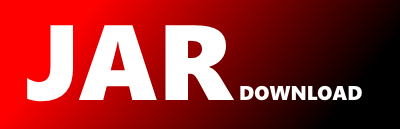
com.amazonaws.services.elasticsearch.model.CreateElasticsearchDomainRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticsearch Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticsearch.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateElasticsearchDomainRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains owned by
* an account within an AWS region. Domain names must start with a lowercase letter and can contain the following
* characters: a-z (lowercase), 0-9, and - (hyphen).
*
*/
private String domainName;
/**
*
* String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more information,
* see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer Guide.
*
*/
private String elasticsearchVersion;
/**
*
* Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in the
* domain cluster.
*
*/
private ElasticsearchClusterConfig elasticsearchClusterConfig;
/**
*
* Options to enable, disable and specify the type and size of EBS storage volumes.
*
*/
private EBSOptions eBSOptions;
/**
*
* IAM access policy as a JSON-formatted string.
*
*/
private String accessPolicies;
/**
*
* Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
*
*/
private SnapshotOptions snapshotOptions;
/**
*
* Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
*
*/
private VPCOptions vPCOptions;
/**
*
* Options to specify the Cognito user and identity pools for Kibana authentication. For more information, see Amazon Cognito Authentication for Kibana.
*
*/
private CognitoOptions cognitoOptions;
/**
*
* Specifies the Encryption At Rest Options.
*
*/
private EncryptionAtRestOptions encryptionAtRestOptions;
/**
*
* Specifies the NodeToNodeEncryptionOptions.
*
*/
private NodeToNodeEncryptionOptions nodeToNodeEncryptionOptions;
/**
*
* Option to allow references to indices in an HTTP request body. Must be false
when configuring access
* to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
*
*/
private java.util.Map advancedOptions;
/**
*
* Map of LogType
and LogPublishingOption
, each containing options to publish a given type
* of Elasticsearch log.
*
*/
private java.util.Map logPublishingOptions;
/**
*
* Options to specify configuration that will be applied to the domain endpoint.
*
*/
private DomainEndpointOptions domainEndpointOptions;
/**
*
* Specifies advanced security options.
*
*/
private AdvancedSecurityOptionsInput advancedSecurityOptions;
/**
*
* The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains owned by
* an account within an AWS region. Domain names must start with a lowercase letter and can contain the following
* characters: a-z (lowercase), 0-9, and - (hyphen).
*
*
* @param domainName
* The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains
* owned by an account within an AWS region. Domain names must start with a lowercase letter and can contain
* the following characters: a-z (lowercase), 0-9, and - (hyphen).
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains owned by
* an account within an AWS region. Domain names must start with a lowercase letter and can contain the following
* characters: a-z (lowercase), 0-9, and - (hyphen).
*
*
* @return The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains
* owned by an account within an AWS region. Domain names must start with a lowercase letter and can contain
* the following characters: a-z (lowercase), 0-9, and - (hyphen).
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains owned by
* an account within an AWS region. Domain names must start with a lowercase letter and can contain the following
* characters: a-z (lowercase), 0-9, and - (hyphen).
*
*
* @param domainName
* The name of the Elasticsearch domain that you are creating. Domain names are unique across the domains
* owned by an account within an AWS region. Domain names must start with a lowercase letter and can contain
* the following characters: a-z (lowercase), 0-9, and - (hyphen).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more information,
* see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer Guide.
*
*
* @param elasticsearchVersion
* String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more
* information, see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer
* Guide.
*/
public void setElasticsearchVersion(String elasticsearchVersion) {
this.elasticsearchVersion = elasticsearchVersion;
}
/**
*
* String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more information,
* see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer Guide.
*
*
* @return String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more
* information, see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer
* Guide.
*/
public String getElasticsearchVersion() {
return this.elasticsearchVersion;
}
/**
*
* String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more information,
* see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer Guide.
*
*
* @param elasticsearchVersion
* String of format X.Y to specify version for the Elasticsearch domain eg. "1.5" or "2.3". For more
* information, see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer
* Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withElasticsearchVersion(String elasticsearchVersion) {
setElasticsearchVersion(elasticsearchVersion);
return this;
}
/**
*
* Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in the
* domain cluster.
*
*
* @param elasticsearchClusterConfig
* Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in
* the domain cluster.
*/
public void setElasticsearchClusterConfig(ElasticsearchClusterConfig elasticsearchClusterConfig) {
this.elasticsearchClusterConfig = elasticsearchClusterConfig;
}
/**
*
* Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in the
* domain cluster.
*
*
* @return Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in
* the domain cluster.
*/
public ElasticsearchClusterConfig getElasticsearchClusterConfig() {
return this.elasticsearchClusterConfig;
}
/**
*
* Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in the
* domain cluster.
*
*
* @param elasticsearchClusterConfig
* Configuration options for an Elasticsearch domain. Specifies the instance type and number of instances in
* the domain cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withElasticsearchClusterConfig(ElasticsearchClusterConfig elasticsearchClusterConfig) {
setElasticsearchClusterConfig(elasticsearchClusterConfig);
return this;
}
/**
*
* Options to enable, disable and specify the type and size of EBS storage volumes.
*
*
* @param eBSOptions
* Options to enable, disable and specify the type and size of EBS storage volumes.
*/
public void setEBSOptions(EBSOptions eBSOptions) {
this.eBSOptions = eBSOptions;
}
/**
*
* Options to enable, disable and specify the type and size of EBS storage volumes.
*
*
* @return Options to enable, disable and specify the type and size of EBS storage volumes.
*/
public EBSOptions getEBSOptions() {
return this.eBSOptions;
}
/**
*
* Options to enable, disable and specify the type and size of EBS storage volumes.
*
*
* @param eBSOptions
* Options to enable, disable and specify the type and size of EBS storage volumes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withEBSOptions(EBSOptions eBSOptions) {
setEBSOptions(eBSOptions);
return this;
}
/**
*
* IAM access policy as a JSON-formatted string.
*
*
* @param accessPolicies
* IAM access policy as a JSON-formatted string.
*/
public void setAccessPolicies(String accessPolicies) {
this.accessPolicies = accessPolicies;
}
/**
*
* IAM access policy as a JSON-formatted string.
*
*
* @return IAM access policy as a JSON-formatted string.
*/
public String getAccessPolicies() {
return this.accessPolicies;
}
/**
*
* IAM access policy as a JSON-formatted string.
*
*
* @param accessPolicies
* IAM access policy as a JSON-formatted string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withAccessPolicies(String accessPolicies) {
setAccessPolicies(accessPolicies);
return this;
}
/**
*
* Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
*
*
* @param snapshotOptions
* Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
*/
public void setSnapshotOptions(SnapshotOptions snapshotOptions) {
this.snapshotOptions = snapshotOptions;
}
/**
*
* Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
*
*
* @return Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
*/
public SnapshotOptions getSnapshotOptions() {
return this.snapshotOptions;
}
/**
*
* Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
*
*
* @param snapshotOptions
* Option to set time, in UTC format, of the daily automated snapshot. Default value is 0 hours.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withSnapshotOptions(SnapshotOptions snapshotOptions) {
setSnapshotOptions(snapshotOptions);
return this;
}
/**
*
* Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
*
*
* @param vPCOptions
* Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
*/
public void setVPCOptions(VPCOptions vPCOptions) {
this.vPCOptions = vPCOptions;
}
/**
*
* Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
*
*
* @return Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
*/
public VPCOptions getVPCOptions() {
return this.vPCOptions;
}
/**
*
* Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
*
*
* @param vPCOptions
* Options to specify the subnets and security groups for VPC endpoint. For more information, see Creating a VPC in VPC Endpoints for Amazon Elasticsearch Service Domains
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withVPCOptions(VPCOptions vPCOptions) {
setVPCOptions(vPCOptions);
return this;
}
/**
*
* Options to specify the Cognito user and identity pools for Kibana authentication. For more information, see Amazon Cognito Authentication for Kibana.
*
*
* @param cognitoOptions
* Options to specify the Cognito user and identity pools for Kibana authentication. For more information,
* see Amazon Cognito Authentication for Kibana.
*/
public void setCognitoOptions(CognitoOptions cognitoOptions) {
this.cognitoOptions = cognitoOptions;
}
/**
*
* Options to specify the Cognito user and identity pools for Kibana authentication. For more information, see Amazon Cognito Authentication for Kibana.
*
*
* @return Options to specify the Cognito user and identity pools for Kibana authentication. For more information,
* see Amazon Cognito Authentication for Kibana.
*/
public CognitoOptions getCognitoOptions() {
return this.cognitoOptions;
}
/**
*
* Options to specify the Cognito user and identity pools for Kibana authentication. For more information, see Amazon Cognito Authentication for Kibana.
*
*
* @param cognitoOptions
* Options to specify the Cognito user and identity pools for Kibana authentication. For more information,
* see Amazon Cognito Authentication for Kibana.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withCognitoOptions(CognitoOptions cognitoOptions) {
setCognitoOptions(cognitoOptions);
return this;
}
/**
*
* Specifies the Encryption At Rest Options.
*
*
* @param encryptionAtRestOptions
* Specifies the Encryption At Rest Options.
*/
public void setEncryptionAtRestOptions(EncryptionAtRestOptions encryptionAtRestOptions) {
this.encryptionAtRestOptions = encryptionAtRestOptions;
}
/**
*
* Specifies the Encryption At Rest Options.
*
*
* @return Specifies the Encryption At Rest Options.
*/
public EncryptionAtRestOptions getEncryptionAtRestOptions() {
return this.encryptionAtRestOptions;
}
/**
*
* Specifies the Encryption At Rest Options.
*
*
* @param encryptionAtRestOptions
* Specifies the Encryption At Rest Options.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withEncryptionAtRestOptions(EncryptionAtRestOptions encryptionAtRestOptions) {
setEncryptionAtRestOptions(encryptionAtRestOptions);
return this;
}
/**
*
* Specifies the NodeToNodeEncryptionOptions.
*
*
* @param nodeToNodeEncryptionOptions
* Specifies the NodeToNodeEncryptionOptions.
*/
public void setNodeToNodeEncryptionOptions(NodeToNodeEncryptionOptions nodeToNodeEncryptionOptions) {
this.nodeToNodeEncryptionOptions = nodeToNodeEncryptionOptions;
}
/**
*
* Specifies the NodeToNodeEncryptionOptions.
*
*
* @return Specifies the NodeToNodeEncryptionOptions.
*/
public NodeToNodeEncryptionOptions getNodeToNodeEncryptionOptions() {
return this.nodeToNodeEncryptionOptions;
}
/**
*
* Specifies the NodeToNodeEncryptionOptions.
*
*
* @param nodeToNodeEncryptionOptions
* Specifies the NodeToNodeEncryptionOptions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withNodeToNodeEncryptionOptions(NodeToNodeEncryptionOptions nodeToNodeEncryptionOptions) {
setNodeToNodeEncryptionOptions(nodeToNodeEncryptionOptions);
return this;
}
/**
*
* Option to allow references to indices in an HTTP request body. Must be false
when configuring access
* to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
*
*
* @return Option to allow references to indices in an HTTP request body. Must be false
when
* configuring access to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
*/
public java.util.Map getAdvancedOptions() {
return advancedOptions;
}
/**
*
* Option to allow references to indices in an HTTP request body. Must be false
when configuring access
* to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
*
*
* @param advancedOptions
* Option to allow references to indices in an HTTP request body. Must be false
when configuring
* access to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
*/
public void setAdvancedOptions(java.util.Map advancedOptions) {
this.advancedOptions = advancedOptions;
}
/**
*
* Option to allow references to indices in an HTTP request body. Must be false
when configuring access
* to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
*
*
* @param advancedOptions
* Option to allow references to indices in an HTTP request body. Must be false
when configuring
* access to individual sub-resources. By default, the value is true
. See Configuration Advanced Options for more information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withAdvancedOptions(java.util.Map advancedOptions) {
setAdvancedOptions(advancedOptions);
return this;
}
public CreateElasticsearchDomainRequest addAdvancedOptionsEntry(String key, String value) {
if (null == this.advancedOptions) {
this.advancedOptions = new java.util.HashMap();
}
if (this.advancedOptions.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.advancedOptions.put(key, value);
return this;
}
/**
* Removes all the entries added into AdvancedOptions.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest clearAdvancedOptionsEntries() {
this.advancedOptions = null;
return this;
}
/**
*
* Map of LogType
and LogPublishingOption
, each containing options to publish a given type
* of Elasticsearch log.
*
*
* @return Map of LogType
and LogPublishingOption
, each containing options to publish a
* given type of Elasticsearch log.
*/
public java.util.Map getLogPublishingOptions() {
return logPublishingOptions;
}
/**
*
* Map of LogType
and LogPublishingOption
, each containing options to publish a given type
* of Elasticsearch log.
*
*
* @param logPublishingOptions
* Map of LogType
and LogPublishingOption
, each containing options to publish a
* given type of Elasticsearch log.
*/
public void setLogPublishingOptions(java.util.Map logPublishingOptions) {
this.logPublishingOptions = logPublishingOptions;
}
/**
*
* Map of LogType
and LogPublishingOption
, each containing options to publish a given type
* of Elasticsearch log.
*
*
* @param logPublishingOptions
* Map of LogType
and LogPublishingOption
, each containing options to publish a
* given type of Elasticsearch log.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withLogPublishingOptions(java.util.Map logPublishingOptions) {
setLogPublishingOptions(logPublishingOptions);
return this;
}
public CreateElasticsearchDomainRequest addLogPublishingOptionsEntry(String key, LogPublishingOption value) {
if (null == this.logPublishingOptions) {
this.logPublishingOptions = new java.util.HashMap();
}
if (this.logPublishingOptions.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.logPublishingOptions.put(key, value);
return this;
}
/**
* Removes all the entries added into LogPublishingOptions.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest clearLogPublishingOptionsEntries() {
this.logPublishingOptions = null;
return this;
}
/**
*
* Options to specify configuration that will be applied to the domain endpoint.
*
*
* @param domainEndpointOptions
* Options to specify configuration that will be applied to the domain endpoint.
*/
public void setDomainEndpointOptions(DomainEndpointOptions domainEndpointOptions) {
this.domainEndpointOptions = domainEndpointOptions;
}
/**
*
* Options to specify configuration that will be applied to the domain endpoint.
*
*
* @return Options to specify configuration that will be applied to the domain endpoint.
*/
public DomainEndpointOptions getDomainEndpointOptions() {
return this.domainEndpointOptions;
}
/**
*
* Options to specify configuration that will be applied to the domain endpoint.
*
*
* @param domainEndpointOptions
* Options to specify configuration that will be applied to the domain endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withDomainEndpointOptions(DomainEndpointOptions domainEndpointOptions) {
setDomainEndpointOptions(domainEndpointOptions);
return this;
}
/**
*
* Specifies advanced security options.
*
*
* @param advancedSecurityOptions
* Specifies advanced security options.
*/
public void setAdvancedSecurityOptions(AdvancedSecurityOptionsInput advancedSecurityOptions) {
this.advancedSecurityOptions = advancedSecurityOptions;
}
/**
*
* Specifies advanced security options.
*
*
* @return Specifies advanced security options.
*/
public AdvancedSecurityOptionsInput getAdvancedSecurityOptions() {
return this.advancedSecurityOptions;
}
/**
*
* Specifies advanced security options.
*
*
* @param advancedSecurityOptions
* Specifies advanced security options.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateElasticsearchDomainRequest withAdvancedSecurityOptions(AdvancedSecurityOptionsInput advancedSecurityOptions) {
setAdvancedSecurityOptions(advancedSecurityOptions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getElasticsearchVersion() != null)
sb.append("ElasticsearchVersion: ").append(getElasticsearchVersion()).append(",");
if (getElasticsearchClusterConfig() != null)
sb.append("ElasticsearchClusterConfig: ").append(getElasticsearchClusterConfig()).append(",");
if (getEBSOptions() != null)
sb.append("EBSOptions: ").append(getEBSOptions()).append(",");
if (getAccessPolicies() != null)
sb.append("AccessPolicies: ").append(getAccessPolicies()).append(",");
if (getSnapshotOptions() != null)
sb.append("SnapshotOptions: ").append(getSnapshotOptions()).append(",");
if (getVPCOptions() != null)
sb.append("VPCOptions: ").append(getVPCOptions()).append(",");
if (getCognitoOptions() != null)
sb.append("CognitoOptions: ").append(getCognitoOptions()).append(",");
if (getEncryptionAtRestOptions() != null)
sb.append("EncryptionAtRestOptions: ").append(getEncryptionAtRestOptions()).append(",");
if (getNodeToNodeEncryptionOptions() != null)
sb.append("NodeToNodeEncryptionOptions: ").append(getNodeToNodeEncryptionOptions()).append(",");
if (getAdvancedOptions() != null)
sb.append("AdvancedOptions: ").append(getAdvancedOptions()).append(",");
if (getLogPublishingOptions() != null)
sb.append("LogPublishingOptions: ").append(getLogPublishingOptions()).append(",");
if (getDomainEndpointOptions() != null)
sb.append("DomainEndpointOptions: ").append(getDomainEndpointOptions()).append(",");
if (getAdvancedSecurityOptions() != null)
sb.append("AdvancedSecurityOptions: ").append(getAdvancedSecurityOptions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateElasticsearchDomainRequest == false)
return false;
CreateElasticsearchDomainRequest other = (CreateElasticsearchDomainRequest) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getElasticsearchVersion() == null ^ this.getElasticsearchVersion() == null)
return false;
if (other.getElasticsearchVersion() != null && other.getElasticsearchVersion().equals(this.getElasticsearchVersion()) == false)
return false;
if (other.getElasticsearchClusterConfig() == null ^ this.getElasticsearchClusterConfig() == null)
return false;
if (other.getElasticsearchClusterConfig() != null && other.getElasticsearchClusterConfig().equals(this.getElasticsearchClusterConfig()) == false)
return false;
if (other.getEBSOptions() == null ^ this.getEBSOptions() == null)
return false;
if (other.getEBSOptions() != null && other.getEBSOptions().equals(this.getEBSOptions()) == false)
return false;
if (other.getAccessPolicies() == null ^ this.getAccessPolicies() == null)
return false;
if (other.getAccessPolicies() != null && other.getAccessPolicies().equals(this.getAccessPolicies()) == false)
return false;
if (other.getSnapshotOptions() == null ^ this.getSnapshotOptions() == null)
return false;
if (other.getSnapshotOptions() != null && other.getSnapshotOptions().equals(this.getSnapshotOptions()) == false)
return false;
if (other.getVPCOptions() == null ^ this.getVPCOptions() == null)
return false;
if (other.getVPCOptions() != null && other.getVPCOptions().equals(this.getVPCOptions()) == false)
return false;
if (other.getCognitoOptions() == null ^ this.getCognitoOptions() == null)
return false;
if (other.getCognitoOptions() != null && other.getCognitoOptions().equals(this.getCognitoOptions()) == false)
return false;
if (other.getEncryptionAtRestOptions() == null ^ this.getEncryptionAtRestOptions() == null)
return false;
if (other.getEncryptionAtRestOptions() != null && other.getEncryptionAtRestOptions().equals(this.getEncryptionAtRestOptions()) == false)
return false;
if (other.getNodeToNodeEncryptionOptions() == null ^ this.getNodeToNodeEncryptionOptions() == null)
return false;
if (other.getNodeToNodeEncryptionOptions() != null && other.getNodeToNodeEncryptionOptions().equals(this.getNodeToNodeEncryptionOptions()) == false)
return false;
if (other.getAdvancedOptions() == null ^ this.getAdvancedOptions() == null)
return false;
if (other.getAdvancedOptions() != null && other.getAdvancedOptions().equals(this.getAdvancedOptions()) == false)
return false;
if (other.getLogPublishingOptions() == null ^ this.getLogPublishingOptions() == null)
return false;
if (other.getLogPublishingOptions() != null && other.getLogPublishingOptions().equals(this.getLogPublishingOptions()) == false)
return false;
if (other.getDomainEndpointOptions() == null ^ this.getDomainEndpointOptions() == null)
return false;
if (other.getDomainEndpointOptions() != null && other.getDomainEndpointOptions().equals(this.getDomainEndpointOptions()) == false)
return false;
if (other.getAdvancedSecurityOptions() == null ^ this.getAdvancedSecurityOptions() == null)
return false;
if (other.getAdvancedSecurityOptions() != null && other.getAdvancedSecurityOptions().equals(this.getAdvancedSecurityOptions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getElasticsearchVersion() == null) ? 0 : getElasticsearchVersion().hashCode());
hashCode = prime * hashCode + ((getElasticsearchClusterConfig() == null) ? 0 : getElasticsearchClusterConfig().hashCode());
hashCode = prime * hashCode + ((getEBSOptions() == null) ? 0 : getEBSOptions().hashCode());
hashCode = prime * hashCode + ((getAccessPolicies() == null) ? 0 : getAccessPolicies().hashCode());
hashCode = prime * hashCode + ((getSnapshotOptions() == null) ? 0 : getSnapshotOptions().hashCode());
hashCode = prime * hashCode + ((getVPCOptions() == null) ? 0 : getVPCOptions().hashCode());
hashCode = prime * hashCode + ((getCognitoOptions() == null) ? 0 : getCognitoOptions().hashCode());
hashCode = prime * hashCode + ((getEncryptionAtRestOptions() == null) ? 0 : getEncryptionAtRestOptions().hashCode());
hashCode = prime * hashCode + ((getNodeToNodeEncryptionOptions() == null) ? 0 : getNodeToNodeEncryptionOptions().hashCode());
hashCode = prime * hashCode + ((getAdvancedOptions() == null) ? 0 : getAdvancedOptions().hashCode());
hashCode = prime * hashCode + ((getLogPublishingOptions() == null) ? 0 : getLogPublishingOptions().hashCode());
hashCode = prime * hashCode + ((getDomainEndpointOptions() == null) ? 0 : getDomainEndpointOptions().hashCode());
hashCode = prime * hashCode + ((getAdvancedSecurityOptions() == null) ? 0 : getAdvancedSecurityOptions().hashCode());
return hashCode;
}
@Override
public CreateElasticsearchDomainRequest clone() {
return (CreateElasticsearchDomainRequest) super.clone();
}
}