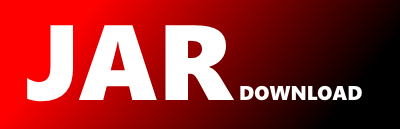
com.amazonaws.services.elasticloadbalancingv2.model.RuleCondition Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancingv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Information about a condition for a rule.
*
*
* Each rule can optionally include up to one of each of the following conditions: http-request-method
,
* host-header
, path-pattern
, and source-ip
. Each rule can also optionally
* include one or more of each of the following conditions: http-header
and query-string
. Note
* that the value for a condition cannot be empty.
*
*
* For more information, see Quotas for your
* Application Load Balancers.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RuleCondition implements Serializable, Cloneable {
/**
*
* The field in the HTTP request. The following are the possible values:
*
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*
*/
private String field;
/**
*
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you can
* specify a single host name (for example, my.example.com) in Values
. A host name is case insensitive,
* can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
, you can
* specify a single path pattern (for example, /img/*) in Values
. A path pattern is case-sensitive, can
* be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*/
private java.util.List values;
/**
*
* Information for a host header condition. Specify only when Field
is host-header
.
*
*/
private HostHeaderConditionConfig hostHeaderConfig;
/**
*
* Information for a path pattern condition. Specify only when Field
is path-pattern
.
*
*/
private PathPatternConditionConfig pathPatternConfig;
/**
*
* Information for an HTTP header condition. Specify only when Field
is http-header
.
*
*/
private HttpHeaderConditionConfig httpHeaderConfig;
/**
*
* Information for a query string condition. Specify only when Field
is query-string
.
*
*/
private QueryStringConditionConfig queryStringConfig;
/**
*
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*
*/
private HttpRequestMethodConditionConfig httpRequestMethodConfig;
/**
*
* Information for a source IP condition. Specify only when Field
is source-ip
.
*
*/
private SourceIpConditionConfig sourceIpConfig;
/**
*
* The field in the HTTP request. The following are the possible values:
*
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*
*
* @param field
* The field in the HTTP request. The following are the possible values:
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*/
public void setField(String field) {
this.field = field;
}
/**
*
* The field in the HTTP request. The following are the possible values:
*
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*
*
* @return The field in the HTTP request. The following are the possible values:
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*/
public String getField() {
return this.field;
}
/**
*
* The field in the HTTP request. The following are the possible values:
*
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*
*
* @param field
* The field in the HTTP request. The following are the possible values:
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withField(String field) {
setField(field);
return this;
}
/**
*
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you can
* specify a single host name (for example, my.example.com) in Values
. A host name is case insensitive,
* can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
, you can
* specify a single path pattern (for example, /img/*) in Values
. A path pattern is case-sensitive, can
* be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* @return The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
* If Field
is host-header
and you are not using HostHeaderConfig
,
* you can specify a single host name (for example, my.example.com) in Values
. A host name is
* case insensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
,
* you can specify a single path pattern (for example, /img/*) in Values
. A path pattern is
* case-sensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*/
public java.util.List getValues() {
return values;
}
/**
*
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you can
* specify a single host name (for example, my.example.com) in Values
. A host name is case insensitive,
* can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
, you can
* specify a single path pattern (for example, /img/*) in Values
. A path pattern is case-sensitive, can
* be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* @param values
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you
* can specify a single host name (for example, my.example.com) in Values
. A host name is case
* insensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
,
* you can specify a single path pattern (for example, /img/*) in Values
. A path pattern is
* case-sensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*/
public void setValues(java.util.Collection values) {
if (values == null) {
this.values = null;
return;
}
this.values = new java.util.ArrayList(values);
}
/**
*
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you can
* specify a single host name (for example, my.example.com) in Values
. A host name is case insensitive,
* can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
, you can
* specify a single path pattern (for example, /img/*) in Values
. A path pattern is case-sensitive, can
* be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValues(java.util.Collection)} or {@link #withValues(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param values
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you
* can specify a single host name (for example, my.example.com) in Values
. A host name is case
* insensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
,
* you can specify a single path pattern (for example, /img/*) in Values
. A path pattern is
* case-sensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withValues(String... values) {
if (this.values == null) {
setValues(new java.util.ArrayList(values.length));
}
for (String ele : values) {
this.values.add(ele);
}
return this;
}
/**
*
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you can
* specify a single host name (for example, my.example.com) in Values
. A host name is case insensitive,
* can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
, you can
* specify a single path pattern (for example, /img/*) in Values
. A path pattern is case-sensitive, can
* be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* @param values
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you
* can specify a single host name (for example, my.example.com) in Values
. A host name is case
* insensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
,
* you can specify a single path pattern (for example, /img/*) in Values
. A path pattern is
* case-sensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withValues(java.util.Collection values) {
setValues(values);
return this;
}
/**
*
* Information for a host header condition. Specify only when Field
is host-header
.
*
*
* @param hostHeaderConfig
* Information for a host header condition. Specify only when Field
is host-header
.
*/
public void setHostHeaderConfig(HostHeaderConditionConfig hostHeaderConfig) {
this.hostHeaderConfig = hostHeaderConfig;
}
/**
*
* Information for a host header condition. Specify only when Field
is host-header
.
*
*
* @return Information for a host header condition. Specify only when Field
is host-header
* .
*/
public HostHeaderConditionConfig getHostHeaderConfig() {
return this.hostHeaderConfig;
}
/**
*
* Information for a host header condition. Specify only when Field
is host-header
.
*
*
* @param hostHeaderConfig
* Information for a host header condition. Specify only when Field
is host-header
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withHostHeaderConfig(HostHeaderConditionConfig hostHeaderConfig) {
setHostHeaderConfig(hostHeaderConfig);
return this;
}
/**
*
* Information for a path pattern condition. Specify only when Field
is path-pattern
.
*
*
* @param pathPatternConfig
* Information for a path pattern condition. Specify only when Field
is
* path-pattern
.
*/
public void setPathPatternConfig(PathPatternConditionConfig pathPatternConfig) {
this.pathPatternConfig = pathPatternConfig;
}
/**
*
* Information for a path pattern condition. Specify only when Field
is path-pattern
.
*
*
* @return Information for a path pattern condition. Specify only when Field
is
* path-pattern
.
*/
public PathPatternConditionConfig getPathPatternConfig() {
return this.pathPatternConfig;
}
/**
*
* Information for a path pattern condition. Specify only when Field
is path-pattern
.
*
*
* @param pathPatternConfig
* Information for a path pattern condition. Specify only when Field
is
* path-pattern
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withPathPatternConfig(PathPatternConditionConfig pathPatternConfig) {
setPathPatternConfig(pathPatternConfig);
return this;
}
/**
*
* Information for an HTTP header condition. Specify only when Field
is http-header
.
*
*
* @param httpHeaderConfig
* Information for an HTTP header condition. Specify only when Field
is http-header
* .
*/
public void setHttpHeaderConfig(HttpHeaderConditionConfig httpHeaderConfig) {
this.httpHeaderConfig = httpHeaderConfig;
}
/**
*
* Information for an HTTP header condition. Specify only when Field
is http-header
.
*
*
* @return Information for an HTTP header condition. Specify only when Field
is
* http-header
.
*/
public HttpHeaderConditionConfig getHttpHeaderConfig() {
return this.httpHeaderConfig;
}
/**
*
* Information for an HTTP header condition. Specify only when Field
is http-header
.
*
*
* @param httpHeaderConfig
* Information for an HTTP header condition. Specify only when Field
is http-header
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withHttpHeaderConfig(HttpHeaderConditionConfig httpHeaderConfig) {
setHttpHeaderConfig(httpHeaderConfig);
return this;
}
/**
*
* Information for a query string condition. Specify only when Field
is query-string
.
*
*
* @param queryStringConfig
* Information for a query string condition. Specify only when Field
is
* query-string
.
*/
public void setQueryStringConfig(QueryStringConditionConfig queryStringConfig) {
this.queryStringConfig = queryStringConfig;
}
/**
*
* Information for a query string condition. Specify only when Field
is query-string
.
*
*
* @return Information for a query string condition. Specify only when Field
is
* query-string
.
*/
public QueryStringConditionConfig getQueryStringConfig() {
return this.queryStringConfig;
}
/**
*
* Information for a query string condition. Specify only when Field
is query-string
.
*
*
* @param queryStringConfig
* Information for a query string condition. Specify only when Field
is
* query-string
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withQueryStringConfig(QueryStringConditionConfig queryStringConfig) {
setQueryStringConfig(queryStringConfig);
return this;
}
/**
*
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*
*
* @param httpRequestMethodConfig
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*/
public void setHttpRequestMethodConfig(HttpRequestMethodConditionConfig httpRequestMethodConfig) {
this.httpRequestMethodConfig = httpRequestMethodConfig;
}
/**
*
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*
*
* @return Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*/
public HttpRequestMethodConditionConfig getHttpRequestMethodConfig() {
return this.httpRequestMethodConfig;
}
/**
*
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*
*
* @param httpRequestMethodConfig
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withHttpRequestMethodConfig(HttpRequestMethodConditionConfig httpRequestMethodConfig) {
setHttpRequestMethodConfig(httpRequestMethodConfig);
return this;
}
/**
*
* Information for a source IP condition. Specify only when Field
is source-ip
.
*
*
* @param sourceIpConfig
* Information for a source IP condition. Specify only when Field
is source-ip
.
*/
public void setSourceIpConfig(SourceIpConditionConfig sourceIpConfig) {
this.sourceIpConfig = sourceIpConfig;
}
/**
*
* Information for a source IP condition. Specify only when Field
is source-ip
.
*
*
* @return Information for a source IP condition. Specify only when Field
is source-ip
.
*/
public SourceIpConditionConfig getSourceIpConfig() {
return this.sourceIpConfig;
}
/**
*
* Information for a source IP condition. Specify only when Field
is source-ip
.
*
*
* @param sourceIpConfig
* Information for a source IP condition. Specify only when Field
is source-ip
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RuleCondition withSourceIpConfig(SourceIpConditionConfig sourceIpConfig) {
setSourceIpConfig(sourceIpConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getField() != null)
sb.append("Field: ").append(getField()).append(",");
if (getValues() != null)
sb.append("Values: ").append(getValues()).append(",");
if (getHostHeaderConfig() != null)
sb.append("HostHeaderConfig: ").append(getHostHeaderConfig()).append(",");
if (getPathPatternConfig() != null)
sb.append("PathPatternConfig: ").append(getPathPatternConfig()).append(",");
if (getHttpHeaderConfig() != null)
sb.append("HttpHeaderConfig: ").append(getHttpHeaderConfig()).append(",");
if (getQueryStringConfig() != null)
sb.append("QueryStringConfig: ").append(getQueryStringConfig()).append(",");
if (getHttpRequestMethodConfig() != null)
sb.append("HttpRequestMethodConfig: ").append(getHttpRequestMethodConfig()).append(",");
if (getSourceIpConfig() != null)
sb.append("SourceIpConfig: ").append(getSourceIpConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RuleCondition == false)
return false;
RuleCondition other = (RuleCondition) obj;
if (other.getField() == null ^ this.getField() == null)
return false;
if (other.getField() != null && other.getField().equals(this.getField()) == false)
return false;
if (other.getValues() == null ^ this.getValues() == null)
return false;
if (other.getValues() != null && other.getValues().equals(this.getValues()) == false)
return false;
if (other.getHostHeaderConfig() == null ^ this.getHostHeaderConfig() == null)
return false;
if (other.getHostHeaderConfig() != null && other.getHostHeaderConfig().equals(this.getHostHeaderConfig()) == false)
return false;
if (other.getPathPatternConfig() == null ^ this.getPathPatternConfig() == null)
return false;
if (other.getPathPatternConfig() != null && other.getPathPatternConfig().equals(this.getPathPatternConfig()) == false)
return false;
if (other.getHttpHeaderConfig() == null ^ this.getHttpHeaderConfig() == null)
return false;
if (other.getHttpHeaderConfig() != null && other.getHttpHeaderConfig().equals(this.getHttpHeaderConfig()) == false)
return false;
if (other.getQueryStringConfig() == null ^ this.getQueryStringConfig() == null)
return false;
if (other.getQueryStringConfig() != null && other.getQueryStringConfig().equals(this.getQueryStringConfig()) == false)
return false;
if (other.getHttpRequestMethodConfig() == null ^ this.getHttpRequestMethodConfig() == null)
return false;
if (other.getHttpRequestMethodConfig() != null && other.getHttpRequestMethodConfig().equals(this.getHttpRequestMethodConfig()) == false)
return false;
if (other.getSourceIpConfig() == null ^ this.getSourceIpConfig() == null)
return false;
if (other.getSourceIpConfig() != null && other.getSourceIpConfig().equals(this.getSourceIpConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getField() == null) ? 0 : getField().hashCode());
hashCode = prime * hashCode + ((getValues() == null) ? 0 : getValues().hashCode());
hashCode = prime * hashCode + ((getHostHeaderConfig() == null) ? 0 : getHostHeaderConfig().hashCode());
hashCode = prime * hashCode + ((getPathPatternConfig() == null) ? 0 : getPathPatternConfig().hashCode());
hashCode = prime * hashCode + ((getHttpHeaderConfig() == null) ? 0 : getHttpHeaderConfig().hashCode());
hashCode = prime * hashCode + ((getQueryStringConfig() == null) ? 0 : getQueryStringConfig().hashCode());
hashCode = prime * hashCode + ((getHttpRequestMethodConfig() == null) ? 0 : getHttpRequestMethodConfig().hashCode());
hashCode = prime * hashCode + ((getSourceIpConfig() == null) ? 0 : getSourceIpConfig().hashCode());
return hashCode;
}
@Override
public RuleCondition clone() {
try {
return (RuleCondition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}