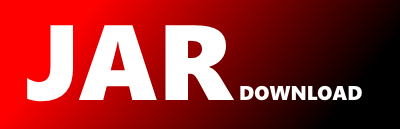
com.amazonaws.services.elasticloadbalancingv2.model.LoadBalancerAttribute Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancingv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Information about a load balancer attribute.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LoadBalancerAttribute implements Serializable, Cloneable {
/**
*
* The name of the attribute.
*
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled. The
* possible values are true
and false
. The default for Network Load Balancers and Gateway
* Load Balancers is false
. The default for Application Load Balancers is true
, and cannot
* be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is true
* or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is required if
* access logs are enabled. The bucket must exist in the same region as the load balancer and have a bucket policy
* that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is set to
* false
for internet-facing load balancers and true
for internal load balancers,
* preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is 60-604800
* seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This attribute is
* required if connection logs are enabled. The bucket must exist in the same region as the load balancer and have a
* bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that might
* pose a security risk to your application. The possible values are monitor
, defensive
,
* and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with invalid header
* fields are removed by the load balancer (true
) or routed to targets (false
). The
* default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer should
* preserve the Host
header in the HTTP request and send it to the target without any change. The
* possible values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers (
* x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information about the
* negotiated TLS version and cipher suite, are added to the client request before sending it to the target. The
* x-amzn-tls-version
header has information about the TLS protocol version negotiated with the client,
* and the x-amzn-tls-cipher-suite
header has information about the cipher suite negotiated with the
* client. Both headers are in OpenSSL format. The possible values for the attribute are true
and
* false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
header
* should preserve the source port that the client used to connect to the load balancer. The possible values are
* true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the request to
* the target. The possible values are append
, preserve
, and remove
. The
* default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the last hop)
* to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the X-Forwarded-For
* header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the X-Forwarded-For
* header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing requires that
* message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route requests to
* targets if it is unable to forward the request to Amazon Web Services WAF. The possible values are
* true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load balancer
* Availability Zones. The possible values are availability_zone_affinity
with 100 percent zonal
* affinity, partial_availability_zone_affinity
with 85 percent zonal affinity, and
* any_availability_zone
with 0 percent zonal affinity.
*
*
*
*/
private String key;
/**
*
* The value of the attribute.
*
*/
private String value;
/**
*
* The name of the attribute.
*
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled. The
* possible values are true
and false
. The default for Network Load Balancers and Gateway
* Load Balancers is false
. The default for Application Load Balancers is true
, and cannot
* be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is true
* or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is required if
* access logs are enabled. The bucket must exist in the same region as the load balancer and have a bucket policy
* that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is set to
* false
for internet-facing load balancers and true
for internal load balancers,
* preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is 60-604800
* seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This attribute is
* required if connection logs are enabled. The bucket must exist in the same region as the load balancer and have a
* bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that might
* pose a security risk to your application. The possible values are monitor
, defensive
,
* and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with invalid header
* fields are removed by the load balancer (true
) or routed to targets (false
). The
* default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer should
* preserve the Host
header in the HTTP request and send it to the target without any change. The
* possible values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers (
* x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information about the
* negotiated TLS version and cipher suite, are added to the client request before sending it to the target. The
* x-amzn-tls-version
header has information about the TLS protocol version negotiated with the client,
* and the x-amzn-tls-cipher-suite
header has information about the cipher suite negotiated with the
* client. Both headers are in OpenSSL format. The possible values for the attribute are true
and
* false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
header
* should preserve the source port that the client used to connect to the load balancer. The possible values are
* true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the request to
* the target. The possible values are append
, preserve
, and remove
. The
* default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the last hop)
* to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the X-Forwarded-For
* header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the X-Forwarded-For
* header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing requires that
* message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route requests to
* targets if it is unable to forward the request to Amazon Web Services WAF. The possible values are
* true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load balancer
* Availability Zones. The possible values are availability_zone_affinity
with 100 percent zonal
* affinity, partial_availability_zone_affinity
with 85 percent zonal affinity, and
* any_availability_zone
with 0 percent zonal affinity.
*
*
*
*
* @param key
* The name of the attribute.
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled.
* The possible values are true
and false
. The default for Network Load Balancers
* and Gateway Load Balancers is false
. The default for Application Load Balancers is
* true
, and cannot be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is
* required if access logs are enabled. The bucket must exist in the same region as the load balancer and
* have a bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is
* set to false
for internet-facing load balancers and true
for internal load
* balancers, preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is
* 60-604800 seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This attribute
* is required if connection logs are enabled. The bucket must exist in the same region as the load balancer
* and have a bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection
* logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that
* might pose a security risk to your application. The possible values are monitor
,
* defensive
, and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with invalid
* header fields are removed by the load balancer (true
) or routed to targets (
* false
). The default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer
* should preserve the Host
header in the HTTP request and send it to the target without any
* change. The possible values are true
and false
. The default is
* false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers
* (x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information
* about the negotiated TLS version and cipher suite, are added to the client request before sending it to
* the target. The x-amzn-tls-version
header has information about the TLS protocol version
* negotiated with the client, and the x-amzn-tls-cipher-suite
header has information about the
* cipher suite negotiated with the client. Both headers are in OpenSSL format. The possible values for the
* attribute are true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
* header should preserve the source port that the client used to connect to the load balancer. The possible
* values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the
* request to the target. The possible values are append
, preserve
, and
* remove
. The default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the last
* hop) to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the
* X-Forwarded-For
header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the
* X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing
* requires that message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route
* requests to targets if it is unable to forward the request to Amazon Web Services WAF. The possible values
* are true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load
* balancer Availability Zones. The possible values are availability_zone_affinity
with 100
* percent zonal affinity, partial_availability_zone_affinity
with 85 percent zonal affinity,
* and any_availability_zone
with 0 percent zonal affinity.
*
*
*/
public void setKey(String key) {
this.key = key;
}
/**
*
* The name of the attribute.
*
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled. The
* possible values are true
and false
. The default for Network Load Balancers and Gateway
* Load Balancers is false
. The default for Application Load Balancers is true
, and cannot
* be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is true
* or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is required if
* access logs are enabled. The bucket must exist in the same region as the load balancer and have a bucket policy
* that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is set to
* false
for internet-facing load balancers and true
for internal load balancers,
* preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is 60-604800
* seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This attribute is
* required if connection logs are enabled. The bucket must exist in the same region as the load balancer and have a
* bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that might
* pose a security risk to your application. The possible values are monitor
, defensive
,
* and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with invalid header
* fields are removed by the load balancer (true
) or routed to targets (false
). The
* default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer should
* preserve the Host
header in the HTTP request and send it to the target without any change. The
* possible values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers (
* x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information about the
* negotiated TLS version and cipher suite, are added to the client request before sending it to the target. The
* x-amzn-tls-version
header has information about the TLS protocol version negotiated with the client,
* and the x-amzn-tls-cipher-suite
header has information about the cipher suite negotiated with the
* client. Both headers are in OpenSSL format. The possible values for the attribute are true
and
* false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
header
* should preserve the source port that the client used to connect to the load balancer. The possible values are
* true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the request to
* the target. The possible values are append
, preserve
, and remove
. The
* default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the last hop)
* to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the X-Forwarded-For
* header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the X-Forwarded-For
* header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing requires that
* message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route requests to
* targets if it is unable to forward the request to Amazon Web Services WAF. The possible values are
* true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load balancer
* Availability Zones. The possible values are availability_zone_affinity
with 100 percent zonal
* affinity, partial_availability_zone_affinity
with 85 percent zonal affinity, and
* any_availability_zone
with 0 percent zonal affinity.
*
*
*
*
* @return The name of the attribute.
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled.
* The possible values are true
and false
. The default for Network Load Balancers
* and Gateway Load Balancers is false
. The default for Application Load Balancers is
* true
, and cannot be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is
* required if access logs are enabled. The bucket must exist in the same region as the load balancer and
* have a bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is
* set to false
for internet-facing load balancers and true
for internal load
* balancers, preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is
* 60-604800 seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This
* attribute is required if connection logs are enabled. The bucket must exist in the same region as the
* load balancer and have a bucket policy that grants Elastic Load Balancing permissions to write to the
* bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection
* logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that
* might pose a security risk to your application. The possible values are monitor
,
* defensive
, and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with
* invalid header fields are removed by the load balancer (true
) or routed to targets (
* false
). The default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer
* should preserve the Host
header in the HTTP request and send it to the target without any
* change. The possible values are true
and false
. The default is
* false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers
* (x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information
* about the negotiated TLS version and cipher suite, are added to the client request before sending it to
* the target. The x-amzn-tls-version
header has information about the TLS protocol version
* negotiated with the client, and the x-amzn-tls-cipher-suite
header has information about the
* cipher suite negotiated with the client. Both headers are in OpenSSL format. The possible values for the
* attribute are true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
* header should preserve the source port that the client used to connect to the load balancer. The possible
* values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the
* request to the target. The possible values are append
, preserve
, and
* remove
. The default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the
* last hop) to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the
* X-Forwarded-For
header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the
* X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing
* requires that message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route
* requests to targets if it is unable to forward the request to Amazon Web Services WAF. The possible
* values are true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load
* balancer Availability Zones. The possible values are availability_zone_affinity
with 100
* percent zonal affinity, partial_availability_zone_affinity
with 85 percent zonal affinity,
* and any_availability_zone
with 0 percent zonal affinity.
*
*
*/
public String getKey() {
return this.key;
}
/**
*
* The name of the attribute.
*
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled. The
* possible values are true
and false
. The default for Network Load Balancers and Gateway
* Load Balancers is false
. The default for Application Load Balancers is true
, and cannot
* be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is true
* or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is required if
* access logs are enabled. The bucket must exist in the same region as the load balancer and have a bucket policy
* that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is set to
* false
for internet-facing load balancers and true
for internal load balancers,
* preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is 60-604800
* seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This attribute is
* required if connection logs are enabled. The bucket must exist in the same region as the load balancer and have a
* bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that might
* pose a security risk to your application. The possible values are monitor
, defensive
,
* and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with invalid header
* fields are removed by the load balancer (true
) or routed to targets (false
). The
* default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer should
* preserve the Host
header in the HTTP request and send it to the target without any change. The
* possible values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers (
* x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information about the
* negotiated TLS version and cipher suite, are added to the client request before sending it to the target. The
* x-amzn-tls-version
header has information about the TLS protocol version negotiated with the client,
* and the x-amzn-tls-cipher-suite
header has information about the cipher suite negotiated with the
* client. Both headers are in OpenSSL format. The possible values for the attribute are true
and
* false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
header
* should preserve the source port that the client used to connect to the load balancer. The possible values are
* true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the request to
* the target. The possible values are append
, preserve
, and remove
. The
* default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the last hop)
* to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the X-Forwarded-For
* header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the X-Forwarded-For
* header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing requires that
* message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route requests to
* targets if it is unable to forward the request to Amazon Web Services WAF. The possible values are
* true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load balancer
* Availability Zones. The possible values are availability_zone_affinity
with 100 percent zonal
* affinity, partial_availability_zone_affinity
with 85 percent zonal affinity, and
* any_availability_zone
with 0 percent zonal affinity.
*
*
*
*
* @param key
* The name of the attribute.
*
* The following attributes are supported by all load balancers:
*
*
* -
*
* deletion_protection.enabled
- Indicates whether deletion protection is enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* load_balancing.cross_zone.enabled
- Indicates whether cross-zone load balancing is enabled.
* The possible values are true
and false
. The default for Network Load Balancers
* and Gateway Load Balancers is false
. The default for Application Load Balancers is
* true
, and cannot be changed.
*
*
*
*
* The following attributes are supported by both Application Load Balancers and Network Load Balancers:
*
*
* -
*
* access_logs.s3.enabled
- Indicates whether access logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* access_logs.s3.bucket
- The name of the S3 bucket for the access logs. This attribute is
* required if access logs are enabled. The bucket must exist in the same region as the load balancer and
* have a bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* access_logs.s3.prefix
- The prefix for the location in the S3 bucket for the access logs.
*
*
* -
*
* ipv6.deny_all_igw_traffic
- Blocks internet gateway (IGW) access to the load balancer. It is
* set to false
for internet-facing load balancers and true
for internal load
* balancers, preventing unintended access to your internal load balancer through an internet gateway.
*
*
*
*
* The following attributes are supported by only Application Load Balancers:
*
*
* -
*
* idle_timeout.timeout_seconds
- The idle timeout value, in seconds. The valid range is 1-4000
* seconds. The default is 60 seconds.
*
*
* -
*
* client_keep_alive.seconds
- The client keep alive value, in seconds. The valid range is
* 60-604800 seconds. The default is 3600 seconds.
*
*
* -
*
* connection_logs.s3.enabled
- Indicates whether connection logs are enabled. The value is
* true
or false
. The default is false
.
*
*
* -
*
* connection_logs.s3.bucket
- The name of the S3 bucket for the connection logs. This attribute
* is required if connection logs are enabled. The bucket must exist in the same region as the load balancer
* and have a bucket policy that grants Elastic Load Balancing permissions to write to the bucket.
*
*
* -
*
* connection_logs.s3.prefix
- The prefix for the location in the S3 bucket for the connection
* logs.
*
*
* -
*
* routing.http.desync_mitigation_mode
- Determines how the load balancer handles requests that
* might pose a security risk to your application. The possible values are monitor
,
* defensive
, and strictest
. The default is defensive
.
*
*
* -
*
* routing.http.drop_invalid_header_fields.enabled
- Indicates whether HTTP headers with invalid
* header fields are removed by the load balancer (true
) or routed to targets (
* false
). The default is false
.
*
*
* -
*
* routing.http.preserve_host_header.enabled
- Indicates whether the Application Load Balancer
* should preserve the Host
header in the HTTP request and send it to the target without any
* change. The possible values are true
and false
. The default is
* false
.
*
*
* -
*
* routing.http.x_amzn_tls_version_and_cipher_suite.enabled
- Indicates whether the two headers
* (x-amzn-tls-version
and x-amzn-tls-cipher-suite
), which contain information
* about the negotiated TLS version and cipher suite, are added to the client request before sending it to
* the target. The x-amzn-tls-version
header has information about the TLS protocol version
* negotiated with the client, and the x-amzn-tls-cipher-suite
header has information about the
* cipher suite negotiated with the client. Both headers are in OpenSSL format. The possible values for the
* attribute are true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_client_port.enabled
- Indicates whether the X-Forwarded-For
* header should preserve the source port that the client used to connect to the load balancer. The possible
* values are true
and false
. The default is false
.
*
*
* -
*
* routing.http.xff_header_processing.mode
- Enables you to modify, preserve, or remove the
* X-Forwarded-For
header in the HTTP request before the Application Load Balancer sends the
* request to the target. The possible values are append
, preserve
, and
* remove
. The default is append
.
*
*
* -
*
* If the value is append
, the Application Load Balancer adds the client IP address (of the last
* hop) to the X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
* -
*
* If the value is preserve
the Application Load Balancer preserves the
* X-Forwarded-For
header in the HTTP request, and sends it to targets without any change.
*
*
* -
*
* If the value is remove
, the Application Load Balancer removes the
* X-Forwarded-For
header in the HTTP request before it sends it to targets.
*
*
*
*
* -
*
* routing.http2.enabled
- Indicates whether HTTP/2 is enabled. The possible values are
* true
and false
. The default is true
. Elastic Load Balancing
* requires that message header names contain only alphanumeric characters and hyphens.
*
*
* -
*
* waf.fail_open.enabled
- Indicates whether to allow a WAF-enabled load balancer to route
* requests to targets if it is unable to forward the request to Amazon Web Services WAF. The possible values
* are true
and false
. The default is false
.
*
*
*
*
* The following attributes are supported by only Network Load Balancers:
*
*
* -
*
* dns_record.client_routing_policy
- Indicates how traffic is distributed among the load
* balancer Availability Zones. The possible values are availability_zone_affinity
with 100
* percent zonal affinity, partial_availability_zone_affinity
with 85 percent zonal affinity,
* and any_availability_zone
with 0 percent zonal affinity.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoadBalancerAttribute withKey(String key) {
setKey(key);
return this;
}
/**
*
* The value of the attribute.
*
*
* @param value
* The value of the attribute.
*/
public void setValue(String value) {
this.value = value;
}
/**
*
* The value of the attribute.
*
*
* @return The value of the attribute.
*/
public String getValue() {
return this.value;
}
/**
*
* The value of the attribute.
*
*
* @param value
* The value of the attribute.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoadBalancerAttribute withValue(String value) {
setValue(value);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getKey() != null)
sb.append("Key: ").append(getKey()).append(",");
if (getValue() != null)
sb.append("Value: ").append(getValue());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LoadBalancerAttribute == false)
return false;
LoadBalancerAttribute other = (LoadBalancerAttribute) obj;
if (other.getKey() == null ^ this.getKey() == null)
return false;
if (other.getKey() != null && other.getKey().equals(this.getKey()) == false)
return false;
if (other.getValue() == null ^ this.getValue() == null)
return false;
if (other.getValue() != null && other.getValue().equals(this.getValue()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getKey() == null) ? 0 : getKey().hashCode());
hashCode = prime * hashCode + ((getValue() == null) ? 0 : getValue().hashCode());
return hashCode;
}
@Override
public LoadBalancerAttribute clone() {
try {
return (LoadBalancerAttribute) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}