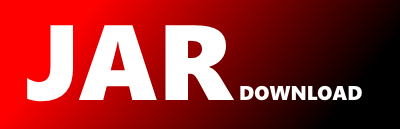
com.amazonaws.services.emrserverless.model.StartJobRunRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-emrserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.emrserverless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartJobRunRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the application on which to run the job.
*
*/
private String applicationId;
/**
*
* The client idempotency token of the job run to start. Its value must be unique for each request.
*
*/
private String clientToken;
/**
*
* The execution role ARN for the job run.
*
*/
private String executionRoleArn;
/**
*
* The job driver for the job run.
*
*/
private JobDriver jobDriver;
/**
*
* The configuration overrides for the job run.
*
*/
private ConfigurationOverrides configurationOverrides;
/**
*
* The tags assigned to the job run.
*
*/
private java.util.Map tags;
/**
*
* The maximum duration for the job run to run. If the job run runs beyond this duration, it will be automatically
* cancelled.
*
*/
private Long executionTimeoutMinutes;
/**
*
* The optional job run name. This doesn't have to be unique.
*
*/
private String name;
/**
*
* The mode of the job run when it starts.
*
*/
private String mode;
/**
*
* The retry policy when job run starts.
*
*/
private RetryPolicy retryPolicy;
/**
*
* The ID of the application on which to run the job.
*
*
* @param applicationId
* The ID of the application on which to run the job.
*/
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
*
* The ID of the application on which to run the job.
*
*
* @return The ID of the application on which to run the job.
*/
public String getApplicationId() {
return this.applicationId;
}
/**
*
* The ID of the application on which to run the job.
*
*
* @param applicationId
* The ID of the application on which to run the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withApplicationId(String applicationId) {
setApplicationId(applicationId);
return this;
}
/**
*
* The client idempotency token of the job run to start. Its value must be unique for each request.
*
*
* @param clientToken
* The client idempotency token of the job run to start. Its value must be unique for each request.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* The client idempotency token of the job run to start. Its value must be unique for each request.
*
*
* @return The client idempotency token of the job run to start. Its value must be unique for each request.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* The client idempotency token of the job run to start. Its value must be unique for each request.
*
*
* @param clientToken
* The client idempotency token of the job run to start. Its value must be unique for each request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The execution role ARN for the job run.
*
*
* @param executionRoleArn
* The execution role ARN for the job run.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The execution role ARN for the job run.
*
*
* @return The execution role ARN for the job run.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The execution role ARN for the job run.
*
*
* @param executionRoleArn
* The execution role ARN for the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* The job driver for the job run.
*
*
* @param jobDriver
* The job driver for the job run.
*/
public void setJobDriver(JobDriver jobDriver) {
this.jobDriver = jobDriver;
}
/**
*
* The job driver for the job run.
*
*
* @return The job driver for the job run.
*/
public JobDriver getJobDriver() {
return this.jobDriver;
}
/**
*
* The job driver for the job run.
*
*
* @param jobDriver
* The job driver for the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withJobDriver(JobDriver jobDriver) {
setJobDriver(jobDriver);
return this;
}
/**
*
* The configuration overrides for the job run.
*
*
* @param configurationOverrides
* The configuration overrides for the job run.
*/
public void setConfigurationOverrides(ConfigurationOverrides configurationOverrides) {
this.configurationOverrides = configurationOverrides;
}
/**
*
* The configuration overrides for the job run.
*
*
* @return The configuration overrides for the job run.
*/
public ConfigurationOverrides getConfigurationOverrides() {
return this.configurationOverrides;
}
/**
*
* The configuration overrides for the job run.
*
*
* @param configurationOverrides
* The configuration overrides for the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withConfigurationOverrides(ConfigurationOverrides configurationOverrides) {
setConfigurationOverrides(configurationOverrides);
return this;
}
/**
*
* The tags assigned to the job run.
*
*
* @return The tags assigned to the job run.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags assigned to the job run.
*
*
* @param tags
* The tags assigned to the job run.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags assigned to the job run.
*
*
* @param tags
* The tags assigned to the job run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see StartJobRunRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* The maximum duration for the job run to run. If the job run runs beyond this duration, it will be automatically
* cancelled.
*
*
* @param executionTimeoutMinutes
* The maximum duration for the job run to run. If the job run runs beyond this duration, it will be
* automatically cancelled.
*/
public void setExecutionTimeoutMinutes(Long executionTimeoutMinutes) {
this.executionTimeoutMinutes = executionTimeoutMinutes;
}
/**
*
* The maximum duration for the job run to run. If the job run runs beyond this duration, it will be automatically
* cancelled.
*
*
* @return The maximum duration for the job run to run. If the job run runs beyond this duration, it will be
* automatically cancelled.
*/
public Long getExecutionTimeoutMinutes() {
return this.executionTimeoutMinutes;
}
/**
*
* The maximum duration for the job run to run. If the job run runs beyond this duration, it will be automatically
* cancelled.
*
*
* @param executionTimeoutMinutes
* The maximum duration for the job run to run. If the job run runs beyond this duration, it will be
* automatically cancelled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withExecutionTimeoutMinutes(Long executionTimeoutMinutes) {
setExecutionTimeoutMinutes(executionTimeoutMinutes);
return this;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @param name
* The optional job run name. This doesn't have to be unique.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @return The optional job run name. This doesn't have to be unique.
*/
public String getName() {
return this.name;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @param name
* The optional job run name. This doesn't have to be unique.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The mode of the job run when it starts.
*
*
* @param mode
* The mode of the job run when it starts.
* @see JobRunMode
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
*
* The mode of the job run when it starts.
*
*
* @return The mode of the job run when it starts.
* @see JobRunMode
*/
public String getMode() {
return this.mode;
}
/**
*
* The mode of the job run when it starts.
*
*
* @param mode
* The mode of the job run when it starts.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobRunMode
*/
public StartJobRunRequest withMode(String mode) {
setMode(mode);
return this;
}
/**
*
* The mode of the job run when it starts.
*
*
* @param mode
* The mode of the job run when it starts.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobRunMode
*/
public StartJobRunRequest withMode(JobRunMode mode) {
this.mode = mode.toString();
return this;
}
/**
*
* The retry policy when job run starts.
*
*
* @param retryPolicy
* The retry policy when job run starts.
*/
public void setRetryPolicy(RetryPolicy retryPolicy) {
this.retryPolicy = retryPolicy;
}
/**
*
* The retry policy when job run starts.
*
*
* @return The retry policy when job run starts.
*/
public RetryPolicy getRetryPolicy() {
return this.retryPolicy;
}
/**
*
* The retry policy when job run starts.
*
*
* @param retryPolicy
* The retry policy when job run starts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartJobRunRequest withRetryPolicy(RetryPolicy retryPolicy) {
setRetryPolicy(retryPolicy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationId() != null)
sb.append("ApplicationId: ").append(getApplicationId()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getJobDriver() != null)
sb.append("JobDriver: ").append(getJobDriver()).append(",");
if (getConfigurationOverrides() != null)
sb.append("ConfigurationOverrides: ").append(getConfigurationOverrides()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getExecutionTimeoutMinutes() != null)
sb.append("ExecutionTimeoutMinutes: ").append(getExecutionTimeoutMinutes()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getMode() != null)
sb.append("Mode: ").append(getMode()).append(",");
if (getRetryPolicy() != null)
sb.append("RetryPolicy: ").append(getRetryPolicy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartJobRunRequest == false)
return false;
StartJobRunRequest other = (StartJobRunRequest) obj;
if (other.getApplicationId() == null ^ this.getApplicationId() == null)
return false;
if (other.getApplicationId() != null && other.getApplicationId().equals(this.getApplicationId()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getJobDriver() == null ^ this.getJobDriver() == null)
return false;
if (other.getJobDriver() != null && other.getJobDriver().equals(this.getJobDriver()) == false)
return false;
if (other.getConfigurationOverrides() == null ^ this.getConfigurationOverrides() == null)
return false;
if (other.getConfigurationOverrides() != null && other.getConfigurationOverrides().equals(this.getConfigurationOverrides()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getExecutionTimeoutMinutes() == null ^ this.getExecutionTimeoutMinutes() == null)
return false;
if (other.getExecutionTimeoutMinutes() != null && other.getExecutionTimeoutMinutes().equals(this.getExecutionTimeoutMinutes()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getMode() == null ^ this.getMode() == null)
return false;
if (other.getMode() != null && other.getMode().equals(this.getMode()) == false)
return false;
if (other.getRetryPolicy() == null ^ this.getRetryPolicy() == null)
return false;
if (other.getRetryPolicy() != null && other.getRetryPolicy().equals(this.getRetryPolicy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationId() == null) ? 0 : getApplicationId().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getJobDriver() == null) ? 0 : getJobDriver().hashCode());
hashCode = prime * hashCode + ((getConfigurationOverrides() == null) ? 0 : getConfigurationOverrides().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getExecutionTimeoutMinutes() == null) ? 0 : getExecutionTimeoutMinutes().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getMode() == null) ? 0 : getMode().hashCode());
hashCode = prime * hashCode + ((getRetryPolicy() == null) ? 0 : getRetryPolicy().hashCode());
return hashCode;
}
@Override
public StartJobRunRequest clone() {
return (StartJobRunRequest) super.clone();
}
}