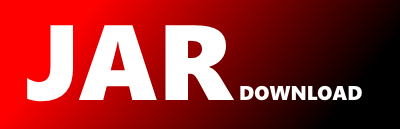
com.amazonaws.services.emrserverless.model.JobRunSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emrserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.emrserverless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The summary of attributes associated with a job run.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class JobRunSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the application the job is running on.
*
*/
private String applicationId;
/**
*
* The ID of the job run.
*
*/
private String id;
/**
*
* The optional job run name. This doesn't have to be unique.
*
*/
private String name;
/**
*
* The mode of the job run.
*
*/
private String mode;
/**
*
* The ARN of the job run.
*
*/
private String arn;
/**
*
* The user who created the job run.
*
*/
private String createdBy;
/**
*
* The date and time when the job run was created.
*
*/
private java.util.Date createdAt;
/**
*
* The date and time when the job run was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The execution role ARN of the job run.
*
*/
private String executionRole;
/**
*
* The state of the job run.
*
*/
private String state;
/**
*
* The state details of the job run.
*
*/
private String stateDetails;
/**
*
* The Amazon EMR release associated with the application your job is running on.
*
*/
private String releaseLabel;
/**
*
* The type of job run, such as Spark or Hive.
*
*/
private String type;
/**
*
* The attempt number of the job run execution.
*
*/
private Integer attempt;
/**
*
* The date and time of when the job run attempt was created.
*
*/
private java.util.Date attemptCreatedAt;
/**
*
* The date and time of when the job run attempt was last updated.
*
*/
private java.util.Date attemptUpdatedAt;
/**
*
* The ID of the application the job is running on.
*
*
* @param applicationId
* The ID of the application the job is running on.
*/
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
*
* The ID of the application the job is running on.
*
*
* @return The ID of the application the job is running on.
*/
public String getApplicationId() {
return this.applicationId;
}
/**
*
* The ID of the application the job is running on.
*
*
* @param applicationId
* The ID of the application the job is running on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withApplicationId(String applicationId) {
setApplicationId(applicationId);
return this;
}
/**
*
* The ID of the job run.
*
*
* @param id
* The ID of the job run.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The ID of the job run.
*
*
* @return The ID of the job run.
*/
public String getId() {
return this.id;
}
/**
*
* The ID of the job run.
*
*
* @param id
* The ID of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withId(String id) {
setId(id);
return this;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @param name
* The optional job run name. This doesn't have to be unique.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @return The optional job run name. This doesn't have to be unique.
*/
public String getName() {
return this.name;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @param name
* The optional job run name. This doesn't have to be unique.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withName(String name) {
setName(name);
return this;
}
/**
*
* The mode of the job run.
*
*
* @param mode
* The mode of the job run.
* @see JobRunMode
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
*
* The mode of the job run.
*
*
* @return The mode of the job run.
* @see JobRunMode
*/
public String getMode() {
return this.mode;
}
/**
*
* The mode of the job run.
*
*
* @param mode
* The mode of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobRunMode
*/
public JobRunSummary withMode(String mode) {
setMode(mode);
return this;
}
/**
*
* The mode of the job run.
*
*
* @param mode
* The mode of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobRunMode
*/
public JobRunSummary withMode(JobRunMode mode) {
this.mode = mode.toString();
return this;
}
/**
*
* The ARN of the job run.
*
*
* @param arn
* The ARN of the job run.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The ARN of the job run.
*
*
* @return The ARN of the job run.
*/
public String getArn() {
return this.arn;
}
/**
*
* The ARN of the job run.
*
*
* @param arn
* The ARN of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The user who created the job run.
*
*
* @param createdBy
* The user who created the job run.
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
*
* The user who created the job run.
*
*
* @return The user who created the job run.
*/
public String getCreatedBy() {
return this.createdBy;
}
/**
*
* The user who created the job run.
*
*
* @param createdBy
* The user who created the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withCreatedBy(String createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
*
* The date and time when the job run was created.
*
*
* @param createdAt
* The date and time when the job run was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time when the job run was created.
*
*
* @return The date and time when the job run was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time when the job run was created.
*
*
* @param createdAt
* The date and time when the job run was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The date and time when the job run was last updated.
*
*
* @param updatedAt
* The date and time when the job run was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The date and time when the job run was last updated.
*
*
* @return The date and time when the job run was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The date and time when the job run was last updated.
*
*
* @param updatedAt
* The date and time when the job run was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* The execution role ARN of the job run.
*
*
* @param executionRole
* The execution role ARN of the job run.
*/
public void setExecutionRole(String executionRole) {
this.executionRole = executionRole;
}
/**
*
* The execution role ARN of the job run.
*
*
* @return The execution role ARN of the job run.
*/
public String getExecutionRole() {
return this.executionRole;
}
/**
*
* The execution role ARN of the job run.
*
*
* @param executionRole
* The execution role ARN of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withExecutionRole(String executionRole) {
setExecutionRole(executionRole);
return this;
}
/**
*
* The state of the job run.
*
*
* @param state
* The state of the job run.
* @see JobRunState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The state of the job run.
*
*
* @return The state of the job run.
* @see JobRunState
*/
public String getState() {
return this.state;
}
/**
*
* The state of the job run.
*
*
* @param state
* The state of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobRunState
*/
public JobRunSummary withState(String state) {
setState(state);
return this;
}
/**
*
* The state of the job run.
*
*
* @param state
* The state of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobRunState
*/
public JobRunSummary withState(JobRunState state) {
this.state = state.toString();
return this;
}
/**
*
* The state details of the job run.
*
*
* @param stateDetails
* The state details of the job run.
*/
public void setStateDetails(String stateDetails) {
this.stateDetails = stateDetails;
}
/**
*
* The state details of the job run.
*
*
* @return The state details of the job run.
*/
public String getStateDetails() {
return this.stateDetails;
}
/**
*
* The state details of the job run.
*
*
* @param stateDetails
* The state details of the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withStateDetails(String stateDetails) {
setStateDetails(stateDetails);
return this;
}
/**
*
* The Amazon EMR release associated with the application your job is running on.
*
*
* @param releaseLabel
* The Amazon EMR release associated with the application your job is running on.
*/
public void setReleaseLabel(String releaseLabel) {
this.releaseLabel = releaseLabel;
}
/**
*
* The Amazon EMR release associated with the application your job is running on.
*
*
* @return The Amazon EMR release associated with the application your job is running on.
*/
public String getReleaseLabel() {
return this.releaseLabel;
}
/**
*
* The Amazon EMR release associated with the application your job is running on.
*
*
* @param releaseLabel
* The Amazon EMR release associated with the application your job is running on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withReleaseLabel(String releaseLabel) {
setReleaseLabel(releaseLabel);
return this;
}
/**
*
* The type of job run, such as Spark or Hive.
*
*
* @param type
* The type of job run, such as Spark or Hive.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of job run, such as Spark or Hive.
*
*
* @return The type of job run, such as Spark or Hive.
*/
public String getType() {
return this.type;
}
/**
*
* The type of job run, such as Spark or Hive.
*
*
* @param type
* The type of job run, such as Spark or Hive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withType(String type) {
setType(type);
return this;
}
/**
*
* The attempt number of the job run execution.
*
*
* @param attempt
* The attempt number of the job run execution.
*/
public void setAttempt(Integer attempt) {
this.attempt = attempt;
}
/**
*
* The attempt number of the job run execution.
*
*
* @return The attempt number of the job run execution.
*/
public Integer getAttempt() {
return this.attempt;
}
/**
*
* The attempt number of the job run execution.
*
*
* @param attempt
* The attempt number of the job run execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withAttempt(Integer attempt) {
setAttempt(attempt);
return this;
}
/**
*
* The date and time of when the job run attempt was created.
*
*
* @param attemptCreatedAt
* The date and time of when the job run attempt was created.
*/
public void setAttemptCreatedAt(java.util.Date attemptCreatedAt) {
this.attemptCreatedAt = attemptCreatedAt;
}
/**
*
* The date and time of when the job run attempt was created.
*
*
* @return The date and time of when the job run attempt was created.
*/
public java.util.Date getAttemptCreatedAt() {
return this.attemptCreatedAt;
}
/**
*
* The date and time of when the job run attempt was created.
*
*
* @param attemptCreatedAt
* The date and time of when the job run attempt was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withAttemptCreatedAt(java.util.Date attemptCreatedAt) {
setAttemptCreatedAt(attemptCreatedAt);
return this;
}
/**
*
* The date and time of when the job run attempt was last updated.
*
*
* @param attemptUpdatedAt
* The date and time of when the job run attempt was last updated.
*/
public void setAttemptUpdatedAt(java.util.Date attemptUpdatedAt) {
this.attemptUpdatedAt = attemptUpdatedAt;
}
/**
*
* The date and time of when the job run attempt was last updated.
*
*
* @return The date and time of when the job run attempt was last updated.
*/
public java.util.Date getAttemptUpdatedAt() {
return this.attemptUpdatedAt;
}
/**
*
* The date and time of when the job run attempt was last updated.
*
*
* @param attemptUpdatedAt
* The date and time of when the job run attempt was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobRunSummary withAttemptUpdatedAt(java.util.Date attemptUpdatedAt) {
setAttemptUpdatedAt(attemptUpdatedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationId() != null)
sb.append("ApplicationId: ").append(getApplicationId()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getMode() != null)
sb.append("Mode: ").append(getMode()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getExecutionRole() != null)
sb.append("ExecutionRole: ").append(getExecutionRole()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getStateDetails() != null)
sb.append("StateDetails: ").append(getStateDetails()).append(",");
if (getReleaseLabel() != null)
sb.append("ReleaseLabel: ").append(getReleaseLabel()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getAttempt() != null)
sb.append("Attempt: ").append(getAttempt()).append(",");
if (getAttemptCreatedAt() != null)
sb.append("AttemptCreatedAt: ").append(getAttemptCreatedAt()).append(",");
if (getAttemptUpdatedAt() != null)
sb.append("AttemptUpdatedAt: ").append(getAttemptUpdatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof JobRunSummary == false)
return false;
JobRunSummary other = (JobRunSummary) obj;
if (other.getApplicationId() == null ^ this.getApplicationId() == null)
return false;
if (other.getApplicationId() != null && other.getApplicationId().equals(this.getApplicationId()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getMode() == null ^ this.getMode() == null)
return false;
if (other.getMode() != null && other.getMode().equals(this.getMode()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getExecutionRole() == null ^ this.getExecutionRole() == null)
return false;
if (other.getExecutionRole() != null && other.getExecutionRole().equals(this.getExecutionRole()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getStateDetails() == null ^ this.getStateDetails() == null)
return false;
if (other.getStateDetails() != null && other.getStateDetails().equals(this.getStateDetails()) == false)
return false;
if (other.getReleaseLabel() == null ^ this.getReleaseLabel() == null)
return false;
if (other.getReleaseLabel() != null && other.getReleaseLabel().equals(this.getReleaseLabel()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getAttempt() == null ^ this.getAttempt() == null)
return false;
if (other.getAttempt() != null && other.getAttempt().equals(this.getAttempt()) == false)
return false;
if (other.getAttemptCreatedAt() == null ^ this.getAttemptCreatedAt() == null)
return false;
if (other.getAttemptCreatedAt() != null && other.getAttemptCreatedAt().equals(this.getAttemptCreatedAt()) == false)
return false;
if (other.getAttemptUpdatedAt() == null ^ this.getAttemptUpdatedAt() == null)
return false;
if (other.getAttemptUpdatedAt() != null && other.getAttemptUpdatedAt().equals(this.getAttemptUpdatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationId() == null) ? 0 : getApplicationId().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getMode() == null) ? 0 : getMode().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getExecutionRole() == null) ? 0 : getExecutionRole().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getStateDetails() == null) ? 0 : getStateDetails().hashCode());
hashCode = prime * hashCode + ((getReleaseLabel() == null) ? 0 : getReleaseLabel().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getAttempt() == null) ? 0 : getAttempt().hashCode());
hashCode = prime * hashCode + ((getAttemptCreatedAt() == null) ? 0 : getAttemptCreatedAt().hashCode());
hashCode = prime * hashCode + ((getAttemptUpdatedAt() == null) ? 0 : getAttemptUpdatedAt().hashCode());
return hashCode;
}
@Override
public JobRunSummary clone() {
try {
return (JobRunSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.emrserverless.model.transform.JobRunSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}