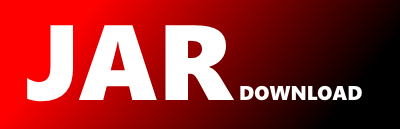
com.amazonaws.services.entityresolution.AWSEntityResolution Maven / Gradle / Ivy
Show all versions of aws-java-sdk-entityresolution Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.entityresolution;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.entityresolution.model.*;
/**
* Interface for accessing AWSEntityResolution.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.entityresolution.AbstractAWSEntityResolution} instead.
*
*
*
* Welcome to the Entity Resolution API Reference.
*
*
* Entity Resolution is an Amazon Web Services service that provides pre-configured entity resolution capabilities that
* enable developers and analysts at advertising and marketing companies to build an accurate and complete view of their
* consumers.
*
*
* With Entity Resolution, you can match source records containing consumer identifiers, such as name, email address,
* and phone number. This is true even when these records have incomplete or conflicting identifiers. For example,
* Entity Resolution can effectively match a source record from a customer relationship management (CRM) system with a
* source record from a marketing system containing campaign information.
*
*
* To learn more about Entity Resolution concepts, procedures, and best practices, see the Entity Resolution User
* Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSEntityResolution {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "entityresolution";
/**
*
* Adds a policy statement object. To retrieve a list of existing policy statements, use the GetPolicy
* API.
*
*
* @param addPolicyStatementRequest
* @return Result of the AddPolicyStatement operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.AddPolicyStatement
* @see AWS API Documentation
*/
AddPolicyStatementResult addPolicyStatement(AddPolicyStatementRequest addPolicyStatementRequest);
/**
*
* Deletes multiple unique IDs in a matching workflow.
*
*
* @param batchDeleteUniqueIdRequest
* @return Result of the BatchDeleteUniqueId operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.BatchDeleteUniqueId
* @see AWS API Documentation
*/
BatchDeleteUniqueIdResult batchDeleteUniqueId(BatchDeleteUniqueIdRequest batchDeleteUniqueIdRequest);
/**
*
* Creates an IdMappingWorkflow
object which stores the configuration of the data processing job to be
* run. Each IdMappingWorkflow
must have a unique workflow name. To modify an existing workflow, use
* the UpdateIdMappingWorkflow
API.
*
*
* @param createIdMappingWorkflowRequest
* @return Result of the CreateIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.CreateIdMappingWorkflow
* @see AWS API Documentation
*/
CreateIdMappingWorkflowResult createIdMappingWorkflow(CreateIdMappingWorkflowRequest createIdMappingWorkflowRequest);
/**
*
* Creates an ID namespace object which will help customers provide metadata explaining their dataset and how to use
* it. Each ID namespace must have a unique name. To modify an existing ID namespace, use the
* UpdateIdNamespace
API.
*
*
* @param createIdNamespaceRequest
* @return Result of the CreateIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.CreateIdNamespace
* @see AWS API Documentation
*/
CreateIdNamespaceResult createIdNamespace(CreateIdNamespaceRequest createIdNamespaceRequest);
/**
*
* Creates a MatchingWorkflow
object which stores the configuration of the data processing job to be
* run. It is important to note that there should not be a pre-existing MatchingWorkflow
with the same
* name. To modify an existing workflow, utilize the UpdateMatchingWorkflow
API.
*
*
* @param createMatchingWorkflowRequest
* @return Result of the CreateMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.CreateMatchingWorkflow
* @see AWS API Documentation
*/
CreateMatchingWorkflowResult createMatchingWorkflow(CreateMatchingWorkflowRequest createMatchingWorkflowRequest);
/**
*
* Creates a schema mapping, which defines the schema of the input customer records table. The
* SchemaMapping
also provides Entity Resolution with some metadata about the table, such as the
* attribute types of the columns and which columns to match on.
*
*
* @param createSchemaMappingRequest
* @return Result of the CreateSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.CreateSchemaMapping
* @see AWS API Documentation
*/
CreateSchemaMappingResult createSchemaMapping(CreateSchemaMappingRequest createSchemaMappingRequest);
/**
*
* Deletes the IdMappingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteIdMappingWorkflowRequest
* @return Result of the DeleteIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.DeleteIdMappingWorkflow
* @see AWS API Documentation
*/
DeleteIdMappingWorkflowResult deleteIdMappingWorkflow(DeleteIdMappingWorkflowRequest deleteIdMappingWorkflowRequest);
/**
*
* Deletes the IdNamespace
with a given name.
*
*
* @param deleteIdNamespaceRequest
* @return Result of the DeleteIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.DeleteIdNamespace
* @see AWS API Documentation
*/
DeleteIdNamespaceResult deleteIdNamespace(DeleteIdNamespaceRequest deleteIdNamespaceRequest);
/**
*
* Deletes the MatchingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteMatchingWorkflowRequest
* @return Result of the DeleteMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.DeleteMatchingWorkflow
* @see AWS API Documentation
*/
DeleteMatchingWorkflowResult deleteMatchingWorkflow(DeleteMatchingWorkflowRequest deleteMatchingWorkflowRequest);
/**
*
* Deletes the policy statement.
*
*
* @param deletePolicyStatementRequest
* @return Result of the DeletePolicyStatement operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.DeletePolicyStatement
* @see AWS API Documentation
*/
DeletePolicyStatementResult deletePolicyStatement(DeletePolicyStatementRequest deletePolicyStatementRequest);
/**
*
* Deletes the SchemaMapping
with a given name. This operation will succeed even if a schema with the
* given name does not exist. This operation will fail if there is a MatchingWorkflow
object that
* references the SchemaMapping
in the workflow's InputSourceConfig
.
*
*
* @param deleteSchemaMappingRequest
* @return Result of the DeleteSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.DeleteSchemaMapping
* @see AWS API Documentation
*/
DeleteSchemaMappingResult deleteSchemaMapping(DeleteSchemaMappingRequest deleteSchemaMappingRequest);
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getIdMappingJobRequest
* @return Result of the GetIdMappingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetIdMappingJob
* @see AWS API Documentation
*/
GetIdMappingJobResult getIdMappingJob(GetIdMappingJobRequest getIdMappingJobRequest);
/**
*
* Returns the IdMappingWorkflow
with a given name, if it exists.
*
*
* @param getIdMappingWorkflowRequest
* @return Result of the GetIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetIdMappingWorkflow
* @see AWS API Documentation
*/
GetIdMappingWorkflowResult getIdMappingWorkflow(GetIdMappingWorkflowRequest getIdMappingWorkflowRequest);
/**
*
* Returns the IdNamespace
with a given name, if it exists.
*
*
* @param getIdNamespaceRequest
* @return Result of the GetIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetIdNamespace
* @see AWS API Documentation
*/
GetIdNamespaceResult getIdNamespace(GetIdNamespaceRequest getIdNamespaceRequest);
/**
*
* Returns the corresponding Match ID of a customer record if the record has been processed.
*
*
* @param getMatchIdRequest
* @return Result of the GetMatchId operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetMatchId
* @see AWS
* API Documentation
*/
GetMatchIdResult getMatchId(GetMatchIdRequest getMatchIdRequest);
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getMatchingJobRequest
* @return Result of the GetMatchingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetMatchingJob
* @see AWS API Documentation
*/
GetMatchingJobResult getMatchingJob(GetMatchingJobRequest getMatchingJobRequest);
/**
*
* Returns the MatchingWorkflow
with a given name, if it exists.
*
*
* @param getMatchingWorkflowRequest
* @return Result of the GetMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetMatchingWorkflow
* @see AWS API Documentation
*/
GetMatchingWorkflowResult getMatchingWorkflow(GetMatchingWorkflowRequest getMatchingWorkflowRequest);
/**
*
* Returns the resource-based policy.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetPolicy
* @see AWS API
* Documentation
*/
GetPolicyResult getPolicy(GetPolicyRequest getPolicyRequest);
/**
*
* Returns the SchemaMapping of a given name.
*
*
* @param getSchemaMappingRequest
* @return Result of the GetSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.GetSchemaMapping
* @see AWS API Documentation
*/
GetSchemaMappingResult getSchemaMapping(GetSchemaMappingRequest getSchemaMappingRequest);
/**
*
* Lists all ID mapping jobs for a given workflow.
*
*
* @param listIdMappingJobsRequest
* @return Result of the ListIdMappingJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListIdMappingJobs
* @see AWS API Documentation
*/
ListIdMappingJobsResult listIdMappingJobs(ListIdMappingJobsRequest listIdMappingJobsRequest);
/**
*
* Returns a list of all the IdMappingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listIdMappingWorkflowsRequest
* @return Result of the ListIdMappingWorkflows operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListIdMappingWorkflows
* @see AWS API Documentation
*/
ListIdMappingWorkflowsResult listIdMappingWorkflows(ListIdMappingWorkflowsRequest listIdMappingWorkflowsRequest);
/**
*
* Returns a list of all ID namespaces.
*
*
* @param listIdNamespacesRequest
* @return Result of the ListIdNamespaces operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListIdNamespaces
* @see AWS API Documentation
*/
ListIdNamespacesResult listIdNamespaces(ListIdNamespacesRequest listIdNamespacesRequest);
/**
*
* Lists all jobs for a given workflow.
*
*
* @param listMatchingJobsRequest
* @return Result of the ListMatchingJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListMatchingJobs
* @see AWS API Documentation
*/
ListMatchingJobsResult listMatchingJobs(ListMatchingJobsRequest listMatchingJobsRequest);
/**
*
* Returns a list of all the MatchingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listMatchingWorkflowsRequest
* @return Result of the ListMatchingWorkflows operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListMatchingWorkflows
* @see AWS API Documentation
*/
ListMatchingWorkflowsResult listMatchingWorkflows(ListMatchingWorkflowsRequest listMatchingWorkflowsRequest);
/**
*
* Returns a list of all the ProviderServices
that are available in this Amazon Web Services Region.
*
*
* @param listProviderServicesRequest
* @return Result of the ListProviderServices operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListProviderServices
* @see AWS API Documentation
*/
ListProviderServicesResult listProviderServices(ListProviderServicesRequest listProviderServicesRequest);
/**
*
* Returns a list of all the SchemaMappings
that have been created for an Amazon Web Services account.
*
*
* @param listSchemaMappingsRequest
* @return Result of the ListSchemaMappings operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListSchemaMappings
* @see AWS API Documentation
*/
ListSchemaMappingsResult listSchemaMappings(ListSchemaMappingsRequest listSchemaMappingsRequest);
/**
*
* Displays the tags associated with an Entity Resolution resource. In Entity Resolution, SchemaMapping
* , and MatchingWorkflow
can be tagged.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Updates the resource-based policy.
*
*
* @param putPolicyRequest
* @return Result of the PutPolicy operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.PutPolicy
* @see AWS API
* Documentation
*/
PutPolicyResult putPolicy(PutPolicyRequest putPolicyRequest);
/**
*
* Starts the IdMappingJob
of a workflow. The workflow must have previously been created using the
* CreateIdMappingWorkflow
endpoint.
*
*
* @param startIdMappingJobRequest
* @return Result of the StartIdMappingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.StartIdMappingJob
* @see AWS API Documentation
*/
StartIdMappingJobResult startIdMappingJob(StartIdMappingJobRequest startIdMappingJobRequest);
/**
*
* Starts the MatchingJob
of a workflow. The workflow must have previously been created using the
* CreateMatchingWorkflow
endpoint.
*
*
* @param startMatchingJobRequest
* @return Result of the StartMatchingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.StartMatchingJob
* @see AWS API Documentation
*/
StartMatchingJobResult startMatchingJob(StartMatchingJobRequest startMatchingJobRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Entity Resolution resource. Tags can help you
* organize and categorize your resources. You can also use them to scope user permissions by granting a user
* permission to access or change only resources with certain tag values. In Entity Resolution,
* SchemaMapping
and MatchingWorkflow
can be tagged. Tags don't have any semantic meaning
* to Amazon Web Services and are interpreted strictly as strings of characters. You can use the
* TagResource
action with a resource that already has tags. If you specify a new tag key, this tag is
* appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes one or more tags from the specified Entity Resolution resource. In Entity Resolution,
* SchemaMapping
, and MatchingWorkflow
can be tagged.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @sample AWSEntityResolution.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an existing IdMappingWorkflow
. This method is identical to
* CreateIdMappingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the IdMappingWorkflow
must already exist for the method to succeed.
*
*
* @param updateIdMappingWorkflowRequest
* @return Result of the UpdateIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.UpdateIdMappingWorkflow
* @see AWS API Documentation
*/
UpdateIdMappingWorkflowResult updateIdMappingWorkflow(UpdateIdMappingWorkflowRequest updateIdMappingWorkflowRequest);
/**
*
* Updates an existing ID namespace.
*
*
* @param updateIdNamespaceRequest
* @return Result of the UpdateIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.UpdateIdNamespace
* @see AWS API Documentation
*/
UpdateIdNamespaceResult updateIdNamespace(UpdateIdNamespaceRequest updateIdNamespaceRequest);
/**
*
* Updates an existing MatchingWorkflow
. This method is identical to
* CreateMatchingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the MatchingWorkflow
must already exist for the method to succeed.
*
*
* @param updateMatchingWorkflowRequest
* @return Result of the UpdateMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.UpdateMatchingWorkflow
* @see AWS API Documentation
*/
UpdateMatchingWorkflowResult updateMatchingWorkflow(UpdateMatchingWorkflowRequest updateMatchingWorkflowRequest);
/**
*
* Updates a schema mapping.
*
*
*
* A schema is immutable if it is being used by a workflow. Therefore, you can't update a schema mapping if it's
* associated with a workflow.
*
*
*
* @param updateSchemaMappingRequest
* @return Result of the UpdateSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @sample AWSEntityResolution.UpdateSchemaMapping
* @see AWS API Documentation
*/
UpdateSchemaMappingResult updateSchemaMapping(UpdateSchemaMappingRequest updateSchemaMappingRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}