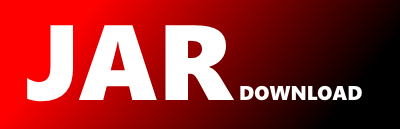
com.amazonaws.services.elasticsearch.AWSElasticsearchAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticsearch Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticsearch;
import com.amazonaws.services.elasticsearch.model.*;
/**
* Interface for accessing Amazon Elasticsearch Service asynchronously. Each
* asynchronous method will return a Java Future object representing the
* asynchronous operation; overloads which accept an {@code AsyncHandler} can be
* used to receive notification when an asynchronous operation completes.
*
* Amazon Elasticsearch Configuration Service
*
* Use the Amazon Elasticsearch configuration API to create, configure, and
* manage Elasticsearch domains.
*
*
* The endpoint for configuration service requests is region-specific:
* es.region.amazonaws.com. For example, es.us-east-1.amazonaws.com. For
* a current list of supported regions and endpoints, see Regions and Endpoints.
*
*/
public interface AWSElasticsearchAsync extends AWSElasticsearch {
/**
*
* Attaches tags to an existing Elasticsearch domain. Tags are a set of
* case-sensitive key value pairs. An Elasticsearch domain may have up to 10
* tags. See Tagging Amazon Elasticsearch Service Domains for more
* information.
*
*
* @param addTagsRequest
* Container for the parameters to the AddTags
* operation. Specify the tags that you want to attach to the
* Elasticsearch domain.
* @return A Java Future containing the result of the AddTags operation
* returned by the service.
* @sample AWSElasticsearchAsync.AddTags
*/
java.util.concurrent.Future addTagsAsync(
AddTagsRequest addTagsRequest);
/**
*
* Attaches tags to an existing Elasticsearch domain. Tags are a set of
* case-sensitive key value pairs. An Elasticsearch domain may have up to 10
* tags. See Tagging Amazon Elasticsearch Service Domains for more
* information.
*
*
* @param addTagsRequest
* Container for the parameters to the AddTags
* operation. Specify the tags that you want to attach to the
* Elasticsearch domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTags operation
* returned by the service.
* @sample AWSElasticsearchAsyncHandler.AddTags
*/
java.util.concurrent.Future addTagsAsync(
AddTagsRequest addTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Elasticsearch domain. For more information, see Creating Elasticsearch Domains in the Amazon
* Elasticsearch Service Developer Guide.
*
*
* @param createElasticsearchDomainRequest
* @return A Java Future containing the result of the
* CreateElasticsearchDomain operation returned by the service.
* @sample AWSElasticsearchAsync.CreateElasticsearchDomain
*/
java.util.concurrent.Future createElasticsearchDomainAsync(
CreateElasticsearchDomainRequest createElasticsearchDomainRequest);
/**
*
* Creates a new Elasticsearch domain. For more information, see Creating Elasticsearch Domains in the Amazon
* Elasticsearch Service Developer Guide.
*
*
* @param createElasticsearchDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateElasticsearchDomain operation returned by the service.
* @sample AWSElasticsearchAsyncHandler.CreateElasticsearchDomain
*/
java.util.concurrent.Future createElasticsearchDomainAsync(
CreateElasticsearchDomainRequest createElasticsearchDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently deletes the specified Elasticsearch domain and all of its
* data. Once a domain is deleted, it cannot be recovered.
*
*
* @param deleteElasticsearchDomainRequest
* Container for the parameters to the
* DeleteElasticsearchDomain
operation. Specifies
* the name of the Elasticsearch domain that you want to delete.
* @return A Java Future containing the result of the
* DeleteElasticsearchDomain operation returned by the service.
* @sample AWSElasticsearchAsync.DeleteElasticsearchDomain
*/
java.util.concurrent.Future deleteElasticsearchDomainAsync(
DeleteElasticsearchDomainRequest deleteElasticsearchDomainRequest);
/**
*
* Permanently deletes the specified Elasticsearch domain and all of its
* data. Once a domain is deleted, it cannot be recovered.
*
*
* @param deleteElasticsearchDomainRequest
* Container for the parameters to the
* DeleteElasticsearchDomain
operation. Specifies
* the name of the Elasticsearch domain that you want to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteElasticsearchDomain operation returned by the service.
* @sample AWSElasticsearchAsyncHandler.DeleteElasticsearchDomain
*/
java.util.concurrent.Future deleteElasticsearchDomainAsync(
DeleteElasticsearchDomainRequest deleteElasticsearchDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns domain configuration information about the specified
* Elasticsearch domain, including the domain ID, domain endpoint, and
* domain ARN.
*
*
* @param describeElasticsearchDomainRequest
* Container for the parameters to the
* DescribeElasticsearchDomain
operation.
* @return A Java Future containing the result of the
* DescribeElasticsearchDomain operation returned by the service.
* @sample AWSElasticsearchAsync.DescribeElasticsearchDomain
*/
java.util.concurrent.Future describeElasticsearchDomainAsync(
DescribeElasticsearchDomainRequest describeElasticsearchDomainRequest);
/**
*
* Returns domain configuration information about the specified
* Elasticsearch domain, including the domain ID, domain endpoint, and
* domain ARN.
*
*
* @param describeElasticsearchDomainRequest
* Container for the parameters to the
* DescribeElasticsearchDomain
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeElasticsearchDomain operation returned by the service.
* @sample AWSElasticsearchAsyncHandler.DescribeElasticsearchDomain
*/
java.util.concurrent.Future describeElasticsearchDomainAsync(
DescribeElasticsearchDomainRequest describeElasticsearchDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides cluster configuration information about the specified
* Elasticsearch domain, such as the state, creation date, update version,
* and update date for cluster options.
*
*
* @param describeElasticsearchDomainConfigRequest
* Container for the parameters to the
* DescribeElasticsearchDomainConfig
operation.
* Specifies the domain name for which you want configuration
* information.
* @return A Java Future containing the result of the
* DescribeElasticsearchDomainConfig operation returned by the
* service.
* @sample AWSElasticsearchAsync.DescribeElasticsearchDomainConfig
*/
java.util.concurrent.Future describeElasticsearchDomainConfigAsync(
DescribeElasticsearchDomainConfigRequest describeElasticsearchDomainConfigRequest);
/**
*
* Provides cluster configuration information about the specified
* Elasticsearch domain, such as the state, creation date, update version,
* and update date for cluster options.
*
*
* @param describeElasticsearchDomainConfigRequest
* Container for the parameters to the
* DescribeElasticsearchDomainConfig
operation.
* Specifies the domain name for which you want configuration
* information.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeElasticsearchDomainConfig operation returned by the
* service.
* @sample AWSElasticsearchAsyncHandler.DescribeElasticsearchDomainConfig
*/
java.util.concurrent.Future describeElasticsearchDomainConfigAsync(
DescribeElasticsearchDomainConfigRequest describeElasticsearchDomainConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns domain configuration information about the specified
* Elasticsearch domains, including the domain ID, domain endpoint, and
* domain ARN.
*
*
* @param describeElasticsearchDomainsRequest
* Container for the parameters to the
* DescribeElasticsearchDomains
operation. By
* default, the API returns the status of all Elasticsearch domains.
* @return A Java Future containing the result of the
* DescribeElasticsearchDomains operation returned by the service.
* @sample AWSElasticsearchAsync.DescribeElasticsearchDomains
*/
java.util.concurrent.Future describeElasticsearchDomainsAsync(
DescribeElasticsearchDomainsRequest describeElasticsearchDomainsRequest);
/**
*
* Returns domain configuration information about the specified
* Elasticsearch domains, including the domain ID, domain endpoint, and
* domain ARN.
*
*
* @param describeElasticsearchDomainsRequest
* Container for the parameters to the
* DescribeElasticsearchDomains
operation. By
* default, the API returns the status of all Elasticsearch domains.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeElasticsearchDomains operation returned by the service.
* @sample AWSElasticsearchAsyncHandler.DescribeElasticsearchDomains
*/
java.util.concurrent.Future describeElasticsearchDomainsAsync(
DescribeElasticsearchDomainsRequest describeElasticsearchDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the name of all Elasticsearch domains owned by the current user's
* account.
*
*
* @param listDomainNamesRequest
* @return A Java Future containing the result of the ListDomainNames
* operation returned by the service.
* @sample AWSElasticsearchAsync.ListDomainNames
*/
java.util.concurrent.Future listDomainNamesAsync(
ListDomainNamesRequest listDomainNamesRequest);
/**
*
* Returns the name of all Elasticsearch domains owned by the current user's
* account.
*
*
* @param listDomainNamesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomainNames
* operation returned by the service.
* @sample AWSElasticsearchAsyncHandler.ListDomainNames
*/
java.util.concurrent.Future listDomainNamesAsync(
ListDomainNamesRequest listDomainNamesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all tags for the given Elasticsearch domain.
*
*
* @param listTagsRequest
* Container for the parameters to the ListTags
* operation. Specify the ARN
for the Elasticsearch
* domain to which the tags are attached that you want to view are
* attached.
* @return A Java Future containing the result of the ListTags operation
* returned by the service.
* @sample AWSElasticsearchAsync.ListTags
*/
java.util.concurrent.Future listTagsAsync(
ListTagsRequest listTagsRequest);
/**
*
* Returns all tags for the given Elasticsearch domain.
*
*
* @param listTagsRequest
* Container for the parameters to the ListTags
* operation. Specify the ARN
for the Elasticsearch
* domain to which the tags are attached that you want to view are
* attached.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTags operation
* returned by the service.
* @sample AWSElasticsearchAsyncHandler.ListTags
*/
java.util.concurrent.Future listTagsAsync(
ListTagsRequest listTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified set of tags from the specified Elasticsearch
* domain.
*
*
* @param removeTagsRequest
* Container for the parameters to the RemoveTags
* operation. Specify the ARN
for the Elasticsearch
* domain from which you want to remove the specified
* TagKey
.
* @return A Java Future containing the result of the RemoveTags operation
* returned by the service.
* @sample AWSElasticsearchAsync.RemoveTags
*/
java.util.concurrent.Future removeTagsAsync(
RemoveTagsRequest removeTagsRequest);
/**
*
* Removes the specified set of tags from the specified Elasticsearch
* domain.
*
*
* @param removeTagsRequest
* Container for the parameters to the RemoveTags
* operation. Specify the ARN
for the Elasticsearch
* domain from which you want to remove the specified
* TagKey
.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTags operation
* returned by the service.
* @sample AWSElasticsearchAsyncHandler.RemoveTags
*/
java.util.concurrent.Future removeTagsAsync(
RemoveTagsRequest removeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the cluster configuration of the specified Elasticsearch domain,
* setting as setting the instance type and the number of instances.
*
*
* @param updateElasticsearchDomainConfigRequest
* Container for the parameters to the
* UpdateElasticsearchDomain
operation. Specifies
* the type and number of instances in the domain cluster.
* @return A Java Future containing the result of the
* UpdateElasticsearchDomainConfig operation returned by the
* service.
* @sample AWSElasticsearchAsync.UpdateElasticsearchDomainConfig
*/
java.util.concurrent.Future updateElasticsearchDomainConfigAsync(
UpdateElasticsearchDomainConfigRequest updateElasticsearchDomainConfigRequest);
/**
*
* Modifies the cluster configuration of the specified Elasticsearch domain,
* setting as setting the instance type and the number of instances.
*
*
* @param updateElasticsearchDomainConfigRequest
* Container for the parameters to the
* UpdateElasticsearchDomain
operation. Specifies
* the type and number of instances in the domain cluster.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* UpdateElasticsearchDomainConfig operation returned by the
* service.
* @sample AWSElasticsearchAsyncHandler.UpdateElasticsearchDomainConfig
*/
java.util.concurrent.Future updateElasticsearchDomainConfigAsync(
UpdateElasticsearchDomainConfigRequest updateElasticsearchDomainConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}