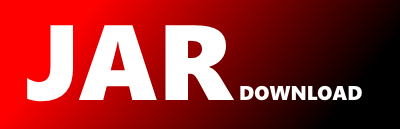
com.amazonaws.services.emrserverless.AWSEMRServerless Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emrserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.emrserverless;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.emrserverless.model.*;
/**
* Interface for accessing EMR Serverless.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.emrserverless.AbstractAWSEMRServerless} instead.
*
*
*
* Amazon EMR Serverless is a new deployment option for Amazon EMR. Amazon EMR Serverless provides a serverless runtime
* environment that simplifies running analytics applications using the latest open source frameworks such as Apache
* Spark and Apache Hive. With Amazon EMR Serverless, you don’t have to configure, optimize, secure, or operate clusters
* to run applications with these frameworks.
*
*
* The API reference to Amazon EMR Serverless is emr-serverless
. The emr-serverless
prefix is
* used in the following scenarios:
*
*
* -
*
* It is the prefix in the CLI commands for Amazon EMR Serverless. For example,
* aws emr-serverless start-job-run
.
*
*
* -
*
* It is the prefix before IAM policy actions for Amazon EMR Serverless. For example,
* "Action": ["emr-serverless:StartJobRun"]
. For more information, see Policy actions for Amazon EMR Serverless.
*
*
* -
*
* It is the prefix used in Amazon EMR Serverless service endpoints. For example,
* emr-serverless.us-east-2.amazonaws.com
.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSEMRServerless {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "emr-serverless";
/**
*
* Cancels a job run.
*
*
* @param cancelJobRunRequest
* @return Result of the CancelJobRun operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.CancelJobRun
* @see AWS
* API Documentation
*/
CancelJobRunResult cancelJobRun(CancelJobRunRequest cancelJobRunRequest);
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @sample AWSEMRServerless.CreateApplication
* @see AWS API Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Deletes an application. An application has to be in a stopped or created state in order to be deleted.
*
*
* @param deleteApplicationRequest
* @return Result of the DeleteApplication operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.DeleteApplication
* @see AWS API Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Displays detailed information about a specified application.
*
*
* @param getApplicationRequest
* @return Result of the GetApplication operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.GetApplication
* @see AWS
* API Documentation
*/
GetApplicationResult getApplication(GetApplicationRequest getApplicationRequest);
/**
*
* Creates and returns a URL that you can use to access the application UIs for a job run.
*
*
* For jobs in a running state, the application UI is a live user interface such as the Spark or Tez web UI. For
* completed jobs, the application UI is a persistent application user interface such as the Spark History Server or
* persistent Tez UI.
*
*
*
* The URL is valid for one hour after you generate it. To access the application UI after that hour elapses, you
* must invoke the API again to generate a new URL.
*
*
*
* @param getDashboardForJobRunRequest
* @return Result of the GetDashboardForJobRun operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.GetDashboardForJobRun
* @see AWS API Documentation
*/
GetDashboardForJobRunResult getDashboardForJobRun(GetDashboardForJobRunRequest getDashboardForJobRunRequest);
/**
*
* Displays detailed information about a job run.
*
*
* @param getJobRunRequest
* @return Result of the GetJobRun operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.GetJobRun
* @see AWS API
* Documentation
*/
GetJobRunResult getJobRun(GetJobRunRequest getJobRunRequest);
/**
*
* Lists applications based on a set of parameters.
*
*
* @param listApplicationsRequest
* @return Result of the ListApplications operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.ListApplications
* @see AWS API Documentation
*/
ListApplicationsResult listApplications(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists all attempt of a job run.
*
*
* @param listJobRunAttemptsRequest
* @return Result of the ListJobRunAttempts operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.ListJobRunAttempts
* @see AWS API Documentation
*/
ListJobRunAttemptsResult listJobRunAttempts(ListJobRunAttemptsRequest listJobRunAttemptsRequest);
/**
*
* Lists job runs based on a set of parameters.
*
*
* @param listJobRunsRequest
* @return Result of the ListJobRuns operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.ListJobRuns
* @see AWS API
* Documentation
*/
ListJobRunsResult listJobRuns(ListJobRunsRequest listJobRunsRequest);
/**
*
* Lists the tags assigned to the resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts a specified application and initializes initial capacity if configured.
*
*
* @param startApplicationRequest
* @return Result of the StartApplication operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @throws ServiceQuotaExceededException
* The maximum number of resources per account has been reached.
* @sample AWSEMRServerless.StartApplication
* @see AWS API Documentation
*/
StartApplicationResult startApplication(StartApplicationRequest startApplicationRequest);
/**
*
* Starts a job run.
*
*
* @param startJobRunRequest
* @return Result of the StartJobRun operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @sample AWSEMRServerless.StartJobRun
* @see AWS API
* Documentation
*/
StartJobRunResult startJobRun(StartJobRunRequest startJobRunRequest);
/**
*
* Stops a specified application and releases initial capacity if configured. All scheduled and running jobs must be
* completed or cancelled before stopping an application.
*
*
* @param stopApplicationRequest
* @return Result of the StopApplication operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.StopApplication
* @see AWS
* API Documentation
*/
StopApplicationResult stopApplication(StopApplicationRequest stopApplicationRequest);
/**
*
* Assigns tags to resources. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists
* of a key and an optional value, both of which you define. Tags enable you to categorize your Amazon Web Services
* resources by attributes such as purpose, owner, or environment. When you have many resources of the same type,
* you can quickly identify a specific resource based on the tags you've assigned to it.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from resources.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a specified application. An application has to be in a stopped or created state in order to be updated.
*
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServerException
* Request processing failed because of an error or failure with the service.
* @sample AWSEMRServerless.UpdateApplication
* @see AWS API Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}