(String serviceUpdateDescription) {
this.serviceUpdateDescription = serviceUpdateDescription;
}
/**
*
* Provides details of the service update
*
*
* @return Provides details of the service update
*/
public String getServiceUpdateDescription() {
return this.serviceUpdateDescription;
}
/**
*
* Provides details of the service update
*
*
* @param serviceUpdateDescription
* Provides details of the service update
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withServiceUpdateDescription(String serviceUpdateDescription) {
setServiceUpdateDescription(serviceUpdateDescription);
return this;
}
/**
*
* Reflects the nature of the service update
*
*
* @param serviceUpdateType
* Reflects the nature of the service update
* @see ServiceUpdateType
*/
public void setServiceUpdateType(String serviceUpdateType) {
this.serviceUpdateType = serviceUpdateType;
}
/**
*
* Reflects the nature of the service update
*
*
* @return Reflects the nature of the service update
* @see ServiceUpdateType
*/
public String getServiceUpdateType() {
return this.serviceUpdateType;
}
/**
*
* Reflects the nature of the service update
*
*
* @param serviceUpdateType
* Reflects the nature of the service update
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceUpdateType
*/
public ServiceUpdate withServiceUpdateType(String serviceUpdateType) {
setServiceUpdateType(serviceUpdateType);
return this;
}
/**
*
* Reflects the nature of the service update
*
*
* @param serviceUpdateType
* Reflects the nature of the service update
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceUpdateType
*/
public ServiceUpdate withServiceUpdateType(ServiceUpdateType serviceUpdateType) {
this.serviceUpdateType = serviceUpdateType.toString();
return this;
}
/**
*
* The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
*
*
* @param engine
* The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
*
*
* @return The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
*
*
* @param engine
* The Elasticache engine to which the update applies. Either Redis OSS or Memcached.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
*
*
* @param engineVersion
* The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
*
*
* @return The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
*
*
* @param engineVersion
* The Elasticache engine version to which the update applies. Either Redis OSS or Memcached engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*
*
* @param autoUpdateAfterRecommendedApplyByDate
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*/
public void setAutoUpdateAfterRecommendedApplyByDate(Boolean autoUpdateAfterRecommendedApplyByDate) {
this.autoUpdateAfterRecommendedApplyByDate = autoUpdateAfterRecommendedApplyByDate;
}
/**
*
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*
*
* @return Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*/
public Boolean getAutoUpdateAfterRecommendedApplyByDate() {
return this.autoUpdateAfterRecommendedApplyByDate;
}
/**
*
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*
*
* @param autoUpdateAfterRecommendedApplyByDate
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withAutoUpdateAfterRecommendedApplyByDate(Boolean autoUpdateAfterRecommendedApplyByDate) {
setAutoUpdateAfterRecommendedApplyByDate(autoUpdateAfterRecommendedApplyByDate);
return this;
}
/**
*
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*
*
* @return Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*/
public Boolean isAutoUpdateAfterRecommendedApplyByDate() {
return this.autoUpdateAfterRecommendedApplyByDate;
}
/**
*
* The estimated length of time the service update will take
*
*
* @param estimatedUpdateTime
* The estimated length of time the service update will take
*/
public void setEstimatedUpdateTime(String estimatedUpdateTime) {
this.estimatedUpdateTime = estimatedUpdateTime;
}
/**
*
* The estimated length of time the service update will take
*
*
* @return The estimated length of time the service update will take
*/
public String getEstimatedUpdateTime() {
return this.estimatedUpdateTime;
}
/**
*
* The estimated length of time the service update will take
*
*
* @param estimatedUpdateTime
* The estimated length of time the service update will take
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceUpdate withEstimatedUpdateTime(String estimatedUpdateTime) {
setEstimatedUpdateTime(estimatedUpdateTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceUpdateName() != null)
sb.append("ServiceUpdateName: ").append(getServiceUpdateName()).append(",");
if (getServiceUpdateReleaseDate() != null)
sb.append("ServiceUpdateReleaseDate: ").append(getServiceUpdateReleaseDate()).append(",");
if (getServiceUpdateEndDate() != null)
sb.append("ServiceUpdateEndDate: ").append(getServiceUpdateEndDate()).append(",");
if (getServiceUpdateSeverity() != null)
sb.append("ServiceUpdateSeverity: ").append(getServiceUpdateSeverity()).append(",");
if (getServiceUpdateRecommendedApplyByDate() != null)
sb.append("ServiceUpdateRecommendedApplyByDate: ").append(getServiceUpdateRecommendedApplyByDate()).append(",");
if (getServiceUpdateStatus() != null)
sb.append("ServiceUpdateStatus: ").append(getServiceUpdateStatus()).append(",");
if (getServiceUpdateDescription() != null)
sb.append("ServiceUpdateDescription: ").append(getServiceUpdateDescription()).append(",");
if (getServiceUpdateType() != null)
sb.append("ServiceUpdateType: ").append(getServiceUpdateType()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAutoUpdateAfterRecommendedApplyByDate() != null)
sb.append("AutoUpdateAfterRecommendedApplyByDate: ").append(getAutoUpdateAfterRecommendedApplyByDate()).append(",");
if (getEstimatedUpdateTime() != null)
sb.append("EstimatedUpdateTime: ").append(getEstimatedUpdateTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceUpdate == false)
return false;
ServiceUpdate other = (ServiceUpdate) obj;
if (other.getServiceUpdateName() == null ^ this.getServiceUpdateName() == null)
return false;
if (other.getServiceUpdateName() != null && other.getServiceUpdateName().equals(this.getServiceUpdateName()) == false)
return false;
if (other.getServiceUpdateReleaseDate() == null ^ this.getServiceUpdateReleaseDate() == null)
return false;
if (other.getServiceUpdateReleaseDate() != null && other.getServiceUpdateReleaseDate().equals(this.getServiceUpdateReleaseDate()) == false)
return false;
if (other.getServiceUpdateEndDate() == null ^ this.getServiceUpdateEndDate() == null)
return false;
if (other.getServiceUpdateEndDate() != null && other.getServiceUpdateEndDate().equals(this.getServiceUpdateEndDate()) == false)
return false;
if (other.getServiceUpdateSeverity() == null ^ this.getServiceUpdateSeverity() == null)
return false;
if (other.getServiceUpdateSeverity() != null && other.getServiceUpdateSeverity().equals(this.getServiceUpdateSeverity()) == false)
return false;
if (other.getServiceUpdateRecommendedApplyByDate() == null ^ this.getServiceUpdateRecommendedApplyByDate() == null)
return false;
if (other.getServiceUpdateRecommendedApplyByDate() != null
&& other.getServiceUpdateRecommendedApplyByDate().equals(this.getServiceUpdateRecommendedApplyByDate()) == false)
return false;
if (other.getServiceUpdateStatus() == null ^ this.getServiceUpdateStatus() == null)
return false;
if (other.getServiceUpdateStatus() != null && other.getServiceUpdateStatus().equals(this.getServiceUpdateStatus()) == false)
return false;
if (other.getServiceUpdateDescription() == null ^ this.getServiceUpdateDescription() == null)
return false;
if (other.getServiceUpdateDescription() != null && other.getServiceUpdateDescription().equals(this.getServiceUpdateDescription()) == false)
return false;
if (other.getServiceUpdateType() == null ^ this.getServiceUpdateType() == null)
return false;
if (other.getServiceUpdateType() != null && other.getServiceUpdateType().equals(this.getServiceUpdateType()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAutoUpdateAfterRecommendedApplyByDate() == null ^ this.getAutoUpdateAfterRecommendedApplyByDate() == null)
return false;
if (other.getAutoUpdateAfterRecommendedApplyByDate() != null
&& other.getAutoUpdateAfterRecommendedApplyByDate().equals(this.getAutoUpdateAfterRecommendedApplyByDate()) == false)
return false;
if (other.getEstimatedUpdateTime() == null ^ this.getEstimatedUpdateTime() == null)
return false;
if (other.getEstimatedUpdateTime() != null && other.getEstimatedUpdateTime().equals(this.getEstimatedUpdateTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceUpdateName() == null) ? 0 : getServiceUpdateName().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateReleaseDate() == null) ? 0 : getServiceUpdateReleaseDate().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateEndDate() == null) ? 0 : getServiceUpdateEndDate().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateSeverity() == null) ? 0 : getServiceUpdateSeverity().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateRecommendedApplyByDate() == null) ? 0 : getServiceUpdateRecommendedApplyByDate().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateStatus() == null) ? 0 : getServiceUpdateStatus().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateDescription() == null) ? 0 : getServiceUpdateDescription().hashCode());
hashCode = prime * hashCode + ((getServiceUpdateType() == null) ? 0 : getServiceUpdateType().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAutoUpdateAfterRecommendedApplyByDate() == null) ? 0 : getAutoUpdateAfterRecommendedApplyByDate().hashCode());
hashCode = prime * hashCode + ((getEstimatedUpdateTime() == null) ? 0 : getEstimatedUpdateTime().hashCode());
return hashCode;
}
@Override
public ServiceUpdate clone() {
try {
return (ServiceUpdate) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}