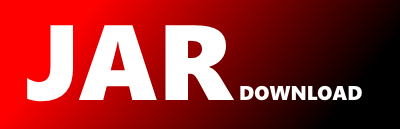
com.amazonaws.services.elasticmapreduce.model.ListInstancesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emr Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticmapreduce.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* This input determines which instances to list.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListInstancesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The identifier of the cluster for which to list the instances.
*
*/
private String clusterId;
/**
*
* The identifier of the instance group for which to list the instances.
*
*/
private String instanceGroupId;
/**
*
* The type of instance group for which to list the instances.
*
*/
private com.amazonaws.internal.SdkInternalList instanceGroupTypes;
/**
*
* The unique identifier of the instance fleet.
*
*/
private String instanceFleetId;
/**
*
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
*
*/
private String instanceFleetType;
/**
*
* A list of instance states that will filter the instances returned with this request.
*
*/
private com.amazonaws.internal.SdkInternalList instanceStates;
/**
*
* The pagination token that indicates the next set of results to retrieve.
*
*/
private String marker;
/**
*
* The identifier of the cluster for which to list the instances.
*
*
* @param clusterId
* The identifier of the cluster for which to list the instances.
*/
public void setClusterId(String clusterId) {
this.clusterId = clusterId;
}
/**
*
* The identifier of the cluster for which to list the instances.
*
*
* @return The identifier of the cluster for which to list the instances.
*/
public String getClusterId() {
return this.clusterId;
}
/**
*
* The identifier of the cluster for which to list the instances.
*
*
* @param clusterId
* The identifier of the cluster for which to list the instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInstancesRequest withClusterId(String clusterId) {
setClusterId(clusterId);
return this;
}
/**
*
* The identifier of the instance group for which to list the instances.
*
*
* @param instanceGroupId
* The identifier of the instance group for which to list the instances.
*/
public void setInstanceGroupId(String instanceGroupId) {
this.instanceGroupId = instanceGroupId;
}
/**
*
* The identifier of the instance group for which to list the instances.
*
*
* @return The identifier of the instance group for which to list the instances.
*/
public String getInstanceGroupId() {
return this.instanceGroupId;
}
/**
*
* The identifier of the instance group for which to list the instances.
*
*
* @param instanceGroupId
* The identifier of the instance group for which to list the instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInstancesRequest withInstanceGroupId(String instanceGroupId) {
setInstanceGroupId(instanceGroupId);
return this;
}
/**
*
* The type of instance group for which to list the instances.
*
*
* @return The type of instance group for which to list the instances.
* @see InstanceGroupType
*/
public java.util.List getInstanceGroupTypes() {
if (instanceGroupTypes == null) {
instanceGroupTypes = new com.amazonaws.internal.SdkInternalList();
}
return instanceGroupTypes;
}
/**
*
* The type of instance group for which to list the instances.
*
*
* @param instanceGroupTypes
* The type of instance group for which to list the instances.
* @see InstanceGroupType
*/
public void setInstanceGroupTypes(java.util.Collection instanceGroupTypes) {
if (instanceGroupTypes == null) {
this.instanceGroupTypes = null;
return;
}
this.instanceGroupTypes = new com.amazonaws.internal.SdkInternalList(instanceGroupTypes);
}
/**
*
* The type of instance group for which to list the instances.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInstanceGroupTypes(java.util.Collection)} or {@link #withInstanceGroupTypes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param instanceGroupTypes
* The type of instance group for which to list the instances.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceGroupType
*/
public ListInstancesRequest withInstanceGroupTypes(String... instanceGroupTypes) {
if (this.instanceGroupTypes == null) {
setInstanceGroupTypes(new com.amazonaws.internal.SdkInternalList(instanceGroupTypes.length));
}
for (String ele : instanceGroupTypes) {
this.instanceGroupTypes.add(ele);
}
return this;
}
/**
*
* The type of instance group for which to list the instances.
*
*
* @param instanceGroupTypes
* The type of instance group for which to list the instances.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceGroupType
*/
public ListInstancesRequest withInstanceGroupTypes(java.util.Collection instanceGroupTypes) {
setInstanceGroupTypes(instanceGroupTypes);
return this;
}
/**
*
* The type of instance group for which to list the instances.
*
*
* @param instanceGroupTypes
* The type of instance group for which to list the instances.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceGroupType
*/
public ListInstancesRequest withInstanceGroupTypes(InstanceGroupType... instanceGroupTypes) {
com.amazonaws.internal.SdkInternalList instanceGroupTypesCopy = new com.amazonaws.internal.SdkInternalList(instanceGroupTypes.length);
for (InstanceGroupType value : instanceGroupTypes) {
instanceGroupTypesCopy.add(value.toString());
}
if (getInstanceGroupTypes() == null) {
setInstanceGroupTypes(instanceGroupTypesCopy);
} else {
getInstanceGroupTypes().addAll(instanceGroupTypesCopy);
}
return this;
}
/**
*
* The unique identifier of the instance fleet.
*
*
* @param instanceFleetId
* The unique identifier of the instance fleet.
*/
public void setInstanceFleetId(String instanceFleetId) {
this.instanceFleetId = instanceFleetId;
}
/**
*
* The unique identifier of the instance fleet.
*
*
* @return The unique identifier of the instance fleet.
*/
public String getInstanceFleetId() {
return this.instanceFleetId;
}
/**
*
* The unique identifier of the instance fleet.
*
*
* @param instanceFleetId
* The unique identifier of the instance fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInstancesRequest withInstanceFleetId(String instanceFleetId) {
setInstanceFleetId(instanceFleetId);
return this;
}
/**
*
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
*
*
* @param instanceFleetType
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
* @see InstanceFleetType
*/
public void setInstanceFleetType(String instanceFleetType) {
this.instanceFleetType = instanceFleetType;
}
/**
*
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
*
*
* @return The node type of the instance fleet. For example MASTER, CORE, or TASK.
* @see InstanceFleetType
*/
public String getInstanceFleetType() {
return this.instanceFleetType;
}
/**
*
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
*
*
* @param instanceFleetType
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceFleetType
*/
public ListInstancesRequest withInstanceFleetType(String instanceFleetType) {
setInstanceFleetType(instanceFleetType);
return this;
}
/**
*
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
*
*
* @param instanceFleetType
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
* @see InstanceFleetType
*/
public void setInstanceFleetType(InstanceFleetType instanceFleetType) {
withInstanceFleetType(instanceFleetType);
}
/**
*
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
*
*
* @param instanceFleetType
* The node type of the instance fleet. For example MASTER, CORE, or TASK.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceFleetType
*/
public ListInstancesRequest withInstanceFleetType(InstanceFleetType instanceFleetType) {
this.instanceFleetType = instanceFleetType.toString();
return this;
}
/**
*
* A list of instance states that will filter the instances returned with this request.
*
*
* @return A list of instance states that will filter the instances returned with this request.
* @see InstanceState
*/
public java.util.List getInstanceStates() {
if (instanceStates == null) {
instanceStates = new com.amazonaws.internal.SdkInternalList();
}
return instanceStates;
}
/**
*
* A list of instance states that will filter the instances returned with this request.
*
*
* @param instanceStates
* A list of instance states that will filter the instances returned with this request.
* @see InstanceState
*/
public void setInstanceStates(java.util.Collection instanceStates) {
if (instanceStates == null) {
this.instanceStates = null;
return;
}
this.instanceStates = new com.amazonaws.internal.SdkInternalList(instanceStates);
}
/**
*
* A list of instance states that will filter the instances returned with this request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInstanceStates(java.util.Collection)} or {@link #withInstanceStates(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param instanceStates
* A list of instance states that will filter the instances returned with this request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceState
*/
public ListInstancesRequest withInstanceStates(String... instanceStates) {
if (this.instanceStates == null) {
setInstanceStates(new com.amazonaws.internal.SdkInternalList(instanceStates.length));
}
for (String ele : instanceStates) {
this.instanceStates.add(ele);
}
return this;
}
/**
*
* A list of instance states that will filter the instances returned with this request.
*
*
* @param instanceStates
* A list of instance states that will filter the instances returned with this request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceState
*/
public ListInstancesRequest withInstanceStates(java.util.Collection instanceStates) {
setInstanceStates(instanceStates);
return this;
}
/**
*
* A list of instance states that will filter the instances returned with this request.
*
*
* @param instanceStates
* A list of instance states that will filter the instances returned with this request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceState
*/
public ListInstancesRequest withInstanceStates(InstanceState... instanceStates) {
com.amazonaws.internal.SdkInternalList instanceStatesCopy = new com.amazonaws.internal.SdkInternalList(instanceStates.length);
for (InstanceState value : instanceStates) {
instanceStatesCopy.add(value.toString());
}
if (getInstanceStates() == null) {
setInstanceStates(instanceStatesCopy);
} else {
getInstanceStates().addAll(instanceStatesCopy);
}
return this;
}
/**
*
* The pagination token that indicates the next set of results to retrieve.
*
*
* @param marker
* The pagination token that indicates the next set of results to retrieve.
*/
public void setMarker(String marker) {
this.marker = marker;
}
/**
*
* The pagination token that indicates the next set of results to retrieve.
*
*
* @return The pagination token that indicates the next set of results to retrieve.
*/
public String getMarker() {
return this.marker;
}
/**
*
* The pagination token that indicates the next set of results to retrieve.
*
*
* @param marker
* The pagination token that indicates the next set of results to retrieve.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListInstancesRequest withMarker(String marker) {
setMarker(marker);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClusterId() != null)
sb.append("ClusterId: ").append(getClusterId()).append(",");
if (getInstanceGroupId() != null)
sb.append("InstanceGroupId: ").append(getInstanceGroupId()).append(",");
if (getInstanceGroupTypes() != null)
sb.append("InstanceGroupTypes: ").append(getInstanceGroupTypes()).append(",");
if (getInstanceFleetId() != null)
sb.append("InstanceFleetId: ").append(getInstanceFleetId()).append(",");
if (getInstanceFleetType() != null)
sb.append("InstanceFleetType: ").append(getInstanceFleetType()).append(",");
if (getInstanceStates() != null)
sb.append("InstanceStates: ").append(getInstanceStates()).append(",");
if (getMarker() != null)
sb.append("Marker: ").append(getMarker());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListInstancesRequest == false)
return false;
ListInstancesRequest other = (ListInstancesRequest) obj;
if (other.getClusterId() == null ^ this.getClusterId() == null)
return false;
if (other.getClusterId() != null && other.getClusterId().equals(this.getClusterId()) == false)
return false;
if (other.getInstanceGroupId() == null ^ this.getInstanceGroupId() == null)
return false;
if (other.getInstanceGroupId() != null && other.getInstanceGroupId().equals(this.getInstanceGroupId()) == false)
return false;
if (other.getInstanceGroupTypes() == null ^ this.getInstanceGroupTypes() == null)
return false;
if (other.getInstanceGroupTypes() != null && other.getInstanceGroupTypes().equals(this.getInstanceGroupTypes()) == false)
return false;
if (other.getInstanceFleetId() == null ^ this.getInstanceFleetId() == null)
return false;
if (other.getInstanceFleetId() != null && other.getInstanceFleetId().equals(this.getInstanceFleetId()) == false)
return false;
if (other.getInstanceFleetType() == null ^ this.getInstanceFleetType() == null)
return false;
if (other.getInstanceFleetType() != null && other.getInstanceFleetType().equals(this.getInstanceFleetType()) == false)
return false;
if (other.getInstanceStates() == null ^ this.getInstanceStates() == null)
return false;
if (other.getInstanceStates() != null && other.getInstanceStates().equals(this.getInstanceStates()) == false)
return false;
if (other.getMarker() == null ^ this.getMarker() == null)
return false;
if (other.getMarker() != null && other.getMarker().equals(this.getMarker()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClusterId() == null) ? 0 : getClusterId().hashCode());
hashCode = prime * hashCode + ((getInstanceGroupId() == null) ? 0 : getInstanceGroupId().hashCode());
hashCode = prime * hashCode + ((getInstanceGroupTypes() == null) ? 0 : getInstanceGroupTypes().hashCode());
hashCode = prime * hashCode + ((getInstanceFleetId() == null) ? 0 : getInstanceFleetId().hashCode());
hashCode = prime * hashCode + ((getInstanceFleetType() == null) ? 0 : getInstanceFleetType().hashCode());
hashCode = prime * hashCode + ((getInstanceStates() == null) ? 0 : getInstanceStates().hashCode());
hashCode = prime * hashCode + ((getMarker() == null) ? 0 : getMarker().hashCode());
return hashCode;
}
@Override
public ListInstancesRequest clone() {
return (ListInstancesRequest) super.clone();
}
}