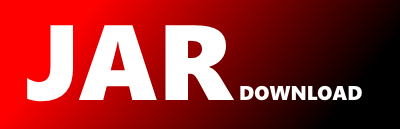
com.amazonaws.services.elasticloadbalancing.AmazonElasticLoadBalancingClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancing Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancing;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.services.elasticloadbalancing.AmazonElasticLoadBalancingClientBuilder;
import com.amazonaws.services.elasticloadbalancing.waiters.AmazonElasticLoadBalancingWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.elasticloadbalancing.model.*;
import com.amazonaws.services.elasticloadbalancing.model.transform.*;
/**
* Client for accessing Elastic Load Balancing. All service calls made using this client are blocking, and will not
* return until the service call completes.
*
* Elastic Load Balancing
*
* A load balancer distributes incoming traffic across your EC2 instances. This enables you to increase the availability
* of your application. The load balancer also monitors the health of its registered instances and ensures that it
* routes traffic only to healthy instances. You configure your load balancer to accept incoming traffic by specifying
* one or more listeners, which are configured with a protocol and port number for connections from clients to the load
* balancer and a protocol and port number for connections from the load balancer to the instances.
*
*
* Elastic Load Balancing supports two types of load balancers: Classic load balancers and Application load balancers
* (new). A Classic load balancer makes routing and load balancing decisions either at the transport layer (TCP/SSL) or
* the application layer (HTTP/HTTPS), and supports either EC2-Classic or a VPC. An Application load balancer makes
* routing and load balancing decisions at the application layer (HTTP/HTTPS), supports path-based routing, and can
* route requests to one or more ports on each EC2 instance or container instance in your virtual private cloud (VPC).
* For more information, see the .
*
*
* This reference covers the 2012-06-01 API, which supports Classic load balancers. The 2015-12-01 API supports
* Application load balancers.
*
*
* To get started, create a load balancer with one or more listeners using CreateLoadBalancer. Register your
* instances with the load balancer using RegisterInstancesWithLoadBalancer.
*
*
* All Elastic Load Balancing operations are idempotent, which means that they complete at most one time. If you
* repeat an operation, it succeeds with a 200 OK response code.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonElasticLoadBalancingClient extends AmazonWebServiceClient implements AmazonElasticLoadBalancing {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonElasticLoadBalancing.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "elasticloadbalancing";
private volatile AmazonElasticLoadBalancingWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
/**
* List of exception unmarshallers for all modeled exceptions
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing. A credentials provider chain will be
* used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonElasticLoadBalancingClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing. A credentials provider chain will be
* used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Elastic Load Balancing (ex: proxy
* settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonElasticLoadBalancingClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing using the specified AWS account
* credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#withCredentials(AWSCredentialsProvider)} for
* example:
* {@code AmazonElasticLoadBalancingClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonElasticLoadBalancingClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing using the specified AWS account
* credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Elastic Load Balancing (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonElasticLoadBalancingClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonElasticLoadBalancingClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing using the specified AWS account
* credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonElasticLoadBalancingClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing using the specified AWS account
* credentials provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Elastic Load Balancing (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonElasticLoadBalancingClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonElasticLoadBalancingClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing using the specified AWS account
* credentials provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Elastic Load Balancing (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonElasticLoadBalancingClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonElasticLoadBalancingClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonElasticLoadBalancingClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonElasticLoadBalancingClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
public static AmazonElasticLoadBalancingClientBuilder builder() {
return AmazonElasticLoadBalancingClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Elastic Load Balancing using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonElasticLoadBalancingClient(AwsSyncClientParams clientParams) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
init();
}
private void init() {
exceptionUnmarshallers.add(new UnsupportedProtocolExceptionUnmarshaller());
exceptionUnmarshallers.add(new LoadBalancerAttributeNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new LoadBalancerNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyLoadBalancersExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidConfigurationRequestExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSecurityGroupExceptionUnmarshaller());
exceptionUnmarshallers.add(new DuplicateLoadBalancerNameExceptionUnmarshaller());
exceptionUnmarshallers.add(new DependencyThrottleExceptionUnmarshaller());
exceptionUnmarshallers.add(new PolicyNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new CertificateNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DuplicateTagKeysExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyTagsExceptionUnmarshaller());
exceptionUnmarshallers.add(new ListenerNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyPoliciesExceptionUnmarshaller());
exceptionUnmarshallers.add(new DuplicatePolicyNameExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubnetNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DuplicateListenerExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSchemeExceptionUnmarshaller());
exceptionUnmarshallers.add(new PolicyTypeNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSubnetExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidInstanceExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.elasticloadbalancing.model.AmazonElasticLoadBalancingException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("elasticloadbalancing.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/elasticloadbalancing/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/elasticloadbalancing/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Adds the specified tags to the specified load balancer. Each load balancer can have a maximum of 10 tags.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the load
* balancer, AddTags
updates its value.
*
*
* For more information, see Tag Your Classic Load
* Balancer in the Classic Load Balancers Guide.
*
*
* @param addTagsRequest
* Contains the parameters for AddTags.
* @return Result of the AddTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TooManyTagsException
* The quota for the number of tags that can be assigned to a load balancer has been reached.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @sample AmazonElasticLoadBalancing.AddTags
* @see AWS
* API Documentation
*/
@Override
public AddTagsResult addTags(AddTagsRequest addTagsRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsRequestMarshaller().marshall(super.beforeMarshalling(addTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new AddTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates one or more security groups with your load balancer in a virtual private cloud (VPC). The specified
* security groups override the previously associated security groups.
*
*
* For more information, see Security Groups for Load Balancers in a VPC in the Classic Load Balancers Guide.
*
*
* @param applySecurityGroupsToLoadBalancerRequest
* Contains the parameters for ApplySecurityGroupsToLoadBalancer.
* @return Result of the ApplySecurityGroupsToLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws InvalidSecurityGroupException
* One or more of the specified security groups do not exist.
* @sample AmazonElasticLoadBalancing.ApplySecurityGroupsToLoadBalancer
* @see AWS API Documentation
*/
@Override
public ApplySecurityGroupsToLoadBalancerResult applySecurityGroupsToLoadBalancer(
ApplySecurityGroupsToLoadBalancerRequest applySecurityGroupsToLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(applySecurityGroupsToLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ApplySecurityGroupsToLoadBalancerRequestMarshaller().marshall(super.beforeMarshalling(applySecurityGroupsToLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ApplySecurityGroupsToLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds one or more subnets to the set of configured subnets for the specified load balancer.
*
*
* The load balancer evenly distributes requests across all registered subnets. For more information, see Add or Remove
* Subnets for Your Load Balancer in a VPC in the Classic Load Balancers Guide.
*
*
* @param attachLoadBalancerToSubnetsRequest
* Contains the parameters for AttachLoaBalancerToSubnets.
* @return Result of the AttachLoadBalancerToSubnets operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SubnetNotFoundException
* One or more of the specified subnets do not exist.
* @throws InvalidSubnetException
* The specified VPC has no associated Internet gateway.
* @sample AmazonElasticLoadBalancing.AttachLoadBalancerToSubnets
* @see AWS API Documentation
*/
@Override
public AttachLoadBalancerToSubnetsResult attachLoadBalancerToSubnets(AttachLoadBalancerToSubnetsRequest attachLoadBalancerToSubnetsRequest) {
ExecutionContext executionContext = createExecutionContext(attachLoadBalancerToSubnetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AttachLoadBalancerToSubnetsRequestMarshaller().marshall(super.beforeMarshalling(attachLoadBalancerToSubnetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AttachLoadBalancerToSubnetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Specifies the health check settings to use when evaluating the health state of your EC2 instances.
*
*
* For more information, see Configure Health
* Checks for Your Load Balancer in the Classic Load Balancers Guide.
*
*
* @param configureHealthCheckRequest
* Contains the parameters for ConfigureHealthCheck.
* @return Result of the ConfigureHealthCheck operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.ConfigureHealthCheck
* @see AWS API Documentation
*/
@Override
public ConfigureHealthCheckResult configureHealthCheck(ConfigureHealthCheckRequest configureHealthCheckRequest) {
ExecutionContext executionContext = createExecutionContext(configureHealthCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ConfigureHealthCheckRequestMarshaller().marshall(super.beforeMarshalling(configureHealthCheckRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ConfigureHealthCheckResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Generates a stickiness policy with sticky session lifetimes that follow that of an application-generated cookie.
* This policy can be associated only with HTTP/HTTPS listeners.
*
*
* This policy is similar to the policy created by CreateLBCookieStickinessPolicy, except that the lifetime
* of the special Elastic Load Balancing cookie, AWSELB
, follows the lifetime of the
* application-generated cookie specified in the policy configuration. The load balancer only inserts a new
* stickiness cookie when the application response includes a new application cookie.
*
*
* If the application cookie is explicitly removed or expires, the session stops being sticky until a new
* application cookie is issued.
*
*
* For more information, see Application-Controlled Session Stickiness in the Classic Load Balancers Guide.
*
*
* @param createAppCookieStickinessPolicyRequest
* Contains the parameters for CreateAppCookieStickinessPolicy.
* @return Result of the CreateAppCookieStickinessPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DuplicatePolicyNameException
* A policy with the specified name already exists for this load balancer.
* @throws TooManyPoliciesException
* The quota for the number of policies for this load balancer has been reached.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.CreateAppCookieStickinessPolicy
* @see AWS API Documentation
*/
@Override
public CreateAppCookieStickinessPolicyResult createAppCookieStickinessPolicy(CreateAppCookieStickinessPolicyRequest createAppCookieStickinessPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createAppCookieStickinessPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppCookieStickinessPolicyRequestMarshaller().marshall(super.beforeMarshalling(createAppCookieStickinessPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateAppCookieStickinessPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Generates a stickiness policy with sticky session lifetimes controlled by the lifetime of the browser
* (user-agent) or a specified expiration period. This policy can be associated only with HTTP/HTTPS listeners.
*
*
* When a load balancer implements this policy, the load balancer uses a special cookie to track the instance for
* each request. When the load balancer receives a request, it first checks to see if this cookie is present in the
* request. If so, the load balancer sends the request to the application server specified in the cookie. If not,
* the load balancer sends the request to a server that is chosen based on the existing load-balancing algorithm.
*
*
* A cookie is inserted into the response for binding subsequent requests from the same user to that server. The
* validity of the cookie is based on the cookie expiration time, which is specified in the policy configuration.
*
*
* For more information, see Duration-Based Session Stickiness in the Classic Load Balancers Guide.
*
*
* @param createLBCookieStickinessPolicyRequest
* Contains the parameters for CreateLBCookieStickinessPolicy.
* @return Result of the CreateLBCookieStickinessPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DuplicatePolicyNameException
* A policy with the specified name already exists for this load balancer.
* @throws TooManyPoliciesException
* The quota for the number of policies for this load balancer has been reached.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.CreateLBCookieStickinessPolicy
* @see AWS API Documentation
*/
@Override
public CreateLBCookieStickinessPolicyResult createLBCookieStickinessPolicy(CreateLBCookieStickinessPolicyRequest createLBCookieStickinessPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createLBCookieStickinessPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLBCookieStickinessPolicyRequestMarshaller().marshall(super.beforeMarshalling(createLBCookieStickinessPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateLBCookieStickinessPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Classic load balancer.
*
*
* You can add listeners, security groups, subnets, and tags when you create your load balancer, or you can add them
* later using CreateLoadBalancerListeners, ApplySecurityGroupsToLoadBalancer,
* AttachLoadBalancerToSubnets, and AddTags.
*
*
* To describe your current load balancers, see DescribeLoadBalancers. When you are finished with a load
* balancer, you can delete it using DeleteLoadBalancer.
*
*
* You can create up to 20 load balancers per region per account. You can request an increase for the number of load
* balancers for your account. For more information, see Limits for Your Classic
* Load Balancer in the Classic Load Balancers Guide.
*
*
* @param createLoadBalancerRequest
* Contains the parameters for CreateLoadBalancer.
* @return Result of the CreateLoadBalancer operation returned by the service.
* @throws DuplicateLoadBalancerNameException
* The specified load balancer name already exists for this account.
* @throws TooManyLoadBalancersException
* The quota for the number of load balancers has been reached.
* @throws CertificateNotFoundException
* The specified ARN does not refer to a valid SSL certificate in AWS Identity and Access Management (IAM)
* or AWS Certificate Manager (ACM). Note that if you recently uploaded the certificate to IAM, this error
* might indicate that the certificate is not fully available yet.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SubnetNotFoundException
* One or more of the specified subnets do not exist.
* @throws InvalidSubnetException
* The specified VPC has no associated Internet gateway.
* @throws InvalidSecurityGroupException
* One or more of the specified security groups do not exist.
* @throws InvalidSchemeException
* The specified value for the schema is not valid. You can only specify a scheme for load balancers in a
* VPC.
* @throws TooManyTagsException
* The quota for the number of tags that can be assigned to a load balancer has been reached.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws UnsupportedProtocolException
* @sample AmazonElasticLoadBalancing.CreateLoadBalancer
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerResult createLoadBalancer(CreateLoadBalancerRequest createLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(createLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLoadBalancerRequestMarshaller().marshall(super.beforeMarshalling(createLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates one or more listeners for the specified load balancer. If a listener with the specified port does not
* already exist, it is created; otherwise, the properties of the new listener must match the properties of the
* existing listener.
*
*
* For more information, see Listeners for Your
* Classic Load Balancer in the Classic Load Balancers Guide.
*
*
* @param createLoadBalancerListenersRequest
* Contains the parameters for CreateLoadBalancerListeners.
* @return Result of the CreateLoadBalancerListeners operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DuplicateListenerException
* A listener already exists for the specified load balancer name and port, but with a different instance
* port, protocol, or SSL certificate.
* @throws CertificateNotFoundException
* The specified ARN does not refer to a valid SSL certificate in AWS Identity and Access Management (IAM)
* or AWS Certificate Manager (ACM). Note that if you recently uploaded the certificate to IAM, this error
* might indicate that the certificate is not fully available yet.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws UnsupportedProtocolException
* @sample AmazonElasticLoadBalancing.CreateLoadBalancerListeners
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerListenersResult createLoadBalancerListeners(CreateLoadBalancerListenersRequest createLoadBalancerListenersRequest) {
ExecutionContext executionContext = createExecutionContext(createLoadBalancerListenersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLoadBalancerListenersRequestMarshaller().marshall(super.beforeMarshalling(createLoadBalancerListenersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateLoadBalancerListenersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a policy with the specified attributes for the specified load balancer.
*
*
* Policies are settings that are saved for your load balancer and that can be applied to the listener or the
* application server, depending on the policy type.
*
*
* @param createLoadBalancerPolicyRequest
* Contains the parameters for CreateLoadBalancerPolicy.
* @return Result of the CreateLoadBalancerPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyTypeNotFoundException
* One or more of the specified policy types do not exist.
* @throws DuplicatePolicyNameException
* A policy with the specified name already exists for this load balancer.
* @throws TooManyPoliciesException
* The quota for the number of policies for this load balancer has been reached.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.CreateLoadBalancerPolicy
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerPolicyResult createLoadBalancerPolicy(CreateLoadBalancerPolicyRequest createLoadBalancerPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createLoadBalancerPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLoadBalancerPolicyRequestMarshaller().marshall(super.beforeMarshalling(createLoadBalancerPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateLoadBalancerPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified load balancer.
*
*
* If you are attempting to recreate a load balancer, you must reconfigure all settings. The DNS name associated
* with a deleted load balancer are no longer usable. The name and associated DNS record of the deleted load
* balancer no longer exist and traffic sent to any of its IP addresses is no longer delivered to your instances.
*
*
* If the load balancer does not exist or has already been deleted, the call to DeleteLoadBalancer
* still succeeds.
*
*
* @param deleteLoadBalancerRequest
* Contains the parameters for DeleteLoadBalancer.
* @return Result of the DeleteLoadBalancer operation returned by the service.
* @sample AmazonElasticLoadBalancing.DeleteLoadBalancer
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerResult deleteLoadBalancer(DeleteLoadBalancerRequest deleteLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLoadBalancerRequestMarshaller().marshall(super.beforeMarshalling(deleteLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified listeners from the specified load balancer.
*
*
* @param deleteLoadBalancerListenersRequest
* Contains the parameters for DeleteLoadBalancerListeners.
* @return Result of the DeleteLoadBalancerListeners operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.DeleteLoadBalancerListeners
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerListenersResult deleteLoadBalancerListeners(DeleteLoadBalancerListenersRequest deleteLoadBalancerListenersRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLoadBalancerListenersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLoadBalancerListenersRequestMarshaller().marshall(super.beforeMarshalling(deleteLoadBalancerListenersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteLoadBalancerListenersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified policy from the specified load balancer. This policy must not be enabled for any listeners.
*
*
* @param deleteLoadBalancerPolicyRequest
* Contains the parameters for DeleteLoadBalancerPolicy.
* @return Result of the DeleteLoadBalancerPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.DeleteLoadBalancerPolicy
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerPolicyResult deleteLoadBalancerPolicy(DeleteLoadBalancerPolicyRequest deleteLoadBalancerPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLoadBalancerPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLoadBalancerPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteLoadBalancerPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteLoadBalancerPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deregisters the specified instances from the specified load balancer. After the instance is deregistered, it no
* longer receives traffic from the load balancer.
*
*
* You can use DescribeLoadBalancers to verify that the instance is deregistered from the load balancer.
*
*
* For more information, see Register or De-Register EC2 Instances in the Classic Load Balancers Guide.
*
*
* @param deregisterInstancesFromLoadBalancerRequest
* Contains the parameters for DeregisterInstancesFromLoadBalancer.
* @return Result of the DeregisterInstancesFromLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidInstanceException
* The specified endpoint is not valid.
* @sample AmazonElasticLoadBalancing.DeregisterInstancesFromLoadBalancer
* @see AWS API Documentation
*/
@Override
public DeregisterInstancesFromLoadBalancerResult deregisterInstancesFromLoadBalancer(
DeregisterInstancesFromLoadBalancerRequest deregisterInstancesFromLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterInstancesFromLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterInstancesFromLoadBalancerRequestMarshaller().marshall(super
.beforeMarshalling(deregisterInstancesFromLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeregisterInstancesFromLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the state of the specified instances with respect to the specified load balancer. If no instances are
* specified, the call describes the state of all instances that are currently registered with the load balancer. If
* instances are specified, their state is returned even if they are no longer registered with the load balancer.
* The state of terminated instances is not returned.
*
*
* @param describeInstanceHealthRequest
* Contains the parameters for DescribeInstanceHealth.
* @return Result of the DescribeInstanceHealth operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidInstanceException
* The specified endpoint is not valid.
* @sample AmazonElasticLoadBalancing.DescribeInstanceHealth
* @see AWS API Documentation
*/
@Override
public DescribeInstanceHealthResult describeInstanceHealth(DescribeInstanceHealthRequest describeInstanceHealthRequest) {
ExecutionContext executionContext = createExecutionContext(describeInstanceHealthRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeInstanceHealthRequestMarshaller().marshall(super.beforeMarshalling(describeInstanceHealthRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeInstanceHealthResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the attributes for the specified load balancer.
*
*
* @param describeLoadBalancerAttributesRequest
* Contains the parameters for DescribeLoadBalancerAttributes.
* @return Result of the DescribeLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws LoadBalancerAttributeNotFoundException
* The specified load balancer attribute does not exist.
* @sample AmazonElasticLoadBalancing.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerAttributesResult describeLoadBalancerAttributes(DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoadBalancerAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoadBalancerAttributesRequestMarshaller().marshall(super.beforeMarshalling(describeLoadBalancerAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeLoadBalancerAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified policies.
*
*
* If you specify a load balancer name, the action returns the descriptions of all policies created for the load
* balancer. If you specify a policy name associated with your load balancer, the action returns the description of
* that policy. If you don't specify a load balancer name, the action returns descriptions of the specified sample
* policies, or descriptions of all sample policies. The names of the sample policies have the
* ELBSample-
prefix.
*
*
* @param describeLoadBalancerPoliciesRequest
* Contains the parameters for DescribeLoadBalancerPolicies.
* @return Result of the DescribeLoadBalancerPolicies operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyNotFoundException
* One or more of the specified policies do not exist.
* @sample AmazonElasticLoadBalancing.DescribeLoadBalancerPolicies
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerPoliciesResult describeLoadBalancerPolicies(DescribeLoadBalancerPoliciesRequest describeLoadBalancerPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoadBalancerPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoadBalancerPoliciesRequestMarshaller().marshall(super.beforeMarshalling(describeLoadBalancerPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeLoadBalancerPoliciesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeLoadBalancerPoliciesResult describeLoadBalancerPolicies() {
return describeLoadBalancerPolicies(new DescribeLoadBalancerPoliciesRequest());
}
/**
*
* Describes the specified load balancer policy types or all load balancer policy types.
*
*
* The description of each type indicates how it can be used. For example, some policies can be used only with layer
* 7 listeners, some policies can be used only with layer 4 listeners, and some policies can be used only with your
* EC2 instances.
*
*
* You can use CreateLoadBalancerPolicy to create a policy configuration for any of these policy types. Then,
* depending on the policy type, use either SetLoadBalancerPoliciesOfListener or
* SetLoadBalancerPoliciesForBackendServer to set the policy.
*
*
* @param describeLoadBalancerPolicyTypesRequest
* Contains the parameters for DescribeLoadBalancerPolicyTypes.
* @return Result of the DescribeLoadBalancerPolicyTypes operation returned by the service.
* @throws PolicyTypeNotFoundException
* One or more of the specified policy types do not exist.
* @sample AmazonElasticLoadBalancing.DescribeLoadBalancerPolicyTypes
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerPolicyTypesResult describeLoadBalancerPolicyTypes(DescribeLoadBalancerPolicyTypesRequest describeLoadBalancerPolicyTypesRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoadBalancerPolicyTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoadBalancerPolicyTypesRequestMarshaller().marshall(super.beforeMarshalling(describeLoadBalancerPolicyTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeLoadBalancerPolicyTypesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeLoadBalancerPolicyTypesResult describeLoadBalancerPolicyTypes() {
return describeLoadBalancerPolicyTypes(new DescribeLoadBalancerPolicyTypesRequest());
}
/**
*
* Describes the specified the load balancers. If no load balancers are specified, the call describes all of your
* load balancers.
*
*
* @param describeLoadBalancersRequest
* Contains the parameters for DescribeLoadBalancers.
* @return Result of the DescribeLoadBalancers operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DependencyThrottleException
* @sample AmazonElasticLoadBalancing.DescribeLoadBalancers
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancersResult describeLoadBalancers(DescribeLoadBalancersRequest describeLoadBalancersRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoadBalancersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoadBalancersRequestMarshaller().marshall(super.beforeMarshalling(describeLoadBalancersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeLoadBalancersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeLoadBalancersResult describeLoadBalancers() {
return describeLoadBalancers(new DescribeLoadBalancersRequest());
}
/**
*
* Describes the tags associated with the specified load balancers.
*
*
* @param describeTagsRequest
* Contains the parameters for DescribeTags.
* @return Result of the DescribeTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTags
* @see AWS API Documentation
*/
@Override
public DescribeTagsResult describeTags(DescribeTagsRequest describeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(describeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTagsRequestMarshaller().marshall(super.beforeMarshalling(describeTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DescribeTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified subnets from the set of configured subnets for the load balancer.
*
*
* After a subnet is removed, all EC2 instances registered with the load balancer in the removed subnet go into the
* OutOfService
state. Then, the load balancer balances the traffic among the remaining routable
* subnets.
*
*
* @param detachLoadBalancerFromSubnetsRequest
* Contains the parameters for DetachLoadBalancerFromSubnets.
* @return Result of the DetachLoadBalancerFromSubnets operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.DetachLoadBalancerFromSubnets
* @see AWS API Documentation
*/
@Override
public DetachLoadBalancerFromSubnetsResult detachLoadBalancerFromSubnets(DetachLoadBalancerFromSubnetsRequest detachLoadBalancerFromSubnetsRequest) {
ExecutionContext executionContext = createExecutionContext(detachLoadBalancerFromSubnetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetachLoadBalancerFromSubnetsRequestMarshaller().marshall(super.beforeMarshalling(detachLoadBalancerFromSubnetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetachLoadBalancerFromSubnetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified Availability Zones from the set of Availability Zones for the specified load balancer.
*
*
* There must be at least one Availability Zone registered with a load balancer at all times. After an Availability
* Zone is removed, all instances registered with the load balancer that are in the removed Availability Zone go
* into the OutOfService
state. Then, the load balancer attempts to equally balance the traffic among
* its remaining Availability Zones.
*
*
* For more information, see Add or Remove
* Availability Zones in the Classic Load Balancers Guide.
*
*
* @param disableAvailabilityZonesForLoadBalancerRequest
* Contains the parameters for DisableAvailabilityZonesForLoadBalancer.
* @return Result of the DisableAvailabilityZonesForLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.DisableAvailabilityZonesForLoadBalancer
* @see AWS API Documentation
*/
@Override
public DisableAvailabilityZonesForLoadBalancerResult disableAvailabilityZonesForLoadBalancer(
DisableAvailabilityZonesForLoadBalancerRequest disableAvailabilityZonesForLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(disableAvailabilityZonesForLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableAvailabilityZonesForLoadBalancerRequestMarshaller().marshall(super
.beforeMarshalling(disableAvailabilityZonesForLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DisableAvailabilityZonesForLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified Availability Zones to the set of Availability Zones for the specified load balancer.
*
*
* The load balancer evenly distributes requests across all its registered Availability Zones that contain
* instances.
*
*
* For more information, see Add or Remove
* Availability Zones in the Classic Load Balancers Guide.
*
*
* @param enableAvailabilityZonesForLoadBalancerRequest
* Contains the parameters for EnableAvailabilityZonesForLoadBalancer.
* @return Result of the EnableAvailabilityZonesForLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.EnableAvailabilityZonesForLoadBalancer
* @see AWS API Documentation
*/
@Override
public EnableAvailabilityZonesForLoadBalancerResult enableAvailabilityZonesForLoadBalancer(
EnableAvailabilityZonesForLoadBalancerRequest enableAvailabilityZonesForLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(enableAvailabilityZonesForLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableAvailabilityZonesForLoadBalancerRequestMarshaller().marshall(super
.beforeMarshalling(enableAvailabilityZonesForLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new EnableAvailabilityZonesForLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the attributes of the specified load balancer.
*
*
* You can modify the load balancer attributes, such as AccessLogs
, ConnectionDraining
,
* and CrossZoneLoadBalancing
by either enabling or disabling them. Or, you can modify the load
* balancer attribute ConnectionSettings
by specifying an idle connection timeout value for your load
* balancer.
*
*
* For more information, see the following in the Classic Load Balancers Guide:
*
*
* -
*
*
* -
*
*
* -
*
* Access
* Logs
*
*
* -
*
*
*
*
* @param modifyLoadBalancerAttributesRequest
* Contains the parameters for ModifyLoadBalancerAttributes.
* @return Result of the ModifyLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws LoadBalancerAttributeNotFoundException
* The specified load balancer attribute does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public ModifyLoadBalancerAttributesResult modifyLoadBalancerAttributes(ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(modifyLoadBalancerAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ModifyLoadBalancerAttributesRequestMarshaller().marshall(super.beforeMarshalling(modifyLoadBalancerAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ModifyLoadBalancerAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified instances to the specified load balancer.
*
*
* The instance must be a running instance in the same network as the load balancer (EC2-Classic or the same VPC).
* If you have EC2-Classic instances and a load balancer in a VPC with ClassicLink enabled, you can link the
* EC2-Classic instances to that VPC and then register the linked EC2-Classic instances with the load balancer in
* the VPC.
*
*
* Note that RegisterInstanceWithLoadBalancer
completes when the request has been registered. Instance
* registration takes a little time to complete. To check the state of the registered instances, use
* DescribeLoadBalancers or DescribeInstanceHealth.
*
*
* After the instance is registered, it starts receiving traffic and requests from the load balancer. Any instance
* that is not in one of the Availability Zones registered for the load balancer is moved to the
* OutOfService
state. If an Availability Zone is added to the load balancer later, any instances
* registered with the load balancer move to the InService
state.
*
*
* To deregister instances from a load balancer, use DeregisterInstancesFromLoadBalancer.
*
*
* For more information, see Register or De-Register EC2 Instances in the Classic Load Balancers Guide.
*
*
* @param registerInstancesWithLoadBalancerRequest
* Contains the parameters for RegisterInstancesWithLoadBalancer.
* @return Result of the RegisterInstancesWithLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidInstanceException
* The specified endpoint is not valid.
* @sample AmazonElasticLoadBalancing.RegisterInstancesWithLoadBalancer
* @see AWS API Documentation
*/
@Override
public RegisterInstancesWithLoadBalancerResult registerInstancesWithLoadBalancer(
RegisterInstancesWithLoadBalancerRequest registerInstancesWithLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(registerInstancesWithLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterInstancesWithLoadBalancerRequestMarshaller().marshall(super.beforeMarshalling(registerInstancesWithLoadBalancerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new RegisterInstancesWithLoadBalancerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more tags from the specified load balancer.
*
*
* @param removeTagsRequest
* Contains the parameters for RemoveTags.
* @return Result of the RemoveTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.RemoveTags
* @see AWS API Documentation
*/
@Override
public RemoveTagsResult removeTags(RemoveTagsRequest removeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(removeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTagsRequestMarshaller().marshall(super.beforeMarshalling(removeTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new RemoveTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets the certificate that terminates the specified listener's SSL connections. The specified certificate replaces
* any prior certificate that was used on the same load balancer and port.
*
*
* For more information about updating your SSL certificate, see Replace the SSL
* Certificate for Your Load Balancer in the Classic Load Balancers Guide.
*
*
* @param setLoadBalancerListenerSSLCertificateRequest
* Contains the parameters for SetLoadBalancerListenerSSLCertificate.
* @return Result of the SetLoadBalancerListenerSSLCertificate operation returned by the service.
* @throws CertificateNotFoundException
* The specified ARN does not refer to a valid SSL certificate in AWS Identity and Access Management (IAM)
* or AWS Certificate Manager (ACM). Note that if you recently uploaded the certificate to IAM, this error
* might indicate that the certificate is not fully available yet.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws ListenerNotFoundException
* The load balancer does not have a listener configured at the specified port.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws UnsupportedProtocolException
* @sample AmazonElasticLoadBalancing.SetLoadBalancerListenerSSLCertificate
* @see AWS API Documentation
*/
@Override
public SetLoadBalancerListenerSSLCertificateResult setLoadBalancerListenerSSLCertificate(
SetLoadBalancerListenerSSLCertificateRequest setLoadBalancerListenerSSLCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(setLoadBalancerListenerSSLCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetLoadBalancerListenerSSLCertificateRequestMarshaller().marshall(super
.beforeMarshalling(setLoadBalancerListenerSSLCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetLoadBalancerListenerSSLCertificateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Replaces the set of policies associated with the specified port on which the EC2 instance is listening with a new
* set of policies. At this time, only the back-end server authentication policy type can be applied to the instance
* ports; this policy type is composed of multiple public key policies.
*
*
* Each time you use SetLoadBalancerPoliciesForBackendServer
to enable the policies, use the
* PolicyNames
parameter to list the policies that you want to enable.
*
*
* You can use DescribeLoadBalancers or DescribeLoadBalancerPolicies to verify that the policy is
* associated with the EC2 instance.
*
*
* For more information about enabling back-end instance authentication, see Configure Back-end Instance Authentication in the Classic Load Balancers Guide. For more information
* about Proxy Protocol, see Configure Proxy
* Protocol Support in the Classic Load Balancers Guide.
*
*
* @param setLoadBalancerPoliciesForBackendServerRequest
* Contains the parameters for SetLoadBalancerPoliciesForBackendServer.
* @return Result of the SetLoadBalancerPoliciesForBackendServer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyNotFoundException
* One or more of the specified policies do not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.SetLoadBalancerPoliciesForBackendServer
* @see AWS API Documentation
*/
@Override
public SetLoadBalancerPoliciesForBackendServerResult setLoadBalancerPoliciesForBackendServer(
SetLoadBalancerPoliciesForBackendServerRequest setLoadBalancerPoliciesForBackendServerRequest) {
ExecutionContext executionContext = createExecutionContext(setLoadBalancerPoliciesForBackendServerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetLoadBalancerPoliciesForBackendServerRequestMarshaller().marshall(super
.beforeMarshalling(setLoadBalancerPoliciesForBackendServerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetLoadBalancerPoliciesForBackendServerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Replaces the current set of policies for the specified load balancer port with the specified set of policies.
*
*
* To enable back-end server authentication, use SetLoadBalancerPoliciesForBackendServer.
*
*
* For more information about setting policies, see Update the SSL
* Negotiation Configuration, Duration-Based Session Stickiness, and Application-Controlled Session Stickiness in the Classic Load Balancers Guide.
*
*
* @param setLoadBalancerPoliciesOfListenerRequest
* Contains the parameters for SetLoadBalancePoliciesOfListener.
* @return Result of the SetLoadBalancerPoliciesOfListener operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyNotFoundException
* One or more of the specified policies do not exist.
* @throws ListenerNotFoundException
* The load balancer does not have a listener configured at the specified port.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @sample AmazonElasticLoadBalancing.SetLoadBalancerPoliciesOfListener
* @see AWS API Documentation
*/
@Override
public SetLoadBalancerPoliciesOfListenerResult setLoadBalancerPoliciesOfListener(
SetLoadBalancerPoliciesOfListenerRequest setLoadBalancerPoliciesOfListenerRequest) {
ExecutionContext executionContext = createExecutionContext(setLoadBalancerPoliciesOfListenerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetLoadBalancerPoliciesOfListenerRequestMarshaller().marshall(super.beforeMarshalling(setLoadBalancerPoliciesOfListenerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetLoadBalancerPoliciesOfListenerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@Override
public AmazonElasticLoadBalancingWaiters waiters() {
if (waiters == null) {
synchronized (this) {
if (waiters == null) {
waiters = new AmazonElasticLoadBalancingWaiters(this);
}
}
}
return waiters;
}
}