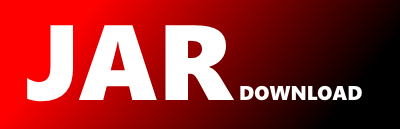
com.amazonaws.services.elasticache.AmazonElastiCacheAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticache;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.elasticache.model.*;
/**
* Interface for accessing AmazonElastiCache asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
* Amazon ElastiCache
* Amazon ElastiCache is a web service that makes it easier to set up,
* operate, and scale a distributed cache in the cloud.
*
*
* With ElastiCache, customers gain all of the benefits of a
* high-performance, in-memory cache with far less of the administrative
* burden of launching and managing a distributed cache. The service
* makes setup, scaling, and cluster failure handling much simpler than
* in a self-managed cache deployment.
*
*
* In addition, through integration with Amazon CloudWatch, customers get
* enhanced visibility into the key performance statistics associated
* with their cache and can receive alarms if a part of their cache runs
* hot.
*
*/
public interface AmazonElastiCacheAsync extends AmazonElastiCache {
/**
*
* The ModifyCacheParameterGroup operation modifies the
* parameters of a cache parameter group. You can modify up to 20
* parameters in a single request by submitting a list parameter name and
* value pairs.
*
*
* @param modifyCacheParameterGroupRequest Container for the necessary
* parameters to execute the ModifyCacheParameterGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* ModifyCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyCacheParameterGroupAsync(ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyCacheParameterGroup operation modifies the
* parameters of a cache parameter group. You can modify up to 20
* parameters in a single request by submitting a list parameter name and
* value pairs.
*
*
* @param modifyCacheParameterGroupRequest Container for the necessary
* parameters to execute the ModifyCacheParameterGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyCacheParameterGroupAsync(ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeReservedCacheNodes operation returns information
* about reserved cache nodes for this account, or about a specified
* reserved cache node.
*
*
* @param describeReservedCacheNodesRequest Container for the necessary
* parameters to execute the DescribeReservedCacheNodes operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeReservedCacheNodes service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedCacheNodesAsync(DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeReservedCacheNodes operation returns information
* about reserved cache nodes for this account, or about a specified
* reserved cache node.
*
*
* @param describeReservedCacheNodesRequest Container for the necessary
* parameters to execute the DescribeReservedCacheNodes operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReservedCacheNodes service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedCacheNodesAsync(DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The AuthorizeCacheSecurityGroupIngress operation allows
* network ingress to a cache security group. Applications using
* ElastiCache must be running on Amazon EC2, and Amazon EC2 security
* groups are used as the authorization mechanism.
*
*
* NOTE:You cannot authorize ingress from an Amazon EC2 security
* group in one region to an ElastiCache cluster in another region.
*
*
* @param authorizeCacheSecurityGroupIngressRequest Container for the
* necessary parameters to execute the AuthorizeCacheSecurityGroupIngress
* operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* AuthorizeCacheSecurityGroupIngress service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeCacheSecurityGroupIngressAsync(AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The AuthorizeCacheSecurityGroupIngress operation allows
* network ingress to a cache security group. Applications using
* ElastiCache must be running on Amazon EC2, and Amazon EC2 security
* groups are used as the authorization mechanism.
*
*
* NOTE:You cannot authorize ingress from an Amazon EC2 security
* group in one region to an ElastiCache cluster in another region.
*
*
* @param authorizeCacheSecurityGroupIngressRequest Container for the
* necessary parameters to execute the AuthorizeCacheSecurityGroupIngress
* operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AuthorizeCacheSecurityGroupIngress service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeCacheSecurityGroupIngressAsync(AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheParameterGroups operation returns a list of
* cache parameter group descriptions. If a cache parameter group name is
* specified, the list will contain only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest Container for the necessary
* parameters to execute the DescribeCacheParameterGroups operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeCacheParameterGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheParameterGroupsAsync(DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheParameterGroups operation returns a list of
* cache parameter group descriptions. If a cache parameter group name is
* specified, the list will contain only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest Container for the necessary
* parameters to execute the DescribeCacheParameterGroups operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCacheParameterGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheParameterGroupsAsync(DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheSecurityGroup operation creates a new cache
* security group. Use a cache security group to control access to one or
* more cache clusters.
*
*
* Cache security groups are only used when you are creating a cache
* cluster outside of an Amazon Virtual Private Cloud (VPC). If you are
* creating a cache cluster inside of a VPC, use a cache subnet group
* instead. For more information, see
* CreateCacheSubnetGroup
* .
*
*
* @param createCacheSecurityGroupRequest Container for the necessary
* parameters to execute the CreateCacheSecurityGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CreateCacheSecurityGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheSecurityGroupAsync(CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheSecurityGroup operation creates a new cache
* security group. Use a cache security group to control access to one or
* more cache clusters.
*
*
* Cache security groups are only used when you are creating a cache
* cluster outside of an Amazon Virtual Private Cloud (VPC). If you are
* creating a cache cluster inside of a VPC, use a cache subnet group
* instead. For more information, see
* CreateCacheSubnetGroup
* .
*
*
* @param createCacheSecurityGroupRequest Container for the necessary
* parameters to execute the CreateCacheSecurityGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateCacheSecurityGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheSecurityGroupAsync(CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteReplicationGroup operation deletes an existing
* cluster. By default, this operation deletes the entire cluster,
* including the primary node group and all of the read replicas. You can
* optionally delete only the read replicas, while retaining the primary
* node group.
*
*
* When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the selected resources; you
* cannot cancel or revert this operation.
*
*
* @param deleteReplicationGroupRequest Container for the necessary
* parameters to execute the DeleteReplicationGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DeleteReplicationGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteReplicationGroupAsync(DeleteReplicationGroupRequest deleteReplicationGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteReplicationGroup operation deletes an existing
* cluster. By default, this operation deletes the entire cluster,
* including the primary node group and all of the read replicas. You can
* optionally delete only the read replicas, while retaining the primary
* node group.
*
*
* When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the selected resources; you
* cannot cancel or revert this operation.
*
*
* @param deleteReplicationGroupRequest Container for the necessary
* parameters to execute the DeleteReplicationGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteReplicationGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteReplicationGroupAsync(DeleteReplicationGroupRequest deleteReplicationGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheParameterGroup operation creates a new cache
* parameter group. A cache parameter group is a collection of parameters
* that you apply to all of the nodes in a cache cluster.
*
*
* @param createCacheParameterGroupRequest Container for the necessary
* parameters to execute the CreateCacheParameterGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CreateCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheParameterGroupAsync(CreateCacheParameterGroupRequest createCacheParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheParameterGroup operation creates a new cache
* parameter group. A cache parameter group is a collection of parameters
* that you apply to all of the nodes in a cache cluster.
*
*
* @param createCacheParameterGroupRequest Container for the necessary
* parameters to execute the CreateCacheParameterGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheParameterGroupAsync(CreateCacheParameterGroupRequest createCacheParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyCacheCluster operation modifies the settings for a
* cache cluster. You can use this operation to change one or more
* cluster configuration parameters by specifying the parameters and the
* new values.
*
*
* @param modifyCacheClusterRequest Container for the necessary
* parameters to execute the ModifyCacheCluster operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* ModifyCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyCacheClusterAsync(ModifyCacheClusterRequest modifyCacheClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyCacheCluster operation modifies the settings for a
* cache cluster. You can use this operation to change one or more
* cluster configuration parameters by specifying the parameters and the
* new values.
*
*
* @param modifyCacheClusterRequest Container for the necessary
* parameters to execute the ModifyCacheCluster operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyCacheClusterAsync(ModifyCacheClusterRequest modifyCacheClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheCluster operation deletes a previously
* provisioned cache cluster. DeleteCacheCluster deletes all
* associated cache nodes, node endpoints and the cache cluster itself.
* When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the cache cluster; you cannot
* cancel or revert this operation.
*
*
* This API cannot be used to delete a cache cluster that is the last
* read replica of a replication group that has automatic failover mode
* enabled.
*
*
* @param deleteCacheClusterRequest Container for the necessary
* parameters to execute the DeleteCacheCluster operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DeleteCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheClusterAsync(DeleteCacheClusterRequest deleteCacheClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheCluster operation deletes a previously
* provisioned cache cluster. DeleteCacheCluster deletes all
* associated cache nodes, node endpoints and the cache cluster itself.
* When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the cache cluster; you cannot
* cancel or revert this operation.
*
*
* This API cannot be used to delete a cache cluster that is the last
* read replica of a replication group that has automatic failover mode
* enabled.
*
*
* @param deleteCacheClusterRequest Container for the necessary
* parameters to execute the DeleteCacheCluster operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheClusterAsync(DeleteCacheClusterRequest deleteCacheClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheSecurityGroup operation deletes a cache
* security group.
*
*
* NOTE:You cannot delete a cache security group if it is
* associated with any cache clusters.
*
*
* @param deleteCacheSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteCacheSecurityGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DeleteCacheSecurityGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheSecurityGroupAsync(DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheSecurityGroup operation deletes a cache
* security group.
*
*
* NOTE:You cannot delete a cache security group if it is
* associated with any cache clusters.
*
*
* @param deleteCacheSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteCacheSecurityGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteCacheSecurityGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheSecurityGroupAsync(DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheParameterGroup operation deletes the specified
* cache parameter group. You cannot delete a cache parameter group if it
* is associated with any cache clusters.
*
*
* @param deleteCacheParameterGroupRequest Container for the necessary
* parameters to execute the DeleteCacheParameterGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DeleteCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheParameterGroupAsync(DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheParameterGroup operation deletes the specified
* cache parameter group. You cannot delete a cache parameter group if it
* is associated with any cache clusters.
*
*
* @param deleteCacheParameterGroupRequest Container for the necessary
* parameters to execute the DeleteCacheParameterGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheParameterGroupAsync(DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateSnapshot operation creates a copy of an entire cache
* cluster at a specific moment in time.
*
*
* @param createSnapshotRequest Container for the necessary parameters to
* execute the CreateSnapshot operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CreateSnapshot service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateSnapshot operation creates a copy of an entire cache
* cluster at a specific moment in time.
*
*
* @param createSnapshotRequest Container for the necessary parameters to
* execute the CreateSnapshot operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateSnapshot service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ResetCacheParameterGroup operation modifies the parameters
* of a cache parameter group to the engine or system default value. You
* can reset specific parameters by submitting a list of parameter names.
* To reset the entire cache parameter group, specify the
* ResetAllParameters and CacheParameterGroupName
* parameters.
*
*
* @param resetCacheParameterGroupRequest Container for the necessary
* parameters to execute the ResetCacheParameterGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* ResetCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future resetCacheParameterGroupAsync(ResetCacheParameterGroupRequest resetCacheParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ResetCacheParameterGroup operation modifies the parameters
* of a cache parameter group to the engine or system default value. You
* can reset specific parameters by submitting a list of parameter names.
* To reset the entire cache parameter group, specify the
* ResetAllParameters and CacheParameterGroupName
* parameters.
*
*
* @param resetCacheParameterGroupRequest Container for the necessary
* parameters to execute the ResetCacheParameterGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ResetCacheParameterGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future resetCacheParameterGroupAsync(ResetCacheParameterGroupRequest resetCacheParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheSubnetGroup operation creates a new cache
* subnet group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon
* Virtual Private Cloud (VPC).
*
*
* @param createCacheSubnetGroupRequest Container for the necessary
* parameters to execute the CreateCacheSubnetGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CreateCacheSubnetGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheSubnetGroupAsync(CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheSubnetGroup operation creates a new cache
* subnet group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon
* Virtual Private Cloud (VPC).
*
*
* @param createCacheSubnetGroupRequest Container for the necessary
* parameters to execute the CreateCacheSubnetGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateCacheSubnetGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheSubnetGroupAsync(CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheCluster operation creates a cache cluster. All
* nodes in the cache cluster run the same protocol-compliant cache
* engine software, either Memcached or Redis.
*
*
* @param createCacheClusterRequest Container for the necessary
* parameters to execute the CreateCacheCluster operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CreateCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheClusterAsync(CreateCacheClusterRequest createCacheClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateCacheCluster operation creates a cache cluster. All
* nodes in the cache cluster run the same protocol-compliant cache
* engine software, either Memcached or Redis.
*
*
* @param createCacheClusterRequest Container for the necessary
* parameters to execute the CreateCacheCluster operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createCacheClusterAsync(CreateCacheClusterRequest createCacheClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheEngineVersions operation returns a list of
* the available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest Container for the necessary
* parameters to execute the DescribeCacheEngineVersions operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeCacheEngineVersions service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheEngineVersionsAsync(DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheEngineVersions operation returns a list of
* the available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest Container for the necessary
* parameters to execute the DescribeCacheEngineVersions operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCacheEngineVersions service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheEngineVersionsAsync(DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheParameters operation returns the detailed
* parameter list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest Container for the necessary
* parameters to execute the DescribeCacheParameters operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeCacheParameters service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheParametersAsync(DescribeCacheParametersRequest describeCacheParametersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheParameters operation returns the detailed
* parameter list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest Container for the necessary
* parameters to execute the DescribeCacheParameters operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCacheParameters service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheParametersAsync(DescribeCacheParametersRequest describeCacheParametersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeReservedCacheNodesOfferings operation lists
* available reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest Container for the
* necessary parameters to execute the
* DescribeReservedCacheNodesOfferings operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeReservedCacheNodesOfferings service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedCacheNodesOfferingsAsync(DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeReservedCacheNodesOfferings operation lists
* available reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest Container for the
* necessary parameters to execute the
* DescribeReservedCacheNodesOfferings operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReservedCacheNodesOfferings service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedCacheNodesOfferingsAsync(DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyCacheSubnetGroup operation modifies an existing
* cache subnet group.
*
*
* @param modifyCacheSubnetGroupRequest Container for the necessary
* parameters to execute the ModifyCacheSubnetGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* ModifyCacheSubnetGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyCacheSubnetGroupAsync(ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyCacheSubnetGroup operation modifies an existing
* cache subnet group.
*
*
* @param modifyCacheSubnetGroupRequest Container for the necessary
* parameters to execute the ModifyCacheSubnetGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyCacheSubnetGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyCacheSubnetGroupAsync(ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyReplicationGroup operation modifies the settings for
* a replication group.
*
*
* @param modifyReplicationGroupRequest Container for the necessary
* parameters to execute the ModifyReplicationGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* ModifyReplicationGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyReplicationGroupAsync(ModifyReplicationGroupRequest modifyReplicationGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ModifyReplicationGroup operation modifies the settings for
* a replication group.
*
*
* @param modifyReplicationGroupRequest Container for the necessary
* parameters to execute the ModifyReplicationGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyReplicationGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyReplicationGroupAsync(ModifyReplicationGroupRequest modifyReplicationGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CopySnapshot operation makes a copy of an existing
* snapshot.
*
*
* @param copySnapshotRequest Container for the necessary parameters to
* execute the CopySnapshot operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CopySnapshot service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CopySnapshot operation makes a copy of an existing
* snapshot.
*
*
* @param copySnapshotRequest Container for the necessary parameters to
* execute the CopySnapshot operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CopySnapshot service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeReplicationGroups operation returns information
* about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups returns information about all
* replication groups.
*
*
* @param describeReplicationGroupsRequest Container for the necessary
* parameters to execute the DescribeReplicationGroups operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeReplicationGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReplicationGroupsAsync(DescribeReplicationGroupsRequest describeReplicationGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeReplicationGroups operation returns information
* about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups returns information about all
* replication groups.
*
*
* @param describeReplicationGroupsRequest Container for the necessary
* parameters to execute the DescribeReplicationGroups operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReplicationGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReplicationGroupsAsync(DescribeReplicationGroupsRequest describeReplicationGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeEngineDefaultParameters operation returns the
* default engine and system parameter information for the specified
* cache engine.
*
*
* @param describeEngineDefaultParametersRequest Container for the
* necessary parameters to execute the DescribeEngineDefaultParameters
* operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeEngineDefaultParameters service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEngineDefaultParametersAsync(DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeEngineDefaultParameters operation returns the
* default engine and system parameter information for the specified
* cache engine.
*
*
* @param describeEngineDefaultParametersRequest Container for the
* necessary parameters to execute the DescribeEngineDefaultParameters
* operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeEngineDefaultParameters service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEngineDefaultParametersAsync(DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheSecurityGroups operation returns a list of
* cache security group descriptions. If a cache security group name is
* specified, the list will contain only the description of that group.
*
*
* @param describeCacheSecurityGroupsRequest Container for the necessary
* parameters to execute the DescribeCacheSecurityGroups operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeCacheSecurityGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheSecurityGroupsAsync(DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheSecurityGroups operation returns a list of
* cache security group descriptions. If a cache security group name is
* specified, the list will contain only the description of that group.
*
*
* @param describeCacheSecurityGroupsRequest Container for the necessary
* parameters to execute the DescribeCacheSecurityGroups operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCacheSecurityGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheSecurityGroupsAsync(DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The PurchaseReservedCacheNodesOffering operation allows you to
* purchase a reserved cache node offering.
*
*
* @param purchaseReservedCacheNodesOfferingRequest Container for the
* necessary parameters to execute the PurchaseReservedCacheNodesOffering
* operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* PurchaseReservedCacheNodesOffering service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future purchaseReservedCacheNodesOfferingAsync(PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The PurchaseReservedCacheNodesOffering operation allows you to
* purchase a reserved cache node offering.
*
*
* @param purchaseReservedCacheNodesOfferingRequest Container for the
* necessary parameters to execute the PurchaseReservedCacheNodesOffering
* operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* PurchaseReservedCacheNodesOffering service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future purchaseReservedCacheNodesOfferingAsync(PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheClusters operation returns information about
* all provisioned cache clusters if no cache cluster identifier is
* specified, or about a specific cache cluster if a cache cluster
* identifier is supplied.
*
*
* By default, abbreviated information about the cache clusters(s) will
* be returned. You can use the optional ShowDetails flag to
* retrieve detailed information about the cache nodes associated with
* the cache clusters. These details include the DNS address and port for
* the cache node endpoint.
*
*
* If the cluster is in the CREATING state, only cluster level
* information will be displayed until all of the nodes are successfully
* provisioned.
*
*
* If the cluster is in the DELETING state, only cluster level
* information will be displayed.
*
*
* If cache nodes are currently being added to the cache cluster, node
* endpoint information and creation time for the additional nodes will
* not be displayed until they are completely provisioned. When the cache
* cluster state is available , the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cache cluster, no
* endpoint information for the removed nodes is displayed.
*
*
* @param describeCacheClustersRequest Container for the necessary
* parameters to execute the DescribeCacheClusters operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeCacheClusters service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheClustersAsync(DescribeCacheClustersRequest describeCacheClustersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheClusters operation returns information about
* all provisioned cache clusters if no cache cluster identifier is
* specified, or about a specific cache cluster if a cache cluster
* identifier is supplied.
*
*
* By default, abbreviated information about the cache clusters(s) will
* be returned. You can use the optional ShowDetails flag to
* retrieve detailed information about the cache nodes associated with
* the cache clusters. These details include the DNS address and port for
* the cache node endpoint.
*
*
* If the cluster is in the CREATING state, only cluster level
* information will be displayed until all of the nodes are successfully
* provisioned.
*
*
* If the cluster is in the DELETING state, only cluster level
* information will be displayed.
*
*
* If cache nodes are currently being added to the cache cluster, node
* endpoint information and creation time for the additional nodes will
* not be displayed until they are completely provisioned. When the cache
* cluster state is available , the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cache cluster, no
* endpoint information for the removed nodes is displayed.
*
*
* @param describeCacheClustersRequest Container for the necessary
* parameters to execute the DescribeCacheClusters operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCacheClusters service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheClustersAsync(DescribeCacheClustersRequest describeCacheClustersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateReplicationGroup operation creates a replication
* group. A replication group is a collection of cache clusters, where
* one of the cache clusters is a read/write primary and the others are
* read-only replicas. Writes to the primary are automatically propagated
* to the replicas.
*
*
* When you create a replication group, you must specify an existing
* cache cluster that is in the primary role. When the replication group
* has been successfully created, you can add one or more read replica
* replicas to it, up to a total of five read replicas.
*
*
* Note: This action is valid only for Redis.
*
*
* @param createReplicationGroupRequest Container for the necessary
* parameters to execute the CreateReplicationGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* CreateReplicationGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createReplicationGroupAsync(CreateReplicationGroupRequest createReplicationGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateReplicationGroup operation creates a replication
* group. A replication group is a collection of cache clusters, where
* one of the cache clusters is a read/write primary and the others are
* read-only replicas. Writes to the primary are automatically propagated
* to the replicas.
*
*
* When you create a replication group, you must specify an existing
* cache cluster that is in the primary role. When the replication group
* has been successfully created, you can add one or more read replica
* replicas to it, up to a total of five read replicas.
*
*
* Note: This action is valid only for Redis.
*
*
* @param createReplicationGroupRequest Container for the necessary
* parameters to execute the CreateReplicationGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateReplicationGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createReplicationGroupAsync(CreateReplicationGroupRequest createReplicationGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The RevokeCacheSecurityGroupIngress operation revokes ingress
* from a cache security group. Use this operation to disallow access
* from an Amazon EC2 security group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest Container for the
* necessary parameters to execute the RevokeCacheSecurityGroupIngress
* operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* RevokeCacheSecurityGroupIngress service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future revokeCacheSecurityGroupIngressAsync(RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The RevokeCacheSecurityGroupIngress operation revokes ingress
* from a cache security group. Use this operation to disallow access
* from an Amazon EC2 security group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest Container for the
* necessary parameters to execute the RevokeCacheSecurityGroupIngress
* operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RevokeCacheSecurityGroupIngress service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future revokeCacheSecurityGroupIngressAsync(RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeSnapshots operation returns information about
* cache cluster snapshots. By default, DescribeSnapshots lists
* all of your snapshots; it can optionally describe a single snapshot,
* or just the snapshots associated with a particular cache cluster.
*
*
* @param describeSnapshotsRequest Container for the necessary parameters
* to execute the DescribeSnapshots operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeSnapshots service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeSnapshots operation returns information about
* cache cluster snapshots. By default, DescribeSnapshots lists
* all of your snapshots; it can optionally describe a single snapshot,
* or just the snapshots associated with a particular cache cluster.
*
*
* @param describeSnapshotsRequest Container for the necessary parameters
* to execute the DescribeSnapshots operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeSnapshots service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeEvents operation returns events related to cache
* clusters, cache security groups, and cache parameter groups. You can
* obtain events specific to a particular cache cluster, cache security
* group, or cache parameter group by providing the name as a parameter.
*
*
* By default, only the events occurring within the last hour are
* returned; however, you can retrieve up to 14 days' worth of events if
* necessary.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeEvents service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventsAsync(DescribeEventsRequest describeEventsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeEvents operation returns events related to cache
* clusters, cache security groups, and cache parameter groups. You can
* obtain events specific to a particular cache cluster, cache security
* group, or cache parameter group by providing the name as a parameter.
*
*
* By default, only the events occurring within the last hour are
* returned; however, you can retrieve up to 14 days' worth of events if
* necessary.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeEvents service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The RebootCacheCluster operation reboots some, or all, of the
* cache nodes within a provisioned cache cluster. This API will apply
* any modified cache parameter groups to the cache cluster. The reboot
* action takes place as soon as possible, and results in a momentary
* outage to the cache cluster. During the reboot, the cache cluster
* status is set to REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node
* being rebooted) to be lost.
*
*
* When the reboot is complete, a cache cluster event is created.
*
*
* @param rebootCacheClusterRequest Container for the necessary
* parameters to execute the RebootCacheCluster operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* RebootCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rebootCacheClusterAsync(RebootCacheClusterRequest rebootCacheClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The RebootCacheCluster operation reboots some, or all, of the
* cache nodes within a provisioned cache cluster. This API will apply
* any modified cache parameter groups to the cache cluster. The reboot
* action takes place as soon as possible, and results in a momentary
* outage to the cache cluster. During the reboot, the cache cluster
* status is set to REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node
* being rebooted) to be lost.
*
*
* When the reboot is complete, a cache cluster event is created.
*
*
* @param rebootCacheClusterRequest Container for the necessary
* parameters to execute the RebootCacheCluster operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RebootCacheCluster service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rebootCacheClusterAsync(RebootCacheClusterRequest rebootCacheClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteSnapshot operation deletes an existing snapshot.
* When you receive a successful response from this operation,
* ElastiCache immediately begins deleting the snapshot; you cannot
* cancel or revert this operation.
*
*
* @param deleteSnapshotRequest Container for the necessary parameters to
* execute the DeleteSnapshot operation on AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DeleteSnapshot service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteSnapshot operation deletes an existing snapshot.
* When you receive a successful response from this operation,
* ElastiCache immediately begins deleting the snapshot; you cannot
* cancel or revert this operation.
*
*
* @param deleteSnapshotRequest Container for the necessary parameters to
* execute the DeleteSnapshot operation on AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteSnapshot service method, as returned by AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheSubnetGroup operation deletes a cache subnet
* group.
*
*
* NOTE:You cannot delete a cache subnet group if it is
* associated with any cache clusters.
*
*
* @param deleteCacheSubnetGroupRequest Container for the necessary
* parameters to execute the DeleteCacheSubnetGroup operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DeleteCacheSubnetGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheSubnetGroupAsync(DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteCacheSubnetGroup operation deletes a cache subnet
* group.
*
*
* NOTE:You cannot delete a cache subnet group if it is
* associated with any cache clusters.
*
*
* @param deleteCacheSubnetGroupRequest Container for the necessary
* parameters to execute the DeleteCacheSubnetGroup operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteCacheSubnetGroup service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteCacheSubnetGroupAsync(DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheSubnetGroups operation returns a list of
* cache subnet group descriptions. If a subnet group name is specified,
* the list will contain only the description of that group.
*
*
* @param describeCacheSubnetGroupsRequest Container for the necessary
* parameters to execute the DescribeCacheSubnetGroups operation on
* AmazonElastiCache.
*
* @return A Java Future object containing the response from the
* DescribeCacheSubnetGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheSubnetGroupsAsync(DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DescribeCacheSubnetGroups operation returns a list of
* cache subnet group descriptions. If a subnet group name is specified,
* the list will contain only the description of that group.
*
*
* @param describeCacheSubnetGroupsRequest Container for the necessary
* parameters to execute the DescribeCacheSubnetGroups operation on
* AmazonElastiCache.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCacheSubnetGroups service method, as returned by
* AmazonElastiCache.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElastiCache indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCacheSubnetGroupsAsync(DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}