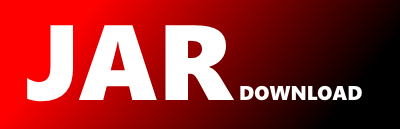
com.amazonaws.services.elasticmapreduce.model.CreateStudioRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emr Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticmapreduce.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateStudioRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A descriptive name for the Amazon EMR Studio.
*
*/
private String name;
/**
*
* A detailed description of the Amazon EMR Studio.
*
*/
private String description;
/**
*
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
*
*/
private String authMode;
/**
*
* The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
*
*/
private String vpcId;
/**
*
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets. The
* subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in any of the
* specified subnets.
*
*/
private com.amazonaws.internal.SdkInternalList subnetIds;
/**
*
* The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
*
*/
private String serviceRole;
/**
*
* The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to the
* UserRole
can be scoped down for each user or group using session policies.
*
*/
private String userRole;
/**
*
* The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound network
* traffic to resources in the Engine security group, and it must be in the same VPC specified by VpcId
* .
*
*/
private String workspaceSecurityGroupId;
/**
*
* The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network traffic
* from the Workspace security group, and it must be in the same VPC specified by VpcId
.
*
*/
private String engineSecurityGroupId;
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*/
private String defaultS3Location;
/**
*
* The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio with the Studio URL and credentials from your IdP. Amazon EMR
* Studio redirects users to this endpoint to enter credentials.
*
*/
private String idpAuthUrl;
/**
*
* The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication and want
* to let federated users log in to a Studio using the Studio URL. The RelayState
parameter differs by
* IdP.
*
*/
private String idpRelayStateParameterName;
/**
*
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist of a
* required key string with a maximum of 128 characters, and an optional value string with a maximum of 256
* characters.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* A descriptive name for the Amazon EMR Studio.
*
*
* @param name
* A descriptive name for the Amazon EMR Studio.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A descriptive name for the Amazon EMR Studio.
*
*
* @return A descriptive name for the Amazon EMR Studio.
*/
public String getName() {
return this.name;
}
/**
*
* A descriptive name for the Amazon EMR Studio.
*
*
* @param name
* A descriptive name for the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withName(String name) {
setName(name);
return this;
}
/**
*
* A detailed description of the Amazon EMR Studio.
*
*
* @param description
* A detailed description of the Amazon EMR Studio.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A detailed description of the Amazon EMR Studio.
*
*
* @return A detailed description of the Amazon EMR Studio.
*/
public String getDescription() {
return this.description;
}
/**
*
* A detailed description of the Amazon EMR Studio.
*
*
* @param description
* A detailed description of the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @param authMode
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
* @see AuthMode
*/
public void setAuthMode(String authMode) {
this.authMode = authMode;
}
/**
*
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @return Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
* @see AuthMode
*/
public String getAuthMode() {
return this.authMode;
}
/**
*
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @param authMode
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthMode
*/
public CreateStudioRequest withAuthMode(String authMode) {
setAuthMode(authMode);
return this;
}
/**
*
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @param authMode
* Specifies whether the Studio authenticates users using IAM or Amazon Web Services SSO.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthMode
*/
public CreateStudioRequest withAuthMode(AuthMode authMode) {
this.authMode = authMode.toString();
return this;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
*
*
* @param vpcId
* The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
*/
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
*
*
* @return The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
*/
public String getVpcId() {
return this.vpcId;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
*
*
* @param vpcId
* The ID of the Amazon Virtual Private Cloud (Amazon VPC) to associate with the Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withVpcId(String vpcId) {
setVpcId(vpcId);
return this;
}
/**
*
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets. The
* subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in any of the
* specified subnets.
*
*
* @return A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets.
* The subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace
* in any of the specified subnets.
*/
public java.util.List getSubnetIds() {
if (subnetIds == null) {
subnetIds = new com.amazonaws.internal.SdkInternalList();
}
return subnetIds;
}
/**
*
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets. The
* subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in any of the
* specified subnets.
*
*
* @param subnetIds
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets.
* The subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in
* any of the specified subnets.
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new com.amazonaws.internal.SdkInternalList(subnetIds);
}
/**
*
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets. The
* subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in any of the
* specified subnets.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets.
* The subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in
* any of the specified subnets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new com.amazonaws.internal.SdkInternalList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
*
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets. The
* subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in any of the
* specified subnets.
*
*
* @param subnetIds
* A list of subnet IDs to associate with the Amazon EMR Studio. A Studio can have a maximum of 5 subnets.
* The subnets must belong to the VPC specified by VpcId
. Studio users can create a Workspace in
* any of the specified subnets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
*
* The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
*
*
* @param serviceRole
* The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
*/
public void setServiceRole(String serviceRole) {
this.serviceRole = serviceRole;
}
/**
*
* The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
*
*
* @return The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
*/
public String getServiceRole() {
return this.serviceRole;
}
/**
*
* The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
*
*
* @param serviceRole
* The IAM role that the Amazon EMR Studio assumes. The service role provides a way for Amazon EMR Studio to
* interoperate with other Amazon Web Services services.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withServiceRole(String serviceRole) {
setServiceRole(serviceRole);
return this;
}
/**
*
* The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to the
* UserRole
can be scoped down for each user or group using session policies.
*
*
* @param userRole
* The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to the
* UserRole
can be scoped down for each user or group using session policies.
*/
public void setUserRole(String userRole) {
this.userRole = userRole;
}
/**
*
* The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to the
* UserRole
can be scoped down for each user or group using session policies.
*
*
* @return The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to
* the UserRole
can be scoped down for each user or group using session policies.
*/
public String getUserRole() {
return this.userRole;
}
/**
*
* The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to the
* UserRole
can be scoped down for each user or group using session policies.
*
*
* @param userRole
* The IAM user role that users and groups assume when logged in to an Amazon EMR Studio. Only specify a
* UserRole
when you use Amazon Web Services SSO authentication. The permissions attached to the
* UserRole
can be scoped down for each user or group using session policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withUserRole(String userRole) {
setUserRole(userRole);
return this;
}
/**
*
* The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound network
* traffic to resources in the Engine security group, and it must be in the same VPC specified by VpcId
* .
*
*
* @param workspaceSecurityGroupId
* The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound
* network traffic to resources in the Engine security group, and it must be in the same VPC specified by
* VpcId
.
*/
public void setWorkspaceSecurityGroupId(String workspaceSecurityGroupId) {
this.workspaceSecurityGroupId = workspaceSecurityGroupId;
}
/**
*
* The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound network
* traffic to resources in the Engine security group, and it must be in the same VPC specified by VpcId
* .
*
*
* @return The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound
* network traffic to resources in the Engine security group, and it must be in the same VPC specified by
* VpcId
.
*/
public String getWorkspaceSecurityGroupId() {
return this.workspaceSecurityGroupId;
}
/**
*
* The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound network
* traffic to resources in the Engine security group, and it must be in the same VPC specified by VpcId
* .
*
*
* @param workspaceSecurityGroupId
* The ID of the Amazon EMR Studio Workspace security group. The Workspace security group allows outbound
* network traffic to resources in the Engine security group, and it must be in the same VPC specified by
* VpcId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withWorkspaceSecurityGroupId(String workspaceSecurityGroupId) {
setWorkspaceSecurityGroupId(workspaceSecurityGroupId);
return this;
}
/**
*
* The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network traffic
* from the Workspace security group, and it must be in the same VPC specified by VpcId
.
*
*
* @param engineSecurityGroupId
* The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network
* traffic from the Workspace security group, and it must be in the same VPC specified by VpcId
.
*/
public void setEngineSecurityGroupId(String engineSecurityGroupId) {
this.engineSecurityGroupId = engineSecurityGroupId;
}
/**
*
* The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network traffic
* from the Workspace security group, and it must be in the same VPC specified by VpcId
.
*
*
* @return The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network
* traffic from the Workspace security group, and it must be in the same VPC specified by VpcId
* .
*/
public String getEngineSecurityGroupId() {
return this.engineSecurityGroupId;
}
/**
*
* The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network traffic
* from the Workspace security group, and it must be in the same VPC specified by VpcId
.
*
*
* @param engineSecurityGroupId
* The ID of the Amazon EMR Studio Engine security group. The Engine security group allows inbound network
* traffic from the Workspace security group, and it must be in the same VPC specified by VpcId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withEngineSecurityGroupId(String engineSecurityGroupId) {
setEngineSecurityGroupId(engineSecurityGroupId);
return this;
}
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*
* @param defaultS3Location
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*/
public void setDefaultS3Location(String defaultS3Location) {
this.defaultS3Location = defaultS3Location;
}
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*
* @return The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*/
public String getDefaultS3Location() {
return this.defaultS3Location;
}
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*
* @param defaultS3Location
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withDefaultS3Location(String defaultS3Location) {
setDefaultS3Location(defaultS3Location);
return this;
}
/**
*
* The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio with the Studio URL and credentials from your IdP. Amazon EMR
* Studio redirects users to this endpoint to enter credentials.
*
*
* @param idpAuthUrl
* The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM
* authentication and want to let federated users log in to a Studio with the Studio URL and credentials from
* your IdP. Amazon EMR Studio redirects users to this endpoint to enter credentials.
*/
public void setIdpAuthUrl(String idpAuthUrl) {
this.idpAuthUrl = idpAuthUrl;
}
/**
*
* The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio with the Studio URL and credentials from your IdP. Amazon EMR
* Studio redirects users to this endpoint to enter credentials.
*
*
* @return The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM
* authentication and want to let federated users log in to a Studio with the Studio URL and credentials
* from your IdP. Amazon EMR Studio redirects users to this endpoint to enter credentials.
*/
public String getIdpAuthUrl() {
return this.idpAuthUrl;
}
/**
*
* The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio with the Studio URL and credentials from your IdP. Amazon EMR
* Studio redirects users to this endpoint to enter credentials.
*
*
* @param idpAuthUrl
* The authentication endpoint of your identity provider (IdP). Specify this value when you use IAM
* authentication and want to let federated users log in to a Studio with the Studio URL and credentials from
* your IdP. Amazon EMR Studio redirects users to this endpoint to enter credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withIdpAuthUrl(String idpAuthUrl) {
setIdpAuthUrl(idpAuthUrl);
return this;
}
/**
*
* The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication and want
* to let federated users log in to a Studio using the Studio URL. The RelayState
parameter differs by
* IdP.
*
*
* @param idpRelayStateParameterName
* The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio using the Studio URL. The RelayState
* parameter differs by IdP.
*/
public void setIdpRelayStateParameterName(String idpRelayStateParameterName) {
this.idpRelayStateParameterName = idpRelayStateParameterName;
}
/**
*
* The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication and want
* to let federated users log in to a Studio using the Studio URL. The RelayState
parameter differs by
* IdP.
*
*
* @return The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio using the Studio URL. The RelayState
* parameter differs by IdP.
*/
public String getIdpRelayStateParameterName() {
return this.idpRelayStateParameterName;
}
/**
*
* The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication and want
* to let federated users log in to a Studio using the Studio URL. The RelayState
parameter differs by
* IdP.
*
*
* @param idpRelayStateParameterName
* The name that your identity provider (IdP) uses for its RelayState
parameter. For example,
* RelayState
or TargetSource
. Specify this value when you use IAM authentication
* and want to let federated users log in to a Studio using the Studio URL. The RelayState
* parameter differs by IdP.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withIdpRelayStateParameterName(String idpRelayStateParameterName) {
setIdpRelayStateParameterName(idpRelayStateParameterName);
return this;
}
/**
*
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist of a
* required key string with a maximum of 128 characters, and an optional value string with a maximum of 256
* characters.
*
*
* @return A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that
* consist of a required key string with a maximum of 128 characters, and an optional value string with a
* maximum of 256 characters.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist of a
* required key string with a maximum of 128 characters, and an optional value string with a maximum of 256
* characters.
*
*
* @param tags
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist
* of a required key string with a maximum of 128 characters, and an optional value string with a maximum of
* 256 characters.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist of a
* required key string with a maximum of 128 characters, and an optional value string with a maximum of 256
* characters.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist
* of a required key string with a maximum of 128 characters, and an optional value string with a maximum of
* 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist of a
* required key string with a maximum of 128 characters, and an optional value string with a maximum of 256
* characters.
*
*
* @param tags
* A list of tags to associate with the Amazon EMR Studio. Tags are user-defined key-value pairs that consist
* of a required key string with a maximum of 128 characters, and an optional value string with a maximum of
* 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStudioRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getAuthMode() != null)
sb.append("AuthMode: ").append(getAuthMode()).append(",");
if (getVpcId() != null)
sb.append("VpcId: ").append(getVpcId()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getServiceRole() != null)
sb.append("ServiceRole: ").append(getServiceRole()).append(",");
if (getUserRole() != null)
sb.append("UserRole: ").append(getUserRole()).append(",");
if (getWorkspaceSecurityGroupId() != null)
sb.append("WorkspaceSecurityGroupId: ").append(getWorkspaceSecurityGroupId()).append(",");
if (getEngineSecurityGroupId() != null)
sb.append("EngineSecurityGroupId: ").append(getEngineSecurityGroupId()).append(",");
if (getDefaultS3Location() != null)
sb.append("DefaultS3Location: ").append(getDefaultS3Location()).append(",");
if (getIdpAuthUrl() != null)
sb.append("IdpAuthUrl: ").append(getIdpAuthUrl()).append(",");
if (getIdpRelayStateParameterName() != null)
sb.append("IdpRelayStateParameterName: ").append(getIdpRelayStateParameterName()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateStudioRequest == false)
return false;
CreateStudioRequest other = (CreateStudioRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getAuthMode() == null ^ this.getAuthMode() == null)
return false;
if (other.getAuthMode() != null && other.getAuthMode().equals(this.getAuthMode()) == false)
return false;
if (other.getVpcId() == null ^ this.getVpcId() == null)
return false;
if (other.getVpcId() != null && other.getVpcId().equals(this.getVpcId()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getServiceRole() == null ^ this.getServiceRole() == null)
return false;
if (other.getServiceRole() != null && other.getServiceRole().equals(this.getServiceRole()) == false)
return false;
if (other.getUserRole() == null ^ this.getUserRole() == null)
return false;
if (other.getUserRole() != null && other.getUserRole().equals(this.getUserRole()) == false)
return false;
if (other.getWorkspaceSecurityGroupId() == null ^ this.getWorkspaceSecurityGroupId() == null)
return false;
if (other.getWorkspaceSecurityGroupId() != null && other.getWorkspaceSecurityGroupId().equals(this.getWorkspaceSecurityGroupId()) == false)
return false;
if (other.getEngineSecurityGroupId() == null ^ this.getEngineSecurityGroupId() == null)
return false;
if (other.getEngineSecurityGroupId() != null && other.getEngineSecurityGroupId().equals(this.getEngineSecurityGroupId()) == false)
return false;
if (other.getDefaultS3Location() == null ^ this.getDefaultS3Location() == null)
return false;
if (other.getDefaultS3Location() != null && other.getDefaultS3Location().equals(this.getDefaultS3Location()) == false)
return false;
if (other.getIdpAuthUrl() == null ^ this.getIdpAuthUrl() == null)
return false;
if (other.getIdpAuthUrl() != null && other.getIdpAuthUrl().equals(this.getIdpAuthUrl()) == false)
return false;
if (other.getIdpRelayStateParameterName() == null ^ this.getIdpRelayStateParameterName() == null)
return false;
if (other.getIdpRelayStateParameterName() != null && other.getIdpRelayStateParameterName().equals(this.getIdpRelayStateParameterName()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getAuthMode() == null) ? 0 : getAuthMode().hashCode());
hashCode = prime * hashCode + ((getVpcId() == null) ? 0 : getVpcId().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getServiceRole() == null) ? 0 : getServiceRole().hashCode());
hashCode = prime * hashCode + ((getUserRole() == null) ? 0 : getUserRole().hashCode());
hashCode = prime * hashCode + ((getWorkspaceSecurityGroupId() == null) ? 0 : getWorkspaceSecurityGroupId().hashCode());
hashCode = prime * hashCode + ((getEngineSecurityGroupId() == null) ? 0 : getEngineSecurityGroupId().hashCode());
hashCode = prime * hashCode + ((getDefaultS3Location() == null) ? 0 : getDefaultS3Location().hashCode());
hashCode = prime * hashCode + ((getIdpAuthUrl() == null) ? 0 : getIdpAuthUrl().hashCode());
hashCode = prime * hashCode + ((getIdpRelayStateParameterName() == null) ? 0 : getIdpRelayStateParameterName().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateStudioRequest clone() {
return (CreateStudioRequest) super.clone();
}
}