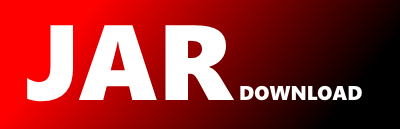
com.amazonaws.services.elasticsearch.AWSElasticsearchClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticsearch Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticsearch;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.elasticsearch.AWSElasticsearchClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.elasticsearch.model.*;
import com.amazonaws.services.elasticsearch.model.transform.*;
/**
* Client for accessing Amazon Elasticsearch Service. All service calls made using this client are blocking, and will
* not return until the service call completes.
*
* Amazon Elasticsearch Configuration Service
*
* Use the Amazon Elasticsearch Configuration API to create, configure, and manage Elasticsearch domains.
*
*
* For sample code that uses the Configuration API, see the Amazon
* Elasticsearch Service Developer Guide. The guide also contains sample code
* for sending signed HTTP requests to the Elasticsearch APIs.
*
*
* The endpoint for configuration service requests is region-specific: es.region.amazonaws.com. For example,
* es.us-east-1.amazonaws.com. For a current list of supported regions and endpoints, see Regions
* and Endpoints.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSElasticsearchClient extends AmazonWebServiceClient implements AWSElasticsearch {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSElasticsearch.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "es";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DisabledOperationException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.DisabledOperationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTypeException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.InvalidTypeExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.ResourceAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.InternalExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidPaginationTokenException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.InvalidPaginationTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BaseException").withExceptionUnmarshaller(
com.amazonaws.services.elasticsearch.model.transform.BaseExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.elasticsearch.model.AWSElasticsearchException.class));
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service. A credentials provider chain
* will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSElasticsearchClientBuilder#defaultClient()}
*/
@Deprecated
public AWSElasticsearchClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service. A credentials provider chain
* will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Elasticsearch Service (ex:
* proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSElasticsearchClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSElasticsearchClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified AWS account
* credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSElasticsearchClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSElasticsearchClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSElasticsearchClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified AWS account
* credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Elasticsearch Service (ex:
* proxy settings, retry counts, etc.).
* @deprecated use {@link AWSElasticsearchClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSElasticsearchClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSElasticsearchClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified AWS account
* credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSElasticsearchClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSElasticsearchClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified AWS account
* credentials provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Elasticsearch Service (ex:
* proxy settings, retry counts, etc.).
* @deprecated use {@link AWSElasticsearchClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSElasticsearchClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSElasticsearchClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified AWS account
* credentials provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Elasticsearch Service (ex:
* proxy settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSElasticsearchClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSElasticsearchClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSElasticsearchClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSElasticsearchClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AWSElasticsearchClientBuilder builder() {
return AWSElasticsearchClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSElasticsearchClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Elasticsearch Service using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSElasticsearchClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("https://es.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/elasticsearch/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/elasticsearch/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Allows the destination domain owner to accept an inbound cross-cluster search connection request.
*
*
* @param acceptInboundCrossClusterSearchConnectionRequest
* Container for the parameters to the AcceptInboundCrossClusterSearchConnection
* operation.
* @return Result of the AcceptInboundCrossClusterSearchConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.AcceptInboundCrossClusterSearchConnection
*/
@Override
public AcceptInboundCrossClusterSearchConnectionResult acceptInboundCrossClusterSearchConnection(AcceptInboundCrossClusterSearchConnectionRequest request) {
request = beforeClientExecution(request);
return executeAcceptInboundCrossClusterSearchConnection(request);
}
@SdkInternalApi
final AcceptInboundCrossClusterSearchConnectionResult executeAcceptInboundCrossClusterSearchConnection(
AcceptInboundCrossClusterSearchConnectionRequest acceptInboundCrossClusterSearchConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(acceptInboundCrossClusterSearchConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptInboundCrossClusterSearchConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(acceptInboundCrossClusterSearchConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptInboundCrossClusterSearchConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AcceptInboundCrossClusterSearchConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches tags to an existing Elasticsearch domain. Tags are a set of case-sensitive key value pairs. An
* Elasticsearch domain may have up to 10 tags. See Tagging Amazon Elasticsearch Service Domains for more information.
*
*
* @param addTagsRequest
* Container for the parameters to the AddTags
operation. Specify the tags that you want
* to attach to the Elasticsearch domain.
* @return Result of the AddTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.AddTags
*/
@Override
public AddTagsResult addTags(AddTagsRequest request) {
request = beforeClientExecution(request);
return executeAddTags(request);
}
@SdkInternalApi
final AddTagsResult executeAddTags(AddTagsRequest addTagsRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(addTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new AddTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a package with an Amazon ES domain.
*
*
* @param associatePackageRequest
* Container for request parameters to AssociatePackage
operation.
* @return Result of the AssociatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use. Returns HTTP
* status code 409.
* @sample AWSElasticsearch.AssociatePackage
*/
@Override
public AssociatePackageResult associatePackage(AssociatePackageRequest request) {
request = beforeClientExecution(request);
return executeAssociatePackage(request);
}
@SdkInternalApi
final AssociatePackageResult executeAssociatePackage(AssociatePackageRequest associatePackageRequest) {
ExecutionContext executionContext = createExecutionContext(associatePackageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePackageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associatePackageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePackage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociatePackageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provides access to an Amazon OpenSearch Service domain through the use of an interface VPC endpoint.
*
*
* @param authorizeVpcEndpointAccessRequest
* Container for request parameters to the AuthorizeVpcEndpointAccess
operation.
* Specifies the account to be permitted to manage VPC endpoints against the domain.
* @return Result of the AuthorizeVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.AuthorizeVpcEndpointAccess
*/
@Override
public AuthorizeVpcEndpointAccessResult authorizeVpcEndpointAccess(AuthorizeVpcEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeAuthorizeVpcEndpointAccess(request);
}
@SdkInternalApi
final AuthorizeVpcEndpointAccessResult executeAuthorizeVpcEndpointAccess(AuthorizeVpcEndpointAccessRequest authorizeVpcEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeVpcEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AuthorizeVpcEndpointAccessRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(authorizeVpcEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AuthorizeVpcEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AuthorizeVpcEndpointAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels a pending configuration change on an Amazon OpenSearch Service domain.
*
*
* @param cancelDomainConfigChangeRequest
* Container for parameters of the CancelDomainConfigChange
operation.
* @return Result of the CancelDomainConfigChange operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.CancelDomainConfigChange
*/
@Override
public CancelDomainConfigChangeResult cancelDomainConfigChange(CancelDomainConfigChangeRequest request) {
request = beforeClientExecution(request);
return executeCancelDomainConfigChange(request);
}
@SdkInternalApi
final CancelDomainConfigChangeResult executeCancelDomainConfigChange(CancelDomainConfigChangeRequest cancelDomainConfigChangeRequest) {
ExecutionContext executionContext = createExecutionContext(cancelDomainConfigChangeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelDomainConfigChangeRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(cancelDomainConfigChangeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelDomainConfigChange");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelDomainConfigChangeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels a scheduled service software update for an Amazon ES domain. You can only perform this operation before
* the AutomatedUpdateDate
and when the UpdateStatus
is in the PENDING_UPDATE
* state.
*
*
* @param cancelElasticsearchServiceSoftwareUpdateRequest
* Container for the parameters to the CancelElasticsearchServiceSoftwareUpdate
* operation. Specifies the name of the Elasticsearch domain that you wish to cancel a service software
* update on.
* @return Result of the CancelElasticsearchServiceSoftwareUpdate operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.CancelElasticsearchServiceSoftwareUpdate
*/
@Override
public CancelElasticsearchServiceSoftwareUpdateResult cancelElasticsearchServiceSoftwareUpdate(CancelElasticsearchServiceSoftwareUpdateRequest request) {
request = beforeClientExecution(request);
return executeCancelElasticsearchServiceSoftwareUpdate(request);
}
@SdkInternalApi
final CancelElasticsearchServiceSoftwareUpdateResult executeCancelElasticsearchServiceSoftwareUpdate(
CancelElasticsearchServiceSoftwareUpdateRequest cancelElasticsearchServiceSoftwareUpdateRequest) {
ExecutionContext executionContext = createExecutionContext(cancelElasticsearchServiceSoftwareUpdateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelElasticsearchServiceSoftwareUpdateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(cancelElasticsearchServiceSoftwareUpdateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelElasticsearchServiceSoftwareUpdate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelElasticsearchServiceSoftwareUpdateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Elasticsearch domain. For more information, see Creating Elasticsearch Domains in the Amazon Elasticsearch Service Developer Guide.
*
*
* @param createElasticsearchDomainRequest
* @return Result of the CreateElasticsearchDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws InvalidTypeException
* An exception for trying to create or access sub-resource that is either invalid or not supported. Gives
* http status code of 409.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.CreateElasticsearchDomain
*/
@Override
public CreateElasticsearchDomainResult createElasticsearchDomain(CreateElasticsearchDomainRequest request) {
request = beforeClientExecution(request);
return executeCreateElasticsearchDomain(request);
}
@SdkInternalApi
final CreateElasticsearchDomainResult executeCreateElasticsearchDomain(CreateElasticsearchDomainRequest createElasticsearchDomainRequest) {
ExecutionContext executionContext = createExecutionContext(createElasticsearchDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateElasticsearchDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createElasticsearchDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateElasticsearchDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateElasticsearchDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new cross-cluster search connection from a source domain to a destination domain.
*
*
* @param createOutboundCrossClusterSearchConnectionRequest
* Container for the parameters to the CreateOutboundCrossClusterSearchConnection
* operation.
* @return Result of the CreateOutboundCrossClusterSearchConnection operation returned by the service.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.CreateOutboundCrossClusterSearchConnection
*/
@Override
public CreateOutboundCrossClusterSearchConnectionResult createOutboundCrossClusterSearchConnection(CreateOutboundCrossClusterSearchConnectionRequest request) {
request = beforeClientExecution(request);
return executeCreateOutboundCrossClusterSearchConnection(request);
}
@SdkInternalApi
final CreateOutboundCrossClusterSearchConnectionResult executeCreateOutboundCrossClusterSearchConnection(
CreateOutboundCrossClusterSearchConnectionRequest createOutboundCrossClusterSearchConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(createOutboundCrossClusterSearchConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateOutboundCrossClusterSearchConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createOutboundCrossClusterSearchConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateOutboundCrossClusterSearchConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateOutboundCrossClusterSearchConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a package for use with Amazon ES domains.
*
*
* @param createPackageRequest
* Container for request parameters to CreatePackage
operation.
* @return Result of the CreatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws InvalidTypeException
* An exception for trying to create or access sub-resource that is either invalid or not supported. Gives
* http status code of 409.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.CreatePackage
*/
@Override
public CreatePackageResult createPackage(CreatePackageRequest request) {
request = beforeClientExecution(request);
return executeCreatePackage(request);
}
@SdkInternalApi
final CreatePackageResult executeCreatePackage(CreatePackageRequest createPackageRequest) {
ExecutionContext executionContext = createExecutionContext(createPackageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePackageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createPackageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePackage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreatePackageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon OpenSearch Service-managed VPC endpoint.
*
*
* @param createVpcEndpointRequest
* Container for the parameters to the CreateVpcEndpointRequest
operation.
* @return Result of the CreateVpcEndpoint operation returned by the service.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use. Returns HTTP
* status code 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.CreateVpcEndpoint
*/
@Override
public CreateVpcEndpointResult createVpcEndpoint(CreateVpcEndpointRequest request) {
request = beforeClientExecution(request);
return executeCreateVpcEndpoint(request);
}
@SdkInternalApi
final CreateVpcEndpointResult executeCreateVpcEndpoint(CreateVpcEndpointRequest createVpcEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(createVpcEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVpcEndpointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVpcEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVpcEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVpcEndpointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes the specified Elasticsearch domain and all of its data. Once a domain is deleted, it cannot
* be recovered.
*
*
* @param deleteElasticsearchDomainRequest
* Container for the parameters to the DeleteElasticsearchDomain
operation. Specifies the
* name of the Elasticsearch domain that you want to delete.
* @return Result of the DeleteElasticsearchDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DeleteElasticsearchDomain
*/
@Override
public DeleteElasticsearchDomainResult deleteElasticsearchDomain(DeleteElasticsearchDomainRequest request) {
request = beforeClientExecution(request);
return executeDeleteElasticsearchDomain(request);
}
@SdkInternalApi
final DeleteElasticsearchDomainResult executeDeleteElasticsearchDomain(DeleteElasticsearchDomainRequest deleteElasticsearchDomainRequest) {
ExecutionContext executionContext = createExecutionContext(deleteElasticsearchDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteElasticsearchDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteElasticsearchDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteElasticsearchDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteElasticsearchDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the service-linked role that Elasticsearch Service uses to manage and maintain VPC domains. Role deletion
* will fail if any existing VPC domains use the role. You must delete any such Elasticsearch domains before
* deleting the role. See Deleting Elasticsearch Service Role in VPC Endpoints for Amazon Elasticsearch Service
* Domains.
*
*
* @param deleteElasticsearchServiceRoleRequest
* @return Result of the DeleteElasticsearchServiceRole operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DeleteElasticsearchServiceRole
*/
@Override
public DeleteElasticsearchServiceRoleResult deleteElasticsearchServiceRole(DeleteElasticsearchServiceRoleRequest request) {
request = beforeClientExecution(request);
return executeDeleteElasticsearchServiceRole(request);
}
@SdkInternalApi
final DeleteElasticsearchServiceRoleResult executeDeleteElasticsearchServiceRole(DeleteElasticsearchServiceRoleRequest deleteElasticsearchServiceRoleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteElasticsearchServiceRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteElasticsearchServiceRoleRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteElasticsearchServiceRoleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteElasticsearchServiceRole");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteElasticsearchServiceRoleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows the destination domain owner to delete an existing inbound cross-cluster search connection.
*
*
* @param deleteInboundCrossClusterSearchConnectionRequest
* Container for the parameters to the DeleteInboundCrossClusterSearchConnection
* operation.
* @return Result of the DeleteInboundCrossClusterSearchConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.DeleteInboundCrossClusterSearchConnection
*/
@Override
public DeleteInboundCrossClusterSearchConnectionResult deleteInboundCrossClusterSearchConnection(DeleteInboundCrossClusterSearchConnectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteInboundCrossClusterSearchConnection(request);
}
@SdkInternalApi
final DeleteInboundCrossClusterSearchConnectionResult executeDeleteInboundCrossClusterSearchConnection(
DeleteInboundCrossClusterSearchConnectionRequest deleteInboundCrossClusterSearchConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInboundCrossClusterSearchConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteInboundCrossClusterSearchConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteInboundCrossClusterSearchConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteInboundCrossClusterSearchConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteInboundCrossClusterSearchConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows the source domain owner to delete an existing outbound cross-cluster search connection.
*
*
* @param deleteOutboundCrossClusterSearchConnectionRequest
* Container for the parameters to the DeleteOutboundCrossClusterSearchConnection
* operation.
* @return Result of the DeleteOutboundCrossClusterSearchConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.DeleteOutboundCrossClusterSearchConnection
*/
@Override
public DeleteOutboundCrossClusterSearchConnectionResult deleteOutboundCrossClusterSearchConnection(DeleteOutboundCrossClusterSearchConnectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteOutboundCrossClusterSearchConnection(request);
}
@SdkInternalApi
final DeleteOutboundCrossClusterSearchConnectionResult executeDeleteOutboundCrossClusterSearchConnection(
DeleteOutboundCrossClusterSearchConnectionRequest deleteOutboundCrossClusterSearchConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteOutboundCrossClusterSearchConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteOutboundCrossClusterSearchConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteOutboundCrossClusterSearchConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteOutboundCrossClusterSearchConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteOutboundCrossClusterSearchConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete the package.
*
*
* @param deletePackageRequest
* Container for request parameters to DeletePackage
operation.
* @return Result of the DeletePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use. Returns HTTP
* status code 409.
* @sample AWSElasticsearch.DeletePackage
*/
@Override
public DeletePackageResult deletePackage(DeletePackageRequest request) {
request = beforeClientExecution(request);
return executeDeletePackage(request);
}
@SdkInternalApi
final DeletePackageResult executeDeletePackage(DeletePackageRequest deletePackageRequest) {
ExecutionContext executionContext = createExecutionContext(deletePackageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePackageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePackageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePackage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeletePackageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
* @param deleteVpcEndpointRequest
* Deletes an Amazon OpenSearch Service-managed interface VPC endpoint.
* @return Result of the DeleteVpcEndpoint operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.DeleteVpcEndpoint
*/
@Override
public DeleteVpcEndpointResult deleteVpcEndpoint(DeleteVpcEndpointRequest request) {
request = beforeClientExecution(request);
return executeDeleteVpcEndpoint(request);
}
@SdkInternalApi
final DeleteVpcEndpointResult executeDeleteVpcEndpoint(DeleteVpcEndpointRequest deleteVpcEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpcEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVpcEndpointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVpcEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVpcEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVpcEndpointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provides scheduled Auto-Tune action details for the Elasticsearch domain, such as Auto-Tune action type,
* description, severity, and scheduled date.
*
*
* @param describeDomainAutoTunesRequest
* Container for the parameters to the DescribeDomainAutoTunes
operation.
* @return Result of the DescribeDomainAutoTunes operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribeDomainAutoTunes
*/
@Override
public DescribeDomainAutoTunesResult describeDomainAutoTunes(DescribeDomainAutoTunesRequest request) {
request = beforeClientExecution(request);
return executeDescribeDomainAutoTunes(request);
}
@SdkInternalApi
final DescribeDomainAutoTunesResult executeDescribeDomainAutoTunes(DescribeDomainAutoTunesRequest describeDomainAutoTunesRequest) {
ExecutionContext executionContext = createExecutionContext(describeDomainAutoTunesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDomainAutoTunesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDomainAutoTunesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDomainAutoTunes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDomainAutoTunesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the current blue/green deployment happening on a domain, including a change ID, status,
* and progress stages.
*
*
* @param describeDomainChangeProgressRequest
* Container for the parameters to the DescribeDomainChangeProgress
operation. Specifies the
* domain name and optional change specific identity for which you want progress information.
* @return Result of the DescribeDomainChangeProgress operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribeDomainChangeProgress
*/
@Override
public DescribeDomainChangeProgressResult describeDomainChangeProgress(DescribeDomainChangeProgressRequest request) {
request = beforeClientExecution(request);
return executeDescribeDomainChangeProgress(request);
}
@SdkInternalApi
final DescribeDomainChangeProgressResult executeDescribeDomainChangeProgress(DescribeDomainChangeProgressRequest describeDomainChangeProgressRequest) {
ExecutionContext executionContext = createExecutionContext(describeDomainChangeProgressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDomainChangeProgressRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDomainChangeProgressRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDomainChangeProgress");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDomainChangeProgressResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns domain configuration information about the specified Elasticsearch domain, including the domain ID,
* domain endpoint, and domain ARN.
*
*
* @param describeElasticsearchDomainRequest
* Container for the parameters to the DescribeElasticsearchDomain
operation.
* @return Result of the DescribeElasticsearchDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchDomain
*/
@Override
public DescribeElasticsearchDomainResult describeElasticsearchDomain(DescribeElasticsearchDomainRequest request) {
request = beforeClientExecution(request);
return executeDescribeElasticsearchDomain(request);
}
@SdkInternalApi
final DescribeElasticsearchDomainResult executeDescribeElasticsearchDomain(DescribeElasticsearchDomainRequest describeElasticsearchDomainRequest) {
ExecutionContext executionContext = createExecutionContext(describeElasticsearchDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeElasticsearchDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeElasticsearchDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeElasticsearchDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeElasticsearchDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provides cluster configuration information about the specified Elasticsearch domain, such as the state, creation
* date, update version, and update date for cluster options.
*
*
* @param describeElasticsearchDomainConfigRequest
* Container for the parameters to the DescribeElasticsearchDomainConfig
operation. Specifies
* the domain name for which you want configuration information.
* @return Result of the DescribeElasticsearchDomainConfig operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchDomainConfig
*/
@Override
public DescribeElasticsearchDomainConfigResult describeElasticsearchDomainConfig(DescribeElasticsearchDomainConfigRequest request) {
request = beforeClientExecution(request);
return executeDescribeElasticsearchDomainConfig(request);
}
@SdkInternalApi
final DescribeElasticsearchDomainConfigResult executeDescribeElasticsearchDomainConfig(
DescribeElasticsearchDomainConfigRequest describeElasticsearchDomainConfigRequest) {
ExecutionContext executionContext = createExecutionContext(describeElasticsearchDomainConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeElasticsearchDomainConfigRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeElasticsearchDomainConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeElasticsearchDomainConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeElasticsearchDomainConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns domain configuration information about the specified Elasticsearch domains, including the domain ID,
* domain endpoint, and domain ARN.
*
*
* @param describeElasticsearchDomainsRequest
* Container for the parameters to the DescribeElasticsearchDomains
operation. By
* default, the API returns the status of all Elasticsearch domains.
* @return Result of the DescribeElasticsearchDomains operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchDomains
*/
@Override
public DescribeElasticsearchDomainsResult describeElasticsearchDomains(DescribeElasticsearchDomainsRequest request) {
request = beforeClientExecution(request);
return executeDescribeElasticsearchDomains(request);
}
@SdkInternalApi
final DescribeElasticsearchDomainsResult executeDescribeElasticsearchDomains(DescribeElasticsearchDomainsRequest describeElasticsearchDomainsRequest) {
ExecutionContext executionContext = createExecutionContext(describeElasticsearchDomainsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeElasticsearchDomainsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeElasticsearchDomainsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeElasticsearchDomains");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeElasticsearchDomainsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describe Elasticsearch Limits for a given InstanceType and ElasticsearchVersion. When modifying existing Domain,
* specify the DomainName
to know what Limits are supported for modifying.
*
*
* @param describeElasticsearchInstanceTypeLimitsRequest
* Container for the parameters to DescribeElasticsearchInstanceTypeLimits
operation.
* @return Result of the DescribeElasticsearchInstanceTypeLimits operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws InvalidTypeException
* An exception for trying to create or access sub-resource that is either invalid or not supported. Gives
* http status code of 409.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribeElasticsearchInstanceTypeLimits
*/
@Override
public DescribeElasticsearchInstanceTypeLimitsResult describeElasticsearchInstanceTypeLimits(DescribeElasticsearchInstanceTypeLimitsRequest request) {
request = beforeClientExecution(request);
return executeDescribeElasticsearchInstanceTypeLimits(request);
}
@SdkInternalApi
final DescribeElasticsearchInstanceTypeLimitsResult executeDescribeElasticsearchInstanceTypeLimits(
DescribeElasticsearchInstanceTypeLimitsRequest describeElasticsearchInstanceTypeLimitsRequest) {
ExecutionContext executionContext = createExecutionContext(describeElasticsearchInstanceTypeLimitsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeElasticsearchInstanceTypeLimitsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeElasticsearchInstanceTypeLimitsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeElasticsearchInstanceTypeLimits");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeElasticsearchInstanceTypeLimitsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the inbound cross-cluster search connections for a destination domain.
*
*
* @param describeInboundCrossClusterSearchConnectionsRequest
* Container for the parameters to the DescribeInboundCrossClusterSearchConnections
* operation.
* @return Result of the DescribeInboundCrossClusterSearchConnections operation returned by the service.
* @throws InvalidPaginationTokenException
* The request processing has failed because of invalid pagination token provided by customer. Returns an
* HTTP status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.DescribeInboundCrossClusterSearchConnections
*/
@Override
public DescribeInboundCrossClusterSearchConnectionsResult describeInboundCrossClusterSearchConnections(
DescribeInboundCrossClusterSearchConnectionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeInboundCrossClusterSearchConnections(request);
}
@SdkInternalApi
final DescribeInboundCrossClusterSearchConnectionsResult executeDescribeInboundCrossClusterSearchConnections(
DescribeInboundCrossClusterSearchConnectionsRequest describeInboundCrossClusterSearchConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeInboundCrossClusterSearchConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeInboundCrossClusterSearchConnectionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeInboundCrossClusterSearchConnectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeInboundCrossClusterSearchConnections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeInboundCrossClusterSearchConnectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the outbound cross-cluster search connections for a source domain.
*
*
* @param describeOutboundCrossClusterSearchConnectionsRequest
* Container for the parameters to the DescribeOutboundCrossClusterSearchConnections
* operation.
* @return Result of the DescribeOutboundCrossClusterSearchConnections operation returned by the service.
* @throws InvalidPaginationTokenException
* The request processing has failed because of invalid pagination token provided by customer. Returns an
* HTTP status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.DescribeOutboundCrossClusterSearchConnections
*/
@Override
public DescribeOutboundCrossClusterSearchConnectionsResult describeOutboundCrossClusterSearchConnections(
DescribeOutboundCrossClusterSearchConnectionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeOutboundCrossClusterSearchConnections(request);
}
@SdkInternalApi
final DescribeOutboundCrossClusterSearchConnectionsResult executeDescribeOutboundCrossClusterSearchConnections(
DescribeOutboundCrossClusterSearchConnectionsRequest describeOutboundCrossClusterSearchConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeOutboundCrossClusterSearchConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeOutboundCrossClusterSearchConnectionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeOutboundCrossClusterSearchConnectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeOutboundCrossClusterSearchConnections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeOutboundCrossClusterSearchConnectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes all packages available to Amazon ES. Includes options for filtering, limiting the number of results,
* and pagination.
*
*
* @param describePackagesRequest
* Container for request parameters to DescribePackage
operation.
* @return Result of the DescribePackages operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.DescribePackages
*/
@Override
public DescribePackagesResult describePackages(DescribePackagesRequest request) {
request = beforeClientExecution(request);
return executeDescribePackages(request);
}
@SdkInternalApi
final DescribePackagesResult executeDescribePackages(DescribePackagesRequest describePackagesRequest) {
ExecutionContext executionContext = createExecutionContext(describePackagesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePackagesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describePackagesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePackages");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribePackagesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists available reserved Elasticsearch instance offerings.
*
*
* @param describeReservedElasticsearchInstanceOfferingsRequest
* Container for parameters to DescribeReservedElasticsearchInstanceOfferings
* @return Result of the DescribeReservedElasticsearchInstanceOfferings operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.DescribeReservedElasticsearchInstanceOfferings
*/
@Override
public DescribeReservedElasticsearchInstanceOfferingsResult describeReservedElasticsearchInstanceOfferings(
DescribeReservedElasticsearchInstanceOfferingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeReservedElasticsearchInstanceOfferings(request);
}
@SdkInternalApi
final DescribeReservedElasticsearchInstanceOfferingsResult executeDescribeReservedElasticsearchInstanceOfferings(
DescribeReservedElasticsearchInstanceOfferingsRequest describeReservedElasticsearchInstanceOfferingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedElasticsearchInstanceOfferingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReservedElasticsearchInstanceOfferingsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeReservedElasticsearchInstanceOfferingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReservedElasticsearchInstanceOfferings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeReservedElasticsearchInstanceOfferingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about reserved Elasticsearch instances for this account.
*
*
* @param describeReservedElasticsearchInstancesRequest
* Container for parameters to DescribeReservedElasticsearchInstances
* @return Result of the DescribeReservedElasticsearchInstances operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.DescribeReservedElasticsearchInstances
*/
@Override
public DescribeReservedElasticsearchInstancesResult describeReservedElasticsearchInstances(DescribeReservedElasticsearchInstancesRequest request) {
request = beforeClientExecution(request);
return executeDescribeReservedElasticsearchInstances(request);
}
@SdkInternalApi
final DescribeReservedElasticsearchInstancesResult executeDescribeReservedElasticsearchInstances(
DescribeReservedElasticsearchInstancesRequest describeReservedElasticsearchInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedElasticsearchInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReservedElasticsearchInstancesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeReservedElasticsearchInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReservedElasticsearchInstances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeReservedElasticsearchInstancesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more Amazon OpenSearch Service-managed VPC endpoints.
*
*
* @param describeVpcEndpointsRequest
* Container for request parameters to the DescribeVpcEndpoints
operation. Specifies the
* list of VPC endpoints to be described.
* @return Result of the DescribeVpcEndpoints operation returned by the service.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.DescribeVpcEndpoints
*/
@Override
public DescribeVpcEndpointsResult describeVpcEndpoints(DescribeVpcEndpointsRequest request) {
request = beforeClientExecution(request);
return executeDescribeVpcEndpoints(request);
}
@SdkInternalApi
final DescribeVpcEndpointsResult executeDescribeVpcEndpoints(DescribeVpcEndpointsRequest describeVpcEndpointsRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpcEndpointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVpcEndpointsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeVpcEndpointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVpcEndpoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeVpcEndpointsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Dissociates a package from the Amazon ES domain.
*
*
* @param dissociatePackageRequest
* Container for request parameters to DissociatePackage
operation.
* @return Result of the DissociatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use. Returns HTTP
* status code 409.
* @sample AWSElasticsearch.DissociatePackage
*/
@Override
public DissociatePackageResult dissociatePackage(DissociatePackageRequest request) {
request = beforeClientExecution(request);
return executeDissociatePackage(request);
}
@SdkInternalApi
final DissociatePackageResult executeDissociatePackage(DissociatePackageRequest dissociatePackageRequest) {
ExecutionContext executionContext = createExecutionContext(dissociatePackageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DissociatePackageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(dissociatePackageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DissociatePackage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DissociatePackageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of upgrade compatible Elastisearch versions. You can optionally pass a
* DomainName
to get all upgrade compatible Elasticsearch versions for that specific domain.
*
*
* @param getCompatibleElasticsearchVersionsRequest
* Container for request parameters to GetCompatibleElasticsearchVersions
operation.
* @return Result of the GetCompatibleElasticsearchVersions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.GetCompatibleElasticsearchVersions
*/
@Override
public GetCompatibleElasticsearchVersionsResult getCompatibleElasticsearchVersions(GetCompatibleElasticsearchVersionsRequest request) {
request = beforeClientExecution(request);
return executeGetCompatibleElasticsearchVersions(request);
}
@SdkInternalApi
final GetCompatibleElasticsearchVersionsResult executeGetCompatibleElasticsearchVersions(
GetCompatibleElasticsearchVersionsRequest getCompatibleElasticsearchVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(getCompatibleElasticsearchVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCompatibleElasticsearchVersionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getCompatibleElasticsearchVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCompatibleElasticsearchVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCompatibleElasticsearchVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of versions of the package, along with their creation time and commit message.
*
*
* @param getPackageVersionHistoryRequest
* Container for request parameters to GetPackageVersionHistory
operation.
* @return Result of the GetPackageVersionHistory operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.GetPackageVersionHistory
*/
@Override
public GetPackageVersionHistoryResult getPackageVersionHistory(GetPackageVersionHistoryRequest request) {
request = beforeClientExecution(request);
return executeGetPackageVersionHistory(request);
}
@SdkInternalApi
final GetPackageVersionHistoryResult executeGetPackageVersionHistory(GetPackageVersionHistoryRequest getPackageVersionHistoryRequest) {
ExecutionContext executionContext = createExecutionContext(getPackageVersionHistoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPackageVersionHistoryRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getPackageVersionHistoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPackageVersionHistory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetPackageVersionHistoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the complete history of the last 10 upgrades that were performed on the domain.
*
*
* @param getUpgradeHistoryRequest
* Container for request parameters to GetUpgradeHistory
operation.
* @return Result of the GetUpgradeHistory operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.GetUpgradeHistory
*/
@Override
public GetUpgradeHistoryResult getUpgradeHistory(GetUpgradeHistoryRequest request) {
request = beforeClientExecution(request);
return executeGetUpgradeHistory(request);
}
@SdkInternalApi
final GetUpgradeHistoryResult executeGetUpgradeHistory(GetUpgradeHistoryRequest getUpgradeHistoryRequest) {
ExecutionContext executionContext = createExecutionContext(getUpgradeHistoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetUpgradeHistoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getUpgradeHistoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetUpgradeHistory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetUpgradeHistoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the latest status of the last upgrade or upgrade eligibility check that was performed on the domain.
*
*
* @param getUpgradeStatusRequest
* Container for request parameters to GetUpgradeStatus
operation.
* @return Result of the GetUpgradeStatus operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.GetUpgradeStatus
*/
@Override
public GetUpgradeStatusResult getUpgradeStatus(GetUpgradeStatusRequest request) {
request = beforeClientExecution(request);
return executeGetUpgradeStatus(request);
}
@SdkInternalApi
final GetUpgradeStatusResult executeGetUpgradeStatus(GetUpgradeStatusRequest getUpgradeStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getUpgradeStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetUpgradeStatusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getUpgradeStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetUpgradeStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetUpgradeStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the name of all Elasticsearch domains owned by the current user's account.
*
*
* @param listDomainNamesRequest
* Container for the parameters to the ListDomainNames
operation.
* @return Result of the ListDomainNames operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.ListDomainNames
*/
@Override
public ListDomainNamesResult listDomainNames(ListDomainNamesRequest request) {
request = beforeClientExecution(request);
return executeListDomainNames(request);
}
@SdkInternalApi
final ListDomainNamesResult executeListDomainNames(ListDomainNamesRequest listDomainNamesRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainNamesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDomainNamesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDomainNamesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDomainNames");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDomainNamesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all Amazon ES domains associated with the package.
*
*
* @param listDomainsForPackageRequest
* Container for request parameters to ListDomainsForPackage
operation.
* @return Result of the ListDomainsForPackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.ListDomainsForPackage
*/
@Override
public ListDomainsForPackageResult listDomainsForPackage(ListDomainsForPackageRequest request) {
request = beforeClientExecution(request);
return executeListDomainsForPackage(request);
}
@SdkInternalApi
final ListDomainsForPackageResult executeListDomainsForPackage(ListDomainsForPackageRequest listDomainsForPackageRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainsForPackageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDomainsForPackageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDomainsForPackageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDomainsForPackage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDomainsForPackageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all Elasticsearch instance types that are supported for given ElasticsearchVersion
*
*
* @param listElasticsearchInstanceTypesRequest
* Container for the parameters to the ListElasticsearchInstanceTypes
operation.
* @return Result of the ListElasticsearchInstanceTypes operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.ListElasticsearchInstanceTypes
*/
@Override
public ListElasticsearchInstanceTypesResult listElasticsearchInstanceTypes(ListElasticsearchInstanceTypesRequest request) {
request = beforeClientExecution(request);
return executeListElasticsearchInstanceTypes(request);
}
@SdkInternalApi
final ListElasticsearchInstanceTypesResult executeListElasticsearchInstanceTypes(ListElasticsearchInstanceTypesRequest listElasticsearchInstanceTypesRequest) {
ExecutionContext executionContext = createExecutionContext(listElasticsearchInstanceTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListElasticsearchInstanceTypesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listElasticsearchInstanceTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListElasticsearchInstanceTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListElasticsearchInstanceTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all supported Elasticsearch versions
*
*
* @param listElasticsearchVersionsRequest
* Container for the parameters to the ListElasticsearchVersions
operation.
*
* Use MaxResults
to control the maximum number of results to retrieve in a single
* call.
*
*
* Use NextToken
in response to retrieve more results. If the received response does
* not contain a NextToken, then there are no more results to retrieve.
*
* @return Result of the ListElasticsearchVersions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.ListElasticsearchVersions
*/
@Override
public ListElasticsearchVersionsResult listElasticsearchVersions(ListElasticsearchVersionsRequest request) {
request = beforeClientExecution(request);
return executeListElasticsearchVersions(request);
}
@SdkInternalApi
final ListElasticsearchVersionsResult executeListElasticsearchVersions(ListElasticsearchVersionsRequest listElasticsearchVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listElasticsearchVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListElasticsearchVersionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listElasticsearchVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListElasticsearchVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListElasticsearchVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all packages associated with the Amazon ES domain.
*
*
* @param listPackagesForDomainRequest
* Container for request parameters to ListPackagesForDomain
operation.
* @return Result of the ListPackagesForDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.ListPackagesForDomain
*/
@Override
public ListPackagesForDomainResult listPackagesForDomain(ListPackagesForDomainRequest request) {
request = beforeClientExecution(request);
return executeListPackagesForDomain(request);
}
@SdkInternalApi
final ListPackagesForDomainResult executeListPackagesForDomain(ListPackagesForDomainRequest listPackagesForDomainRequest) {
ExecutionContext executionContext = createExecutionContext(listPackagesForDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPackagesForDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPackagesForDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPackagesForDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPackagesForDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns all tags for the given Elasticsearch domain.
*
*
* @param listTagsRequest
* Container for the parameters to the ListTags
operation. Specify the ARN
* for the Elasticsearch domain to which the tags are attached that you want to view are attached.
* @return Result of the ListTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.ListTags
*/
@Override
public ListTagsResult listTags(ListTagsRequest request) {
request = beforeClientExecution(request);
return executeListTags(request);
}
@SdkInternalApi
final ListTagsResult executeListTags(ListTagsRequest listTagsRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about each principal that is allowed to access a given Amazon OpenSearch Service domain
* through the use of an interface VPC endpoint.
*
*
* @param listVpcEndpointAccessRequest
* Retrieves information about each principal that is allowed to access a given Amazon OpenSearch Service
* domain through the use of an interface VPC endpoint
* @return Result of the ListVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.ListVpcEndpointAccess
*/
@Override
public ListVpcEndpointAccessResult listVpcEndpointAccess(ListVpcEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeListVpcEndpointAccess(request);
}
@SdkInternalApi
final ListVpcEndpointAccessResult executeListVpcEndpointAccess(ListVpcEndpointAccessRequest listVpcEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(listVpcEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVpcEndpointAccessRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVpcEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVpcEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListVpcEndpointAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves all Amazon OpenSearch Service-managed VPC endpoints in the current account and Region.
*
*
* @param listVpcEndpointsRequest
* Container for request parameters to the ListVpcEndpoints
operation.
* @return Result of the ListVpcEndpoints operation returned by the service.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.ListVpcEndpoints
*/
@Override
public ListVpcEndpointsResult listVpcEndpoints(ListVpcEndpointsRequest request) {
request = beforeClientExecution(request);
return executeListVpcEndpoints(request);
}
@SdkInternalApi
final ListVpcEndpointsResult executeListVpcEndpoints(ListVpcEndpointsRequest listVpcEndpointsRequest) {
ExecutionContext executionContext = createExecutionContext(listVpcEndpointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVpcEndpointsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVpcEndpointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVpcEndpoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVpcEndpointsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves all Amazon OpenSearch Service-managed VPC endpoints associated with a particular domain.
*
*
* @param listVpcEndpointsForDomainRequest
* Container for request parameters to the ListVpcEndpointsForDomain
operation. Specifies
* the domain whose VPC endpoints will be listed.
* @return Result of the ListVpcEndpointsForDomain operation returned by the service.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.ListVpcEndpointsForDomain
*/
@Override
public ListVpcEndpointsForDomainResult listVpcEndpointsForDomain(ListVpcEndpointsForDomainRequest request) {
request = beforeClientExecution(request);
return executeListVpcEndpointsForDomain(request);
}
@SdkInternalApi
final ListVpcEndpointsForDomainResult executeListVpcEndpointsForDomain(ListVpcEndpointsForDomainRequest listVpcEndpointsForDomainRequest) {
ExecutionContext executionContext = createExecutionContext(listVpcEndpointsForDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVpcEndpointsForDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listVpcEndpointsForDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVpcEndpointsForDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListVpcEndpointsForDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows you to purchase reserved Elasticsearch instances.
*
*
* @param purchaseReservedElasticsearchInstanceOfferingRequest
* Container for parameters to PurchaseReservedElasticsearchInstanceOffering
* @return Result of the PurchaseReservedElasticsearchInstanceOffering operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists. Gives http status code of 400.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.PurchaseReservedElasticsearchInstanceOffering
*/
@Override
public PurchaseReservedElasticsearchInstanceOfferingResult purchaseReservedElasticsearchInstanceOffering(
PurchaseReservedElasticsearchInstanceOfferingRequest request) {
request = beforeClientExecution(request);
return executePurchaseReservedElasticsearchInstanceOffering(request);
}
@SdkInternalApi
final PurchaseReservedElasticsearchInstanceOfferingResult executePurchaseReservedElasticsearchInstanceOffering(
PurchaseReservedElasticsearchInstanceOfferingRequest purchaseReservedElasticsearchInstanceOfferingRequest) {
ExecutionContext executionContext = createExecutionContext(purchaseReservedElasticsearchInstanceOfferingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PurchaseReservedElasticsearchInstanceOfferingRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(purchaseReservedElasticsearchInstanceOfferingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PurchaseReservedElasticsearchInstanceOffering");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PurchaseReservedElasticsearchInstanceOfferingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows the destination domain owner to reject an inbound cross-cluster search connection request.
*
*
* @param rejectInboundCrossClusterSearchConnectionRequest
* Container for the parameters to the RejectInboundCrossClusterSearchConnection
* operation.
* @return Result of the RejectInboundCrossClusterSearchConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @sample AWSElasticsearch.RejectInboundCrossClusterSearchConnection
*/
@Override
public RejectInboundCrossClusterSearchConnectionResult rejectInboundCrossClusterSearchConnection(RejectInboundCrossClusterSearchConnectionRequest request) {
request = beforeClientExecution(request);
return executeRejectInboundCrossClusterSearchConnection(request);
}
@SdkInternalApi
final RejectInboundCrossClusterSearchConnectionResult executeRejectInboundCrossClusterSearchConnection(
RejectInboundCrossClusterSearchConnectionRequest rejectInboundCrossClusterSearchConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(rejectInboundCrossClusterSearchConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RejectInboundCrossClusterSearchConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(rejectInboundCrossClusterSearchConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RejectInboundCrossClusterSearchConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RejectInboundCrossClusterSearchConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified set of tags from the specified Elasticsearch domain.
*
*
* @param removeTagsRequest
* Container for the parameters to the RemoveTags
operation. Specify the ARN
* for the Elasticsearch domain from which you want to remove the specified TagKey
.
* @return Result of the RemoveTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.RemoveTags
*/
@Override
public RemoveTagsResult removeTags(RemoveTagsRequest request) {
request = beforeClientExecution(request);
return executeRemoveTags(request);
}
@SdkInternalApi
final RemoveTagsResult executeRemoveTags(RemoveTagsRequest removeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(removeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemoveTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Revokes access to an Amazon OpenSearch Service domain that was provided through an interface VPC endpoint.
*
*
* @param revokeVpcEndpointAccessRequest
* Revokes access to an Amazon OpenSearch Service domain that was provided through an interface VPC endpoint.
* @return Result of the RevokeVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.RevokeVpcEndpointAccess
*/
@Override
public RevokeVpcEndpointAccessResult revokeVpcEndpointAccess(RevokeVpcEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeRevokeVpcEndpointAccess(request);
}
@SdkInternalApi
final RevokeVpcEndpointAccessResult executeRevokeVpcEndpointAccess(RevokeVpcEndpointAccessRequest revokeVpcEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(revokeVpcEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RevokeVpcEndpointAccessRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(revokeVpcEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RevokeVpcEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RevokeVpcEndpointAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Schedules a service software update for an Amazon ES domain.
*
*
* @param startElasticsearchServiceSoftwareUpdateRequest
* Container for the parameters to the StartElasticsearchServiceSoftwareUpdate
operation.
* Specifies the name of the Elasticsearch domain that you wish to schedule a service software update on.
* @return Result of the StartElasticsearchServiceSoftwareUpdate operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.StartElasticsearchServiceSoftwareUpdate
*/
@Override
public StartElasticsearchServiceSoftwareUpdateResult startElasticsearchServiceSoftwareUpdate(StartElasticsearchServiceSoftwareUpdateRequest request) {
request = beforeClientExecution(request);
return executeStartElasticsearchServiceSoftwareUpdate(request);
}
@SdkInternalApi
final StartElasticsearchServiceSoftwareUpdateResult executeStartElasticsearchServiceSoftwareUpdate(
StartElasticsearchServiceSoftwareUpdateRequest startElasticsearchServiceSoftwareUpdateRequest) {
ExecutionContext executionContext = createExecutionContext(startElasticsearchServiceSoftwareUpdateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartElasticsearchServiceSoftwareUpdateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startElasticsearchServiceSoftwareUpdateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartElasticsearchServiceSoftwareUpdate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartElasticsearchServiceSoftwareUpdateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the cluster configuration of the specified Elasticsearch domain, setting as setting the instance type
* and the number of instances.
*
*
* @param updateElasticsearchDomainConfigRequest
* Container for the parameters to the UpdateElasticsearchDomain
operation. Specifies the
* type and number of instances in the domain cluster.
* @return Result of the UpdateElasticsearchDomainConfig operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws InvalidTypeException
* An exception for trying to create or access sub-resource that is either invalid or not supported. Gives
* http status code of 409.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.UpdateElasticsearchDomainConfig
*/
@Override
public UpdateElasticsearchDomainConfigResult updateElasticsearchDomainConfig(UpdateElasticsearchDomainConfigRequest request) {
request = beforeClientExecution(request);
return executeUpdateElasticsearchDomainConfig(request);
}
@SdkInternalApi
final UpdateElasticsearchDomainConfigResult executeUpdateElasticsearchDomainConfig(
UpdateElasticsearchDomainConfigRequest updateElasticsearchDomainConfigRequest) {
ExecutionContext executionContext = createExecutionContext(updateElasticsearchDomainConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateElasticsearchDomainConfigRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateElasticsearchDomainConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateElasticsearchDomainConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateElasticsearchDomainConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a package for use with Amazon ES domains.
*
*
* @param updatePackageRequest
* Container for request parameters to UpdatePackage
operation.
* @return Result of the UpdatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws LimitExceededException
* An exception for trying to create more than allowed resources or sub-resources. Gives http status code of
* 409.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws AccessDeniedException
* An error occurred because user does not have permissions to access the resource. Returns HTTP status code
* 403.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @sample AWSElasticsearch.UpdatePackage
*/
@Override
public UpdatePackageResult updatePackage(UpdatePackageRequest request) {
request = beforeClientExecution(request);
return executeUpdatePackage(request);
}
@SdkInternalApi
final UpdatePackageResult executeUpdatePackage(UpdatePackageRequest updatePackageRequest) {
ExecutionContext executionContext = createExecutionContext(updatePackageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePackageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updatePackageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePackage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdatePackageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
* @param updateVpcEndpointRequest
* Modifies an Amazon OpenSearch Service-managed interface VPC endpoint.
* @return Result of the UpdateVpcEndpoint operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use. Returns HTTP
* status code 409.
* @throws BaseException
* An error occurred while processing the request.
* @sample AWSElasticsearch.UpdateVpcEndpoint
*/
@Override
public UpdateVpcEndpointResult updateVpcEndpoint(UpdateVpcEndpointRequest request) {
request = beforeClientExecution(request);
return executeUpdateVpcEndpoint(request);
}
@SdkInternalApi
final UpdateVpcEndpointResult executeUpdateVpcEndpoint(UpdateVpcEndpointRequest updateVpcEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(updateVpcEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVpcEndpointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateVpcEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateVpcEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateVpcEndpointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows you to either upgrade your domain or perform an Upgrade eligibility check to a compatible Elasticsearch
* version.
*
*
* @param upgradeElasticsearchDomainRequest
* Container for request parameters to UpgradeElasticsearchDomain
operation.
* @return Result of the UpgradeElasticsearchDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist. Gives http status code of 400.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists. Gives http status code of 400.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation. Gives http status code of
* 409.
* @throws ValidationException
* An exception for missing / invalid input fields. Gives http status code of 400.
* @throws InternalException
* The request processing has failed because of an unknown error, exception or failure (the failure is
* internal to the service) . Gives http status code of 500.
* @sample AWSElasticsearch.UpgradeElasticsearchDomain
*/
@Override
public UpgradeElasticsearchDomainResult upgradeElasticsearchDomain(UpgradeElasticsearchDomainRequest request) {
request = beforeClientExecution(request);
return executeUpgradeElasticsearchDomain(request);
}
@SdkInternalApi
final UpgradeElasticsearchDomainResult executeUpgradeElasticsearchDomain(UpgradeElasticsearchDomainRequest upgradeElasticsearchDomainRequest) {
ExecutionContext executionContext = createExecutionContext(upgradeElasticsearchDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpgradeElasticsearchDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(upgradeElasticsearchDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Elasticsearch Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpgradeElasticsearchDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpgradeElasticsearchDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}