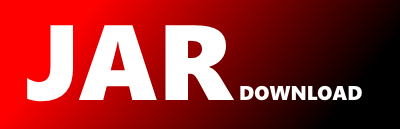
com.amazonaws.services.elasticache.model.CreateReplicationGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticache Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticache.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.elasticache.AmazonElastiCache#createReplicationGroup(CreateReplicationGroupRequest) CreateReplicationGroup operation}.
*
* The CreateReplicationGroup operation creates a replication
* group. A replication group is a collection of cache clusters, where
* one of the cache clusters is a read/write primary and the others are
* read-only replicas. Writes to the primary are automatically propagated
* to the replicas.
*
*
* When you create a replication group, you must specify an existing
* cache cluster that is in the primary role. When the replication group
* has been successfully created, you can add one or more read replica
* replicas to it, up to a total of five read replicas.
*
*
* Note: This action is valid only for Redis.
*
*
* @see com.amazonaws.services.elasticache.AmazonElastiCache#createReplicationGroup(CreateReplicationGroupRequest)
*/
public class CreateReplicationGroupRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The replication group identifier. This parameter is stored as a
* lowercase string. Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*/
private String replicationGroupId;
/**
* A user-created description for the replication group.
*/
private String replicationGroupDescription;
/**
* The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available. This parameter is not required if
* NumCacheClusters is specified.
*/
private String primaryClusterId;
/**
* Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*/
private Boolean automaticFailoverEnabled;
/**
* The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*/
private Integer numCacheClusters;
/**
* A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*/
private com.amazonaws.internal.ListWithAutoConstructFlag preferredCacheClusterAZs;
/**
* The compute and memory capacity of the nodes in the node group.
* Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*/
private String cacheNodeType;
/**
* The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*/
private String engine;
/**
* The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*/
private String engineVersion;
/**
* The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*/
private String cacheParameterGroupName;
/**
* The name of the cache subnet group to be used for the replication
* group.
*/
private String cacheSubnetGroupName;
/**
* A list of cache security group names to associate with this
* replication group.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag cacheSecurityGroupNames;
/**
* One or more Amazon VPC security groups associated with this
* replication group. Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*/
private com.amazonaws.internal.ListWithAutoConstructFlag securityGroupIds;
/**
* A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
* Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
private com.amazonaws.internal.ListWithAutoConstructFlag snapshotArns;
/**
* The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created. Note: This parameter is
* only valid if the Engine
parameter is redis
.
*/
private String snapshotName;
/**
* The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*/
private String preferredMaintenanceWindow;
/**
* The port number on which each member of the replication group will
* accept connections.
*/
private Integer port;
/**
* The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*/
private String notificationTopicArn;
/**
* Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*/
private Boolean autoMinorVersionUpgrade;
/**
* The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*/
private Integer snapshotRetentionLimit;
/**
* The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*/
private String snapshotWindow;
/**
* The replication group identifier. This parameter is stored as a
* lowercase string.
Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*
* @return The replication group identifier. This parameter is stored as a
* lowercase string. Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*/
public String getReplicationGroupId() {
return replicationGroupId;
}
/**
* The replication group identifier. This parameter is stored as a
* lowercase string. Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*
* @param replicationGroupId The replication group identifier. This parameter is stored as a
* lowercase string. Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*/
public void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
/**
* The replication group identifier. This parameter is stored as a
* lowercase string. Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param replicationGroupId The replication group identifier. This parameter is stored as a
* lowercase string.
Constraints:
- A name must contain from 1
* to 20 alphanumeric characters or hyphens.
- The first character
* must be a letter.
- A name cannot end with a hyphen or contain
* two consecutive hyphens.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
return this;
}
/**
* A user-created description for the replication group.
*
* @return A user-created description for the replication group.
*/
public String getReplicationGroupDescription() {
return replicationGroupDescription;
}
/**
* A user-created description for the replication group.
*
* @param replicationGroupDescription A user-created description for the replication group.
*/
public void setReplicationGroupDescription(String replicationGroupDescription) {
this.replicationGroupDescription = replicationGroupDescription;
}
/**
* A user-created description for the replication group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param replicationGroupDescription A user-created description for the replication group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withReplicationGroupDescription(String replicationGroupDescription) {
this.replicationGroupDescription = replicationGroupDescription;
return this;
}
/**
* The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available.
This parameter is not required if
* NumCacheClusters is specified.
*
* @return The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available.
This parameter is not required if
* NumCacheClusters is specified.
*/
public String getPrimaryClusterId() {
return primaryClusterId;
}
/**
* The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available.
This parameter is not required if
* NumCacheClusters is specified.
*
* @param primaryClusterId The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available.
This parameter is not required if
* NumCacheClusters is specified.
*/
public void setPrimaryClusterId(String primaryClusterId) {
this.primaryClusterId = primaryClusterId;
}
/**
* The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available.
This parameter is not required if
* NumCacheClusters is specified.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param primaryClusterId The identifier of the cache cluster that will serve as the primary for
* this replication group. This cache cluster must already exist and have
* a status of available.
This parameter is not required if
* NumCacheClusters is specified.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withPrimaryClusterId(String primaryClusterId) {
this.primaryClusterId = primaryClusterId;
return this;
}
/**
* Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*
* @return Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*/
public Boolean isAutomaticFailoverEnabled() {
return automaticFailoverEnabled;
}
/**
* Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*
* @param automaticFailoverEnabled Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*/
public void setAutomaticFailoverEnabled(Boolean automaticFailoverEnabled) {
this.automaticFailoverEnabled = automaticFailoverEnabled;
}
/**
* Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param automaticFailoverEnabled Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withAutomaticFailoverEnabled(Boolean automaticFailoverEnabled) {
this.automaticFailoverEnabled = automaticFailoverEnabled;
return this;
}
/**
* Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*
* @return Specifies whether a read-only replica will be automatically promoted
* to read/write primary if the existing primary fails.
If
* true
, automatic failover is enabled for this replication
* group. If false
, automatic failover is disabled for this
* replication group.
Default: false ElastiCache Multi-AZ
* replication groups is not supported on:
- Redis version
* 2.6.
- T1 and T2 cache node types.
*/
public Boolean getAutomaticFailoverEnabled() {
return automaticFailoverEnabled;
}
/**
* The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*
* @return The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*/
public Integer getNumCacheClusters() {
return numCacheClusters;
}
/**
* The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*
* @param numCacheClusters The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*/
public void setNumCacheClusters(Integer numCacheClusters) {
this.numCacheClusters = numCacheClusters;
}
/**
* The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param numCacheClusters The number of cache clusters this replication group will initially
* have.
If AutomaticFailover is enabled
, the
* value of this parameter must be at least 2.
The maximum permitted
* value for NumCacheClusters is 6 (primary plus 5 replicas). If
* you need to exceed this limit, please fill out the ElastiCache Limit
* Increase Request forrm at http://aws.amazon.com/contact-us/elasticache-node-limit-request.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withNumCacheClusters(Integer numCacheClusters) {
this.numCacheClusters = numCacheClusters;
return this;
}
/**
* A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*
* @return A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*/
public java.util.List getPreferredCacheClusterAZs() {
if (preferredCacheClusterAZs == null) {
preferredCacheClusterAZs = new com.amazonaws.internal.ListWithAutoConstructFlag();
preferredCacheClusterAZs.setAutoConstruct(true);
}
return preferredCacheClusterAZs;
}
/**
* A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*
* @param preferredCacheClusterAZs A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*/
public void setPreferredCacheClusterAZs(java.util.Collection preferredCacheClusterAZs) {
if (preferredCacheClusterAZs == null) {
this.preferredCacheClusterAZs = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag preferredCacheClusterAZsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(preferredCacheClusterAZs.size());
preferredCacheClusterAZsCopy.addAll(preferredCacheClusterAZs);
this.preferredCacheClusterAZs = preferredCacheClusterAZsCopy;
}
/**
* A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param preferredCacheClusterAZs A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withPreferredCacheClusterAZs(String... preferredCacheClusterAZs) {
if (getPreferredCacheClusterAZs() == null) setPreferredCacheClusterAZs(new java.util.ArrayList(preferredCacheClusterAZs.length));
for (String value : preferredCacheClusterAZs) {
getPreferredCacheClusterAZs().add(value);
}
return this;
}
/**
* A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param preferredCacheClusterAZs A list of EC2 availability zones in which the replication group's
* cache clusters will be created. The order of the availability zones in
* the list is not important. If you are creating your replication
* group in an Amazon VPC (recommended), you can only locate cache
* clusters in availability zones associated with the subnets in the
* selected subnet group. The number of availability zones listed must
* equal the value of NumCacheClusters.
Default: system
* chosen availability zones.
Example: One Redis cache cluster in each
* of three availability zones.
* PreferredAvailabilityZones.member.1=us-east-1a
* PreferredAvailabilityZones.member.2=us-east-1c
* PreferredAvailabilityZones.member.3=us-east-1d
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withPreferredCacheClusterAZs(java.util.Collection preferredCacheClusterAZs) {
if (preferredCacheClusterAZs == null) {
this.preferredCacheClusterAZs = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag preferredCacheClusterAZsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(preferredCacheClusterAZs.size());
preferredCacheClusterAZsCopy.addAll(preferredCacheClusterAZs);
this.preferredCacheClusterAZs = preferredCacheClusterAZsCopy;
}
return this;
}
/**
* The compute and memory capacity of the nodes in the node group.
* Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*
* @return The compute and memory capacity of the nodes in the node group.
*
Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*/
public String getCacheNodeType() {
return cacheNodeType;
}
/**
* The compute and memory capacity of the nodes in the node group.
*
Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*
* @param cacheNodeType The compute and memory capacity of the nodes in the node group.
*
Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*/
public void setCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
}
/**
* The compute and memory capacity of the nodes in the node group.
*
Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param cacheNodeType The compute and memory capacity of the nodes in the node group.
*
Valid node types are as follows:
- General purpose:
* - Current generation:
cache.t2.micro
,
* cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
* - Previous generation:
cache.t1.micro
,
* cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
- Compute optimized:
cache.c1.xlarge
* - Memory optimized
- Current generation:
*
cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
,
* cache.r3.8xlarge
- Previous generation:
*
cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
Notes:
*
- All t2 instances are created in an Amazon Virtual Private
* Cloud (VPC).
- Redis backup/restore is not supported for t2
* instances.
- Redis Append-only files (AOF) functionality is not
* supported for t1 or t2 instances.
For a complete listing
* of cache node types and specifications, see Amazon ElastiCache
* Product Features and Details and Cache
* Node Type-Specific Parameters for Memcached or Cache
* Node Type-Specific Parameters for Redis.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
return this;
}
/**
* The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*
* @return The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*/
public String getEngine() {
return engine;
}
/**
* The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*
* @param engine The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
* The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param engine The name of the cache engine to be used for the cache clusters in this
* replication group.
Default: redis
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withEngine(String engine) {
this.engine = engine;
return this;
}
/**
* The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* @return The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*/
public String getEngineVersion() {
return engineVersion;
}
/**
* The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* @param engineVersion The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
* The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param engineVersion The version number of the cach engine to be used for the cache
* clusters in this replication group. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
/**
* The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*
* @return The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*/
public String getCacheParameterGroupName() {
return cacheParameterGroupName;
}
/**
* The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*
* @param cacheParameterGroupName The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*/
public void setCacheParameterGroupName(String cacheParameterGroupName) {
this.cacheParameterGroupName = cacheParameterGroupName;
}
/**
* The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param cacheParameterGroupName The name of the parameter group to associate with this replication
* group. If this argument is omitted, the default cache parameter group
* for the specified engine is used.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withCacheParameterGroupName(String cacheParameterGroupName) {
this.cacheParameterGroupName = cacheParameterGroupName;
return this;
}
/**
* The name of the cache subnet group to be used for the replication
* group.
*
* @return The name of the cache subnet group to be used for the replication
* group.
*/
public String getCacheSubnetGroupName() {
return cacheSubnetGroupName;
}
/**
* The name of the cache subnet group to be used for the replication
* group.
*
* @param cacheSubnetGroupName The name of the cache subnet group to be used for the replication
* group.
*/
public void setCacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
}
/**
* The name of the cache subnet group to be used for the replication
* group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param cacheSubnetGroupName The name of the cache subnet group to be used for the replication
* group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withCacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
return this;
}
/**
* A list of cache security group names to associate with this
* replication group.
*
* @return A list of cache security group names to associate with this
* replication group.
*/
public java.util.List getCacheSecurityGroupNames() {
if (cacheSecurityGroupNames == null) {
cacheSecurityGroupNames = new com.amazonaws.internal.ListWithAutoConstructFlag();
cacheSecurityGroupNames.setAutoConstruct(true);
}
return cacheSecurityGroupNames;
}
/**
* A list of cache security group names to associate with this
* replication group.
*
* @param cacheSecurityGroupNames A list of cache security group names to associate with this
* replication group.
*/
public void setCacheSecurityGroupNames(java.util.Collection cacheSecurityGroupNames) {
if (cacheSecurityGroupNames == null) {
this.cacheSecurityGroupNames = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag cacheSecurityGroupNamesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(cacheSecurityGroupNames.size());
cacheSecurityGroupNamesCopy.addAll(cacheSecurityGroupNames);
this.cacheSecurityGroupNames = cacheSecurityGroupNamesCopy;
}
/**
* A list of cache security group names to associate with this
* replication group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param cacheSecurityGroupNames A list of cache security group names to associate with this
* replication group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withCacheSecurityGroupNames(String... cacheSecurityGroupNames) {
if (getCacheSecurityGroupNames() == null) setCacheSecurityGroupNames(new java.util.ArrayList(cacheSecurityGroupNames.length));
for (String value : cacheSecurityGroupNames) {
getCacheSecurityGroupNames().add(value);
}
return this;
}
/**
* A list of cache security group names to associate with this
* replication group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param cacheSecurityGroupNames A list of cache security group names to associate with this
* replication group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withCacheSecurityGroupNames(java.util.Collection cacheSecurityGroupNames) {
if (cacheSecurityGroupNames == null) {
this.cacheSecurityGroupNames = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag cacheSecurityGroupNamesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(cacheSecurityGroupNames.size());
cacheSecurityGroupNamesCopy.addAll(cacheSecurityGroupNames);
this.cacheSecurityGroupNames = cacheSecurityGroupNamesCopy;
}
return this;
}
/**
* One or more Amazon VPC security groups associated with this
* replication group. Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*
* @return One or more Amazon VPC security groups associated with this
* replication group.
Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.ListWithAutoConstructFlag();
securityGroupIds.setAutoConstruct(true);
}
return securityGroupIds;
}
/**
* One or more Amazon VPC security groups associated with this
* replication group. Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*
* @param securityGroupIds One or more Amazon VPC security groups associated with this
* replication group.
Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag securityGroupIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(securityGroupIds.size());
securityGroupIdsCopy.addAll(securityGroupIds);
this.securityGroupIds = securityGroupIdsCopy;
}
/**
* One or more Amazon VPC security groups associated with this
* replication group. Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param securityGroupIds One or more Amazon VPC security groups associated with this
* replication group.
Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSecurityGroupIds(String... securityGroupIds) {
if (getSecurityGroupIds() == null) setSecurityGroupIds(new java.util.ArrayList(securityGroupIds.length));
for (String value : securityGroupIds) {
getSecurityGroupIds().add(value);
}
return this;
}
/**
* One or more Amazon VPC security groups associated with this
* replication group. Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param securityGroupIds One or more Amazon VPC security groups associated with this
* replication group.
Use this parameter only when you are creating a
* replication group in an Amazon Virtual Private Cloud (VPC).
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag securityGroupIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(securityGroupIds.size());
securityGroupIdsCopy.addAll(securityGroupIds);
this.securityGroupIds = securityGroupIdsCopy;
}
return this;
}
/**
* A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
* Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
* @return A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
public java.util.List getSnapshotArns() {
if (snapshotArns == null) {
snapshotArns = new com.amazonaws.internal.ListWithAutoConstructFlag();
snapshotArns.setAutoConstruct(true);
}
return snapshotArns;
}
/**
* A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
* Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
* @param snapshotArns A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
public void setSnapshotArns(java.util.Collection snapshotArns) {
if (snapshotArns == null) {
this.snapshotArns = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag snapshotArnsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(snapshotArns.size());
snapshotArnsCopy.addAll(snapshotArns);
this.snapshotArns = snapshotArnsCopy;
}
/**
* A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
* Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param snapshotArns A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSnapshotArns(String... snapshotArns) {
if (getSnapshotArns() == null) setSnapshotArns(new java.util.ArrayList(snapshotArns.length));
for (String value : snapshotArns) {
getSnapshotArns().add(value);
}
return this;
}
/**
* A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
* Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param snapshotArns A single-element string list containing an Amazon Resource Name (ARN)
* that uniquely identifies a Redis RDB snapshot file stored in Amazon
* S3. The snapshot file will be used to populate the node group. The
* Amazon S3 object name in the ARN cannot contain any commas.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Example of an
* Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSnapshotArns(java.util.Collection snapshotArns) {
if (snapshotArns == null) {
this.snapshotArns = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag snapshotArnsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(snapshotArns.size());
snapshotArnsCopy.addAll(snapshotArns);
this.snapshotArns = snapshotArnsCopy;
}
return this;
}
/**
* The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created. Note: This parameter is
* only valid if the Engine
parameter is redis
.
*
* @return The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created.
Note: This parameter is
* only valid if the Engine
parameter is redis
.
*/
public String getSnapshotName() {
return snapshotName;
}
/**
* The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created.
Note: This parameter is
* only valid if the Engine
parameter is redis
.
*
* @param snapshotName The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created.
Note: This parameter is
* only valid if the Engine
parameter is redis
.
*/
public void setSnapshotName(String snapshotName) {
this.snapshotName = snapshotName;
}
/**
* The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created.
Note: This parameter is
* only valid if the Engine
parameter is redis
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param snapshotName The name of a snapshot from which to restore data into the new node
* group. The snapshot status changes to restoring
while the
* new node group is being created.
Note: This parameter is
* only valid if the Engine
parameter is redis
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSnapshotName(String snapshotName) {
this.snapshotName = snapshotName;
return this;
}
/**
* The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*
* @return The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*/
public String getPreferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
* The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*
* @param preferredMaintenanceWindow The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
* The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param preferredMaintenanceWindow The weekly time range (in UTC) during which system maintenance can
* occur.
Example: sun:05:00-sun:09:00
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
/**
* The port number on which each member of the replication group will
* accept connections.
*
* @return The port number on which each member of the replication group will
* accept connections.
*/
public Integer getPort() {
return port;
}
/**
* The port number on which each member of the replication group will
* accept connections.
*
* @param port The port number on which each member of the replication group will
* accept connections.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
* The port number on which each member of the replication group will
* accept connections.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param port The port number on which each member of the replication group will
* accept connections.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withPort(Integer port) {
this.port = port;
return this;
}
/**
* The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*
* @return The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*/
public String getNotificationTopicArn() {
return notificationTopicArn;
}
/**
* The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*
* @param notificationTopicArn The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*/
public void setNotificationTopicArn(String notificationTopicArn) {
this.notificationTopicArn = notificationTopicArn;
}
/**
* The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param notificationTopicArn The Amazon Resource Name (ARN) of the Amazon Simple Notification
* Service (SNS) topic to which notifications will be sent. The
* Amazon SNS topic owner must be the same as the cache cluster
* owner.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withNotificationTopicArn(String notificationTopicArn) {
this.notificationTopicArn = notificationTopicArn;
return this;
}
/**
* Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*
* @return Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*/
public Boolean isAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
* Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*
* @param autoMinorVersionUpgrade Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
* Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param autoMinorVersionUpgrade Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
/**
* Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*
* @return Determines whether minor engine upgrades will be applied automatically
* to the node group during the maintenance window. A value of
* true
allows these upgrades to occur; false
* disables automatic upgrades.
Default: true
*/
public Boolean getAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
* The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*
* @return The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*/
public Integer getSnapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
* The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*
* @param snapshotRetentionLimit The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*/
public void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
/**
* The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param snapshotRetentionLimit The number of days for which ElastiCache will retain automatic
* snapshots before deleting them. For example, if you set
* SnapshotRetentionLimit
to 5, then a snapshot that was
* taken today will be retained for 5 days before being deleted.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
Default: 0
* (i.e., automatic backups are disabled for this cache cluster).
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
return this;
}
/**
* The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*
* @return The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*/
public String getSnapshotWindow() {
return snapshotWindow;
}
/**
* The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*
* @param snapshotWindow The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*/
public void setSnapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
}
/**
* The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param snapshotWindow The daily time range (in UTC) during which ElastiCache will begin
* taking a daily snapshot of your node group.
Example:
* 05:00-09:00
If you do not specify this parameter, then
* ElastiCache will automatically choose an appropriate time range.
*
Note: This parameter is only valid if the
* Engine
parameter is redis
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateReplicationGroupRequest withSnapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationGroupId() != null) sb.append("ReplicationGroupId: " + getReplicationGroupId() + ",");
if (getReplicationGroupDescription() != null) sb.append("ReplicationGroupDescription: " + getReplicationGroupDescription() + ",");
if (getPrimaryClusterId() != null) sb.append("PrimaryClusterId: " + getPrimaryClusterId() + ",");
if (isAutomaticFailoverEnabled() != null) sb.append("AutomaticFailoverEnabled: " + isAutomaticFailoverEnabled() + ",");
if (getNumCacheClusters() != null) sb.append("NumCacheClusters: " + getNumCacheClusters() + ",");
if (getPreferredCacheClusterAZs() != null) sb.append("PreferredCacheClusterAZs: " + getPreferredCacheClusterAZs() + ",");
if (getCacheNodeType() != null) sb.append("CacheNodeType: " + getCacheNodeType() + ",");
if (getEngine() != null) sb.append("Engine: " + getEngine() + ",");
if (getEngineVersion() != null) sb.append("EngineVersion: " + getEngineVersion() + ",");
if (getCacheParameterGroupName() != null) sb.append("CacheParameterGroupName: " + getCacheParameterGroupName() + ",");
if (getCacheSubnetGroupName() != null) sb.append("CacheSubnetGroupName: " + getCacheSubnetGroupName() + ",");
if (getCacheSecurityGroupNames() != null) sb.append("CacheSecurityGroupNames: " + getCacheSecurityGroupNames() + ",");
if (getSecurityGroupIds() != null) sb.append("SecurityGroupIds: " + getSecurityGroupIds() + ",");
if (getSnapshotArns() != null) sb.append("SnapshotArns: " + getSnapshotArns() + ",");
if (getSnapshotName() != null) sb.append("SnapshotName: " + getSnapshotName() + ",");
if (getPreferredMaintenanceWindow() != null) sb.append("PreferredMaintenanceWindow: " + getPreferredMaintenanceWindow() + ",");
if (getPort() != null) sb.append("Port: " + getPort() + ",");
if (getNotificationTopicArn() != null) sb.append("NotificationTopicArn: " + getNotificationTopicArn() + ",");
if (isAutoMinorVersionUpgrade() != null) sb.append("AutoMinorVersionUpgrade: " + isAutoMinorVersionUpgrade() + ",");
if (getSnapshotRetentionLimit() != null) sb.append("SnapshotRetentionLimit: " + getSnapshotRetentionLimit() + ",");
if (getSnapshotWindow() != null) sb.append("SnapshotWindow: " + getSnapshotWindow() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationGroupId() == null) ? 0 : getReplicationGroupId().hashCode());
hashCode = prime * hashCode + ((getReplicationGroupDescription() == null) ? 0 : getReplicationGroupDescription().hashCode());
hashCode = prime * hashCode + ((getPrimaryClusterId() == null) ? 0 : getPrimaryClusterId().hashCode());
hashCode = prime * hashCode + ((isAutomaticFailoverEnabled() == null) ? 0 : isAutomaticFailoverEnabled().hashCode());
hashCode = prime * hashCode + ((getNumCacheClusters() == null) ? 0 : getNumCacheClusters().hashCode());
hashCode = prime * hashCode + ((getPreferredCacheClusterAZs() == null) ? 0 : getPreferredCacheClusterAZs().hashCode());
hashCode = prime * hashCode + ((getCacheNodeType() == null) ? 0 : getCacheNodeType().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getCacheParameterGroupName() == null) ? 0 : getCacheParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getCacheSubnetGroupName() == null) ? 0 : getCacheSubnetGroupName().hashCode());
hashCode = prime * hashCode + ((getCacheSecurityGroupNames() == null) ? 0 : getCacheSecurityGroupNames().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getSnapshotArns() == null) ? 0 : getSnapshotArns().hashCode());
hashCode = prime * hashCode + ((getSnapshotName() == null) ? 0 : getSnapshotName().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getNotificationTopicArn() == null) ? 0 : getNotificationTopicArn().hashCode());
hashCode = prime * hashCode + ((isAutoMinorVersionUpgrade() == null) ? 0 : isAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getSnapshotRetentionLimit() == null) ? 0 : getSnapshotRetentionLimit().hashCode());
hashCode = prime * hashCode + ((getSnapshotWindow() == null) ? 0 : getSnapshotWindow().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof CreateReplicationGroupRequest == false) return false;
CreateReplicationGroupRequest other = (CreateReplicationGroupRequest)obj;
if (other.getReplicationGroupId() == null ^ this.getReplicationGroupId() == null) return false;
if (other.getReplicationGroupId() != null && other.getReplicationGroupId().equals(this.getReplicationGroupId()) == false) return false;
if (other.getReplicationGroupDescription() == null ^ this.getReplicationGroupDescription() == null) return false;
if (other.getReplicationGroupDescription() != null && other.getReplicationGroupDescription().equals(this.getReplicationGroupDescription()) == false) return false;
if (other.getPrimaryClusterId() == null ^ this.getPrimaryClusterId() == null) return false;
if (other.getPrimaryClusterId() != null && other.getPrimaryClusterId().equals(this.getPrimaryClusterId()) == false) return false;
if (other.isAutomaticFailoverEnabled() == null ^ this.isAutomaticFailoverEnabled() == null) return false;
if (other.isAutomaticFailoverEnabled() != null && other.isAutomaticFailoverEnabled().equals(this.isAutomaticFailoverEnabled()) == false) return false;
if (other.getNumCacheClusters() == null ^ this.getNumCacheClusters() == null) return false;
if (other.getNumCacheClusters() != null && other.getNumCacheClusters().equals(this.getNumCacheClusters()) == false) return false;
if (other.getPreferredCacheClusterAZs() == null ^ this.getPreferredCacheClusterAZs() == null) return false;
if (other.getPreferredCacheClusterAZs() != null && other.getPreferredCacheClusterAZs().equals(this.getPreferredCacheClusterAZs()) == false) return false;
if (other.getCacheNodeType() == null ^ this.getCacheNodeType() == null) return false;
if (other.getCacheNodeType() != null && other.getCacheNodeType().equals(this.getCacheNodeType()) == false) return false;
if (other.getEngine() == null ^ this.getEngine() == null) return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false) return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null) return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false) return false;
if (other.getCacheParameterGroupName() == null ^ this.getCacheParameterGroupName() == null) return false;
if (other.getCacheParameterGroupName() != null && other.getCacheParameterGroupName().equals(this.getCacheParameterGroupName()) == false) return false;
if (other.getCacheSubnetGroupName() == null ^ this.getCacheSubnetGroupName() == null) return false;
if (other.getCacheSubnetGroupName() != null && other.getCacheSubnetGroupName().equals(this.getCacheSubnetGroupName()) == false) return false;
if (other.getCacheSecurityGroupNames() == null ^ this.getCacheSecurityGroupNames() == null) return false;
if (other.getCacheSecurityGroupNames() != null && other.getCacheSecurityGroupNames().equals(this.getCacheSecurityGroupNames()) == false) return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null) return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false) return false;
if (other.getSnapshotArns() == null ^ this.getSnapshotArns() == null) return false;
if (other.getSnapshotArns() != null && other.getSnapshotArns().equals(this.getSnapshotArns()) == false) return false;
if (other.getSnapshotName() == null ^ this.getSnapshotName() == null) return false;
if (other.getSnapshotName() != null && other.getSnapshotName().equals(this.getSnapshotName()) == false) return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null) return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false) return false;
if (other.getPort() == null ^ this.getPort() == null) return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false) return false;
if (other.getNotificationTopicArn() == null ^ this.getNotificationTopicArn() == null) return false;
if (other.getNotificationTopicArn() != null && other.getNotificationTopicArn().equals(this.getNotificationTopicArn()) == false) return false;
if (other.isAutoMinorVersionUpgrade() == null ^ this.isAutoMinorVersionUpgrade() == null) return false;
if (other.isAutoMinorVersionUpgrade() != null && other.isAutoMinorVersionUpgrade().equals(this.isAutoMinorVersionUpgrade()) == false) return false;
if (other.getSnapshotRetentionLimit() == null ^ this.getSnapshotRetentionLimit() == null) return false;
if (other.getSnapshotRetentionLimit() != null && other.getSnapshotRetentionLimit().equals(this.getSnapshotRetentionLimit()) == false) return false;
if (other.getSnapshotWindow() == null ^ this.getSnapshotWindow() == null) return false;
if (other.getSnapshotWindow() != null && other.getSnapshotWindow().equals(this.getSnapshotWindow()) == false) return false;
return true;
}
}