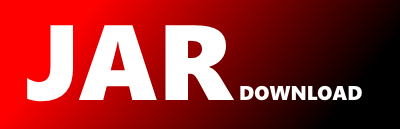
com.amazonaws.services.elasticloadbalancingv2.model.TargetHealthDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticloadbalancingv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Information about the health of a target.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TargetHealthDescription implements Serializable, Cloneable {
/**
*
* The description of the target.
*
*/
private TargetDescription target;
/**
*
* The port to use to connect with the target.
*
*/
private String healthCheckPort;
/**
*
* The health information for the target.
*
*/
private TargetHealth targetHealth;
/**
*
* The anomaly detection result for the target.
*
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
*
*/
private AnomalyDetection anomalyDetection;
/**
*
* The description of the target.
*
*
* @param target
* The description of the target.
*/
public void setTarget(TargetDescription target) {
this.target = target;
}
/**
*
* The description of the target.
*
*
* @return The description of the target.
*/
public TargetDescription getTarget() {
return this.target;
}
/**
*
* The description of the target.
*
*
* @param target
* The description of the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TargetHealthDescription withTarget(TargetDescription target) {
setTarget(target);
return this;
}
/**
*
* The port to use to connect with the target.
*
*
* @param healthCheckPort
* The port to use to connect with the target.
*/
public void setHealthCheckPort(String healthCheckPort) {
this.healthCheckPort = healthCheckPort;
}
/**
*
* The port to use to connect with the target.
*
*
* @return The port to use to connect with the target.
*/
public String getHealthCheckPort() {
return this.healthCheckPort;
}
/**
*
* The port to use to connect with the target.
*
*
* @param healthCheckPort
* The port to use to connect with the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TargetHealthDescription withHealthCheckPort(String healthCheckPort) {
setHealthCheckPort(healthCheckPort);
return this;
}
/**
*
* The health information for the target.
*
*
* @param targetHealth
* The health information for the target.
*/
public void setTargetHealth(TargetHealth targetHealth) {
this.targetHealth = targetHealth;
}
/**
*
* The health information for the target.
*
*
* @return The health information for the target.
*/
public TargetHealth getTargetHealth() {
return this.targetHealth;
}
/**
*
* The health information for the target.
*
*
* @param targetHealth
* The health information for the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TargetHealthDescription withTargetHealth(TargetHealth targetHealth) {
setTargetHealth(targetHealth);
return this;
}
/**
*
* The anomaly detection result for the target.
*
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
*
*
* @param anomalyDetection
* The anomaly detection result for the target.
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
*/
public void setAnomalyDetection(AnomalyDetection anomalyDetection) {
this.anomalyDetection = anomalyDetection;
}
/**
*
* The anomaly detection result for the target.
*
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
*
*
* @return The anomaly detection result for the target.
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
*/
public AnomalyDetection getAnomalyDetection() {
return this.anomalyDetection;
}
/**
*
* The anomaly detection result for the target.
*
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
*
*
* @param anomalyDetection
* The anomaly detection result for the target.
*
* If no anomalies were detected, the result is normal
.
*
*
* If anomalies were detected, the result is anomalous
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TargetHealthDescription withAnomalyDetection(AnomalyDetection anomalyDetection) {
setAnomalyDetection(anomalyDetection);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTarget() != null)
sb.append("Target: ").append(getTarget()).append(",");
if (getHealthCheckPort() != null)
sb.append("HealthCheckPort: ").append(getHealthCheckPort()).append(",");
if (getTargetHealth() != null)
sb.append("TargetHealth: ").append(getTargetHealth()).append(",");
if (getAnomalyDetection() != null)
sb.append("AnomalyDetection: ").append(getAnomalyDetection());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TargetHealthDescription == false)
return false;
TargetHealthDescription other = (TargetHealthDescription) obj;
if (other.getTarget() == null ^ this.getTarget() == null)
return false;
if (other.getTarget() != null && other.getTarget().equals(this.getTarget()) == false)
return false;
if (other.getHealthCheckPort() == null ^ this.getHealthCheckPort() == null)
return false;
if (other.getHealthCheckPort() != null && other.getHealthCheckPort().equals(this.getHealthCheckPort()) == false)
return false;
if (other.getTargetHealth() == null ^ this.getTargetHealth() == null)
return false;
if (other.getTargetHealth() != null && other.getTargetHealth().equals(this.getTargetHealth()) == false)
return false;
if (other.getAnomalyDetection() == null ^ this.getAnomalyDetection() == null)
return false;
if (other.getAnomalyDetection() != null && other.getAnomalyDetection().equals(this.getAnomalyDetection()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTarget() == null) ? 0 : getTarget().hashCode());
hashCode = prime * hashCode + ((getHealthCheckPort() == null) ? 0 : getHealthCheckPort().hashCode());
hashCode = prime * hashCode + ((getTargetHealth() == null) ? 0 : getTargetHealth().hashCode());
hashCode = prime * hashCode + ((getAnomalyDetection() == null) ? 0 : getAnomalyDetection().hashCode());
return hashCode;
}
@Override
public TargetHealthDescription clone() {
try {
return (TargetHealthDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}