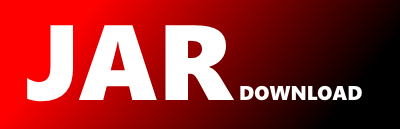
com.amazonaws.services.elasticsearch.model.ChangeProgressStatusDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-elasticsearch Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticsearch.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The progress details of a specific domain configuration change.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ChangeProgressStatusDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique change identifier associated with a specific domain configuration change.
*
*/
private String changeId;
/**
*
* The time at which the configuration change is made on the domain.
*
*/
private java.util.Date startTime;
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*/
private String status;
/**
*
* The list of properties involved in the domain configuration change that are still in pending.
*
*/
private java.util.List pendingProperties;
/**
*
* The list of properties involved in the domain configuration change that are completed.
*
*/
private java.util.List completedProperties;
/**
*
* The total number of stages required for the configuration change.
*
*/
private Integer totalNumberOfStages;
/**
*
* The specific stages that the domain is going through to perform the configuration change.
*
*/
private java.util.List changeProgressStages;
/**
*
* The current status of the configuration change.
*
*/
private String configChangeStatus;
/**
*
* The last time that the status of the configuration change was updated.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* The IAM principal who initiated the configuration change.
*
*/
private String initiatedBy;
/**
*
* The unique change identifier associated with a specific domain configuration change.
*
*
* @param changeId
* The unique change identifier associated with a specific domain configuration change.
*/
public void setChangeId(String changeId) {
this.changeId = changeId;
}
/**
*
* The unique change identifier associated with a specific domain configuration change.
*
*
* @return The unique change identifier associated with a specific domain configuration change.
*/
public String getChangeId() {
return this.changeId;
}
/**
*
* The unique change identifier associated with a specific domain configuration change.
*
*
* @param changeId
* The unique change identifier associated with a specific domain configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withChangeId(String changeId) {
setChangeId(changeId);
return this;
}
/**
*
* The time at which the configuration change is made on the domain.
*
*
* @param startTime
* The time at which the configuration change is made on the domain.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time at which the configuration change is made on the domain.
*
*
* @return The time at which the configuration change is made on the domain.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time at which the configuration change is made on the domain.
*
*
* @param startTime
* The time at which the configuration change is made on the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*
* @param status
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
* @see OverallChangeStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*
* @return The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
* @see OverallChangeStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*
* @param status
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
* @return Returns a reference to this object so that method calls can be chained together.
* @see OverallChangeStatus
*/
public ChangeProgressStatusDetails withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*
* @param status
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
* @return Returns a reference to this object so that method calls can be chained together.
* @see OverallChangeStatus
*/
public ChangeProgressStatusDetails withStatus(OverallChangeStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The list of properties involved in the domain configuration change that are still in pending.
*
*
* @return The list of properties involved in the domain configuration change that are still in pending.
*/
public java.util.List getPendingProperties() {
return pendingProperties;
}
/**
*
* The list of properties involved in the domain configuration change that are still in pending.
*
*
* @param pendingProperties
* The list of properties involved in the domain configuration change that are still in pending.
*/
public void setPendingProperties(java.util.Collection pendingProperties) {
if (pendingProperties == null) {
this.pendingProperties = null;
return;
}
this.pendingProperties = new java.util.ArrayList(pendingProperties);
}
/**
*
* The list of properties involved in the domain configuration change that are still in pending.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPendingProperties(java.util.Collection)} or {@link #withPendingProperties(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param pendingProperties
* The list of properties involved in the domain configuration change that are still in pending.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withPendingProperties(String... pendingProperties) {
if (this.pendingProperties == null) {
setPendingProperties(new java.util.ArrayList(pendingProperties.length));
}
for (String ele : pendingProperties) {
this.pendingProperties.add(ele);
}
return this;
}
/**
*
* The list of properties involved in the domain configuration change that are still in pending.
*
*
* @param pendingProperties
* The list of properties involved in the domain configuration change that are still in pending.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withPendingProperties(java.util.Collection pendingProperties) {
setPendingProperties(pendingProperties);
return this;
}
/**
*
* The list of properties involved in the domain configuration change that are completed.
*
*
* @return The list of properties involved in the domain configuration change that are completed.
*/
public java.util.List getCompletedProperties() {
return completedProperties;
}
/**
*
* The list of properties involved in the domain configuration change that are completed.
*
*
* @param completedProperties
* The list of properties involved in the domain configuration change that are completed.
*/
public void setCompletedProperties(java.util.Collection completedProperties) {
if (completedProperties == null) {
this.completedProperties = null;
return;
}
this.completedProperties = new java.util.ArrayList(completedProperties);
}
/**
*
* The list of properties involved in the domain configuration change that are completed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCompletedProperties(java.util.Collection)} or {@link #withCompletedProperties(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param completedProperties
* The list of properties involved in the domain configuration change that are completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withCompletedProperties(String... completedProperties) {
if (this.completedProperties == null) {
setCompletedProperties(new java.util.ArrayList(completedProperties.length));
}
for (String ele : completedProperties) {
this.completedProperties.add(ele);
}
return this;
}
/**
*
* The list of properties involved in the domain configuration change that are completed.
*
*
* @param completedProperties
* The list of properties involved in the domain configuration change that are completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withCompletedProperties(java.util.Collection completedProperties) {
setCompletedProperties(completedProperties);
return this;
}
/**
*
* The total number of stages required for the configuration change.
*
*
* @param totalNumberOfStages
* The total number of stages required for the configuration change.
*/
public void setTotalNumberOfStages(Integer totalNumberOfStages) {
this.totalNumberOfStages = totalNumberOfStages;
}
/**
*
* The total number of stages required for the configuration change.
*
*
* @return The total number of stages required for the configuration change.
*/
public Integer getTotalNumberOfStages() {
return this.totalNumberOfStages;
}
/**
*
* The total number of stages required for the configuration change.
*
*
* @param totalNumberOfStages
* The total number of stages required for the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withTotalNumberOfStages(Integer totalNumberOfStages) {
setTotalNumberOfStages(totalNumberOfStages);
return this;
}
/**
*
* The specific stages that the domain is going through to perform the configuration change.
*
*
* @return The specific stages that the domain is going through to perform the configuration change.
*/
public java.util.List getChangeProgressStages() {
return changeProgressStages;
}
/**
*
* The specific stages that the domain is going through to perform the configuration change.
*
*
* @param changeProgressStages
* The specific stages that the domain is going through to perform the configuration change.
*/
public void setChangeProgressStages(java.util.Collection changeProgressStages) {
if (changeProgressStages == null) {
this.changeProgressStages = null;
return;
}
this.changeProgressStages = new java.util.ArrayList(changeProgressStages);
}
/**
*
* The specific stages that the domain is going through to perform the configuration change.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChangeProgressStages(java.util.Collection)} or {@link #withChangeProgressStages(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param changeProgressStages
* The specific stages that the domain is going through to perform the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withChangeProgressStages(ChangeProgressStage... changeProgressStages) {
if (this.changeProgressStages == null) {
setChangeProgressStages(new java.util.ArrayList(changeProgressStages.length));
}
for (ChangeProgressStage ele : changeProgressStages) {
this.changeProgressStages.add(ele);
}
return this;
}
/**
*
* The specific stages that the domain is going through to perform the configuration change.
*
*
* @param changeProgressStages
* The specific stages that the domain is going through to perform the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withChangeProgressStages(java.util.Collection changeProgressStages) {
setChangeProgressStages(changeProgressStages);
return this;
}
/**
*
* The current status of the configuration change.
*
*
* @param configChangeStatus
* The current status of the configuration change.
* @see ConfigChangeStatus
*/
public void setConfigChangeStatus(String configChangeStatus) {
this.configChangeStatus = configChangeStatus;
}
/**
*
* The current status of the configuration change.
*
*
* @return The current status of the configuration change.
* @see ConfigChangeStatus
*/
public String getConfigChangeStatus() {
return this.configChangeStatus;
}
/**
*
* The current status of the configuration change.
*
*
* @param configChangeStatus
* The current status of the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigChangeStatus
*/
public ChangeProgressStatusDetails withConfigChangeStatus(String configChangeStatus) {
setConfigChangeStatus(configChangeStatus);
return this;
}
/**
*
* The current status of the configuration change.
*
*
* @param configChangeStatus
* The current status of the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigChangeStatus
*/
public ChangeProgressStatusDetails withConfigChangeStatus(ConfigChangeStatus configChangeStatus) {
this.configChangeStatus = configChangeStatus.toString();
return this;
}
/**
*
* The last time that the status of the configuration change was updated.
*
*
* @param lastUpdatedTime
* The last time that the status of the configuration change was updated.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* The last time that the status of the configuration change was updated.
*
*
* @return The last time that the status of the configuration change was updated.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* The last time that the status of the configuration change was updated.
*
*
* @param lastUpdatedTime
* The last time that the status of the configuration change was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ChangeProgressStatusDetails withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
*
* The IAM principal who initiated the configuration change.
*
*
* @param initiatedBy
* The IAM principal who initiated the configuration change.
* @see InitiatedBy
*/
public void setInitiatedBy(String initiatedBy) {
this.initiatedBy = initiatedBy;
}
/**
*
* The IAM principal who initiated the configuration change.
*
*
* @return The IAM principal who initiated the configuration change.
* @see InitiatedBy
*/
public String getInitiatedBy() {
return this.initiatedBy;
}
/**
*
* The IAM principal who initiated the configuration change.
*
*
* @param initiatedBy
* The IAM principal who initiated the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InitiatedBy
*/
public ChangeProgressStatusDetails withInitiatedBy(String initiatedBy) {
setInitiatedBy(initiatedBy);
return this;
}
/**
*
* The IAM principal who initiated the configuration change.
*
*
* @param initiatedBy
* The IAM principal who initiated the configuration change.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InitiatedBy
*/
public ChangeProgressStatusDetails withInitiatedBy(InitiatedBy initiatedBy) {
this.initiatedBy = initiatedBy.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getChangeId() != null)
sb.append("ChangeId: ").append(getChangeId()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getPendingProperties() != null)
sb.append("PendingProperties: ").append(getPendingProperties()).append(",");
if (getCompletedProperties() != null)
sb.append("CompletedProperties: ").append(getCompletedProperties()).append(",");
if (getTotalNumberOfStages() != null)
sb.append("TotalNumberOfStages: ").append(getTotalNumberOfStages()).append(",");
if (getChangeProgressStages() != null)
sb.append("ChangeProgressStages: ").append(getChangeProgressStages()).append(",");
if (getConfigChangeStatus() != null)
sb.append("ConfigChangeStatus: ").append(getConfigChangeStatus()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime()).append(",");
if (getInitiatedBy() != null)
sb.append("InitiatedBy: ").append(getInitiatedBy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ChangeProgressStatusDetails == false)
return false;
ChangeProgressStatusDetails other = (ChangeProgressStatusDetails) obj;
if (other.getChangeId() == null ^ this.getChangeId() == null)
return false;
if (other.getChangeId() != null && other.getChangeId().equals(this.getChangeId()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getPendingProperties() == null ^ this.getPendingProperties() == null)
return false;
if (other.getPendingProperties() != null && other.getPendingProperties().equals(this.getPendingProperties()) == false)
return false;
if (other.getCompletedProperties() == null ^ this.getCompletedProperties() == null)
return false;
if (other.getCompletedProperties() != null && other.getCompletedProperties().equals(this.getCompletedProperties()) == false)
return false;
if (other.getTotalNumberOfStages() == null ^ this.getTotalNumberOfStages() == null)
return false;
if (other.getTotalNumberOfStages() != null && other.getTotalNumberOfStages().equals(this.getTotalNumberOfStages()) == false)
return false;
if (other.getChangeProgressStages() == null ^ this.getChangeProgressStages() == null)
return false;
if (other.getChangeProgressStages() != null && other.getChangeProgressStages().equals(this.getChangeProgressStages()) == false)
return false;
if (other.getConfigChangeStatus() == null ^ this.getConfigChangeStatus() == null)
return false;
if (other.getConfigChangeStatus() != null && other.getConfigChangeStatus().equals(this.getConfigChangeStatus()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
if (other.getInitiatedBy() == null ^ this.getInitiatedBy() == null)
return false;
if (other.getInitiatedBy() != null && other.getInitiatedBy().equals(this.getInitiatedBy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getChangeId() == null) ? 0 : getChangeId().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getPendingProperties() == null) ? 0 : getPendingProperties().hashCode());
hashCode = prime * hashCode + ((getCompletedProperties() == null) ? 0 : getCompletedProperties().hashCode());
hashCode = prime * hashCode + ((getTotalNumberOfStages() == null) ? 0 : getTotalNumberOfStages().hashCode());
hashCode = prime * hashCode + ((getChangeProgressStages() == null) ? 0 : getChangeProgressStages().hashCode());
hashCode = prime * hashCode + ((getConfigChangeStatus() == null) ? 0 : getConfigChangeStatus().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getInitiatedBy() == null) ? 0 : getInitiatedBy().hashCode());
return hashCode;
}
@Override
public ChangeProgressStatusDetails clone() {
try {
return (ChangeProgressStatusDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.elasticsearch.model.transform.ChangeProgressStatusDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}