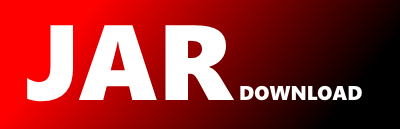
com.amazonaws.services.emrserverless.AWSEMRServerlessAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emrserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.emrserverless;
import javax.annotation.Generated;
import com.amazonaws.services.emrserverless.model.*;
/**
* Interface for accessing EMR Serverless asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.emrserverless.AbstractAWSEMRServerlessAsync} instead.
*
*
*
* Amazon EMR Serverless is a new deployment option for Amazon EMR. Amazon EMR Serverless provides a serverless runtime
* environment that simplifies running analytics applications using the latest open source frameworks such as Apache
* Spark and Apache Hive. With Amazon EMR Serverless, you don’t have to configure, optimize, secure, or operate clusters
* to run applications with these frameworks.
*
*
* The API reference to Amazon EMR Serverless is emr-serverless
. The emr-serverless
prefix is
* used in the following scenarios:
*
*
* -
*
* It is the prefix in the CLI commands for Amazon EMR Serverless. For example,
* aws emr-serverless start-job-run
.
*
*
* -
*
* It is the prefix before IAM policy actions for Amazon EMR Serverless. For example,
* "Action": ["emr-serverless:StartJobRun"]
. For more information, see Policy actions for Amazon EMR Serverless.
*
*
* -
*
* It is the prefix used in Amazon EMR Serverless service endpoints. For example,
* emr-serverless.us-east-2.amazonaws.com
.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSEMRServerlessAsync extends AWSEMRServerless {
/**
*
* Cancels a job run.
*
*
* @param cancelJobRunRequest
* @return A Java Future containing the result of the CancelJobRun operation returned by the service.
* @sample AWSEMRServerlessAsync.CancelJobRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelJobRunAsync(CancelJobRunRequest cancelJobRunRequest);
/**
*
* Cancels a job run.
*
*
* @param cancelJobRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelJobRun operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.CancelJobRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelJobRunAsync(CancelJobRunRequest cancelJobRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSEMRServerlessAsync.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an application. An application has to be in a stopped or created state in order to be deleted.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSEMRServerlessAsync.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes an application. An application has to be in a stopped or created state in order to be deleted.
*
*
* @param deleteApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays detailed information about a specified application.
*
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AWSEMRServerlessAsync.GetApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest);
/**
*
* Displays detailed information about a specified application.
*
*
* @param getApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.GetApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and returns a URL that you can use to access the application UIs for a job run.
*
*
* For jobs in a running state, the application UI is a live user interface such as the Spark or Tez web UI. For
* completed jobs, the application UI is a persistent application user interface such as the Spark History Server or
* persistent Tez UI.
*
*
*
* The URL is valid for one hour after you generate it. To access the application UI after that hour elapses, you
* must invoke the API again to generate a new URL.
*
*
*
* @param getDashboardForJobRunRequest
* @return A Java Future containing the result of the GetDashboardForJobRun operation returned by the service.
* @sample AWSEMRServerlessAsync.GetDashboardForJobRun
* @see AWS API Documentation
*/
java.util.concurrent.Future getDashboardForJobRunAsync(GetDashboardForJobRunRequest getDashboardForJobRunRequest);
/**
*
* Creates and returns a URL that you can use to access the application UIs for a job run.
*
*
* For jobs in a running state, the application UI is a live user interface such as the Spark or Tez web UI. For
* completed jobs, the application UI is a persistent application user interface such as the Spark History Server or
* persistent Tez UI.
*
*
*
* The URL is valid for one hour after you generate it. To access the application UI after that hour elapses, you
* must invoke the API again to generate a new URL.
*
*
*
* @param getDashboardForJobRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDashboardForJobRun operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.GetDashboardForJobRun
* @see AWS API Documentation
*/
java.util.concurrent.Future getDashboardForJobRunAsync(GetDashboardForJobRunRequest getDashboardForJobRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays detailed information about a job run.
*
*
* @param getJobRunRequest
* @return A Java Future containing the result of the GetJobRun operation returned by the service.
* @sample AWSEMRServerlessAsync.GetJobRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobRunAsync(GetJobRunRequest getJobRunRequest);
/**
*
* Displays detailed information about a job run.
*
*
* @param getJobRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetJobRun operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.GetJobRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobRunAsync(GetJobRunRequest getJobRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists applications based on a set of parameters.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSEMRServerlessAsync.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists applications based on a set of parameters.
*
*
* @param listApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all attempt of a job run.
*
*
* @param listJobRunAttemptsRequest
* @return A Java Future containing the result of the ListJobRunAttempts operation returned by the service.
* @sample AWSEMRServerlessAsync.ListJobRunAttempts
* @see AWS API Documentation
*/
java.util.concurrent.Future listJobRunAttemptsAsync(ListJobRunAttemptsRequest listJobRunAttemptsRequest);
/**
*
* Lists all attempt of a job run.
*
*
* @param listJobRunAttemptsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListJobRunAttempts operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.ListJobRunAttempts
* @see AWS API Documentation
*/
java.util.concurrent.Future listJobRunAttemptsAsync(ListJobRunAttemptsRequest listJobRunAttemptsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists job runs based on a set of parameters.
*
*
* @param listJobRunsRequest
* @return A Java Future containing the result of the ListJobRuns operation returned by the service.
* @sample AWSEMRServerlessAsync.ListJobRuns
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobRunsAsync(ListJobRunsRequest listJobRunsRequest);
/**
*
* Lists job runs based on a set of parameters.
*
*
* @param listJobRunsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListJobRuns operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.ListJobRuns
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobRunsAsync(ListJobRunsRequest listJobRunsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags assigned to the resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSEMRServerlessAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags assigned to the resources.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a specified application and initializes initial capacity if configured.
*
*
* @param startApplicationRequest
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* @sample AWSEMRServerlessAsync.StartApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future startApplicationAsync(StartApplicationRequest startApplicationRequest);
/**
*
* Starts a specified application and initializes initial capacity if configured.
*
*
* @param startApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.StartApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future startApplicationAsync(StartApplicationRequest startApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a job run.
*
*
* @param startJobRunRequest
* @return A Java Future containing the result of the StartJobRun operation returned by the service.
* @sample AWSEMRServerlessAsync.StartJobRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startJobRunAsync(StartJobRunRequest startJobRunRequest);
/**
*
* Starts a job run.
*
*
* @param startJobRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartJobRun operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.StartJobRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startJobRunAsync(StartJobRunRequest startJobRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a specified application and releases initial capacity if configured. All scheduled and running jobs must be
* completed or cancelled before stopping an application.
*
*
* @param stopApplicationRequest
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* @sample AWSEMRServerlessAsync.StopApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopApplicationAsync(StopApplicationRequest stopApplicationRequest);
/**
*
* Stops a specified application and releases initial capacity if configured. All scheduled and running jobs must be
* completed or cancelled before stopping an application.
*
*
* @param stopApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.StopApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopApplicationAsync(StopApplicationRequest stopApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns tags to resources. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists
* of a key and an optional value, both of which you define. Tags enable you to categorize your Amazon Web Services
* resources by attributes such as purpose, owner, or environment. When you have many resources of the same type,
* you can quickly identify a specific resource based on the tags you've assigned to it.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSEMRServerlessAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns tags to resources. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists
* of a key and an optional value, both of which you define. Tags enable you to categorize your Amazon Web Services
* resources by attributes such as purpose, owner, or environment. When you have many resources of the same type,
* you can quickly identify a specific resource based on the tags you've assigned to it.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from resources.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSEMRServerlessAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from resources.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a specified application. An application has to be in a stopped or created state in order to be updated.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSEMRServerlessAsync.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates a specified application. An application has to be in a stopped or created state in order to be updated.
*
*
* @param updateApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSEMRServerlessAsyncHandler.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}