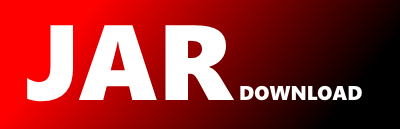
com.amazonaws.services.emrserverless.model.Application Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emrserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.emrserverless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about an application. Amazon EMR Serverless uses applications to run jobs.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Application implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the application.
*
*/
private String applicationId;
/**
*
* The name of the application.
*
*/
private String name;
/**
*
* The ARN of the application.
*
*/
private String arn;
/**
*
* The Amazon EMR release associated with the application.
*
*/
private String releaseLabel;
/**
*
* The type of application, such as Spark or Hive.
*
*/
private String type;
/**
*
* The state of the application.
*
*/
private String state;
/**
*
* The state details of the application.
*
*/
private String stateDetails;
/**
*
* The initial capacity of the application.
*
*/
private java.util.Map initialCapacity;
/**
*
* The maximum capacity of the application. This is cumulative across all workers at any given point in time during
* the lifespan of the application is created. No new resources will be created once any one of the defined limits
* is hit.
*
*/
private MaximumAllowedResources maximumCapacity;
/**
*
* The date and time when the application run was created.
*
*/
private java.util.Date createdAt;
/**
*
* The date and time when the application run was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The tags assigned to the application.
*
*/
private java.util.Map tags;
/**
*
* The configuration for an application to automatically start on job submission.
*
*/
private AutoStartConfig autoStartConfiguration;
/**
*
* The configuration for an application to automatically stop after a certain amount of time being idle.
*
*/
private AutoStopConfig autoStopConfiguration;
/**
*
* The network configuration for customer VPC connectivity for the application.
*
*/
private NetworkConfiguration networkConfiguration;
/**
*
* The CPU architecture of an application.
*
*/
private String architecture;
/**
*
* The image configuration applied to all worker types.
*
*/
private ImageConfiguration imageConfiguration;
/**
*
* The specification applied to each worker type.
*
*/
private java.util.Map workerTypeSpecifications;
/**
*
* The Configuration
* specifications of an application. Each configuration consists of a classification and properties. You use this
* parameter when creating or updating an application. To see the runtimeConfiguration object of an application, run
* the GetApplication
* API operation.
*
*/
private java.util.List runtimeConfiguration;
private MonitoringConfiguration monitoringConfiguration;
/**
*
* The interactive configuration object that enables the interactive use cases for an application.
*
*/
private InteractiveConfiguration interactiveConfiguration;
/**
*
* The ID of the application.
*
*
* @param applicationId
* The ID of the application.
*/
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
*
* The ID of the application.
*
*
* @return The ID of the application.
*/
public String getApplicationId() {
return this.applicationId;
}
/**
*
* The ID of the application.
*
*
* @param applicationId
* The ID of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withApplicationId(String applicationId) {
setApplicationId(applicationId);
return this;
}
/**
*
* The name of the application.
*
*
* @param name
* The name of the application.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the application.
*
*
* @return The name of the application.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the application.
*
*
* @param name
* The name of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withName(String name) {
setName(name);
return this;
}
/**
*
* The ARN of the application.
*
*
* @param arn
* The ARN of the application.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The ARN of the application.
*
*
* @return The ARN of the application.
*/
public String getArn() {
return this.arn;
}
/**
*
* The ARN of the application.
*
*
* @param arn
* The ARN of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The Amazon EMR release associated with the application.
*
*
* @param releaseLabel
* The Amazon EMR release associated with the application.
*/
public void setReleaseLabel(String releaseLabel) {
this.releaseLabel = releaseLabel;
}
/**
*
* The Amazon EMR release associated with the application.
*
*
* @return The Amazon EMR release associated with the application.
*/
public String getReleaseLabel() {
return this.releaseLabel;
}
/**
*
* The Amazon EMR release associated with the application.
*
*
* @param releaseLabel
* The Amazon EMR release associated with the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withReleaseLabel(String releaseLabel) {
setReleaseLabel(releaseLabel);
return this;
}
/**
*
* The type of application, such as Spark or Hive.
*
*
* @param type
* The type of application, such as Spark or Hive.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of application, such as Spark or Hive.
*
*
* @return The type of application, such as Spark or Hive.
*/
public String getType() {
return this.type;
}
/**
*
* The type of application, such as Spark or Hive.
*
*
* @param type
* The type of application, such as Spark or Hive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withType(String type) {
setType(type);
return this;
}
/**
*
* The state of the application.
*
*
* @param state
* The state of the application.
* @see ApplicationState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The state of the application.
*
*
* @return The state of the application.
* @see ApplicationState
*/
public String getState() {
return this.state;
}
/**
*
* The state of the application.
*
*
* @param state
* The state of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ApplicationState
*/
public Application withState(String state) {
setState(state);
return this;
}
/**
*
* The state of the application.
*
*
* @param state
* The state of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ApplicationState
*/
public Application withState(ApplicationState state) {
this.state = state.toString();
return this;
}
/**
*
* The state details of the application.
*
*
* @param stateDetails
* The state details of the application.
*/
public void setStateDetails(String stateDetails) {
this.stateDetails = stateDetails;
}
/**
*
* The state details of the application.
*
*
* @return The state details of the application.
*/
public String getStateDetails() {
return this.stateDetails;
}
/**
*
* The state details of the application.
*
*
* @param stateDetails
* The state details of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withStateDetails(String stateDetails) {
setStateDetails(stateDetails);
return this;
}
/**
*
* The initial capacity of the application.
*
*
* @return The initial capacity of the application.
*/
public java.util.Map getInitialCapacity() {
return initialCapacity;
}
/**
*
* The initial capacity of the application.
*
*
* @param initialCapacity
* The initial capacity of the application.
*/
public void setInitialCapacity(java.util.Map initialCapacity) {
this.initialCapacity = initialCapacity;
}
/**
*
* The initial capacity of the application.
*
*
* @param initialCapacity
* The initial capacity of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withInitialCapacity(java.util.Map initialCapacity) {
setInitialCapacity(initialCapacity);
return this;
}
/**
* Add a single InitialCapacity entry
*
* @see Application#withInitialCapacity
* @returns a reference to this object so that method calls can be chained together.
*/
public Application addInitialCapacityEntry(String key, InitialCapacityConfig value) {
if (null == this.initialCapacity) {
this.initialCapacity = new java.util.HashMap();
}
if (this.initialCapacity.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.initialCapacity.put(key, value);
return this;
}
/**
* Removes all the entries added into InitialCapacity.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application clearInitialCapacityEntries() {
this.initialCapacity = null;
return this;
}
/**
*
* The maximum capacity of the application. This is cumulative across all workers at any given point in time during
* the lifespan of the application is created. No new resources will be created once any one of the defined limits
* is hit.
*
*
* @param maximumCapacity
* The maximum capacity of the application. This is cumulative across all workers at any given point in time
* during the lifespan of the application is created. No new resources will be created once any one of the
* defined limits is hit.
*/
public void setMaximumCapacity(MaximumAllowedResources maximumCapacity) {
this.maximumCapacity = maximumCapacity;
}
/**
*
* The maximum capacity of the application. This is cumulative across all workers at any given point in time during
* the lifespan of the application is created. No new resources will be created once any one of the defined limits
* is hit.
*
*
* @return The maximum capacity of the application. This is cumulative across all workers at any given point in time
* during the lifespan of the application is created. No new resources will be created once any one of the
* defined limits is hit.
*/
public MaximumAllowedResources getMaximumCapacity() {
return this.maximumCapacity;
}
/**
*
* The maximum capacity of the application. This is cumulative across all workers at any given point in time during
* the lifespan of the application is created. No new resources will be created once any one of the defined limits
* is hit.
*
*
* @param maximumCapacity
* The maximum capacity of the application. This is cumulative across all workers at any given point in time
* during the lifespan of the application is created. No new resources will be created once any one of the
* defined limits is hit.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withMaximumCapacity(MaximumAllowedResources maximumCapacity) {
setMaximumCapacity(maximumCapacity);
return this;
}
/**
*
* The date and time when the application run was created.
*
*
* @param createdAt
* The date and time when the application run was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time when the application run was created.
*
*
* @return The date and time when the application run was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time when the application run was created.
*
*
* @param createdAt
* The date and time when the application run was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The date and time when the application run was last updated.
*
*
* @param updatedAt
* The date and time when the application run was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The date and time when the application run was last updated.
*
*
* @return The date and time when the application run was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The date and time when the application run was last updated.
*
*
* @param updatedAt
* The date and time when the application run was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* The tags assigned to the application.
*
*
* @return The tags assigned to the application.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags assigned to the application.
*
*
* @param tags
* The tags assigned to the application.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags assigned to the application.
*
*
* @param tags
* The tags assigned to the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see Application#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public Application addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* The configuration for an application to automatically start on job submission.
*
*
* @param autoStartConfiguration
* The configuration for an application to automatically start on job submission.
*/
public void setAutoStartConfiguration(AutoStartConfig autoStartConfiguration) {
this.autoStartConfiguration = autoStartConfiguration;
}
/**
*
* The configuration for an application to automatically start on job submission.
*
*
* @return The configuration for an application to automatically start on job submission.
*/
public AutoStartConfig getAutoStartConfiguration() {
return this.autoStartConfiguration;
}
/**
*
* The configuration for an application to automatically start on job submission.
*
*
* @param autoStartConfiguration
* The configuration for an application to automatically start on job submission.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withAutoStartConfiguration(AutoStartConfig autoStartConfiguration) {
setAutoStartConfiguration(autoStartConfiguration);
return this;
}
/**
*
* The configuration for an application to automatically stop after a certain amount of time being idle.
*
*
* @param autoStopConfiguration
* The configuration for an application to automatically stop after a certain amount of time being idle.
*/
public void setAutoStopConfiguration(AutoStopConfig autoStopConfiguration) {
this.autoStopConfiguration = autoStopConfiguration;
}
/**
*
* The configuration for an application to automatically stop after a certain amount of time being idle.
*
*
* @return The configuration for an application to automatically stop after a certain amount of time being idle.
*/
public AutoStopConfig getAutoStopConfiguration() {
return this.autoStopConfiguration;
}
/**
*
* The configuration for an application to automatically stop after a certain amount of time being idle.
*
*
* @param autoStopConfiguration
* The configuration for an application to automatically stop after a certain amount of time being idle.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withAutoStopConfiguration(AutoStopConfig autoStopConfiguration) {
setAutoStopConfiguration(autoStopConfiguration);
return this;
}
/**
*
* The network configuration for customer VPC connectivity for the application.
*
*
* @param networkConfiguration
* The network configuration for customer VPC connectivity for the application.
*/
public void setNetworkConfiguration(NetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* The network configuration for customer VPC connectivity for the application.
*
*
* @return The network configuration for customer VPC connectivity for the application.
*/
public NetworkConfiguration getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* The network configuration for customer VPC connectivity for the application.
*
*
* @param networkConfiguration
* The network configuration for customer VPC connectivity for the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withNetworkConfiguration(NetworkConfiguration networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
* The CPU architecture of an application.
*
*
* @param architecture
* The CPU architecture of an application.
* @see Architecture
*/
public void setArchitecture(String architecture) {
this.architecture = architecture;
}
/**
*
* The CPU architecture of an application.
*
*
* @return The CPU architecture of an application.
* @see Architecture
*/
public String getArchitecture() {
return this.architecture;
}
/**
*
* The CPU architecture of an application.
*
*
* @param architecture
* The CPU architecture of an application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Architecture
*/
public Application withArchitecture(String architecture) {
setArchitecture(architecture);
return this;
}
/**
*
* The CPU architecture of an application.
*
*
* @param architecture
* The CPU architecture of an application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Architecture
*/
public Application withArchitecture(Architecture architecture) {
this.architecture = architecture.toString();
return this;
}
/**
*
* The image configuration applied to all worker types.
*
*
* @param imageConfiguration
* The image configuration applied to all worker types.
*/
public void setImageConfiguration(ImageConfiguration imageConfiguration) {
this.imageConfiguration = imageConfiguration;
}
/**
*
* The image configuration applied to all worker types.
*
*
* @return The image configuration applied to all worker types.
*/
public ImageConfiguration getImageConfiguration() {
return this.imageConfiguration;
}
/**
*
* The image configuration applied to all worker types.
*
*
* @param imageConfiguration
* The image configuration applied to all worker types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withImageConfiguration(ImageConfiguration imageConfiguration) {
setImageConfiguration(imageConfiguration);
return this;
}
/**
*
* The specification applied to each worker type.
*
*
* @return The specification applied to each worker type.
*/
public java.util.Map getWorkerTypeSpecifications() {
return workerTypeSpecifications;
}
/**
*
* The specification applied to each worker type.
*
*
* @param workerTypeSpecifications
* The specification applied to each worker type.
*/
public void setWorkerTypeSpecifications(java.util.Map workerTypeSpecifications) {
this.workerTypeSpecifications = workerTypeSpecifications;
}
/**
*
* The specification applied to each worker type.
*
*
* @param workerTypeSpecifications
* The specification applied to each worker type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withWorkerTypeSpecifications(java.util.Map workerTypeSpecifications) {
setWorkerTypeSpecifications(workerTypeSpecifications);
return this;
}
/**
* Add a single WorkerTypeSpecifications entry
*
* @see Application#withWorkerTypeSpecifications
* @returns a reference to this object so that method calls can be chained together.
*/
public Application addWorkerTypeSpecificationsEntry(String key, WorkerTypeSpecification value) {
if (null == this.workerTypeSpecifications) {
this.workerTypeSpecifications = new java.util.HashMap();
}
if (this.workerTypeSpecifications.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.workerTypeSpecifications.put(key, value);
return this;
}
/**
* Removes all the entries added into WorkerTypeSpecifications.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application clearWorkerTypeSpecificationsEntries() {
this.workerTypeSpecifications = null;
return this;
}
/**
*
* The Configuration
* specifications of an application. Each configuration consists of a classification and properties. You use this
* parameter when creating or updating an application. To see the runtimeConfiguration object of an application, run
* the GetApplication
* API operation.
*
*
* @return The Configuration
* specifications of an application. Each configuration consists of a classification and properties.
* You use this parameter when creating or updating an application. To see the runtimeConfiguration object
* of an application, run the GetApplication API operation.
*/
public java.util.List getRuntimeConfiguration() {
return runtimeConfiguration;
}
/**
*
* The Configuration
* specifications of an application. Each configuration consists of a classification and properties. You use this
* parameter when creating or updating an application. To see the runtimeConfiguration object of an application, run
* the GetApplication
* API operation.
*
*
* @param runtimeConfiguration
* The
* Configuration specifications of an application. Each configuration consists of a classification and
* properties. You use this parameter when creating or updating an application. To see the
* runtimeConfiguration object of an application, run the GetApplication API operation.
*/
public void setRuntimeConfiguration(java.util.Collection runtimeConfiguration) {
if (runtimeConfiguration == null) {
this.runtimeConfiguration = null;
return;
}
this.runtimeConfiguration = new java.util.ArrayList(runtimeConfiguration);
}
/**
*
* The Configuration
* specifications of an application. Each configuration consists of a classification and properties. You use this
* parameter when creating or updating an application. To see the runtimeConfiguration object of an application, run
* the GetApplication
* API operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRuntimeConfiguration(java.util.Collection)} or {@link #withRuntimeConfiguration(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param runtimeConfiguration
* The
* Configuration specifications of an application. Each configuration consists of a classification and
* properties. You use this parameter when creating or updating an application. To see the
* runtimeConfiguration object of an application, run the GetApplication API operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withRuntimeConfiguration(Configuration... runtimeConfiguration) {
if (this.runtimeConfiguration == null) {
setRuntimeConfiguration(new java.util.ArrayList(runtimeConfiguration.length));
}
for (Configuration ele : runtimeConfiguration) {
this.runtimeConfiguration.add(ele);
}
return this;
}
/**
*
* The Configuration
* specifications of an application. Each configuration consists of a classification and properties. You use this
* parameter when creating or updating an application. To see the runtimeConfiguration object of an application, run
* the GetApplication
* API operation.
*
*
* @param runtimeConfiguration
* The
* Configuration specifications of an application. Each configuration consists of a classification and
* properties. You use this parameter when creating or updating an application. To see the
* runtimeConfiguration object of an application, run the GetApplication API operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withRuntimeConfiguration(java.util.Collection runtimeConfiguration) {
setRuntimeConfiguration(runtimeConfiguration);
return this;
}
/**
* @param monitoringConfiguration
*/
public void setMonitoringConfiguration(MonitoringConfiguration monitoringConfiguration) {
this.monitoringConfiguration = monitoringConfiguration;
}
/**
* @return
*/
public MonitoringConfiguration getMonitoringConfiguration() {
return this.monitoringConfiguration;
}
/**
* @param monitoringConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withMonitoringConfiguration(MonitoringConfiguration monitoringConfiguration) {
setMonitoringConfiguration(monitoringConfiguration);
return this;
}
/**
*
* The interactive configuration object that enables the interactive use cases for an application.
*
*
* @param interactiveConfiguration
* The interactive configuration object that enables the interactive use cases for an application.
*/
public void setInteractiveConfiguration(InteractiveConfiguration interactiveConfiguration) {
this.interactiveConfiguration = interactiveConfiguration;
}
/**
*
* The interactive configuration object that enables the interactive use cases for an application.
*
*
* @return The interactive configuration object that enables the interactive use cases for an application.
*/
public InteractiveConfiguration getInteractiveConfiguration() {
return this.interactiveConfiguration;
}
/**
*
* The interactive configuration object that enables the interactive use cases for an application.
*
*
* @param interactiveConfiguration
* The interactive configuration object that enables the interactive use cases for an application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Application withInteractiveConfiguration(InteractiveConfiguration interactiveConfiguration) {
setInteractiveConfiguration(interactiveConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationId() != null)
sb.append("ApplicationId: ").append(getApplicationId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getReleaseLabel() != null)
sb.append("ReleaseLabel: ").append(getReleaseLabel()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getStateDetails() != null)
sb.append("StateDetails: ").append(getStateDetails()).append(",");
if (getInitialCapacity() != null)
sb.append("InitialCapacity: ").append(getInitialCapacity()).append(",");
if (getMaximumCapacity() != null)
sb.append("MaximumCapacity: ").append(getMaximumCapacity()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAutoStartConfiguration() != null)
sb.append("AutoStartConfiguration: ").append(getAutoStartConfiguration()).append(",");
if (getAutoStopConfiguration() != null)
sb.append("AutoStopConfiguration: ").append(getAutoStopConfiguration()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getArchitecture() != null)
sb.append("Architecture: ").append(getArchitecture()).append(",");
if (getImageConfiguration() != null)
sb.append("ImageConfiguration: ").append(getImageConfiguration()).append(",");
if (getWorkerTypeSpecifications() != null)
sb.append("WorkerTypeSpecifications: ").append(getWorkerTypeSpecifications()).append(",");
if (getRuntimeConfiguration() != null)
sb.append("RuntimeConfiguration: ").append(getRuntimeConfiguration()).append(",");
if (getMonitoringConfiguration() != null)
sb.append("MonitoringConfiguration: ").append(getMonitoringConfiguration()).append(",");
if (getInteractiveConfiguration() != null)
sb.append("InteractiveConfiguration: ").append(getInteractiveConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Application == false)
return false;
Application other = (Application) obj;
if (other.getApplicationId() == null ^ this.getApplicationId() == null)
return false;
if (other.getApplicationId() != null && other.getApplicationId().equals(this.getApplicationId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getReleaseLabel() == null ^ this.getReleaseLabel() == null)
return false;
if (other.getReleaseLabel() != null && other.getReleaseLabel().equals(this.getReleaseLabel()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getStateDetails() == null ^ this.getStateDetails() == null)
return false;
if (other.getStateDetails() != null && other.getStateDetails().equals(this.getStateDetails()) == false)
return false;
if (other.getInitialCapacity() == null ^ this.getInitialCapacity() == null)
return false;
if (other.getInitialCapacity() != null && other.getInitialCapacity().equals(this.getInitialCapacity()) == false)
return false;
if (other.getMaximumCapacity() == null ^ this.getMaximumCapacity() == null)
return false;
if (other.getMaximumCapacity() != null && other.getMaximumCapacity().equals(this.getMaximumCapacity()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAutoStartConfiguration() == null ^ this.getAutoStartConfiguration() == null)
return false;
if (other.getAutoStartConfiguration() != null && other.getAutoStartConfiguration().equals(this.getAutoStartConfiguration()) == false)
return false;
if (other.getAutoStopConfiguration() == null ^ this.getAutoStopConfiguration() == null)
return false;
if (other.getAutoStopConfiguration() != null && other.getAutoStopConfiguration().equals(this.getAutoStopConfiguration()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getArchitecture() == null ^ this.getArchitecture() == null)
return false;
if (other.getArchitecture() != null && other.getArchitecture().equals(this.getArchitecture()) == false)
return false;
if (other.getImageConfiguration() == null ^ this.getImageConfiguration() == null)
return false;
if (other.getImageConfiguration() != null && other.getImageConfiguration().equals(this.getImageConfiguration()) == false)
return false;
if (other.getWorkerTypeSpecifications() == null ^ this.getWorkerTypeSpecifications() == null)
return false;
if (other.getWorkerTypeSpecifications() != null && other.getWorkerTypeSpecifications().equals(this.getWorkerTypeSpecifications()) == false)
return false;
if (other.getRuntimeConfiguration() == null ^ this.getRuntimeConfiguration() == null)
return false;
if (other.getRuntimeConfiguration() != null && other.getRuntimeConfiguration().equals(this.getRuntimeConfiguration()) == false)
return false;
if (other.getMonitoringConfiguration() == null ^ this.getMonitoringConfiguration() == null)
return false;
if (other.getMonitoringConfiguration() != null && other.getMonitoringConfiguration().equals(this.getMonitoringConfiguration()) == false)
return false;
if (other.getInteractiveConfiguration() == null ^ this.getInteractiveConfiguration() == null)
return false;
if (other.getInteractiveConfiguration() != null && other.getInteractiveConfiguration().equals(this.getInteractiveConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationId() == null) ? 0 : getApplicationId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getReleaseLabel() == null) ? 0 : getReleaseLabel().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getStateDetails() == null) ? 0 : getStateDetails().hashCode());
hashCode = prime * hashCode + ((getInitialCapacity() == null) ? 0 : getInitialCapacity().hashCode());
hashCode = prime * hashCode + ((getMaximumCapacity() == null) ? 0 : getMaximumCapacity().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAutoStartConfiguration() == null) ? 0 : getAutoStartConfiguration().hashCode());
hashCode = prime * hashCode + ((getAutoStopConfiguration() == null) ? 0 : getAutoStopConfiguration().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getArchitecture() == null) ? 0 : getArchitecture().hashCode());
hashCode = prime * hashCode + ((getImageConfiguration() == null) ? 0 : getImageConfiguration().hashCode());
hashCode = prime * hashCode + ((getWorkerTypeSpecifications() == null) ? 0 : getWorkerTypeSpecifications().hashCode());
hashCode = prime * hashCode + ((getRuntimeConfiguration() == null) ? 0 : getRuntimeConfiguration().hashCode());
hashCode = prime * hashCode + ((getMonitoringConfiguration() == null) ? 0 : getMonitoringConfiguration().hashCode());
hashCode = prime * hashCode + ((getInteractiveConfiguration() == null) ? 0 : getInteractiveConfiguration().hashCode());
return hashCode;
}
@Override
public Application clone() {
try {
return (Application) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.emrserverless.model.transform.ApplicationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}