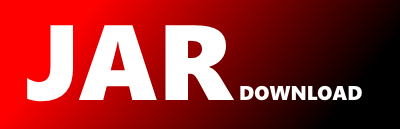
com.amazonaws.services.elasticmapreduce.model.Studio Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emr Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.elasticmapreduce.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details for an Amazon EMR Studio including ID, creation time, name, and so on.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Studio implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the Amazon EMR Studio.
*
*/
private String studioId;
/**
*
* The Amazon Resource Name (ARN) of the Amazon EMR Studio.
*
*/
private String studioArn;
/**
*
* The name of the Amazon EMR Studio.
*
*/
private String name;
/**
*
* The detailed description of the Amazon EMR Studio.
*
*/
private String description;
/**
*
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
*
*/
private String authMode;
/**
*
* The ID of the VPC associated with the Amazon EMR Studio.
*
*/
private String vpcId;
/**
*
* The list of IDs of the subnets associated with the Amazon EMR Studio.
*
*/
private com.amazonaws.internal.SdkInternalList subnetIds;
/**
*
* The name of the IAM role assumed by the Amazon EMR Studio.
*
*/
private String serviceRole;
/**
*
* The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
*
*/
private String userRole;
/**
*
* The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security group allows
* outbound network traffic to resources in the Engine security group and to the internet.
*
*/
private String workspaceSecurityGroupId;
/**
*
* The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group allows
* inbound network traffic from resources in the Workspace security group.
*
*/
private String engineSecurityGroupId;
/**
*
* The unique access URL of the Amazon EMR Studio.
*
*/
private String url;
/**
*
* The time the Amazon EMR Studio was created.
*
*/
private java.util.Date creationTime;
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*/
private String defaultS3Location;
/**
*
* Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this endpoint
* for authentication when logging in to a Studio with the Studio URL.
*
*/
private String idpAuthUrl;
/**
*
* The name of your identity provider's RelayState
parameter.
*
*/
private String idpRelayStateParameterName;
/**
*
* A list of tags associated with the Amazon EMR Studio.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The ID of the Amazon EMR Studio.
*
*
* @param studioId
* The ID of the Amazon EMR Studio.
*/
public void setStudioId(String studioId) {
this.studioId = studioId;
}
/**
*
* The ID of the Amazon EMR Studio.
*
*
* @return The ID of the Amazon EMR Studio.
*/
public String getStudioId() {
return this.studioId;
}
/**
*
* The ID of the Amazon EMR Studio.
*
*
* @param studioId
* The ID of the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withStudioId(String studioId) {
setStudioId(studioId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon EMR Studio.
*
*
* @param studioArn
* The Amazon Resource Name (ARN) of the Amazon EMR Studio.
*/
public void setStudioArn(String studioArn) {
this.studioArn = studioArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon EMR Studio.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon EMR Studio.
*/
public String getStudioArn() {
return this.studioArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon EMR Studio.
*
*
* @param studioArn
* The Amazon Resource Name (ARN) of the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withStudioArn(String studioArn) {
setStudioArn(studioArn);
return this;
}
/**
*
* The name of the Amazon EMR Studio.
*
*
* @param name
* The name of the Amazon EMR Studio.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the Amazon EMR Studio.
*
*
* @return The name of the Amazon EMR Studio.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the Amazon EMR Studio.
*
*
* @param name
* The name of the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withName(String name) {
setName(name);
return this;
}
/**
*
* The detailed description of the Amazon EMR Studio.
*
*
* @param description
* The detailed description of the Amazon EMR Studio.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The detailed description of the Amazon EMR Studio.
*
*
* @return The detailed description of the Amazon EMR Studio.
*/
public String getDescription() {
return this.description;
}
/**
*
* The detailed description of the Amazon EMR Studio.
*
*
* @param description
* The detailed description of the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @param authMode
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
* @see AuthMode
*/
public void setAuthMode(String authMode) {
this.authMode = authMode;
}
/**
*
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @return Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
* @see AuthMode
*/
public String getAuthMode() {
return this.authMode;
}
/**
*
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @param authMode
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthMode
*/
public Studio withAuthMode(String authMode) {
setAuthMode(authMode);
return this;
}
/**
*
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
*
*
* @param authMode
* Specifies whether the Amazon EMR Studio authenticates users using IAM or Amazon Web Services SSO.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthMode
*/
public Studio withAuthMode(AuthMode authMode) {
this.authMode = authMode.toString();
return this;
}
/**
*
* The ID of the VPC associated with the Amazon EMR Studio.
*
*
* @param vpcId
* The ID of the VPC associated with the Amazon EMR Studio.
*/
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
/**
*
* The ID of the VPC associated with the Amazon EMR Studio.
*
*
* @return The ID of the VPC associated with the Amazon EMR Studio.
*/
public String getVpcId() {
return this.vpcId;
}
/**
*
* The ID of the VPC associated with the Amazon EMR Studio.
*
*
* @param vpcId
* The ID of the VPC associated with the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withVpcId(String vpcId) {
setVpcId(vpcId);
return this;
}
/**
*
* The list of IDs of the subnets associated with the Amazon EMR Studio.
*
*
* @return The list of IDs of the subnets associated with the Amazon EMR Studio.
*/
public java.util.List getSubnetIds() {
if (subnetIds == null) {
subnetIds = new com.amazonaws.internal.SdkInternalList();
}
return subnetIds;
}
/**
*
* The list of IDs of the subnets associated with the Amazon EMR Studio.
*
*
* @param subnetIds
* The list of IDs of the subnets associated with the Amazon EMR Studio.
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new com.amazonaws.internal.SdkInternalList(subnetIds);
}
/**
*
* The list of IDs of the subnets associated with the Amazon EMR Studio.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* The list of IDs of the subnets associated with the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new com.amazonaws.internal.SdkInternalList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
*
* The list of IDs of the subnets associated with the Amazon EMR Studio.
*
*
* @param subnetIds
* The list of IDs of the subnets associated with the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
*
* The name of the IAM role assumed by the Amazon EMR Studio.
*
*
* @param serviceRole
* The name of the IAM role assumed by the Amazon EMR Studio.
*/
public void setServiceRole(String serviceRole) {
this.serviceRole = serviceRole;
}
/**
*
* The name of the IAM role assumed by the Amazon EMR Studio.
*
*
* @return The name of the IAM role assumed by the Amazon EMR Studio.
*/
public String getServiceRole() {
return this.serviceRole;
}
/**
*
* The name of the IAM role assumed by the Amazon EMR Studio.
*
*
* @param serviceRole
* The name of the IAM role assumed by the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withServiceRole(String serviceRole) {
setServiceRole(serviceRole);
return this;
}
/**
*
* The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
*
*
* @param userRole
* The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
*/
public void setUserRole(String userRole) {
this.userRole = userRole;
}
/**
*
* The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
*
*
* @return The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
*/
public String getUserRole() {
return this.userRole;
}
/**
*
* The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
*
*
* @param userRole
* The name of the IAM role assumed by users logged in to the Amazon EMR Studio. A Studio only requires a
* UserRole
when you use IAM authentication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withUserRole(String userRole) {
setUserRole(userRole);
return this;
}
/**
*
* The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security group allows
* outbound network traffic to resources in the Engine security group and to the internet.
*
*
* @param workspaceSecurityGroupId
* The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security group
* allows outbound network traffic to resources in the Engine security group and to the internet.
*/
public void setWorkspaceSecurityGroupId(String workspaceSecurityGroupId) {
this.workspaceSecurityGroupId = workspaceSecurityGroupId;
}
/**
*
* The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security group allows
* outbound network traffic to resources in the Engine security group and to the internet.
*
*
* @return The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security
* group allows outbound network traffic to resources in the Engine security group and to the internet.
*/
public String getWorkspaceSecurityGroupId() {
return this.workspaceSecurityGroupId;
}
/**
*
* The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security group allows
* outbound network traffic to resources in the Engine security group and to the internet.
*
*
* @param workspaceSecurityGroupId
* The ID of the Workspace security group associated with the Amazon EMR Studio. The Workspace security group
* allows outbound network traffic to resources in the Engine security group and to the internet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withWorkspaceSecurityGroupId(String workspaceSecurityGroupId) {
setWorkspaceSecurityGroupId(workspaceSecurityGroupId);
return this;
}
/**
*
* The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group allows
* inbound network traffic from resources in the Workspace security group.
*
*
* @param engineSecurityGroupId
* The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group
* allows inbound network traffic from resources in the Workspace security group.
*/
public void setEngineSecurityGroupId(String engineSecurityGroupId) {
this.engineSecurityGroupId = engineSecurityGroupId;
}
/**
*
* The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group allows
* inbound network traffic from resources in the Workspace security group.
*
*
* @return The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group
* allows inbound network traffic from resources in the Workspace security group.
*/
public String getEngineSecurityGroupId() {
return this.engineSecurityGroupId;
}
/**
*
* The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group allows
* inbound network traffic from resources in the Workspace security group.
*
*
* @param engineSecurityGroupId
* The ID of the Engine security group associated with the Amazon EMR Studio. The Engine security group
* allows inbound network traffic from resources in the Workspace security group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withEngineSecurityGroupId(String engineSecurityGroupId) {
setEngineSecurityGroupId(engineSecurityGroupId);
return this;
}
/**
*
* The unique access URL of the Amazon EMR Studio.
*
*
* @param url
* The unique access URL of the Amazon EMR Studio.
*/
public void setUrl(String url) {
this.url = url;
}
/**
*
* The unique access URL of the Amazon EMR Studio.
*
*
* @return The unique access URL of the Amazon EMR Studio.
*/
public String getUrl() {
return this.url;
}
/**
*
* The unique access URL of the Amazon EMR Studio.
*
*
* @param url
* The unique access URL of the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withUrl(String url) {
setUrl(url);
return this;
}
/**
*
* The time the Amazon EMR Studio was created.
*
*
* @param creationTime
* The time the Amazon EMR Studio was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The time the Amazon EMR Studio was created.
*
*
* @return The time the Amazon EMR Studio was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The time the Amazon EMR Studio was created.
*
*
* @param creationTime
* The time the Amazon EMR Studio was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*
* @param defaultS3Location
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*/
public void setDefaultS3Location(String defaultS3Location) {
this.defaultS3Location = defaultS3Location;
}
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*
* @return The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*/
public String getDefaultS3Location() {
return this.defaultS3Location;
}
/**
*
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
*
*
* @param defaultS3Location
* The Amazon S3 location to back up Amazon EMR Studio Workspaces and notebook files.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withDefaultS3Location(String defaultS3Location) {
setDefaultS3Location(defaultS3Location);
return this;
}
/**
*
* Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this endpoint
* for authentication when logging in to a Studio with the Studio URL.
*
*
* @param idpAuthUrl
* Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this
* endpoint for authentication when logging in to a Studio with the Studio URL.
*/
public void setIdpAuthUrl(String idpAuthUrl) {
this.idpAuthUrl = idpAuthUrl;
}
/**
*
* Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this endpoint
* for authentication when logging in to a Studio with the Studio URL.
*
*
* @return Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this
* endpoint for authentication when logging in to a Studio with the Studio URL.
*/
public String getIdpAuthUrl() {
return this.idpAuthUrl;
}
/**
*
* Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this endpoint
* for authentication when logging in to a Studio with the Studio URL.
*
*
* @param idpAuthUrl
* Your identity provider's authentication endpoint. Amazon EMR Studio redirects federated users to this
* endpoint for authentication when logging in to a Studio with the Studio URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withIdpAuthUrl(String idpAuthUrl) {
setIdpAuthUrl(idpAuthUrl);
return this;
}
/**
*
* The name of your identity provider's RelayState
parameter.
*
*
* @param idpRelayStateParameterName
* The name of your identity provider's RelayState
parameter.
*/
public void setIdpRelayStateParameterName(String idpRelayStateParameterName) {
this.idpRelayStateParameterName = idpRelayStateParameterName;
}
/**
*
* The name of your identity provider's RelayState
parameter.
*
*
* @return The name of your identity provider's RelayState
parameter.
*/
public String getIdpRelayStateParameterName() {
return this.idpRelayStateParameterName;
}
/**
*
* The name of your identity provider's RelayState
parameter.
*
*
* @param idpRelayStateParameterName
* The name of your identity provider's RelayState
parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withIdpRelayStateParameterName(String idpRelayStateParameterName) {
setIdpRelayStateParameterName(idpRelayStateParameterName);
return this;
}
/**
*
* A list of tags associated with the Amazon EMR Studio.
*
*
* @return A list of tags associated with the Amazon EMR Studio.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* A list of tags associated with the Amazon EMR Studio.
*
*
* @param tags
* A list of tags associated with the Amazon EMR Studio.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* A list of tags associated with the Amazon EMR Studio.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags associated with the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags associated with the Amazon EMR Studio.
*
*
* @param tags
* A list of tags associated with the Amazon EMR Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Studio withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStudioId() != null)
sb.append("StudioId: ").append(getStudioId()).append(",");
if (getStudioArn() != null)
sb.append("StudioArn: ").append(getStudioArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getAuthMode() != null)
sb.append("AuthMode: ").append(getAuthMode()).append(",");
if (getVpcId() != null)
sb.append("VpcId: ").append(getVpcId()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getServiceRole() != null)
sb.append("ServiceRole: ").append(getServiceRole()).append(",");
if (getUserRole() != null)
sb.append("UserRole: ").append(getUserRole()).append(",");
if (getWorkspaceSecurityGroupId() != null)
sb.append("WorkspaceSecurityGroupId: ").append(getWorkspaceSecurityGroupId()).append(",");
if (getEngineSecurityGroupId() != null)
sb.append("EngineSecurityGroupId: ").append(getEngineSecurityGroupId()).append(",");
if (getUrl() != null)
sb.append("Url: ").append(getUrl()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getDefaultS3Location() != null)
sb.append("DefaultS3Location: ").append(getDefaultS3Location()).append(",");
if (getIdpAuthUrl() != null)
sb.append("IdpAuthUrl: ").append(getIdpAuthUrl()).append(",");
if (getIdpRelayStateParameterName() != null)
sb.append("IdpRelayStateParameterName: ").append(getIdpRelayStateParameterName()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Studio == false)
return false;
Studio other = (Studio) obj;
if (other.getStudioId() == null ^ this.getStudioId() == null)
return false;
if (other.getStudioId() != null && other.getStudioId().equals(this.getStudioId()) == false)
return false;
if (other.getStudioArn() == null ^ this.getStudioArn() == null)
return false;
if (other.getStudioArn() != null && other.getStudioArn().equals(this.getStudioArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getAuthMode() == null ^ this.getAuthMode() == null)
return false;
if (other.getAuthMode() != null && other.getAuthMode().equals(this.getAuthMode()) == false)
return false;
if (other.getVpcId() == null ^ this.getVpcId() == null)
return false;
if (other.getVpcId() != null && other.getVpcId().equals(this.getVpcId()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getServiceRole() == null ^ this.getServiceRole() == null)
return false;
if (other.getServiceRole() != null && other.getServiceRole().equals(this.getServiceRole()) == false)
return false;
if (other.getUserRole() == null ^ this.getUserRole() == null)
return false;
if (other.getUserRole() != null && other.getUserRole().equals(this.getUserRole()) == false)
return false;
if (other.getWorkspaceSecurityGroupId() == null ^ this.getWorkspaceSecurityGroupId() == null)
return false;
if (other.getWorkspaceSecurityGroupId() != null && other.getWorkspaceSecurityGroupId().equals(this.getWorkspaceSecurityGroupId()) == false)
return false;
if (other.getEngineSecurityGroupId() == null ^ this.getEngineSecurityGroupId() == null)
return false;
if (other.getEngineSecurityGroupId() != null && other.getEngineSecurityGroupId().equals(this.getEngineSecurityGroupId()) == false)
return false;
if (other.getUrl() == null ^ this.getUrl() == null)
return false;
if (other.getUrl() != null && other.getUrl().equals(this.getUrl()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getDefaultS3Location() == null ^ this.getDefaultS3Location() == null)
return false;
if (other.getDefaultS3Location() != null && other.getDefaultS3Location().equals(this.getDefaultS3Location()) == false)
return false;
if (other.getIdpAuthUrl() == null ^ this.getIdpAuthUrl() == null)
return false;
if (other.getIdpAuthUrl() != null && other.getIdpAuthUrl().equals(this.getIdpAuthUrl()) == false)
return false;
if (other.getIdpRelayStateParameterName() == null ^ this.getIdpRelayStateParameterName() == null)
return false;
if (other.getIdpRelayStateParameterName() != null && other.getIdpRelayStateParameterName().equals(this.getIdpRelayStateParameterName()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStudioId() == null) ? 0 : getStudioId().hashCode());
hashCode = prime * hashCode + ((getStudioArn() == null) ? 0 : getStudioArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getAuthMode() == null) ? 0 : getAuthMode().hashCode());
hashCode = prime * hashCode + ((getVpcId() == null) ? 0 : getVpcId().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getServiceRole() == null) ? 0 : getServiceRole().hashCode());
hashCode = prime * hashCode + ((getUserRole() == null) ? 0 : getUserRole().hashCode());
hashCode = prime * hashCode + ((getWorkspaceSecurityGroupId() == null) ? 0 : getWorkspaceSecurityGroupId().hashCode());
hashCode = prime * hashCode + ((getEngineSecurityGroupId() == null) ? 0 : getEngineSecurityGroupId().hashCode());
hashCode = prime * hashCode + ((getUrl() == null) ? 0 : getUrl().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getDefaultS3Location() == null) ? 0 : getDefaultS3Location().hashCode());
hashCode = prime * hashCode + ((getIdpAuthUrl() == null) ? 0 : getIdpAuthUrl().hashCode());
hashCode = prime * hashCode + ((getIdpRelayStateParameterName() == null) ? 0 : getIdpRelayStateParameterName().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public Studio clone() {
try {
return (Studio) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.elasticmapreduce.model.transform.StudioMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}