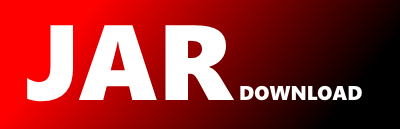
com.amazonaws.services.elasticsearch.AWSElasticsearch Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticsearch Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticsearch;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.elasticsearch.model.*;
/**
* Interface for accessing Amazon Elasticsearch Service.
*
* Amazon Elasticsearch Configuration Service
*
* Use the Amazon Elasticsearch configuration API to create, configure, and
* manage Elasticsearch domains.
*
*
* The endpoint for configuration service requests is region-specific:
* es.region.amazonaws.com. For example, es.us-east-1.amazonaws.com. For
* a current list of supported regions and endpoints, see Regions and Endpoints.
*
*/
public interface AWSElasticsearch {
/**
* Overrides the default endpoint for this client
* ("https://es.us-east-1.amazonaws.com"). Callers can use this method to
* control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "es.us-east-1.amazonaws.com")
* or a full URL, including the protocol (ex:
* "https://es.us-east-1.amazonaws.com"). If the protocol is not specified
* here, the default protocol from this client's {@link ClientConfiguration}
* will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see: http://developer.amazonwebservices.com/connect/entry.jspa?externalID=
* 3912
*
* This method is not threadsafe. An endpoint should be configured when
* the client is created and before any service requests are made. Changing
* it afterwards creates inevitable race conditions for any service requests
* in transit or retrying.
*
* @param endpoint
* The endpoint (ex: "es.us-east-1.amazonaws.com") or a full URL,
* including the protocol (ex: "https://es.us-east-1.amazonaws.com")
* of the region specific AWS endpoint this client will communicate
* with.
*/
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSElasticsearch#setEndpoint(String)}, sets the
* regional endpoint for this client's service calls. Callers can use this
* method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region. Must not be null and must be a region
* where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class,
* com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
*/
void setRegion(Region region);
/**
*
* Attaches tags to an existing Elasticsearch domain. Tags are a set of
* case-sensitive key value pairs. An Elasticsearch domain may have up to 10
* tags. See Tagging Amazon Elasticsearch Service Domains for more
* information.
*
*
* @param addTagsRequest
* Container for the parameters to the AddTags
* operation. Specify the tags that you want to attach to the
* Elasticsearch domain.
* @return Result of the AddTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or
* sub-resources. Gives http status code of 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @sample AWSElasticsearch.AddTags
*/
AddTagsResult addTags(AddTagsRequest addTagsRequest);
/**
*
* Creates a new Elasticsearch domain. For more information, see Creating Elasticsearch Domains in the Amazon
* Elasticsearch Service Developer Guide.
*
*
* @param createElasticsearchDomainRequest
* @return Result of the CreateElasticsearchDomain operation returned by the
* service.
* @throws BaseException
* An error occurred while processing the request.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not
* supported operation. Gives http status code of 409.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @throws InvalidTypeException
* An exception for trying to create or access sub-resource that is
* either invalid or not supported. Gives http status code of 409.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or
* sub-resources. Gives http status code of 409.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists. Gives
* http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.CreateElasticsearchDomain
*/
CreateElasticsearchDomainResult createElasticsearchDomain(
CreateElasticsearchDomainRequest createElasticsearchDomainRequest);
/**
*
* Permanently deletes the specified Elasticsearch domain and all of its
* data. Once a domain is deleted, it cannot be recovered.
*
*
* @param deleteElasticsearchDomainRequest
* Container for the parameters to the
* DeleteElasticsearchDomain
operation. Specifies
* the name of the Elasticsearch domain that you want to delete.
* @return Result of the DeleteElasticsearchDomain operation returned by the
* service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not
* exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.DeleteElasticsearchDomain
*/
DeleteElasticsearchDomainResult deleteElasticsearchDomain(
DeleteElasticsearchDomainRequest deleteElasticsearchDomainRequest);
/**
*
* Returns domain configuration information about the specified
* Elasticsearch domain, including the domain ID, domain endpoint, and
* domain ARN.
*
*
* @param describeElasticsearchDomainRequest
* Container for the parameters to the
* DescribeElasticsearchDomain
operation.
* @return Result of the DescribeElasticsearchDomain operation returned by
* the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not
* exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchDomain
*/
DescribeElasticsearchDomainResult describeElasticsearchDomain(
DescribeElasticsearchDomainRequest describeElasticsearchDomainRequest);
/**
*
* Provides cluster configuration information about the specified
* Elasticsearch domain, such as the state, creation date, update version,
* and update date for cluster options.
*
*
* @param describeElasticsearchDomainConfigRequest
* Container for the parameters to the
* DescribeElasticsearchDomainConfig
operation.
* Specifies the domain name for which you want configuration
* information.
* @return Result of the DescribeElasticsearchDomainConfig operation
* returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not
* exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchDomainConfig
*/
DescribeElasticsearchDomainConfigResult describeElasticsearchDomainConfig(
DescribeElasticsearchDomainConfigRequest describeElasticsearchDomainConfigRequest);
/**
*
* Returns domain configuration information about the specified
* Elasticsearch domains, including the domain ID, domain endpoint, and
* domain ARN.
*
*
* @param describeElasticsearchDomainsRequest
* Container for the parameters to the
* DescribeElasticsearchDomains
operation. By
* default, the API returns the status of all Elasticsearch domains.
* @return Result of the DescribeElasticsearchDomains operation returned by
* the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchDomains
*/
DescribeElasticsearchDomainsResult describeElasticsearchDomains(
DescribeElasticsearchDomainsRequest describeElasticsearchDomainsRequest);
/**
*
* Returns the name of all Elasticsearch domains owned by the current user's
* account.
*
*
* @param listDomainNamesRequest
* @return Result of the ListDomainNames operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.ListDomainNames
*/
ListDomainNamesResult listDomainNames(
ListDomainNamesRequest listDomainNamesRequest);
/**
*
* Returns all tags for the given Elasticsearch domain.
*
*
* @param listTagsRequest
* Container for the parameters to the ListTags
* operation. Specify the ARN
for the Elasticsearch
* domain to which the tags are attached that you want to view are
* attached.
* @return Result of the ListTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not
* exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @sample AWSElasticsearch.ListTags
*/
ListTagsResult listTags(ListTagsRequest listTagsRequest);
/**
*
* Removes the specified set of tags from the specified Elasticsearch
* domain.
*
*
* @param removeTagsRequest
* Container for the parameters to the RemoveTags
* operation. Specify the ARN
for the Elasticsearch
* domain from which you want to remove the specified
* TagKey
.
* @return Result of the RemoveTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @sample AWSElasticsearch.RemoveTags
*/
RemoveTagsResult removeTags(RemoveTagsRequest removeTagsRequest);
/**
*
* Modifies the cluster configuration of the specified Elasticsearch domain,
* setting as setting the instance type and the number of instances.
*
*
* @param updateElasticsearchDomainConfigRequest
* Container for the parameters to the
* UpdateElasticsearchDomain
operation. Specifies
* the type and number of instances in the domain cluster.
* @return Result of the UpdateElasticsearchDomainConfig operation returned
* by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error,
* exception or failure (the failure is internal to the service) .
* Gives http status code of 500.
* @throws InvalidTypeException
* An exception for trying to create or access sub-resource that is
* either invalid or not supported. Gives http status code of 409.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or
* sub-resources. Gives http status code of 409.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not
* exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http
* status code of 400.
* @sample AWSElasticsearch.UpdateElasticsearchDomainConfig
*/
UpdateElasticsearchDomainConfigResult updateElasticsearchDomainConfig(
UpdateElasticsearchDomainConfigRequest updateElasticsearchDomainConfigRequest);
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request,
* typically used for debugging issues where a service isn't acting as
* expected. This data isn't considered part of the result data returned by
* an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}