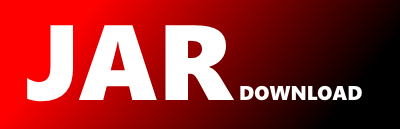
com.amazonaws.services.elasticbeanstalk.model.BuildConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticbeanstalk Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Settings for an AWS CodeBuild build.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class BuildConfiguration implements Serializable, Cloneable {
/**
*
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact in the
* S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label-artifact
* -name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*
*/
private String artifactName;
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*
*/
private String codeBuildServiceRole;
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*/
private String computeType;
/**
*
* The ID of the Docker image to use for this build project.
*
*/
private String image;
/**
*
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build that
* does not get marked as completed. The default is 60 minutes.
*
*/
private Integer timeoutInMinutes;
/**
*
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact in the
* S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label-artifact
* -name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*
*
* @param artifactName
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact
* in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label<
* /i>-artifact-name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3
* location S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*/
public void setArtifactName(String artifactName) {
this.artifactName = artifactName;
}
/**
*
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact in the
* S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label-artifact
* -name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*
*
* @return The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact
* in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label
* -artifact-name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3
* location S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*/
public String getArtifactName() {
return this.artifactName;
}
/**
*
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact in the
* S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label-artifact
* -name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*
*
* @param artifactName
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact
* in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label<
* /i>-artifact-name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3
* location S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BuildConfiguration withArtifactName(String artifactName) {
setArtifactName(artifactName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*
*
* @param codeBuildServiceRole
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS
* CodeBuild to interact with dependent AWS services on behalf of the AWS account.
*/
public void setCodeBuildServiceRole(String codeBuildServiceRole) {
this.codeBuildServiceRole = codeBuildServiceRole;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*
*
* @return The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS
* CodeBuild to interact with dependent AWS services on behalf of the AWS account.
*/
public String getCodeBuildServiceRole() {
return this.codeBuildServiceRole;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*
*
* @param codeBuildServiceRole
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS
* CodeBuild to interact with dependent AWS services on behalf of the AWS account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BuildConfiguration withCodeBuildServiceRole(String codeBuildServiceRole) {
setCodeBuildServiceRole(codeBuildServiceRole);
return this;
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* @param computeType
* Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @see ComputeType
*/
public void setComputeType(String computeType) {
this.computeType = computeType;
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* @return Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @see ComputeType
*/
public String getComputeType() {
return this.computeType;
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* @param computeType
* Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputeType
*/
public BuildConfiguration withComputeType(String computeType) {
setComputeType(computeType);
return this;
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* @param computeType
* Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @see ComputeType
*/
public void setComputeType(ComputeType computeType) {
withComputeType(computeType);
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* @param computeType
* Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputeType
*/
public BuildConfiguration withComputeType(ComputeType computeType) {
this.computeType = computeType.toString();
return this;
}
/**
*
* The ID of the Docker image to use for this build project.
*
*
* @param image
* The ID of the Docker image to use for this build project.
*/
public void setImage(String image) {
this.image = image;
}
/**
*
* The ID of the Docker image to use for this build project.
*
*
* @return The ID of the Docker image to use for this build project.
*/
public String getImage() {
return this.image;
}
/**
*
* The ID of the Docker image to use for this build project.
*
*
* @param image
* The ID of the Docker image to use for this build project.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BuildConfiguration withImage(String image) {
setImage(image);
return this;
}
/**
*
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build that
* does not get marked as completed. The default is 60 minutes.
*
*
* @param timeoutInMinutes
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build
* that does not get marked as completed. The default is 60 minutes.
*/
public void setTimeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
}
/**
*
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build that
* does not get marked as completed. The default is 60 minutes.
*
*
* @return How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related
* build that does not get marked as completed. The default is 60 minutes.
*/
public Integer getTimeoutInMinutes() {
return this.timeoutInMinutes;
}
/**
*
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build that
* does not get marked as completed. The default is 60 minutes.
*
*
* @param timeoutInMinutes
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build
* that does not get marked as completed. The default is 60 minutes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BuildConfiguration withTimeoutInMinutes(Integer timeoutInMinutes) {
setTimeoutInMinutes(timeoutInMinutes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArtifactName() != null)
sb.append("ArtifactName: ").append(getArtifactName()).append(",");
if (getCodeBuildServiceRole() != null)
sb.append("CodeBuildServiceRole: ").append(getCodeBuildServiceRole()).append(",");
if (getComputeType() != null)
sb.append("ComputeType: ").append(getComputeType()).append(",");
if (getImage() != null)
sb.append("Image: ").append(getImage()).append(",");
if (getTimeoutInMinutes() != null)
sb.append("TimeoutInMinutes: ").append(getTimeoutInMinutes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof BuildConfiguration == false)
return false;
BuildConfiguration other = (BuildConfiguration) obj;
if (other.getArtifactName() == null ^ this.getArtifactName() == null)
return false;
if (other.getArtifactName() != null && other.getArtifactName().equals(this.getArtifactName()) == false)
return false;
if (other.getCodeBuildServiceRole() == null ^ this.getCodeBuildServiceRole() == null)
return false;
if (other.getCodeBuildServiceRole() != null && other.getCodeBuildServiceRole().equals(this.getCodeBuildServiceRole()) == false)
return false;
if (other.getComputeType() == null ^ this.getComputeType() == null)
return false;
if (other.getComputeType() != null && other.getComputeType().equals(this.getComputeType()) == false)
return false;
if (other.getImage() == null ^ this.getImage() == null)
return false;
if (other.getImage() != null && other.getImage().equals(this.getImage()) == false)
return false;
if (other.getTimeoutInMinutes() == null ^ this.getTimeoutInMinutes() == null)
return false;
if (other.getTimeoutInMinutes() != null && other.getTimeoutInMinutes().equals(this.getTimeoutInMinutes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArtifactName() == null) ? 0 : getArtifactName().hashCode());
hashCode = prime * hashCode + ((getCodeBuildServiceRole() == null) ? 0 : getCodeBuildServiceRole().hashCode());
hashCode = prime * hashCode + ((getComputeType() == null) ? 0 : getComputeType().hashCode());
hashCode = prime * hashCode + ((getImage() == null) ? 0 : getImage().hashCode());
hashCode = prime * hashCode + ((getTimeoutInMinutes() == null) ? 0 : getTimeoutInMinutes().hashCode());
return hashCode;
}
@Override
public BuildConfiguration clone() {
try {
return (BuildConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}