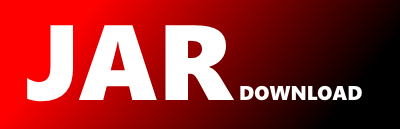
com.amazonaws.services.elasticbeanstalk.model.PlatformBranchSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticbeanstalk Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Summary information about a platform branch.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PlatformBranchSummary implements Serializable, Cloneable {
/**
*
* The name of the platform to which this platform branch belongs.
*
*/
private String platformName;
/**
*
* The name of the platform branch.
*
*/
private String branchName;
/**
*
* The support life cycle state of the platform branch.
*
*
* Possible values: beta
| supported
| deprecated
| retired
*
*/
private String lifecycleState;
/**
*
* An ordinal number that designates the order in which platform branches have been added to a platform. This can be
* helpful, for example, if your code calls the ListPlatformBranches
action and then displays a list of
* platform branches.
*
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*
*/
private Integer branchOrder;
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*/
private com.amazonaws.internal.SdkInternalList supportedTierList;
/**
*
* The name of the platform to which this platform branch belongs.
*
*
* @param platformName
* The name of the platform to which this platform branch belongs.
*/
public void setPlatformName(String platformName) {
this.platformName = platformName;
}
/**
*
* The name of the platform to which this platform branch belongs.
*
*
* @return The name of the platform to which this platform branch belongs.
*/
public String getPlatformName() {
return this.platformName;
}
/**
*
* The name of the platform to which this platform branch belongs.
*
*
* @param platformName
* The name of the platform to which this platform branch belongs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlatformBranchSummary withPlatformName(String platformName) {
setPlatformName(platformName);
return this;
}
/**
*
* The name of the platform branch.
*
*
* @param branchName
* The name of the platform branch.
*/
public void setBranchName(String branchName) {
this.branchName = branchName;
}
/**
*
* The name of the platform branch.
*
*
* @return The name of the platform branch.
*/
public String getBranchName() {
return this.branchName;
}
/**
*
* The name of the platform branch.
*
*
* @param branchName
* The name of the platform branch.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlatformBranchSummary withBranchName(String branchName) {
setBranchName(branchName);
return this;
}
/**
*
* The support life cycle state of the platform branch.
*
*
* Possible values: beta
| supported
| deprecated
| retired
*
*
* @param lifecycleState
* The support life cycle state of the platform branch.
*
* Possible values: beta
| supported
| deprecated
|
* retired
*/
public void setLifecycleState(String lifecycleState) {
this.lifecycleState = lifecycleState;
}
/**
*
* The support life cycle state of the platform branch.
*
*
* Possible values: beta
| supported
| deprecated
| retired
*
*
* @return The support life cycle state of the platform branch.
*
* Possible values: beta
| supported
| deprecated
|
* retired
*/
public String getLifecycleState() {
return this.lifecycleState;
}
/**
*
* The support life cycle state of the platform branch.
*
*
* Possible values: beta
| supported
| deprecated
| retired
*
*
* @param lifecycleState
* The support life cycle state of the platform branch.
*
* Possible values: beta
| supported
| deprecated
|
* retired
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlatformBranchSummary withLifecycleState(String lifecycleState) {
setLifecycleState(lifecycleState);
return this;
}
/**
*
* An ordinal number that designates the order in which platform branches have been added to a platform. This can be
* helpful, for example, if your code calls the ListPlatformBranches
action and then displays a list of
* platform branches.
*
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*
*
* @param branchOrder
* An ordinal number that designates the order in which platform branches have been added to a platform. This
* can be helpful, for example, if your code calls the ListPlatformBranches
action and then
* displays a list of platform branches.
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*/
public void setBranchOrder(Integer branchOrder) {
this.branchOrder = branchOrder;
}
/**
*
* An ordinal number that designates the order in which platform branches have been added to a platform. This can be
* helpful, for example, if your code calls the ListPlatformBranches
action and then displays a list of
* platform branches.
*
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*
*
* @return An ordinal number that designates the order in which platform branches have been added to a platform.
* This can be helpful, for example, if your code calls the ListPlatformBranches
action and
* then displays a list of platform branches.
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*/
public Integer getBranchOrder() {
return this.branchOrder;
}
/**
*
* An ordinal number that designates the order in which platform branches have been added to a platform. This can be
* helpful, for example, if your code calls the ListPlatformBranches
action and then displays a list of
* platform branches.
*
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*
*
* @param branchOrder
* An ordinal number that designates the order in which platform branches have been added to a platform. This
* can be helpful, for example, if your code calls the ListPlatformBranches
action and then
* displays a list of platform branches.
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlatformBranchSummary withBranchOrder(Integer branchOrder) {
setBranchOrder(branchOrder);
return this;
}
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* @return The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*/
public java.util.List getSupportedTierList() {
if (supportedTierList == null) {
supportedTierList = new com.amazonaws.internal.SdkInternalList();
}
return supportedTierList;
}
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* @param supportedTierList
* The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*/
public void setSupportedTierList(java.util.Collection supportedTierList) {
if (supportedTierList == null) {
this.supportedTierList = null;
return;
}
this.supportedTierList = new com.amazonaws.internal.SdkInternalList(supportedTierList);
}
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedTierList(java.util.Collection)} or {@link #withSupportedTierList(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param supportedTierList
* The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlatformBranchSummary withSupportedTierList(String... supportedTierList) {
if (this.supportedTierList == null) {
setSupportedTierList(new com.amazonaws.internal.SdkInternalList(supportedTierList.length));
}
for (String ele : supportedTierList) {
this.supportedTierList.add(ele);
}
return this;
}
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* @param supportedTierList
* The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlatformBranchSummary withSupportedTierList(java.util.Collection supportedTierList) {
setSupportedTierList(supportedTierList);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPlatformName() != null)
sb.append("PlatformName: ").append(getPlatformName()).append(",");
if (getBranchName() != null)
sb.append("BranchName: ").append(getBranchName()).append(",");
if (getLifecycleState() != null)
sb.append("LifecycleState: ").append(getLifecycleState()).append(",");
if (getBranchOrder() != null)
sb.append("BranchOrder: ").append(getBranchOrder()).append(",");
if (getSupportedTierList() != null)
sb.append("SupportedTierList: ").append(getSupportedTierList());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PlatformBranchSummary == false)
return false;
PlatformBranchSummary other = (PlatformBranchSummary) obj;
if (other.getPlatformName() == null ^ this.getPlatformName() == null)
return false;
if (other.getPlatformName() != null && other.getPlatformName().equals(this.getPlatformName()) == false)
return false;
if (other.getBranchName() == null ^ this.getBranchName() == null)
return false;
if (other.getBranchName() != null && other.getBranchName().equals(this.getBranchName()) == false)
return false;
if (other.getLifecycleState() == null ^ this.getLifecycleState() == null)
return false;
if (other.getLifecycleState() != null && other.getLifecycleState().equals(this.getLifecycleState()) == false)
return false;
if (other.getBranchOrder() == null ^ this.getBranchOrder() == null)
return false;
if (other.getBranchOrder() != null && other.getBranchOrder().equals(this.getBranchOrder()) == false)
return false;
if (other.getSupportedTierList() == null ^ this.getSupportedTierList() == null)
return false;
if (other.getSupportedTierList() != null && other.getSupportedTierList().equals(this.getSupportedTierList()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPlatformName() == null) ? 0 : getPlatformName().hashCode());
hashCode = prime * hashCode + ((getBranchName() == null) ? 0 : getBranchName().hashCode());
hashCode = prime * hashCode + ((getLifecycleState() == null) ? 0 : getLifecycleState().hashCode());
hashCode = prime * hashCode + ((getBranchOrder() == null) ? 0 : getBranchOrder().hashCode());
hashCode = prime * hashCode + ((getSupportedTierList() == null) ? 0 : getSupportedTierList().hashCode());
return hashCode;
}
@Override
public PlatformBranchSummary clone() {
try {
return (PlatformBranchSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}