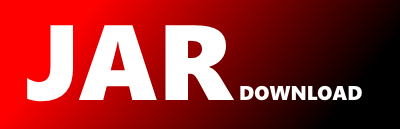
com.amazonaws.services.elasticsearch.model.SAMLOptionsInput Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticsearch Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticsearch.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Specifies the SAML application configuration for the domain.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SAMLOptionsInput implements Serializable, Cloneable, StructuredPojo {
/**
*
* True if SAML is enabled.
*
*/
private Boolean enabled;
/**
*
* Specifies the SAML Identity Provider's information.
*
*/
private SAMLIdp idp;
/**
*
* The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
*
*/
private String masterUserName;
/**
*
* The backend role to which the SAML master user is mapped to.
*
*/
private String masterBackendRole;
/**
*
* The key to use for matching the SAML Subject attribute.
*
*/
private String subjectKey;
/**
*
* The key to use for matching the SAML Roles attribute.
*
*/
private String rolesKey;
/**
*
* The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1 and 1440,
* and the default value is 60.
*
*/
private Integer sessionTimeoutMinutes;
/**
*
* True if SAML is enabled.
*
*
* @param enabled
* True if SAML is enabled.
*/
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
/**
*
* True if SAML is enabled.
*
*
* @return True if SAML is enabled.
*/
public Boolean getEnabled() {
return this.enabled;
}
/**
*
* True if SAML is enabled.
*
*
* @param enabled
* True if SAML is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withEnabled(Boolean enabled) {
setEnabled(enabled);
return this;
}
/**
*
* True if SAML is enabled.
*
*
* @return True if SAML is enabled.
*/
public Boolean isEnabled() {
return this.enabled;
}
/**
*
* Specifies the SAML Identity Provider's information.
*
*
* @param idp
* Specifies the SAML Identity Provider's information.
*/
public void setIdp(SAMLIdp idp) {
this.idp = idp;
}
/**
*
* Specifies the SAML Identity Provider's information.
*
*
* @return Specifies the SAML Identity Provider's information.
*/
public SAMLIdp getIdp() {
return this.idp;
}
/**
*
* Specifies the SAML Identity Provider's information.
*
*
* @param idp
* Specifies the SAML Identity Provider's information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withIdp(SAMLIdp idp) {
setIdp(idp);
return this;
}
/**
*
* The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
*
*
* @param masterUserName
* The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
*/
public void setMasterUserName(String masterUserName) {
this.masterUserName = masterUserName;
}
/**
*
* The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
*
*
* @return The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
*/
public String getMasterUserName() {
return this.masterUserName;
}
/**
*
* The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
*
*
* @param masterUserName
* The SAML master username, which is stored in the Amazon Elasticsearch Service domain's internal database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withMasterUserName(String masterUserName) {
setMasterUserName(masterUserName);
return this;
}
/**
*
* The backend role to which the SAML master user is mapped to.
*
*
* @param masterBackendRole
* The backend role to which the SAML master user is mapped to.
*/
public void setMasterBackendRole(String masterBackendRole) {
this.masterBackendRole = masterBackendRole;
}
/**
*
* The backend role to which the SAML master user is mapped to.
*
*
* @return The backend role to which the SAML master user is mapped to.
*/
public String getMasterBackendRole() {
return this.masterBackendRole;
}
/**
*
* The backend role to which the SAML master user is mapped to.
*
*
* @param masterBackendRole
* The backend role to which the SAML master user is mapped to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withMasterBackendRole(String masterBackendRole) {
setMasterBackendRole(masterBackendRole);
return this;
}
/**
*
* The key to use for matching the SAML Subject attribute.
*
*
* @param subjectKey
* The key to use for matching the SAML Subject attribute.
*/
public void setSubjectKey(String subjectKey) {
this.subjectKey = subjectKey;
}
/**
*
* The key to use for matching the SAML Subject attribute.
*
*
* @return The key to use for matching the SAML Subject attribute.
*/
public String getSubjectKey() {
return this.subjectKey;
}
/**
*
* The key to use for matching the SAML Subject attribute.
*
*
* @param subjectKey
* The key to use for matching the SAML Subject attribute.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withSubjectKey(String subjectKey) {
setSubjectKey(subjectKey);
return this;
}
/**
*
* The key to use for matching the SAML Roles attribute.
*
*
* @param rolesKey
* The key to use for matching the SAML Roles attribute.
*/
public void setRolesKey(String rolesKey) {
this.rolesKey = rolesKey;
}
/**
*
* The key to use for matching the SAML Roles attribute.
*
*
* @return The key to use for matching the SAML Roles attribute.
*/
public String getRolesKey() {
return this.rolesKey;
}
/**
*
* The key to use for matching the SAML Roles attribute.
*
*
* @param rolesKey
* The key to use for matching the SAML Roles attribute.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withRolesKey(String rolesKey) {
setRolesKey(rolesKey);
return this;
}
/**
*
* The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1 and 1440,
* and the default value is 60.
*
*
* @param sessionTimeoutMinutes
* The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1 and
* 1440, and the default value is 60.
*/
public void setSessionTimeoutMinutes(Integer sessionTimeoutMinutes) {
this.sessionTimeoutMinutes = sessionTimeoutMinutes;
}
/**
*
* The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1 and 1440,
* and the default value is 60.
*
*
* @return The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1
* and 1440, and the default value is 60.
*/
public Integer getSessionTimeoutMinutes() {
return this.sessionTimeoutMinutes;
}
/**
*
* The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1 and 1440,
* and the default value is 60.
*
*
* @param sessionTimeoutMinutes
* The duration, in minutes, after which a user session becomes inactive. Acceptable values are between 1 and
* 1440, and the default value is 60.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SAMLOptionsInput withSessionTimeoutMinutes(Integer sessionTimeoutMinutes) {
setSessionTimeoutMinutes(sessionTimeoutMinutes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnabled() != null)
sb.append("Enabled: ").append(getEnabled()).append(",");
if (getIdp() != null)
sb.append("Idp: ").append(getIdp()).append(",");
if (getMasterUserName() != null)
sb.append("MasterUserName: ").append("***Sensitive Data Redacted***").append(",");
if (getMasterBackendRole() != null)
sb.append("MasterBackendRole: ").append(getMasterBackendRole()).append(",");
if (getSubjectKey() != null)
sb.append("SubjectKey: ").append(getSubjectKey()).append(",");
if (getRolesKey() != null)
sb.append("RolesKey: ").append(getRolesKey()).append(",");
if (getSessionTimeoutMinutes() != null)
sb.append("SessionTimeoutMinutes: ").append(getSessionTimeoutMinutes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SAMLOptionsInput == false)
return false;
SAMLOptionsInput other = (SAMLOptionsInput) obj;
if (other.getEnabled() == null ^ this.getEnabled() == null)
return false;
if (other.getEnabled() != null && other.getEnabled().equals(this.getEnabled()) == false)
return false;
if (other.getIdp() == null ^ this.getIdp() == null)
return false;
if (other.getIdp() != null && other.getIdp().equals(this.getIdp()) == false)
return false;
if (other.getMasterUserName() == null ^ this.getMasterUserName() == null)
return false;
if (other.getMasterUserName() != null && other.getMasterUserName().equals(this.getMasterUserName()) == false)
return false;
if (other.getMasterBackendRole() == null ^ this.getMasterBackendRole() == null)
return false;
if (other.getMasterBackendRole() != null && other.getMasterBackendRole().equals(this.getMasterBackendRole()) == false)
return false;
if (other.getSubjectKey() == null ^ this.getSubjectKey() == null)
return false;
if (other.getSubjectKey() != null && other.getSubjectKey().equals(this.getSubjectKey()) == false)
return false;
if (other.getRolesKey() == null ^ this.getRolesKey() == null)
return false;
if (other.getRolesKey() != null && other.getRolesKey().equals(this.getRolesKey()) == false)
return false;
if (other.getSessionTimeoutMinutes() == null ^ this.getSessionTimeoutMinutes() == null)
return false;
if (other.getSessionTimeoutMinutes() != null && other.getSessionTimeoutMinutes().equals(this.getSessionTimeoutMinutes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnabled() == null) ? 0 : getEnabled().hashCode());
hashCode = prime * hashCode + ((getIdp() == null) ? 0 : getIdp().hashCode());
hashCode = prime * hashCode + ((getMasterUserName() == null) ? 0 : getMasterUserName().hashCode());
hashCode = prime * hashCode + ((getMasterBackendRole() == null) ? 0 : getMasterBackendRole().hashCode());
hashCode = prime * hashCode + ((getSubjectKey() == null) ? 0 : getSubjectKey().hashCode());
hashCode = prime * hashCode + ((getRolesKey() == null) ? 0 : getRolesKey().hashCode());
hashCode = prime * hashCode + ((getSessionTimeoutMinutes() == null) ? 0 : getSessionTimeoutMinutes().hashCode());
return hashCode;
}
@Override
public SAMLOptionsInput clone() {
try {
return (SAMLOptionsInput) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.elasticsearch.model.transform.SAMLOptionsInputMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}