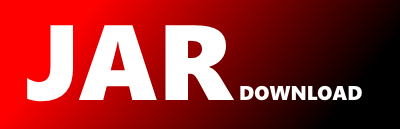
com.amazonaws.services.elasticbeanstalk.AWSElasticBeanstalk Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticbeanstalk Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.elasticbeanstalk.model.*;
import com.amazonaws.services.elasticbeanstalk.waiters.AWSElasticBeanstalkWaiters;
/**
* Interface for accessing Elastic Beanstalk.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.elasticbeanstalk.AbstractAWSElasticBeanstalk} instead.
*
*
* AWS Elastic Beanstalk
*
* AWS Elastic Beanstalk makes it easy for you to create, deploy, and manage scalable, fault-tolerant applications
* running on the Amazon Web Services cloud.
*
*
* For more information about this product, go to the AWS Elastic
* Beanstalk details page. The location of the latest AWS Elastic Beanstalk WSDL is https://elasticbeanstalk.s3.amazonaws.com/doc/2010-12-01/AWSElasticBeanstalk.wsdl. To install the Software
* Development Kits (SDKs), Integrated Development Environment (IDE) Toolkits, and command line tools that enable you to
* access the API, go to Tools for Amazon Web Services.
*
*
* Endpoints
*
*
* For a list of region-specific endpoints that AWS Elastic Beanstalk supports, go to Regions and Endpoints in
* the Amazon Web Services Glossary.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSElasticBeanstalk {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "elasticbeanstalk";
/**
* Overrides the default endpoint for this client ("https://elasticbeanstalk.us-east-1.amazonaws.com"). Callers can
* use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "elasticbeanstalk.us-east-1.amazonaws.com") or a full URL, including
* the protocol (ex: "https://elasticbeanstalk.us-east-1.amazonaws.com"). If the protocol is not specified here, the
* default protocol from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "elasticbeanstalk.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://elasticbeanstalk.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSElasticBeanstalk#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Cancels in-progress environment configuration update or application version deployment.
*
*
* @param abortEnvironmentUpdateRequest
* @return Result of the AbortEnvironmentUpdate operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.AbortEnvironmentUpdate
* @see AWS API Documentation
*/
AbortEnvironmentUpdateResult abortEnvironmentUpdate(AbortEnvironmentUpdateRequest abortEnvironmentUpdateRequest);
/**
* Simplified method form for invoking the AbortEnvironmentUpdate operation.
*
* @see #abortEnvironmentUpdate(AbortEnvironmentUpdateRequest)
*/
AbortEnvironmentUpdateResult abortEnvironmentUpdate();
/**
*
* Applies a scheduled managed action immediately. A managed action can be applied only if its status is
* Scheduled
. Get the status and action ID of a managed action with
* DescribeEnvironmentManagedActions.
*
*
* @param applyEnvironmentManagedActionRequest
* Request to execute a scheduled managed action immediately.
* @return Result of the ApplyEnvironmentManagedAction operation returned by the service.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @throws ManagedActionInvalidStateException
* Cannot modify the managed action in its current state.
* @sample AWSElasticBeanstalk.ApplyEnvironmentManagedAction
* @see AWS API Documentation
*/
ApplyEnvironmentManagedActionResult applyEnvironmentManagedAction(ApplyEnvironmentManagedActionRequest applyEnvironmentManagedActionRequest);
/**
*
* Add or change the operations role used by an environment. After this call is made, Elastic Beanstalk uses the
* associated operations role for permissions to downstream services during subsequent calls acting on this
* environment. For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
* @param associateEnvironmentOperationsRoleRequest
* Request to add or change the operations role used by an environment.
* @return Result of the AssociateEnvironmentOperationsRole operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.AssociateEnvironmentOperationsRole
* @see AWS API Documentation
*/
AssociateEnvironmentOperationsRoleResult associateEnvironmentOperationsRole(
AssociateEnvironmentOperationsRoleRequest associateEnvironmentOperationsRoleRequest);
/**
*
* Checks if the specified CNAME is available.
*
*
* @param checkDNSAvailabilityRequest
* Results message indicating whether a CNAME is available.
* @return Result of the CheckDNSAvailability operation returned by the service.
* @sample AWSElasticBeanstalk.CheckDNSAvailability
* @see AWS API Documentation
*/
CheckDNSAvailabilityResult checkDNSAvailability(CheckDNSAvailabilityRequest checkDNSAvailabilityRequest);
/**
*
* Create or update a group of environments that each run a separate component of a single application. Takes a list
* of version labels that specify application source bundles for each of the environments to create or update. The
* name of each environment and other required information must be included in the source bundles in an environment
* manifest named env.yaml
. See Compose
* Environments for details.
*
*
* @param composeEnvironmentsRequest
* Request to create or update a group of environments.
* @return Result of the ComposeEnvironments operation returned by the service.
* @throws TooManyEnvironmentsException
* The specified account has reached its limit of environments.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.ComposeEnvironments
* @see AWS API Documentation
*/
ComposeEnvironmentsResult composeEnvironments(ComposeEnvironmentsRequest composeEnvironmentsRequest);
/**
*
* Creates an application that has one configuration template named default
and no application
* versions.
*
*
* @param createApplicationRequest
* Request to create an application.
* @return Result of the CreateApplication operation returned by the service.
* @throws TooManyApplicationsException
* The specified account has reached its limit of applications.
* @sample AWSElasticBeanstalk.CreateApplication
* @see AWS API Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates an application version for the specified application. You can create an application version from a source
* bundle in Amazon S3, a commit in AWS CodeCommit, or the output of an AWS CodeBuild build as follows:
*
*
* Specify a commit in an AWS CodeCommit repository with SourceBuildInformation
.
*
*
* Specify a build in an AWS CodeBuild with SourceBuildInformation
and BuildConfiguration
.
*
*
* Specify a source bundle in S3 with SourceBundle
*
*
* Omit both SourceBuildInformation
and SourceBundle
to use the default sample
* application.
*
*
*
* After you create an application version with a specified Amazon S3 bucket and key location, you can't change that
* Amazon S3 location. If you change the Amazon S3 location, you receive an exception when you attempt to launch an
* environment from the application version.
*
*
*
* @param createApplicationVersionRequest
* @return Result of the CreateApplicationVersion operation returned by the service.
* @throws TooManyApplicationsException
* The specified account has reached its limit of applications.
* @throws TooManyApplicationVersionsException
* The specified account has reached its limit of application versions.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws S3LocationNotInServiceRegionException
* The specified S3 bucket does not belong to the S3 region in which the service is running. The following
* regions are supported:
*
* -
*
* IAD/us-east-1
*
*
* -
*
* PDX/us-west-2
*
*
* -
*
* DUB/eu-west-1
*
*
* @throws CodeBuildNotInServiceRegionException
* AWS CodeBuild is not available in the specified region.
* @sample AWSElasticBeanstalk.CreateApplicationVersion
* @see AWS API Documentation
*/
CreateApplicationVersionResult createApplicationVersion(CreateApplicationVersionRequest createApplicationVersionRequest);
/**
*
* Creates an AWS Elastic Beanstalk configuration template, associated with a specific Elastic Beanstalk
* application. You define application configuration settings in a configuration template. You can then use the
* configuration template to deploy different versions of the application with the same configuration settings.
*
*
* Templates aren't associated with any environment. The EnvironmentName
response element is always
* null
.
*
*
* Related Topics
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createConfigurationTemplateRequest
* Request to create a configuration template.
* @return Result of the CreateConfigurationTemplate operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @throws TooManyConfigurationTemplatesException
* The specified account has reached its limit of configuration templates.
* @sample AWSElasticBeanstalk.CreateConfigurationTemplate
* @see AWS API Documentation
*/
CreateConfigurationTemplateResult createConfigurationTemplate(CreateConfigurationTemplateRequest createConfigurationTemplateRequest);
/**
*
* Launches an AWS Elastic Beanstalk environment for the specified application using the specified configuration.
*
*
* @param createEnvironmentRequest
* @return Result of the CreateEnvironment operation returned by the service.
* @throws TooManyEnvironmentsException
* The specified account has reached its limit of environments.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.CreateEnvironment
* @see AWS API Documentation
*/
CreateEnvironmentResult createEnvironment(CreateEnvironmentRequest createEnvironmentRequest);
/**
*
* Create a new version of your custom platform.
*
*
* @param createPlatformVersionRequest
* Request to create a new platform version.
* @return Result of the CreatePlatformVersion operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @throws TooManyPlatformsException
* You have exceeded the maximum number of allowed platforms associated with the account.
* @sample AWSElasticBeanstalk.CreatePlatformVersion
* @see AWS API Documentation
*/
CreatePlatformVersionResult createPlatformVersion(CreatePlatformVersionRequest createPlatformVersionRequest);
/**
*
* Creates a bucket in Amazon S3 to store application versions, logs, and other files used by Elastic Beanstalk
* environments. The Elastic Beanstalk console and EB CLI call this API the first time you create an environment in
* a region. If the storage location already exists, CreateStorageLocation
still returns the bucket
* name but does not create a new bucket.
*
*
* @param createStorageLocationRequest
* @return Result of the CreateStorageLocation operation returned by the service.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @throws S3SubscriptionRequiredException
* The specified account does not have a subscription to Amazon S3.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.CreateStorageLocation
* @see AWS API Documentation
*/
CreateStorageLocationResult createStorageLocation(CreateStorageLocationRequest createStorageLocationRequest);
/**
* Simplified method form for invoking the CreateStorageLocation operation.
*
* @see #createStorageLocation(CreateStorageLocationRequest)
*/
CreateStorageLocationResult createStorageLocation();
/**
*
* Deletes the specified application along with all associated versions and configurations. The application versions
* will not be deleted from your Amazon S3 bucket.
*
*
*
* You cannot delete an application that has a running environment.
*
*
*
* @param deleteApplicationRequest
* Request to delete an application.
* @return Result of the DeleteApplication operation returned by the service.
* @throws OperationInProgressException
* Unable to perform the specified operation because another operation that effects an element in this
* activity is already in progress.
* @sample AWSElasticBeanstalk.DeleteApplication
* @see AWS API Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes the specified version from the specified application.
*
*
*
* You cannot delete an application version that is associated with a running environment.
*
*
*
* @param deleteApplicationVersionRequest
* Request to delete an application version.
* @return Result of the DeleteApplicationVersion operation returned by the service.
* @throws SourceBundleDeletionException
* Unable to delete the Amazon S3 source bundle associated with the application version. The application
* version was deleted successfully.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws OperationInProgressException
* Unable to perform the specified operation because another operation that effects an element in this
* activity is already in progress.
* @throws S3LocationNotInServiceRegionException
* The specified S3 bucket does not belong to the S3 region in which the service is running. The following
* regions are supported:
*
* -
*
* IAD/us-east-1
*
*
* -
*
* PDX/us-west-2
*
*
* -
*
* DUB/eu-west-1
*
*
* @sample AWSElasticBeanstalk.DeleteApplicationVersion
* @see AWS API Documentation
*/
DeleteApplicationVersionResult deleteApplicationVersion(DeleteApplicationVersionRequest deleteApplicationVersionRequest);
/**
*
* Deletes the specified configuration template.
*
*
*
* When you launch an environment using a configuration template, the environment gets a copy of the template. You
* can delete or modify the environment's copy of the template without affecting the running environment.
*
*
*
* @param deleteConfigurationTemplateRequest
* Request to delete a configuration template.
* @return Result of the DeleteConfigurationTemplate operation returned by the service.
* @throws OperationInProgressException
* Unable to perform the specified operation because another operation that effects an element in this
* activity is already in progress.
* @sample AWSElasticBeanstalk.DeleteConfigurationTemplate
* @see AWS API Documentation
*/
DeleteConfigurationTemplateResult deleteConfigurationTemplate(DeleteConfigurationTemplateRequest deleteConfigurationTemplateRequest);
/**
*
* Deletes the draft configuration associated with the running environment.
*
*
* Updating a running environment with any configuration changes creates a draft configuration set. You can get the
* draft configuration using DescribeConfigurationSettings while the update is in progress or if the update
* fails. The DeploymentStatus
for the draft configuration indicates whether the deployment is in
* process or has failed. The draft configuration remains in existence until it is deleted with this action.
*
*
* @param deleteEnvironmentConfigurationRequest
* Request to delete a draft environment configuration.
* @return Result of the DeleteEnvironmentConfiguration operation returned by the service.
* @sample AWSElasticBeanstalk.DeleteEnvironmentConfiguration
* @see AWS API Documentation
*/
DeleteEnvironmentConfigurationResult deleteEnvironmentConfiguration(DeleteEnvironmentConfigurationRequest deleteEnvironmentConfigurationRequest);
/**
*
* Deletes the specified version of a custom platform.
*
*
* @param deletePlatformVersionRequest
* @return Result of the DeletePlatformVersion operation returned by the service.
* @throws OperationInProgressException
* Unable to perform the specified operation because another operation that effects an element in this
* activity is already in progress.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @throws PlatformVersionStillReferencedException
* You cannot delete the platform version because there are still environments running on it.
* @sample AWSElasticBeanstalk.DeletePlatformVersion
* @see AWS API Documentation
*/
DeletePlatformVersionResult deletePlatformVersion(DeletePlatformVersionRequest deletePlatformVersionRequest);
/**
*
* Returns attributes related to AWS Elastic Beanstalk that are associated with the calling AWS account.
*
*
* The result currently has one set of attributes—resource quotas.
*
*
* @param describeAccountAttributesRequest
* @return Result of the DescribeAccountAttributes operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.DescribeAccountAttributes
* @see AWS API Documentation
*/
DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Retrieve a list of application versions.
*
*
* @param describeApplicationVersionsRequest
* Request to describe application versions.
* @return Result of the DescribeApplicationVersions operation returned by the service.
* @sample AWSElasticBeanstalk.DescribeApplicationVersions
* @see AWS API Documentation
*/
DescribeApplicationVersionsResult describeApplicationVersions(DescribeApplicationVersionsRequest describeApplicationVersionsRequest);
/**
* Simplified method form for invoking the DescribeApplicationVersions operation.
*
* @see #describeApplicationVersions(DescribeApplicationVersionsRequest)
*/
DescribeApplicationVersionsResult describeApplicationVersions();
/**
*
* Returns the descriptions of existing applications.
*
*
* @param describeApplicationsRequest
* Request to describe one or more applications.
* @return Result of the DescribeApplications operation returned by the service.
* @sample AWSElasticBeanstalk.DescribeApplications
* @see AWS API Documentation
*/
DescribeApplicationsResult describeApplications(DescribeApplicationsRequest describeApplicationsRequest);
/**
* Simplified method form for invoking the DescribeApplications operation.
*
* @see #describeApplications(DescribeApplicationsRequest)
*/
DescribeApplicationsResult describeApplications();
/**
*
* Describes the configuration options that are used in a particular configuration template or environment, or that
* a specified solution stack defines. The description includes the values the options, their default values, and an
* indication of the required action on a running environment if an option value is changed.
*
*
* @param describeConfigurationOptionsRequest
* Result message containing a list of application version descriptions.
* @return Result of the DescribeConfigurationOptions operation returned by the service.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @sample AWSElasticBeanstalk.DescribeConfigurationOptions
* @see AWS API Documentation
*/
DescribeConfigurationOptionsResult describeConfigurationOptions(DescribeConfigurationOptionsRequest describeConfigurationOptionsRequest);
/**
*
* Returns a description of the settings for the specified configuration set, that is, either a configuration
* template or the configuration set associated with a running environment.
*
*
* When describing the settings for the configuration set associated with a running environment, it is possible to
* receive two sets of setting descriptions. One is the deployed configuration set, and the other is a draft
* configuration of an environment that is either in the process of deployment or that failed to deploy.
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param describeConfigurationSettingsRequest
* Result message containing all of the configuration settings for a specified solution stack or
* configuration template.
* @return Result of the DescribeConfigurationSettings operation returned by the service.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @sample AWSElasticBeanstalk.DescribeConfigurationSettings
* @see AWS API Documentation
*/
DescribeConfigurationSettingsResult describeConfigurationSettings(DescribeConfigurationSettingsRequest describeConfigurationSettingsRequest);
/**
*
* Returns information about the overall health of the specified environment. The DescribeEnvironmentHealth
* operation is only available with AWS Elastic Beanstalk Enhanced Health.
*
*
* @param describeEnvironmentHealthRequest
* See the example below to learn how to create a request body.
* @return Result of the DescribeEnvironmentHealth operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters is not valid. Please correct the input parameters and try the operation
* again.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @sample AWSElasticBeanstalk.DescribeEnvironmentHealth
* @see AWS API Documentation
*/
DescribeEnvironmentHealthResult describeEnvironmentHealth(DescribeEnvironmentHealthRequest describeEnvironmentHealthRequest);
/**
*
* Lists an environment's completed and failed managed actions.
*
*
* @param describeEnvironmentManagedActionHistoryRequest
* Request to list completed and failed managed actions.
* @return Result of the DescribeEnvironmentManagedActionHistory operation returned by the service.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @sample AWSElasticBeanstalk.DescribeEnvironmentManagedActionHistory
* @see AWS API Documentation
*/
DescribeEnvironmentManagedActionHistoryResult describeEnvironmentManagedActionHistory(
DescribeEnvironmentManagedActionHistoryRequest describeEnvironmentManagedActionHistoryRequest);
/**
*
* Lists an environment's upcoming and in-progress managed actions.
*
*
* @param describeEnvironmentManagedActionsRequest
* Request to list an environment's upcoming and in-progress managed actions.
* @return Result of the DescribeEnvironmentManagedActions operation returned by the service.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @sample AWSElasticBeanstalk.DescribeEnvironmentManagedActions
* @see AWS API Documentation
*/
DescribeEnvironmentManagedActionsResult describeEnvironmentManagedActions(DescribeEnvironmentManagedActionsRequest describeEnvironmentManagedActionsRequest);
/**
*
* Returns AWS resources for this environment.
*
*
* @param describeEnvironmentResourcesRequest
* Request to describe the resources in an environment.
* @return Result of the DescribeEnvironmentResources operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.DescribeEnvironmentResources
* @see AWS API Documentation
*/
DescribeEnvironmentResourcesResult describeEnvironmentResources(DescribeEnvironmentResourcesRequest describeEnvironmentResourcesRequest);
/**
*
* Returns descriptions for existing environments.
*
*
* @param describeEnvironmentsRequest
* Request to describe one or more environments.
* @return Result of the DescribeEnvironments operation returned by the service.
* @sample AWSElasticBeanstalk.DescribeEnvironments
* @see AWS API Documentation
*/
DescribeEnvironmentsResult describeEnvironments(DescribeEnvironmentsRequest describeEnvironmentsRequest);
/**
* Simplified method form for invoking the DescribeEnvironments operation.
*
* @see #describeEnvironments(DescribeEnvironmentsRequest)
*/
DescribeEnvironmentsResult describeEnvironments();
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* @param describeEventsRequest
* Request to retrieve a list of events for an environment.
* @return Result of the DescribeEvents operation returned by the service.
* @sample AWSElasticBeanstalk.DescribeEvents
* @see AWS API Documentation
*/
DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest);
/**
* Simplified method form for invoking the DescribeEvents operation.
*
* @see #describeEvents(DescribeEventsRequest)
*/
DescribeEventsResult describeEvents();
/**
*
* Retrieves detailed information about the health of instances in your AWS Elastic Beanstalk. This operation
* requires enhanced health
* reporting.
*
*
* @param describeInstancesHealthRequest
* Parameters for a call to DescribeInstancesHealth
.
* @return Result of the DescribeInstancesHealth operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters is not valid. Please correct the input parameters and try the operation
* again.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @sample AWSElasticBeanstalk.DescribeInstancesHealth
* @see AWS API Documentation
*/
DescribeInstancesHealthResult describeInstancesHealth(DescribeInstancesHealthRequest describeInstancesHealthRequest);
/**
*
* Describes a platform version. Provides full details. Compare to ListPlatformVersions, which provides
* summary information about a list of platform versions.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @param describePlatformVersionRequest
* @return Result of the DescribePlatformVersion operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @sample AWSElasticBeanstalk.DescribePlatformVersion
* @see AWS API Documentation
*/
DescribePlatformVersionResult describePlatformVersion(DescribePlatformVersionRequest describePlatformVersionRequest);
/**
*
* Disassociate the operations role from an environment. After this call is made, Elastic Beanstalk uses the
* caller's permissions for permissions to downstream services during subsequent calls acting on this environment.
* For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
* @param disassociateEnvironmentOperationsRoleRequest
* Request to disassociate the operations role from an environment.
* @return Result of the DisassociateEnvironmentOperationsRole operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.DisassociateEnvironmentOperationsRole
* @see AWS API Documentation
*/
DisassociateEnvironmentOperationsRoleResult disassociateEnvironmentOperationsRole(
DisassociateEnvironmentOperationsRoleRequest disassociateEnvironmentOperationsRoleRequest);
/**
*
* Returns a list of the available solution stack names, with the public version first and then in reverse
* chronological order.
*
*
* @param listAvailableSolutionStacksRequest
* @return Result of the ListAvailableSolutionStacks operation returned by the service.
* @sample AWSElasticBeanstalk.ListAvailableSolutionStacks
* @see AWS API Documentation
*/
ListAvailableSolutionStacksResult listAvailableSolutionStacks(ListAvailableSolutionStacksRequest listAvailableSolutionStacksRequest);
/**
* Simplified method form for invoking the ListAvailableSolutionStacks operation.
*
* @see #listAvailableSolutionStacks(ListAvailableSolutionStacksRequest)
*/
ListAvailableSolutionStacksResult listAvailableSolutionStacks();
/**
*
* Lists the platform branches available for your account in an AWS Region. Provides summary information about each
* platform branch.
*
*
* For definitions of platform branch and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @param listPlatformBranchesRequest
* @return Result of the ListPlatformBranches operation returned by the service.
* @sample AWSElasticBeanstalk.ListPlatformBranches
* @see AWS API Documentation
*/
ListPlatformBranchesResult listPlatformBranches(ListPlatformBranchesRequest listPlatformBranchesRequest);
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @param listPlatformVersionsRequest
* @return Result of the ListPlatformVersions operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws ElasticBeanstalkServiceException
* A generic service exception has occurred.
* @sample AWSElasticBeanstalk.ListPlatformVersions
* @see AWS API Documentation
*/
ListPlatformVersionsResult listPlatformVersions(ListPlatformVersionsRequest listPlatformVersionsRequest);
/**
*
* Return the tags applied to an AWS Elastic Beanstalk resource. The response contains a list of tag key-value
* pairs.
*
*
* Elastic Beanstalk supports tagging of all of its resources. For details about resource tagging, see Tagging
* Application Resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN).
* @throws ResourceTypeNotSupportedException
* The type of the specified Amazon Resource Name (ARN) isn't supported for this operation.
* @sample AWSElasticBeanstalk.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Deletes and recreates all of the AWS resources (for example: the Auto Scaling group, load balancer, etc.) for a
* specified environment and forces a restart.
*
*
* @param rebuildEnvironmentRequest
* @return Result of the RebuildEnvironment operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.RebuildEnvironment
* @see AWS API Documentation
*/
RebuildEnvironmentResult rebuildEnvironment(RebuildEnvironmentRequest rebuildEnvironmentRequest);
/**
*
* Initiates a request to compile the specified type of information of the deployed environment.
*
*
* Setting the InfoType
to tail
compiles the last lines from the application server log
* files of every Amazon EC2 instance in your environment.
*
*
* Setting the InfoType
to bundle
compresses the application server log files for every
* Amazon EC2 instance into a .zip
file. Legacy and .NET containers do not support bundle logs.
*
*
* Use RetrieveEnvironmentInfo to obtain the set of logs.
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param requestEnvironmentInfoRequest
* Request to retrieve logs from an environment and store them in your Elastic Beanstalk storage bucket.
* @return Result of the RequestEnvironmentInfo operation returned by the service.
* @sample AWSElasticBeanstalk.RequestEnvironmentInfo
* @see AWS API Documentation
*/
RequestEnvironmentInfoResult requestEnvironmentInfo(RequestEnvironmentInfoRequest requestEnvironmentInfoRequest);
/**
*
* Causes the environment to restart the application container server running on each Amazon EC2 instance.
*
*
* @param restartAppServerRequest
* @return Result of the RestartAppServer operation returned by the service.
* @sample AWSElasticBeanstalk.RestartAppServer
* @see AWS API Documentation
*/
RestartAppServerResult restartAppServer(RestartAppServerRequest restartAppServerRequest);
/**
*
* Retrieves the compiled information from a RequestEnvironmentInfo request.
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param retrieveEnvironmentInfoRequest
* Request to download logs retrieved with RequestEnvironmentInfo.
* @return Result of the RetrieveEnvironmentInfo operation returned by the service.
* @sample AWSElasticBeanstalk.RetrieveEnvironmentInfo
* @see AWS API Documentation
*/
RetrieveEnvironmentInfoResult retrieveEnvironmentInfo(RetrieveEnvironmentInfoRequest retrieveEnvironmentInfoRequest);
/**
*
* Swaps the CNAMEs of two environments.
*
*
* @param swapEnvironmentCNAMEsRequest
* Swaps the CNAMEs of two environments.
* @return Result of the SwapEnvironmentCNAMEs operation returned by the service.
* @sample AWSElasticBeanstalk.SwapEnvironmentCNAMEs
* @see AWS API Documentation
*/
SwapEnvironmentCNAMEsResult swapEnvironmentCNAMEs(SwapEnvironmentCNAMEsRequest swapEnvironmentCNAMEsRequest);
/**
* Simplified method form for invoking the SwapEnvironmentCNAMEs operation.
*
* @see #swapEnvironmentCNAMEs(SwapEnvironmentCNAMEsRequest)
*/
SwapEnvironmentCNAMEsResult swapEnvironmentCNAMEs();
/**
*
* Terminates the specified environment.
*
*
* @param terminateEnvironmentRequest
* Request to terminate an environment.
* @return Result of the TerminateEnvironment operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.TerminateEnvironment
* @see AWS API Documentation
*/
TerminateEnvironmentResult terminateEnvironment(TerminateEnvironmentRequest terminateEnvironmentRequest);
/**
*
* Updates the specified application to have the specified properties.
*
*
*
* If a property (for example, description
) is not provided, the value remains unchanged. To clear
* these properties, specify an empty string.
*
*
*
* @param updateApplicationRequest
* Request to update an application.
* @return Result of the UpdateApplication operation returned by the service.
* @sample AWSElasticBeanstalk.UpdateApplication
* @see AWS API Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Modifies lifecycle settings for an application.
*
*
* @param updateApplicationResourceLifecycleRequest
* @return Result of the UpdateApplicationResourceLifecycle operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @sample AWSElasticBeanstalk.UpdateApplicationResourceLifecycle
* @see AWS API Documentation
*/
UpdateApplicationResourceLifecycleResult updateApplicationResourceLifecycle(
UpdateApplicationResourceLifecycleRequest updateApplicationResourceLifecycleRequest);
/**
*
* Updates the specified application version to have the specified properties.
*
*
*
* If a property (for example, description
) is not provided, the value remains unchanged. To clear
* properties, specify an empty string.
*
*
*
* @param updateApplicationVersionRequest
* @return Result of the UpdateApplicationVersion operation returned by the service.
* @sample AWSElasticBeanstalk.UpdateApplicationVersion
* @see AWS API Documentation
*/
UpdateApplicationVersionResult updateApplicationVersion(UpdateApplicationVersionRequest updateApplicationVersionRequest);
/**
*
* Updates the specified configuration template to have the specified properties or configuration option values.
*
*
*
* If a property (for example, ApplicationName
) is not provided, its value remains unchanged. To clear
* such properties, specify an empty string.
*
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param updateConfigurationTemplateRequest
* The result message containing the options for the specified solution stack.
* @return Result of the UpdateConfigurationTemplate operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @sample AWSElasticBeanstalk.UpdateConfigurationTemplate
* @see AWS API Documentation
*/
UpdateConfigurationTemplateResult updateConfigurationTemplate(UpdateConfigurationTemplateRequest updateConfigurationTemplateRequest);
/**
*
* Updates the environment description, deploys a new application version, updates the configuration settings to an
* entirely new configuration template, or updates select configuration option values in the running environment.
*
*
* Attempting to update both the release and configuration is not allowed and AWS Elastic Beanstalk returns an
* InvalidParameterCombination
error.
*
*
* When updating the configuration settings to a new template or individual settings, a draft configuration is
* created and DescribeConfigurationSettings for this environment returns two setting descriptions with
* different DeploymentStatus
values.
*
*
* @param updateEnvironmentRequest
* Request to update an environment.
* @return Result of the UpdateEnvironment operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @sample AWSElasticBeanstalk.UpdateEnvironment
* @see AWS API Documentation
*/
UpdateEnvironmentResult updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest);
/**
*
* Update the list of tags applied to an AWS Elastic Beanstalk resource. Two lists can be passed:
* TagsToAdd
for tags to add or update, and TagsToRemove
.
*
*
* Elastic Beanstalk supports tagging of all of its resources. For details about resource tagging, see Tagging
* Application Resources.
*
*
* If you create a custom IAM user policy to control permission to this operation, specify one of the following two
* virtual actions (or both) instead of the API operation name:
*
*
* - elasticbeanstalk:AddTags
* -
*
* Controls permission to call UpdateTagsForResource
and pass a list of tags to add in the
* TagsToAdd
parameter.
*
*
* - elasticbeanstalk:RemoveTags
* -
*
* Controls permission to call UpdateTagsForResource
and pass a list of tag keys to remove in the
* TagsToRemove
parameter.
*
*
*
*
* For details about creating a custom user policy, see Creating a Custom User Policy.
*
*
* @param updateTagsForResourceRequest
* @return Result of the UpdateTagsForResource operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws OperationInProgressException
* Unable to perform the specified operation because another operation that effects an element in this
* activity is already in progress.
* @throws TooManyTagsException
* The number of tags in the resource would exceed the number of tags that each resource can have.
*
* To calculate this, the operation considers both the number of tags the resource already has and the tags
* this operation would add if it succeeded.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN).
* @throws ResourceTypeNotSupportedException
* The type of the specified Amazon Resource Name (ARN) isn't supported for this operation.
* @sample AWSElasticBeanstalk.UpdateTagsForResource
* @see AWS API Documentation
*/
UpdateTagsForResourceResult updateTagsForResource(UpdateTagsForResourceRequest updateTagsForResourceRequest);
/**
*
* Takes a set of configuration settings and either a configuration template or environment, and determines whether
* those values are valid.
*
*
* This action returns a list of messages indicating any errors or warnings associated with the selection of option
* values.
*
*
* @param validateConfigurationSettingsRequest
* A list of validation messages for a specified configuration template.
* @return Result of the ValidateConfigurationSettings operation returned by the service.
* @throws InsufficientPrivilegesException
* The specified account does not have sufficient privileges for one or more AWS services.
* @throws TooManyBucketsException
* The specified account has reached its limit of Amazon S3 buckets.
* @sample AWSElasticBeanstalk.ValidateConfigurationSettings
* @see AWS API Documentation
*/
ValidateConfigurationSettingsResult validateConfigurationSettings(ValidateConfigurationSettingsRequest validateConfigurationSettingsRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AWSElasticBeanstalkWaiters waiters();
}