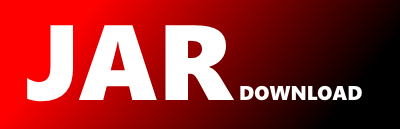
com.amazonaws.services.elasticbeanstalk.model.ConfigurationSettingsDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticbeanstalk Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Describes the settings for a configuration set.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ConfigurationSettingsDescription implements Serializable, Cloneable {
/**
*
* The name of the solution stack this configuration set uses.
*
*/
private String solutionStackName;
/**
*
* The ARN of the platform version.
*
*/
private String platformArn;
/**
*
* The name of the application associated with this configuration set.
*
*/
private String applicationName;
/**
*
* If not null
, the name of the configuration template for this configuration set.
*
*/
private String templateName;
/**
*
* Describes this configuration set.
*
*/
private String description;
/**
*
* If not null
, the name of the environment for this configuration set.
*
*/
private String environmentName;
/**
*
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but is in
* the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
*
*/
private String deploymentStatus;
/**
*
* The date (in UTC time) when this configuration set was created.
*
*/
private java.util.Date dateCreated;
/**
*
* The date (in UTC time) when this configuration set was last modified.
*
*/
private java.util.Date dateUpdated;
/**
*
* A list of the configuration options and their values in this configuration set.
*
*/
private com.amazonaws.internal.SdkInternalList optionSettings;
/**
*
* The name of the solution stack this configuration set uses.
*
*
* @param solutionStackName
* The name of the solution stack this configuration set uses.
*/
public void setSolutionStackName(String solutionStackName) {
this.solutionStackName = solutionStackName;
}
/**
*
* The name of the solution stack this configuration set uses.
*
*
* @return The name of the solution stack this configuration set uses.
*/
public String getSolutionStackName() {
return this.solutionStackName;
}
/**
*
* The name of the solution stack this configuration set uses.
*
*
* @param solutionStackName
* The name of the solution stack this configuration set uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withSolutionStackName(String solutionStackName) {
setSolutionStackName(solutionStackName);
return this;
}
/**
*
* The ARN of the platform version.
*
*
* @param platformArn
* The ARN of the platform version.
*/
public void setPlatformArn(String platformArn) {
this.platformArn = platformArn;
}
/**
*
* The ARN of the platform version.
*
*
* @return The ARN of the platform version.
*/
public String getPlatformArn() {
return this.platformArn;
}
/**
*
* The ARN of the platform version.
*
*
* @param platformArn
* The ARN of the platform version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withPlatformArn(String platformArn) {
setPlatformArn(platformArn);
return this;
}
/**
*
* The name of the application associated with this configuration set.
*
*
* @param applicationName
* The name of the application associated with this configuration set.
*/
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
/**
*
* The name of the application associated with this configuration set.
*
*
* @return The name of the application associated with this configuration set.
*/
public String getApplicationName() {
return this.applicationName;
}
/**
*
* The name of the application associated with this configuration set.
*
*
* @param applicationName
* The name of the application associated with this configuration set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withApplicationName(String applicationName) {
setApplicationName(applicationName);
return this;
}
/**
*
* If not null
, the name of the configuration template for this configuration set.
*
*
* @param templateName
* If not null
, the name of the configuration template for this configuration set.
*/
public void setTemplateName(String templateName) {
this.templateName = templateName;
}
/**
*
* If not null
, the name of the configuration template for this configuration set.
*
*
* @return If not null
, the name of the configuration template for this configuration set.
*/
public String getTemplateName() {
return this.templateName;
}
/**
*
* If not null
, the name of the configuration template for this configuration set.
*
*
* @param templateName
* If not null
, the name of the configuration template for this configuration set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withTemplateName(String templateName) {
setTemplateName(templateName);
return this;
}
/**
*
* Describes this configuration set.
*
*
* @param description
* Describes this configuration set.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Describes this configuration set.
*
*
* @return Describes this configuration set.
*/
public String getDescription() {
return this.description;
}
/**
*
* Describes this configuration set.
*
*
* @param description
* Describes this configuration set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* If not null
, the name of the environment for this configuration set.
*
*
* @param environmentName
* If not null
, the name of the environment for this configuration set.
*/
public void setEnvironmentName(String environmentName) {
this.environmentName = environmentName;
}
/**
*
* If not null
, the name of the environment for this configuration set.
*
*
* @return If not null
, the name of the environment for this configuration set.
*/
public String getEnvironmentName() {
return this.environmentName;
}
/**
*
* If not null
, the name of the environment for this configuration set.
*
*
* @param environmentName
* If not null
, the name of the environment for this configuration set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withEnvironmentName(String environmentName) {
setEnvironmentName(environmentName);
return this;
}
/**
*
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but is in
* the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
*
*
* @param deploymentStatus
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but
* is in the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
* @see ConfigurationDeploymentStatus
*/
public void setDeploymentStatus(String deploymentStatus) {
this.deploymentStatus = deploymentStatus;
}
/**
*
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but is in
* the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
*
*
* @return If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment
* but is in the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
* @see ConfigurationDeploymentStatus
*/
public String getDeploymentStatus() {
return this.deploymentStatus;
}
/**
*
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but is in
* the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
*
*
* @param deploymentStatus
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but
* is in the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigurationDeploymentStatus
*/
public ConfigurationSettingsDescription withDeploymentStatus(String deploymentStatus) {
setDeploymentStatus(deploymentStatus);
return this;
}
/**
*
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but is in
* the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
*
*
* @param deploymentStatus
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but
* is in the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
* @see ConfigurationDeploymentStatus
*/
public void setDeploymentStatus(ConfigurationDeploymentStatus deploymentStatus) {
withDeploymentStatus(deploymentStatus);
}
/**
*
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but is in
* the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
*
*
* @param deploymentStatus
* If this configuration set is associated with an environment, the DeploymentStatus
parameter
* indicates the deployment status of this configuration set:
*
* -
*
* null
: This configuration is not associated with a running environment.
*
*
* -
*
* pending
: This is a draft configuration that is not deployed to the associated environment but
* is in the process of deploying.
*
*
* -
*
* deployed
: This is the configuration that is currently deployed to the associated running
* environment.
*
*
* -
*
* failed
: This is a draft configuration that failed to successfully deploy.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigurationDeploymentStatus
*/
public ConfigurationSettingsDescription withDeploymentStatus(ConfigurationDeploymentStatus deploymentStatus) {
this.deploymentStatus = deploymentStatus.toString();
return this;
}
/**
*
* The date (in UTC time) when this configuration set was created.
*
*
* @param dateCreated
* The date (in UTC time) when this configuration set was created.
*/
public void setDateCreated(java.util.Date dateCreated) {
this.dateCreated = dateCreated;
}
/**
*
* The date (in UTC time) when this configuration set was created.
*
*
* @return The date (in UTC time) when this configuration set was created.
*/
public java.util.Date getDateCreated() {
return this.dateCreated;
}
/**
*
* The date (in UTC time) when this configuration set was created.
*
*
* @param dateCreated
* The date (in UTC time) when this configuration set was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withDateCreated(java.util.Date dateCreated) {
setDateCreated(dateCreated);
return this;
}
/**
*
* The date (in UTC time) when this configuration set was last modified.
*
*
* @param dateUpdated
* The date (in UTC time) when this configuration set was last modified.
*/
public void setDateUpdated(java.util.Date dateUpdated) {
this.dateUpdated = dateUpdated;
}
/**
*
* The date (in UTC time) when this configuration set was last modified.
*
*
* @return The date (in UTC time) when this configuration set was last modified.
*/
public java.util.Date getDateUpdated() {
return this.dateUpdated;
}
/**
*
* The date (in UTC time) when this configuration set was last modified.
*
*
* @param dateUpdated
* The date (in UTC time) when this configuration set was last modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withDateUpdated(java.util.Date dateUpdated) {
setDateUpdated(dateUpdated);
return this;
}
/**
*
* A list of the configuration options and their values in this configuration set.
*
*
* @return A list of the configuration options and their values in this configuration set.
*/
public java.util.List getOptionSettings() {
if (optionSettings == null) {
optionSettings = new com.amazonaws.internal.SdkInternalList();
}
return optionSettings;
}
/**
*
* A list of the configuration options and their values in this configuration set.
*
*
* @param optionSettings
* A list of the configuration options and their values in this configuration set.
*/
public void setOptionSettings(java.util.Collection optionSettings) {
if (optionSettings == null) {
this.optionSettings = null;
return;
}
this.optionSettings = new com.amazonaws.internal.SdkInternalList(optionSettings);
}
/**
*
* A list of the configuration options and their values in this configuration set.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOptionSettings(java.util.Collection)} or {@link #withOptionSettings(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param optionSettings
* A list of the configuration options and their values in this configuration set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withOptionSettings(ConfigurationOptionSetting... optionSettings) {
if (this.optionSettings == null) {
setOptionSettings(new com.amazonaws.internal.SdkInternalList(optionSettings.length));
}
for (ConfigurationOptionSetting ele : optionSettings) {
this.optionSettings.add(ele);
}
return this;
}
/**
*
* A list of the configuration options and their values in this configuration set.
*
*
* @param optionSettings
* A list of the configuration options and their values in this configuration set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationSettingsDescription withOptionSettings(java.util.Collection optionSettings) {
setOptionSettings(optionSettings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSolutionStackName() != null)
sb.append("SolutionStackName: ").append(getSolutionStackName()).append(",");
if (getPlatformArn() != null)
sb.append("PlatformArn: ").append(getPlatformArn()).append(",");
if (getApplicationName() != null)
sb.append("ApplicationName: ").append(getApplicationName()).append(",");
if (getTemplateName() != null)
sb.append("TemplateName: ").append(getTemplateName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEnvironmentName() != null)
sb.append("EnvironmentName: ").append(getEnvironmentName()).append(",");
if (getDeploymentStatus() != null)
sb.append("DeploymentStatus: ").append(getDeploymentStatus()).append(",");
if (getDateCreated() != null)
sb.append("DateCreated: ").append(getDateCreated()).append(",");
if (getDateUpdated() != null)
sb.append("DateUpdated: ").append(getDateUpdated()).append(",");
if (getOptionSettings() != null)
sb.append("OptionSettings: ").append(getOptionSettings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ConfigurationSettingsDescription == false)
return false;
ConfigurationSettingsDescription other = (ConfigurationSettingsDescription) obj;
if (other.getSolutionStackName() == null ^ this.getSolutionStackName() == null)
return false;
if (other.getSolutionStackName() != null && other.getSolutionStackName().equals(this.getSolutionStackName()) == false)
return false;
if (other.getPlatformArn() == null ^ this.getPlatformArn() == null)
return false;
if (other.getPlatformArn() != null && other.getPlatformArn().equals(this.getPlatformArn()) == false)
return false;
if (other.getApplicationName() == null ^ this.getApplicationName() == null)
return false;
if (other.getApplicationName() != null && other.getApplicationName().equals(this.getApplicationName()) == false)
return false;
if (other.getTemplateName() == null ^ this.getTemplateName() == null)
return false;
if (other.getTemplateName() != null && other.getTemplateName().equals(this.getTemplateName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEnvironmentName() == null ^ this.getEnvironmentName() == null)
return false;
if (other.getEnvironmentName() != null && other.getEnvironmentName().equals(this.getEnvironmentName()) == false)
return false;
if (other.getDeploymentStatus() == null ^ this.getDeploymentStatus() == null)
return false;
if (other.getDeploymentStatus() != null && other.getDeploymentStatus().equals(this.getDeploymentStatus()) == false)
return false;
if (other.getDateCreated() == null ^ this.getDateCreated() == null)
return false;
if (other.getDateCreated() != null && other.getDateCreated().equals(this.getDateCreated()) == false)
return false;
if (other.getDateUpdated() == null ^ this.getDateUpdated() == null)
return false;
if (other.getDateUpdated() != null && other.getDateUpdated().equals(this.getDateUpdated()) == false)
return false;
if (other.getOptionSettings() == null ^ this.getOptionSettings() == null)
return false;
if (other.getOptionSettings() != null && other.getOptionSettings().equals(this.getOptionSettings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSolutionStackName() == null) ? 0 : getSolutionStackName().hashCode());
hashCode = prime * hashCode + ((getPlatformArn() == null) ? 0 : getPlatformArn().hashCode());
hashCode = prime * hashCode + ((getApplicationName() == null) ? 0 : getApplicationName().hashCode());
hashCode = prime * hashCode + ((getTemplateName() == null) ? 0 : getTemplateName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEnvironmentName() == null) ? 0 : getEnvironmentName().hashCode());
hashCode = prime * hashCode + ((getDeploymentStatus() == null) ? 0 : getDeploymentStatus().hashCode());
hashCode = prime * hashCode + ((getDateCreated() == null) ? 0 : getDateCreated().hashCode());
hashCode = prime * hashCode + ((getDateUpdated() == null) ? 0 : getDateUpdated().hashCode());
hashCode = prime * hashCode + ((getOptionSettings() == null) ? 0 : getOptionSettings().hashCode());
return hashCode;
}
@Override
public ConfigurationSettingsDescription clone() {
try {
return (ConfigurationSettingsDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}