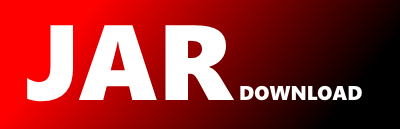
com.amazonaws.services.elasticloadbalancingv2.AmazonElasticLoadBalancingAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancingv2 Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancingv2;
import com.amazonaws.services.elasticloadbalancingv2.model.*;
/**
* Interface for accessing Elastic Load Balancing v2 asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Elastic Load Balancing
*
* A load balancer distributes incoming traffic across targets, such as your EC2 instances. This enables you to increase
* the availability of your application. The load balancer also monitors the health of its registered targets and
* ensures that it routes traffic only to healthy targets. You configure your load balancer to accept incoming traffic
* by specifying one or more listeners, which are configured with a protocol and port number for connections from
* clients to the load balancer. You configure a target group with a protocol and port number for connections from the
* load balancer to the targets, and with health check settings to be used when checking the health status of the
* targets.
*
*
* Elastic Load Balancing supports two types of load balancers: Classic load balancers and Application load balancers
* (new). A Classic load balancer makes routing and load balancing decisions either at the transport layer (TCP/SSL) or
* the application layer (HTTP/HTTPS), and supports either EC2-Classic or a VPC. An Application load balancer makes
* routing and load balancing decisions at the application layer (HTTP/HTTPS), supports path-based routing, and can
* route requests to one or more ports on each EC2 instance or container instance in your virtual private cloud (VPC).
* For more information, see the Elastic
* Load Balancing User Guide.
*
*
* This reference covers the 2015-12-01 API, which supports Application load balancers. The 2012-06-01 API supports
* Classic load balancers.
*
*
* To get started with an Application load balancer, complete the following tasks:
*
*
* -
*
* Create a load balancer using CreateLoadBalancer.
*
*
* -
*
* Create a target group using CreateTargetGroup.
*
*
* -
*
* Register targets for the target group using RegisterTargets.
*
*
* -
*
* Create one or more listeners for your load balancer using CreateListener.
*
*
* -
*
* (Optional) Create one or more rules for content routing based on URL using CreateRule.
*
*
*
*
* To delete an Application load balancer and its related resources, complete the following tasks:
*
*
* -
*
* Delete the load balancer using DeleteLoadBalancer.
*
*
* -
*
* Delete the target group using DeleteTargetGroup.
*
*
*
*
* All Elastic Load Balancing operations are idempotent, which means that they complete at most one time. If you repeat
* an operation, it succeeds.
*
*/
public interface AmazonElasticLoadBalancingAsync extends AmazonElasticLoadBalancing {
/**
*
* Adds the specified tags to the specified resource. You can tag your Application load balancers and your target
* groups.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
* To list the current tags for your resources, use DescribeTags. To remove tags from your resources, use
* RemoveTags.
*
*
* @param addTagsRequest
* Contains the parameters for AddTags.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.AddTags
*/
java.util.concurrent.Future addTagsAsync(AddTagsRequest addTagsRequest);
/**
*
* Adds the specified tags to the specified resource. You can tag your Application load balancers and your target
* groups.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
* To list the current tags for your resources, use DescribeTags. To remove tags from your resources, use
* RemoveTags.
*
*
* @param addTagsRequest
* Contains the parameters for AddTags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.AddTags
*/
java.util.concurrent.Future addTagsAsync(AddTagsRequest addTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a listener for the specified Application load balancer.
*
*
* To update a listener, use ModifyListener. When you are finished with a listener, you can delete it using
* DeleteListener. If you are finished with both the listener and the load balancer, you can delete them both
* using DeleteLoadBalancer.
*
*
* For more information, see Listeners
* for Your Application Load Balancers in the Application Load Balancers Guide.
*
*
* @param createListenerRequest
* Contains the parameters for CreateListener.
* @return A Java Future containing the result of the CreateListener operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.CreateListener
*/
java.util.concurrent.Future createListenerAsync(CreateListenerRequest createListenerRequest);
/**
*
* Creates a listener for the specified Application load balancer.
*
*
* To update a listener, use ModifyListener. When you are finished with a listener, you can delete it using
* DeleteListener. If you are finished with both the listener and the load balancer, you can delete them both
* using DeleteLoadBalancer.
*
*
* For more information, see Listeners
* for Your Application Load Balancers in the Application Load Balancers Guide.
*
*
* @param createListenerRequest
* Contains the parameters for CreateListener.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateListener operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.CreateListener
*/
java.util.concurrent.Future createListenerAsync(CreateListenerRequest createListenerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Application load balancer.
*
*
* To create listeners for your load balancer, use CreateListener. You can add security groups, subnets, and
* tags when you create your load balancer, or you can add them later using SetSecurityGroups,
* SetSubnets, and AddTags.
*
*
* To describe your current load balancers, see DescribeLoadBalancers. When you are finished with a load
* balancer, you can delete it using DeleteLoadBalancer.
*
*
* You can create up to 20 load balancers per region per account. You can request an increase for the number of load
* balancers for your account. For more information, see Limits for
* Your Application Load Balancer in the Application Load Balancers Guide.
*
*
* @param createLoadBalancerRequest
* Contains the parameters for CreateLoadBalancer.
* @return A Java Future containing the result of the CreateLoadBalancer operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.CreateLoadBalancer
*/
java.util.concurrent.Future createLoadBalancerAsync(CreateLoadBalancerRequest createLoadBalancerRequest);
/**
*
* Creates an Application load balancer.
*
*
* To create listeners for your load balancer, use CreateListener. You can add security groups, subnets, and
* tags when you create your load balancer, or you can add them later using SetSecurityGroups,
* SetSubnets, and AddTags.
*
*
* To describe your current load balancers, see DescribeLoadBalancers. When you are finished with a load
* balancer, you can delete it using DeleteLoadBalancer.
*
*
* You can create up to 20 load balancers per region per account. You can request an increase for the number of load
* balancers for your account. For more information, see Limits for
* Your Application Load Balancer in the Application Load Balancers Guide.
*
*
* @param createLoadBalancerRequest
* Contains the parameters for CreateLoadBalancer.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLoadBalancer operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.CreateLoadBalancer
*/
java.util.concurrent.Future createLoadBalancerAsync(CreateLoadBalancerRequest createLoadBalancerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a rule for the specified listener.
*
*
* A rule consists conditions and actions. Rules are evaluated in priority order, from the lowest value to the
* highest value. When the conditions for a rule are met, the specified actions are taken. If no rule's conditions
* are met, the default actions for the listener are taken.
*
*
* To view your current rules, use DescribeRules. To update a rule, use ModifyRule. To set the
* priorities of your rules, use SetRulePriorities. To delete a rule, use DeleteRule.
*
*
* @param createRuleRequest
* Contains the parameters for CreateRule.
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.CreateRule
*/
java.util.concurrent.Future createRuleAsync(CreateRuleRequest createRuleRequest);
/**
*
* Creates a rule for the specified listener.
*
*
* A rule consists conditions and actions. Rules are evaluated in priority order, from the lowest value to the
* highest value. When the conditions for a rule are met, the specified actions are taken. If no rule's conditions
* are met, the default actions for the listener are taken.
*
*
* To view your current rules, use DescribeRules. To update a rule, use ModifyRule. To set the
* priorities of your rules, use SetRulePriorities. To delete a rule, use DeleteRule.
*
*
* @param createRuleRequest
* Contains the parameters for CreateRule.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.CreateRule
*/
java.util.concurrent.Future createRuleAsync(CreateRuleRequest createRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a target group.
*
*
* To register targets with the target group, use RegisterTargets. To update the health check settings for
* the target group, use ModifyTargetGroup. To monitor the health of targets in the target group, use
* DescribeTargetHealth.
*
*
* To route traffic to the targets in a target group, specify the target group in an action using
* CreateListener or CreateRule.
*
*
* To delete a target group, use DeleteTargetGroup.
*
*
* For more information, see Target
* Groups for Your Application Load Balancers in the Application Load Balancers Guide.
*
*
* @param createTargetGroupRequest
* Contains the parameters for CreateTargetGroup.
* @return A Java Future containing the result of the CreateTargetGroup operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.CreateTargetGroup
*/
java.util.concurrent.Future createTargetGroupAsync(CreateTargetGroupRequest createTargetGroupRequest);
/**
*
* Creates a target group.
*
*
* To register targets with the target group, use RegisterTargets. To update the health check settings for
* the target group, use ModifyTargetGroup. To monitor the health of targets in the target group, use
* DescribeTargetHealth.
*
*
* To route traffic to the targets in a target group, specify the target group in an action using
* CreateListener or CreateRule.
*
*
* To delete a target group, use DeleteTargetGroup.
*
*
* For more information, see Target
* Groups for Your Application Load Balancers in the Application Load Balancers Guide.
*
*
* @param createTargetGroupRequest
* Contains the parameters for CreateTargetGroup.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTargetGroup operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.CreateTargetGroup
*/
java.util.concurrent.Future createTargetGroupAsync(CreateTargetGroupRequest createTargetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer it is attached to using
* DeleteLoadBalancer.
*
*
* @param deleteListenerRequest
* Contains the parameters for DeleteListener.
* @return A Java Future containing the result of the DeleteListener operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DeleteListener
*/
java.util.concurrent.Future deleteListenerAsync(DeleteListenerRequest deleteListenerRequest);
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer it is attached to using
* DeleteLoadBalancer.
*
*
* @param deleteListenerRequest
* Contains the parameters for DeleteListener.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteListener operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DeleteListener
*/
java.util.concurrent.Future deleteListenerAsync(DeleteListenerRequest deleteListenerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified load balancer and its attached listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
* @param deleteLoadBalancerRequest
* Contains the parameters for DeleteLoadBalancer.
* @return A Java Future containing the result of the DeleteLoadBalancer operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DeleteLoadBalancer
*/
java.util.concurrent.Future deleteLoadBalancerAsync(DeleteLoadBalancerRequest deleteLoadBalancerRequest);
/**
*
* Deletes the specified load balancer and its attached listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
* @param deleteLoadBalancerRequest
* Contains the parameters for DeleteLoadBalancer.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLoadBalancer operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DeleteLoadBalancer
*/
java.util.concurrent.Future deleteLoadBalancerAsync(DeleteLoadBalancerRequest deleteLoadBalancerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified rule.
*
*
* @param deleteRuleRequest
* Contains the parameters for DeleteRule.
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DeleteRule
*/
java.util.concurrent.Future deleteRuleAsync(DeleteRuleRequest deleteRuleRequest);
/**
*
* Deletes the specified rule.
*
*
* @param deleteRuleRequest
* Contains the parameters for DeleteRule.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DeleteRule
*/
java.util.concurrent.Future deleteRuleAsync(DeleteRuleRequest deleteRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks.
*
*
* @param deleteTargetGroupRequest
* Contains the parameters for DeleteTargetGroup.
* @return A Java Future containing the result of the DeleteTargetGroup operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DeleteTargetGroup
*/
java.util.concurrent.Future deleteTargetGroupAsync(DeleteTargetGroupRequest deleteTargetGroupRequest);
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks.
*
*
* @param deleteTargetGroupRequest
* Contains the parameters for DeleteTargetGroup.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTargetGroup operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DeleteTargetGroup
*/
java.util.concurrent.Future deleteTargetGroupAsync(DeleteTargetGroupRequest deleteTargetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* @param deregisterTargetsRequest
* Contains the parameters for DeregisterTargets.
* @return A Java Future containing the result of the DeregisterTargets operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DeregisterTargets
*/
java.util.concurrent.Future deregisterTargetsAsync(DeregisterTargetsRequest deregisterTargetsRequest);
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* @param deregisterTargetsRequest
* Contains the parameters for DeregisterTargets.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterTargets operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DeregisterTargets
*/
java.util.concurrent.Future deregisterTargetsAsync(DeregisterTargetsRequest deregisterTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified listeners or the listeners for the specified load balancer. You must specify either a
* load balancer or one or more listeners.
*
*
* @param describeListenersRequest
* Contains the parameters for DescribeListeners.
* @return A Java Future containing the result of the DescribeListeners operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeListeners
*/
java.util.concurrent.Future describeListenersAsync(DescribeListenersRequest describeListenersRequest);
/**
*
* Describes the specified listeners or the listeners for the specified load balancer. You must specify either a
* load balancer or one or more listeners.
*
*
* @param describeListenersRequest
* Contains the parameters for DescribeListeners.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeListeners operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeListeners
*/
java.util.concurrent.Future describeListenersAsync(DescribeListenersRequest describeListenersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the attributes for the specified load balancer.
*
*
* @param describeLoadBalancerAttributesRequest
* Contains the parameters for DescribeLoadBalancerAttributes.
* @return A Java Future containing the result of the DescribeLoadBalancerAttributes operation returned by the
* service.
* @sample AmazonElasticLoadBalancingAsync.DescribeLoadBalancerAttributes
*/
java.util.concurrent.Future describeLoadBalancerAttributesAsync(
DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest);
/**
*
* Describes the attributes for the specified load balancer.
*
*
* @param describeLoadBalancerAttributesRequest
* Contains the parameters for DescribeLoadBalancerAttributes.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLoadBalancerAttributes operation returned by the
* service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeLoadBalancerAttributes
*/
java.util.concurrent.Future describeLoadBalancerAttributesAsync(
DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified Application load balancers or all of your Application load balancers.
*
*
* To describe the listeners for a load balancer, use DescribeListeners. To describe the attributes for a
* load balancer, use DescribeLoadBalancerAttributes.
*
*
* @param describeLoadBalancersRequest
* Contains the parameters for DescribeLoadBalancers.
* @return A Java Future containing the result of the DescribeLoadBalancers operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeLoadBalancers
*/
java.util.concurrent.Future describeLoadBalancersAsync(DescribeLoadBalancersRequest describeLoadBalancersRequest);
/**
*
* Describes the specified Application load balancers or all of your Application load balancers.
*
*
* To describe the listeners for a load balancer, use DescribeListeners. To describe the attributes for a
* load balancer, use DescribeLoadBalancerAttributes.
*
*
* @param describeLoadBalancersRequest
* Contains the parameters for DescribeLoadBalancers.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLoadBalancers operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeLoadBalancers
*/
java.util.concurrent.Future describeLoadBalancersAsync(DescribeLoadBalancersRequest describeLoadBalancersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
* @param describeRulesRequest
* Contains the parameters for DescribeRules.
* @return A Java Future containing the result of the DescribeRules operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeRules
*/
java.util.concurrent.Future describeRulesAsync(DescribeRulesRequest describeRulesRequest);
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
* @param describeRulesRequest
* Contains the parameters for DescribeRules.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRules operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeRules
*/
java.util.concurrent.Future describeRulesAsync(DescribeRulesRequest describeRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* Note that the only supported policy at this time is ELBSecurityPolicy-2015-05.
*
*
* @param describeSSLPoliciesRequest
* Contains the parameters for DescribeSSLPolicies.
* @return A Java Future containing the result of the DescribeSSLPolicies operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeSSLPolicies
*/
java.util.concurrent.Future describeSSLPoliciesAsync(DescribeSSLPoliciesRequest describeSSLPoliciesRequest);
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* Note that the only supported policy at this time is ELBSecurityPolicy-2015-05.
*
*
* @param describeSSLPoliciesRequest
* Contains the parameters for DescribeSSLPolicies.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSSLPolicies operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeSSLPolicies
*/
java.util.concurrent.Future describeSSLPoliciesAsync(DescribeSSLPoliciesRequest describeSSLPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the tags for the specified resources.
*
*
* @param describeTagsRequest
* Contains the parameters for DescribeTags.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest);
/**
*
* Describes the tags for the specified resources.
*
*
* @param describeTagsRequest
* Contains the parameters for DescribeTags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the attributes for the specified target group.
*
*
* @param describeTargetGroupAttributesRequest
* Contains the parameters for DescribeTargetGroupAttributes.
* @return A Java Future containing the result of the DescribeTargetGroupAttributes operation returned by the
* service.
* @sample AmazonElasticLoadBalancingAsync.DescribeTargetGroupAttributes
*/
java.util.concurrent.Future describeTargetGroupAttributesAsync(
DescribeTargetGroupAttributesRequest describeTargetGroupAttributesRequest);
/**
*
* Describes the attributes for the specified target group.
*
*
* @param describeTargetGroupAttributesRequest
* Contains the parameters for DescribeTargetGroupAttributes.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTargetGroupAttributes operation returned by the
* service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeTargetGroupAttributes
*/
java.util.concurrent.Future describeTargetGroupAttributesAsync(
DescribeTargetGroupAttributesRequest describeTargetGroupAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* To describe the targets for a target group, use DescribeTargetHealth. To describe the attributes of a
* target group, use DescribeTargetGroupAttributes.
*
*
* @param describeTargetGroupsRequest
* Contains the parameters for DescribeTargetGroups.
* @return A Java Future containing the result of the DescribeTargetGroups operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeTargetGroups
*/
java.util.concurrent.Future describeTargetGroupsAsync(DescribeTargetGroupsRequest describeTargetGroupsRequest);
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* To describe the targets for a target group, use DescribeTargetHealth. To describe the attributes of a
* target group, use DescribeTargetGroupAttributes.
*
*
* @param describeTargetGroupsRequest
* Contains the parameters for DescribeTargetGroups.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTargetGroups operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeTargetGroups
*/
java.util.concurrent.Future describeTargetGroupsAsync(DescribeTargetGroupsRequest describeTargetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
* @param describeTargetHealthRequest
* Contains the parameters for DescribeTargetHealth.
* @return A Java Future containing the result of the DescribeTargetHealth operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.DescribeTargetHealth
*/
java.util.concurrent.Future describeTargetHealthAsync(DescribeTargetHealthRequest describeTargetHealthRequest);
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
* @param describeTargetHealthRequest
* Contains the parameters for DescribeTargetHealth.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTargetHealth operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.DescribeTargetHealth
*/
java.util.concurrent.Future describeTargetHealthAsync(DescribeTargetHealthRequest describeTargetHealthRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified properties of the specified listener.
*
*
* Any properties that you do not specify retain their current values. However, changing the protocol from HTTPS to
* HTTP removes the security policy and SSL certificate properties. If you change the protocol from HTTP to HTTPS,
* you must add the security policy.
*
*
* @param modifyListenerRequest
* Contains the parameters for ModifyListener.
* @return A Java Future containing the result of the ModifyListener operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.ModifyListener
*/
java.util.concurrent.Future modifyListenerAsync(ModifyListenerRequest modifyListenerRequest);
/**
*
* Modifies the specified properties of the specified listener.
*
*
* Any properties that you do not specify retain their current values. However, changing the protocol from HTTPS to
* HTTP removes the security policy and SSL certificate properties. If you change the protocol from HTTP to HTTPS,
* you must add the security policy.
*
*
* @param modifyListenerRequest
* Contains the parameters for ModifyListener.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyListener operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.ModifyListener
*/
java.util.concurrent.Future modifyListenerAsync(ModifyListenerRequest modifyListenerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified attributes of the specified load balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
* @param modifyLoadBalancerAttributesRequest
* Contains the parameters for ModifyLoadBalancerAttributes.
* @return A Java Future containing the result of the ModifyLoadBalancerAttributes operation returned by the
* service.
* @sample AmazonElasticLoadBalancingAsync.ModifyLoadBalancerAttributes
*/
java.util.concurrent.Future modifyLoadBalancerAttributesAsync(
ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest);
/**
*
* Modifies the specified attributes of the specified load balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
* @param modifyLoadBalancerAttributesRequest
* Contains the parameters for ModifyLoadBalancerAttributes.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyLoadBalancerAttributes operation returned by the
* service.
* @sample AmazonElasticLoadBalancingAsyncHandler.ModifyLoadBalancerAttributes
*/
java.util.concurrent.Future modifyLoadBalancerAttributesAsync(
ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified rule.
*
*
* Any existing properties that you do not modify retain their current values.
*
*
* To modify the default action, use ModifyListener.
*
*
* @param modifyRuleRequest
* Contains the parameters for ModifyRules.
* @return A Java Future containing the result of the ModifyRule operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.ModifyRule
*/
java.util.concurrent.Future modifyRuleAsync(ModifyRuleRequest modifyRuleRequest);
/**
*
* Modifies the specified rule.
*
*
* Any existing properties that you do not modify retain their current values.
*
*
* To modify the default action, use ModifyListener.
*
*
* @param modifyRuleRequest
* Contains the parameters for ModifyRules.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyRule operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.ModifyRule
*/
java.util.concurrent.Future modifyRuleAsync(ModifyRuleRequest modifyRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
* To monitor the health of the targets, use DescribeTargetHealth.
*
*
* @param modifyTargetGroupRequest
* Contains the parameters for ModifyTargetGroup.
* @return A Java Future containing the result of the ModifyTargetGroup operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.ModifyTargetGroup
*/
java.util.concurrent.Future modifyTargetGroupAsync(ModifyTargetGroupRequest modifyTargetGroupRequest);
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
* To monitor the health of the targets, use DescribeTargetHealth.
*
*
* @param modifyTargetGroupRequest
* Contains the parameters for ModifyTargetGroup.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyTargetGroup operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.ModifyTargetGroup
*/
java.util.concurrent.Future modifyTargetGroupAsync(ModifyTargetGroupRequest modifyTargetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified attributes of the specified target group.
*
*
* @param modifyTargetGroupAttributesRequest
* Contains the parameters for ModifyTargetGroupAttributes.
* @return A Java Future containing the result of the ModifyTargetGroupAttributes operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.ModifyTargetGroupAttributes
*/
java.util.concurrent.Future modifyTargetGroupAttributesAsync(
ModifyTargetGroupAttributesRequest modifyTargetGroupAttributesRequest);
/**
*
* Modifies the specified attributes of the specified target group.
*
*
* @param modifyTargetGroupAttributesRequest
* Contains the parameters for ModifyTargetGroupAttributes.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyTargetGroupAttributes operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.ModifyTargetGroupAttributes
*/
java.util.concurrent.Future modifyTargetGroupAttributesAsync(
ModifyTargetGroupAttributesRequest modifyTargetGroupAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers the specified targets with the specified target group.
*
*
* The target must be in the virtual private cloud (VPC) that you specified for the target group.
*
*
* To remove a target from a target group, use DeregisterTargets.
*
*
* @param registerTargetsRequest
* Contains the parameters for RegisterTargets.
* @return A Java Future containing the result of the RegisterTargets operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.RegisterTargets
*/
java.util.concurrent.Future registerTargetsAsync(RegisterTargetsRequest registerTargetsRequest);
/**
*
* Registers the specified targets with the specified target group.
*
*
* The target must be in the virtual private cloud (VPC) that you specified for the target group.
*
*
* To remove a target from a target group, use DeregisterTargets.
*
*
* @param registerTargetsRequest
* Contains the parameters for RegisterTargets.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterTargets operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.RegisterTargets
*/
java.util.concurrent.Future registerTargetsAsync(RegisterTargetsRequest registerTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from the specified resource.
*
*
* To list the current tags for your resources, use DescribeTags.
*
*
* @param removeTagsRequest
* Contains the parameters for RemoveTags.
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.RemoveTags
*/
java.util.concurrent.Future removeTagsAsync(RemoveTagsRequest removeTagsRequest);
/**
*
* Removes the specified tags from the specified resource.
*
*
* To list the current tags for your resources, use DescribeTags.
*
*
* @param removeTagsRequest
* Contains the parameters for RemoveTags.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.RemoveTags
*/
java.util.concurrent.Future removeTagsAsync(RemoveTagsRequest removeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
* @param setRulePrioritiesRequest
* Contains the parameters for SetRulePriorities.
* @return A Java Future containing the result of the SetRulePriorities operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.SetRulePriorities
*/
java.util.concurrent.Future setRulePrioritiesAsync(SetRulePrioritiesRequest setRulePrioritiesRequest);
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
* @param setRulePrioritiesRequest
* Contains the parameters for SetRulePriorities.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetRulePriorities operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.SetRulePriorities
*/
java.util.concurrent.Future setRulePrioritiesAsync(SetRulePrioritiesRequest setRulePrioritiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified security groups with the specified load balancer. The specified security groups override
* the previously associated security groups.
*
*
* @param setSecurityGroupsRequest
* Contains the parameters for SetSecurityGroups.
* @return A Java Future containing the result of the SetSecurityGroups operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.SetSecurityGroups
*/
java.util.concurrent.Future setSecurityGroupsAsync(SetSecurityGroupsRequest setSecurityGroupsRequest);
/**
*
* Associates the specified security groups with the specified load balancer. The specified security groups override
* the previously associated security groups.
*
*
* @param setSecurityGroupsRequest
* Contains the parameters for SetSecurityGroups.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetSecurityGroups operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.SetSecurityGroups
*/
java.util.concurrent.Future setSecurityGroupsAsync(SetSecurityGroupsRequest setSecurityGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables the Availability Zone for the specified subnets for the specified load balancer. The specified subnets
* replace the previously enabled subnets.
*
*
* @param setSubnetsRequest
* Contains the parameters for SetSubnets.
* @return A Java Future containing the result of the SetSubnets operation returned by the service.
* @sample AmazonElasticLoadBalancingAsync.SetSubnets
*/
java.util.concurrent.Future setSubnetsAsync(SetSubnetsRequest setSubnetsRequest);
/**
*
* Enables the Availability Zone for the specified subnets for the specified load balancer. The specified subnets
* replace the previously enabled subnets.
*
*
* @param setSubnetsRequest
* Contains the parameters for SetSubnets.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetSubnets operation returned by the service.
* @sample AmazonElasticLoadBalancingAsyncHandler.SetSubnets
*/
java.util.concurrent.Future setSubnetsAsync(SetSubnetsRequest setSubnetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}