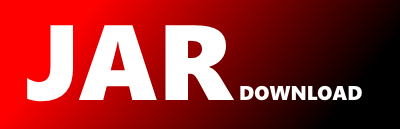
com.amazonaws.services.elasticloadbalancingv2.model.Action Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancingv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Information about an action.
*
*
* Each rule must include exactly one of the following types of actions: forward
,
* fixed-response
, or redirect
, and it must be the last action to be performed.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Action implements Serializable, Cloneable {
/**
*
* The type of action.
*
*/
private String type;
/**
*
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is forward
* and you want to route to a single target group. To route to one or more target groups, use
* ForwardConfig
instead.
*
*/
private String targetGroupArn;
/**
*
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC). Specify
* only when Type
is authenticate-oidc
.
*
*/
private AuthenticateOidcActionConfig authenticateOidcConfig;
/**
*
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when Type
* is authenticate-cognito
.
*
*/
private AuthenticateCognitoActionConfig authenticateCognitoConfig;
/**
*
* The order for the action. This value is required for rules with multiple actions. The action with the lowest
* value for order is performed first.
*
*/
private Integer order;
/**
*
* [Application Load Balancer] Information for creating a redirect action. Specify only when Type
is
* redirect
.
*
*/
private RedirectActionConfig redirectConfig;
/**
*
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response. Specify only
* when Type
is fixed-response
.
*
*/
private FixedResponseActionConfig fixedResponseConfig;
/**
*
* Information for creating an action that distributes requests among one or more target groups. For Network Load
* Balancers, you can specify a single target group. Specify only when Type
is forward
. If
* you specify both ForwardConfig
and TargetGroupArn
, you can specify only one target
* group using ForwardConfig
and it must be the same target group specified in
* TargetGroupArn
.
*
*/
private ForwardActionConfig forwardConfig;
/**
*
* The type of action.
*
*
* @param type
* The type of action.
* @see ActionTypeEnum
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of action.
*
*
* @return The type of action.
* @see ActionTypeEnum
*/
public String getType() {
return this.type;
}
/**
*
* The type of action.
*
*
* @param type
* The type of action.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActionTypeEnum
*/
public Action withType(String type) {
setType(type);
return this;
}
/**
*
* The type of action.
*
*
* @param type
* The type of action.
* @see ActionTypeEnum
*/
public void setType(ActionTypeEnum type) {
withType(type);
}
/**
*
* The type of action.
*
*
* @param type
* The type of action.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActionTypeEnum
*/
public Action withType(ActionTypeEnum type) {
this.type = type.toString();
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is forward
* and you want to route to a single target group. To route to one or more target groups, use
* ForwardConfig
instead.
*
*
* @param targetGroupArn
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is
* forward
and you want to route to a single target group. To route to one or more target
* groups, use ForwardConfig
instead.
*/
public void setTargetGroupArn(String targetGroupArn) {
this.targetGroupArn = targetGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is forward
* and you want to route to a single target group. To route to one or more target groups, use
* ForwardConfig
instead.
*
*
* @return The Amazon Resource Name (ARN) of the target group. Specify only when Type
is
* forward
and you want to route to a single target group. To route to one or more target
* groups, use ForwardConfig
instead.
*/
public String getTargetGroupArn() {
return this.targetGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is forward
* and you want to route to a single target group. To route to one or more target groups, use
* ForwardConfig
instead.
*
*
* @param targetGroupArn
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is
* forward
and you want to route to a single target group. To route to one or more target
* groups, use ForwardConfig
instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withTargetGroupArn(String targetGroupArn) {
setTargetGroupArn(targetGroupArn);
return this;
}
/**
*
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC). Specify
* only when Type
is authenticate-oidc
.
*
*
* @param authenticateOidcConfig
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC).
* Specify only when Type
is authenticate-oidc
.
*/
public void setAuthenticateOidcConfig(AuthenticateOidcActionConfig authenticateOidcConfig) {
this.authenticateOidcConfig = authenticateOidcConfig;
}
/**
*
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC). Specify
* only when Type
is authenticate-oidc
.
*
*
* @return [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC).
* Specify only when Type
is authenticate-oidc
.
*/
public AuthenticateOidcActionConfig getAuthenticateOidcConfig() {
return this.authenticateOidcConfig;
}
/**
*
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC). Specify
* only when Type
is authenticate-oidc
.
*
*
* @param authenticateOidcConfig
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC).
* Specify only when Type
is authenticate-oidc
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withAuthenticateOidcConfig(AuthenticateOidcActionConfig authenticateOidcConfig) {
setAuthenticateOidcConfig(authenticateOidcConfig);
return this;
}
/**
*
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when Type
* is authenticate-cognito
.
*
*
* @param authenticateCognitoConfig
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when
* Type
is authenticate-cognito
.
*/
public void setAuthenticateCognitoConfig(AuthenticateCognitoActionConfig authenticateCognitoConfig) {
this.authenticateCognitoConfig = authenticateCognitoConfig;
}
/**
*
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when Type
* is authenticate-cognito
.
*
*
* @return [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when
* Type
is authenticate-cognito
.
*/
public AuthenticateCognitoActionConfig getAuthenticateCognitoConfig() {
return this.authenticateCognitoConfig;
}
/**
*
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when Type
* is authenticate-cognito
.
*
*
* @param authenticateCognitoConfig
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when
* Type
is authenticate-cognito
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withAuthenticateCognitoConfig(AuthenticateCognitoActionConfig authenticateCognitoConfig) {
setAuthenticateCognitoConfig(authenticateCognitoConfig);
return this;
}
/**
*
* The order for the action. This value is required for rules with multiple actions. The action with the lowest
* value for order is performed first.
*
*
* @param order
* The order for the action. This value is required for rules with multiple actions. The action with the
* lowest value for order is performed first.
*/
public void setOrder(Integer order) {
this.order = order;
}
/**
*
* The order for the action. This value is required for rules with multiple actions. The action with the lowest
* value for order is performed first.
*
*
* @return The order for the action. This value is required for rules with multiple actions. The action with the
* lowest value for order is performed first.
*/
public Integer getOrder() {
return this.order;
}
/**
*
* The order for the action. This value is required for rules with multiple actions. The action with the lowest
* value for order is performed first.
*
*
* @param order
* The order for the action. This value is required for rules with multiple actions. The action with the
* lowest value for order is performed first.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withOrder(Integer order) {
setOrder(order);
return this;
}
/**
*
* [Application Load Balancer] Information for creating a redirect action. Specify only when Type
is
* redirect
.
*
*
* @param redirectConfig
* [Application Load Balancer] Information for creating a redirect action. Specify only when
* Type
is redirect
.
*/
public void setRedirectConfig(RedirectActionConfig redirectConfig) {
this.redirectConfig = redirectConfig;
}
/**
*
* [Application Load Balancer] Information for creating a redirect action. Specify only when Type
is
* redirect
.
*
*
* @return [Application Load Balancer] Information for creating a redirect action. Specify only when
* Type
is redirect
.
*/
public RedirectActionConfig getRedirectConfig() {
return this.redirectConfig;
}
/**
*
* [Application Load Balancer] Information for creating a redirect action. Specify only when Type
is
* redirect
.
*
*
* @param redirectConfig
* [Application Load Balancer] Information for creating a redirect action. Specify only when
* Type
is redirect
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withRedirectConfig(RedirectActionConfig redirectConfig) {
setRedirectConfig(redirectConfig);
return this;
}
/**
*
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response. Specify only
* when Type
is fixed-response
.
*
*
* @param fixedResponseConfig
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response.
* Specify only when Type
is fixed-response
.
*/
public void setFixedResponseConfig(FixedResponseActionConfig fixedResponseConfig) {
this.fixedResponseConfig = fixedResponseConfig;
}
/**
*
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response. Specify only
* when Type
is fixed-response
.
*
*
* @return [Application Load Balancer] Information for creating an action that returns a custom HTTP response.
* Specify only when Type
is fixed-response
.
*/
public FixedResponseActionConfig getFixedResponseConfig() {
return this.fixedResponseConfig;
}
/**
*
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response. Specify only
* when Type
is fixed-response
.
*
*
* @param fixedResponseConfig
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response.
* Specify only when Type
is fixed-response
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withFixedResponseConfig(FixedResponseActionConfig fixedResponseConfig) {
setFixedResponseConfig(fixedResponseConfig);
return this;
}
/**
*
* Information for creating an action that distributes requests among one or more target groups. For Network Load
* Balancers, you can specify a single target group. Specify only when Type
is forward
. If
* you specify both ForwardConfig
and TargetGroupArn
, you can specify only one target
* group using ForwardConfig
and it must be the same target group specified in
* TargetGroupArn
.
*
*
* @param forwardConfig
* Information for creating an action that distributes requests among one or more target groups. For Network
* Load Balancers, you can specify a single target group. Specify only when Type
is
* forward
. If you specify both ForwardConfig
and TargetGroupArn
, you
* can specify only one target group using ForwardConfig
and it must be the same target group
* specified in TargetGroupArn
.
*/
public void setForwardConfig(ForwardActionConfig forwardConfig) {
this.forwardConfig = forwardConfig;
}
/**
*
* Information for creating an action that distributes requests among one or more target groups. For Network Load
* Balancers, you can specify a single target group. Specify only when Type
is forward
. If
* you specify both ForwardConfig
and TargetGroupArn
, you can specify only one target
* group using ForwardConfig
and it must be the same target group specified in
* TargetGroupArn
.
*
*
* @return Information for creating an action that distributes requests among one or more target groups. For Network
* Load Balancers, you can specify a single target group. Specify only when Type
is
* forward
. If you specify both ForwardConfig
and TargetGroupArn
, you
* can specify only one target group using ForwardConfig
and it must be the same target group
* specified in TargetGroupArn
.
*/
public ForwardActionConfig getForwardConfig() {
return this.forwardConfig;
}
/**
*
* Information for creating an action that distributes requests among one or more target groups. For Network Load
* Balancers, you can specify a single target group. Specify only when Type
is forward
. If
* you specify both ForwardConfig
and TargetGroupArn
, you can specify only one target
* group using ForwardConfig
and it must be the same target group specified in
* TargetGroupArn
.
*
*
* @param forwardConfig
* Information for creating an action that distributes requests among one or more target groups. For Network
* Load Balancers, you can specify a single target group. Specify only when Type
is
* forward
. If you specify both ForwardConfig
and TargetGroupArn
, you
* can specify only one target group using ForwardConfig
and it must be the same target group
* specified in TargetGroupArn
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withForwardConfig(ForwardActionConfig forwardConfig) {
setForwardConfig(forwardConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getTargetGroupArn() != null)
sb.append("TargetGroupArn: ").append(getTargetGroupArn()).append(",");
if (getAuthenticateOidcConfig() != null)
sb.append("AuthenticateOidcConfig: ").append(getAuthenticateOidcConfig()).append(",");
if (getAuthenticateCognitoConfig() != null)
sb.append("AuthenticateCognitoConfig: ").append(getAuthenticateCognitoConfig()).append(",");
if (getOrder() != null)
sb.append("Order: ").append(getOrder()).append(",");
if (getRedirectConfig() != null)
sb.append("RedirectConfig: ").append(getRedirectConfig()).append(",");
if (getFixedResponseConfig() != null)
sb.append("FixedResponseConfig: ").append(getFixedResponseConfig()).append(",");
if (getForwardConfig() != null)
sb.append("ForwardConfig: ").append(getForwardConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Action == false)
return false;
Action other = (Action) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getTargetGroupArn() == null ^ this.getTargetGroupArn() == null)
return false;
if (other.getTargetGroupArn() != null && other.getTargetGroupArn().equals(this.getTargetGroupArn()) == false)
return false;
if (other.getAuthenticateOidcConfig() == null ^ this.getAuthenticateOidcConfig() == null)
return false;
if (other.getAuthenticateOidcConfig() != null && other.getAuthenticateOidcConfig().equals(this.getAuthenticateOidcConfig()) == false)
return false;
if (other.getAuthenticateCognitoConfig() == null ^ this.getAuthenticateCognitoConfig() == null)
return false;
if (other.getAuthenticateCognitoConfig() != null && other.getAuthenticateCognitoConfig().equals(this.getAuthenticateCognitoConfig()) == false)
return false;
if (other.getOrder() == null ^ this.getOrder() == null)
return false;
if (other.getOrder() != null && other.getOrder().equals(this.getOrder()) == false)
return false;
if (other.getRedirectConfig() == null ^ this.getRedirectConfig() == null)
return false;
if (other.getRedirectConfig() != null && other.getRedirectConfig().equals(this.getRedirectConfig()) == false)
return false;
if (other.getFixedResponseConfig() == null ^ this.getFixedResponseConfig() == null)
return false;
if (other.getFixedResponseConfig() != null && other.getFixedResponseConfig().equals(this.getFixedResponseConfig()) == false)
return false;
if (other.getForwardConfig() == null ^ this.getForwardConfig() == null)
return false;
if (other.getForwardConfig() != null && other.getForwardConfig().equals(this.getForwardConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getTargetGroupArn() == null) ? 0 : getTargetGroupArn().hashCode());
hashCode = prime * hashCode + ((getAuthenticateOidcConfig() == null) ? 0 : getAuthenticateOidcConfig().hashCode());
hashCode = prime * hashCode + ((getAuthenticateCognitoConfig() == null) ? 0 : getAuthenticateCognitoConfig().hashCode());
hashCode = prime * hashCode + ((getOrder() == null) ? 0 : getOrder().hashCode());
hashCode = prime * hashCode + ((getRedirectConfig() == null) ? 0 : getRedirectConfig().hashCode());
hashCode = prime * hashCode + ((getFixedResponseConfig() == null) ? 0 : getFixedResponseConfig().hashCode());
hashCode = prime * hashCode + ((getForwardConfig() == null) ? 0 : getForwardConfig().hashCode());
return hashCode;
}
@Override
public Action clone() {
try {
return (Action) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}