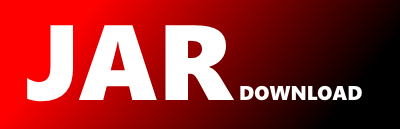
com.amazonaws.services.elasticinference.AmazonElasticInferenceAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticinference;
import javax.annotation.Generated;
import com.amazonaws.services.elasticinference.model.*;
/**
* Interface for accessing Amazon Elastic Inference asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.elasticinference.AbstractAmazonElasticInferenceAsync} instead.
*
*
*
* Elastic Inference public APIs.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After April
* 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon SageMaker, Amazon
* ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past 30-day period are
* considered current customers and will be able to continue using the service.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonElasticInferenceAsync extends AmazonElasticInference {
/**
*
* Describes the locations in which a given accelerator type or set of types is present in a given region.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param describeAcceleratorOfferingsRequest
* @return A Java Future containing the result of the DescribeAcceleratorOfferings operation returned by the
* service.
* @sample AmazonElasticInferenceAsync.DescribeAcceleratorOfferings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAcceleratorOfferingsAsync(
DescribeAcceleratorOfferingsRequest describeAcceleratorOfferingsRequest);
/**
*
* Describes the locations in which a given accelerator type or set of types is present in a given region.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param describeAcceleratorOfferingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAcceleratorOfferings operation returned by the
* service.
* @sample AmazonElasticInferenceAsyncHandler.DescribeAcceleratorOfferings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAcceleratorOfferingsAsync(
DescribeAcceleratorOfferingsRequest describeAcceleratorOfferingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the accelerator types available in a given region, as well as their characteristics, such as memory and
* throughput.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param describeAcceleratorTypesRequest
* @return A Java Future containing the result of the DescribeAcceleratorTypes operation returned by the service.
* @sample AmazonElasticInferenceAsync.DescribeAcceleratorTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAcceleratorTypesAsync(DescribeAcceleratorTypesRequest describeAcceleratorTypesRequest);
/**
*
* Describes the accelerator types available in a given region, as well as their characteristics, such as memory and
* throughput.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param describeAcceleratorTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAcceleratorTypes operation returned by the service.
* @sample AmazonElasticInferenceAsyncHandler.DescribeAcceleratorTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAcceleratorTypesAsync(DescribeAcceleratorTypesRequest describeAcceleratorTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes information over a provided set of accelerators belonging to an account.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param describeAcceleratorsRequest
* @return A Java Future containing the result of the DescribeAccelerators operation returned by the service.
* @sample AmazonElasticInferenceAsync.DescribeAccelerators
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAcceleratorsAsync(DescribeAcceleratorsRequest describeAcceleratorsRequest);
/**
*
* Describes information over a provided set of accelerators belonging to an account.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param describeAcceleratorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccelerators operation returned by the service.
* @sample AmazonElasticInferenceAsyncHandler.DescribeAccelerators
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAcceleratorsAsync(DescribeAcceleratorsRequest describeAcceleratorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all tags of an Elastic Inference Accelerator.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonElasticInferenceAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns all tags of an Elastic Inference Accelerator.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonElasticInferenceAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified tags to an Elastic Inference Accelerator.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonElasticInferenceAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds the specified tags to an Elastic Inference Accelerator.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonElasticInferenceAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from an Elastic Inference Accelerator.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonElasticInferenceAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the specified tags from an Elastic Inference Accelerator.
*
*
* February 15, 2023: Starting April 15, 2023, AWS will not onboard new customers to Amazon Elastic Inference (EI),
* and will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonElasticInferenceAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}