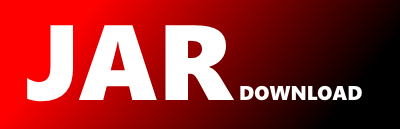
com.amazonaws.services.elasticloadbalancingv2.AmazonElasticLoadBalancing Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancingv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancingv2;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.elasticloadbalancingv2.model.*;
import com.amazonaws.services.elasticloadbalancingv2.waiters.AmazonElasticLoadBalancingWaiters;
/**
* Interface for accessing Elastic Load Balancing v2.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.elasticloadbalancingv2.AbstractAmazonElasticLoadBalancing} instead.
*
*
* Elastic Load Balancing
*
* A load balancer distributes incoming traffic across targets, such as your EC2 instances. This enables you to increase
* the availability of your application. The load balancer also monitors the health of its registered targets and
* ensures that it routes traffic only to healthy targets. You configure your load balancer to accept incoming traffic
* by specifying one or more listeners, which are configured with a protocol and port number for connections from
* clients to the load balancer. You configure a target group with a protocol and port number for connections from the
* load balancer to the targets, and with health check settings to be used when checking the health status of the
* targets.
*
*
* Elastic Load Balancing supports the following types of load balancers: Application Load Balancers, Network Load
* Balancers, Gateway Load Balancers, and Classic Load Balancers. This reference covers the following load balancer
* types:
*
*
* -
*
* Application Load Balancer - Operates at the application layer (layer 7) and supports HTTP and HTTPS.
*
*
* -
*
* Network Load Balancer - Operates at the transport layer (layer 4) and supports TCP, TLS, and UDP.
*
*
* -
*
* Gateway Load Balancer - Operates at the network layer (layer 3).
*
*
*
*
* For more information, see the Elastic
* Load Balancing User Guide.
*
*
* All Elastic Load Balancing operations are idempotent, which means that they complete at most one time. If you repeat
* an operation, it succeeds.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonElasticLoadBalancing {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "elasticloadbalancing";
/**
* Overrides the default endpoint for this client ("elasticloadbalancing.us-east-1.amazonaws.com"). Callers can use
* this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "elasticloadbalancing.us-east-1.amazonaws.com") or a full URL,
* including the protocol (ex: "elasticloadbalancing.us-east-1.amazonaws.com"). If the protocol is not specified
* here, the default protocol from this client's {@link ClientConfiguration} will be used, which by default is
* HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "elasticloadbalancing.us-east-1.amazonaws.com") or a full URL, including the protocol
* (ex: "elasticloadbalancing.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonElasticLoadBalancing#setEndpoint(String)}, sets the regional endpoint for this
* client's service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Adds the specified SSL server certificate to the certificate list for the specified HTTPS or TLS listener.
*
*
* If the certificate in already in the certificate list, the call is successful but the certificate is not added
* again.
*
*
* For more information, see HTTPS
* listeners in the Application Load Balancers Guide or TLS listeners
* in the Network Load Balancers Guide.
*
*
* @param addListenerCertificatesRequest
* @return Result of the AddListenerCertificates operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws TooManyCertificatesException
* You've reached the limit on the number of certificates per load balancer.
* @throws CertificateNotFoundException
* The specified certificate does not exist.
* @sample AmazonElasticLoadBalancing.AddListenerCertificates
* @see AWS API Documentation
*/
AddListenerCertificatesResult addListenerCertificates(AddListenerCertificatesRequest addListenerCertificatesRequest);
/**
*
* Adds the specified tags to the specified Elastic Load Balancing resource. You can tag your Application Load
* Balancers, Network Load Balancers, Gateway Load Balancers, target groups, trust stores, listeners, and rules.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
* @param addTagsRequest
* @return Result of the AddTags operation returned by the service.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @sample AmazonElasticLoadBalancing.AddTags
* @see AWS
* API Documentation
*/
AddTagsResult addTags(AddTagsRequest addTagsRequest);
/**
*
* Adds the specified revocation file to the specified trust store.
*
*
* @param addTrustStoreRevocationsRequest
* @return Result of the AddTrustStoreRevocations operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws InvalidRevocationContentException
* The provided revocation file is an invalid format, or uses an incorrect algorithm.
* @throws TooManyTrustStoreRevocationEntriesException
* The specified trust store has too many revocation entries.
* @throws RevocationContentNotFoundException
* The specified revocation file does not exist.
* @sample AmazonElasticLoadBalancing.AddTrustStoreRevocations
* @see AWS API Documentation
*/
AddTrustStoreRevocationsResult addTrustStoreRevocations(AddTrustStoreRevocationsRequest addTrustStoreRevocationsRequest);
/**
*
* Creates a listener for the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* listeners with the same settings, each call succeeds.
*
*
* @param createListenerRequest
* @return Result of the CreateListener operation returned by the service.
* @throws DuplicateListenerException
* A listener with the specified port already exists.
* @throws TooManyListenersException
* You've reached the limit on the number of listeners per load balancer.
* @throws TooManyCertificatesException
* You've reached the limit on the number of certificates per load balancer.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws SSLPolicyNotFoundException
* The specified SSL policy does not exist.
* @throws CertificateNotFoundException
* The specified certificate does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws ALPNPolicyNotSupportedException
* The specified ALPN policy is not supported.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws TrustStoreNotReadyException
* The specified trust store is not active.
* @sample AmazonElasticLoadBalancing.CreateListener
* @see AWS API Documentation
*/
CreateListenerResult createListener(CreateListenerRequest createListenerRequest);
/**
*
* Creates an Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* load balancers with the same settings, each call succeeds.
*
*
* @param createLoadBalancerRequest
* @return Result of the CreateLoadBalancer operation returned by the service.
* @throws DuplicateLoadBalancerNameException
* A load balancer with the specified name already exists.
* @throws TooManyLoadBalancersException
* You've reached the limit on the number of load balancers for your Amazon Web Services account.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SubnetNotFoundException
* The specified subnet does not exist.
* @throws InvalidSubnetException
* The specified subnet is out of available addresses.
* @throws InvalidSecurityGroupException
* The specified security group does not exist.
* @throws InvalidSchemeException
* The requested scheme is not valid.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws ResourceInUseException
* A specified resource is in use.
* @throws AllocationIdNotFoundException
* The specified allocation ID does not exist.
* @throws AvailabilityZoneNotSupportedException
* The specified Availability Zone is not supported.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AmazonElasticLoadBalancing.CreateLoadBalancer
* @see AWS API Documentation
*/
CreateLoadBalancerResult createLoadBalancer(CreateLoadBalancerRequest createLoadBalancerRequest);
/**
*
* Creates a rule for the specified listener. The listener must be associated with an Application Load Balancer.
*
*
* Each rule consists of a priority, one or more actions, and one or more conditions. Rules are evaluated in
* priority order, from the lowest value to the highest value. When the conditions for a rule are met, its actions
* are performed. If the conditions for no rules are met, the actions for the default rule are performed. For more
* information, see Listener rules in the Application Load Balancers Guide.
*
*
* @param createRuleRequest
* @return Result of the CreateRule operation returned by the service.
* @throws PriorityInUseException
* The specified priority is in use.
* @throws TooManyTargetGroupsException
* You've reached the limit on the number of target groups for your Amazon Web Services account.
* @throws TooManyRulesException
* You've reached the limit on the number of rules per load balancer.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @sample AmazonElasticLoadBalancing.CreateRule
* @see AWS API Documentation
*/
CreateRuleResult createRule(CreateRuleRequest createRuleRequest);
/**
*
* Creates a target group.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* target groups with the same settings, each call succeeds.
*
*
* @param createTargetGroupRequest
* @return Result of the CreateTargetGroup operation returned by the service.
* @throws DuplicateTargetGroupNameException
* A target group with the specified name already exists.
* @throws TooManyTargetGroupsException
* You've reached the limit on the number of target groups for your Amazon Web Services account.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @sample AmazonElasticLoadBalancing.CreateTargetGroup
* @see AWS API Documentation
*/
CreateTargetGroupResult createTargetGroup(CreateTargetGroupRequest createTargetGroupRequest);
/**
*
* Creates a trust store.
*
*
* @param createTrustStoreRequest
* @return Result of the CreateTrustStore operation returned by the service.
* @throws DuplicateTrustStoreNameException
* A trust store with the specified name already exists.
* @throws TooManyTrustStoresException
* You've reached the limit on the number of trust stores for your Amazon Web Services account.
* @throws InvalidCaCertificatesBundleException
* The specified ca certificate bundle is in an invalid format, or corrupt.
* @throws CaCertificatesBundleNotFoundException
* The specified ca certificate bundle does not exist.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @sample AmazonElasticLoadBalancing.CreateTrustStore
* @see AWS API Documentation
*/
CreateTrustStoreResult createTrustStore(CreateTrustStoreRequest createTrustStoreRequest);
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer to which it is attached.
*
*
* @param deleteListenerRequest
* @return Result of the DeleteListener operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws ResourceInUseException
* A specified resource is in use.
* @sample AmazonElasticLoadBalancing.DeleteListener
* @see AWS API Documentation
*/
DeleteListenerResult deleteListener(DeleteListenerRequest deleteListenerRequest);
/**
*
* Deletes the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer. Deleting a load
* balancer also deletes its listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
* @param deleteLoadBalancerRequest
* @return Result of the DeleteLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ResourceInUseException
* A specified resource is in use.
* @sample AmazonElasticLoadBalancing.DeleteLoadBalancer
* @see AWS API Documentation
*/
DeleteLoadBalancerResult deleteLoadBalancer(DeleteLoadBalancerRequest deleteLoadBalancerRequest);
/**
*
* Deletes the specified rule.
*
*
* You can't delete the default rule.
*
*
* @param deleteRuleRequest
* @return Result of the DeleteRule operation returned by the service.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AmazonElasticLoadBalancing.DeleteRule
* @see AWS API Documentation
*/
DeleteRuleResult deleteRule(DeleteRuleRequest deleteRuleRequest);
/**
*
* Deletes a shared trust store association.
*
*
* @param deleteSharedTrustStoreAssociationRequest
* @return Result of the DeleteSharedTrustStoreAssociation operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws DeleteAssociationSameAccountException
* The specified association cannot be within the same account.
* @throws TrustStoreAssociationNotFoundException
* The specified association does not exist.
* @sample AmazonElasticLoadBalancing.DeleteSharedTrustStoreAssociation
* @see AWS API Documentation
*/
DeleteSharedTrustStoreAssociationResult deleteSharedTrustStoreAssociation(DeleteSharedTrustStoreAssociationRequest deleteSharedTrustStoreAssociationRequest);
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks. Deleting a target group does not affect its registered targets. For example, any EC2
* instances continue to run until you stop or terminate them.
*
*
* @param deleteTargetGroupRequest
* @return Result of the DeleteTargetGroup operation returned by the service.
* @throws ResourceInUseException
* A specified resource is in use.
* @sample AmazonElasticLoadBalancing.DeleteTargetGroup
* @see AWS API Documentation
*/
DeleteTargetGroupResult deleteTargetGroup(DeleteTargetGroupRequest deleteTargetGroupRequest);
/**
*
* Deletes a trust store.
*
*
* @param deleteTrustStoreRequest
* @return Result of the DeleteTrustStore operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws TrustStoreInUseException
* The specified trust store is currently in use.
* @sample AmazonElasticLoadBalancing.DeleteTrustStore
* @see AWS API Documentation
*/
DeleteTrustStoreResult deleteTrustStore(DeleteTrustStoreRequest deleteTrustStoreRequest);
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* The load balancer stops sending requests to targets that are deregistering, but uses connection draining to
* ensure that in-flight traffic completes on the existing connections. This deregistration delay is configured by
* default but can be updated for each target group.
*
*
* For more information, see the following:
*
*
* -
*
* Deregistration delay in the Application Load Balancers User Guide
*
*
* -
*
* Deregistration delay in the Network Load Balancers User Guide
*
*
* -
*
*
* Deregistration delay in the Gateway Load Balancers User Guide
*
*
*
*
* Note: If the specified target does not exist, the action returns successfully.
*
*
* @param deregisterTargetsRequest
* @return Result of the DeregisterTargets operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidTargetException
* The specified target does not exist, is not in the same VPC as the target group, or has an unsupported
* instance type.
* @sample AmazonElasticLoadBalancing.DeregisterTargets
* @see AWS API Documentation
*/
DeregisterTargetsResult deregisterTargets(DeregisterTargetsRequest deregisterTargetsRequest);
/**
*
* Describes the current Elastic Load Balancing resource limits for your Amazon Web Services account.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param describeAccountLimitsRequest
* @return Result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonElasticLoadBalancing.DescribeAccountLimits
* @see AWS API Documentation
*/
DescribeAccountLimitsResult describeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest);
/**
*
* Describes the default certificate and the certificate list for the specified HTTPS or TLS listener.
*
*
* If the default certificate is also in the certificate list, it appears twice in the results (once with
* IsDefault
set to true and once with IsDefault
set to false).
*
*
* For more information, see SSL certificates in the Application Load Balancers Guide or Server certificates in the Network Load Balancers Guide.
*
*
* @param describeListenerCertificatesRequest
* @return Result of the DescribeListenerCertificates operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @sample AmazonElasticLoadBalancing.DescribeListenerCertificates
* @see AWS API Documentation
*/
DescribeListenerCertificatesResult describeListenerCertificates(DescribeListenerCertificatesRequest describeListenerCertificatesRequest);
/**
*
* Describes the specified listeners or the listeners for the specified Application Load Balancer, Network Load
* Balancer, or Gateway Load Balancer. You must specify either a load balancer or one or more listeners.
*
*
* @param describeListenersRequest
* @return Result of the DescribeListeners operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @sample AmazonElasticLoadBalancing.DescribeListeners
* @see AWS API Documentation
*/
DescribeListenersResult describeListeners(DescribeListenersRequest describeListenersRequest);
/**
*
* Describes the attributes for the specified Application Load Balancer, Network Load Balancer, or Gateway Load
* Balancer.
*
*
* For more information, see the following:
*
*
* -
*
* Load balancer attributes in the Application Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Network Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Gateway Load Balancers Guide
*
*
*
*
* @param describeLoadBalancerAttributesRequest
* @return Result of the DescribeLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
DescribeLoadBalancerAttributesResult describeLoadBalancerAttributes(DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest);
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
* @param describeLoadBalancersRequest
* @return Result of the DescribeLoadBalancers operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @sample AmazonElasticLoadBalancing.DescribeLoadBalancers
* @see AWS API Documentation
*/
DescribeLoadBalancersResult describeLoadBalancers(DescribeLoadBalancersRequest describeLoadBalancersRequest);
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
* @param describeRulesRequest
* @return Result of the DescribeRules operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @sample AmazonElasticLoadBalancing.DescribeRules
* @see AWS API Documentation
*/
DescribeRulesResult describeRules(DescribeRulesRequest describeRulesRequest);
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* For more information, see Security policies in the Application Load Balancers Guide or Security policies in the Network Load Balancers Guide.
*
*
* @param describeSSLPoliciesRequest
* @return Result of the DescribeSSLPolicies operation returned by the service.
* @throws SSLPolicyNotFoundException
* The specified SSL policy does not exist.
* @sample AmazonElasticLoadBalancing.DescribeSSLPolicies
* @see AWS API Documentation
*/
DescribeSSLPoliciesResult describeSSLPolicies(DescribeSSLPoliciesRequest describeSSLPoliciesRequest);
/**
*
* Describes the tags for the specified Elastic Load Balancing resources. You can describe the tags for one or more
* Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or rules.
*
*
* @param describeTagsRequest
* @return Result of the DescribeTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTags
* @see AWS API Documentation
*/
DescribeTagsResult describeTags(DescribeTagsRequest describeTagsRequest);
/**
*
* Describes the attributes for the specified target group.
*
*
* For more information, see the following:
*
*
* -
*
* Target group attributes in the Application Load Balancers Guide
*
*
* -
*
* Target group attributes in the Network Load Balancers Guide
*
*
* -
*
* Target group attributes in the Gateway Load Balancers Guide
*
*
*
*
* @param describeTargetGroupAttributesRequest
* @return Result of the DescribeTargetGroupAttributes operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTargetGroupAttributes
* @see AWS API Documentation
*/
DescribeTargetGroupAttributesResult describeTargetGroupAttributes(DescribeTargetGroupAttributesRequest describeTargetGroupAttributesRequest);
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* @param describeTargetGroupsRequest
* @return Result of the DescribeTargetGroups operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTargetGroups
* @see AWS API Documentation
*/
DescribeTargetGroupsResult describeTargetGroups(DescribeTargetGroupsRequest describeTargetGroupsRequest);
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
* @param describeTargetHealthRequest
* @return Result of the DescribeTargetHealth operation returned by the service.
* @throws InvalidTargetException
* The specified target does not exist, is not in the same VPC as the target group, or has an unsupported
* instance type.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws HealthUnavailableException
* The health of the specified targets could not be retrieved due to an internal error.
* @sample AmazonElasticLoadBalancing.DescribeTargetHealth
* @see AWS API Documentation
*/
DescribeTargetHealthResult describeTargetHealth(DescribeTargetHealthRequest describeTargetHealthRequest);
/**
*
* Describes all resources associated with the specified trust store.
*
*
* @param describeTrustStoreAssociationsRequest
* @return Result of the DescribeTrustStoreAssociations operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTrustStoreAssociations
* @see AWS API Documentation
*/
DescribeTrustStoreAssociationsResult describeTrustStoreAssociations(DescribeTrustStoreAssociationsRequest describeTrustStoreAssociationsRequest);
/**
*
* Describes the revocation files in use by the specified trust store or revocation files.
*
*
* @param describeTrustStoreRevocationsRequest
* @return Result of the DescribeTrustStoreRevocations operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws RevocationIdNotFoundException
* The specified revocation ID does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTrustStoreRevocations
* @see AWS API Documentation
*/
DescribeTrustStoreRevocationsResult describeTrustStoreRevocations(DescribeTrustStoreRevocationsRequest describeTrustStoreRevocationsRequest);
/**
*
* Describes all trust stores for the specified account.
*
*
* @param describeTrustStoresRequest
* @return Result of the DescribeTrustStores operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @sample AmazonElasticLoadBalancing.DescribeTrustStores
* @see AWS API Documentation
*/
DescribeTrustStoresResult describeTrustStores(DescribeTrustStoresRequest describeTrustStoresRequest);
/**
*
* Retrieves the resource policy for a specified resource.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonElasticLoadBalancing.GetResourcePolicy
* @see AWS API Documentation
*/
GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest);
/**
*
* Retrieves the ca certificate bundle.
*
*
* This action returns a pre-signed S3 URI which is active for ten minutes.
*
*
* @param getTrustStoreCaCertificatesBundleRequest
* @return Result of the GetTrustStoreCaCertificatesBundle operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @sample AmazonElasticLoadBalancing.GetTrustStoreCaCertificatesBundle
* @see AWS API Documentation
*/
GetTrustStoreCaCertificatesBundleResult getTrustStoreCaCertificatesBundle(GetTrustStoreCaCertificatesBundleRequest getTrustStoreCaCertificatesBundleRequest);
/**
*
* Retrieves the specified revocation file.
*
*
* This action returns a pre-signed S3 URI which is active for ten minutes.
*
*
* @param getTrustStoreRevocationContentRequest
* @return Result of the GetTrustStoreRevocationContent operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws RevocationIdNotFoundException
* The specified revocation ID does not exist.
* @sample AmazonElasticLoadBalancing.GetTrustStoreRevocationContent
* @see AWS API Documentation
*/
GetTrustStoreRevocationContentResult getTrustStoreRevocationContent(GetTrustStoreRevocationContentRequest getTrustStoreRevocationContentRequest);
/**
*
* Replaces the specified properties of the specified listener. Any properties that you do not specify remain
* unchanged.
*
*
* Changing the protocol from HTTPS to HTTP, or from TLS to TCP, removes the security policy and default certificate
* properties. If you change the protocol from HTTP to HTTPS, or from TCP to TLS, you must add the security policy
* and default certificate properties.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyListenerRequest
* @return Result of the ModifyListener operation returned by the service.
* @throws DuplicateListenerException
* A listener with the specified port already exists.
* @throws TooManyListenersException
* You've reached the limit on the number of listeners per load balancer.
* @throws TooManyCertificatesException
* You've reached the limit on the number of certificates per load balancer.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws SSLPolicyNotFoundException
* The specified SSL policy does not exist.
* @throws CertificateNotFoundException
* The specified certificate does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws ALPNPolicyNotSupportedException
* The specified ALPN policy is not supported.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws TrustStoreNotReadyException
* The specified trust store is not active.
* @sample AmazonElasticLoadBalancing.ModifyListener
* @see AWS API Documentation
*/
ModifyListenerResult modifyListener(ModifyListenerRequest modifyListenerRequest);
/**
*
* Modifies the specified attributes of the specified Application Load Balancer, Network Load Balancer, or Gateway
* Load Balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
* @param modifyLoadBalancerAttributesRequest
* @return Result of the ModifyLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @sample AmazonElasticLoadBalancing.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
ModifyLoadBalancerAttributesResult modifyLoadBalancerAttributes(ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest);
/**
*
* Replaces the specified properties of the specified rule. Any properties that you do not specify are unchanged.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyRuleRequest
* @return Result of the ModifyRule operation returned by the service.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @sample AmazonElasticLoadBalancing.ModifyRule
* @see AWS API Documentation
*/
ModifyRuleResult modifyRule(ModifyRuleRequest modifyRuleRequest);
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
* @param modifyTargetGroupRequest
* @return Result of the ModifyTargetGroup operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @sample AmazonElasticLoadBalancing.ModifyTargetGroup
* @see AWS API Documentation
*/
ModifyTargetGroupResult modifyTargetGroup(ModifyTargetGroupRequest modifyTargetGroupRequest);
/**
*
* Modifies the specified attributes of the specified target group.
*
*
* @param modifyTargetGroupAttributesRequest
* @return Result of the ModifyTargetGroupAttributes operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @sample AmazonElasticLoadBalancing.ModifyTargetGroupAttributes
* @see AWS API Documentation
*/
ModifyTargetGroupAttributesResult modifyTargetGroupAttributes(ModifyTargetGroupAttributesRequest modifyTargetGroupAttributesRequest);
/**
*
* Update the ca certificate bundle for the specified trust store.
*
*
* @param modifyTrustStoreRequest
* @return Result of the ModifyTrustStore operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws InvalidCaCertificatesBundleException
* The specified ca certificate bundle is in an invalid format, or corrupt.
* @throws CaCertificatesBundleNotFoundException
* The specified ca certificate bundle does not exist.
* @sample AmazonElasticLoadBalancing.ModifyTrustStore
* @see AWS API Documentation
*/
ModifyTrustStoreResult modifyTrustStore(ModifyTrustStoreRequest modifyTrustStoreRequest);
/**
*
* Registers the specified targets with the specified target group.
*
*
* If the target is an EC2 instance, it must be in the running
state when you register it.
*
*
* By default, the load balancer routes requests to registered targets using the protocol and port for the target
* group. Alternatively, you can override the port for a target when you register it. You can register each EC2
* instance or IP address with the same target group multiple times using different ports.
*
*
* With a Network Load Balancer, you cannot register instances by instance ID if they have the following instance
* types: C1, CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1. You can register instances of
* these types by IP address.
*
*
* @param registerTargetsRequest
* @return Result of the RegisterTargets operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws InvalidTargetException
* The specified target does not exist, is not in the same VPC as the target group, or has an unsupported
* instance type.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @sample AmazonElasticLoadBalancing.RegisterTargets
* @see AWS API Documentation
*/
RegisterTargetsResult registerTargets(RegisterTargetsRequest registerTargetsRequest);
/**
*
* Removes the specified certificate from the certificate list for the specified HTTPS or TLS listener.
*
*
* @param removeListenerCertificatesRequest
* @return Result of the RemoveListenerCertificates operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AmazonElasticLoadBalancing.RemoveListenerCertificates
* @see AWS API Documentation
*/
RemoveListenerCertificatesResult removeListenerCertificates(RemoveListenerCertificatesRequest removeListenerCertificatesRequest);
/**
*
* Removes the specified tags from the specified Elastic Load Balancing resources. You can remove the tags for one
* or more Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or
* rules.
*
*
* @param removeTagsRequest
* @return Result of the RemoveTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws TooManyTagsException
* You've reached the limit on the number of tags for this resource.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @sample AmazonElasticLoadBalancing.RemoveTags
* @see AWS API Documentation
*/
RemoveTagsResult removeTags(RemoveTagsRequest removeTagsRequest);
/**
*
* Removes the specified revocation file from the specified trust store.
*
*
* @param removeTrustStoreRevocationsRequest
* @return Result of the RemoveTrustStoreRevocations operation returned by the service.
* @throws TrustStoreNotFoundException
* The specified trust store does not exist.
* @throws RevocationIdNotFoundException
* The specified revocation ID does not exist.
* @sample AmazonElasticLoadBalancing.RemoveTrustStoreRevocations
* @see AWS API Documentation
*/
RemoveTrustStoreRevocationsResult removeTrustStoreRevocations(RemoveTrustStoreRevocationsRequest removeTrustStoreRevocationsRequest);
/**
*
* Sets the type of IP addresses used by the subnets of the specified load balancer.
*
*
* @param setIpAddressTypeRequest
* @return Result of the SetIpAddressType operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws InvalidSubnetException
* The specified subnet is out of available addresses.
* @sample AmazonElasticLoadBalancing.SetIpAddressType
* @see AWS API Documentation
*/
SetIpAddressTypeResult setIpAddressType(SetIpAddressTypeRequest setIpAddressTypeRequest);
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
* @param setRulePrioritiesRequest
* @return Result of the SetRulePriorities operation returned by the service.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws PriorityInUseException
* The specified priority is in use.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AmazonElasticLoadBalancing.SetRulePriorities
* @see AWS API Documentation
*/
SetRulePrioritiesResult setRulePriorities(SetRulePrioritiesRequest setRulePrioritiesRequest);
/**
*
* Associates the specified security groups with the specified Application Load Balancer or Network Load Balancer.
* The specified security groups override the previously associated security groups.
*
*
* You can't perform this operation on a Network Load Balancer unless you specified a security group for the load
* balancer when you created it.
*
*
* You can't associate a security group with a Gateway Load Balancer.
*
*
* @param setSecurityGroupsRequest
* @return Result of the SetSecurityGroups operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws InvalidSecurityGroupException
* The specified security group does not exist.
* @sample AmazonElasticLoadBalancing.SetSecurityGroups
* @see AWS API Documentation
*/
SetSecurityGroupsResult setSecurityGroups(SetSecurityGroupsRequest setSecurityGroupsRequest);
/**
*
* Enables the Availability Zones for the specified public subnets for the specified Application Load Balancer,
* Network Load Balancer or Gateway Load Balancer. The specified subnets replace the previously enabled subnets.
*
*
* When you specify subnets for a Network Load Balancer, or Gateway Load Balancer you must include all subnets that
* were enabled previously, with their existing configurations, plus any additional subnets.
*
*
* @param setSubnetsRequest
* @return Result of the SetSubnets operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SubnetNotFoundException
* The specified subnet does not exist.
* @throws InvalidSubnetException
* The specified subnet is out of available addresses.
* @throws AllocationIdNotFoundException
* The specified allocation ID does not exist.
* @throws AvailabilityZoneNotSupportedException
* The specified Availability Zone is not supported.
* @sample AmazonElasticLoadBalancing.SetSubnets
* @see AWS API Documentation
*/
SetSubnetsResult setSubnets(SetSubnetsRequest setSubnetsRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonElasticLoadBalancingWaiters waiters();
}